Writing Python lists to columns in csv
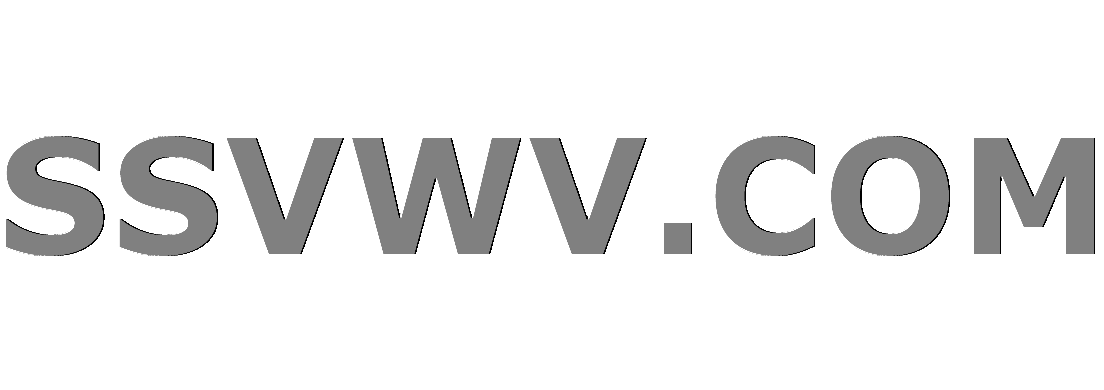
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I have 5 lists, all of the same length, and I'd like to write them to 5 columns in a CSV. So far, I can only write one to a column with this code:
with open('test.csv', 'wb') as f:
writer = csv.writer(f)
for val in test_list:
writer.writerow([val])
If I add another for
loop, it just writes that list to the same column. Anyone know a good way to get five separate columns?
python list csv
add a comment |
I have 5 lists, all of the same length, and I'd like to write them to 5 columns in a CSV. So far, I can only write one to a column with this code:
with open('test.csv', 'wb') as f:
writer = csv.writer(f)
for val in test_list:
writer.writerow([val])
If I add another for
loop, it just writes that list to the same column. Anyone know a good way to get five separate columns?
python list csv
add a comment |
I have 5 lists, all of the same length, and I'd like to write them to 5 columns in a CSV. So far, I can only write one to a column with this code:
with open('test.csv', 'wb') as f:
writer = csv.writer(f)
for val in test_list:
writer.writerow([val])
If I add another for
loop, it just writes that list to the same column. Anyone know a good way to get five separate columns?
python list csv
I have 5 lists, all of the same length, and I'd like to write them to 5 columns in a CSV. So far, I can only write one to a column with this code:
with open('test.csv', 'wb') as f:
writer = csv.writer(f)
for val in test_list:
writer.writerow([val])
If I add another for
loop, it just writes that list to the same column. Anyone know a good way to get five separate columns?
python list csv
python list csv
edited Jul 17 '13 at 16:49
Mike Mertsock
9,43273266
9,43273266
asked Jul 17 '13 at 15:40


Alex ChumbleyAlex Chumbley
2843714
2843714
add a comment |
add a comment |
6 Answers
6
active
oldest
votes
change them to rows
rows = zip(list1,list2,list3,list4,list5)
then just
import csv
with open(newfilePath, "w") as f:
writer = csv.writer(f)
for row in rows:
writer.writerow(row)
I got something like: array([-23.], dtype=float32), array([-0.39999986], dtype=float32... how get pure values without types?
– Brans Ds
Nov 30 '16 at 13:05
add a comment |
The following code writes python lists into columns in csv
import csv
from itertools import zip_longest
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j']
d = [list1, list2]
export_data = zip_longest(*d, fillvalue = '')
with open('numbers.csv', 'w', encoding="ISO-8859-1", newline='') as myfile:
wr = csv.writer(myfile)
wr.writerow(("List1", "List2"))
wr.writerows(export_data)
myfile.close()
The output looks like this
add a comment |
You can use izip
to combine your lists, and then iterate them
for val in itertools.izip(l1,l2,l3,l4,l5):
writer.writerow(val)
5
It's worth noting thatitertools.izip()
doesn't exist in Python 3.x, and thezip()
built-in gives an iterator, so that is a drop-in replacement. Also note that if you have a list of lists, you can use the unpacking operator (zip(*lists)
). This is better design than having variablesl1
,l2
, etc...
– Gareth Latty
Jul 17 '13 at 15:47
add a comment |
import csv
dic = {firstcol,secondcol} #dictionary
csv = open('result.csv', "w")
for key in dic.keys():
row ="n"+ str(key) + "," + str(dic[key])
csv.write(row)
2
Your contribution would be more meaningful if you would include some explanation about why this approach should work and be an improvement over what has already been proposed and accepted as the answer.
– Cindy Meister
Oct 13 '18 at 21:17
add a comment |
If you are happy to use a 3rd party library, you can do this with Pandas. The benefits include seamless access to specialized methods and row / column labeling:
import pandas as pd
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
df = pd.DataFrame(list(zip(*[list1, list2, list3]))).add_prefix('Col')
df.to_csv('file.csv', index=False)
print(df)
Col0 Col1 Col2
0 1 4 7
1 2 5 8
2 3 6 9
add a comment |
I didn't want to import anything other than csv, and all my lists have the same number of items. The top answer here seems to make the lists into one row each, instead of one column each. Thus I took the answers here and came up with this:
import csv
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j','k']
with open('C:/test/numbers.csv', 'wb+') as myfile:
wr = csv.writer(myfile)
wr.writerow(("list1", "list2"))
rcount = 0
for row in list1:
wr.writerow((list1[rcount], list2[rcount]))
rcount = rcount + 1
myfile.close()
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17704244%2fwriting-python-lists-to-columns-in-csv%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
change them to rows
rows = zip(list1,list2,list3,list4,list5)
then just
import csv
with open(newfilePath, "w") as f:
writer = csv.writer(f)
for row in rows:
writer.writerow(row)
I got something like: array([-23.], dtype=float32), array([-0.39999986], dtype=float32... how get pure values without types?
– Brans Ds
Nov 30 '16 at 13:05
add a comment |
change them to rows
rows = zip(list1,list2,list3,list4,list5)
then just
import csv
with open(newfilePath, "w") as f:
writer = csv.writer(f)
for row in rows:
writer.writerow(row)
I got something like: array([-23.], dtype=float32), array([-0.39999986], dtype=float32... how get pure values without types?
– Brans Ds
Nov 30 '16 at 13:05
add a comment |
change them to rows
rows = zip(list1,list2,list3,list4,list5)
then just
import csv
with open(newfilePath, "w") as f:
writer = csv.writer(f)
for row in rows:
writer.writerow(row)
change them to rows
rows = zip(list1,list2,list3,list4,list5)
then just
import csv
with open(newfilePath, "w") as f:
writer = csv.writer(f)
for row in rows:
writer.writerow(row)
edited Sep 20 '17 at 3:00
NRR
500617
500617
answered Jul 17 '13 at 15:43
Joran BeasleyJoran Beasley
74.2k683122
74.2k683122
I got something like: array([-23.], dtype=float32), array([-0.39999986], dtype=float32... how get pure values without types?
– Brans Ds
Nov 30 '16 at 13:05
add a comment |
I got something like: array([-23.], dtype=float32), array([-0.39999986], dtype=float32... how get pure values without types?
– Brans Ds
Nov 30 '16 at 13:05
I got something like: array([-23.], dtype=float32), array([-0.39999986], dtype=float32... how get pure values without types?
– Brans Ds
Nov 30 '16 at 13:05
I got something like: array([-23.], dtype=float32), array([-0.39999986], dtype=float32... how get pure values without types?
– Brans Ds
Nov 30 '16 at 13:05
add a comment |
The following code writes python lists into columns in csv
import csv
from itertools import zip_longest
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j']
d = [list1, list2]
export_data = zip_longest(*d, fillvalue = '')
with open('numbers.csv', 'w', encoding="ISO-8859-1", newline='') as myfile:
wr = csv.writer(myfile)
wr.writerow(("List1", "List2"))
wr.writerows(export_data)
myfile.close()
The output looks like this
add a comment |
The following code writes python lists into columns in csv
import csv
from itertools import zip_longest
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j']
d = [list1, list2]
export_data = zip_longest(*d, fillvalue = '')
with open('numbers.csv', 'w', encoding="ISO-8859-1", newline='') as myfile:
wr = csv.writer(myfile)
wr.writerow(("List1", "List2"))
wr.writerows(export_data)
myfile.close()
The output looks like this
add a comment |
The following code writes python lists into columns in csv
import csv
from itertools import zip_longest
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j']
d = [list1, list2]
export_data = zip_longest(*d, fillvalue = '')
with open('numbers.csv', 'w', encoding="ISO-8859-1", newline='') as myfile:
wr = csv.writer(myfile)
wr.writerow(("List1", "List2"))
wr.writerows(export_data)
myfile.close()
The output looks like this
The following code writes python lists into columns in csv
import csv
from itertools import zip_longest
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j']
d = [list1, list2]
export_data = zip_longest(*d, fillvalue = '')
with open('numbers.csv', 'w', encoding="ISO-8859-1", newline='') as myfile:
wr = csv.writer(myfile)
wr.writerow(("List1", "List2"))
wr.writerows(export_data)
myfile.close()
The output looks like this
answered Nov 17 '17 at 13:40
Ashok Kumar JayaramanAshok Kumar Jayaraman
1,10511219
1,10511219
add a comment |
add a comment |
You can use izip
to combine your lists, and then iterate them
for val in itertools.izip(l1,l2,l3,l4,l5):
writer.writerow(val)
5
It's worth noting thatitertools.izip()
doesn't exist in Python 3.x, and thezip()
built-in gives an iterator, so that is a drop-in replacement. Also note that if you have a list of lists, you can use the unpacking operator (zip(*lists)
). This is better design than having variablesl1
,l2
, etc...
– Gareth Latty
Jul 17 '13 at 15:47
add a comment |
You can use izip
to combine your lists, and then iterate them
for val in itertools.izip(l1,l2,l3,l4,l5):
writer.writerow(val)
5
It's worth noting thatitertools.izip()
doesn't exist in Python 3.x, and thezip()
built-in gives an iterator, so that is a drop-in replacement. Also note that if you have a list of lists, you can use the unpacking operator (zip(*lists)
). This is better design than having variablesl1
,l2
, etc...
– Gareth Latty
Jul 17 '13 at 15:47
add a comment |
You can use izip
to combine your lists, and then iterate them
for val in itertools.izip(l1,l2,l3,l4,l5):
writer.writerow(val)
You can use izip
to combine your lists, and then iterate them
for val in itertools.izip(l1,l2,l3,l4,l5):
writer.writerow(val)
answered Jul 17 '13 at 15:45
jh314jh314
20.6k134870
20.6k134870
5
It's worth noting thatitertools.izip()
doesn't exist in Python 3.x, and thezip()
built-in gives an iterator, so that is a drop-in replacement. Also note that if you have a list of lists, you can use the unpacking operator (zip(*lists)
). This is better design than having variablesl1
,l2
, etc...
– Gareth Latty
Jul 17 '13 at 15:47
add a comment |
5
It's worth noting thatitertools.izip()
doesn't exist in Python 3.x, and thezip()
built-in gives an iterator, so that is a drop-in replacement. Also note that if you have a list of lists, you can use the unpacking operator (zip(*lists)
). This is better design than having variablesl1
,l2
, etc...
– Gareth Latty
Jul 17 '13 at 15:47
5
5
It's worth noting that
itertools.izip()
doesn't exist in Python 3.x, and the zip()
built-in gives an iterator, so that is a drop-in replacement. Also note that if you have a list of lists, you can use the unpacking operator (zip(*lists)
). This is better design than having variables l1
, l2
, etc...– Gareth Latty
Jul 17 '13 at 15:47
It's worth noting that
itertools.izip()
doesn't exist in Python 3.x, and the zip()
built-in gives an iterator, so that is a drop-in replacement. Also note that if you have a list of lists, you can use the unpacking operator (zip(*lists)
). This is better design than having variables l1
, l2
, etc...– Gareth Latty
Jul 17 '13 at 15:47
add a comment |
import csv
dic = {firstcol,secondcol} #dictionary
csv = open('result.csv', "w")
for key in dic.keys():
row ="n"+ str(key) + "," + str(dic[key])
csv.write(row)
2
Your contribution would be more meaningful if you would include some explanation about why this approach should work and be an improvement over what has already been proposed and accepted as the answer.
– Cindy Meister
Oct 13 '18 at 21:17
add a comment |
import csv
dic = {firstcol,secondcol} #dictionary
csv = open('result.csv', "w")
for key in dic.keys():
row ="n"+ str(key) + "," + str(dic[key])
csv.write(row)
2
Your contribution would be more meaningful if you would include some explanation about why this approach should work and be an improvement over what has already been proposed and accepted as the answer.
– Cindy Meister
Oct 13 '18 at 21:17
add a comment |
import csv
dic = {firstcol,secondcol} #dictionary
csv = open('result.csv', "w")
for key in dic.keys():
row ="n"+ str(key) + "," + str(dic[key])
csv.write(row)
import csv
dic = {firstcol,secondcol} #dictionary
csv = open('result.csv', "w")
for key in dic.keys():
row ="n"+ str(key) + "," + str(dic[key])
csv.write(row)
answered Oct 13 '18 at 18:45
Shb8086Shb8086
111
111
2
Your contribution would be more meaningful if you would include some explanation about why this approach should work and be an improvement over what has already been proposed and accepted as the answer.
– Cindy Meister
Oct 13 '18 at 21:17
add a comment |
2
Your contribution would be more meaningful if you would include some explanation about why this approach should work and be an improvement over what has already been proposed and accepted as the answer.
– Cindy Meister
Oct 13 '18 at 21:17
2
2
Your contribution would be more meaningful if you would include some explanation about why this approach should work and be an improvement over what has already been proposed and accepted as the answer.
– Cindy Meister
Oct 13 '18 at 21:17
Your contribution would be more meaningful if you would include some explanation about why this approach should work and be an improvement over what has already been proposed and accepted as the answer.
– Cindy Meister
Oct 13 '18 at 21:17
add a comment |
If you are happy to use a 3rd party library, you can do this with Pandas. The benefits include seamless access to specialized methods and row / column labeling:
import pandas as pd
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
df = pd.DataFrame(list(zip(*[list1, list2, list3]))).add_prefix('Col')
df.to_csv('file.csv', index=False)
print(df)
Col0 Col1 Col2
0 1 4 7
1 2 5 8
2 3 6 9
add a comment |
If you are happy to use a 3rd party library, you can do this with Pandas. The benefits include seamless access to specialized methods and row / column labeling:
import pandas as pd
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
df = pd.DataFrame(list(zip(*[list1, list2, list3]))).add_prefix('Col')
df.to_csv('file.csv', index=False)
print(df)
Col0 Col1 Col2
0 1 4 7
1 2 5 8
2 3 6 9
add a comment |
If you are happy to use a 3rd party library, you can do this with Pandas. The benefits include seamless access to specialized methods and row / column labeling:
import pandas as pd
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
df = pd.DataFrame(list(zip(*[list1, list2, list3]))).add_prefix('Col')
df.to_csv('file.csv', index=False)
print(df)
Col0 Col1 Col2
0 1 4 7
1 2 5 8
2 3 6 9
If you are happy to use a 3rd party library, you can do this with Pandas. The benefits include seamless access to specialized methods and row / column labeling:
import pandas as pd
list1 = [1, 2, 3]
list2 = [4, 5, 6]
list3 = [7, 8, 9]
df = pd.DataFrame(list(zip(*[list1, list2, list3]))).add_prefix('Col')
df.to_csv('file.csv', index=False)
print(df)
Col0 Col1 Col2
0 1 4 7
1 2 5 8
2 3 6 9
answered Nov 22 '18 at 12:20


jppjpp
103k2166116
103k2166116
add a comment |
add a comment |
I didn't want to import anything other than csv, and all my lists have the same number of items. The top answer here seems to make the lists into one row each, instead of one column each. Thus I took the answers here and came up with this:
import csv
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j','k']
with open('C:/test/numbers.csv', 'wb+') as myfile:
wr = csv.writer(myfile)
wr.writerow(("list1", "list2"))
rcount = 0
for row in list1:
wr.writerow((list1[rcount], list2[rcount]))
rcount = rcount + 1
myfile.close()
add a comment |
I didn't want to import anything other than csv, and all my lists have the same number of items. The top answer here seems to make the lists into one row each, instead of one column each. Thus I took the answers here and came up with this:
import csv
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j','k']
with open('C:/test/numbers.csv', 'wb+') as myfile:
wr = csv.writer(myfile)
wr.writerow(("list1", "list2"))
rcount = 0
for row in list1:
wr.writerow((list1[rcount], list2[rcount]))
rcount = rcount + 1
myfile.close()
add a comment |
I didn't want to import anything other than csv, and all my lists have the same number of items. The top answer here seems to make the lists into one row each, instead of one column each. Thus I took the answers here and came up with this:
import csv
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j','k']
with open('C:/test/numbers.csv', 'wb+') as myfile:
wr = csv.writer(myfile)
wr.writerow(("list1", "list2"))
rcount = 0
for row in list1:
wr.writerow((list1[rcount], list2[rcount]))
rcount = rcount + 1
myfile.close()
I didn't want to import anything other than csv, and all my lists have the same number of items. The top answer here seems to make the lists into one row each, instead of one column each. Thus I took the answers here and came up with this:
import csv
list1 = ['a', 'b', 'c', 'd', 'e']
list2 = ['f', 'g', 'i', 'j','k']
with open('C:/test/numbers.csv', 'wb+') as myfile:
wr = csv.writer(myfile)
wr.writerow(("list1", "list2"))
rcount = 0
for row in list1:
wr.writerow((list1[rcount], list2[rcount]))
rcount = rcount + 1
myfile.close()
edited Feb 1 at 16:46
answered Feb 1 at 16:40
BartatsBartats
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f17704244%2fwriting-python-lists-to-columns-in-csv%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FWssiaxIy4 J6qpi3uA1WPwD qPOJ,xEP51