undefined method `image' for #
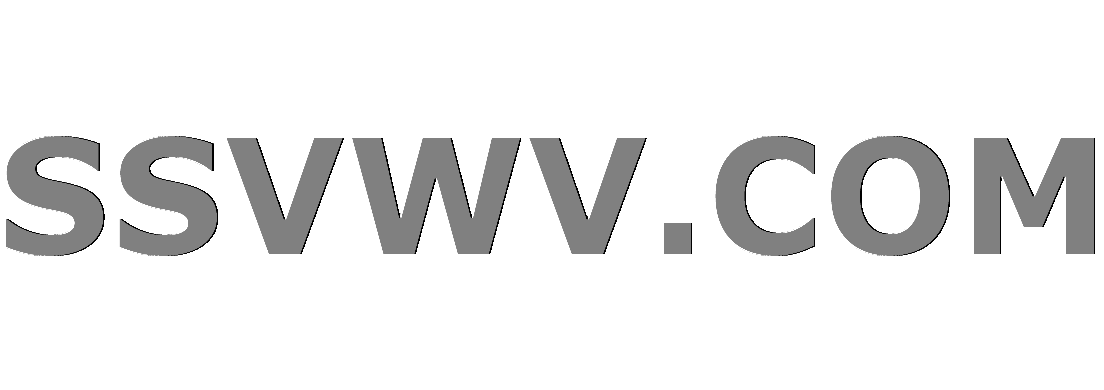
Multi tool use
I am using the Paperclip gem. A user can submit a location with an image. I do not want the user to duplicate an already submitted location, but they can add an image to it.
I have been trying to figure it out. I will leave the important code below:
location.rb
class Location < ApplicationRecord
has_many :submissions
has_many :users, through: :submissions
# Allows submission objects
accepts_nested_attributes_for :submissions
submission.rb
class Submission < ApplicationRecord
belongs_to :user
belongs_to :location
has_attached_file :image, styles: { large: "600x600>", medium: "300x300>", thumb: "150x150#" }
validates_attachment_content_type :image, content_type: /Aimage/.*z/
locations_controller.rb
class LocationsController < ApplicationController
# Before actions get routed and ran, find_location will occur
before_action :find_location, only: [:show, :edit, :update, :destroy]
# For the Locations/index.html.erb
def index
@locations = Location.all
end
# Binds submission object to the form in new.html.erb
def new
@locations = Location.new
@locations.submissions.build
end
# For creating a new location in the new.html.erb form
def create
@locations = Location.new(user_params)
# Everything went well. User will be sent to @locations show page
if @locations.save
# Redirects to user submitted locations
redirect_to @locations
else
render 'new'
end
end
# Finds new location user submitted by its unique id
def show
end
# Allowing user to update their submitted location
def edit
end
# Updates users edited submission
def update
if @locations.update(user_params)
# Redirects to user submitted locations
redirect_to @locations
else
render 'edit'
end
end
# Deletes users submission
def destroy
@locations.destroy
# Redirects to user submitted locations
redirect_to @locations
end
private
# Used for finding user submitted location (Prevents DRY)
def find_location
@locations = Location.find(params[:id])
end
# Strong parameters for security - Defines what can be update/created in location model
def user_params
params.require(:location).permit(:city, :state, :submissions_attributes => [:image])
end
end
_form.html.erb
<%= form_for @locations, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for :submissions do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
show.html.erb
<h3>Image: <%= image_tag @locations.image.url(:medium) %></h3>
I get an error:
"undefined method `image' for.."
ruby-on-rails forms paperclip
add a comment |
I am using the Paperclip gem. A user can submit a location with an image. I do not want the user to duplicate an already submitted location, but they can add an image to it.
I have been trying to figure it out. I will leave the important code below:
location.rb
class Location < ApplicationRecord
has_many :submissions
has_many :users, through: :submissions
# Allows submission objects
accepts_nested_attributes_for :submissions
submission.rb
class Submission < ApplicationRecord
belongs_to :user
belongs_to :location
has_attached_file :image, styles: { large: "600x600>", medium: "300x300>", thumb: "150x150#" }
validates_attachment_content_type :image, content_type: /Aimage/.*z/
locations_controller.rb
class LocationsController < ApplicationController
# Before actions get routed and ran, find_location will occur
before_action :find_location, only: [:show, :edit, :update, :destroy]
# For the Locations/index.html.erb
def index
@locations = Location.all
end
# Binds submission object to the form in new.html.erb
def new
@locations = Location.new
@locations.submissions.build
end
# For creating a new location in the new.html.erb form
def create
@locations = Location.new(user_params)
# Everything went well. User will be sent to @locations show page
if @locations.save
# Redirects to user submitted locations
redirect_to @locations
else
render 'new'
end
end
# Finds new location user submitted by its unique id
def show
end
# Allowing user to update their submitted location
def edit
end
# Updates users edited submission
def update
if @locations.update(user_params)
# Redirects to user submitted locations
redirect_to @locations
else
render 'edit'
end
end
# Deletes users submission
def destroy
@locations.destroy
# Redirects to user submitted locations
redirect_to @locations
end
private
# Used for finding user submitted location (Prevents DRY)
def find_location
@locations = Location.find(params[:id])
end
# Strong parameters for security - Defines what can be update/created in location model
def user_params
params.require(:location).permit(:city, :state, :submissions_attributes => [:image])
end
end
_form.html.erb
<%= form_for @locations, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for :submissions do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
show.html.erb
<h3>Image: <%= image_tag @locations.image.url(:medium) %></h3>
I get an error:
"undefined method `image' for.."
ruby-on-rails forms paperclip
add a comment |
I am using the Paperclip gem. A user can submit a location with an image. I do not want the user to duplicate an already submitted location, but they can add an image to it.
I have been trying to figure it out. I will leave the important code below:
location.rb
class Location < ApplicationRecord
has_many :submissions
has_many :users, through: :submissions
# Allows submission objects
accepts_nested_attributes_for :submissions
submission.rb
class Submission < ApplicationRecord
belongs_to :user
belongs_to :location
has_attached_file :image, styles: { large: "600x600>", medium: "300x300>", thumb: "150x150#" }
validates_attachment_content_type :image, content_type: /Aimage/.*z/
locations_controller.rb
class LocationsController < ApplicationController
# Before actions get routed and ran, find_location will occur
before_action :find_location, only: [:show, :edit, :update, :destroy]
# For the Locations/index.html.erb
def index
@locations = Location.all
end
# Binds submission object to the form in new.html.erb
def new
@locations = Location.new
@locations.submissions.build
end
# For creating a new location in the new.html.erb form
def create
@locations = Location.new(user_params)
# Everything went well. User will be sent to @locations show page
if @locations.save
# Redirects to user submitted locations
redirect_to @locations
else
render 'new'
end
end
# Finds new location user submitted by its unique id
def show
end
# Allowing user to update their submitted location
def edit
end
# Updates users edited submission
def update
if @locations.update(user_params)
# Redirects to user submitted locations
redirect_to @locations
else
render 'edit'
end
end
# Deletes users submission
def destroy
@locations.destroy
# Redirects to user submitted locations
redirect_to @locations
end
private
# Used for finding user submitted location (Prevents DRY)
def find_location
@locations = Location.find(params[:id])
end
# Strong parameters for security - Defines what can be update/created in location model
def user_params
params.require(:location).permit(:city, :state, :submissions_attributes => [:image])
end
end
_form.html.erb
<%= form_for @locations, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for :submissions do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
show.html.erb
<h3>Image: <%= image_tag @locations.image.url(:medium) %></h3>
I get an error:
"undefined method `image' for.."
ruby-on-rails forms paperclip
I am using the Paperclip gem. A user can submit a location with an image. I do not want the user to duplicate an already submitted location, but they can add an image to it.
I have been trying to figure it out. I will leave the important code below:
location.rb
class Location < ApplicationRecord
has_many :submissions
has_many :users, through: :submissions
# Allows submission objects
accepts_nested_attributes_for :submissions
submission.rb
class Submission < ApplicationRecord
belongs_to :user
belongs_to :location
has_attached_file :image, styles: { large: "600x600>", medium: "300x300>", thumb: "150x150#" }
validates_attachment_content_type :image, content_type: /Aimage/.*z/
locations_controller.rb
class LocationsController < ApplicationController
# Before actions get routed and ran, find_location will occur
before_action :find_location, only: [:show, :edit, :update, :destroy]
# For the Locations/index.html.erb
def index
@locations = Location.all
end
# Binds submission object to the form in new.html.erb
def new
@locations = Location.new
@locations.submissions.build
end
# For creating a new location in the new.html.erb form
def create
@locations = Location.new(user_params)
# Everything went well. User will be sent to @locations show page
if @locations.save
# Redirects to user submitted locations
redirect_to @locations
else
render 'new'
end
end
# Finds new location user submitted by its unique id
def show
end
# Allowing user to update their submitted location
def edit
end
# Updates users edited submission
def update
if @locations.update(user_params)
# Redirects to user submitted locations
redirect_to @locations
else
render 'edit'
end
end
# Deletes users submission
def destroy
@locations.destroy
# Redirects to user submitted locations
redirect_to @locations
end
private
# Used for finding user submitted location (Prevents DRY)
def find_location
@locations = Location.find(params[:id])
end
# Strong parameters for security - Defines what can be update/created in location model
def user_params
params.require(:location).permit(:city, :state, :submissions_attributes => [:image])
end
end
_form.html.erb
<%= form_for @locations, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for :submissions do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
show.html.erb
<h3>Image: <%= image_tag @locations.image.url(:medium) %></h3>
I get an error:
"undefined method `image' for.."
ruby-on-rails forms paperclip
ruby-on-rails forms paperclip
edited Nov 21 '18 at 5:42


sawa
132k29205304
132k29205304
asked Nov 21 '18 at 2:32
Felix DoeFelix Doe
1511112
1511112
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
It looks like you're trying to do form tags for collections of records, which I don't think will work. Instead, I think you need a structure something like:
<% @locations.each do |location| %>
<%= form_for location, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for location.submissions.new do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
both the form_for
and fields_for
need to point to a singular resource.
Side note: Paperclip has been deprecated, and it is recommended that you instead use the Rails internal ActiveStorage.
So I took your advise and converted to ActiveStorage. I think my main problem is that I have a Many-To-Many relationship which is throwing me off. I think I am able to add an image but I am having trouble displaying it. In my show.html.erb for locations I have "<%= image_tag(@locations.image) %>" and I am still getting the same error as above. Do you have any clue on what I should do here?
– Felix Doe
Nov 22 '18 at 18:25
So the thing to keep in mind here is that you're dealing with an array of locations that each have an array of submissions with images. So you need to loop over the locations, then loop over each location's submissions, then render an image tag for each of those. Something like<% @locations.each do |location| %><% location.submissions.each do |submission| %><%= image_tag(submission.image) %><% end %><% end %>
.
– lobati
Nov 22 '18 at 23:17
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404524%2fundefined-method-image-for-location-0x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It looks like you're trying to do form tags for collections of records, which I don't think will work. Instead, I think you need a structure something like:
<% @locations.each do |location| %>
<%= form_for location, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for location.submissions.new do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
both the form_for
and fields_for
need to point to a singular resource.
Side note: Paperclip has been deprecated, and it is recommended that you instead use the Rails internal ActiveStorage.
So I took your advise and converted to ActiveStorage. I think my main problem is that I have a Many-To-Many relationship which is throwing me off. I think I am able to add an image but I am having trouble displaying it. In my show.html.erb for locations I have "<%= image_tag(@locations.image) %>" and I am still getting the same error as above. Do you have any clue on what I should do here?
– Felix Doe
Nov 22 '18 at 18:25
So the thing to keep in mind here is that you're dealing with an array of locations that each have an array of submissions with images. So you need to loop over the locations, then loop over each location's submissions, then render an image tag for each of those. Something like<% @locations.each do |location| %><% location.submissions.each do |submission| %><%= image_tag(submission.image) %><% end %><% end %>
.
– lobati
Nov 22 '18 at 23:17
add a comment |
It looks like you're trying to do form tags for collections of records, which I don't think will work. Instead, I think you need a structure something like:
<% @locations.each do |location| %>
<%= form_for location, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for location.submissions.new do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
both the form_for
and fields_for
need to point to a singular resource.
Side note: Paperclip has been deprecated, and it is recommended that you instead use the Rails internal ActiveStorage.
So I took your advise and converted to ActiveStorage. I think my main problem is that I have a Many-To-Many relationship which is throwing me off. I think I am able to add an image but I am having trouble displaying it. In my show.html.erb for locations I have "<%= image_tag(@locations.image) %>" and I am still getting the same error as above. Do you have any clue on what I should do here?
– Felix Doe
Nov 22 '18 at 18:25
So the thing to keep in mind here is that you're dealing with an array of locations that each have an array of submissions with images. So you need to loop over the locations, then loop over each location's submissions, then render an image tag for each of those. Something like<% @locations.each do |location| %><% location.submissions.each do |submission| %><%= image_tag(submission.image) %><% end %><% end %>
.
– lobati
Nov 22 '18 at 23:17
add a comment |
It looks like you're trying to do form tags for collections of records, which I don't think will work. Instead, I think you need a structure something like:
<% @locations.each do |location| %>
<%= form_for location, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for location.submissions.new do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
both the form_for
and fields_for
need to point to a singular resource.
Side note: Paperclip has been deprecated, and it is recommended that you instead use the Rails internal ActiveStorage.
It looks like you're trying to do form tags for collections of records, which I don't think will work. Instead, I think you need a structure something like:
<% @locations.each do |location| %>
<%= form_for location, html: {multipart: true} do |f| %>
.
.
.
<!-- User enters image-->
<%= f.fields_for location.submissions.new do |s| %>
<div class="form-group">
<h3>Upload Image:</h3>
<%= s.file_field :image, class: 'form-control' %>
</div>
<% end %>
<% end %>
both the form_for
and fields_for
need to point to a singular resource.
Side note: Paperclip has been deprecated, and it is recommended that you instead use the Rails internal ActiveStorage.
answered Nov 21 '18 at 4:46
lobatilobati
2,58432241
2,58432241
So I took your advise and converted to ActiveStorage. I think my main problem is that I have a Many-To-Many relationship which is throwing me off. I think I am able to add an image but I am having trouble displaying it. In my show.html.erb for locations I have "<%= image_tag(@locations.image) %>" and I am still getting the same error as above. Do you have any clue on what I should do here?
– Felix Doe
Nov 22 '18 at 18:25
So the thing to keep in mind here is that you're dealing with an array of locations that each have an array of submissions with images. So you need to loop over the locations, then loop over each location's submissions, then render an image tag for each of those. Something like<% @locations.each do |location| %><% location.submissions.each do |submission| %><%= image_tag(submission.image) %><% end %><% end %>
.
– lobati
Nov 22 '18 at 23:17
add a comment |
So I took your advise and converted to ActiveStorage. I think my main problem is that I have a Many-To-Many relationship which is throwing me off. I think I am able to add an image but I am having trouble displaying it. In my show.html.erb for locations I have "<%= image_tag(@locations.image) %>" and I am still getting the same error as above. Do you have any clue on what I should do here?
– Felix Doe
Nov 22 '18 at 18:25
So the thing to keep in mind here is that you're dealing with an array of locations that each have an array of submissions with images. So you need to loop over the locations, then loop over each location's submissions, then render an image tag for each of those. Something like<% @locations.each do |location| %><% location.submissions.each do |submission| %><%= image_tag(submission.image) %><% end %><% end %>
.
– lobati
Nov 22 '18 at 23:17
So I took your advise and converted to ActiveStorage. I think my main problem is that I have a Many-To-Many relationship which is throwing me off. I think I am able to add an image but I am having trouble displaying it. In my show.html.erb for locations I have "<%= image_tag(@locations.image) %>" and I am still getting the same error as above. Do you have any clue on what I should do here?
– Felix Doe
Nov 22 '18 at 18:25
So I took your advise and converted to ActiveStorage. I think my main problem is that I have a Many-To-Many relationship which is throwing me off. I think I am able to add an image but I am having trouble displaying it. In my show.html.erb for locations I have "<%= image_tag(@locations.image) %>" and I am still getting the same error as above. Do you have any clue on what I should do here?
– Felix Doe
Nov 22 '18 at 18:25
So the thing to keep in mind here is that you're dealing with an array of locations that each have an array of submissions with images. So you need to loop over the locations, then loop over each location's submissions, then render an image tag for each of those. Something like
<% @locations.each do |location| %><% location.submissions.each do |submission| %><%= image_tag(submission.image) %><% end %><% end %>
.– lobati
Nov 22 '18 at 23:17
So the thing to keep in mind here is that you're dealing with an array of locations that each have an array of submissions with images. So you need to loop over the locations, then loop over each location's submissions, then render an image tag for each of those. Something like
<% @locations.each do |location| %><% location.submissions.each do |submission| %><%= image_tag(submission.image) %><% end %><% end %>
.– lobati
Nov 22 '18 at 23:17
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53404524%2fundefined-method-image-for-location-0x%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IBs,0 9OKGQkN,0 X WJcq6GNyWIBInr377BUt,VBtd8D5dK s,G9hgQw,qcdjr4t17E7oQE 1,PInOt66KewwoeFsfrns,15,EDr