How to initialize a ctypes array in Python from command line
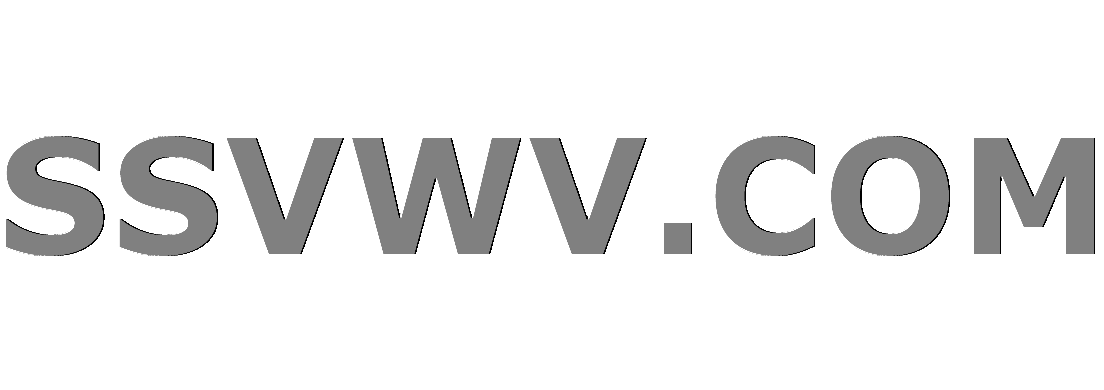
Multi tool use
So I have been tasked with writing a Python script which accesses a Win 32 DLL to perform some functionality. This script needs to accept parameters from the command line then output other parameters.
I am using ctypes since it is the easiest way I have found to pass parameters to the Win 32 DLL methods but I was lucky enough to have a fixed array of values from the command line which I solved by doing such:
seed = (ctypes.c_ubyte * 12)(
ctypes.c_ubyte(int(sys.argv[3], 16)), ctypes.c_ubyte(int(sys.argv[4], 16)),
ctypes.c_ubyte(int(sys.argv[5], 16)), ctypes.c_ubyte(int(sys.argv[6], 16)),
ctypes.c_ubyte(int(sys.argv[7], 16)), ctypes.c_ubyte(int(sys.argv[8], 16)),
ctypes.c_ubyte(int(sys.argv[9], 16)), ctypes.c_ubyte(int(sys.argv[10], 16)),
ctypes.c_ubyte(int(sys.argv[11], 16)), ctypes.c_ubyte(int(sys.argv[12], 16)),
ctypes.c_ubyte(int(sys.argv[13], 16)), ctypes.c_ubyte(int(sys.argv[14], 16))
)
But this is not dynamic in anyway and I tried to perform this array initialization with a for loop inside the array initialization but without success.
I am completely new to Python and find it rather difficult to do what I consider simple tasks in other languages (not bashing the language, just not finding it as intuitive as other languages for such tasks).
So, is there a way to simplify initializing this array where there could be a variable amount of entries per se?
I have searched and searched and nothing I have found has solved my problem.
All positive and negative comments are always appreciated and both will always serve as a learning experience :)
python ctypes python-2.5
add a comment |
So I have been tasked with writing a Python script which accesses a Win 32 DLL to perform some functionality. This script needs to accept parameters from the command line then output other parameters.
I am using ctypes since it is the easiest way I have found to pass parameters to the Win 32 DLL methods but I was lucky enough to have a fixed array of values from the command line which I solved by doing such:
seed = (ctypes.c_ubyte * 12)(
ctypes.c_ubyte(int(sys.argv[3], 16)), ctypes.c_ubyte(int(sys.argv[4], 16)),
ctypes.c_ubyte(int(sys.argv[5], 16)), ctypes.c_ubyte(int(sys.argv[6], 16)),
ctypes.c_ubyte(int(sys.argv[7], 16)), ctypes.c_ubyte(int(sys.argv[8], 16)),
ctypes.c_ubyte(int(sys.argv[9], 16)), ctypes.c_ubyte(int(sys.argv[10], 16)),
ctypes.c_ubyte(int(sys.argv[11], 16)), ctypes.c_ubyte(int(sys.argv[12], 16)),
ctypes.c_ubyte(int(sys.argv[13], 16)), ctypes.c_ubyte(int(sys.argv[14], 16))
)
But this is not dynamic in anyway and I tried to perform this array initialization with a for loop inside the array initialization but without success.
I am completely new to Python and find it rather difficult to do what I consider simple tasks in other languages (not bashing the language, just not finding it as intuitive as other languages for such tasks).
So, is there a way to simplify initializing this array where there could be a variable amount of entries per se?
I have searched and searched and nothing I have found has solved my problem.
All positive and negative comments are always appreciated and both will always serve as a learning experience :)
python ctypes python-2.5
add a comment |
So I have been tasked with writing a Python script which accesses a Win 32 DLL to perform some functionality. This script needs to accept parameters from the command line then output other parameters.
I am using ctypes since it is the easiest way I have found to pass parameters to the Win 32 DLL methods but I was lucky enough to have a fixed array of values from the command line which I solved by doing such:
seed = (ctypes.c_ubyte * 12)(
ctypes.c_ubyte(int(sys.argv[3], 16)), ctypes.c_ubyte(int(sys.argv[4], 16)),
ctypes.c_ubyte(int(sys.argv[5], 16)), ctypes.c_ubyte(int(sys.argv[6], 16)),
ctypes.c_ubyte(int(sys.argv[7], 16)), ctypes.c_ubyte(int(sys.argv[8], 16)),
ctypes.c_ubyte(int(sys.argv[9], 16)), ctypes.c_ubyte(int(sys.argv[10], 16)),
ctypes.c_ubyte(int(sys.argv[11], 16)), ctypes.c_ubyte(int(sys.argv[12], 16)),
ctypes.c_ubyte(int(sys.argv[13], 16)), ctypes.c_ubyte(int(sys.argv[14], 16))
)
But this is not dynamic in anyway and I tried to perform this array initialization with a for loop inside the array initialization but without success.
I am completely new to Python and find it rather difficult to do what I consider simple tasks in other languages (not bashing the language, just not finding it as intuitive as other languages for such tasks).
So, is there a way to simplify initializing this array where there could be a variable amount of entries per se?
I have searched and searched and nothing I have found has solved my problem.
All positive and negative comments are always appreciated and both will always serve as a learning experience :)
python ctypes python-2.5
So I have been tasked with writing a Python script which accesses a Win 32 DLL to perform some functionality. This script needs to accept parameters from the command line then output other parameters.
I am using ctypes since it is the easiest way I have found to pass parameters to the Win 32 DLL methods but I was lucky enough to have a fixed array of values from the command line which I solved by doing such:
seed = (ctypes.c_ubyte * 12)(
ctypes.c_ubyte(int(sys.argv[3], 16)), ctypes.c_ubyte(int(sys.argv[4], 16)),
ctypes.c_ubyte(int(sys.argv[5], 16)), ctypes.c_ubyte(int(sys.argv[6], 16)),
ctypes.c_ubyte(int(sys.argv[7], 16)), ctypes.c_ubyte(int(sys.argv[8], 16)),
ctypes.c_ubyte(int(sys.argv[9], 16)), ctypes.c_ubyte(int(sys.argv[10], 16)),
ctypes.c_ubyte(int(sys.argv[11], 16)), ctypes.c_ubyte(int(sys.argv[12], 16)),
ctypes.c_ubyte(int(sys.argv[13], 16)), ctypes.c_ubyte(int(sys.argv[14], 16))
)
But this is not dynamic in anyway and I tried to perform this array initialization with a for loop inside the array initialization but without success.
I am completely new to Python and find it rather difficult to do what I consider simple tasks in other languages (not bashing the language, just not finding it as intuitive as other languages for such tasks).
So, is there a way to simplify initializing this array where there could be a variable amount of entries per se?
I have searched and searched and nothing I have found has solved my problem.
All positive and negative comments are always appreciated and both will always serve as a learning experience :)
python ctypes python-2.5
python ctypes python-2.5
edited Nov 21 '18 at 6:08
Mad Lee
537317
537317
asked Nov 21 '18 at 0:54
CCSCCS
189112
189112
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
From your example it looks like you have 14 parameters and the last 12 are hexadecimal bytes.
import ctypes
import sys
# Convert parameters 3-14 from hexadecimal strings to integers using a list comprehension.
L = [int(i,16) for i in sys.argv[3:15]]
# Initialize a byte array with those 12 parameters.
# *L passes each element of L as a separate parameter.
seed = (ctypes.c_ubyte * 12)(*L)
Thank you for your solution, I chose it as the answer because it is more anonymous method like, and only took two lines of code :) Thanks again for taking the time to answer, much appreciate!
– CCS
Nov 21 '18 at 22:19
add a comment |
Try this:
seed_list =
for i in sys.argv[3:]:
seed_list.append(ctypes.c_ubyte(int(i, 16)))
seed = (ctypes.c_ubyte * len(seed_list))(*seed_list)
I wish I could select both response as solutions because they both worked as expected. Thank you for your solution.
– CCS
Nov 21 '18 at 22:16
But this did allow me to initialize a large array which much less code, thanks again!
– CCS
Nov 21 '18 at 22:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403825%2fhow-to-initialize-a-ctypes-array-in-python-from-command-line%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
From your example it looks like you have 14 parameters and the last 12 are hexadecimal bytes.
import ctypes
import sys
# Convert parameters 3-14 from hexadecimal strings to integers using a list comprehension.
L = [int(i,16) for i in sys.argv[3:15]]
# Initialize a byte array with those 12 parameters.
# *L passes each element of L as a separate parameter.
seed = (ctypes.c_ubyte * 12)(*L)
Thank you for your solution, I chose it as the answer because it is more anonymous method like, and only took two lines of code :) Thanks again for taking the time to answer, much appreciate!
– CCS
Nov 21 '18 at 22:19
add a comment |
From your example it looks like you have 14 parameters and the last 12 are hexadecimal bytes.
import ctypes
import sys
# Convert parameters 3-14 from hexadecimal strings to integers using a list comprehension.
L = [int(i,16) for i in sys.argv[3:15]]
# Initialize a byte array with those 12 parameters.
# *L passes each element of L as a separate parameter.
seed = (ctypes.c_ubyte * 12)(*L)
Thank you for your solution, I chose it as the answer because it is more anonymous method like, and only took two lines of code :) Thanks again for taking the time to answer, much appreciate!
– CCS
Nov 21 '18 at 22:19
add a comment |
From your example it looks like you have 14 parameters and the last 12 are hexadecimal bytes.
import ctypes
import sys
# Convert parameters 3-14 from hexadecimal strings to integers using a list comprehension.
L = [int(i,16) for i in sys.argv[3:15]]
# Initialize a byte array with those 12 parameters.
# *L passes each element of L as a separate parameter.
seed = (ctypes.c_ubyte * 12)(*L)
From your example it looks like you have 14 parameters and the last 12 are hexadecimal bytes.
import ctypes
import sys
# Convert parameters 3-14 from hexadecimal strings to integers using a list comprehension.
L = [int(i,16) for i in sys.argv[3:15]]
# Initialize a byte array with those 12 parameters.
# *L passes each element of L as a separate parameter.
seed = (ctypes.c_ubyte * 12)(*L)
answered Nov 21 '18 at 8:53


Mark TolonenMark Tolonen
94.6k12114176
94.6k12114176
Thank you for your solution, I chose it as the answer because it is more anonymous method like, and only took two lines of code :) Thanks again for taking the time to answer, much appreciate!
– CCS
Nov 21 '18 at 22:19
add a comment |
Thank you for your solution, I chose it as the answer because it is more anonymous method like, and only took two lines of code :) Thanks again for taking the time to answer, much appreciate!
– CCS
Nov 21 '18 at 22:19
Thank you for your solution, I chose it as the answer because it is more anonymous method like, and only took two lines of code :) Thanks again for taking the time to answer, much appreciate!
– CCS
Nov 21 '18 at 22:19
Thank you for your solution, I chose it as the answer because it is more anonymous method like, and only took two lines of code :) Thanks again for taking the time to answer, much appreciate!
– CCS
Nov 21 '18 at 22:19
add a comment |
Try this:
seed_list =
for i in sys.argv[3:]:
seed_list.append(ctypes.c_ubyte(int(i, 16)))
seed = (ctypes.c_ubyte * len(seed_list))(*seed_list)
I wish I could select both response as solutions because they both worked as expected. Thank you for your solution.
– CCS
Nov 21 '18 at 22:16
But this did allow me to initialize a large array which much less code, thanks again!
– CCS
Nov 21 '18 at 22:45
add a comment |
Try this:
seed_list =
for i in sys.argv[3:]:
seed_list.append(ctypes.c_ubyte(int(i, 16)))
seed = (ctypes.c_ubyte * len(seed_list))(*seed_list)
I wish I could select both response as solutions because they both worked as expected. Thank you for your solution.
– CCS
Nov 21 '18 at 22:16
But this did allow me to initialize a large array which much less code, thanks again!
– CCS
Nov 21 '18 at 22:45
add a comment |
Try this:
seed_list =
for i in sys.argv[3:]:
seed_list.append(ctypes.c_ubyte(int(i, 16)))
seed = (ctypes.c_ubyte * len(seed_list))(*seed_list)
Try this:
seed_list =
for i in sys.argv[3:]:
seed_list.append(ctypes.c_ubyte(int(i, 16)))
seed = (ctypes.c_ubyte * len(seed_list))(*seed_list)
answered Nov 21 '18 at 5:31
Mad LeeMad Lee
537317
537317
I wish I could select both response as solutions because they both worked as expected. Thank you for your solution.
– CCS
Nov 21 '18 at 22:16
But this did allow me to initialize a large array which much less code, thanks again!
– CCS
Nov 21 '18 at 22:45
add a comment |
I wish I could select both response as solutions because they both worked as expected. Thank you for your solution.
– CCS
Nov 21 '18 at 22:16
But this did allow me to initialize a large array which much less code, thanks again!
– CCS
Nov 21 '18 at 22:45
I wish I could select both response as solutions because they both worked as expected. Thank you for your solution.
– CCS
Nov 21 '18 at 22:16
I wish I could select both response as solutions because they both worked as expected. Thank you for your solution.
– CCS
Nov 21 '18 at 22:16
But this did allow me to initialize a large array which much less code, thanks again!
– CCS
Nov 21 '18 at 22:45
But this did allow me to initialize a large array which much less code, thanks again!
– CCS
Nov 21 '18 at 22:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403825%2fhow-to-initialize-a-ctypes-array-in-python-from-command-line%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
szy,CE18PSpi U,v,UpWN7WOCaoptd,LknsCoqEU91OMRdnibGhY2ogIuB