How to stop & start Pastel Gradient Animation between views
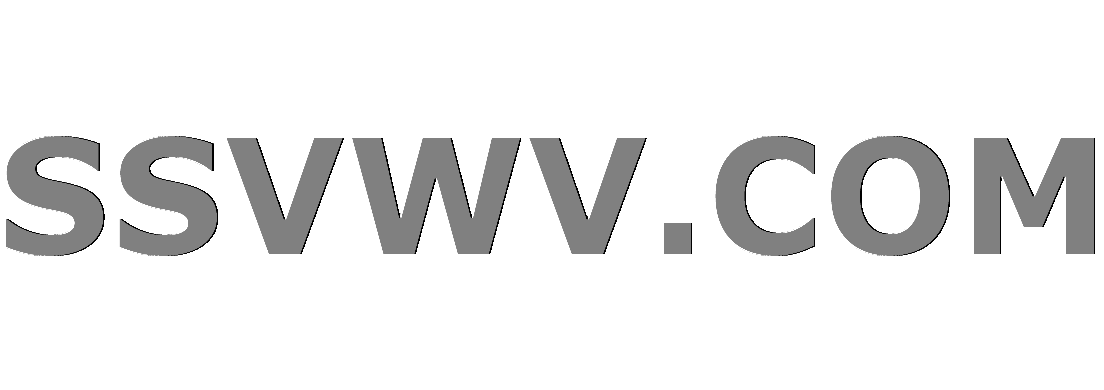
Multi tool use
I'm using a pod from github called Pastel
https://github.com/cruisediary/Pastel
It allows for an easy gradient color animation for background views. My problem is the animated gradient stops when moving to another UIView and going back to the original. Any idea on how to start and stop this animation while going to other tabs or views?
This is the main part of pod file `
import UIKit
open class PastelView: UIView {
private struct Animation {
static let keyPath = "colors"
static let key = "ColorChange"
}
//MARK: - Custom Direction
open var startPoint: CGPoint = PastelPoint.topRight.point
open var endPoint: CGPoint = PastelPoint.bottomLeft.point
open var startPastelPoint = PastelPoint.topRight {
didSet {
startPoint = startPastelPoint.point
}
}
open var endPastelPoint = PastelPoint.bottomLeft {
didSet {
endPoint = endPastelPoint.point
}
}
//MARK: - Custom Duration
open var animationDuration: TimeInterval = 5.0
fileprivate let gradient = CAGradientLayer()
private var currentGradient: Int = 0
private var colors: [UIColor] = [UIColor(red: 156/255, green: 39/255, blue: 176/255, alpha: 1.0),
UIColor(red: 255/255, green: 64/255, blue: 129/255, alpha: 1.0),
UIColor(red: 123/255, green: 31/255, blue: 162/255, alpha: 1.0),
UIColor(red: 32/255, green: 76/255, blue: 255/255, alpha: 1.0),
UIColor(red: 32/255, green: 158/255, blue: 255/255, alpha: 1.0),
UIColor(red: 90/255, green: 120/255, blue: 127/255, alpha: 1.0),
UIColor(red: 58/255, green: 255/255, blue: 217/255, alpha: 1.0)]
public override init(frame: CGRect) {
super.init(frame: frame)
}
public required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
open override func awakeFromNib() {
super.awakeFromNib()
}
open override func layoutSubviews() {
super.layoutSubviews()
gradient.frame = bounds
}
public func startAnimation() {
gradient.removeAllAnimations()
setup()
animateGradient()
}
fileprivate func setup() {
gradient.frame = bounds
gradient.colors = currentGradientSet()
gradient.startPoint = startPoint
gradient.endPoint = endPoint
gradient.drawsAsynchronously = true
layer.insertSublayer(gradient, at: 0)
}
fileprivate func currentGradientSet() -> [CGColor] {
guard colors.count > 0 else { return }
return [colors[currentGradient % colors.count].cgColor,
colors[(currentGradient + 1) % colors.count].cgColor]
}
public func setColors(_ colors: [UIColor]) {
guard colors.count > 0 else { return }
self.colors = colors
}
public func setPastelGradient(_ gradient: PastelGradient) {
setColors(gradient.colors())
}
public func addcolor(_ color: UIColor) {
self.colors.append(color)
}
func animateGradient() {
currentGradient += 1
let animation = CABasicAnimation(keyPath: Animation.keyPath)
animation.duration = animationDuration
animation.toValue = currentGradientSet()
animation.fillMode = kCAFillModeForwards
animation.isRemovedOnCompletion = false
animation.delegate = self
animation.autoreverses = true
animation.repeatCount = Float.infinity
gradient.add(animation, forKey: Animation.key)
}
open override func removeFromSuperview() {
super.removeFromSuperview()
gradient.removeAllAnimations()
gradient.removeFromSuperlayer()
extension PastelView: CAAnimationDelegate {
public func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
if flag {
gradient.colors = currentGradientSet()
animateGradient()
}
}
and is shown in view controller like
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
//Moving Gradient View
let pastelView = PastelView(frame: view.bounds)
//MARK: - Custom Direction
pastelView.startPastelPoint = .bottomRight
pastelView.endPastelPoint = .topLeft
//MARK: - Custom Duration
pastelView.animationDuration = 1.60
//MARK: - Custom Color
pastelView.setColors([UIColor(red: 255/255, green: 30/255, blue: 140/255, alpha: 1.0),
UIColor(red: 255/255, green: 48/255, blue: 129/255, alpha: 1.0),
UIColor(red: 254/255, green: 66/255, blue: 121/255, alpha: 1.0),
UIColor(red: 253/255, green: 91/255, blue: 114/255, alpha: 1.0),
UIColor(red: 253/255, green: 105/255, blue: 109/255, alpha: 1.0),
])
pastelView.startAnimation()
view.insertSubview(pastelView, at: 0)
}
swift xcode gradient caanimation cagradientlayer
add a comment |
I'm using a pod from github called Pastel
https://github.com/cruisediary/Pastel
It allows for an easy gradient color animation for background views. My problem is the animated gradient stops when moving to another UIView and going back to the original. Any idea on how to start and stop this animation while going to other tabs or views?
This is the main part of pod file `
import UIKit
open class PastelView: UIView {
private struct Animation {
static let keyPath = "colors"
static let key = "ColorChange"
}
//MARK: - Custom Direction
open var startPoint: CGPoint = PastelPoint.topRight.point
open var endPoint: CGPoint = PastelPoint.bottomLeft.point
open var startPastelPoint = PastelPoint.topRight {
didSet {
startPoint = startPastelPoint.point
}
}
open var endPastelPoint = PastelPoint.bottomLeft {
didSet {
endPoint = endPastelPoint.point
}
}
//MARK: - Custom Duration
open var animationDuration: TimeInterval = 5.0
fileprivate let gradient = CAGradientLayer()
private var currentGradient: Int = 0
private var colors: [UIColor] = [UIColor(red: 156/255, green: 39/255, blue: 176/255, alpha: 1.0),
UIColor(red: 255/255, green: 64/255, blue: 129/255, alpha: 1.0),
UIColor(red: 123/255, green: 31/255, blue: 162/255, alpha: 1.0),
UIColor(red: 32/255, green: 76/255, blue: 255/255, alpha: 1.0),
UIColor(red: 32/255, green: 158/255, blue: 255/255, alpha: 1.0),
UIColor(red: 90/255, green: 120/255, blue: 127/255, alpha: 1.0),
UIColor(red: 58/255, green: 255/255, blue: 217/255, alpha: 1.0)]
public override init(frame: CGRect) {
super.init(frame: frame)
}
public required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
open override func awakeFromNib() {
super.awakeFromNib()
}
open override func layoutSubviews() {
super.layoutSubviews()
gradient.frame = bounds
}
public func startAnimation() {
gradient.removeAllAnimations()
setup()
animateGradient()
}
fileprivate func setup() {
gradient.frame = bounds
gradient.colors = currentGradientSet()
gradient.startPoint = startPoint
gradient.endPoint = endPoint
gradient.drawsAsynchronously = true
layer.insertSublayer(gradient, at: 0)
}
fileprivate func currentGradientSet() -> [CGColor] {
guard colors.count > 0 else { return }
return [colors[currentGradient % colors.count].cgColor,
colors[(currentGradient + 1) % colors.count].cgColor]
}
public func setColors(_ colors: [UIColor]) {
guard colors.count > 0 else { return }
self.colors = colors
}
public func setPastelGradient(_ gradient: PastelGradient) {
setColors(gradient.colors())
}
public func addcolor(_ color: UIColor) {
self.colors.append(color)
}
func animateGradient() {
currentGradient += 1
let animation = CABasicAnimation(keyPath: Animation.keyPath)
animation.duration = animationDuration
animation.toValue = currentGradientSet()
animation.fillMode = kCAFillModeForwards
animation.isRemovedOnCompletion = false
animation.delegate = self
animation.autoreverses = true
animation.repeatCount = Float.infinity
gradient.add(animation, forKey: Animation.key)
}
open override func removeFromSuperview() {
super.removeFromSuperview()
gradient.removeAllAnimations()
gradient.removeFromSuperlayer()
extension PastelView: CAAnimationDelegate {
public func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
if flag {
gradient.colors = currentGradientSet()
animateGradient()
}
}
and is shown in view controller like
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
//Moving Gradient View
let pastelView = PastelView(frame: view.bounds)
//MARK: - Custom Direction
pastelView.startPastelPoint = .bottomRight
pastelView.endPastelPoint = .topLeft
//MARK: - Custom Duration
pastelView.animationDuration = 1.60
//MARK: - Custom Color
pastelView.setColors([UIColor(red: 255/255, green: 30/255, blue: 140/255, alpha: 1.0),
UIColor(red: 255/255, green: 48/255, blue: 129/255, alpha: 1.0),
UIColor(red: 254/255, green: 66/255, blue: 121/255, alpha: 1.0),
UIColor(red: 253/255, green: 91/255, blue: 114/255, alpha: 1.0),
UIColor(red: 253/255, green: 105/255, blue: 109/255, alpha: 1.0),
])
pastelView.startAnimation()
view.insertSubview(pastelView, at: 0)
}
swift xcode gradient caanimation cagradientlayer
add a comment |
I'm using a pod from github called Pastel
https://github.com/cruisediary/Pastel
It allows for an easy gradient color animation for background views. My problem is the animated gradient stops when moving to another UIView and going back to the original. Any idea on how to start and stop this animation while going to other tabs or views?
This is the main part of pod file `
import UIKit
open class PastelView: UIView {
private struct Animation {
static let keyPath = "colors"
static let key = "ColorChange"
}
//MARK: - Custom Direction
open var startPoint: CGPoint = PastelPoint.topRight.point
open var endPoint: CGPoint = PastelPoint.bottomLeft.point
open var startPastelPoint = PastelPoint.topRight {
didSet {
startPoint = startPastelPoint.point
}
}
open var endPastelPoint = PastelPoint.bottomLeft {
didSet {
endPoint = endPastelPoint.point
}
}
//MARK: - Custom Duration
open var animationDuration: TimeInterval = 5.0
fileprivate let gradient = CAGradientLayer()
private var currentGradient: Int = 0
private var colors: [UIColor] = [UIColor(red: 156/255, green: 39/255, blue: 176/255, alpha: 1.0),
UIColor(red: 255/255, green: 64/255, blue: 129/255, alpha: 1.0),
UIColor(red: 123/255, green: 31/255, blue: 162/255, alpha: 1.0),
UIColor(red: 32/255, green: 76/255, blue: 255/255, alpha: 1.0),
UIColor(red: 32/255, green: 158/255, blue: 255/255, alpha: 1.0),
UIColor(red: 90/255, green: 120/255, blue: 127/255, alpha: 1.0),
UIColor(red: 58/255, green: 255/255, blue: 217/255, alpha: 1.0)]
public override init(frame: CGRect) {
super.init(frame: frame)
}
public required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
open override func awakeFromNib() {
super.awakeFromNib()
}
open override func layoutSubviews() {
super.layoutSubviews()
gradient.frame = bounds
}
public func startAnimation() {
gradient.removeAllAnimations()
setup()
animateGradient()
}
fileprivate func setup() {
gradient.frame = bounds
gradient.colors = currentGradientSet()
gradient.startPoint = startPoint
gradient.endPoint = endPoint
gradient.drawsAsynchronously = true
layer.insertSublayer(gradient, at: 0)
}
fileprivate func currentGradientSet() -> [CGColor] {
guard colors.count > 0 else { return }
return [colors[currentGradient % colors.count].cgColor,
colors[(currentGradient + 1) % colors.count].cgColor]
}
public func setColors(_ colors: [UIColor]) {
guard colors.count > 0 else { return }
self.colors = colors
}
public func setPastelGradient(_ gradient: PastelGradient) {
setColors(gradient.colors())
}
public func addcolor(_ color: UIColor) {
self.colors.append(color)
}
func animateGradient() {
currentGradient += 1
let animation = CABasicAnimation(keyPath: Animation.keyPath)
animation.duration = animationDuration
animation.toValue = currentGradientSet()
animation.fillMode = kCAFillModeForwards
animation.isRemovedOnCompletion = false
animation.delegate = self
animation.autoreverses = true
animation.repeatCount = Float.infinity
gradient.add(animation, forKey: Animation.key)
}
open override func removeFromSuperview() {
super.removeFromSuperview()
gradient.removeAllAnimations()
gradient.removeFromSuperlayer()
extension PastelView: CAAnimationDelegate {
public func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
if flag {
gradient.colors = currentGradientSet()
animateGradient()
}
}
and is shown in view controller like
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
//Moving Gradient View
let pastelView = PastelView(frame: view.bounds)
//MARK: - Custom Direction
pastelView.startPastelPoint = .bottomRight
pastelView.endPastelPoint = .topLeft
//MARK: - Custom Duration
pastelView.animationDuration = 1.60
//MARK: - Custom Color
pastelView.setColors([UIColor(red: 255/255, green: 30/255, blue: 140/255, alpha: 1.0),
UIColor(red: 255/255, green: 48/255, blue: 129/255, alpha: 1.0),
UIColor(red: 254/255, green: 66/255, blue: 121/255, alpha: 1.0),
UIColor(red: 253/255, green: 91/255, blue: 114/255, alpha: 1.0),
UIColor(red: 253/255, green: 105/255, blue: 109/255, alpha: 1.0),
])
pastelView.startAnimation()
view.insertSubview(pastelView, at: 0)
}
swift xcode gradient caanimation cagradientlayer
I'm using a pod from github called Pastel
https://github.com/cruisediary/Pastel
It allows for an easy gradient color animation for background views. My problem is the animated gradient stops when moving to another UIView and going back to the original. Any idea on how to start and stop this animation while going to other tabs or views?
This is the main part of pod file `
import UIKit
open class PastelView: UIView {
private struct Animation {
static let keyPath = "colors"
static let key = "ColorChange"
}
//MARK: - Custom Direction
open var startPoint: CGPoint = PastelPoint.topRight.point
open var endPoint: CGPoint = PastelPoint.bottomLeft.point
open var startPastelPoint = PastelPoint.topRight {
didSet {
startPoint = startPastelPoint.point
}
}
open var endPastelPoint = PastelPoint.bottomLeft {
didSet {
endPoint = endPastelPoint.point
}
}
//MARK: - Custom Duration
open var animationDuration: TimeInterval = 5.0
fileprivate let gradient = CAGradientLayer()
private var currentGradient: Int = 0
private var colors: [UIColor] = [UIColor(red: 156/255, green: 39/255, blue: 176/255, alpha: 1.0),
UIColor(red: 255/255, green: 64/255, blue: 129/255, alpha: 1.0),
UIColor(red: 123/255, green: 31/255, blue: 162/255, alpha: 1.0),
UIColor(red: 32/255, green: 76/255, blue: 255/255, alpha: 1.0),
UIColor(red: 32/255, green: 158/255, blue: 255/255, alpha: 1.0),
UIColor(red: 90/255, green: 120/255, blue: 127/255, alpha: 1.0),
UIColor(red: 58/255, green: 255/255, blue: 217/255, alpha: 1.0)]
public override init(frame: CGRect) {
super.init(frame: frame)
}
public required init?(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
}
open override func awakeFromNib() {
super.awakeFromNib()
}
open override func layoutSubviews() {
super.layoutSubviews()
gradient.frame = bounds
}
public func startAnimation() {
gradient.removeAllAnimations()
setup()
animateGradient()
}
fileprivate func setup() {
gradient.frame = bounds
gradient.colors = currentGradientSet()
gradient.startPoint = startPoint
gradient.endPoint = endPoint
gradient.drawsAsynchronously = true
layer.insertSublayer(gradient, at: 0)
}
fileprivate func currentGradientSet() -> [CGColor] {
guard colors.count > 0 else { return }
return [colors[currentGradient % colors.count].cgColor,
colors[(currentGradient + 1) % colors.count].cgColor]
}
public func setColors(_ colors: [UIColor]) {
guard colors.count > 0 else { return }
self.colors = colors
}
public func setPastelGradient(_ gradient: PastelGradient) {
setColors(gradient.colors())
}
public func addcolor(_ color: UIColor) {
self.colors.append(color)
}
func animateGradient() {
currentGradient += 1
let animation = CABasicAnimation(keyPath: Animation.keyPath)
animation.duration = animationDuration
animation.toValue = currentGradientSet()
animation.fillMode = kCAFillModeForwards
animation.isRemovedOnCompletion = false
animation.delegate = self
animation.autoreverses = true
animation.repeatCount = Float.infinity
gradient.add(animation, forKey: Animation.key)
}
open override func removeFromSuperview() {
super.removeFromSuperview()
gradient.removeAllAnimations()
gradient.removeFromSuperlayer()
extension PastelView: CAAnimationDelegate {
public func animationDidStop(_ anim: CAAnimation, finished flag: Bool) {
if flag {
gradient.colors = currentGradientSet()
animateGradient()
}
}
and is shown in view controller like
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
//Moving Gradient View
let pastelView = PastelView(frame: view.bounds)
//MARK: - Custom Direction
pastelView.startPastelPoint = .bottomRight
pastelView.endPastelPoint = .topLeft
//MARK: - Custom Duration
pastelView.animationDuration = 1.60
//MARK: - Custom Color
pastelView.setColors([UIColor(red: 255/255, green: 30/255, blue: 140/255, alpha: 1.0),
UIColor(red: 255/255, green: 48/255, blue: 129/255, alpha: 1.0),
UIColor(red: 254/255, green: 66/255, blue: 121/255, alpha: 1.0),
UIColor(red: 253/255, green: 91/255, blue: 114/255, alpha: 1.0),
UIColor(red: 253/255, green: 105/255, blue: 109/255, alpha: 1.0),
])
pastelView.startAnimation()
view.insertSubview(pastelView, at: 0)
}
swift xcode gradient caanimation cagradientlayer
swift xcode gradient caanimation cagradientlayer
asked Nov 20 '18 at 7:43


Robert HarrisonRobert Harrison
154
154
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53388323%2fhow-to-stop-start-pastel-gradient-animation-between-views%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53388323%2fhow-to-stop-start-pastel-gradient-animation-between-views%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vODf8fwQBNGb,ry fERYWC9jbK2EE U PGZC,ftS4th05v0410 KP39,E7 OTD3SlJk1mR43IgzEyp5Ov0BpJdG5ZQJgnhUCjLdalJJMEC