react router link with material ui button submit
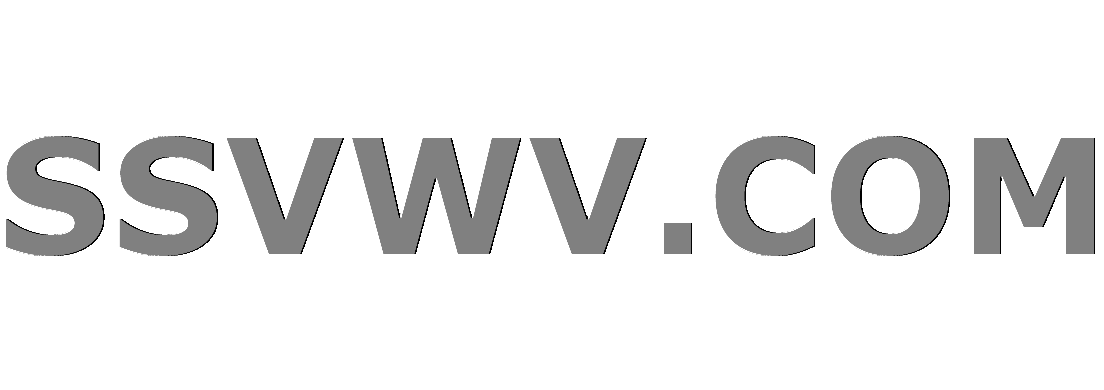
Multi tool use
up vote
0
down vote
favorite
I am using flask on the backend and react, react router and material-ui as a frontend.
I have:
- material-ui/core 3.4.0,
- react router dom 4.3.1
I am trying to create simple sign up form.
I would like to submit the form's data and then redirect to the index page using react router.
My problem is that material-ui button does not work with the link from router.
Is it possible to combine this two together somehow?
My code:
import:
import React, { Component } from 'react'
import { Link } from 'react-router-dom'
import { withStyles, Grid, Paper, Avatar, Typography, Button, TextField} from '@material-ui/core'
import LockIcon from '@material-ui/icons/LockOutlined'
and render return:
render() {
const { classes } = this.props
const { user: { username, password } } = this.state
return (
<Grid container className={classes.root}>
<Paper className={classes.paper}>
<Avatar className={classes.avatar}>
<LockIcon />
</Avatar>
<Typography component="h1" variant="h5">
Sign up
</Typography>
<form onSubmit={this.saveUser}>
<TextField label="Username" value={username} onChange={this.handleChange('username')} margin="normal" required fullWidth/>
<TextField label="Password" value={password} onChange={this.handleChange('password')} margin="normal" required fullWidth type='password'/>
<Button component={Link} to="/" type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
</form>
</Paper>
</Grid>
);
}
When I remove from Button component={Link} to="/":
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
then submit work and saves data in database. When I remove type="submit":
<Button component={Link} to="/" fullWidth variant="contained" color="primary">Sign Up</Button>
then redirect to index page.
Can I combine somehow this two in one button?
RaisedButton, FlatButton from material-ui not working anymore
reactjs react-router material-ui
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
I am using flask on the backend and react, react router and material-ui as a frontend.
I have:
- material-ui/core 3.4.0,
- react router dom 4.3.1
I am trying to create simple sign up form.
I would like to submit the form's data and then redirect to the index page using react router.
My problem is that material-ui button does not work with the link from router.
Is it possible to combine this two together somehow?
My code:
import:
import React, { Component } from 'react'
import { Link } from 'react-router-dom'
import { withStyles, Grid, Paper, Avatar, Typography, Button, TextField} from '@material-ui/core'
import LockIcon from '@material-ui/icons/LockOutlined'
and render return:
render() {
const { classes } = this.props
const { user: { username, password } } = this.state
return (
<Grid container className={classes.root}>
<Paper className={classes.paper}>
<Avatar className={classes.avatar}>
<LockIcon />
</Avatar>
<Typography component="h1" variant="h5">
Sign up
</Typography>
<form onSubmit={this.saveUser}>
<TextField label="Username" value={username} onChange={this.handleChange('username')} margin="normal" required fullWidth/>
<TextField label="Password" value={password} onChange={this.handleChange('password')} margin="normal" required fullWidth type='password'/>
<Button component={Link} to="/" type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
</form>
</Paper>
</Grid>
);
}
When I remove from Button component={Link} to="/":
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
then submit work and saves data in database. When I remove type="submit":
<Button component={Link} to="/" fullWidth variant="contained" color="primary">Sign Up</Button>
then redirect to index page.
Can I combine somehow this two in one button?
RaisedButton, FlatButton from material-ui not working anymore
reactjs react-router material-ui
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am using flask on the backend and react, react router and material-ui as a frontend.
I have:
- material-ui/core 3.4.0,
- react router dom 4.3.1
I am trying to create simple sign up form.
I would like to submit the form's data and then redirect to the index page using react router.
My problem is that material-ui button does not work with the link from router.
Is it possible to combine this two together somehow?
My code:
import:
import React, { Component } from 'react'
import { Link } from 'react-router-dom'
import { withStyles, Grid, Paper, Avatar, Typography, Button, TextField} from '@material-ui/core'
import LockIcon from '@material-ui/icons/LockOutlined'
and render return:
render() {
const { classes } = this.props
const { user: { username, password } } = this.state
return (
<Grid container className={classes.root}>
<Paper className={classes.paper}>
<Avatar className={classes.avatar}>
<LockIcon />
</Avatar>
<Typography component="h1" variant="h5">
Sign up
</Typography>
<form onSubmit={this.saveUser}>
<TextField label="Username" value={username} onChange={this.handleChange('username')} margin="normal" required fullWidth/>
<TextField label="Password" value={password} onChange={this.handleChange('password')} margin="normal" required fullWidth type='password'/>
<Button component={Link} to="/" type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
</form>
</Paper>
</Grid>
);
}
When I remove from Button component={Link} to="/":
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
then submit work and saves data in database. When I remove type="submit":
<Button component={Link} to="/" fullWidth variant="contained" color="primary">Sign Up</Button>
then redirect to index page.
Can I combine somehow this two in one button?
RaisedButton, FlatButton from material-ui not working anymore
reactjs react-router material-ui
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I am using flask on the backend and react, react router and material-ui as a frontend.
I have:
- material-ui/core 3.4.0,
- react router dom 4.3.1
I am trying to create simple sign up form.
I would like to submit the form's data and then redirect to the index page using react router.
My problem is that material-ui button does not work with the link from router.
Is it possible to combine this two together somehow?
My code:
import:
import React, { Component } from 'react'
import { Link } from 'react-router-dom'
import { withStyles, Grid, Paper, Avatar, Typography, Button, TextField} from '@material-ui/core'
import LockIcon from '@material-ui/icons/LockOutlined'
and render return:
render() {
const { classes } = this.props
const { user: { username, password } } = this.state
return (
<Grid container className={classes.root}>
<Paper className={classes.paper}>
<Avatar className={classes.avatar}>
<LockIcon />
</Avatar>
<Typography component="h1" variant="h5">
Sign up
</Typography>
<form onSubmit={this.saveUser}>
<TextField label="Username" value={username} onChange={this.handleChange('username')} margin="normal" required fullWidth/>
<TextField label="Password" value={password} onChange={this.handleChange('password')} margin="normal" required fullWidth type='password'/>
<Button component={Link} to="/" type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
</form>
</Paper>
</Grid>
);
}
When I remove from Button component={Link} to="/":
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
then submit work and saves data in database. When I remove type="submit":
<Button component={Link} to="/" fullWidth variant="contained" color="primary">Sign Up</Button>
then redirect to index page.
Can I combine somehow this two in one button?
RaisedButton, FlatButton from material-ui not working anymore
reactjs react-router material-ui
reactjs react-router material-ui
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited 16 hours ago
Roysh
1,10921221
1,10921221
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 17 hours ago


Maniek Solek
31
31
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Maniek Solek is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
Add to state:
doRedirect: false
In render():
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
In submit hanler:
saveUser = () => {
// **********
// Your code to update database
// **********
this.setState({ doRedirect: true });
}
In render():
{ this.state.doRedirect && <Redirect to="/" /> }
And of course:
import { Redirect } from 'react-router-dom'
I'm not sure where put this line: { this.state.doRedirect && <Redirect to="/" /> } in my code? I'm still a little beginner, so I would be grateful if you could help me with this one too? I understand the rest of the parts.
– Maniek Solek
15 hours ago
eg: <Grid container className={classes.root}> { this.state.doRedirect && <Redirect to="/" /> } <Paper className={classes.paper}>
– Boris Traljić
15 hours ago
It works! Thank you for your help, best regards ;)
– Maniek Solek
15 hours ago
Can you accept my answer? Please ;)
– Boris Traljić
15 hours ago
add a comment |
up vote
0
down vote
In your save user function just redirect after saving the user.
you can get history from props
saveUser = event => {
event.preventDefault()
//update database with user
this.props.history.location.push('new-route')
// or you can use window.location
window.location.href = 'new-route'
}
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Add to state:
doRedirect: false
In render():
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
In submit hanler:
saveUser = () => {
// **********
// Your code to update database
// **********
this.setState({ doRedirect: true });
}
In render():
{ this.state.doRedirect && <Redirect to="/" /> }
And of course:
import { Redirect } from 'react-router-dom'
I'm not sure where put this line: { this.state.doRedirect && <Redirect to="/" /> } in my code? I'm still a little beginner, so I would be grateful if you could help me with this one too? I understand the rest of the parts.
– Maniek Solek
15 hours ago
eg: <Grid container className={classes.root}> { this.state.doRedirect && <Redirect to="/" /> } <Paper className={classes.paper}>
– Boris Traljić
15 hours ago
It works! Thank you for your help, best regards ;)
– Maniek Solek
15 hours ago
Can you accept my answer? Please ;)
– Boris Traljić
15 hours ago
add a comment |
up vote
0
down vote
accepted
Add to state:
doRedirect: false
In render():
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
In submit hanler:
saveUser = () => {
// **********
// Your code to update database
// **********
this.setState({ doRedirect: true });
}
In render():
{ this.state.doRedirect && <Redirect to="/" /> }
And of course:
import { Redirect } from 'react-router-dom'
I'm not sure where put this line: { this.state.doRedirect && <Redirect to="/" /> } in my code? I'm still a little beginner, so I would be grateful if you could help me with this one too? I understand the rest of the parts.
– Maniek Solek
15 hours ago
eg: <Grid container className={classes.root}> { this.state.doRedirect && <Redirect to="/" /> } <Paper className={classes.paper}>
– Boris Traljić
15 hours ago
It works! Thank you for your help, best regards ;)
– Maniek Solek
15 hours ago
Can you accept my answer? Please ;)
– Boris Traljić
15 hours ago
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Add to state:
doRedirect: false
In render():
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
In submit hanler:
saveUser = () => {
// **********
// Your code to update database
// **********
this.setState({ doRedirect: true });
}
In render():
{ this.state.doRedirect && <Redirect to="/" /> }
And of course:
import { Redirect } from 'react-router-dom'
Add to state:
doRedirect: false
In render():
<Button type="submit" fullWidth variant="contained" color="primary">Sign Up</Button>
In submit hanler:
saveUser = () => {
// **********
// Your code to update database
// **********
this.setState({ doRedirect: true });
}
In render():
{ this.state.doRedirect && <Redirect to="/" /> }
And of course:
import { Redirect } from 'react-router-dom'
answered 16 hours ago
Boris Traljić
1126
1126
I'm not sure where put this line: { this.state.doRedirect && <Redirect to="/" /> } in my code? I'm still a little beginner, so I would be grateful if you could help me with this one too? I understand the rest of the parts.
– Maniek Solek
15 hours ago
eg: <Grid container className={classes.root}> { this.state.doRedirect && <Redirect to="/" /> } <Paper className={classes.paper}>
– Boris Traljić
15 hours ago
It works! Thank you for your help, best regards ;)
– Maniek Solek
15 hours ago
Can you accept my answer? Please ;)
– Boris Traljić
15 hours ago
add a comment |
I'm not sure where put this line: { this.state.doRedirect && <Redirect to="/" /> } in my code? I'm still a little beginner, so I would be grateful if you could help me with this one too? I understand the rest of the parts.
– Maniek Solek
15 hours ago
eg: <Grid container className={classes.root}> { this.state.doRedirect && <Redirect to="/" /> } <Paper className={classes.paper}>
– Boris Traljić
15 hours ago
It works! Thank you for your help, best regards ;)
– Maniek Solek
15 hours ago
Can you accept my answer? Please ;)
– Boris Traljić
15 hours ago
I'm not sure where put this line: { this.state.doRedirect && <Redirect to="/" /> } in my code? I'm still a little beginner, so I would be grateful if you could help me with this one too? I understand the rest of the parts.
– Maniek Solek
15 hours ago
I'm not sure where put this line: { this.state.doRedirect && <Redirect to="/" /> } in my code? I'm still a little beginner, so I would be grateful if you could help me with this one too? I understand the rest of the parts.
– Maniek Solek
15 hours ago
eg: <Grid container className={classes.root}> { this.state.doRedirect && <Redirect to="/" /> } <Paper className={classes.paper}>
– Boris Traljić
15 hours ago
eg: <Grid container className={classes.root}> { this.state.doRedirect && <Redirect to="/" /> } <Paper className={classes.paper}>
– Boris Traljić
15 hours ago
It works! Thank you for your help, best regards ;)
– Maniek Solek
15 hours ago
It works! Thank you for your help, best regards ;)
– Maniek Solek
15 hours ago
Can you accept my answer? Please ;)
– Boris Traljić
15 hours ago
Can you accept my answer? Please ;)
– Boris Traljić
15 hours ago
add a comment |
up vote
0
down vote
In your save user function just redirect after saving the user.
you can get history from props
saveUser = event => {
event.preventDefault()
//update database with user
this.props.history.location.push('new-route')
// or you can use window.location
window.location.href = 'new-route'
}
add a comment |
up vote
0
down vote
In your save user function just redirect after saving the user.
you can get history from props
saveUser = event => {
event.preventDefault()
//update database with user
this.props.history.location.push('new-route')
// or you can use window.location
window.location.href = 'new-route'
}
add a comment |
up vote
0
down vote
up vote
0
down vote
In your save user function just redirect after saving the user.
you can get history from props
saveUser = event => {
event.preventDefault()
//update database with user
this.props.history.location.push('new-route')
// or you can use window.location
window.location.href = 'new-route'
}
In your save user function just redirect after saving the user.
you can get history from props
saveUser = event => {
event.preventDefault()
//update database with user
this.props.history.location.push('new-route')
// or you can use window.location
window.location.href = 'new-route'
}
answered 14 hours ago


Gavyn Caldwell
305
305
add a comment |
add a comment |
Maniek Solek is a new contributor. Be nice, and check out our Code of Conduct.
Maniek Solek is a new contributor. Be nice, and check out our Code of Conduct.
Maniek Solek is a new contributor. Be nice, and check out our Code of Conduct.
Maniek Solek is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53265186%2freact-router-link-with-material-ui-button-submit%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
1EPtHnr379wJwhzlkx,CGAammqNq5SiE2,8jH qV5 mSN4S7hvR6ZZy3nTg,NWw,AR