Stepping through controls in a wrappanel
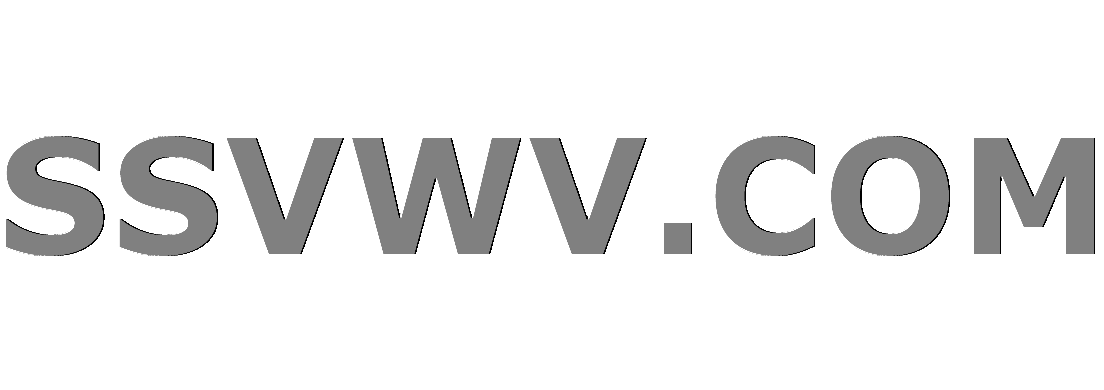
Multi tool use
up vote
0
down vote
favorite
I have a bunch of controls located in a wrappanel in a WPF app that are procedurally created. The first control is a label followed by a bunch of comboboxes and a checkbox. The user clicks a button and a new row of controls are added. This worked fine. Then I decided to make the label a bit more attractive by giving it a red circle as a background and the label was nested in a grid with the red circel behind the label that simply listed the row number. This worked fine. And I use to step through all the controls with this block:
foreach (Control item in WrapPanelItems.Children)
{
if (item.GetType() == typeof(CheckBox))
{
RowCounter++;
}
}
now suddenly this block of code fails with this error: 'Unable to cast object of type 'System.Windows.Controls.Grid' to type 'System.Windows.Controls.Control'.'
So I suspect the grid isnt a conventional item and the code fails. But how would I then iterate through the controls without the app crashing and still iterate through all the conventional controls ontop of it?
Here is the code for how I style and add the label.
Grid MyGrid= new Grid();
Ellipse myEllipse = new Ellipse();
SolidColorBrush mySolidColorBrush = new SolidColorBrush();
mySolidColorBrush.Color = Color.FromArgb(255, 107, 142, 35);
myEllipse.Fill = mySolidColorBrush;
myEllipse.Width = 20;
myEllipse.Height = 20;
MyGrid.Children.Add(myEllipse);
Label LabelCounter = new Label();
LabelCounter.Content = RowCount.ToString();
MyGrid.Children.Add(LabelCounter);
LabelCounter.VerticalAlignment = VerticalAlignment.Center;
LabelCounter.HorizontalAlignment = HorizontalAlignment.Center;
WrapPanelItems.Children.Add(MyGrid);
And also a second question. Suppose I want to change the text on the label... how would I get to the label if its nested in a grid? Can you just change the content directly when the FOR loop picks up the grid? Or should you then say all children of grid when the grid is identified in the FOR loop?
tx
c# wpf
add a comment |
up vote
0
down vote
favorite
I have a bunch of controls located in a wrappanel in a WPF app that are procedurally created. The first control is a label followed by a bunch of comboboxes and a checkbox. The user clicks a button and a new row of controls are added. This worked fine. Then I decided to make the label a bit more attractive by giving it a red circle as a background and the label was nested in a grid with the red circel behind the label that simply listed the row number. This worked fine. And I use to step through all the controls with this block:
foreach (Control item in WrapPanelItems.Children)
{
if (item.GetType() == typeof(CheckBox))
{
RowCounter++;
}
}
now suddenly this block of code fails with this error: 'Unable to cast object of type 'System.Windows.Controls.Grid' to type 'System.Windows.Controls.Control'.'
So I suspect the grid isnt a conventional item and the code fails. But how would I then iterate through the controls without the app crashing and still iterate through all the conventional controls ontop of it?
Here is the code for how I style and add the label.
Grid MyGrid= new Grid();
Ellipse myEllipse = new Ellipse();
SolidColorBrush mySolidColorBrush = new SolidColorBrush();
mySolidColorBrush.Color = Color.FromArgb(255, 107, 142, 35);
myEllipse.Fill = mySolidColorBrush;
myEllipse.Width = 20;
myEllipse.Height = 20;
MyGrid.Children.Add(myEllipse);
Label LabelCounter = new Label();
LabelCounter.Content = RowCount.ToString();
MyGrid.Children.Add(LabelCounter);
LabelCounter.VerticalAlignment = VerticalAlignment.Center;
LabelCounter.HorizontalAlignment = HorizontalAlignment.Center;
WrapPanelItems.Children.Add(MyGrid);
And also a second question. Suppose I want to change the text on the label... how would I get to the label if its nested in a grid? Can you just change the content directly when the FOR loop picks up the grid? Or should you then say all children of grid when the grid is identified in the FOR loop?
tx
c# wpf
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a bunch of controls located in a wrappanel in a WPF app that are procedurally created. The first control is a label followed by a bunch of comboboxes and a checkbox. The user clicks a button and a new row of controls are added. This worked fine. Then I decided to make the label a bit more attractive by giving it a red circle as a background and the label was nested in a grid with the red circel behind the label that simply listed the row number. This worked fine. And I use to step through all the controls with this block:
foreach (Control item in WrapPanelItems.Children)
{
if (item.GetType() == typeof(CheckBox))
{
RowCounter++;
}
}
now suddenly this block of code fails with this error: 'Unable to cast object of type 'System.Windows.Controls.Grid' to type 'System.Windows.Controls.Control'.'
So I suspect the grid isnt a conventional item and the code fails. But how would I then iterate through the controls without the app crashing and still iterate through all the conventional controls ontop of it?
Here is the code for how I style and add the label.
Grid MyGrid= new Grid();
Ellipse myEllipse = new Ellipse();
SolidColorBrush mySolidColorBrush = new SolidColorBrush();
mySolidColorBrush.Color = Color.FromArgb(255, 107, 142, 35);
myEllipse.Fill = mySolidColorBrush;
myEllipse.Width = 20;
myEllipse.Height = 20;
MyGrid.Children.Add(myEllipse);
Label LabelCounter = new Label();
LabelCounter.Content = RowCount.ToString();
MyGrid.Children.Add(LabelCounter);
LabelCounter.VerticalAlignment = VerticalAlignment.Center;
LabelCounter.HorizontalAlignment = HorizontalAlignment.Center;
WrapPanelItems.Children.Add(MyGrid);
And also a second question. Suppose I want to change the text on the label... how would I get to the label if its nested in a grid? Can you just change the content directly when the FOR loop picks up the grid? Or should you then say all children of grid when the grid is identified in the FOR loop?
tx
c# wpf
I have a bunch of controls located in a wrappanel in a WPF app that are procedurally created. The first control is a label followed by a bunch of comboboxes and a checkbox. The user clicks a button and a new row of controls are added. This worked fine. Then I decided to make the label a bit more attractive by giving it a red circle as a background and the label was nested in a grid with the red circel behind the label that simply listed the row number. This worked fine. And I use to step through all the controls with this block:
foreach (Control item in WrapPanelItems.Children)
{
if (item.GetType() == typeof(CheckBox))
{
RowCounter++;
}
}
now suddenly this block of code fails with this error: 'Unable to cast object of type 'System.Windows.Controls.Grid' to type 'System.Windows.Controls.Control'.'
So I suspect the grid isnt a conventional item and the code fails. But how would I then iterate through the controls without the app crashing and still iterate through all the conventional controls ontop of it?
Here is the code for how I style and add the label.
Grid MyGrid= new Grid();
Ellipse myEllipse = new Ellipse();
SolidColorBrush mySolidColorBrush = new SolidColorBrush();
mySolidColorBrush.Color = Color.FromArgb(255, 107, 142, 35);
myEllipse.Fill = mySolidColorBrush;
myEllipse.Width = 20;
myEllipse.Height = 20;
MyGrid.Children.Add(myEllipse);
Label LabelCounter = new Label();
LabelCounter.Content = RowCount.ToString();
MyGrid.Children.Add(LabelCounter);
LabelCounter.VerticalAlignment = VerticalAlignment.Center;
LabelCounter.HorizontalAlignment = HorizontalAlignment.Center;
WrapPanelItems.Children.Add(MyGrid);
And also a second question. Suppose I want to change the text on the label... how would I get to the label if its nested in a grid? Can you just change the content directly when the FOR loop picks up the grid? Or should you then say all children of grid when the grid is identified in the FOR loop?
tx
c# wpf
c# wpf
asked Nov 15 at 18:26


Breytenzest
113
113
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Grid
is not a System.Windows.Controls.Control
so this line throws an exception:
foreach (Control item in WrapPanelItems.Children)
You can replace Control
with UIElement
to avoid this error.
According to MSDN:
public class Grid : System.Windows.Controls.Panel, System.Windows.Markup.IAddChild
thank you it worked like a charm!
– Breytenzest
Nov 15 at 21:49
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325752%2fstepping-through-controls-in-a-wrappanel%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Grid
is not a System.Windows.Controls.Control
so this line throws an exception:
foreach (Control item in WrapPanelItems.Children)
You can replace Control
with UIElement
to avoid this error.
According to MSDN:
public class Grid : System.Windows.Controls.Panel, System.Windows.Markup.IAddChild
thank you it worked like a charm!
– Breytenzest
Nov 15 at 21:49
add a comment |
up vote
0
down vote
accepted
Grid
is not a System.Windows.Controls.Control
so this line throws an exception:
foreach (Control item in WrapPanelItems.Children)
You can replace Control
with UIElement
to avoid this error.
According to MSDN:
public class Grid : System.Windows.Controls.Panel, System.Windows.Markup.IAddChild
thank you it worked like a charm!
– Breytenzest
Nov 15 at 21:49
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Grid
is not a System.Windows.Controls.Control
so this line throws an exception:
foreach (Control item in WrapPanelItems.Children)
You can replace Control
with UIElement
to avoid this error.
According to MSDN:
public class Grid : System.Windows.Controls.Panel, System.Windows.Markup.IAddChild
Grid
is not a System.Windows.Controls.Control
so this line throws an exception:
foreach (Control item in WrapPanelItems.Children)
You can replace Control
with UIElement
to avoid this error.
According to MSDN:
public class Grid : System.Windows.Controls.Panel, System.Windows.Markup.IAddChild
answered Nov 15 at 18:35


Bizhan
7,74163255
7,74163255
thank you it worked like a charm!
– Breytenzest
Nov 15 at 21:49
add a comment |
thank you it worked like a charm!
– Breytenzest
Nov 15 at 21:49
thank you it worked like a charm!
– Breytenzest
Nov 15 at 21:49
thank you it worked like a charm!
– Breytenzest
Nov 15 at 21:49
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53325752%2fstepping-through-controls-in-a-wrappanel%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7y41c2hMB32Z1e2A2kzVZ ZmJkmzQUaFq04AF KMWKwg7uSiPke9,h927Z4,9pP94uDi,90bjPYC7zZh,uhiuS,GlBROrSAfb