SQL Server: quick and dirty data validation query?
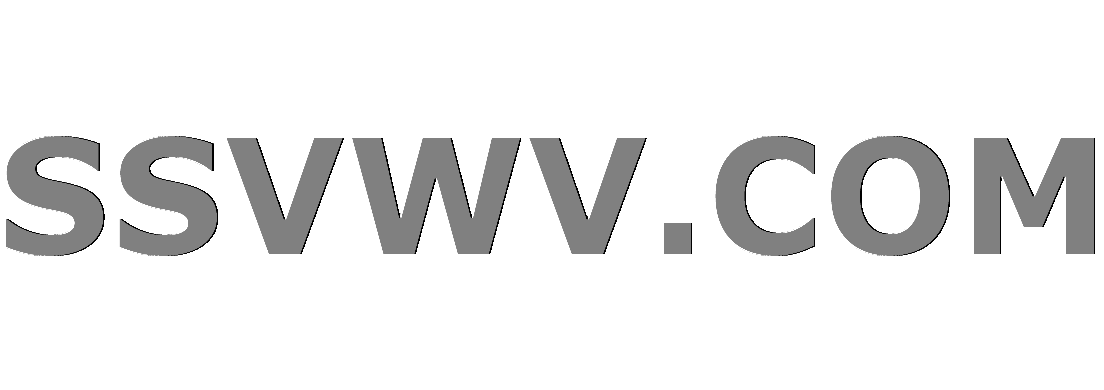
Multi tool use
up vote
2
down vote
favorite
Getting frequent updates on a database that is going through major changes. Need a quick way to check for abnormalities in the data. Over 1,000 tables; about 200
actually being used.
Would like to have a list of the tables with the most records at the top. Found and modified this code that seems to work:
select
object_name(object_id) AS TableName,
sum(rows) AS TableRowCount
from
sys.partitions
where
index_id in (0, 1)
group by
object_id
order by
TableRowCount desc
However, it would be useful to also have an indication if columns are populated properly. Would be great to have percentages of valid rows as well as percentages of NULLs, blanks and zeros.
Stumbled across this snippet:
SELECT
TABLE_NAME,
COLUMN_NAME,
dbo.ExecuteScalarToInt('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
However, this throws an error:
Cannot find either column "dbo" or the user-defined function or aggregate "dbo.ExecuteScalarToInt", or the name is ambiguous.
Hmmm. I deleted the dbo.ExecuteScalarToInt
part:
SELECT
TABLE_NAME,
COLUMN_NAME,
('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) AS Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
ORDER BY
TABLE_NAME ASC, COLUMN_NAME ASC
This works, but for the percentage column, a SQL query shows up. Tried the resulting SQL query--it works.
Tried substituting "exec", "sp_executesql", and "exec sp_executesql".
Got errors.
Here is what I'm imagining what the output should look similar to:
TableName TableRows ColumnName PctPopulatedRows PctNULLs PctBlanks PctZeros
LineItems 4,649,764 InventryNum 100.0% 0.0% 0.0% 0.0%
LineItems 4,649,764 Description 99.9% 0.0% 0.1% 0.0%
LineItems 4,649,764 Price 99.7% 0.0% 0.1% 0.2%
.
.
.
Invoices 385,532 InvoiceNum 100.0% 0.0% 0.0% 0.0%
Invoices 385,532 DateTime 99.6% 0.2% 0.1% 0.1%
Invoices 385,532 TotalAmt 99.8% 0.0% 0.2% 0.0%
.
.
.
Customers 64,906 CustomerNum 100.0% 0.0% 0.0% 0.0%
Customers 64,906 CustStreet 99.8% 0.0% 0.2% 0.0%
Customers 64,906 CustZip 99.7% 0.1% 0.2% 0.0%
Tired of banging my head against this. Can anyone straighten me out to get closer to a solution?
Many thanks for taking a look.
sql

add a comment |
up vote
2
down vote
favorite
Getting frequent updates on a database that is going through major changes. Need a quick way to check for abnormalities in the data. Over 1,000 tables; about 200
actually being used.
Would like to have a list of the tables with the most records at the top. Found and modified this code that seems to work:
select
object_name(object_id) AS TableName,
sum(rows) AS TableRowCount
from
sys.partitions
where
index_id in (0, 1)
group by
object_id
order by
TableRowCount desc
However, it would be useful to also have an indication if columns are populated properly. Would be great to have percentages of valid rows as well as percentages of NULLs, blanks and zeros.
Stumbled across this snippet:
SELECT
TABLE_NAME,
COLUMN_NAME,
dbo.ExecuteScalarToInt('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
However, this throws an error:
Cannot find either column "dbo" or the user-defined function or aggregate "dbo.ExecuteScalarToInt", or the name is ambiguous.
Hmmm. I deleted the dbo.ExecuteScalarToInt
part:
SELECT
TABLE_NAME,
COLUMN_NAME,
('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) AS Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
ORDER BY
TABLE_NAME ASC, COLUMN_NAME ASC
This works, but for the percentage column, a SQL query shows up. Tried the resulting SQL query--it works.
Tried substituting "exec", "sp_executesql", and "exec sp_executesql".
Got errors.
Here is what I'm imagining what the output should look similar to:
TableName TableRows ColumnName PctPopulatedRows PctNULLs PctBlanks PctZeros
LineItems 4,649,764 InventryNum 100.0% 0.0% 0.0% 0.0%
LineItems 4,649,764 Description 99.9% 0.0% 0.1% 0.0%
LineItems 4,649,764 Price 99.7% 0.0% 0.1% 0.2%
.
.
.
Invoices 385,532 InvoiceNum 100.0% 0.0% 0.0% 0.0%
Invoices 385,532 DateTime 99.6% 0.2% 0.1% 0.1%
Invoices 385,532 TotalAmt 99.8% 0.0% 0.2% 0.0%
.
.
.
Customers 64,906 CustomerNum 100.0% 0.0% 0.0% 0.0%
Customers 64,906 CustStreet 99.8% 0.0% 0.2% 0.0%
Customers 64,906 CustZip 99.7% 0.1% 0.2% 0.0%
Tired of banging my head against this. Can anyone straighten me out to get closer to a solution?
Many thanks for taking a look.
sql

ExecuteScalarToInt
is a custom scalar user defined function. Wherever you lifted that from might have the definition for it. You'd need to create it in the same database. If a NULL or blank is legitimately an invalid piece of data then you should have constraints stopping that. Of course in the real world that can be difficult.
– Nick.McDermaid
Nov 13 at 3:47
What you required can be done but not in one single query but utilize dynamic sql + temp table. Looks like it will be a complex query (the dynamic part)
– Squirrel
Nov 13 at 4:12
You basically need to use a cursor to populate some dynamic SQL... like this stackoverflow.com/questions/25559088/…
– Nick.McDermaid
Nov 13 at 11:08
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
Getting frequent updates on a database that is going through major changes. Need a quick way to check for abnormalities in the data. Over 1,000 tables; about 200
actually being used.
Would like to have a list of the tables with the most records at the top. Found and modified this code that seems to work:
select
object_name(object_id) AS TableName,
sum(rows) AS TableRowCount
from
sys.partitions
where
index_id in (0, 1)
group by
object_id
order by
TableRowCount desc
However, it would be useful to also have an indication if columns are populated properly. Would be great to have percentages of valid rows as well as percentages of NULLs, blanks and zeros.
Stumbled across this snippet:
SELECT
TABLE_NAME,
COLUMN_NAME,
dbo.ExecuteScalarToInt('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
However, this throws an error:
Cannot find either column "dbo" or the user-defined function or aggregate "dbo.ExecuteScalarToInt", or the name is ambiguous.
Hmmm. I deleted the dbo.ExecuteScalarToInt
part:
SELECT
TABLE_NAME,
COLUMN_NAME,
('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) AS Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
ORDER BY
TABLE_NAME ASC, COLUMN_NAME ASC
This works, but for the percentage column, a SQL query shows up. Tried the resulting SQL query--it works.
Tried substituting "exec", "sp_executesql", and "exec sp_executesql".
Got errors.
Here is what I'm imagining what the output should look similar to:
TableName TableRows ColumnName PctPopulatedRows PctNULLs PctBlanks PctZeros
LineItems 4,649,764 InventryNum 100.0% 0.0% 0.0% 0.0%
LineItems 4,649,764 Description 99.9% 0.0% 0.1% 0.0%
LineItems 4,649,764 Price 99.7% 0.0% 0.1% 0.2%
.
.
.
Invoices 385,532 InvoiceNum 100.0% 0.0% 0.0% 0.0%
Invoices 385,532 DateTime 99.6% 0.2% 0.1% 0.1%
Invoices 385,532 TotalAmt 99.8% 0.0% 0.2% 0.0%
.
.
.
Customers 64,906 CustomerNum 100.0% 0.0% 0.0% 0.0%
Customers 64,906 CustStreet 99.8% 0.0% 0.2% 0.0%
Customers 64,906 CustZip 99.7% 0.1% 0.2% 0.0%
Tired of banging my head against this. Can anyone straighten me out to get closer to a solution?
Many thanks for taking a look.
sql

Getting frequent updates on a database that is going through major changes. Need a quick way to check for abnormalities in the data. Over 1,000 tables; about 200
actually being used.
Would like to have a list of the tables with the most records at the top. Found and modified this code that seems to work:
select
object_name(object_id) AS TableName,
sum(rows) AS TableRowCount
from
sys.partitions
where
index_id in (0, 1)
group by
object_id
order by
TableRowCount desc
However, it would be useful to also have an indication if columns are populated properly. Would be great to have percentages of valid rows as well as percentages of NULLs, blanks and zeros.
Stumbled across this snippet:
SELECT
TABLE_NAME,
COLUMN_NAME,
dbo.ExecuteScalarToInt('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
However, this throws an error:
Cannot find either column "dbo" or the user-defined function or aggregate "dbo.ExecuteScalarToInt", or the name is ambiguous.
Hmmm. I deleted the dbo.ExecuteScalarToInt
part:
SELECT
TABLE_NAME,
COLUMN_NAME,
('SELECT ISNULL(SUM(CASE WHEN ' + QUOTENAME(COLUMN_NAME) + ' IS NULL THEN 1 ELSE 0 END) * 100.00/count(*), 0) Percentage FROM ' + QUOTENAME(TABLE_SCHEMA) + '.' + QUOTENAME(TABLE_NAME)) AS Percentage
FROM
INFORMATION_SCHEMA.COLUMNS
ORDER BY
TABLE_NAME ASC, COLUMN_NAME ASC
This works, but for the percentage column, a SQL query shows up. Tried the resulting SQL query--it works.
Tried substituting "exec", "sp_executesql", and "exec sp_executesql".
Got errors.
Here is what I'm imagining what the output should look similar to:
TableName TableRows ColumnName PctPopulatedRows PctNULLs PctBlanks PctZeros
LineItems 4,649,764 InventryNum 100.0% 0.0% 0.0% 0.0%
LineItems 4,649,764 Description 99.9% 0.0% 0.1% 0.0%
LineItems 4,649,764 Price 99.7% 0.0% 0.1% 0.2%
.
.
.
Invoices 385,532 InvoiceNum 100.0% 0.0% 0.0% 0.0%
Invoices 385,532 DateTime 99.6% 0.2% 0.1% 0.1%
Invoices 385,532 TotalAmt 99.8% 0.0% 0.2% 0.0%
.
.
.
Customers 64,906 CustomerNum 100.0% 0.0% 0.0% 0.0%
Customers 64,906 CustStreet 99.8% 0.0% 0.2% 0.0%
Customers 64,906 CustZip 99.7% 0.1% 0.2% 0.0%
Tired of banging my head against this. Can anyone straighten me out to get closer to a solution?
Many thanks for taking a look.
sql

sql

edited Nov 13 at 5:40
marc_s
566k12610921245
566k12610921245
asked Nov 13 at 3:22
SquareDonut
182
182
ExecuteScalarToInt
is a custom scalar user defined function. Wherever you lifted that from might have the definition for it. You'd need to create it in the same database. If a NULL or blank is legitimately an invalid piece of data then you should have constraints stopping that. Of course in the real world that can be difficult.
– Nick.McDermaid
Nov 13 at 3:47
What you required can be done but not in one single query but utilize dynamic sql + temp table. Looks like it will be a complex query (the dynamic part)
– Squirrel
Nov 13 at 4:12
You basically need to use a cursor to populate some dynamic SQL... like this stackoverflow.com/questions/25559088/…
– Nick.McDermaid
Nov 13 at 11:08
add a comment |
ExecuteScalarToInt
is a custom scalar user defined function. Wherever you lifted that from might have the definition for it. You'd need to create it in the same database. If a NULL or blank is legitimately an invalid piece of data then you should have constraints stopping that. Of course in the real world that can be difficult.
– Nick.McDermaid
Nov 13 at 3:47
What you required can be done but not in one single query but utilize dynamic sql + temp table. Looks like it will be a complex query (the dynamic part)
– Squirrel
Nov 13 at 4:12
You basically need to use a cursor to populate some dynamic SQL... like this stackoverflow.com/questions/25559088/…
– Nick.McDermaid
Nov 13 at 11:08
ExecuteScalarToInt
is a custom scalar user defined function. Wherever you lifted that from might have the definition for it. You'd need to create it in the same database. If a NULL or blank is legitimately an invalid piece of data then you should have constraints stopping that. Of course in the real world that can be difficult.– Nick.McDermaid
Nov 13 at 3:47
ExecuteScalarToInt
is a custom scalar user defined function. Wherever you lifted that from might have the definition for it. You'd need to create it in the same database. If a NULL or blank is legitimately an invalid piece of data then you should have constraints stopping that. Of course in the real world that can be difficult.– Nick.McDermaid
Nov 13 at 3:47
What you required can be done but not in one single query but utilize dynamic sql + temp table. Looks like it will be a complex query (the dynamic part)
– Squirrel
Nov 13 at 4:12
What you required can be done but not in one single query but utilize dynamic sql + temp table. Looks like it will be a complex query (the dynamic part)
– Squirrel
Nov 13 at 4:12
You basically need to use a cursor to populate some dynamic SQL... like this stackoverflow.com/questions/25559088/…
– Nick.McDermaid
Nov 13 at 11:08
You basically need to use a cursor to populate some dynamic SQL... like this stackoverflow.com/questions/25559088/…
– Nick.McDermaid
Nov 13 at 11:08
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53273322%2fsql-server-quick-and-dirty-data-validation-query%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NUTI,D IVImXShBgL7B1,tAicE17PWMWc,kO09
ExecuteScalarToInt
is a custom scalar user defined function. Wherever you lifted that from might have the definition for it. You'd need to create it in the same database. If a NULL or blank is legitimately an invalid piece of data then you should have constraints stopping that. Of course in the real world that can be difficult.– Nick.McDermaid
Nov 13 at 3:47
What you required can be done but not in one single query but utilize dynamic sql + temp table. Looks like it will be a complex query (the dynamic part)
– Squirrel
Nov 13 at 4:12
You basically need to use a cursor to populate some dynamic SQL... like this stackoverflow.com/questions/25559088/…
– Nick.McDermaid
Nov 13 at 11:08