Only receives one cookie in fetch response on Android but not on iOS
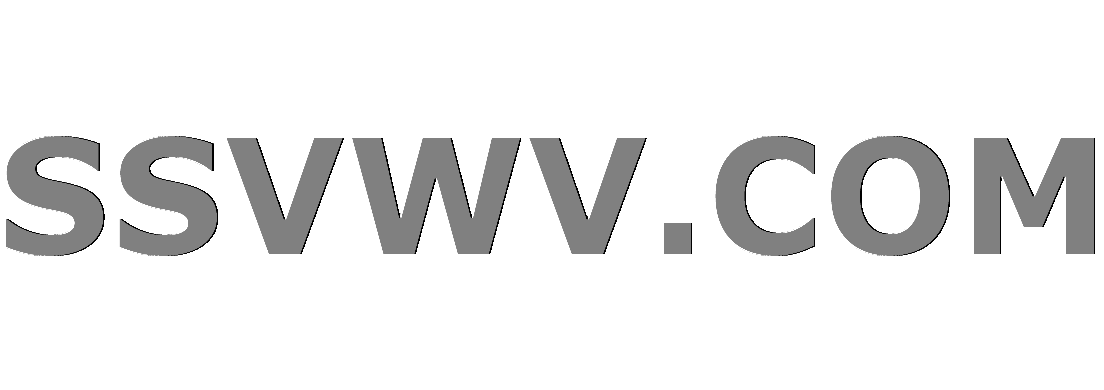
Multi tool use
up vote
3
down vote
favorite
When I send a POST fetch request to my website to login it all works on iOS. The fetch response set-cookie have all the cookies I need to proceed and make future requests. The problem is on Android I only receive one cookie in set-cookie, even though there is more. It seems like it gets cut and I can't seem to access the raw response.
My fetch code:
const myRequest = new Request('MYURL',
{method: 'POST', body: parameters,
headers: {
"Referer": 'MYURL.com/login',
'Origin': 'MYURL',
'Content-Type': 'application/x-www-form-urlencoded'
}});
fetch(myRequest)
.then(
function(response) {
try {
console.log("JSON RESPONSE: " + response.json());
console.log(JSON.stringify(response, null, 2));
console.log(response.headers.get('Content-Type'));
console.log(response.headers.get('Date'));
} catch(err) {
console.log("ERROR: " + err);
}
}
)
.catch(function(err) {
console.log('Fetch Error', err);
});
I know that android gets correctly logged in cause the server redirects me to the menu page and also recognizes the clients personal ID.
Here is the response from iOS(the one that works):
"set-cookie": "session=CENSORED; Domain=.MYURL; Path=/; HttpOnly, clientID=123; expires=Thu, 07-Nov-2019 09:27:20 GMT; domain=.MYURL; path=/",
Perfect response. Everything works.
Here is the response from android:
"set-cookie": "clientID=123; expires=Thu, 07-Nov-2019 09:34:00 GMT; domain=.MYURL; path=/",
I've tried for several days to understand the problem but to no avail. I've tried to test cookies without httponly to see if that was the error but no.
I hope some experienced react-native users can shine light on this problem. I haven't experienced it before when I created native apps for android and IOS. Only with react-native. :-(
I hope to get to this to work since I would rather avoid using native code for this small part.
EDIT: Tested fetch function with reddit login api and same problem occurs. Only 1 cookie in response set-cookio on Android but all cookies shown on iOS.

add a comment |
up vote
3
down vote
favorite
When I send a POST fetch request to my website to login it all works on iOS. The fetch response set-cookie have all the cookies I need to proceed and make future requests. The problem is on Android I only receive one cookie in set-cookie, even though there is more. It seems like it gets cut and I can't seem to access the raw response.
My fetch code:
const myRequest = new Request('MYURL',
{method: 'POST', body: parameters,
headers: {
"Referer": 'MYURL.com/login',
'Origin': 'MYURL',
'Content-Type': 'application/x-www-form-urlencoded'
}});
fetch(myRequest)
.then(
function(response) {
try {
console.log("JSON RESPONSE: " + response.json());
console.log(JSON.stringify(response, null, 2));
console.log(response.headers.get('Content-Type'));
console.log(response.headers.get('Date'));
} catch(err) {
console.log("ERROR: " + err);
}
}
)
.catch(function(err) {
console.log('Fetch Error', err);
});
I know that android gets correctly logged in cause the server redirects me to the menu page and also recognizes the clients personal ID.
Here is the response from iOS(the one that works):
"set-cookie": "session=CENSORED; Domain=.MYURL; Path=/; HttpOnly, clientID=123; expires=Thu, 07-Nov-2019 09:27:20 GMT; domain=.MYURL; path=/",
Perfect response. Everything works.
Here is the response from android:
"set-cookie": "clientID=123; expires=Thu, 07-Nov-2019 09:34:00 GMT; domain=.MYURL; path=/",
I've tried for several days to understand the problem but to no avail. I've tried to test cookies without httponly to see if that was the error but no.
I hope some experienced react-native users can shine light on this problem. I haven't experienced it before when I created native apps for android and IOS. Only with react-native. :-(
I hope to get to this to work since I would rather avoid using native code for this small part.
EDIT: Tested fetch function with reddit login api and same problem occurs. Only 1 cookie in response set-cookio on Android but all cookies shown on iOS.

add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
When I send a POST fetch request to my website to login it all works on iOS. The fetch response set-cookie have all the cookies I need to proceed and make future requests. The problem is on Android I only receive one cookie in set-cookie, even though there is more. It seems like it gets cut and I can't seem to access the raw response.
My fetch code:
const myRequest = new Request('MYURL',
{method: 'POST', body: parameters,
headers: {
"Referer": 'MYURL.com/login',
'Origin': 'MYURL',
'Content-Type': 'application/x-www-form-urlencoded'
}});
fetch(myRequest)
.then(
function(response) {
try {
console.log("JSON RESPONSE: " + response.json());
console.log(JSON.stringify(response, null, 2));
console.log(response.headers.get('Content-Type'));
console.log(response.headers.get('Date'));
} catch(err) {
console.log("ERROR: " + err);
}
}
)
.catch(function(err) {
console.log('Fetch Error', err);
});
I know that android gets correctly logged in cause the server redirects me to the menu page and also recognizes the clients personal ID.
Here is the response from iOS(the one that works):
"set-cookie": "session=CENSORED; Domain=.MYURL; Path=/; HttpOnly, clientID=123; expires=Thu, 07-Nov-2019 09:27:20 GMT; domain=.MYURL; path=/",
Perfect response. Everything works.
Here is the response from android:
"set-cookie": "clientID=123; expires=Thu, 07-Nov-2019 09:34:00 GMT; domain=.MYURL; path=/",
I've tried for several days to understand the problem but to no avail. I've tried to test cookies without httponly to see if that was the error but no.
I hope some experienced react-native users can shine light on this problem. I haven't experienced it before when I created native apps for android and IOS. Only with react-native. :-(
I hope to get to this to work since I would rather avoid using native code for this small part.
EDIT: Tested fetch function with reddit login api and same problem occurs. Only 1 cookie in response set-cookio on Android but all cookies shown on iOS.

When I send a POST fetch request to my website to login it all works on iOS. The fetch response set-cookie have all the cookies I need to proceed and make future requests. The problem is on Android I only receive one cookie in set-cookie, even though there is more. It seems like it gets cut and I can't seem to access the raw response.
My fetch code:
const myRequest = new Request('MYURL',
{method: 'POST', body: parameters,
headers: {
"Referer": 'MYURL.com/login',
'Origin': 'MYURL',
'Content-Type': 'application/x-www-form-urlencoded'
}});
fetch(myRequest)
.then(
function(response) {
try {
console.log("JSON RESPONSE: " + response.json());
console.log(JSON.stringify(response, null, 2));
console.log(response.headers.get('Content-Type'));
console.log(response.headers.get('Date'));
} catch(err) {
console.log("ERROR: " + err);
}
}
)
.catch(function(err) {
console.log('Fetch Error', err);
});
I know that android gets correctly logged in cause the server redirects me to the menu page and also recognizes the clients personal ID.
Here is the response from iOS(the one that works):
"set-cookie": "session=CENSORED; Domain=.MYURL; Path=/; HttpOnly, clientID=123; expires=Thu, 07-Nov-2019 09:27:20 GMT; domain=.MYURL; path=/",
Perfect response. Everything works.
Here is the response from android:
"set-cookie": "clientID=123; expires=Thu, 07-Nov-2019 09:34:00 GMT; domain=.MYURL; path=/",
I've tried for several days to understand the problem but to no avail. I've tried to test cookies without httponly to see if that was the error but no.
I hope some experienced react-native users can shine light on this problem. I haven't experienced it before when I created native apps for android and IOS. Only with react-native. :-(
I hope to get to this to work since I would rather avoid using native code for this small part.
EDIT: Tested fetch function with reddit login api and same problem occurs. Only 1 cookie in response set-cookio on Android but all cookies shown on iOS.


edited Nov 7 at 11:44
asked Nov 7 at 9:39
Anton B
5912
5912
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Look into the official documents of React Native and MDN:
- https://facebook.github.io/react-native/docs/network
- https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch
The problem here is fetch
won't send or receive any cookie from server by default. So you have to enable it by add credentials
. And there are some libraries that can help you manage cookies:
- https://github.com/joeferraro/react-native-cookies
I added 'credentials': 'include' to my fetch request but it changes nothing. I still got the same response on iOS and android. iOS still works fine and android still doesn't work.
– Anton B
Nov 14 at 14:16
1
And how does it explain why I still receive the cookies properly on iOS but not on android? And it's not like I don't receive any. I still receive one cookie but only 1 on android. Thanks for the links, though!
– Anton B
Nov 14 at 14:27
This must be a bug of RN. Which version of RN are you using? I found a report here and it's not fixed: github.com/facebook/react-native/issues/21795
– Louis Solo
Nov 15 at 3:32
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Look into the official documents of React Native and MDN:
- https://facebook.github.io/react-native/docs/network
- https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch
The problem here is fetch
won't send or receive any cookie from server by default. So you have to enable it by add credentials
. And there are some libraries that can help you manage cookies:
- https://github.com/joeferraro/react-native-cookies
I added 'credentials': 'include' to my fetch request but it changes nothing. I still got the same response on iOS and android. iOS still works fine and android still doesn't work.
– Anton B
Nov 14 at 14:16
1
And how does it explain why I still receive the cookies properly on iOS but not on android? And it's not like I don't receive any. I still receive one cookie but only 1 on android. Thanks for the links, though!
– Anton B
Nov 14 at 14:27
This must be a bug of RN. Which version of RN are you using? I found a report here and it's not fixed: github.com/facebook/react-native/issues/21795
– Louis Solo
Nov 15 at 3:32
add a comment |
up vote
0
down vote
Look into the official documents of React Native and MDN:
- https://facebook.github.io/react-native/docs/network
- https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch
The problem here is fetch
won't send or receive any cookie from server by default. So you have to enable it by add credentials
. And there are some libraries that can help you manage cookies:
- https://github.com/joeferraro/react-native-cookies
I added 'credentials': 'include' to my fetch request but it changes nothing. I still got the same response on iOS and android. iOS still works fine and android still doesn't work.
– Anton B
Nov 14 at 14:16
1
And how does it explain why I still receive the cookies properly on iOS but not on android? And it's not like I don't receive any. I still receive one cookie but only 1 on android. Thanks for the links, though!
– Anton B
Nov 14 at 14:27
This must be a bug of RN. Which version of RN are you using? I found a report here and it's not fixed: github.com/facebook/react-native/issues/21795
– Louis Solo
Nov 15 at 3:32
add a comment |
up vote
0
down vote
up vote
0
down vote
Look into the official documents of React Native and MDN:
- https://facebook.github.io/react-native/docs/network
- https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch
The problem here is fetch
won't send or receive any cookie from server by default. So you have to enable it by add credentials
. And there are some libraries that can help you manage cookies:
- https://github.com/joeferraro/react-native-cookies
Look into the official documents of React Native and MDN:
- https://facebook.github.io/react-native/docs/network
- https://developer.mozilla.org/en-US/docs/Web/API/Fetch_API/Using_Fetch
The problem here is fetch
won't send or receive any cookie from server by default. So you have to enable it by add credentials
. And there are some libraries that can help you manage cookies:
- https://github.com/joeferraro/react-native-cookies
answered Nov 13 at 3:25
Louis Solo
1162
1162
I added 'credentials': 'include' to my fetch request but it changes nothing. I still got the same response on iOS and android. iOS still works fine and android still doesn't work.
– Anton B
Nov 14 at 14:16
1
And how does it explain why I still receive the cookies properly on iOS but not on android? And it's not like I don't receive any. I still receive one cookie but only 1 on android. Thanks for the links, though!
– Anton B
Nov 14 at 14:27
This must be a bug of RN. Which version of RN are you using? I found a report here and it's not fixed: github.com/facebook/react-native/issues/21795
– Louis Solo
Nov 15 at 3:32
add a comment |
I added 'credentials': 'include' to my fetch request but it changes nothing. I still got the same response on iOS and android. iOS still works fine and android still doesn't work.
– Anton B
Nov 14 at 14:16
1
And how does it explain why I still receive the cookies properly on iOS but not on android? And it's not like I don't receive any. I still receive one cookie but only 1 on android. Thanks for the links, though!
– Anton B
Nov 14 at 14:27
This must be a bug of RN. Which version of RN are you using? I found a report here and it's not fixed: github.com/facebook/react-native/issues/21795
– Louis Solo
Nov 15 at 3:32
I added 'credentials': 'include' to my fetch request but it changes nothing. I still got the same response on iOS and android. iOS still works fine and android still doesn't work.
– Anton B
Nov 14 at 14:16
I added 'credentials': 'include' to my fetch request but it changes nothing. I still got the same response on iOS and android. iOS still works fine and android still doesn't work.
– Anton B
Nov 14 at 14:16
1
1
And how does it explain why I still receive the cookies properly on iOS but not on android? And it's not like I don't receive any. I still receive one cookie but only 1 on android. Thanks for the links, though!
– Anton B
Nov 14 at 14:27
And how does it explain why I still receive the cookies properly on iOS but not on android? And it's not like I don't receive any. I still receive one cookie but only 1 on android. Thanks for the links, though!
– Anton B
Nov 14 at 14:27
This must be a bug of RN. Which version of RN are you using? I found a report here and it's not fixed: github.com/facebook/react-native/issues/21795
– Louis Solo
Nov 15 at 3:32
This must be a bug of RN. Which version of RN are you using? I found a report here and it's not fixed: github.com/facebook/react-native/issues/21795
– Louis Solo
Nov 15 at 3:32
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53186826%2fonly-receives-one-cookie-in-fetch-response-on-android-but-not-on-ios%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9MiJ8Lcvl 1Hhorf FuBOoVgTT5dWUQLZMEyCVzJx