How can I intentionally discard a [[nodiscard]] return value?
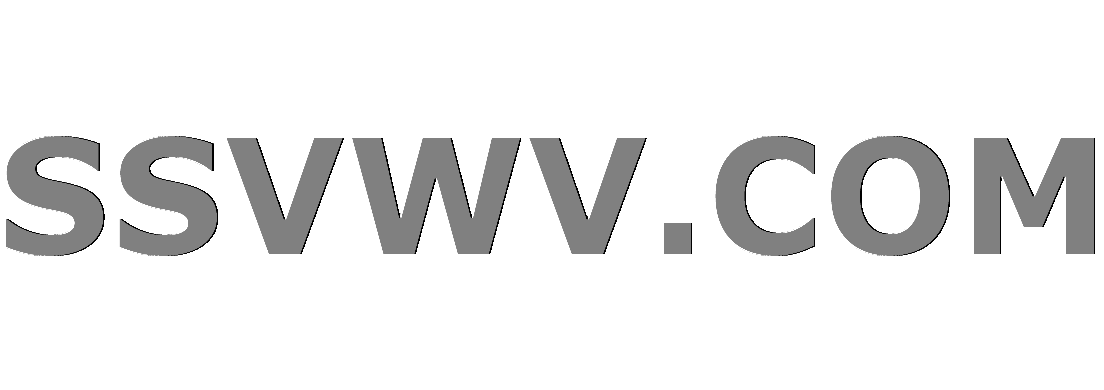
Multi tool use
Say I have
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
foo ();
}
then
error: ignoring return value of ‘int foo()’, declared with attribute nodiscard [-Werror=unused-result]
but if
int x = foo ();
then
error: unused variable ‘x’ [-Werror=unused-variable]
Is there a clean way of telling the compiler "I want to discard this [[nodiscard]]
value"?
c++ c++17
add a comment |
Say I have
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
foo ();
}
then
error: ignoring return value of ‘int foo()’, declared with attribute nodiscard [-Werror=unused-result]
but if
int x = foo ();
then
error: unused variable ‘x’ [-Werror=unused-variable]
Is there a clean way of telling the compiler "I want to discard this [[nodiscard]]
value"?
c++ c++17
Sorry if I'm nosy, but why do you want to discard the value? If it makes sense to run the code and discard the value, the function shouldn't be[[nodiscard]]
, right? I can think of up two reasons to call a[[nodiscard]]
function for side effects, unit testing and cache warming. In the UT case, you normally want to compare the return value against some expected result, though.
– Arne Vogel
Dec 3 '18 at 10:14
In my particular case, a list container erase method returns the iterator to the subsequent list node, but that subsequent node iterator is already known to the calling function.
– spraff
Dec 3 '18 at 11:34
add a comment |
Say I have
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
foo ();
}
then
error: ignoring return value of ‘int foo()’, declared with attribute nodiscard [-Werror=unused-result]
but if
int x = foo ();
then
error: unused variable ‘x’ [-Werror=unused-variable]
Is there a clean way of telling the compiler "I want to discard this [[nodiscard]]
value"?
c++ c++17
Say I have
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
foo ();
}
then
error: ignoring return value of ‘int foo()’, declared with attribute nodiscard [-Werror=unused-result]
but if
int x = foo ();
then
error: unused variable ‘x’ [-Werror=unused-variable]
Is there a clean way of telling the compiler "I want to discard this [[nodiscard]]
value"?
c++ c++17
c++ c++17
edited Dec 2 '18 at 15:37
Matthieu Brucher
12.2k22139
12.2k22139
asked Dec 2 '18 at 15:31
spraff
17.7k1485164
17.7k1485164
Sorry if I'm nosy, but why do you want to discard the value? If it makes sense to run the code and discard the value, the function shouldn't be[[nodiscard]]
, right? I can think of up two reasons to call a[[nodiscard]]
function for side effects, unit testing and cache warming. In the UT case, you normally want to compare the return value against some expected result, though.
– Arne Vogel
Dec 3 '18 at 10:14
In my particular case, a list container erase method returns the iterator to the subsequent list node, but that subsequent node iterator is already known to the calling function.
– spraff
Dec 3 '18 at 11:34
add a comment |
Sorry if I'm nosy, but why do you want to discard the value? If it makes sense to run the code and discard the value, the function shouldn't be[[nodiscard]]
, right? I can think of up two reasons to call a[[nodiscard]]
function for side effects, unit testing and cache warming. In the UT case, you normally want to compare the return value against some expected result, though.
– Arne Vogel
Dec 3 '18 at 10:14
In my particular case, a list container erase method returns the iterator to the subsequent list node, but that subsequent node iterator is already known to the calling function.
– spraff
Dec 3 '18 at 11:34
Sorry if I'm nosy, but why do you want to discard the value? If it makes sense to run the code and discard the value, the function shouldn't be
[[nodiscard]]
, right? I can think of up two reasons to call a [[nodiscard]]
function for side effects, unit testing and cache warming. In the UT case, you normally want to compare the return value against some expected result, though.– Arne Vogel
Dec 3 '18 at 10:14
Sorry if I'm nosy, but why do you want to discard the value? If it makes sense to run the code and discard the value, the function shouldn't be
[[nodiscard]]
, right? I can think of up two reasons to call a [[nodiscard]]
function for side effects, unit testing and cache warming. In the UT case, you normally want to compare the return value against some expected result, though.– Arne Vogel
Dec 3 '18 at 10:14
In my particular case, a list container erase method returns the iterator to the subsequent list node, but that subsequent node iterator is already known to the calling function.
– spraff
Dec 3 '18 at 11:34
In my particular case, a list container erase method returns the iterator to the subsequent list node, but that subsequent node iterator is already known to the calling function.
– spraff
Dec 3 '18 at 11:34
add a comment |
3 Answers
3
active
oldest
votes
The WG14 nodiscard proposal discusses the rationale for allowing the diagnostic to be silenced by casting to void. It says casting to void is the encouraged (if non-normative) way to silence it which follows what the existing implementation do with __attribute__((warn_unused_result))
:
The [[nodiscard]] attribute has extensive real-world use, being implemented by Clang and GCC as __attribute__((warn_unused_result))
, but was standardized under the name [[nodiscard]] by WG21. This proposal chose the identifier nodiscard
because deviation from this name would create a needless incompatibility with C++.
The semantics of this attribute rely heavily on the notion of a use,
the definition of which is left to implementation discretion. However,
the non-normative guidance specified by WG21 is to encourage
implementations to emit a warning diagnostic when a nodiscard function
call is used in a potentially-evalulated discarded-value expression
unless it is an explicit cast to void. This means that an
implementation is not encouraged to perform dataflow analysis (like an
initialized-but- unused local variable diagnostic would require).
...
The C++ way would be static_cast<void>
.
See the draft C++ standard [[dcl.attr.nodiscard]p2:
[ Note: A nodiscard call is a function call expression that calls a function previously declared nodiscard, or whose return type is a possibly cv-qualified class or enumeration type marked nodiscard.
Appearance of a nodiscard call as a potentially-evaluated discarded-value expression is discouraged unless explicitly cast to void.
Implementations should issue a warning in such cases.
This is typically because discarding the return value of a nodiscard call has surprising consequences.
— end note]
This is a note, so non-normative but basically this is what existing implementations do with __attribute__((warn_unused_result))
. Also, note a diagnostic for nodiscard is also also non-normative, so a diagnostic for violating nodiscard is not ill-formed but a quality of implementation just like suppressing via a cast to void is.
see the clang document on nodiscard, warn_unused_result:
Clang supports the ability to diagnose when the results of a function call expression are discarded under suspicious circumstances. A diagnostic is generated when a function or its return type is marked with [[nodiscard]] (or __attribute__((warn_unused_result))) and the function call appears as a potentially-evaluated discarded-value expression that is not explicitly cast to void.
add a comment |
Cast it to void
:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
static_cast<void>(foo());
}
This basically tells the compiler "Yes I know I'm discarding this, yes I'm sure of it."
If only it had been made mandatory in the language years, even decades, ago to have to cast-to-void ignored return values! Think how many bugs could have been averted in the edit-compile cycle before even getting to link/run/debug (much less production)!!
– davidbak
Dec 2 '18 at 16:11
Don't worry, each answer here has one downvote :) Not sure if all were done by the same user.
– Ruslan
Dec 2 '18 at 20:08
@Ruslan Yeah probably. It just annoys me that my comments asking for clarification got deleted.
– Sombrero Chicken
Dec 2 '18 at 20:53
1
@davidbak Except that there was novoid
in the original C language, and it is common practice to ignore the return value from some library functions (likeprintf
). Adding a requirement to explicitly discard the return value from these functions would have broken almost every program in existence at the time. And made for some very inelegant looking code.
– 1201ProgramAlarm
Dec 3 '18 at 3:45
add a comment |
You can also tag the returned int
with another tag:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
[[maybe_unused]] int i = foo ();
}
Could be useful if you have some debug-only code that requires the value.
I just turn off the unused-variable warning with a pragma, globally. There is no more useless warning from compilers than that one. Oh wait, yes there is: Unused parameter (with the implied suggestion that you remove the parameter's identifier from the signature)!
– davidbak
Dec 2 '18 at 16:17
2
Actually, I don't agree. I turned the warning on on purpose on my app, because I don't want to have clutter everywhere, except maybe when I know that with this attribute. Different policies, different approaches ;)
– Matthieu Brucher
Dec 2 '18 at 16:19
1
I liked this solution better than casting to void which I find a bit unintuitive. I also don't find the unused variable/parameter to be useless, in fact, it sometimes catches a bug in a large legacy code base where functions are in the thousands of lines (yeah I know x-). Just my 2c.
– Anders
Dec 2 '18 at 16:23
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53581744%2fhow-can-i-intentionally-discard-a-nodiscard-return-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
The WG14 nodiscard proposal discusses the rationale for allowing the diagnostic to be silenced by casting to void. It says casting to void is the encouraged (if non-normative) way to silence it which follows what the existing implementation do with __attribute__((warn_unused_result))
:
The [[nodiscard]] attribute has extensive real-world use, being implemented by Clang and GCC as __attribute__((warn_unused_result))
, but was standardized under the name [[nodiscard]] by WG21. This proposal chose the identifier nodiscard
because deviation from this name would create a needless incompatibility with C++.
The semantics of this attribute rely heavily on the notion of a use,
the definition of which is left to implementation discretion. However,
the non-normative guidance specified by WG21 is to encourage
implementations to emit a warning diagnostic when a nodiscard function
call is used in a potentially-evalulated discarded-value expression
unless it is an explicit cast to void. This means that an
implementation is not encouraged to perform dataflow analysis (like an
initialized-but- unused local variable diagnostic would require).
...
The C++ way would be static_cast<void>
.
See the draft C++ standard [[dcl.attr.nodiscard]p2:
[ Note: A nodiscard call is a function call expression that calls a function previously declared nodiscard, or whose return type is a possibly cv-qualified class or enumeration type marked nodiscard.
Appearance of a nodiscard call as a potentially-evaluated discarded-value expression is discouraged unless explicitly cast to void.
Implementations should issue a warning in such cases.
This is typically because discarding the return value of a nodiscard call has surprising consequences.
— end note]
This is a note, so non-normative but basically this is what existing implementations do with __attribute__((warn_unused_result))
. Also, note a diagnostic for nodiscard is also also non-normative, so a diagnostic for violating nodiscard is not ill-formed but a quality of implementation just like suppressing via a cast to void is.
see the clang document on nodiscard, warn_unused_result:
Clang supports the ability to diagnose when the results of a function call expression are discarded under suspicious circumstances. A diagnostic is generated when a function or its return type is marked with [[nodiscard]] (or __attribute__((warn_unused_result))) and the function call appears as a potentially-evaluated discarded-value expression that is not explicitly cast to void.
add a comment |
The WG14 nodiscard proposal discusses the rationale for allowing the diagnostic to be silenced by casting to void. It says casting to void is the encouraged (if non-normative) way to silence it which follows what the existing implementation do with __attribute__((warn_unused_result))
:
The [[nodiscard]] attribute has extensive real-world use, being implemented by Clang and GCC as __attribute__((warn_unused_result))
, but was standardized under the name [[nodiscard]] by WG21. This proposal chose the identifier nodiscard
because deviation from this name would create a needless incompatibility with C++.
The semantics of this attribute rely heavily on the notion of a use,
the definition of which is left to implementation discretion. However,
the non-normative guidance specified by WG21 is to encourage
implementations to emit a warning diagnostic when a nodiscard function
call is used in a potentially-evalulated discarded-value expression
unless it is an explicit cast to void. This means that an
implementation is not encouraged to perform dataflow analysis (like an
initialized-but- unused local variable diagnostic would require).
...
The C++ way would be static_cast<void>
.
See the draft C++ standard [[dcl.attr.nodiscard]p2:
[ Note: A nodiscard call is a function call expression that calls a function previously declared nodiscard, or whose return type is a possibly cv-qualified class or enumeration type marked nodiscard.
Appearance of a nodiscard call as a potentially-evaluated discarded-value expression is discouraged unless explicitly cast to void.
Implementations should issue a warning in such cases.
This is typically because discarding the return value of a nodiscard call has surprising consequences.
— end note]
This is a note, so non-normative but basically this is what existing implementations do with __attribute__((warn_unused_result))
. Also, note a diagnostic for nodiscard is also also non-normative, so a diagnostic for violating nodiscard is not ill-formed but a quality of implementation just like suppressing via a cast to void is.
see the clang document on nodiscard, warn_unused_result:
Clang supports the ability to diagnose when the results of a function call expression are discarded under suspicious circumstances. A diagnostic is generated when a function or its return type is marked with [[nodiscard]] (or __attribute__((warn_unused_result))) and the function call appears as a potentially-evaluated discarded-value expression that is not explicitly cast to void.
add a comment |
The WG14 nodiscard proposal discusses the rationale for allowing the diagnostic to be silenced by casting to void. It says casting to void is the encouraged (if non-normative) way to silence it which follows what the existing implementation do with __attribute__((warn_unused_result))
:
The [[nodiscard]] attribute has extensive real-world use, being implemented by Clang and GCC as __attribute__((warn_unused_result))
, but was standardized under the name [[nodiscard]] by WG21. This proposal chose the identifier nodiscard
because deviation from this name would create a needless incompatibility with C++.
The semantics of this attribute rely heavily on the notion of a use,
the definition of which is left to implementation discretion. However,
the non-normative guidance specified by WG21 is to encourage
implementations to emit a warning diagnostic when a nodiscard function
call is used in a potentially-evalulated discarded-value expression
unless it is an explicit cast to void. This means that an
implementation is not encouraged to perform dataflow analysis (like an
initialized-but- unused local variable diagnostic would require).
...
The C++ way would be static_cast<void>
.
See the draft C++ standard [[dcl.attr.nodiscard]p2:
[ Note: A nodiscard call is a function call expression that calls a function previously declared nodiscard, or whose return type is a possibly cv-qualified class or enumeration type marked nodiscard.
Appearance of a nodiscard call as a potentially-evaluated discarded-value expression is discouraged unless explicitly cast to void.
Implementations should issue a warning in such cases.
This is typically because discarding the return value of a nodiscard call has surprising consequences.
— end note]
This is a note, so non-normative but basically this is what existing implementations do with __attribute__((warn_unused_result))
. Also, note a diagnostic for nodiscard is also also non-normative, so a diagnostic for violating nodiscard is not ill-formed but a quality of implementation just like suppressing via a cast to void is.
see the clang document on nodiscard, warn_unused_result:
Clang supports the ability to diagnose when the results of a function call expression are discarded under suspicious circumstances. A diagnostic is generated when a function or its return type is marked with [[nodiscard]] (or __attribute__((warn_unused_result))) and the function call appears as a potentially-evaluated discarded-value expression that is not explicitly cast to void.
The WG14 nodiscard proposal discusses the rationale for allowing the diagnostic to be silenced by casting to void. It says casting to void is the encouraged (if non-normative) way to silence it which follows what the existing implementation do with __attribute__((warn_unused_result))
:
The [[nodiscard]] attribute has extensive real-world use, being implemented by Clang and GCC as __attribute__((warn_unused_result))
, but was standardized under the name [[nodiscard]] by WG21. This proposal chose the identifier nodiscard
because deviation from this name would create a needless incompatibility with C++.
The semantics of this attribute rely heavily on the notion of a use,
the definition of which is left to implementation discretion. However,
the non-normative guidance specified by WG21 is to encourage
implementations to emit a warning diagnostic when a nodiscard function
call is used in a potentially-evalulated discarded-value expression
unless it is an explicit cast to void. This means that an
implementation is not encouraged to perform dataflow analysis (like an
initialized-but- unused local variable diagnostic would require).
...
The C++ way would be static_cast<void>
.
See the draft C++ standard [[dcl.attr.nodiscard]p2:
[ Note: A nodiscard call is a function call expression that calls a function previously declared nodiscard, or whose return type is a possibly cv-qualified class or enumeration type marked nodiscard.
Appearance of a nodiscard call as a potentially-evaluated discarded-value expression is discouraged unless explicitly cast to void.
Implementations should issue a warning in such cases.
This is typically because discarding the return value of a nodiscard call has surprising consequences.
— end note]
This is a note, so non-normative but basically this is what existing implementations do with __attribute__((warn_unused_result))
. Also, note a diagnostic for nodiscard is also also non-normative, so a diagnostic for violating nodiscard is not ill-formed but a quality of implementation just like suppressing via a cast to void is.
see the clang document on nodiscard, warn_unused_result:
Clang supports the ability to diagnose when the results of a function call expression are discarded under suspicious circumstances. A diagnostic is generated when a function or its return type is marked with [[nodiscard]] (or __attribute__((warn_unused_result))) and the function call appears as a potentially-evaluated discarded-value expression that is not explicitly cast to void.
edited Dec 5 '18 at 18:27
answered Dec 2 '18 at 15:54
Shafik Yaghmour
125k23322532
125k23322532
add a comment |
add a comment |
Cast it to void
:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
static_cast<void>(foo());
}
This basically tells the compiler "Yes I know I'm discarding this, yes I'm sure of it."
If only it had been made mandatory in the language years, even decades, ago to have to cast-to-void ignored return values! Think how many bugs could have been averted in the edit-compile cycle before even getting to link/run/debug (much less production)!!
– davidbak
Dec 2 '18 at 16:11
Don't worry, each answer here has one downvote :) Not sure if all were done by the same user.
– Ruslan
Dec 2 '18 at 20:08
@Ruslan Yeah probably. It just annoys me that my comments asking for clarification got deleted.
– Sombrero Chicken
Dec 2 '18 at 20:53
1
@davidbak Except that there was novoid
in the original C language, and it is common practice to ignore the return value from some library functions (likeprintf
). Adding a requirement to explicitly discard the return value from these functions would have broken almost every program in existence at the time. And made for some very inelegant looking code.
– 1201ProgramAlarm
Dec 3 '18 at 3:45
add a comment |
Cast it to void
:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
static_cast<void>(foo());
}
This basically tells the compiler "Yes I know I'm discarding this, yes I'm sure of it."
If only it had been made mandatory in the language years, even decades, ago to have to cast-to-void ignored return values! Think how many bugs could have been averted in the edit-compile cycle before even getting to link/run/debug (much less production)!!
– davidbak
Dec 2 '18 at 16:11
Don't worry, each answer here has one downvote :) Not sure if all were done by the same user.
– Ruslan
Dec 2 '18 at 20:08
@Ruslan Yeah probably. It just annoys me that my comments asking for clarification got deleted.
– Sombrero Chicken
Dec 2 '18 at 20:53
1
@davidbak Except that there was novoid
in the original C language, and it is common practice to ignore the return value from some library functions (likeprintf
). Adding a requirement to explicitly discard the return value from these functions would have broken almost every program in existence at the time. And made for some very inelegant looking code.
– 1201ProgramAlarm
Dec 3 '18 at 3:45
add a comment |
Cast it to void
:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
static_cast<void>(foo());
}
This basically tells the compiler "Yes I know I'm discarding this, yes I'm sure of it."
Cast it to void
:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
static_cast<void>(foo());
}
This basically tells the compiler "Yes I know I'm discarding this, yes I'm sure of it."
edited Dec 2 '18 at 15:41
answered Dec 2 '18 at 15:34


Sombrero Chicken
23.4k33077
23.4k33077
If only it had been made mandatory in the language years, even decades, ago to have to cast-to-void ignored return values! Think how many bugs could have been averted in the edit-compile cycle before even getting to link/run/debug (much less production)!!
– davidbak
Dec 2 '18 at 16:11
Don't worry, each answer here has one downvote :) Not sure if all were done by the same user.
– Ruslan
Dec 2 '18 at 20:08
@Ruslan Yeah probably. It just annoys me that my comments asking for clarification got deleted.
– Sombrero Chicken
Dec 2 '18 at 20:53
1
@davidbak Except that there was novoid
in the original C language, and it is common practice to ignore the return value from some library functions (likeprintf
). Adding a requirement to explicitly discard the return value from these functions would have broken almost every program in existence at the time. And made for some very inelegant looking code.
– 1201ProgramAlarm
Dec 3 '18 at 3:45
add a comment |
If only it had been made mandatory in the language years, even decades, ago to have to cast-to-void ignored return values! Think how many bugs could have been averted in the edit-compile cycle before even getting to link/run/debug (much less production)!!
– davidbak
Dec 2 '18 at 16:11
Don't worry, each answer here has one downvote :) Not sure if all were done by the same user.
– Ruslan
Dec 2 '18 at 20:08
@Ruslan Yeah probably. It just annoys me that my comments asking for clarification got deleted.
– Sombrero Chicken
Dec 2 '18 at 20:53
1
@davidbak Except that there was novoid
in the original C language, and it is common practice to ignore the return value from some library functions (likeprintf
). Adding a requirement to explicitly discard the return value from these functions would have broken almost every program in existence at the time. And made for some very inelegant looking code.
– 1201ProgramAlarm
Dec 3 '18 at 3:45
If only it had been made mandatory in the language years, even decades, ago to have to cast-to-void ignored return values! Think how many bugs could have been averted in the edit-compile cycle before even getting to link/run/debug (much less production)!!
– davidbak
Dec 2 '18 at 16:11
If only it had been made mandatory in the language years, even decades, ago to have to cast-to-void ignored return values! Think how many bugs could have been averted in the edit-compile cycle before even getting to link/run/debug (much less production)!!
– davidbak
Dec 2 '18 at 16:11
Don't worry, each answer here has one downvote :) Not sure if all were done by the same user.
– Ruslan
Dec 2 '18 at 20:08
Don't worry, each answer here has one downvote :) Not sure if all were done by the same user.
– Ruslan
Dec 2 '18 at 20:08
@Ruslan Yeah probably. It just annoys me that my comments asking for clarification got deleted.
– Sombrero Chicken
Dec 2 '18 at 20:53
@Ruslan Yeah probably. It just annoys me that my comments asking for clarification got deleted.
– Sombrero Chicken
Dec 2 '18 at 20:53
1
1
@davidbak Except that there was no
void
in the original C language, and it is common practice to ignore the return value from some library functions (like printf
). Adding a requirement to explicitly discard the return value from these functions would have broken almost every program in existence at the time. And made for some very inelegant looking code.– 1201ProgramAlarm
Dec 3 '18 at 3:45
@davidbak Except that there was no
void
in the original C language, and it is common practice to ignore the return value from some library functions (like printf
). Adding a requirement to explicitly discard the return value from these functions would have broken almost every program in existence at the time. And made for some very inelegant looking code.– 1201ProgramAlarm
Dec 3 '18 at 3:45
add a comment |
You can also tag the returned int
with another tag:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
[[maybe_unused]] int i = foo ();
}
Could be useful if you have some debug-only code that requires the value.
I just turn off the unused-variable warning with a pragma, globally. There is no more useless warning from compilers than that one. Oh wait, yes there is: Unused parameter (with the implied suggestion that you remove the parameter's identifier from the signature)!
– davidbak
Dec 2 '18 at 16:17
2
Actually, I don't agree. I turned the warning on on purpose on my app, because I don't want to have clutter everywhere, except maybe when I know that with this attribute. Different policies, different approaches ;)
– Matthieu Brucher
Dec 2 '18 at 16:19
1
I liked this solution better than casting to void which I find a bit unintuitive. I also don't find the unused variable/parameter to be useless, in fact, it sometimes catches a bug in a large legacy code base where functions are in the thousands of lines (yeah I know x-). Just my 2c.
– Anders
Dec 2 '18 at 16:23
add a comment |
You can also tag the returned int
with another tag:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
[[maybe_unused]] int i = foo ();
}
Could be useful if you have some debug-only code that requires the value.
I just turn off the unused-variable warning with a pragma, globally. There is no more useless warning from compilers than that one. Oh wait, yes there is: Unused parameter (with the implied suggestion that you remove the parameter's identifier from the signature)!
– davidbak
Dec 2 '18 at 16:17
2
Actually, I don't agree. I turned the warning on on purpose on my app, because I don't want to have clutter everywhere, except maybe when I know that with this attribute. Different policies, different approaches ;)
– Matthieu Brucher
Dec 2 '18 at 16:19
1
I liked this solution better than casting to void which I find a bit unintuitive. I also don't find the unused variable/parameter to be useless, in fact, it sometimes catches a bug in a large legacy code base where functions are in the thousands of lines (yeah I know x-). Just my 2c.
– Anders
Dec 2 '18 at 16:23
add a comment |
You can also tag the returned int
with another tag:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
[[maybe_unused]] int i = foo ();
}
Could be useful if you have some debug-only code that requires the value.
You can also tag the returned int
with another tag:
[[nodiscard]] int foo ()
{
return 0;
}
int main ()
{
[[maybe_unused]] int i = foo ();
}
Could be useful if you have some debug-only code that requires the value.
edited Dec 2 '18 at 15:52
answered Dec 2 '18 at 15:36
Matthieu Brucher
12.2k22139
12.2k22139
I just turn off the unused-variable warning with a pragma, globally. There is no more useless warning from compilers than that one. Oh wait, yes there is: Unused parameter (with the implied suggestion that you remove the parameter's identifier from the signature)!
– davidbak
Dec 2 '18 at 16:17
2
Actually, I don't agree. I turned the warning on on purpose on my app, because I don't want to have clutter everywhere, except maybe when I know that with this attribute. Different policies, different approaches ;)
– Matthieu Brucher
Dec 2 '18 at 16:19
1
I liked this solution better than casting to void which I find a bit unintuitive. I also don't find the unused variable/parameter to be useless, in fact, it sometimes catches a bug in a large legacy code base where functions are in the thousands of lines (yeah I know x-). Just my 2c.
– Anders
Dec 2 '18 at 16:23
add a comment |
I just turn off the unused-variable warning with a pragma, globally. There is no more useless warning from compilers than that one. Oh wait, yes there is: Unused parameter (with the implied suggestion that you remove the parameter's identifier from the signature)!
– davidbak
Dec 2 '18 at 16:17
2
Actually, I don't agree. I turned the warning on on purpose on my app, because I don't want to have clutter everywhere, except maybe when I know that with this attribute. Different policies, different approaches ;)
– Matthieu Brucher
Dec 2 '18 at 16:19
1
I liked this solution better than casting to void which I find a bit unintuitive. I also don't find the unused variable/parameter to be useless, in fact, it sometimes catches a bug in a large legacy code base where functions are in the thousands of lines (yeah I know x-). Just my 2c.
– Anders
Dec 2 '18 at 16:23
I just turn off the unused-variable warning with a pragma, globally. There is no more useless warning from compilers than that one. Oh wait, yes there is: Unused parameter (with the implied suggestion that you remove the parameter's identifier from the signature)!
– davidbak
Dec 2 '18 at 16:17
I just turn off the unused-variable warning with a pragma, globally. There is no more useless warning from compilers than that one. Oh wait, yes there is: Unused parameter (with the implied suggestion that you remove the parameter's identifier from the signature)!
– davidbak
Dec 2 '18 at 16:17
2
2
Actually, I don't agree. I turned the warning on on purpose on my app, because I don't want to have clutter everywhere, except maybe when I know that with this attribute. Different policies, different approaches ;)
– Matthieu Brucher
Dec 2 '18 at 16:19
Actually, I don't agree. I turned the warning on on purpose on my app, because I don't want to have clutter everywhere, except maybe when I know that with this attribute. Different policies, different approaches ;)
– Matthieu Brucher
Dec 2 '18 at 16:19
1
1
I liked this solution better than casting to void which I find a bit unintuitive. I also don't find the unused variable/parameter to be useless, in fact, it sometimes catches a bug in a large legacy code base where functions are in the thousands of lines (yeah I know x-). Just my 2c.
– Anders
Dec 2 '18 at 16:23
I liked this solution better than casting to void which I find a bit unintuitive. I also don't find the unused variable/parameter to be useless, in fact, it sometimes catches a bug in a large legacy code base where functions are in the thousands of lines (yeah I know x-). Just my 2c.
– Anders
Dec 2 '18 at 16:23
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53581744%2fhow-can-i-intentionally-discard-a-nodiscard-return-value%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FyS,K3lokbmJHf2BlOrb QIb 1,U5D
Sorry if I'm nosy, but why do you want to discard the value? If it makes sense to run the code and discard the value, the function shouldn't be
[[nodiscard]]
, right? I can think of up two reasons to call a[[nodiscard]]
function for side effects, unit testing and cache warming. In the UT case, you normally want to compare the return value against some expected result, though.– Arne Vogel
Dec 3 '18 at 10:14
In my particular case, a list container erase method returns the iterator to the subsequent list node, but that subsequent node iterator is already known to the calling function.
– spraff
Dec 3 '18 at 11:34