Cant make connection with MariaDB and Java
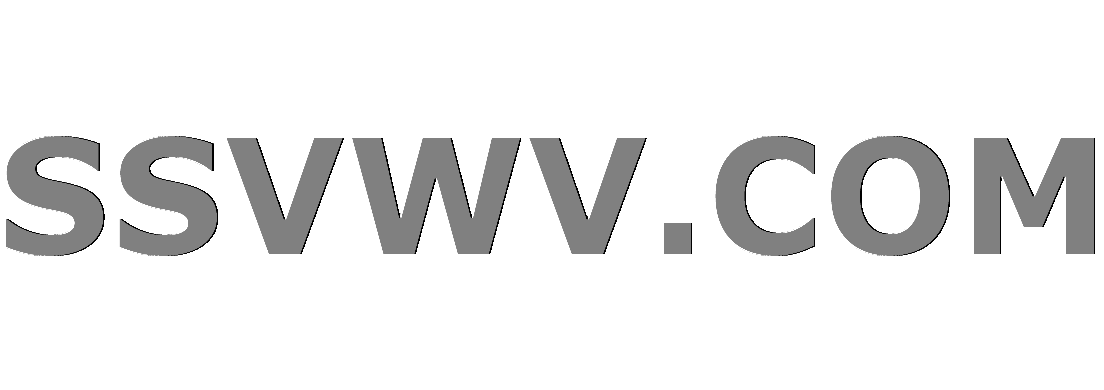
Multi tool use
Im trying to create web app using java and mariadb but i encountered problem when tried to implement mariadb to login. Here my code:
initSql:
package dao;
import java.sql.Connection;
import java.sql.DriverManager;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
@WebServlet("/initSql")
public class initSql extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public initSql() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see Servlet#init(ServletConfig)
*/
Connection conn = null;
public void init(ServletConfig config) throws ServletException {
// TODO Auto-generated method stub
try {
Class.forName("org.mariadb.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mariadb://localhost:3306/baza_new", "root","root");
System.out.println("db povezana");
}catch(Exception e){
//JOptionPane.showMessageDialog(null, e);
System.out.println("db NIiiJE povezana");
//return null;
}
}
}
LoginDAO:
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import dao.initSql;
public class LoginDAO {
static Connection con = null;
public static boolean validate(String username, String password, String type) {
boolean status = false;
try {
con = initSql.init();
System.out.println("1");
String query = "select * from users where username=? and password=?";
PreparedStatement pst = con.prepareStatement(query);
//pst.setString(1, type);
pst.setString(1, username);
pst.setString(2, password);
ResultSet rs = pst.executeQuery();
status= rs.next();
con.close();
}catch(Exception e) {System.out.print(e);}
return status;
}
}
and i get markers:
Cannot make static reference to non-static method from type generic servler
Type mistmatch cannot connect from void to Connection
I'm little bit stuck with this problem.Can someone help me with my code?
java mariadb
add a comment |
Im trying to create web app using java and mariadb but i encountered problem when tried to implement mariadb to login. Here my code:
initSql:
package dao;
import java.sql.Connection;
import java.sql.DriverManager;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
@WebServlet("/initSql")
public class initSql extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public initSql() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see Servlet#init(ServletConfig)
*/
Connection conn = null;
public void init(ServletConfig config) throws ServletException {
// TODO Auto-generated method stub
try {
Class.forName("org.mariadb.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mariadb://localhost:3306/baza_new", "root","root");
System.out.println("db povezana");
}catch(Exception e){
//JOptionPane.showMessageDialog(null, e);
System.out.println("db NIiiJE povezana");
//return null;
}
}
}
LoginDAO:
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import dao.initSql;
public class LoginDAO {
static Connection con = null;
public static boolean validate(String username, String password, String type) {
boolean status = false;
try {
con = initSql.init();
System.out.println("1");
String query = "select * from users where username=? and password=?";
PreparedStatement pst = con.prepareStatement(query);
//pst.setString(1, type);
pst.setString(1, username);
pst.setString(2, password);
ResultSet rs = pst.executeQuery();
status= rs.next();
con.close();
}catch(Exception e) {System.out.print(e);}
return status;
}
}
and i get markers:
Cannot make static reference to non-static method from type generic servler
Type mistmatch cannot connect from void to Connection
I'm little bit stuck with this problem.Can someone help me with my code?
java mariadb
Unrelated to the problem, you can use the MySQL driver here instead of the MariaDB one for a MariaDB connection, usually the driver for MySQL is kept more sanely.
– Rogue
Nov 21 '18 at 14:24
add a comment |
Im trying to create web app using java and mariadb but i encountered problem when tried to implement mariadb to login. Here my code:
initSql:
package dao;
import java.sql.Connection;
import java.sql.DriverManager;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
@WebServlet("/initSql")
public class initSql extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public initSql() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see Servlet#init(ServletConfig)
*/
Connection conn = null;
public void init(ServletConfig config) throws ServletException {
// TODO Auto-generated method stub
try {
Class.forName("org.mariadb.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mariadb://localhost:3306/baza_new", "root","root");
System.out.println("db povezana");
}catch(Exception e){
//JOptionPane.showMessageDialog(null, e);
System.out.println("db NIiiJE povezana");
//return null;
}
}
}
LoginDAO:
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import dao.initSql;
public class LoginDAO {
static Connection con = null;
public static boolean validate(String username, String password, String type) {
boolean status = false;
try {
con = initSql.init();
System.out.println("1");
String query = "select * from users where username=? and password=?";
PreparedStatement pst = con.prepareStatement(query);
//pst.setString(1, type);
pst.setString(1, username);
pst.setString(2, password);
ResultSet rs = pst.executeQuery();
status= rs.next();
con.close();
}catch(Exception e) {System.out.print(e);}
return status;
}
}
and i get markers:
Cannot make static reference to non-static method from type generic servler
Type mistmatch cannot connect from void to Connection
I'm little bit stuck with this problem.Can someone help me with my code?
java mariadb
Im trying to create web app using java and mariadb but i encountered problem when tried to implement mariadb to login. Here my code:
initSql:
package dao;
import java.sql.Connection;
import java.sql.DriverManager;
import javax.servlet.ServletConfig;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
@WebServlet("/initSql")
public class initSql extends HttpServlet {
private static final long serialVersionUID = 1L;
/**
* @see HttpServlet#HttpServlet()
*/
public initSql() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see Servlet#init(ServletConfig)
*/
Connection conn = null;
public void init(ServletConfig config) throws ServletException {
// TODO Auto-generated method stub
try {
Class.forName("org.mariadb.jdbc.Driver");
Connection conn = DriverManager.getConnection("jdbc:mariadb://localhost:3306/baza_new", "root","root");
System.out.println("db povezana");
}catch(Exception e){
//JOptionPane.showMessageDialog(null, e);
System.out.println("db NIiiJE povezana");
//return null;
}
}
}
LoginDAO:
package dao;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import dao.initSql;
public class LoginDAO {
static Connection con = null;
public static boolean validate(String username, String password, String type) {
boolean status = false;
try {
con = initSql.init();
System.out.println("1");
String query = "select * from users where username=? and password=?";
PreparedStatement pst = con.prepareStatement(query);
//pst.setString(1, type);
pst.setString(1, username);
pst.setString(2, password);
ResultSet rs = pst.executeQuery();
status= rs.next();
con.close();
}catch(Exception e) {System.out.print(e);}
return status;
}
}
and i get markers:
Cannot make static reference to non-static method from type generic servler
Type mistmatch cannot connect from void to Connection
I'm little bit stuck with this problem.Can someone help me with my code?
java mariadb
java mariadb
edited Nov 21 '18 at 14:18
Rishikesh Dhokare
2,3441429
2,3441429
asked Nov 21 '18 at 14:16


louseDepolouseDepo
33
33
Unrelated to the problem, you can use the MySQL driver here instead of the MariaDB one for a MariaDB connection, usually the driver for MySQL is kept more sanely.
– Rogue
Nov 21 '18 at 14:24
add a comment |
Unrelated to the problem, you can use the MySQL driver here instead of the MariaDB one for a MariaDB connection, usually the driver for MySQL is kept more sanely.
– Rogue
Nov 21 '18 at 14:24
Unrelated to the problem, you can use the MySQL driver here instead of the MariaDB one for a MariaDB connection, usually the driver for MySQL is kept more sanely.
– Rogue
Nov 21 '18 at 14:24
Unrelated to the problem, you can use the MySQL driver here instead of the MariaDB one for a MariaDB connection, usually the driver for MySQL is kept more sanely.
– Rogue
Nov 21 '18 at 14:24
add a comment |
3 Answers
3
active
oldest
votes
People seem to be neglecting the more broad-scale issues in your code. There are standards to follow like capitalization etc but overall you have some bigger issues.
You shouldn't be making erroneous instances of initSql
as it's an HttpServlet
, it just doesn't make sense. You also have static/non-static references to a Connection
field when you don't need it. To start with, change initSql#init
to return a Connection
, and while I normally wouldn't recommend abusing static this way, make the method itself static:
//returns a connection, requires no class instance
public static Connection init(ServletConfig config) { ... }
From there, we can now retrieve a Connection instance by calling this method:
Connection con = initSql.init();
Overall you should have a proper class or design for handling this, but for simple learning this is "okay".
Secondly, you're not quite using ResultSet
correctly. #next
will determine if there is an available row to point to from the SQL results, and if so it moves the marker to the next row. You would use it in order to check if you can retrieve results:
ResultSet set = /* some sql query */;
String someField;
if (set.next()) {
//gets the value of the column "my_field"
someField = set.getString("my_field");
} else {
//no results!
someField = null;
}
Alternatively, if you were looking for multiple results you can loop over #next
while (set.next()) {
//just one value out of many
String myField = set.getString("my_field");
}
In this use-case it's alright to check if the row exists, but I would personally check against something like user permissions or somesuch. If you relied on code like this for something sensitive you might expose something you don't want to.
Overall, I would work a little more on your logical structure for the code, and maybe go over some of the basics for Java and common coding standards for it (Google and Oracle have good guides for this).
add a comment |
Firstly, your class name initSql should have Capitalized first letter to follow conventions.
Secondly, you should either create an instance/object of InitSql and then call the method init() on that object or make the init() method static.
add a comment |
initSql.init()
isn't static, which is not a problem of MariaDB and its connection from Java :) To fix this error you can add static
to the mentioned method. But: As there are multiple errors in your code (e.g. assigning the result of a void method to a variable), it will not work then either..
How should i fix this?
– louseDepo
Nov 21 '18 at 14:20
remove static from your validate method, and call it on an instance of your LoginDAO class
– JoSSte
Nov 21 '18 at 14:24
@louseDepo I moved my comment to my answer. Feel free to mark it (or any other answer for that matter) as accepted, to resolve this question. Questions without accepted answers remain "open", which is undesired. Ask a new question (after searching the existing questions/answers, obviously) in case there's something unclear with the remaining problems
– crusy
Nov 22 '18 at 7:06
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414042%2fcant-make-connection-with-mariadb-and-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
People seem to be neglecting the more broad-scale issues in your code. There are standards to follow like capitalization etc but overall you have some bigger issues.
You shouldn't be making erroneous instances of initSql
as it's an HttpServlet
, it just doesn't make sense. You also have static/non-static references to a Connection
field when you don't need it. To start with, change initSql#init
to return a Connection
, and while I normally wouldn't recommend abusing static this way, make the method itself static:
//returns a connection, requires no class instance
public static Connection init(ServletConfig config) { ... }
From there, we can now retrieve a Connection instance by calling this method:
Connection con = initSql.init();
Overall you should have a proper class or design for handling this, but for simple learning this is "okay".
Secondly, you're not quite using ResultSet
correctly. #next
will determine if there is an available row to point to from the SQL results, and if so it moves the marker to the next row. You would use it in order to check if you can retrieve results:
ResultSet set = /* some sql query */;
String someField;
if (set.next()) {
//gets the value of the column "my_field"
someField = set.getString("my_field");
} else {
//no results!
someField = null;
}
Alternatively, if you were looking for multiple results you can loop over #next
while (set.next()) {
//just one value out of many
String myField = set.getString("my_field");
}
In this use-case it's alright to check if the row exists, but I would personally check against something like user permissions or somesuch. If you relied on code like this for something sensitive you might expose something you don't want to.
Overall, I would work a little more on your logical structure for the code, and maybe go over some of the basics for Java and common coding standards for it (Google and Oracle have good guides for this).
add a comment |
People seem to be neglecting the more broad-scale issues in your code. There are standards to follow like capitalization etc but overall you have some bigger issues.
You shouldn't be making erroneous instances of initSql
as it's an HttpServlet
, it just doesn't make sense. You also have static/non-static references to a Connection
field when you don't need it. To start with, change initSql#init
to return a Connection
, and while I normally wouldn't recommend abusing static this way, make the method itself static:
//returns a connection, requires no class instance
public static Connection init(ServletConfig config) { ... }
From there, we can now retrieve a Connection instance by calling this method:
Connection con = initSql.init();
Overall you should have a proper class or design for handling this, but for simple learning this is "okay".
Secondly, you're not quite using ResultSet
correctly. #next
will determine if there is an available row to point to from the SQL results, and if so it moves the marker to the next row. You would use it in order to check if you can retrieve results:
ResultSet set = /* some sql query */;
String someField;
if (set.next()) {
//gets the value of the column "my_field"
someField = set.getString("my_field");
} else {
//no results!
someField = null;
}
Alternatively, if you were looking for multiple results you can loop over #next
while (set.next()) {
//just one value out of many
String myField = set.getString("my_field");
}
In this use-case it's alright to check if the row exists, but I would personally check against something like user permissions or somesuch. If you relied on code like this for something sensitive you might expose something you don't want to.
Overall, I would work a little more on your logical structure for the code, and maybe go over some of the basics for Java and common coding standards for it (Google and Oracle have good guides for this).
add a comment |
People seem to be neglecting the more broad-scale issues in your code. There are standards to follow like capitalization etc but overall you have some bigger issues.
You shouldn't be making erroneous instances of initSql
as it's an HttpServlet
, it just doesn't make sense. You also have static/non-static references to a Connection
field when you don't need it. To start with, change initSql#init
to return a Connection
, and while I normally wouldn't recommend abusing static this way, make the method itself static:
//returns a connection, requires no class instance
public static Connection init(ServletConfig config) { ... }
From there, we can now retrieve a Connection instance by calling this method:
Connection con = initSql.init();
Overall you should have a proper class or design for handling this, but for simple learning this is "okay".
Secondly, you're not quite using ResultSet
correctly. #next
will determine if there is an available row to point to from the SQL results, and if so it moves the marker to the next row. You would use it in order to check if you can retrieve results:
ResultSet set = /* some sql query */;
String someField;
if (set.next()) {
//gets the value of the column "my_field"
someField = set.getString("my_field");
} else {
//no results!
someField = null;
}
Alternatively, if you were looking for multiple results you can loop over #next
while (set.next()) {
//just one value out of many
String myField = set.getString("my_field");
}
In this use-case it's alright to check if the row exists, but I would personally check against something like user permissions or somesuch. If you relied on code like this for something sensitive you might expose something you don't want to.
Overall, I would work a little more on your logical structure for the code, and maybe go over some of the basics for Java and common coding standards for it (Google and Oracle have good guides for this).
People seem to be neglecting the more broad-scale issues in your code. There are standards to follow like capitalization etc but overall you have some bigger issues.
You shouldn't be making erroneous instances of initSql
as it's an HttpServlet
, it just doesn't make sense. You also have static/non-static references to a Connection
field when you don't need it. To start with, change initSql#init
to return a Connection
, and while I normally wouldn't recommend abusing static this way, make the method itself static:
//returns a connection, requires no class instance
public static Connection init(ServletConfig config) { ... }
From there, we can now retrieve a Connection instance by calling this method:
Connection con = initSql.init();
Overall you should have a proper class or design for handling this, but for simple learning this is "okay".
Secondly, you're not quite using ResultSet
correctly. #next
will determine if there is an available row to point to from the SQL results, and if so it moves the marker to the next row. You would use it in order to check if you can retrieve results:
ResultSet set = /* some sql query */;
String someField;
if (set.next()) {
//gets the value of the column "my_field"
someField = set.getString("my_field");
} else {
//no results!
someField = null;
}
Alternatively, if you were looking for multiple results you can loop over #next
while (set.next()) {
//just one value out of many
String myField = set.getString("my_field");
}
In this use-case it's alright to check if the row exists, but I would personally check against something like user permissions or somesuch. If you relied on code like this for something sensitive you might expose something you don't want to.
Overall, I would work a little more on your logical structure for the code, and maybe go over some of the basics for Java and common coding standards for it (Google and Oracle have good guides for this).
answered Nov 21 '18 at 14:32


RogueRogue
7,03332854
7,03332854
add a comment |
add a comment |
Firstly, your class name initSql should have Capitalized first letter to follow conventions.
Secondly, you should either create an instance/object of InitSql and then call the method init() on that object or make the init() method static.
add a comment |
Firstly, your class name initSql should have Capitalized first letter to follow conventions.
Secondly, you should either create an instance/object of InitSql and then call the method init() on that object or make the init() method static.
add a comment |
Firstly, your class name initSql should have Capitalized first letter to follow conventions.
Secondly, you should either create an instance/object of InitSql and then call the method init() on that object or make the init() method static.
Firstly, your class name initSql should have Capitalized first letter to follow conventions.
Secondly, you should either create an instance/object of InitSql and then call the method init() on that object or make the init() method static.
answered Nov 21 '18 at 14:24
Ankur ChrungooAnkur Chrungoo
60839
60839
add a comment |
add a comment |
initSql.init()
isn't static, which is not a problem of MariaDB and its connection from Java :) To fix this error you can add static
to the mentioned method. But: As there are multiple errors in your code (e.g. assigning the result of a void method to a variable), it will not work then either..
How should i fix this?
– louseDepo
Nov 21 '18 at 14:20
remove static from your validate method, and call it on an instance of your LoginDAO class
– JoSSte
Nov 21 '18 at 14:24
@louseDepo I moved my comment to my answer. Feel free to mark it (or any other answer for that matter) as accepted, to resolve this question. Questions without accepted answers remain "open", which is undesired. Ask a new question (after searching the existing questions/answers, obviously) in case there's something unclear with the remaining problems
– crusy
Nov 22 '18 at 7:06
add a comment |
initSql.init()
isn't static, which is not a problem of MariaDB and its connection from Java :) To fix this error you can add static
to the mentioned method. But: As there are multiple errors in your code (e.g. assigning the result of a void method to a variable), it will not work then either..
How should i fix this?
– louseDepo
Nov 21 '18 at 14:20
remove static from your validate method, and call it on an instance of your LoginDAO class
– JoSSte
Nov 21 '18 at 14:24
@louseDepo I moved my comment to my answer. Feel free to mark it (or any other answer for that matter) as accepted, to resolve this question. Questions without accepted answers remain "open", which is undesired. Ask a new question (after searching the existing questions/answers, obviously) in case there's something unclear with the remaining problems
– crusy
Nov 22 '18 at 7:06
add a comment |
initSql.init()
isn't static, which is not a problem of MariaDB and its connection from Java :) To fix this error you can add static
to the mentioned method. But: As there are multiple errors in your code (e.g. assigning the result of a void method to a variable), it will not work then either..
initSql.init()
isn't static, which is not a problem of MariaDB and its connection from Java :) To fix this error you can add static
to the mentioned method. But: As there are multiple errors in your code (e.g. assigning the result of a void method to a variable), it will not work then either..
edited Nov 22 '18 at 7:03
answered Nov 21 '18 at 14:18
crusycrusy
3051424
3051424
How should i fix this?
– louseDepo
Nov 21 '18 at 14:20
remove static from your validate method, and call it on an instance of your LoginDAO class
– JoSSte
Nov 21 '18 at 14:24
@louseDepo I moved my comment to my answer. Feel free to mark it (or any other answer for that matter) as accepted, to resolve this question. Questions without accepted answers remain "open", which is undesired. Ask a new question (after searching the existing questions/answers, obviously) in case there's something unclear with the remaining problems
– crusy
Nov 22 '18 at 7:06
add a comment |
How should i fix this?
– louseDepo
Nov 21 '18 at 14:20
remove static from your validate method, and call it on an instance of your LoginDAO class
– JoSSte
Nov 21 '18 at 14:24
@louseDepo I moved my comment to my answer. Feel free to mark it (or any other answer for that matter) as accepted, to resolve this question. Questions without accepted answers remain "open", which is undesired. Ask a new question (after searching the existing questions/answers, obviously) in case there's something unclear with the remaining problems
– crusy
Nov 22 '18 at 7:06
How should i fix this?
– louseDepo
Nov 21 '18 at 14:20
How should i fix this?
– louseDepo
Nov 21 '18 at 14:20
remove static from your validate method, and call it on an instance of your LoginDAO class
– JoSSte
Nov 21 '18 at 14:24
remove static from your validate method, and call it on an instance of your LoginDAO class
– JoSSte
Nov 21 '18 at 14:24
@louseDepo I moved my comment to my answer. Feel free to mark it (or any other answer for that matter) as accepted, to resolve this question. Questions without accepted answers remain "open", which is undesired. Ask a new question (after searching the existing questions/answers, obviously) in case there's something unclear with the remaining problems
– crusy
Nov 22 '18 at 7:06
@louseDepo I moved my comment to my answer. Feel free to mark it (or any other answer for that matter) as accepted, to resolve this question. Questions without accepted answers remain "open", which is undesired. Ask a new question (after searching the existing questions/answers, obviously) in case there's something unclear with the remaining problems
– crusy
Nov 22 '18 at 7:06
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414042%2fcant-make-connection-with-mariadb-and-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
C 3GXdF4G BrU GL4MlHjhTqS8h36vIwC,muzRvXLy2kwn4mII0CapE0OrKqDgsQ
Unrelated to the problem, you can use the MySQL driver here instead of the MariaDB one for a MariaDB connection, usually the driver for MySQL is kept more sanely.
– Rogue
Nov 21 '18 at 14:24