Using foreach to show only certain elements in a list
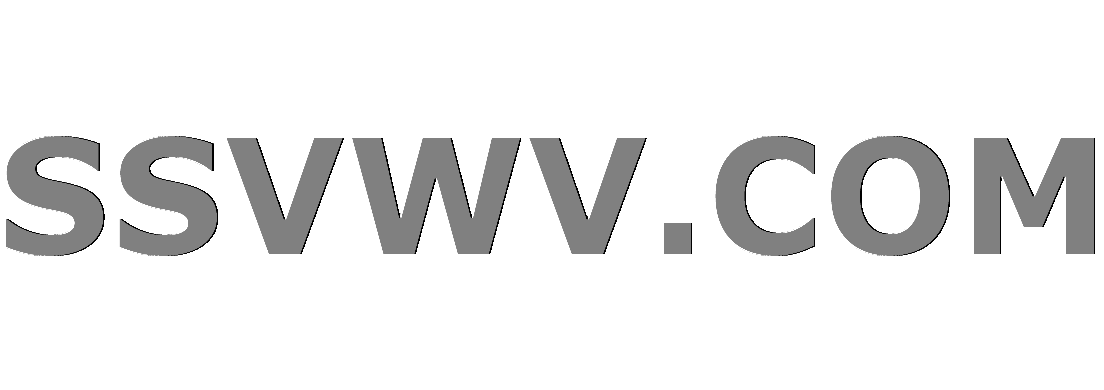
Multi tool use
I'm quite new to c# and I've been trying to search for an answer/solution online but couldn't.
Basically, I've read a csv file in C#, separated the lines by a comma (',') and stored the three sets of data in a table. For eg, one line of the csv file is like: name, date1, date2
You can see the code below (a RHM class has also been created):
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
}
Now that I've imported it successfully, I just want to display e.g. the 5 line in the Name list. So far, I've only managed to display all the names using a foreach loop:
foreach (var RMH in data1)
{
Console.WriteLine(RMH.Name);
}
I've tried using .skip but that will only remove one element from the list. I need a way to show for eg, lines 23, 34, 15.
c#
add a comment |
I'm quite new to c# and I've been trying to search for an answer/solution online but couldn't.
Basically, I've read a csv file in C#, separated the lines by a comma (',') and stored the three sets of data in a table. For eg, one line of the csv file is like: name, date1, date2
You can see the code below (a RHM class has also been created):
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
}
Now that I've imported it successfully, I just want to display e.g. the 5 line in the Name list. So far, I've only managed to display all the names using a foreach loop:
foreach (var RMH in data1)
{
Console.WriteLine(RMH.Name);
}
I've tried using .skip but that will only remove one element from the list. I need a way to show for eg, lines 23, 34, 15.
c#
4
You can use your variable data1 as it was an array data1[4] is the fifth element (an RHM) added to that list, and thus data1[4].Name
– Steve
Nov 20 '18 at 14:42
add a comment |
I'm quite new to c# and I've been trying to search for an answer/solution online but couldn't.
Basically, I've read a csv file in C#, separated the lines by a comma (',') and stored the three sets of data in a table. For eg, one line of the csv file is like: name, date1, date2
You can see the code below (a RHM class has also been created):
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
}
Now that I've imported it successfully, I just want to display e.g. the 5 line in the Name list. So far, I've only managed to display all the names using a foreach loop:
foreach (var RMH in data1)
{
Console.WriteLine(RMH.Name);
}
I've tried using .skip but that will only remove one element from the list. I need a way to show for eg, lines 23, 34, 15.
c#
I'm quite new to c# and I've been trying to search for an answer/solution online but couldn't.
Basically, I've read a csv file in C#, separated the lines by a comma (',') and stored the three sets of data in a table. For eg, one line of the csv file is like: name, date1, date2
You can see the code below (a RHM class has also been created):
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
}
Now that I've imported it successfully, I just want to display e.g. the 5 line in the Name list. So far, I've only managed to display all the names using a foreach loop:
foreach (var RMH in data1)
{
Console.WriteLine(RMH.Name);
}
I've tried using .skip but that will only remove one element from the list. I need a way to show for eg, lines 23, 34, 15.
c#
c#
edited Nov 20 '18 at 14:52
Rand Random
2,97573163
2,97573163
asked Nov 20 '18 at 14:39


RefreshRefresh
31
31
4
You can use your variable data1 as it was an array data1[4] is the fifth element (an RHM) added to that list, and thus data1[4].Name
– Steve
Nov 20 '18 at 14:42
add a comment |
4
You can use your variable data1 as it was an array data1[4] is the fifth element (an RHM) added to that list, and thus data1[4].Name
– Steve
Nov 20 '18 at 14:42
4
4
You can use your variable data1 as it was an array data1[4] is the fifth element (an RHM) added to that list, and thus data1[4].Name
– Steve
Nov 20 '18 at 14:42
You can use your variable data1 as it was an array data1[4] is the fifth element (an RHM) added to that list, and thus data1[4].Name
– Steve
Nov 20 '18 at 14:42
add a comment |
2 Answers
2
active
oldest
votes
You can access object in the list by index. So if you want the 23rd element you simply do list[22]
. Index is 0
based that means list[0]
will be the first element in the list. Here is an example how to access and use your object in list:
RHM 23rdItemInList = data1[22];
Console.WriteLine($"Name of item in list on index 22 is: {23rdItemInList.Name}");
Note if you remove an item from the list, the index will not correspond to actual line in the file. If it matters I would suggest to store line number in your object.
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
int lineNumber = 1; //Here probably should be 2 because you skipping the first line in file with lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Line = lineNumber,
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
lineNumber++;
}
Now you can get object by line number like so:
var 23rdLineData = data1.FirstOrDefault(x=>x.Line == 23);
FirstOrDefault
will return object of null if there is no record in the list with Line == 23.
Check if object is found before using it
if(23rdLineData != null)
Console.WriteLine($"Name on line 23 is {23rdLineData.Name}");
else
Console.WriteLine("There is no data on line 23");
I've tried that before but for some reason it outputs the namespace name. I've also tried doing that in the foreach loop but that outputs the first character
– Refresh
Nov 20 '18 at 14:53
I updated my answer with example and added some notes
– Reniuz
Nov 20 '18 at 15:04
He should check if the item index exists to avoid an exception
– crawletas
Nov 20 '18 at 15:35
add a comment |
There are multiple ways to achive your goal.
You could for example filter your list by any of your object fields by using LINQ like this:
List<RMH> results = data1.Where(d => d.Name == "HPBaxxter");
To filter by linenumber you can use TryGetValue for example:
RMH niceRMH;
int index = 23;
data1.TryGetValue(index, out niceRMH);
TryGetValue
is method ofDictionary
not aList
– Reniuz
Nov 20 '18 at 15:05
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395431%2fusing-foreach-to-show-only-certain-elements-in-a-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can access object in the list by index. So if you want the 23rd element you simply do list[22]
. Index is 0
based that means list[0]
will be the first element in the list. Here is an example how to access and use your object in list:
RHM 23rdItemInList = data1[22];
Console.WriteLine($"Name of item in list on index 22 is: {23rdItemInList.Name}");
Note if you remove an item from the list, the index will not correspond to actual line in the file. If it matters I would suggest to store line number in your object.
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
int lineNumber = 1; //Here probably should be 2 because you skipping the first line in file with lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Line = lineNumber,
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
lineNumber++;
}
Now you can get object by line number like so:
var 23rdLineData = data1.FirstOrDefault(x=>x.Line == 23);
FirstOrDefault
will return object of null if there is no record in the list with Line == 23.
Check if object is found before using it
if(23rdLineData != null)
Console.WriteLine($"Name on line 23 is {23rdLineData.Name}");
else
Console.WriteLine("There is no data on line 23");
I've tried that before but for some reason it outputs the namespace name. I've also tried doing that in the foreach loop but that outputs the first character
– Refresh
Nov 20 '18 at 14:53
I updated my answer with example and added some notes
– Reniuz
Nov 20 '18 at 15:04
He should check if the item index exists to avoid an exception
– crawletas
Nov 20 '18 at 15:35
add a comment |
You can access object in the list by index. So if you want the 23rd element you simply do list[22]
. Index is 0
based that means list[0]
will be the first element in the list. Here is an example how to access and use your object in list:
RHM 23rdItemInList = data1[22];
Console.WriteLine($"Name of item in list on index 22 is: {23rdItemInList.Name}");
Note if you remove an item from the list, the index will not correspond to actual line in the file. If it matters I would suggest to store line number in your object.
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
int lineNumber = 1; //Here probably should be 2 because you skipping the first line in file with lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Line = lineNumber,
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
lineNumber++;
}
Now you can get object by line number like so:
var 23rdLineData = data1.FirstOrDefault(x=>x.Line == 23);
FirstOrDefault
will return object of null if there is no record in the list with Line == 23.
Check if object is found before using it
if(23rdLineData != null)
Console.WriteLine($"Name on line 23 is {23rdLineData.Name}");
else
Console.WriteLine("There is no data on line 23");
I've tried that before but for some reason it outputs the namespace name. I've also tried doing that in the foreach loop but that outputs the first character
– Refresh
Nov 20 '18 at 14:53
I updated my answer with example and added some notes
– Reniuz
Nov 20 '18 at 15:04
He should check if the item index exists to avoid an exception
– crawletas
Nov 20 '18 at 15:35
add a comment |
You can access object in the list by index. So if you want the 23rd element you simply do list[22]
. Index is 0
based that means list[0]
will be the first element in the list. Here is an example how to access and use your object in list:
RHM 23rdItemInList = data1[22];
Console.WriteLine($"Name of item in list on index 22 is: {23rdItemInList.Name}");
Note if you remove an item from the list, the index will not correspond to actual line in the file. If it matters I would suggest to store line number in your object.
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
int lineNumber = 1; //Here probably should be 2 because you skipping the first line in file with lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Line = lineNumber,
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
lineNumber++;
}
Now you can get object by line number like so:
var 23rdLineData = data1.FirstOrDefault(x=>x.Line == 23);
FirstOrDefault
will return object of null if there is no record in the list with Line == 23.
Check if object is found before using it
if(23rdLineData != null)
Console.WriteLine($"Name on line 23 is {23rdLineData.Name}");
else
Console.WriteLine("There is no data on line 23");
You can access object in the list by index. So if you want the 23rd element you simply do list[22]
. Index is 0
based that means list[0]
will be the first element in the list. Here is an example how to access and use your object in list:
RHM 23rdItemInList = data1[22];
Console.WriteLine($"Name of item in list on index 22 is: {23rdItemInList.Name}");
Note if you remove an item from the list, the index will not correspond to actual line in the file. If it matters I would suggest to store line number in your object.
List<RHM> data1 = new List<RHM>();
List<string> lines = File.ReadAllLines(filePath).ToList();
lines.RemoveAt(0);
int lineNumber = 1; //Here probably should be 2 because you skipping the first line in file with lines.RemoveAt(0);
foreach (var line in lines)
{
string entries = line.Split(',');
RHM nRHM = new RMH
{
Line = lineNumber,
Name = entries[0],
Date1 = entries[1],
Date2 = entries[2]
};
data1.Add(nRMH);
lineNumber++;
}
Now you can get object by line number like so:
var 23rdLineData = data1.FirstOrDefault(x=>x.Line == 23);
FirstOrDefault
will return object of null if there is no record in the list with Line == 23.
Check if object is found before using it
if(23rdLineData != null)
Console.WriteLine($"Name on line 23 is {23rdLineData.Name}");
else
Console.WriteLine("There is no data on line 23");
edited Nov 20 '18 at 15:00
answered Nov 20 '18 at 14:45
ReniuzReniuz
10.2k13555
10.2k13555
I've tried that before but for some reason it outputs the namespace name. I've also tried doing that in the foreach loop but that outputs the first character
– Refresh
Nov 20 '18 at 14:53
I updated my answer with example and added some notes
– Reniuz
Nov 20 '18 at 15:04
He should check if the item index exists to avoid an exception
– crawletas
Nov 20 '18 at 15:35
add a comment |
I've tried that before but for some reason it outputs the namespace name. I've also tried doing that in the foreach loop but that outputs the first character
– Refresh
Nov 20 '18 at 14:53
I updated my answer with example and added some notes
– Reniuz
Nov 20 '18 at 15:04
He should check if the item index exists to avoid an exception
– crawletas
Nov 20 '18 at 15:35
I've tried that before but for some reason it outputs the namespace name. I've also tried doing that in the foreach loop but that outputs the first character
– Refresh
Nov 20 '18 at 14:53
I've tried that before but for some reason it outputs the namespace name. I've also tried doing that in the foreach loop but that outputs the first character
– Refresh
Nov 20 '18 at 14:53
I updated my answer with example and added some notes
– Reniuz
Nov 20 '18 at 15:04
I updated my answer with example and added some notes
– Reniuz
Nov 20 '18 at 15:04
He should check if the item index exists to avoid an exception
– crawletas
Nov 20 '18 at 15:35
He should check if the item index exists to avoid an exception
– crawletas
Nov 20 '18 at 15:35
add a comment |
There are multiple ways to achive your goal.
You could for example filter your list by any of your object fields by using LINQ like this:
List<RMH> results = data1.Where(d => d.Name == "HPBaxxter");
To filter by linenumber you can use TryGetValue for example:
RMH niceRMH;
int index = 23;
data1.TryGetValue(index, out niceRMH);
TryGetValue
is method ofDictionary
not aList
– Reniuz
Nov 20 '18 at 15:05
add a comment |
There are multiple ways to achive your goal.
You could for example filter your list by any of your object fields by using LINQ like this:
List<RMH> results = data1.Where(d => d.Name == "HPBaxxter");
To filter by linenumber you can use TryGetValue for example:
RMH niceRMH;
int index = 23;
data1.TryGetValue(index, out niceRMH);
TryGetValue
is method ofDictionary
not aList
– Reniuz
Nov 20 '18 at 15:05
add a comment |
There are multiple ways to achive your goal.
You could for example filter your list by any of your object fields by using LINQ like this:
List<RMH> results = data1.Where(d => d.Name == "HPBaxxter");
To filter by linenumber you can use TryGetValue for example:
RMH niceRMH;
int index = 23;
data1.TryGetValue(index, out niceRMH);
There are multiple ways to achive your goal.
You could for example filter your list by any of your object fields by using LINQ like this:
List<RMH> results = data1.Where(d => d.Name == "HPBaxxter");
To filter by linenumber you can use TryGetValue for example:
RMH niceRMH;
int index = 23;
data1.TryGetValue(index, out niceRMH);
answered Nov 20 '18 at 14:51
epanalepsisepanalepsis
1069
1069
TryGetValue
is method ofDictionary
not aList
– Reniuz
Nov 20 '18 at 15:05
add a comment |
TryGetValue
is method ofDictionary
not aList
– Reniuz
Nov 20 '18 at 15:05
TryGetValue
is method of Dictionary
not a List
– Reniuz
Nov 20 '18 at 15:05
TryGetValue
is method of Dictionary
not a List
– Reniuz
Nov 20 '18 at 15:05
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53395431%2fusing-foreach-to-show-only-certain-elements-in-a-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
S1UL0eX1H KiwiOvkGjfvBXhAJi,f8,fGyc 9sF waxKx,saG4j02Cv
4
You can use your variable data1 as it was an array data1[4] is the fifth element (an RHM) added to that list, and thus data1[4].Name
– Steve
Nov 20 '18 at 14:42