The 465 Arrangement
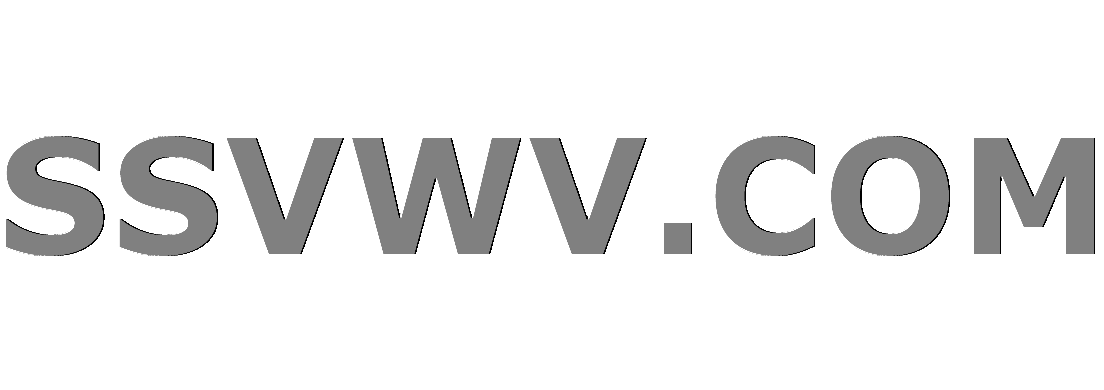
Multi tool use
$begingroup$
Here's the challenge. Write some code to output all the integers in a range. Sounds easy, but here's the tricky part. It will start with the lowest number, then the highest. Then the lowest number which isn't yet in the array. Then the highest which isn't yet in it.
Example:
Lets take 1 to 5 as our start
The numbers are [1, 2, 3, 4, 5].
We take the first, so [1]. Remaining numbers are [2, 3, 4, 5].
We take the last, new array is [1, 5]. Remaining numbers are [2, 3, 4].
We take the first, new array is [1, 5, 2]. Remaining numbers are [3, 4].
We take the last, new array is [1, 5, 2, 4]. Remaining numbers are [3].
We take the first, new array is [1, 5, 2, 4, 3]. No numbers remaining, we're done.
Output [1, 5, 2, 4, 3]
Rules:
- This is code golf, write it in the fewest bytes, any language.
- No standard loopholes.
- Links to an online interpreter, please? (E.g. https://tio.run/)
- Two inputs, both integers. Low end of range, and high end of range.
- I don't mind what the data type of the output is, but it must show the numbers in the correct order.
Examples
Low: 4
High: 6
Result:
4
6
5
Low: 1
High: 5
Result:
1
5
2
4
3
Low: -1
High: 1
Result:
-1
1
0
Low: -1
high: 2
Result:
-1
2
0
1
Low: -50
High: 50
Result:
-50
50
-49
49
-48
48
-47
47
-46
46
-45
45
-44
44
-43
43
-42
42
-41
41
-40
40
-39
39
-38
38
-37
37
-36
36
-35
35
-34
34
-33
33
-32
32
-31
31
-30
30
-29
29
-28
28
-27
27
-26
26
-25
25
-24
24
-23
23
-22
22
-21
21
-20
20
-19
19
-18
18
-17
17
-16
16
-15
15
-14
14
-13
13
-12
12
-11
11
-10
10
-9
9
-8
8
-7
7
-6
6
-5
5
-4
4
-3
3
-2
2
-1
1
0
Happy golfing!
code-golf
$endgroup$
|
show 2 more comments
$begingroup$
Here's the challenge. Write some code to output all the integers in a range. Sounds easy, but here's the tricky part. It will start with the lowest number, then the highest. Then the lowest number which isn't yet in the array. Then the highest which isn't yet in it.
Example:
Lets take 1 to 5 as our start
The numbers are [1, 2, 3, 4, 5].
We take the first, so [1]. Remaining numbers are [2, 3, 4, 5].
We take the last, new array is [1, 5]. Remaining numbers are [2, 3, 4].
We take the first, new array is [1, 5, 2]. Remaining numbers are [3, 4].
We take the last, new array is [1, 5, 2, 4]. Remaining numbers are [3].
We take the first, new array is [1, 5, 2, 4, 3]. No numbers remaining, we're done.
Output [1, 5, 2, 4, 3]
Rules:
- This is code golf, write it in the fewest bytes, any language.
- No standard loopholes.
- Links to an online interpreter, please? (E.g. https://tio.run/)
- Two inputs, both integers. Low end of range, and high end of range.
- I don't mind what the data type of the output is, but it must show the numbers in the correct order.
Examples
Low: 4
High: 6
Result:
4
6
5
Low: 1
High: 5
Result:
1
5
2
4
3
Low: -1
High: 1
Result:
-1
1
0
Low: -1
high: 2
Result:
-1
2
0
1
Low: -50
High: 50
Result:
-50
50
-49
49
-48
48
-47
47
-46
46
-45
45
-44
44
-43
43
-42
42
-41
41
-40
40
-39
39
-38
38
-37
37
-36
36
-35
35
-34
34
-33
33
-32
32
-31
31
-30
30
-29
29
-28
28
-27
27
-26
26
-25
25
-24
24
-23
23
-22
22
-21
21
-20
20
-19
19
-18
18
-17
17
-16
16
-15
15
-14
14
-13
13
-12
12
-11
11
-10
10
-9
9
-8
8
-7
7
-6
6
-5
5
-4
4
-3
3
-2
2
-1
1
0
Happy golfing!
code-golf
$endgroup$
2
$begingroup$
Almost duplicate (the difference being that this one requires reversing the second half before merging).
$endgroup$
– Peter Taylor
Feb 13 at 15:13
$begingroup$
is the input always going to be in the order of low end, high end?
$endgroup$
– Sumner18
Feb 13 at 16:14
1
$begingroup$
@Sumner18 yes. The community here is dead-set against input validation, and I haven’t asked for a reverse-order input, so we can assume it’ll always be low - high.
$endgroup$
– AJFaraday
Feb 13 at 16:21
1
$begingroup$
@Sumner18 How these challenges usually work is that we don't care how invalid inputs are handled. Your code is only judged to be successful by how it deals with valid inputs (i.e. both are integers, the first is lower than the second)
$endgroup$
– AJFaraday
Feb 13 at 16:36
1
$begingroup$
@AJFaraday: you should add a note to the main post indicating that X will be always strictly lower than Y (i.e. X != Y), I missed this comment ;)
$endgroup$
– digEmAll
Feb 13 at 18:23
|
show 2 more comments
$begingroup$
Here's the challenge. Write some code to output all the integers in a range. Sounds easy, but here's the tricky part. It will start with the lowest number, then the highest. Then the lowest number which isn't yet in the array. Then the highest which isn't yet in it.
Example:
Lets take 1 to 5 as our start
The numbers are [1, 2, 3, 4, 5].
We take the first, so [1]. Remaining numbers are [2, 3, 4, 5].
We take the last, new array is [1, 5]. Remaining numbers are [2, 3, 4].
We take the first, new array is [1, 5, 2]. Remaining numbers are [3, 4].
We take the last, new array is [1, 5, 2, 4]. Remaining numbers are [3].
We take the first, new array is [1, 5, 2, 4, 3]. No numbers remaining, we're done.
Output [1, 5, 2, 4, 3]
Rules:
- This is code golf, write it in the fewest bytes, any language.
- No standard loopholes.
- Links to an online interpreter, please? (E.g. https://tio.run/)
- Two inputs, both integers. Low end of range, and high end of range.
- I don't mind what the data type of the output is, but it must show the numbers in the correct order.
Examples
Low: 4
High: 6
Result:
4
6
5
Low: 1
High: 5
Result:
1
5
2
4
3
Low: -1
High: 1
Result:
-1
1
0
Low: -1
high: 2
Result:
-1
2
0
1
Low: -50
High: 50
Result:
-50
50
-49
49
-48
48
-47
47
-46
46
-45
45
-44
44
-43
43
-42
42
-41
41
-40
40
-39
39
-38
38
-37
37
-36
36
-35
35
-34
34
-33
33
-32
32
-31
31
-30
30
-29
29
-28
28
-27
27
-26
26
-25
25
-24
24
-23
23
-22
22
-21
21
-20
20
-19
19
-18
18
-17
17
-16
16
-15
15
-14
14
-13
13
-12
12
-11
11
-10
10
-9
9
-8
8
-7
7
-6
6
-5
5
-4
4
-3
3
-2
2
-1
1
0
Happy golfing!
code-golf
$endgroup$
Here's the challenge. Write some code to output all the integers in a range. Sounds easy, but here's the tricky part. It will start with the lowest number, then the highest. Then the lowest number which isn't yet in the array. Then the highest which isn't yet in it.
Example:
Lets take 1 to 5 as our start
The numbers are [1, 2, 3, 4, 5].
We take the first, so [1]. Remaining numbers are [2, 3, 4, 5].
We take the last, new array is [1, 5]. Remaining numbers are [2, 3, 4].
We take the first, new array is [1, 5, 2]. Remaining numbers are [3, 4].
We take the last, new array is [1, 5, 2, 4]. Remaining numbers are [3].
We take the first, new array is [1, 5, 2, 4, 3]. No numbers remaining, we're done.
Output [1, 5, 2, 4, 3]
Rules:
- This is code golf, write it in the fewest bytes, any language.
- No standard loopholes.
- Links to an online interpreter, please? (E.g. https://tio.run/)
- Two inputs, both integers. Low end of range, and high end of range.
- I don't mind what the data type of the output is, but it must show the numbers in the correct order.
Examples
Low: 4
High: 6
Result:
4
6
5
Low: 1
High: 5
Result:
1
5
2
4
3
Low: -1
High: 1
Result:
-1
1
0
Low: -1
high: 2
Result:
-1
2
0
1
Low: -50
High: 50
Result:
-50
50
-49
49
-48
48
-47
47
-46
46
-45
45
-44
44
-43
43
-42
42
-41
41
-40
40
-39
39
-38
38
-37
37
-36
36
-35
35
-34
34
-33
33
-32
32
-31
31
-30
30
-29
29
-28
28
-27
27
-26
26
-25
25
-24
24
-23
23
-22
22
-21
21
-20
20
-19
19
-18
18
-17
17
-16
16
-15
15
-14
14
-13
13
-12
12
-11
11
-10
10
-9
9
-8
8
-7
7
-6
6
-5
5
-4
4
-3
3
-2
2
-1
1
0
Happy golfing!
code-golf
code-golf
asked Feb 13 at 15:08
AJFaradayAJFaraday
3,47643159
3,47643159
2
$begingroup$
Almost duplicate (the difference being that this one requires reversing the second half before merging).
$endgroup$
– Peter Taylor
Feb 13 at 15:13
$begingroup$
is the input always going to be in the order of low end, high end?
$endgroup$
– Sumner18
Feb 13 at 16:14
1
$begingroup$
@Sumner18 yes. The community here is dead-set against input validation, and I haven’t asked for a reverse-order input, so we can assume it’ll always be low - high.
$endgroup$
– AJFaraday
Feb 13 at 16:21
1
$begingroup$
@Sumner18 How these challenges usually work is that we don't care how invalid inputs are handled. Your code is only judged to be successful by how it deals with valid inputs (i.e. both are integers, the first is lower than the second)
$endgroup$
– AJFaraday
Feb 13 at 16:36
1
$begingroup$
@AJFaraday: you should add a note to the main post indicating that X will be always strictly lower than Y (i.e. X != Y), I missed this comment ;)
$endgroup$
– digEmAll
Feb 13 at 18:23
|
show 2 more comments
2
$begingroup$
Almost duplicate (the difference being that this one requires reversing the second half before merging).
$endgroup$
– Peter Taylor
Feb 13 at 15:13
$begingroup$
is the input always going to be in the order of low end, high end?
$endgroup$
– Sumner18
Feb 13 at 16:14
1
$begingroup$
@Sumner18 yes. The community here is dead-set against input validation, and I haven’t asked for a reverse-order input, so we can assume it’ll always be low - high.
$endgroup$
– AJFaraday
Feb 13 at 16:21
1
$begingroup$
@Sumner18 How these challenges usually work is that we don't care how invalid inputs are handled. Your code is only judged to be successful by how it deals with valid inputs (i.e. both are integers, the first is lower than the second)
$endgroup$
– AJFaraday
Feb 13 at 16:36
1
$begingroup$
@AJFaraday: you should add a note to the main post indicating that X will be always strictly lower than Y (i.e. X != Y), I missed this comment ;)
$endgroup$
– digEmAll
Feb 13 at 18:23
2
2
$begingroup$
Almost duplicate (the difference being that this one requires reversing the second half before merging).
$endgroup$
– Peter Taylor
Feb 13 at 15:13
$begingroup$
Almost duplicate (the difference being that this one requires reversing the second half before merging).
$endgroup$
– Peter Taylor
Feb 13 at 15:13
$begingroup$
is the input always going to be in the order of low end, high end?
$endgroup$
– Sumner18
Feb 13 at 16:14
$begingroup$
is the input always going to be in the order of low end, high end?
$endgroup$
– Sumner18
Feb 13 at 16:14
1
1
$begingroup$
@Sumner18 yes. The community here is dead-set against input validation, and I haven’t asked for a reverse-order input, so we can assume it’ll always be low - high.
$endgroup$
– AJFaraday
Feb 13 at 16:21
$begingroup$
@Sumner18 yes. The community here is dead-set against input validation, and I haven’t asked for a reverse-order input, so we can assume it’ll always be low - high.
$endgroup$
– AJFaraday
Feb 13 at 16:21
1
1
$begingroup$
@Sumner18 How these challenges usually work is that we don't care how invalid inputs are handled. Your code is only judged to be successful by how it deals with valid inputs (i.e. both are integers, the first is lower than the second)
$endgroup$
– AJFaraday
Feb 13 at 16:36
$begingroup$
@Sumner18 How these challenges usually work is that we don't care how invalid inputs are handled. Your code is only judged to be successful by how it deals with valid inputs (i.e. both are integers, the first is lower than the second)
$endgroup$
– AJFaraday
Feb 13 at 16:36
1
1
$begingroup$
@AJFaraday: you should add a note to the main post indicating that X will be always strictly lower than Y (i.e. X != Y), I missed this comment ;)
$endgroup$
– digEmAll
Feb 13 at 18:23
$begingroup$
@AJFaraday: you should add a note to the main post indicating that X will be always strictly lower than Y (i.e. X != Y), I missed this comment ;)
$endgroup$
– digEmAll
Feb 13 at 18:23
|
show 2 more comments
50 Answers
50
active
oldest
votes
1 2
next
$begingroup$
R, 38 37 36 bytes
function(a,b)rbind(a:b,b:a)[a:b-a+1]
Try it online!
- -1 byte thanks to @user2390246
- -1 byte thanks to @Kirill L.
Exploiting the fact that R stores matrices column-wise
$endgroup$
$begingroup$
Usingrbind
is much better than my approach, but you can save 1 byte by using[seq(a:b)]
instead ofunique
.
$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
You're right, I missed the comment where has been specified that a < b (never equal), so we can useseq(a:b)
$endgroup$
– digEmAll
Feb 13 at 18:06
$begingroup$
@digEmAll My solution was essentially a literal interpretation of the puzzle, I never would have even thought of doing something such as this. Impressive, have an upvote!
$endgroup$
– Sumner18
Feb 13 at 18:07
1
$begingroup$
-1 more
$endgroup$
– Kirill L.
Feb 13 at 20:32
add a comment |
$begingroup$
Haskell, 30 bytes
a%b=a:take(b-a)(b:(a+1)%(b-1))
Try it online!
$endgroup$
$begingroup$
Dammit! I have just found the exact same solution. Oh well
$endgroup$
– proud haskeller
Feb 14 at 13:49
add a comment |
$begingroup$
Haskell, 39 bytes
a#b|a>b=a:b#(a-1)|a<b=a:b#(a+1)|1>0=[a]
Try it online!
$endgroup$
add a comment |
$begingroup$
R, 65 64 61 60 bytes
-1 byte thanks to Robert S.
-4 more thanks to digEmAll
x=scan();z=x:x[2];while(sum(z|1)){cat(z[1],"");z=rev(z[-1])}
Try it online!
$endgroup$
$begingroup$
You can replacelength(z)
withsum(z|1)
to save 1 byte :)
$endgroup$
– Robert S.
Feb 13 at 17:09
$begingroup$
I don't understand how that works but I guess it does. sum(z|1) seems like it would always evaluate to at least 1, which would cause the while loop to loop endlessly. but apparently not
$endgroup$
– Sumner18
Feb 13 at 17:27
3
$begingroup$
z is a vector. each element of that vector is|
ed with 1. Which is always equal to 1. When you take the sum, you have a vector filled withTRUE
s so the result is equal to the length of the vector. If the vector is empty, you the is nothing to|
with so the output vector islogical(0)
. When you take that sum, it's 0
$endgroup$
– OganM
Feb 13 at 23:59
add a comment |
$begingroup$
Python 2, 44 bytes
f=lambda a,b:[a]*(a==b)or[a]+f(b,a-cmp(a,b))
Try it online!
$endgroup$
add a comment |
$begingroup$
PowerShell, 59 48 bytes
param($a,$b)(($z=0..($b-$a))|%{$a+$_;$b-$_})[$z]
Try it online!
(Seems long...)
Takes input $a
and $b
, constructs the range 0 .. ($b-$a)
, stores that into $z
, then loops through that range. The looping through that range is just used as a counter to ensure we get enough iterations. Each iteration, we put $a
and $b
on the pipeline with addition/subtraction. That gives us something like 1,5,2,4,3,3,4,2,5,1
so we need to slice into that from 0
up to the $b-$a
(i.e., the count) of the original array so we're only left with the appropriate elements. That's left on the pipeline and output is implicit.
-11 bytes thanks to mazzy.
$endgroup$
$begingroup$
48 bytes
$endgroup$
– mazzy
Feb 13 at 17:29
$begingroup$
@mazzy Ah, I like that$b-$a
trick -- that's clever!
$endgroup$
– AdmBorkBork
Feb 13 at 17:40
add a comment |
$begingroup$
05AB1E, 6 bytes
ŸDvć,R
Try it online!
Explanation
Ÿ # push range [min ... max]
D # duplicate
v # for each element in the copy
ć, # extract and print the head of the original list
R # and then reverse it
$endgroup$
$begingroup$
Ÿ2ä`R.ι non iterative using interleave, but this is still much better.
$endgroup$
– Magic Octopus Urn
Feb 14 at 2:36
1
$begingroup$
@MagicOctopusUrn: I tried a non-iterative solution first, but it was even worse since I didn't know about.ι
;)
$endgroup$
– Emigna
Feb 14 at 7:36
$begingroup$
Similar as what I had in mind, so obvious +1 from me. I do like your alternative 7-byter as well through, @MagicOctopusUrn. :)
$endgroup$
– Kevin Cruijssen
Feb 14 at 8:28
1
$begingroup$
@KristianWilliams: Seems to be working for me.
$endgroup$
– Emigna
Feb 14 at 9:08
1
$begingroup$
@KevinCruijssen: I switched to a pair instead as that felt more intuitive anyways :)
$endgroup$
– Emigna
Feb 14 at 10:50
|
show 3 more comments
$begingroup$
Wolfram Language (Mathematica), 56 54 bytes
This is my first time golfing!
f[a_,b_]:=(c=a~Range~b;Drop[c~Riffle~Reverse@c,a-b-1])
Try it online!
Saved 2 bytes using infix notation.
Explanation:
f[a_,b_]:= function of two variables
c=a~Range~b; list of integers from a to b
Reverse@c same list in reverse
c~Riffle~Reverse@c interleave the two lists
Drop[c~Riffle~Reverse@c,a-b-1] drop last |a-b-1| elements (note a-b-1 < 0)
Alternatively, we could use Take[...,b-a+1]
for the same result.
Tests:
f[4, 6]
f[1, 5]
f[-1, 1]
f[-1, 2]
Ouput:
{4, 6, 5}
{1, 5, 2, 4, 3}
{-1, 1, 0}
{-1, 2, 0, 1}
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
The "Try it online" link returns a 403. "Sorry, you do not have permission to access this item."
$endgroup$
– Rohit Namjoshi
Feb 15 at 0:02
$begingroup$
@RohitNamjoshi I updated the link
$endgroup$
– Kai
Feb 15 at 0:24
$begingroup$
note that on TIO you can place header and footer code in the text boxes above and below the actual code box. This makes the code look cleaner, as well as allow you to take advantage the PPCG answer formatter (esc-s-g). Try it online!
$endgroup$
– Jo King
Feb 15 at 0:46
$begingroup$
@JoKing much appreciated, I had never used it before!
$endgroup$
– Kai
Feb 15 at 0:50
add a comment |
$begingroup$
Japt, 14 bytes
òV
íUs w)c vUl
Try it online!
$endgroup$
$begingroup$
13 bytes, using the same approach.
$endgroup$
– Shaggy
Feb 13 at 23:01
add a comment |
$begingroup$
Stax, 7 bytes
É╓ÅìΔà▲
Run and debug it
$endgroup$
add a comment |
$begingroup$
cQuents, 19 bytes
#|B-A+1&A+k-1,B-k+1
Try it online!
Note that it does not work on TIO right now because TIO's interpreter is not up to date.
Explanation
#|B-A+1&A+k-1,B-k+1
A is the first input, B is the second input
#|B-A+1 n = B - A + 1
& Print the first n terms of the sequence
k starts at 1 and increments whenever we return to the first term
A+k-1, Terms alternate between A + k - 1 and
B-k+1 B - k + 1
increment k
$endgroup$
add a comment |
$begingroup$
Haskell, 39 bytes
f(a:b)=a:f(reverse b)
f x=x
a#b=f[a..b]
Try it online!
$endgroup$
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 46 bytes
a=>b=>{for(;a<=b;Write(a+(b>a++?b--+"":"")));}
Saved 4 bytes thanks to dana!
Try it online!
C# (Visual C# Interactive Compiler), 65 bytes
void z(int a,int b){if(a<=b){Write(a+(b>a?b+"":""));z(a+1,b-1);}}
Try it online!
$endgroup$
$begingroup$
I didn't notice the extra empty string there, thanks!
$endgroup$
– Embodiment of Ignorance
Feb 14 at 16:23
add a comment |
$begingroup$
APL (dzaima/APL), 21 bytes
⌈⊢+.5×-+∘(⌽ׯ1*)∘⍳1+-
Try it online!
$endgroup$
add a comment |
$begingroup$
Japt, 7 bytes
Takes input as an array.
rõ
ÊÆÔv
Try it or run all test cases
:Implicit input of array U=[low,high]
r :Reduce by
õ : Inclusive, reversed range (giving the range [high,low])
n :Reassign to U
Ê :Length
Æ :Map the range [0,Ê)
Ô : Reverse U
v : Remove the first element
$endgroup$
add a comment |
$begingroup$
R, 51 bytes
function(x,y,z=x:y)matrix(c(z,rev(z)),2,,T)[seq(z)]
Try it online!
Explanation:
For a sequence x:y
of length N
, create a two-by-N matrix consisting of the sequence x:y in the top row and y:x in the bottom row matrix(c(z,rev(z)),2,,T)
. If we select the first N
elements of the matrix [seq(z)]
, they will be chosen by column, giving the required output.
Outgolfed by digEmAll
$endgroup$
1
$begingroup$
I just posted a very similar approach 30 seconds before you :D
$endgroup$
– digEmAll
Feb 13 at 18:01
$begingroup$
@digEmAll Yes, but yours is a lot better!
$endgroup$
– user2390246
Feb 13 at 18:03
add a comment |
$begingroup$
MATL, 8 bytes
&:t"1&)P
Try it online!
Explanation
&: % Take two inputs (implicit). Two-input range
t % Duplicate
" % For each
1&) % Push first element, then an array with the rest
P % Reverse array
% End (implicit). Display (implicit)
$endgroup$
add a comment |
$begingroup$
JavaScript, 40 bytes
l=>g=h=>h>l?[l++,h--,...g(h)]:h<l?:[l]
Try It Online!
$endgroup$
add a comment |
$begingroup$
Forth (gforth), 52 bytes
: f 2dup - 1+ 0 do dup . i 2 mod 2* 1- - swap loop ;
Try it online!
Explanation
Loop from 0 to (End - Start). Place End and Start on top of the stack.
Each Iteration:
- Output the current number
- Add (or subtract) 1 from the current number
- Switch the current number with the other number
Code Explanation
: f start new word definition
2dup - get the size of the range (total number of integers)
1+ 0 add 1 to the size because forth loops are [Inclusive, Exclusive)
do start counted loop from 0 to size+1
dup . output the current top of the stack
i 2 mod get the index of the loop modulus 2
2* 1- convert from 0,1 to -1,1
- subtract result from top of stack (adds 1 to lower bound and subtracts 1 from upper)
swap swap the top two stack numbers
loop end the counted loop
; end the word definition
$endgroup$
add a comment |
$begingroup$
Julia 0.7, 29 bytes
f(a,b)=[a:b b:-1:a]'[1:1+b-a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Haskell, 30 bytes
l%h=l:take(h-l)(h:(l+1)%(h-1))
Usage: 3%7
gives `[3,7,4,6,5]
For the inputs l, h
the function calls recursively with the inputs l+1, h-1
, and adds l,h
to the beggining.
Instead of any halting condition, the code uses take(h-l)
to shorten the sequence to the right length (which would otherwise be an infinite sequence of increasing and decreasing numbers).
$endgroup$
add a comment |
$begingroup$
JVM bytecode (OpenJDK asmtools JASM), 449 bytes
enum b{const #1=Method java/io/PrintStream.print:(I)V;static Method a:(II)V stack 2 locals 4{getstatic java/lang/System.out:"Ljava/io/PrintStream;";astore 3;ldc 0;istore 2;l:iload 2;ldc 1;if_icmpeq t;aload 3;iload 0;invokevirtual #1;iinc 0,1;iinc 2,1;goto c;t:aload 3;iload 1;invokevirtual #1;iinc 1,-1;iinc 2,-1;c:aload 3;ldc 32;i2c;invokevirtual java/io/PrintStream.print:(C)V;iload 0;iload 1;if_icmpne l;aload 3;iload 0;invokevirtual #1;return;}}
Ungolfed (and slightly cleaner)
enum b {
public static Method "a":(II)V stack 5 locals 4 {
getstatic "java/lang/System"."out":"Ljava/io/PrintStream;";
astore 3;
ldc 0;
istore 2;
loop:
iload 2;
ldc 1;
if_icmpeq true;
false:
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 0,1;
iinc 2,1;
goto cond;
true:
aload 3;
iload 1;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 1,-1;
iinc 2,-1;
goto cond;
cond:
iload 0;
iload 1;
if_icmpne loop;
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
return;
}
}
Standalone function, needs to be called from Java as b.a(num1,num2)
.
Explanation
This code uses the method parameters as variables, as well as a boolean in local #3 deciding which number to output. Each loop iteration either the left or right is output, and that number is incremented for the left or decremented for the right. Loop continues until both numbers are equal, then that number is output.
...I have a distinct feeling I'm massively outgunned on the byte count
$endgroup$
add a comment |
$begingroup$
Ink, 46 bytes
=h(I,A)
{I<=A:{I} {I<A:{A} ->h(I+1,A-1)}}->->
(I don't think there's an online interpreter for Ink, sorry)
Defines a stitch called h, which takes two arguments I and A, which are the bounds of the range.
Outputs by printing values, separated by spaces, to stdout.
Explanation
=h(min, max) // Define the stitch.
{min <= max:{min}/* print min unless it's greater than max */{min < max: {max} /*Also print max if it's greater than min*/->h(min+1, max-1)/*Then divert, with the arguments changed*/}}
->-> // If we didn't divert earlier, divert to wherever the stitch was called from
$endgroup$
add a comment |
$begingroup$
Perl 5 -ln
, 37 bytes
@.=$_..<>;say shift@.,$/,pop@.while@.
Try it online!
$endgroup$
$begingroup$
33bytes
$endgroup$
– Nahuel Fouilleul
Feb 14 at 14:47
add a comment |
$begingroup$
Java (JDK), 52 bytes
(l,h,o)->{for(int i=0;l<=h;i^=1)o.add(i<1?l++:h--);}
Try it online!
$endgroup$
add a comment |
$begingroup$
Clean, 48 bytes
import StdEnv
$a b|a<>b=[a: $b(a+sign(b-a))]=[a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Ruby, 37 36 33 bytes
f=->a,b{a>b ?:[a,b]|f[a+1,b-1]}
Try it online!
Recursive version with 3 bytes saved by G B.
Ruby, 38 bytes
->a,b{d=*c=a..b;c.map{d.reverse!.pop}}
Try it online!
Non-recursive version.
$endgroup$
add a comment |
$begingroup$
Cubix, 16 bytes
;w(.II>sO-?@;)^/
Try it here
Cubified
; w
( .
I I > s O - ? @
; ) ^ / . . . .
. .
. .
Explanation
Basically, this moves the two bounds closer together one step at a time until they meet. Each time through the loop, we s
wap the bounds, O
utput, take the difference, and increment with )
or decrement with (
based on the sign.
$endgroup$
add a comment |
$begingroup$
Pyth, 10 8 bytes
{.iF_B}F
Try it here
Explanation
{.iF_B}F
}FQ Generate the range between the (implicit) inputs.
.iF_B Interleave it with its reverse.
{ Deduplicate.
$endgroup$
add a comment |
$begingroup$
JavaScript, 35 bytes
f=(a,b,s=1)=>a-b?a+[,f(b,a+s,-s)]:a
Try it online!
Thanks to Arnauld, 1 byte saved.
$endgroup$
add a comment |
1 2
next
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "200"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f179852%2fthe-465-arrangement%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
50 Answers
50
active
oldest
votes
50 Answers
50
active
oldest
votes
active
oldest
votes
active
oldest
votes
1 2
next
$begingroup$
R, 38 37 36 bytes
function(a,b)rbind(a:b,b:a)[a:b-a+1]
Try it online!
- -1 byte thanks to @user2390246
- -1 byte thanks to @Kirill L.
Exploiting the fact that R stores matrices column-wise
$endgroup$
$begingroup$
Usingrbind
is much better than my approach, but you can save 1 byte by using[seq(a:b)]
instead ofunique
.
$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
You're right, I missed the comment where has been specified that a < b (never equal), so we can useseq(a:b)
$endgroup$
– digEmAll
Feb 13 at 18:06
$begingroup$
@digEmAll My solution was essentially a literal interpretation of the puzzle, I never would have even thought of doing something such as this. Impressive, have an upvote!
$endgroup$
– Sumner18
Feb 13 at 18:07
1
$begingroup$
-1 more
$endgroup$
– Kirill L.
Feb 13 at 20:32
add a comment |
$begingroup$
R, 38 37 36 bytes
function(a,b)rbind(a:b,b:a)[a:b-a+1]
Try it online!
- -1 byte thanks to @user2390246
- -1 byte thanks to @Kirill L.
Exploiting the fact that R stores matrices column-wise
$endgroup$
$begingroup$
Usingrbind
is much better than my approach, but you can save 1 byte by using[seq(a:b)]
instead ofunique
.
$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
You're right, I missed the comment where has been specified that a < b (never equal), so we can useseq(a:b)
$endgroup$
– digEmAll
Feb 13 at 18:06
$begingroup$
@digEmAll My solution was essentially a literal interpretation of the puzzle, I never would have even thought of doing something such as this. Impressive, have an upvote!
$endgroup$
– Sumner18
Feb 13 at 18:07
1
$begingroup$
-1 more
$endgroup$
– Kirill L.
Feb 13 at 20:32
add a comment |
$begingroup$
R, 38 37 36 bytes
function(a,b)rbind(a:b,b:a)[a:b-a+1]
Try it online!
- -1 byte thanks to @user2390246
- -1 byte thanks to @Kirill L.
Exploiting the fact that R stores matrices column-wise
$endgroup$
R, 38 37 36 bytes
function(a,b)rbind(a:b,b:a)[a:b-a+1]
Try it online!
- -1 byte thanks to @user2390246
- -1 byte thanks to @Kirill L.
Exploiting the fact that R stores matrices column-wise
edited Feb 13 at 20:57
answered Feb 13 at 17:59
digEmAlldigEmAll
3,369415
3,369415
$begingroup$
Usingrbind
is much better than my approach, but you can save 1 byte by using[seq(a:b)]
instead ofunique
.
$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
You're right, I missed the comment where has been specified that a < b (never equal), so we can useseq(a:b)
$endgroup$
– digEmAll
Feb 13 at 18:06
$begingroup$
@digEmAll My solution was essentially a literal interpretation of the puzzle, I never would have even thought of doing something such as this. Impressive, have an upvote!
$endgroup$
– Sumner18
Feb 13 at 18:07
1
$begingroup$
-1 more
$endgroup$
– Kirill L.
Feb 13 at 20:32
add a comment |
$begingroup$
Usingrbind
is much better than my approach, but you can save 1 byte by using[seq(a:b)]
instead ofunique
.
$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
You're right, I missed the comment where has been specified that a < b (never equal), so we can useseq(a:b)
$endgroup$
– digEmAll
Feb 13 at 18:06
$begingroup$
@digEmAll My solution was essentially a literal interpretation of the puzzle, I never would have even thought of doing something such as this. Impressive, have an upvote!
$endgroup$
– Sumner18
Feb 13 at 18:07
1
$begingroup$
-1 more
$endgroup$
– Kirill L.
Feb 13 at 20:32
$begingroup$
Using
rbind
is much better than my approach, but you can save 1 byte by using [seq(a:b)]
instead of unique
.$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
Using
rbind
is much better than my approach, but you can save 1 byte by using [seq(a:b)]
instead of unique
.$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
You're right, I missed the comment where has been specified that a < b (never equal), so we can use
seq(a:b)
$endgroup$
– digEmAll
Feb 13 at 18:06
$begingroup$
You're right, I missed the comment where has been specified that a < b (never equal), so we can use
seq(a:b)
$endgroup$
– digEmAll
Feb 13 at 18:06
$begingroup$
@digEmAll My solution was essentially a literal interpretation of the puzzle, I never would have even thought of doing something such as this. Impressive, have an upvote!
$endgroup$
– Sumner18
Feb 13 at 18:07
$begingroup$
@digEmAll My solution was essentially a literal interpretation of the puzzle, I never would have even thought of doing something such as this. Impressive, have an upvote!
$endgroup$
– Sumner18
Feb 13 at 18:07
1
1
$begingroup$
-1 more
$endgroup$
– Kirill L.
Feb 13 at 20:32
$begingroup$
-1 more
$endgroup$
– Kirill L.
Feb 13 at 20:32
add a comment |
$begingroup$
Haskell, 30 bytes
a%b=a:take(b-a)(b:(a+1)%(b-1))
Try it online!
$endgroup$
$begingroup$
Dammit! I have just found the exact same solution. Oh well
$endgroup$
– proud haskeller
Feb 14 at 13:49
add a comment |
$begingroup$
Haskell, 30 bytes
a%b=a:take(b-a)(b:(a+1)%(b-1))
Try it online!
$endgroup$
$begingroup$
Dammit! I have just found the exact same solution. Oh well
$endgroup$
– proud haskeller
Feb 14 at 13:49
add a comment |
$begingroup$
Haskell, 30 bytes
a%b=a:take(b-a)(b:(a+1)%(b-1))
Try it online!
$endgroup$
Haskell, 30 bytes
a%b=a:take(b-a)(b:(a+1)%(b-1))
Try it online!
answered Feb 14 at 0:56


xnorxnor
90.9k18186441
90.9k18186441
$begingroup$
Dammit! I have just found the exact same solution. Oh well
$endgroup$
– proud haskeller
Feb 14 at 13:49
add a comment |
$begingroup$
Dammit! I have just found the exact same solution. Oh well
$endgroup$
– proud haskeller
Feb 14 at 13:49
$begingroup$
Dammit! I have just found the exact same solution. Oh well
$endgroup$
– proud haskeller
Feb 14 at 13:49
$begingroup$
Dammit! I have just found the exact same solution. Oh well
$endgroup$
– proud haskeller
Feb 14 at 13:49
add a comment |
$begingroup$
Haskell, 39 bytes
a#b|a>b=a:b#(a-1)|a<b=a:b#(a+1)|1>0=[a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Haskell, 39 bytes
a#b|a>b=a:b#(a-1)|a<b=a:b#(a+1)|1>0=[a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Haskell, 39 bytes
a#b|a>b=a:b#(a-1)|a<b=a:b#(a+1)|1>0=[a]
Try it online!
$endgroup$
Haskell, 39 bytes
a#b|a>b=a:b#(a-1)|a<b=a:b#(a+1)|1>0=[a]
Try it online!
answered Feb 13 at 17:15
ovsovs
19k21159
19k21159
add a comment |
add a comment |
$begingroup$
R, 65 64 61 60 bytes
-1 byte thanks to Robert S.
-4 more thanks to digEmAll
x=scan();z=x:x[2];while(sum(z|1)){cat(z[1],"");z=rev(z[-1])}
Try it online!
$endgroup$
$begingroup$
You can replacelength(z)
withsum(z|1)
to save 1 byte :)
$endgroup$
– Robert S.
Feb 13 at 17:09
$begingroup$
I don't understand how that works but I guess it does. sum(z|1) seems like it would always evaluate to at least 1, which would cause the while loop to loop endlessly. but apparently not
$endgroup$
– Sumner18
Feb 13 at 17:27
3
$begingroup$
z is a vector. each element of that vector is|
ed with 1. Which is always equal to 1. When you take the sum, you have a vector filled withTRUE
s so the result is equal to the length of the vector. If the vector is empty, you the is nothing to|
with so the output vector islogical(0)
. When you take that sum, it's 0
$endgroup$
– OganM
Feb 13 at 23:59
add a comment |
$begingroup$
R, 65 64 61 60 bytes
-1 byte thanks to Robert S.
-4 more thanks to digEmAll
x=scan();z=x:x[2];while(sum(z|1)){cat(z[1],"");z=rev(z[-1])}
Try it online!
$endgroup$
$begingroup$
You can replacelength(z)
withsum(z|1)
to save 1 byte :)
$endgroup$
– Robert S.
Feb 13 at 17:09
$begingroup$
I don't understand how that works but I guess it does. sum(z|1) seems like it would always evaluate to at least 1, which would cause the while loop to loop endlessly. but apparently not
$endgroup$
– Sumner18
Feb 13 at 17:27
3
$begingroup$
z is a vector. each element of that vector is|
ed with 1. Which is always equal to 1. When you take the sum, you have a vector filled withTRUE
s so the result is equal to the length of the vector. If the vector is empty, you the is nothing to|
with so the output vector islogical(0)
. When you take that sum, it's 0
$endgroup$
– OganM
Feb 13 at 23:59
add a comment |
$begingroup$
R, 65 64 61 60 bytes
-1 byte thanks to Robert S.
-4 more thanks to digEmAll
x=scan();z=x:x[2];while(sum(z|1)){cat(z[1],"");z=rev(z[-1])}
Try it online!
$endgroup$
R, 65 64 61 60 bytes
-1 byte thanks to Robert S.
-4 more thanks to digEmAll
x=scan();z=x:x[2];while(sum(z|1)){cat(z[1],"");z=rev(z[-1])}
Try it online!
edited Feb 13 at 18:15
answered Feb 13 at 16:58


Sumner18Sumner18
5507
5507
$begingroup$
You can replacelength(z)
withsum(z|1)
to save 1 byte :)
$endgroup$
– Robert S.
Feb 13 at 17:09
$begingroup$
I don't understand how that works but I guess it does. sum(z|1) seems like it would always evaluate to at least 1, which would cause the while loop to loop endlessly. but apparently not
$endgroup$
– Sumner18
Feb 13 at 17:27
3
$begingroup$
z is a vector. each element of that vector is|
ed with 1. Which is always equal to 1. When you take the sum, you have a vector filled withTRUE
s so the result is equal to the length of the vector. If the vector is empty, you the is nothing to|
with so the output vector islogical(0)
. When you take that sum, it's 0
$endgroup$
– OganM
Feb 13 at 23:59
add a comment |
$begingroup$
You can replacelength(z)
withsum(z|1)
to save 1 byte :)
$endgroup$
– Robert S.
Feb 13 at 17:09
$begingroup$
I don't understand how that works but I guess it does. sum(z|1) seems like it would always evaluate to at least 1, which would cause the while loop to loop endlessly. but apparently not
$endgroup$
– Sumner18
Feb 13 at 17:27
3
$begingroup$
z is a vector. each element of that vector is|
ed with 1. Which is always equal to 1. When you take the sum, you have a vector filled withTRUE
s so the result is equal to the length of the vector. If the vector is empty, you the is nothing to|
with so the output vector islogical(0)
. When you take that sum, it's 0
$endgroup$
– OganM
Feb 13 at 23:59
$begingroup$
You can replace
length(z)
with sum(z|1)
to save 1 byte :)$endgroup$
– Robert S.
Feb 13 at 17:09
$begingroup$
You can replace
length(z)
with sum(z|1)
to save 1 byte :)$endgroup$
– Robert S.
Feb 13 at 17:09
$begingroup$
I don't understand how that works but I guess it does. sum(z|1) seems like it would always evaluate to at least 1, which would cause the while loop to loop endlessly. but apparently not
$endgroup$
– Sumner18
Feb 13 at 17:27
$begingroup$
I don't understand how that works but I guess it does. sum(z|1) seems like it would always evaluate to at least 1, which would cause the while loop to loop endlessly. but apparently not
$endgroup$
– Sumner18
Feb 13 at 17:27
3
3
$begingroup$
z is a vector. each element of that vector is
|
ed with 1. Which is always equal to 1. When you take the sum, you have a vector filled with TRUE
s so the result is equal to the length of the vector. If the vector is empty, you the is nothing to |
with so the output vector is logical(0)
. When you take that sum, it's 0$endgroup$
– OganM
Feb 13 at 23:59
$begingroup$
z is a vector. each element of that vector is
|
ed with 1. Which is always equal to 1. When you take the sum, you have a vector filled with TRUE
s so the result is equal to the length of the vector. If the vector is empty, you the is nothing to |
with so the output vector is logical(0)
. When you take that sum, it's 0$endgroup$
– OganM
Feb 13 at 23:59
add a comment |
$begingroup$
Python 2, 44 bytes
f=lambda a,b:[a]*(a==b)or[a]+f(b,a-cmp(a,b))
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 44 bytes
f=lambda a,b:[a]*(a==b)or[a]+f(b,a-cmp(a,b))
Try it online!
$endgroup$
add a comment |
$begingroup$
Python 2, 44 bytes
f=lambda a,b:[a]*(a==b)or[a]+f(b,a-cmp(a,b))
Try it online!
$endgroup$
Python 2, 44 bytes
f=lambda a,b:[a]*(a==b)or[a]+f(b,a-cmp(a,b))
Try it online!
answered Feb 13 at 16:58
ovsovs
19k21159
19k21159
add a comment |
add a comment |
$begingroup$
PowerShell, 59 48 bytes
param($a,$b)(($z=0..($b-$a))|%{$a+$_;$b-$_})[$z]
Try it online!
(Seems long...)
Takes input $a
and $b
, constructs the range 0 .. ($b-$a)
, stores that into $z
, then loops through that range. The looping through that range is just used as a counter to ensure we get enough iterations. Each iteration, we put $a
and $b
on the pipeline with addition/subtraction. That gives us something like 1,5,2,4,3,3,4,2,5,1
so we need to slice into that from 0
up to the $b-$a
(i.e., the count) of the original array so we're only left with the appropriate elements. That's left on the pipeline and output is implicit.
-11 bytes thanks to mazzy.
$endgroup$
$begingroup$
48 bytes
$endgroup$
– mazzy
Feb 13 at 17:29
$begingroup$
@mazzy Ah, I like that$b-$a
trick -- that's clever!
$endgroup$
– AdmBorkBork
Feb 13 at 17:40
add a comment |
$begingroup$
PowerShell, 59 48 bytes
param($a,$b)(($z=0..($b-$a))|%{$a+$_;$b-$_})[$z]
Try it online!
(Seems long...)
Takes input $a
and $b
, constructs the range 0 .. ($b-$a)
, stores that into $z
, then loops through that range. The looping through that range is just used as a counter to ensure we get enough iterations. Each iteration, we put $a
and $b
on the pipeline with addition/subtraction. That gives us something like 1,5,2,4,3,3,4,2,5,1
so we need to slice into that from 0
up to the $b-$a
(i.e., the count) of the original array so we're only left with the appropriate elements. That's left on the pipeline and output is implicit.
-11 bytes thanks to mazzy.
$endgroup$
$begingroup$
48 bytes
$endgroup$
– mazzy
Feb 13 at 17:29
$begingroup$
@mazzy Ah, I like that$b-$a
trick -- that's clever!
$endgroup$
– AdmBorkBork
Feb 13 at 17:40
add a comment |
$begingroup$
PowerShell, 59 48 bytes
param($a,$b)(($z=0..($b-$a))|%{$a+$_;$b-$_})[$z]
Try it online!
(Seems long...)
Takes input $a
and $b
, constructs the range 0 .. ($b-$a)
, stores that into $z
, then loops through that range. The looping through that range is just used as a counter to ensure we get enough iterations. Each iteration, we put $a
and $b
on the pipeline with addition/subtraction. That gives us something like 1,5,2,4,3,3,4,2,5,1
so we need to slice into that from 0
up to the $b-$a
(i.e., the count) of the original array so we're only left with the appropriate elements. That's left on the pipeline and output is implicit.
-11 bytes thanks to mazzy.
$endgroup$
PowerShell, 59 48 bytes
param($a,$b)(($z=0..($b-$a))|%{$a+$_;$b-$_})[$z]
Try it online!
(Seems long...)
Takes input $a
and $b
, constructs the range 0 .. ($b-$a)
, stores that into $z
, then loops through that range. The looping through that range is just used as a counter to ensure we get enough iterations. Each iteration, we put $a
and $b
on the pipeline with addition/subtraction. That gives us something like 1,5,2,4,3,3,4,2,5,1
so we need to slice into that from 0
up to the $b-$a
(i.e., the count) of the original array so we're only left with the appropriate elements. That's left on the pipeline and output is implicit.
-11 bytes thanks to mazzy.
edited Feb 13 at 17:40
answered Feb 13 at 15:51


AdmBorkBorkAdmBorkBork
27.2k466234
27.2k466234
$begingroup$
48 bytes
$endgroup$
– mazzy
Feb 13 at 17:29
$begingroup$
@mazzy Ah, I like that$b-$a
trick -- that's clever!
$endgroup$
– AdmBorkBork
Feb 13 at 17:40
add a comment |
$begingroup$
48 bytes
$endgroup$
– mazzy
Feb 13 at 17:29
$begingroup$
@mazzy Ah, I like that$b-$a
trick -- that's clever!
$endgroup$
– AdmBorkBork
Feb 13 at 17:40
$begingroup$
48 bytes
$endgroup$
– mazzy
Feb 13 at 17:29
$begingroup$
48 bytes
$endgroup$
– mazzy
Feb 13 at 17:29
$begingroup$
@mazzy Ah, I like that
$b-$a
trick -- that's clever!$endgroup$
– AdmBorkBork
Feb 13 at 17:40
$begingroup$
@mazzy Ah, I like that
$b-$a
trick -- that's clever!$endgroup$
– AdmBorkBork
Feb 13 at 17:40
add a comment |
$begingroup$
05AB1E, 6 bytes
ŸDvć,R
Try it online!
Explanation
Ÿ # push range [min ... max]
D # duplicate
v # for each element in the copy
ć, # extract and print the head of the original list
R # and then reverse it
$endgroup$
$begingroup$
Ÿ2ä`R.ι non iterative using interleave, but this is still much better.
$endgroup$
– Magic Octopus Urn
Feb 14 at 2:36
1
$begingroup$
@MagicOctopusUrn: I tried a non-iterative solution first, but it was even worse since I didn't know about.ι
;)
$endgroup$
– Emigna
Feb 14 at 7:36
$begingroup$
Similar as what I had in mind, so obvious +1 from me. I do like your alternative 7-byter as well through, @MagicOctopusUrn. :)
$endgroup$
– Kevin Cruijssen
Feb 14 at 8:28
1
$begingroup$
@KristianWilliams: Seems to be working for me.
$endgroup$
– Emigna
Feb 14 at 9:08
1
$begingroup$
@KevinCruijssen: I switched to a pair instead as that felt more intuitive anyways :)
$endgroup$
– Emigna
Feb 14 at 10:50
|
show 3 more comments
$begingroup$
05AB1E, 6 bytes
ŸDvć,R
Try it online!
Explanation
Ÿ # push range [min ... max]
D # duplicate
v # for each element in the copy
ć, # extract and print the head of the original list
R # and then reverse it
$endgroup$
$begingroup$
Ÿ2ä`R.ι non iterative using interleave, but this is still much better.
$endgroup$
– Magic Octopus Urn
Feb 14 at 2:36
1
$begingroup$
@MagicOctopusUrn: I tried a non-iterative solution first, but it was even worse since I didn't know about.ι
;)
$endgroup$
– Emigna
Feb 14 at 7:36
$begingroup$
Similar as what I had in mind, so obvious +1 from me. I do like your alternative 7-byter as well through, @MagicOctopusUrn. :)
$endgroup$
– Kevin Cruijssen
Feb 14 at 8:28
1
$begingroup$
@KristianWilliams: Seems to be working for me.
$endgroup$
– Emigna
Feb 14 at 9:08
1
$begingroup$
@KevinCruijssen: I switched to a pair instead as that felt more intuitive anyways :)
$endgroup$
– Emigna
Feb 14 at 10:50
|
show 3 more comments
$begingroup$
05AB1E, 6 bytes
ŸDvć,R
Try it online!
Explanation
Ÿ # push range [min ... max]
D # duplicate
v # for each element in the copy
ć, # extract and print the head of the original list
R # and then reverse it
$endgroup$
05AB1E, 6 bytes
ŸDvć,R
Try it online!
Explanation
Ÿ # push range [min ... max]
D # duplicate
v # for each element in the copy
ć, # extract and print the head of the original list
R # and then reverse it
edited Feb 14 at 10:36
answered Feb 13 at 17:02


EmignaEmigna
46.3k432141
46.3k432141
$begingroup$
Ÿ2ä`R.ι non iterative using interleave, but this is still much better.
$endgroup$
– Magic Octopus Urn
Feb 14 at 2:36
1
$begingroup$
@MagicOctopusUrn: I tried a non-iterative solution first, but it was even worse since I didn't know about.ι
;)
$endgroup$
– Emigna
Feb 14 at 7:36
$begingroup$
Similar as what I had in mind, so obvious +1 from me. I do like your alternative 7-byter as well through, @MagicOctopusUrn. :)
$endgroup$
– Kevin Cruijssen
Feb 14 at 8:28
1
$begingroup$
@KristianWilliams: Seems to be working for me.
$endgroup$
– Emigna
Feb 14 at 9:08
1
$begingroup$
@KevinCruijssen: I switched to a pair instead as that felt more intuitive anyways :)
$endgroup$
– Emigna
Feb 14 at 10:50
|
show 3 more comments
$begingroup$
Ÿ2ä`R.ι non iterative using interleave, but this is still much better.
$endgroup$
– Magic Octopus Urn
Feb 14 at 2:36
1
$begingroup$
@MagicOctopusUrn: I tried a non-iterative solution first, but it was even worse since I didn't know about.ι
;)
$endgroup$
– Emigna
Feb 14 at 7:36
$begingroup$
Similar as what I had in mind, so obvious +1 from me. I do like your alternative 7-byter as well through, @MagicOctopusUrn. :)
$endgroup$
– Kevin Cruijssen
Feb 14 at 8:28
1
$begingroup$
@KristianWilliams: Seems to be working for me.
$endgroup$
– Emigna
Feb 14 at 9:08
1
$begingroup$
@KevinCruijssen: I switched to a pair instead as that felt more intuitive anyways :)
$endgroup$
– Emigna
Feb 14 at 10:50
$begingroup$
Ÿ2ä`R.ι non iterative using interleave, but this is still much better.
$endgroup$
– Magic Octopus Urn
Feb 14 at 2:36
$begingroup$
Ÿ2ä`R.ι non iterative using interleave, but this is still much better.
$endgroup$
– Magic Octopus Urn
Feb 14 at 2:36
1
1
$begingroup$
@MagicOctopusUrn: I tried a non-iterative solution first, but it was even worse since I didn't know about
.ι
;)$endgroup$
– Emigna
Feb 14 at 7:36
$begingroup$
@MagicOctopusUrn: I tried a non-iterative solution first, but it was even worse since I didn't know about
.ι
;)$endgroup$
– Emigna
Feb 14 at 7:36
$begingroup$
Similar as what I had in mind, so obvious +1 from me. I do like your alternative 7-byter as well through, @MagicOctopusUrn. :)
$endgroup$
– Kevin Cruijssen
Feb 14 at 8:28
$begingroup$
Similar as what I had in mind, so obvious +1 from me. I do like your alternative 7-byter as well through, @MagicOctopusUrn. :)
$endgroup$
– Kevin Cruijssen
Feb 14 at 8:28
1
1
$begingroup$
@KristianWilliams: Seems to be working for me.
$endgroup$
– Emigna
Feb 14 at 9:08
$begingroup$
@KristianWilliams: Seems to be working for me.
$endgroup$
– Emigna
Feb 14 at 9:08
1
1
$begingroup$
@KevinCruijssen: I switched to a pair instead as that felt more intuitive anyways :)
$endgroup$
– Emigna
Feb 14 at 10:50
$begingroup$
@KevinCruijssen: I switched to a pair instead as that felt more intuitive anyways :)
$endgroup$
– Emigna
Feb 14 at 10:50
|
show 3 more comments
$begingroup$
Wolfram Language (Mathematica), 56 54 bytes
This is my first time golfing!
f[a_,b_]:=(c=a~Range~b;Drop[c~Riffle~Reverse@c,a-b-1])
Try it online!
Saved 2 bytes using infix notation.
Explanation:
f[a_,b_]:= function of two variables
c=a~Range~b; list of integers from a to b
Reverse@c same list in reverse
c~Riffle~Reverse@c interleave the two lists
Drop[c~Riffle~Reverse@c,a-b-1] drop last |a-b-1| elements (note a-b-1 < 0)
Alternatively, we could use Take[...,b-a+1]
for the same result.
Tests:
f[4, 6]
f[1, 5]
f[-1, 1]
f[-1, 2]
Ouput:
{4, 6, 5}
{1, 5, 2, 4, 3}
{-1, 1, 0}
{-1, 2, 0, 1}
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
The "Try it online" link returns a 403. "Sorry, you do not have permission to access this item."
$endgroup$
– Rohit Namjoshi
Feb 15 at 0:02
$begingroup$
@RohitNamjoshi I updated the link
$endgroup$
– Kai
Feb 15 at 0:24
$begingroup$
note that on TIO you can place header and footer code in the text boxes above and below the actual code box. This makes the code look cleaner, as well as allow you to take advantage the PPCG answer formatter (esc-s-g). Try it online!
$endgroup$
– Jo King
Feb 15 at 0:46
$begingroup$
@JoKing much appreciated, I had never used it before!
$endgroup$
– Kai
Feb 15 at 0:50
add a comment |
$begingroup$
Wolfram Language (Mathematica), 56 54 bytes
This is my first time golfing!
f[a_,b_]:=(c=a~Range~b;Drop[c~Riffle~Reverse@c,a-b-1])
Try it online!
Saved 2 bytes using infix notation.
Explanation:
f[a_,b_]:= function of two variables
c=a~Range~b; list of integers from a to b
Reverse@c same list in reverse
c~Riffle~Reverse@c interleave the two lists
Drop[c~Riffle~Reverse@c,a-b-1] drop last |a-b-1| elements (note a-b-1 < 0)
Alternatively, we could use Take[...,b-a+1]
for the same result.
Tests:
f[4, 6]
f[1, 5]
f[-1, 1]
f[-1, 2]
Ouput:
{4, 6, 5}
{1, 5, 2, 4, 3}
{-1, 1, 0}
{-1, 2, 0, 1}
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
$begingroup$
The "Try it online" link returns a 403. "Sorry, you do not have permission to access this item."
$endgroup$
– Rohit Namjoshi
Feb 15 at 0:02
$begingroup$
@RohitNamjoshi I updated the link
$endgroup$
– Kai
Feb 15 at 0:24
$begingroup$
note that on TIO you can place header and footer code in the text boxes above and below the actual code box. This makes the code look cleaner, as well as allow you to take advantage the PPCG answer formatter (esc-s-g). Try it online!
$endgroup$
– Jo King
Feb 15 at 0:46
$begingroup$
@JoKing much appreciated, I had never used it before!
$endgroup$
– Kai
Feb 15 at 0:50
add a comment |
$begingroup$
Wolfram Language (Mathematica), 56 54 bytes
This is my first time golfing!
f[a_,b_]:=(c=a~Range~b;Drop[c~Riffle~Reverse@c,a-b-1])
Try it online!
Saved 2 bytes using infix notation.
Explanation:
f[a_,b_]:= function of two variables
c=a~Range~b; list of integers from a to b
Reverse@c same list in reverse
c~Riffle~Reverse@c interleave the two lists
Drop[c~Riffle~Reverse@c,a-b-1] drop last |a-b-1| elements (note a-b-1 < 0)
Alternatively, we could use Take[...,b-a+1]
for the same result.
Tests:
f[4, 6]
f[1, 5]
f[-1, 1]
f[-1, 2]
Ouput:
{4, 6, 5}
{1, 5, 2, 4, 3}
{-1, 1, 0}
{-1, 2, 0, 1}
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
Wolfram Language (Mathematica), 56 54 bytes
This is my first time golfing!
f[a_,b_]:=(c=a~Range~b;Drop[c~Riffle~Reverse@c,a-b-1])
Try it online!
Saved 2 bytes using infix notation.
Explanation:
f[a_,b_]:= function of two variables
c=a~Range~b; list of integers from a to b
Reverse@c same list in reverse
c~Riffle~Reverse@c interleave the two lists
Drop[c~Riffle~Reverse@c,a-b-1] drop last |a-b-1| elements (note a-b-1 < 0)
Alternatively, we could use Take[...,b-a+1]
for the same result.
Tests:
f[4, 6]
f[1, 5]
f[-1, 1]
f[-1, 2]
Ouput:
{4, 6, 5}
{1, 5, 2, 4, 3}
{-1, 1, 0}
{-1, 2, 0, 1}
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited Feb 15 at 1:08
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered Feb 14 at 21:50


KaiKai
1713
1713
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Kai is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$begingroup$
The "Try it online" link returns a 403. "Sorry, you do not have permission to access this item."
$endgroup$
– Rohit Namjoshi
Feb 15 at 0:02
$begingroup$
@RohitNamjoshi I updated the link
$endgroup$
– Kai
Feb 15 at 0:24
$begingroup$
note that on TIO you can place header and footer code in the text boxes above and below the actual code box. This makes the code look cleaner, as well as allow you to take advantage the PPCG answer formatter (esc-s-g). Try it online!
$endgroup$
– Jo King
Feb 15 at 0:46
$begingroup$
@JoKing much appreciated, I had never used it before!
$endgroup$
– Kai
Feb 15 at 0:50
add a comment |
$begingroup$
The "Try it online" link returns a 403. "Sorry, you do not have permission to access this item."
$endgroup$
– Rohit Namjoshi
Feb 15 at 0:02
$begingroup$
@RohitNamjoshi I updated the link
$endgroup$
– Kai
Feb 15 at 0:24
$begingroup$
note that on TIO you can place header and footer code in the text boxes above and below the actual code box. This makes the code look cleaner, as well as allow you to take advantage the PPCG answer formatter (esc-s-g). Try it online!
$endgroup$
– Jo King
Feb 15 at 0:46
$begingroup$
@JoKing much appreciated, I had never used it before!
$endgroup$
– Kai
Feb 15 at 0:50
$begingroup$
The "Try it online" link returns a 403. "Sorry, you do not have permission to access this item."
$endgroup$
– Rohit Namjoshi
Feb 15 at 0:02
$begingroup$
The "Try it online" link returns a 403. "Sorry, you do not have permission to access this item."
$endgroup$
– Rohit Namjoshi
Feb 15 at 0:02
$begingroup$
@RohitNamjoshi I updated the link
$endgroup$
– Kai
Feb 15 at 0:24
$begingroup$
@RohitNamjoshi I updated the link
$endgroup$
– Kai
Feb 15 at 0:24
$begingroup$
note that on TIO you can place header and footer code in the text boxes above and below the actual code box. This makes the code look cleaner, as well as allow you to take advantage the PPCG answer formatter (esc-s-g). Try it online!
$endgroup$
– Jo King
Feb 15 at 0:46
$begingroup$
note that on TIO you can place header and footer code in the text boxes above and below the actual code box. This makes the code look cleaner, as well as allow you to take advantage the PPCG answer formatter (esc-s-g). Try it online!
$endgroup$
– Jo King
Feb 15 at 0:46
$begingroup$
@JoKing much appreciated, I had never used it before!
$endgroup$
– Kai
Feb 15 at 0:50
$begingroup$
@JoKing much appreciated, I had never used it before!
$endgroup$
– Kai
Feb 15 at 0:50
add a comment |
$begingroup$
Japt, 14 bytes
òV
íUs w)c vUl
Try it online!
$endgroup$
$begingroup$
13 bytes, using the same approach.
$endgroup$
– Shaggy
Feb 13 at 23:01
add a comment |
$begingroup$
Japt, 14 bytes
òV
íUs w)c vUl
Try it online!
$endgroup$
$begingroup$
13 bytes, using the same approach.
$endgroup$
– Shaggy
Feb 13 at 23:01
add a comment |
$begingroup$
Japt, 14 bytes
òV
íUs w)c vUl
Try it online!
$endgroup$
Japt, 14 bytes
òV
íUs w)c vUl
Try it online!
answered Feb 13 at 15:42


Luis felipe De jesus MunozLuis felipe De jesus Munoz
4,79721465
4,79721465
$begingroup$
13 bytes, using the same approach.
$endgroup$
– Shaggy
Feb 13 at 23:01
add a comment |
$begingroup$
13 bytes, using the same approach.
$endgroup$
– Shaggy
Feb 13 at 23:01
$begingroup$
13 bytes, using the same approach.
$endgroup$
– Shaggy
Feb 13 at 23:01
$begingroup$
13 bytes, using the same approach.
$endgroup$
– Shaggy
Feb 13 at 23:01
add a comment |
$begingroup$
Stax, 7 bytes
É╓ÅìΔà▲
Run and debug it
$endgroup$
add a comment |
$begingroup$
Stax, 7 bytes
É╓ÅìΔà▲
Run and debug it
$endgroup$
add a comment |
$begingroup$
Stax, 7 bytes
É╓ÅìΔà▲
Run and debug it
$endgroup$
Stax, 7 bytes
É╓ÅìΔà▲
Run and debug it
answered Feb 13 at 16:57
recursiverecursive
5,3091322
5,3091322
add a comment |
add a comment |
$begingroup$
cQuents, 19 bytes
#|B-A+1&A+k-1,B-k+1
Try it online!
Note that it does not work on TIO right now because TIO's interpreter is not up to date.
Explanation
#|B-A+1&A+k-1,B-k+1
A is the first input, B is the second input
#|B-A+1 n = B - A + 1
& Print the first n terms of the sequence
k starts at 1 and increments whenever we return to the first term
A+k-1, Terms alternate between A + k - 1 and
B-k+1 B - k + 1
increment k
$endgroup$
add a comment |
$begingroup$
cQuents, 19 bytes
#|B-A+1&A+k-1,B-k+1
Try it online!
Note that it does not work on TIO right now because TIO's interpreter is not up to date.
Explanation
#|B-A+1&A+k-1,B-k+1
A is the first input, B is the second input
#|B-A+1 n = B - A + 1
& Print the first n terms of the sequence
k starts at 1 and increments whenever we return to the first term
A+k-1, Terms alternate between A + k - 1 and
B-k+1 B - k + 1
increment k
$endgroup$
add a comment |
$begingroup$
cQuents, 19 bytes
#|B-A+1&A+k-1,B-k+1
Try it online!
Note that it does not work on TIO right now because TIO's interpreter is not up to date.
Explanation
#|B-A+1&A+k-1,B-k+1
A is the first input, B is the second input
#|B-A+1 n = B - A + 1
& Print the first n terms of the sequence
k starts at 1 and increments whenever we return to the first term
A+k-1, Terms alternate between A + k - 1 and
B-k+1 B - k + 1
increment k
$endgroup$
cQuents, 19 bytes
#|B-A+1&A+k-1,B-k+1
Try it online!
Note that it does not work on TIO right now because TIO's interpreter is not up to date.
Explanation
#|B-A+1&A+k-1,B-k+1
A is the first input, B is the second input
#|B-A+1 n = B - A + 1
& Print the first n terms of the sequence
k starts at 1 and increments whenever we return to the first term
A+k-1, Terms alternate between A + k - 1 and
B-k+1 B - k + 1
increment k
answered Feb 13 at 18:21


StephenStephen
7,34823295
7,34823295
add a comment |
add a comment |
$begingroup$
Haskell, 39 bytes
f(a:b)=a:f(reverse b)
f x=x
a#b=f[a..b]
Try it online!
$endgroup$
add a comment |
$begingroup$
Haskell, 39 bytes
f(a:b)=a:f(reverse b)
f x=x
a#b=f[a..b]
Try it online!
$endgroup$
add a comment |
$begingroup$
Haskell, 39 bytes
f(a:b)=a:f(reverse b)
f x=x
a#b=f[a..b]
Try it online!
$endgroup$
Haskell, 39 bytes
f(a:b)=a:f(reverse b)
f x=x
a#b=f[a..b]
Try it online!
answered Feb 13 at 19:09
niminimi
31.8k32285
31.8k32285
add a comment |
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 46 bytes
a=>b=>{for(;a<=b;Write(a+(b>a++?b--+"":"")));}
Saved 4 bytes thanks to dana!
Try it online!
C# (Visual C# Interactive Compiler), 65 bytes
void z(int a,int b){if(a<=b){Write(a+(b>a?b+"":""));z(a+1,b-1);}}
Try it online!
$endgroup$
$begingroup$
I didn't notice the extra empty string there, thanks!
$endgroup$
– Embodiment of Ignorance
Feb 14 at 16:23
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 46 bytes
a=>b=>{for(;a<=b;Write(a+(b>a++?b--+"":"")));}
Saved 4 bytes thanks to dana!
Try it online!
C# (Visual C# Interactive Compiler), 65 bytes
void z(int a,int b){if(a<=b){Write(a+(b>a?b+"":""));z(a+1,b-1);}}
Try it online!
$endgroup$
$begingroup$
I didn't notice the extra empty string there, thanks!
$endgroup$
– Embodiment of Ignorance
Feb 14 at 16:23
add a comment |
$begingroup$
C# (Visual C# Interactive Compiler), 46 bytes
a=>b=>{for(;a<=b;Write(a+(b>a++?b--+"":"")));}
Saved 4 bytes thanks to dana!
Try it online!
C# (Visual C# Interactive Compiler), 65 bytes
void z(int a,int b){if(a<=b){Write(a+(b>a?b+"":""));z(a+1,b-1);}}
Try it online!
$endgroup$
C# (Visual C# Interactive Compiler), 46 bytes
a=>b=>{for(;a<=b;Write(a+(b>a++?b--+"":"")));}
Saved 4 bytes thanks to dana!
Try it online!
C# (Visual C# Interactive Compiler), 65 bytes
void z(int a,int b){if(a<=b){Write(a+(b>a?b+"":""));z(a+1,b-1);}}
Try it online!
edited Feb 14 at 16:25
answered Feb 13 at 16:50
Embodiment of IgnoranceEmbodiment of Ignorance
1,100119
1,100119
$begingroup$
I didn't notice the extra empty string there, thanks!
$endgroup$
– Embodiment of Ignorance
Feb 14 at 16:23
add a comment |
$begingroup$
I didn't notice the extra empty string there, thanks!
$endgroup$
– Embodiment of Ignorance
Feb 14 at 16:23
$begingroup$
I didn't notice the extra empty string there, thanks!
$endgroup$
– Embodiment of Ignorance
Feb 14 at 16:23
$begingroup$
I didn't notice the extra empty string there, thanks!
$endgroup$
– Embodiment of Ignorance
Feb 14 at 16:23
add a comment |
$begingroup$
APL (dzaima/APL), 21 bytes
⌈⊢+.5×-+∘(⌽ׯ1*)∘⍳1+-
Try it online!
$endgroup$
add a comment |
$begingroup$
APL (dzaima/APL), 21 bytes
⌈⊢+.5×-+∘(⌽ׯ1*)∘⍳1+-
Try it online!
$endgroup$
add a comment |
$begingroup$
APL (dzaima/APL), 21 bytes
⌈⊢+.5×-+∘(⌽ׯ1*)∘⍳1+-
Try it online!
$endgroup$
APL (dzaima/APL), 21 bytes
⌈⊢+.5×-+∘(⌽ׯ1*)∘⍳1+-
Try it online!
answered Feb 13 at 16:03


dzaimadzaima
15.1k21856
15.1k21856
add a comment |
add a comment |
$begingroup$
Japt, 7 bytes
Takes input as an array.
rõ
ÊÆÔv
Try it or run all test cases
:Implicit input of array U=[low,high]
r :Reduce by
õ : Inclusive, reversed range (giving the range [high,low])
n :Reassign to U
Ê :Length
Æ :Map the range [0,Ê)
Ô : Reverse U
v : Remove the first element
$endgroup$
add a comment |
$begingroup$
Japt, 7 bytes
Takes input as an array.
rõ
ÊÆÔv
Try it or run all test cases
:Implicit input of array U=[low,high]
r :Reduce by
õ : Inclusive, reversed range (giving the range [high,low])
n :Reassign to U
Ê :Length
Æ :Map the range [0,Ê)
Ô : Reverse U
v : Remove the first element
$endgroup$
add a comment |
$begingroup$
Japt, 7 bytes
Takes input as an array.
rõ
ÊÆÔv
Try it or run all test cases
:Implicit input of array U=[low,high]
r :Reduce by
õ : Inclusive, reversed range (giving the range [high,low])
n :Reassign to U
Ê :Length
Æ :Map the range [0,Ê)
Ô : Reverse U
v : Remove the first element
$endgroup$
Japt, 7 bytes
Takes input as an array.
rõ
ÊÆÔv
Try it or run all test cases
:Implicit input of array U=[low,high]
r :Reduce by
õ : Inclusive, reversed range (giving the range [high,low])
n :Reassign to U
Ê :Length
Æ :Map the range [0,Ê)
Ô : Reverse U
v : Remove the first element
edited Feb 13 at 17:31
answered Feb 13 at 17:20


ShaggyShaggy
20.1k21667
20.1k21667
add a comment |
add a comment |
$begingroup$
R, 51 bytes
function(x,y,z=x:y)matrix(c(z,rev(z)),2,,T)[seq(z)]
Try it online!
Explanation:
For a sequence x:y
of length N
, create a two-by-N matrix consisting of the sequence x:y in the top row and y:x in the bottom row matrix(c(z,rev(z)),2,,T)
. If we select the first N
elements of the matrix [seq(z)]
, they will be chosen by column, giving the required output.
Outgolfed by digEmAll
$endgroup$
1
$begingroup$
I just posted a very similar approach 30 seconds before you :D
$endgroup$
– digEmAll
Feb 13 at 18:01
$begingroup$
@digEmAll Yes, but yours is a lot better!
$endgroup$
– user2390246
Feb 13 at 18:03
add a comment |
$begingroup$
R, 51 bytes
function(x,y,z=x:y)matrix(c(z,rev(z)),2,,T)[seq(z)]
Try it online!
Explanation:
For a sequence x:y
of length N
, create a two-by-N matrix consisting of the sequence x:y in the top row and y:x in the bottom row matrix(c(z,rev(z)),2,,T)
. If we select the first N
elements of the matrix [seq(z)]
, they will be chosen by column, giving the required output.
Outgolfed by digEmAll
$endgroup$
1
$begingroup$
I just posted a very similar approach 30 seconds before you :D
$endgroup$
– digEmAll
Feb 13 at 18:01
$begingroup$
@digEmAll Yes, but yours is a lot better!
$endgroup$
– user2390246
Feb 13 at 18:03
add a comment |
$begingroup$
R, 51 bytes
function(x,y,z=x:y)matrix(c(z,rev(z)),2,,T)[seq(z)]
Try it online!
Explanation:
For a sequence x:y
of length N
, create a two-by-N matrix consisting of the sequence x:y in the top row and y:x in the bottom row matrix(c(z,rev(z)),2,,T)
. If we select the first N
elements of the matrix [seq(z)]
, they will be chosen by column, giving the required output.
Outgolfed by digEmAll
$endgroup$
R, 51 bytes
function(x,y,z=x:y)matrix(c(z,rev(z)),2,,T)[seq(z)]
Try it online!
Explanation:
For a sequence x:y
of length N
, create a two-by-N matrix consisting of the sequence x:y in the top row and y:x in the bottom row matrix(c(z,rev(z)),2,,T)
. If we select the first N
elements of the matrix [seq(z)]
, they will be chosen by column, giving the required output.
Outgolfed by digEmAll
edited Feb 13 at 18:04
answered Feb 13 at 17:59
user2390246user2390246
1,264111
1,264111
1
$begingroup$
I just posted a very similar approach 30 seconds before you :D
$endgroup$
– digEmAll
Feb 13 at 18:01
$begingroup$
@digEmAll Yes, but yours is a lot better!
$endgroup$
– user2390246
Feb 13 at 18:03
add a comment |
1
$begingroup$
I just posted a very similar approach 30 seconds before you :D
$endgroup$
– digEmAll
Feb 13 at 18:01
$begingroup$
@digEmAll Yes, but yours is a lot better!
$endgroup$
– user2390246
Feb 13 at 18:03
1
1
$begingroup$
I just posted a very similar approach 30 seconds before you :D
$endgroup$
– digEmAll
Feb 13 at 18:01
$begingroup$
I just posted a very similar approach 30 seconds before you :D
$endgroup$
– digEmAll
Feb 13 at 18:01
$begingroup$
@digEmAll Yes, but yours is a lot better!
$endgroup$
– user2390246
Feb 13 at 18:03
$begingroup$
@digEmAll Yes, but yours is a lot better!
$endgroup$
– user2390246
Feb 13 at 18:03
add a comment |
$begingroup$
MATL, 8 bytes
&:t"1&)P
Try it online!
Explanation
&: % Take two inputs (implicit). Two-input range
t % Duplicate
" % For each
1&) % Push first element, then an array with the rest
P % Reverse array
% End (implicit). Display (implicit)
$endgroup$
add a comment |
$begingroup$
MATL, 8 bytes
&:t"1&)P
Try it online!
Explanation
&: % Take two inputs (implicit). Two-input range
t % Duplicate
" % For each
1&) % Push first element, then an array with the rest
P % Reverse array
% End (implicit). Display (implicit)
$endgroup$
add a comment |
$begingroup$
MATL, 8 bytes
&:t"1&)P
Try it online!
Explanation
&: % Take two inputs (implicit). Two-input range
t % Duplicate
" % For each
1&) % Push first element, then an array with the rest
P % Reverse array
% End (implicit). Display (implicit)
$endgroup$
MATL, 8 bytes
&:t"1&)P
Try it online!
Explanation
&: % Take two inputs (implicit). Two-input range
t % Duplicate
" % For each
1&) % Push first element, then an array with the rest
P % Reverse array
% End (implicit). Display (implicit)
edited Feb 13 at 18:11
answered Feb 13 at 17:57


Luis MendoLuis Mendo
74.5k888291
74.5k888291
add a comment |
add a comment |
$begingroup$
JavaScript, 40 bytes
l=>g=h=>h>l?[l++,h--,...g(h)]:h<l?:[l]
Try It Online!
$endgroup$
add a comment |
$begingroup$
JavaScript, 40 bytes
l=>g=h=>h>l?[l++,h--,...g(h)]:h<l?:[l]
Try It Online!
$endgroup$
add a comment |
$begingroup$
JavaScript, 40 bytes
l=>g=h=>h>l?[l++,h--,...g(h)]:h<l?:[l]
Try It Online!
$endgroup$
JavaScript, 40 bytes
l=>g=h=>h>l?[l++,h--,...g(h)]:h<l?:[l]
Try It Online!
edited Feb 13 at 20:13
answered Feb 13 at 18:13


ShaggyShaggy
20.1k21667
20.1k21667
add a comment |
add a comment |
$begingroup$
Forth (gforth), 52 bytes
: f 2dup - 1+ 0 do dup . i 2 mod 2* 1- - swap loop ;
Try it online!
Explanation
Loop from 0 to (End - Start). Place End and Start on top of the stack.
Each Iteration:
- Output the current number
- Add (or subtract) 1 from the current number
- Switch the current number with the other number
Code Explanation
: f start new word definition
2dup - get the size of the range (total number of integers)
1+ 0 add 1 to the size because forth loops are [Inclusive, Exclusive)
do start counted loop from 0 to size+1
dup . output the current top of the stack
i 2 mod get the index of the loop modulus 2
2* 1- convert from 0,1 to -1,1
- subtract result from top of stack (adds 1 to lower bound and subtracts 1 from upper)
swap swap the top two stack numbers
loop end the counted loop
; end the word definition
$endgroup$
add a comment |
$begingroup$
Forth (gforth), 52 bytes
: f 2dup - 1+ 0 do dup . i 2 mod 2* 1- - swap loop ;
Try it online!
Explanation
Loop from 0 to (End - Start). Place End and Start on top of the stack.
Each Iteration:
- Output the current number
- Add (or subtract) 1 from the current number
- Switch the current number with the other number
Code Explanation
: f start new word definition
2dup - get the size of the range (total number of integers)
1+ 0 add 1 to the size because forth loops are [Inclusive, Exclusive)
do start counted loop from 0 to size+1
dup . output the current top of the stack
i 2 mod get the index of the loop modulus 2
2* 1- convert from 0,1 to -1,1
- subtract result from top of stack (adds 1 to lower bound and subtracts 1 from upper)
swap swap the top two stack numbers
loop end the counted loop
; end the word definition
$endgroup$
add a comment |
$begingroup$
Forth (gforth), 52 bytes
: f 2dup - 1+ 0 do dup . i 2 mod 2* 1- - swap loop ;
Try it online!
Explanation
Loop from 0 to (End - Start). Place End and Start on top of the stack.
Each Iteration:
- Output the current number
- Add (or subtract) 1 from the current number
- Switch the current number with the other number
Code Explanation
: f start new word definition
2dup - get the size of the range (total number of integers)
1+ 0 add 1 to the size because forth loops are [Inclusive, Exclusive)
do start counted loop from 0 to size+1
dup . output the current top of the stack
i 2 mod get the index of the loop modulus 2
2* 1- convert from 0,1 to -1,1
- subtract result from top of stack (adds 1 to lower bound and subtracts 1 from upper)
swap swap the top two stack numbers
loop end the counted loop
; end the word definition
$endgroup$
Forth (gforth), 52 bytes
: f 2dup - 1+ 0 do dup . i 2 mod 2* 1- - swap loop ;
Try it online!
Explanation
Loop from 0 to (End - Start). Place End and Start on top of the stack.
Each Iteration:
- Output the current number
- Add (or subtract) 1 from the current number
- Switch the current number with the other number
Code Explanation
: f start new word definition
2dup - get the size of the range (total number of integers)
1+ 0 add 1 to the size because forth loops are [Inclusive, Exclusive)
do start counted loop from 0 to size+1
dup . output the current top of the stack
i 2 mod get the index of the loop modulus 2
2* 1- convert from 0,1 to -1,1
- subtract result from top of stack (adds 1 to lower bound and subtracts 1 from upper)
swap swap the top two stack numbers
loop end the counted loop
; end the word definition
edited Feb 13 at 20:23
answered Feb 13 at 18:02
reffureffu
61126
61126
add a comment |
add a comment |
$begingroup$
Julia 0.7, 29 bytes
f(a,b)=[a:b b:-1:a]'[1:1+b-a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Julia 0.7, 29 bytes
f(a,b)=[a:b b:-1:a]'[1:1+b-a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Julia 0.7, 29 bytes
f(a,b)=[a:b b:-1:a]'[1:1+b-a]
Try it online!
$endgroup$
Julia 0.7, 29 bytes
f(a,b)=[a:b b:-1:a]'[1:1+b-a]
Try it online!
answered Feb 13 at 20:30
Kirill L.Kirill L.
4,4151523
4,4151523
add a comment |
add a comment |
$begingroup$
Haskell, 30 bytes
l%h=l:take(h-l)(h:(l+1)%(h-1))
Usage: 3%7
gives `[3,7,4,6,5]
For the inputs l, h
the function calls recursively with the inputs l+1, h-1
, and adds l,h
to the beggining.
Instead of any halting condition, the code uses take(h-l)
to shorten the sequence to the right length (which would otherwise be an infinite sequence of increasing and decreasing numbers).
$endgroup$
add a comment |
$begingroup$
Haskell, 30 bytes
l%h=l:take(h-l)(h:(l+1)%(h-1))
Usage: 3%7
gives `[3,7,4,6,5]
For the inputs l, h
the function calls recursively with the inputs l+1, h-1
, and adds l,h
to the beggining.
Instead of any halting condition, the code uses take(h-l)
to shorten the sequence to the right length (which would otherwise be an infinite sequence of increasing and decreasing numbers).
$endgroup$
add a comment |
$begingroup$
Haskell, 30 bytes
l%h=l:take(h-l)(h:(l+1)%(h-1))
Usage: 3%7
gives `[3,7,4,6,5]
For the inputs l, h
the function calls recursively with the inputs l+1, h-1
, and adds l,h
to the beggining.
Instead of any halting condition, the code uses take(h-l)
to shorten the sequence to the right length (which would otherwise be an infinite sequence of increasing and decreasing numbers).
$endgroup$
Haskell, 30 bytes
l%h=l:take(h-l)(h:(l+1)%(h-1))
Usage: 3%7
gives `[3,7,4,6,5]
For the inputs l, h
the function calls recursively with the inputs l+1, h-1
, and adds l,h
to the beggining.
Instead of any halting condition, the code uses take(h-l)
to shorten the sequence to the right length (which would otherwise be an infinite sequence of increasing and decreasing numbers).
answered Feb 14 at 13:48
proud haskellerproud haskeller
5,71611536
5,71611536
add a comment |
add a comment |
$begingroup$
JVM bytecode (OpenJDK asmtools JASM), 449 bytes
enum b{const #1=Method java/io/PrintStream.print:(I)V;static Method a:(II)V stack 2 locals 4{getstatic java/lang/System.out:"Ljava/io/PrintStream;";astore 3;ldc 0;istore 2;l:iload 2;ldc 1;if_icmpeq t;aload 3;iload 0;invokevirtual #1;iinc 0,1;iinc 2,1;goto c;t:aload 3;iload 1;invokevirtual #1;iinc 1,-1;iinc 2,-1;c:aload 3;ldc 32;i2c;invokevirtual java/io/PrintStream.print:(C)V;iload 0;iload 1;if_icmpne l;aload 3;iload 0;invokevirtual #1;return;}}
Ungolfed (and slightly cleaner)
enum b {
public static Method "a":(II)V stack 5 locals 4 {
getstatic "java/lang/System"."out":"Ljava/io/PrintStream;";
astore 3;
ldc 0;
istore 2;
loop:
iload 2;
ldc 1;
if_icmpeq true;
false:
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 0,1;
iinc 2,1;
goto cond;
true:
aload 3;
iload 1;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 1,-1;
iinc 2,-1;
goto cond;
cond:
iload 0;
iload 1;
if_icmpne loop;
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
return;
}
}
Standalone function, needs to be called from Java as b.a(num1,num2)
.
Explanation
This code uses the method parameters as variables, as well as a boolean in local #3 deciding which number to output. Each loop iteration either the left or right is output, and that number is incremented for the left or decremented for the right. Loop continues until both numbers are equal, then that number is output.
...I have a distinct feeling I'm massively outgunned on the byte count
$endgroup$
add a comment |
$begingroup$
JVM bytecode (OpenJDK asmtools JASM), 449 bytes
enum b{const #1=Method java/io/PrintStream.print:(I)V;static Method a:(II)V stack 2 locals 4{getstatic java/lang/System.out:"Ljava/io/PrintStream;";astore 3;ldc 0;istore 2;l:iload 2;ldc 1;if_icmpeq t;aload 3;iload 0;invokevirtual #1;iinc 0,1;iinc 2,1;goto c;t:aload 3;iload 1;invokevirtual #1;iinc 1,-1;iinc 2,-1;c:aload 3;ldc 32;i2c;invokevirtual java/io/PrintStream.print:(C)V;iload 0;iload 1;if_icmpne l;aload 3;iload 0;invokevirtual #1;return;}}
Ungolfed (and slightly cleaner)
enum b {
public static Method "a":(II)V stack 5 locals 4 {
getstatic "java/lang/System"."out":"Ljava/io/PrintStream;";
astore 3;
ldc 0;
istore 2;
loop:
iload 2;
ldc 1;
if_icmpeq true;
false:
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 0,1;
iinc 2,1;
goto cond;
true:
aload 3;
iload 1;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 1,-1;
iinc 2,-1;
goto cond;
cond:
iload 0;
iload 1;
if_icmpne loop;
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
return;
}
}
Standalone function, needs to be called from Java as b.a(num1,num2)
.
Explanation
This code uses the method parameters as variables, as well as a boolean in local #3 deciding which number to output. Each loop iteration either the left or right is output, and that number is incremented for the left or decremented for the right. Loop continues until both numbers are equal, then that number is output.
...I have a distinct feeling I'm massively outgunned on the byte count
$endgroup$
add a comment |
$begingroup$
JVM bytecode (OpenJDK asmtools JASM), 449 bytes
enum b{const #1=Method java/io/PrintStream.print:(I)V;static Method a:(II)V stack 2 locals 4{getstatic java/lang/System.out:"Ljava/io/PrintStream;";astore 3;ldc 0;istore 2;l:iload 2;ldc 1;if_icmpeq t;aload 3;iload 0;invokevirtual #1;iinc 0,1;iinc 2,1;goto c;t:aload 3;iload 1;invokevirtual #1;iinc 1,-1;iinc 2,-1;c:aload 3;ldc 32;i2c;invokevirtual java/io/PrintStream.print:(C)V;iload 0;iload 1;if_icmpne l;aload 3;iload 0;invokevirtual #1;return;}}
Ungolfed (and slightly cleaner)
enum b {
public static Method "a":(II)V stack 5 locals 4 {
getstatic "java/lang/System"."out":"Ljava/io/PrintStream;";
astore 3;
ldc 0;
istore 2;
loop:
iload 2;
ldc 1;
if_icmpeq true;
false:
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 0,1;
iinc 2,1;
goto cond;
true:
aload 3;
iload 1;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 1,-1;
iinc 2,-1;
goto cond;
cond:
iload 0;
iload 1;
if_icmpne loop;
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
return;
}
}
Standalone function, needs to be called from Java as b.a(num1,num2)
.
Explanation
This code uses the method parameters as variables, as well as a boolean in local #3 deciding which number to output. Each loop iteration either the left or right is output, and that number is incremented for the left or decremented for the right. Loop continues until both numbers are equal, then that number is output.
...I have a distinct feeling I'm massively outgunned on the byte count
$endgroup$
JVM bytecode (OpenJDK asmtools JASM), 449 bytes
enum b{const #1=Method java/io/PrintStream.print:(I)V;static Method a:(II)V stack 2 locals 4{getstatic java/lang/System.out:"Ljava/io/PrintStream;";astore 3;ldc 0;istore 2;l:iload 2;ldc 1;if_icmpeq t;aload 3;iload 0;invokevirtual #1;iinc 0,1;iinc 2,1;goto c;t:aload 3;iload 1;invokevirtual #1;iinc 1,-1;iinc 2,-1;c:aload 3;ldc 32;i2c;invokevirtual java/io/PrintStream.print:(C)V;iload 0;iload 1;if_icmpne l;aload 3;iload 0;invokevirtual #1;return;}}
Ungolfed (and slightly cleaner)
enum b {
public static Method "a":(II)V stack 5 locals 4 {
getstatic "java/lang/System"."out":"Ljava/io/PrintStream;";
astore 3;
ldc 0;
istore 2;
loop:
iload 2;
ldc 1;
if_icmpeq true;
false:
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 0,1;
iinc 2,1;
goto cond;
true:
aload 3;
iload 1;
invokevirtual "java/io/PrintStream"."print":"(I)V";
iinc 1,-1;
iinc 2,-1;
goto cond;
cond:
iload 0;
iload 1;
if_icmpne loop;
aload 3;
iload 0;
invokevirtual "java/io/PrintStream"."print":"(I)V";
return;
}
}
Standalone function, needs to be called from Java as b.a(num1,num2)
.
Explanation
This code uses the method parameters as variables, as well as a boolean in local #3 deciding which number to output. Each loop iteration either the left or right is output, and that number is incremented for the left or decremented for the right. Loop continues until both numbers are equal, then that number is output.
...I have a distinct feeling I'm massively outgunned on the byte count
answered Feb 14 at 22:30


Archduke LiamusArchduke Liamus
1412
1412
add a comment |
add a comment |
$begingroup$
Ink, 46 bytes
=h(I,A)
{I<=A:{I} {I<A:{A} ->h(I+1,A-1)}}->->
(I don't think there's an online interpreter for Ink, sorry)
Defines a stitch called h, which takes two arguments I and A, which are the bounds of the range.
Outputs by printing values, separated by spaces, to stdout.
Explanation
=h(min, max) // Define the stitch.
{min <= max:{min}/* print min unless it's greater than max */{min < max: {max} /*Also print max if it's greater than min*/->h(min+1, max-1)/*Then divert, with the arguments changed*/}}
->-> // If we didn't divert earlier, divert to wherever the stitch was called from
$endgroup$
add a comment |
$begingroup$
Ink, 46 bytes
=h(I,A)
{I<=A:{I} {I<A:{A} ->h(I+1,A-1)}}->->
(I don't think there's an online interpreter for Ink, sorry)
Defines a stitch called h, which takes two arguments I and A, which are the bounds of the range.
Outputs by printing values, separated by spaces, to stdout.
Explanation
=h(min, max) // Define the stitch.
{min <= max:{min}/* print min unless it's greater than max */{min < max: {max} /*Also print max if it's greater than min*/->h(min+1, max-1)/*Then divert, with the arguments changed*/}}
->-> // If we didn't divert earlier, divert to wherever the stitch was called from
$endgroup$
add a comment |
$begingroup$
Ink, 46 bytes
=h(I,A)
{I<=A:{I} {I<A:{A} ->h(I+1,A-1)}}->->
(I don't think there's an online interpreter for Ink, sorry)
Defines a stitch called h, which takes two arguments I and A, which are the bounds of the range.
Outputs by printing values, separated by spaces, to stdout.
Explanation
=h(min, max) // Define the stitch.
{min <= max:{min}/* print min unless it's greater than max */{min < max: {max} /*Also print max if it's greater than min*/->h(min+1, max-1)/*Then divert, with the arguments changed*/}}
->-> // If we didn't divert earlier, divert to wherever the stitch was called from
$endgroup$
Ink, 46 bytes
=h(I,A)
{I<=A:{I} {I<A:{A} ->h(I+1,A-1)}}->->
(I don't think there's an online interpreter for Ink, sorry)
Defines a stitch called h, which takes two arguments I and A, which are the bounds of the range.
Outputs by printing values, separated by spaces, to stdout.
Explanation
=h(min, max) // Define the stitch.
{min <= max:{min}/* print min unless it's greater than max */{min < max: {max} /*Also print max if it's greater than min*/->h(min+1, max-1)/*Then divert, with the arguments changed*/}}
->-> // If we didn't divert earlier, divert to wherever the stitch was called from
answered Feb 13 at 19:01
Sara JSara J
613
613
add a comment |
add a comment |
$begingroup$
Perl 5 -ln
, 37 bytes
@.=$_..<>;say shift@.,$/,pop@.while@.
Try it online!
$endgroup$
$begingroup$
33bytes
$endgroup$
– Nahuel Fouilleul
Feb 14 at 14:47
add a comment |
$begingroup$
Perl 5 -ln
, 37 bytes
@.=$_..<>;say shift@.,$/,pop@.while@.
Try it online!
$endgroup$
$begingroup$
33bytes
$endgroup$
– Nahuel Fouilleul
Feb 14 at 14:47
add a comment |
$begingroup$
Perl 5 -ln
, 37 bytes
@.=$_..<>;say shift@.,$/,pop@.while@.
Try it online!
$endgroup$
Perl 5 -ln
, 37 bytes
@.=$_..<>;say shift@.,$/,pop@.while@.
Try it online!
answered Feb 13 at 20:02
XcaliXcali
5,335520
5,335520
$begingroup$
33bytes
$endgroup$
– Nahuel Fouilleul
Feb 14 at 14:47
add a comment |
$begingroup$
33bytes
$endgroup$
– Nahuel Fouilleul
Feb 14 at 14:47
$begingroup$
33bytes
$endgroup$
– Nahuel Fouilleul
Feb 14 at 14:47
$begingroup$
33bytes
$endgroup$
– Nahuel Fouilleul
Feb 14 at 14:47
add a comment |
$begingroup$
Java (JDK), 52 bytes
(l,h,o)->{for(int i=0;l<=h;i^=1)o.add(i<1?l++:h--);}
Try it online!
$endgroup$
add a comment |
$begingroup$
Java (JDK), 52 bytes
(l,h,o)->{for(int i=0;l<=h;i^=1)o.add(i<1?l++:h--);}
Try it online!
$endgroup$
add a comment |
$begingroup$
Java (JDK), 52 bytes
(l,h,o)->{for(int i=0;l<=h;i^=1)o.add(i<1?l++:h--);}
Try it online!
$endgroup$
Java (JDK), 52 bytes
(l,h,o)->{for(int i=0;l<=h;i^=1)o.add(i<1?l++:h--);}
Try it online!
edited Feb 13 at 20:44
answered Feb 13 at 17:25


Olivier GrégoireOlivier Grégoire
9,10511943
9,10511943
add a comment |
add a comment |
$begingroup$
Clean, 48 bytes
import StdEnv
$a b|a<>b=[a: $b(a+sign(b-a))]=[a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Clean, 48 bytes
import StdEnv
$a b|a<>b=[a: $b(a+sign(b-a))]=[a]
Try it online!
$endgroup$
add a comment |
$begingroup$
Clean, 48 bytes
import StdEnv
$a b|a<>b=[a: $b(a+sign(b-a))]=[a]
Try it online!
$endgroup$
Clean, 48 bytes
import StdEnv
$a b|a<>b=[a: $b(a+sign(b-a))]=[a]
Try it online!
answered Feb 14 at 1:29
ΟurousΟurous
7,12111035
7,12111035
add a comment |
add a comment |
$begingroup$
Ruby, 37 36 33 bytes
f=->a,b{a>b ?:[a,b]|f[a+1,b-1]}
Try it online!
Recursive version with 3 bytes saved by G B.
Ruby, 38 bytes
->a,b{d=*c=a..b;c.map{d.reverse!.pop}}
Try it online!
Non-recursive version.
$endgroup$
add a comment |
$begingroup$
Ruby, 37 36 33 bytes
f=->a,b{a>b ?:[a,b]|f[a+1,b-1]}
Try it online!
Recursive version with 3 bytes saved by G B.
Ruby, 38 bytes
->a,b{d=*c=a..b;c.map{d.reverse!.pop}}
Try it online!
Non-recursive version.
$endgroup$
add a comment |
$begingroup$
Ruby, 37 36 33 bytes
f=->a,b{a>b ?:[a,b]|f[a+1,b-1]}
Try it online!
Recursive version with 3 bytes saved by G B.
Ruby, 38 bytes
->a,b{d=*c=a..b;c.map{d.reverse!.pop}}
Try it online!
Non-recursive version.
$endgroup$
Ruby, 37 36 33 bytes
f=->a,b{a>b ?:[a,b]|f[a+1,b-1]}
Try it online!
Recursive version with 3 bytes saved by G B.
Ruby, 38 bytes
->a,b{d=*c=a..b;c.map{d.reverse!.pop}}
Try it online!
Non-recursive version.
edited Feb 14 at 15:11
answered Feb 14 at 10:56
Kirill L.Kirill L.
4,4151523
4,4151523
add a comment |
add a comment |
$begingroup$
Cubix, 16 bytes
;w(.II>sO-?@;)^/
Try it here
Cubified
; w
( .
I I > s O - ? @
; ) ^ / . . . .
. .
. .
Explanation
Basically, this moves the two bounds closer together one step at a time until they meet. Each time through the loop, we s
wap the bounds, O
utput, take the difference, and increment with )
or decrement with (
based on the sign.
$endgroup$
add a comment |
$begingroup$
Cubix, 16 bytes
;w(.II>sO-?@;)^/
Try it here
Cubified
; w
( .
I I > s O - ? @
; ) ^ / . . . .
. .
. .
Explanation
Basically, this moves the two bounds closer together one step at a time until they meet. Each time through the loop, we s
wap the bounds, O
utput, take the difference, and increment with )
or decrement with (
based on the sign.
$endgroup$
add a comment |
$begingroup$
Cubix, 16 bytes
;w(.II>sO-?@;)^/
Try it here
Cubified
; w
( .
I I > s O - ? @
; ) ^ / . . . .
. .
. .
Explanation
Basically, this moves the two bounds closer together one step at a time until they meet. Each time through the loop, we s
wap the bounds, O
utput, take the difference, and increment with )
or decrement with (
based on the sign.
$endgroup$
Cubix, 16 bytes
;w(.II>sO-?@;)^/
Try it here
Cubified
; w
( .
I I > s O - ? @
; ) ^ / . . . .
. .
. .
Explanation
Basically, this moves the two bounds closer together one step at a time until they meet. Each time through the loop, we s
wap the bounds, O
utput, take the difference, and increment with )
or decrement with (
based on the sign.
answered Feb 14 at 16:41
MnemonicMnemonic
4,7351730
4,7351730
add a comment |
add a comment |
$begingroup$
Pyth, 10 8 bytes
{.iF_B}F
Try it here
Explanation
{.iF_B}F
}FQ Generate the range between the (implicit) inputs.
.iF_B Interleave it with its reverse.
{ Deduplicate.
$endgroup$
add a comment |
$begingroup$
Pyth, 10 8 bytes
{.iF_B}F
Try it here
Explanation
{.iF_B}F
}FQ Generate the range between the (implicit) inputs.
.iF_B Interleave it with its reverse.
{ Deduplicate.
$endgroup$
add a comment |
$begingroup$
Pyth, 10 8 bytes
{.iF_B}F
Try it here
Explanation
{.iF_B}F
}FQ Generate the range between the (implicit) inputs.
.iF_B Interleave it with its reverse.
{ Deduplicate.
$endgroup$
Pyth, 10 8 bytes
{.iF_B}F
Try it here
Explanation
{.iF_B}F
}FQ Generate the range between the (implicit) inputs.
.iF_B Interleave it with its reverse.
{ Deduplicate.
edited Feb 14 at 17:44
answered Feb 13 at 22:08
MnemonicMnemonic
4,7351730
4,7351730
add a comment |
add a comment |
$begingroup$
JavaScript, 35 bytes
f=(a,b,s=1)=>a-b?a+[,f(b,a+s,-s)]:a
Try it online!
Thanks to Arnauld, 1 byte saved.
$endgroup$
add a comment |
$begingroup$
JavaScript, 35 bytes
f=(a,b,s=1)=>a-b?a+[,f(b,a+s,-s)]:a
Try it online!
Thanks to Arnauld, 1 byte saved.
$endgroup$
add a comment |
$begingroup$
JavaScript, 35 bytes
f=(a,b,s=1)=>a-b?a+[,f(b,a+s,-s)]:a
Try it online!
Thanks to Arnauld, 1 byte saved.
$endgroup$
JavaScript, 35 bytes
f=(a,b,s=1)=>a-b?a+[,f(b,a+s,-s)]:a
Try it online!
Thanks to Arnauld, 1 byte saved.
edited Feb 15 at 1:49
answered Feb 14 at 3:24
tshtsh
9,06511650
9,06511650
add a comment |
add a comment |
1 2
next
If this is an answer to a challenge…
…Be sure to follow the challenge specification. However, please refrain from exploiting obvious loopholes. Answers abusing any of the standard loopholes are considered invalid. If you think a specification is unclear or underspecified, comment on the question instead.
…Try to optimize your score. For instance, answers to code-golf challenges should attempt to be as short as possible. You can always include a readable version of the code in addition to the competitive one.
Explanations of your answer make it more interesting to read and are very much encouraged.…Include a short header which indicates the language(s) of your code and its score, as defined by the challenge.
More generally…
…Please make sure to answer the question and provide sufficient detail.
…Avoid asking for help, clarification or responding to other answers (use comments instead).
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodegolf.stackexchange.com%2fquestions%2f179852%2fthe-465-arrangement%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QmnephLAwr,buQcZk PTIHGzre6EYTMdk,Hja3,p5FrQ H9Z7HZD1RoPB6GUkNWC5hY8fL3ZNClSXw0o3Q7y,Qc6KajmKqkN,wVLX
2
$begingroup$
Almost duplicate (the difference being that this one requires reversing the second half before merging).
$endgroup$
– Peter Taylor
Feb 13 at 15:13
$begingroup$
is the input always going to be in the order of low end, high end?
$endgroup$
– Sumner18
Feb 13 at 16:14
1
$begingroup$
@Sumner18 yes. The community here is dead-set against input validation, and I haven’t asked for a reverse-order input, so we can assume it’ll always be low - high.
$endgroup$
– AJFaraday
Feb 13 at 16:21
1
$begingroup$
@Sumner18 How these challenges usually work is that we don't care how invalid inputs are handled. Your code is only judged to be successful by how it deals with valid inputs (i.e. both are integers, the first is lower than the second)
$endgroup$
– AJFaraday
Feb 13 at 16:36
1
$begingroup$
@AJFaraday: you should add a note to the main post indicating that X will be always strictly lower than Y (i.e. X != Y), I missed this comment ;)
$endgroup$
– digEmAll
Feb 13 at 18:23