Restrict security authentication for read access
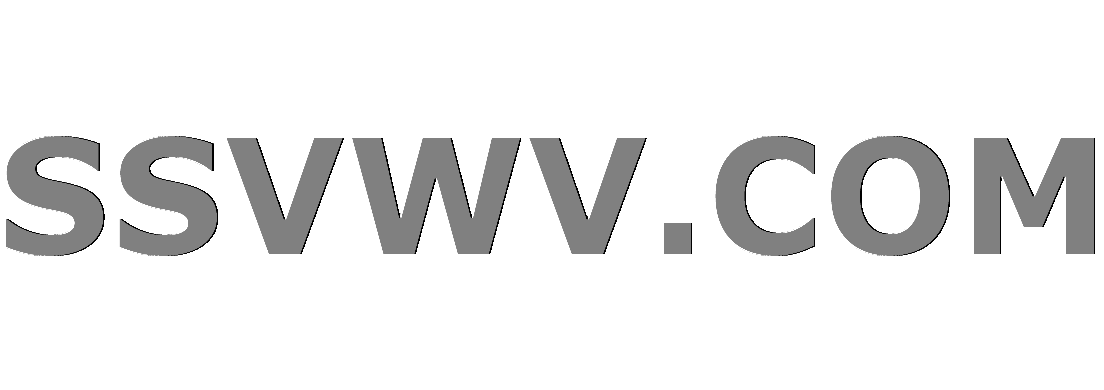
Multi tool use
I created a web application using a sample project in GitHub. However, it required authentication for all crud operations. I want to restrict this security checking for all read DB operations. What changes do I need?
These are the related classes:
protected void configure(HttpSecurity http) throws Exception {
http
.cors()
.and()
.csrf()
.disable()
.exceptionHandling()
.authenticationEntryPoint(unauthorizedHandler)
.and()
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers("/",
"/favicon.ico",
"/**/*.png",
"/**/*.gif",
"/**/*.svg",
"/**/*.jpg",
"/**/*.html",
"/**/*.css",
"/**/*.js")
.permitAll()
.antMatchers("/api/auth/**")
.permitAll()
.antMatchers("/api/user/checkUsernameAvailability", "/api/user/checkEmailAvailability")
.permitAll()
.antMatchers(HttpMethod.GET, "/api/polls/**", "/api/users/**")
.permitAll()
.anyRequest()
.authenticated();
// Add our custom JWT security filter
http.addFilterBefore(jwtAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
}
java spring spring-boot spring-security
add a comment |
I created a web application using a sample project in GitHub. However, it required authentication for all crud operations. I want to restrict this security checking for all read DB operations. What changes do I need?
These are the related classes:
protected void configure(HttpSecurity http) throws Exception {
http
.cors()
.and()
.csrf()
.disable()
.exceptionHandling()
.authenticationEntryPoint(unauthorizedHandler)
.and()
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers("/",
"/favicon.ico",
"/**/*.png",
"/**/*.gif",
"/**/*.svg",
"/**/*.jpg",
"/**/*.html",
"/**/*.css",
"/**/*.js")
.permitAll()
.antMatchers("/api/auth/**")
.permitAll()
.antMatchers("/api/user/checkUsernameAvailability", "/api/user/checkEmailAvailability")
.permitAll()
.antMatchers(HttpMethod.GET, "/api/polls/**", "/api/users/**")
.permitAll()
.anyRequest()
.authenticated();
// Add our custom JWT security filter
http.addFilterBefore(jwtAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
}
java spring spring-boot spring-security
add a comment |
I created a web application using a sample project in GitHub. However, it required authentication for all crud operations. I want to restrict this security checking for all read DB operations. What changes do I need?
These are the related classes:
protected void configure(HttpSecurity http) throws Exception {
http
.cors()
.and()
.csrf()
.disable()
.exceptionHandling()
.authenticationEntryPoint(unauthorizedHandler)
.and()
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers("/",
"/favicon.ico",
"/**/*.png",
"/**/*.gif",
"/**/*.svg",
"/**/*.jpg",
"/**/*.html",
"/**/*.css",
"/**/*.js")
.permitAll()
.antMatchers("/api/auth/**")
.permitAll()
.antMatchers("/api/user/checkUsernameAvailability", "/api/user/checkEmailAvailability")
.permitAll()
.antMatchers(HttpMethod.GET, "/api/polls/**", "/api/users/**")
.permitAll()
.anyRequest()
.authenticated();
// Add our custom JWT security filter
http.addFilterBefore(jwtAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
}
java spring spring-boot spring-security
I created a web application using a sample project in GitHub. However, it required authentication for all crud operations. I want to restrict this security checking for all read DB operations. What changes do I need?
These are the related classes:
protected void configure(HttpSecurity http) throws Exception {
http
.cors()
.and()
.csrf()
.disable()
.exceptionHandling()
.authenticationEntryPoint(unauthorizedHandler)
.and()
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.authorizeRequests()
.antMatchers("/",
"/favicon.ico",
"/**/*.png",
"/**/*.gif",
"/**/*.svg",
"/**/*.jpg",
"/**/*.html",
"/**/*.css",
"/**/*.js")
.permitAll()
.antMatchers("/api/auth/**")
.permitAll()
.antMatchers("/api/user/checkUsernameAvailability", "/api/user/checkEmailAvailability")
.permitAll()
.antMatchers(HttpMethod.GET, "/api/polls/**", "/api/users/**")
.permitAll()
.anyRequest()
.authenticated();
// Add our custom JWT security filter
http.addFilterBefore(jwtAuthenticationFilter(), UsernamePasswordAuthenticationFilter.class);
}
}
java spring spring-boot spring-security
java spring spring-boot spring-security
asked Nov 20 '18 at 16:29


chk.buddichk.buddi
10912
10912
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
As far as i know there is no way to magically create a read only user.However, you can create a role such as ROLE_UPDATE
and make all of your methods that perform creates/updates/deletes be secured via @Secured("ROLE_UPDATE")
. Then, if a user is not granted the ROLE_UPDATE
authority, they will not be able to call any of the 'writing' methods, and therefore it will be restricted to only call 'read' methods.
Thank you Alien
– chk.buddi
Nov 22 '18 at 15:33
add a comment |
Generally, Spring Security doesn't have such feature. You can do as @Alien suggested create some role (ex. ROLE_WRITE
and then check on the resources if the user who is trying to access the resource has the correct role
@PreAuthorize("hasRole('ROLE_WRITE')")
public String someWriteOperation() {
}
The other way (but it's only applying when your JPA framework allows you such feature) it's create a Filter
in spring and then before processing your request further in the chain create transaction read-only:
@Component
@Order(1)
public class TransactionFilter implements Filter {
@Override
public void doFilterServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
//Create read only transaction
// ex. if(isUserReadOnly(Security ....getUser())) {DBSession.setReadOnly(true);}
//Remember it will work only if your JPA framework have the feature - explore your code/framework before
chain.doFilter(request, response);
}
}
Remember filter order should be after Spring Security Filter
Thank you Andrew Sasha
– chk.buddi
Nov 22 '18 at 15:34
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53397387%2frestrict-security-authentication-for-read-access%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
As far as i know there is no way to magically create a read only user.However, you can create a role such as ROLE_UPDATE
and make all of your methods that perform creates/updates/deletes be secured via @Secured("ROLE_UPDATE")
. Then, if a user is not granted the ROLE_UPDATE
authority, they will not be able to call any of the 'writing' methods, and therefore it will be restricted to only call 'read' methods.
Thank you Alien
– chk.buddi
Nov 22 '18 at 15:33
add a comment |
As far as i know there is no way to magically create a read only user.However, you can create a role such as ROLE_UPDATE
and make all of your methods that perform creates/updates/deletes be secured via @Secured("ROLE_UPDATE")
. Then, if a user is not granted the ROLE_UPDATE
authority, they will not be able to call any of the 'writing' methods, and therefore it will be restricted to only call 'read' methods.
Thank you Alien
– chk.buddi
Nov 22 '18 at 15:33
add a comment |
As far as i know there is no way to magically create a read only user.However, you can create a role such as ROLE_UPDATE
and make all of your methods that perform creates/updates/deletes be secured via @Secured("ROLE_UPDATE")
. Then, if a user is not granted the ROLE_UPDATE
authority, they will not be able to call any of the 'writing' methods, and therefore it will be restricted to only call 'read' methods.
As far as i know there is no way to magically create a read only user.However, you can create a role such as ROLE_UPDATE
and make all of your methods that perform creates/updates/deletes be secured via @Secured("ROLE_UPDATE")
. Then, if a user is not granted the ROLE_UPDATE
authority, they will not be able to call any of the 'writing' methods, and therefore it will be restricted to only call 'read' methods.
answered Nov 20 '18 at 18:24


AlienAlien
5,15131126
5,15131126
Thank you Alien
– chk.buddi
Nov 22 '18 at 15:33
add a comment |
Thank you Alien
– chk.buddi
Nov 22 '18 at 15:33
Thank you Alien
– chk.buddi
Nov 22 '18 at 15:33
Thank you Alien
– chk.buddi
Nov 22 '18 at 15:33
add a comment |
Generally, Spring Security doesn't have such feature. You can do as @Alien suggested create some role (ex. ROLE_WRITE
and then check on the resources if the user who is trying to access the resource has the correct role
@PreAuthorize("hasRole('ROLE_WRITE')")
public String someWriteOperation() {
}
The other way (but it's only applying when your JPA framework allows you such feature) it's create a Filter
in spring and then before processing your request further in the chain create transaction read-only:
@Component
@Order(1)
public class TransactionFilter implements Filter {
@Override
public void doFilterServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
//Create read only transaction
// ex. if(isUserReadOnly(Security ....getUser())) {DBSession.setReadOnly(true);}
//Remember it will work only if your JPA framework have the feature - explore your code/framework before
chain.doFilter(request, response);
}
}
Remember filter order should be after Spring Security Filter
Thank you Andrew Sasha
– chk.buddi
Nov 22 '18 at 15:34
add a comment |
Generally, Spring Security doesn't have such feature. You can do as @Alien suggested create some role (ex. ROLE_WRITE
and then check on the resources if the user who is trying to access the resource has the correct role
@PreAuthorize("hasRole('ROLE_WRITE')")
public String someWriteOperation() {
}
The other way (but it's only applying when your JPA framework allows you such feature) it's create a Filter
in spring and then before processing your request further in the chain create transaction read-only:
@Component
@Order(1)
public class TransactionFilter implements Filter {
@Override
public void doFilterServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
//Create read only transaction
// ex. if(isUserReadOnly(Security ....getUser())) {DBSession.setReadOnly(true);}
//Remember it will work only if your JPA framework have the feature - explore your code/framework before
chain.doFilter(request, response);
}
}
Remember filter order should be after Spring Security Filter
Thank you Andrew Sasha
– chk.buddi
Nov 22 '18 at 15:34
add a comment |
Generally, Spring Security doesn't have such feature. You can do as @Alien suggested create some role (ex. ROLE_WRITE
and then check on the resources if the user who is trying to access the resource has the correct role
@PreAuthorize("hasRole('ROLE_WRITE')")
public String someWriteOperation() {
}
The other way (but it's only applying when your JPA framework allows you such feature) it's create a Filter
in spring and then before processing your request further in the chain create transaction read-only:
@Component
@Order(1)
public class TransactionFilter implements Filter {
@Override
public void doFilterServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
//Create read only transaction
// ex. if(isUserReadOnly(Security ....getUser())) {DBSession.setReadOnly(true);}
//Remember it will work only if your JPA framework have the feature - explore your code/framework before
chain.doFilter(request, response);
}
}
Remember filter order should be after Spring Security Filter
Generally, Spring Security doesn't have such feature. You can do as @Alien suggested create some role (ex. ROLE_WRITE
and then check on the resources if the user who is trying to access the resource has the correct role
@PreAuthorize("hasRole('ROLE_WRITE')")
public String someWriteOperation() {
}
The other way (but it's only applying when your JPA framework allows you such feature) it's create a Filter
in spring and then before processing your request further in the chain create transaction read-only:
@Component
@Order(1)
public class TransactionFilter implements Filter {
@Override
public void doFilterServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException {
//Create read only transaction
// ex. if(isUserReadOnly(Security ....getUser())) {DBSession.setReadOnly(true);}
//Remember it will work only if your JPA framework have the feature - explore your code/framework before
chain.doFilter(request, response);
}
}
Remember filter order should be after Spring Security Filter
edited Nov 22 '18 at 15:35
answered Nov 22 '18 at 15:31
Andrew SashaAndrew Sasha
554214
554214
Thank you Andrew Sasha
– chk.buddi
Nov 22 '18 at 15:34
add a comment |
Thank you Andrew Sasha
– chk.buddi
Nov 22 '18 at 15:34
Thank you Andrew Sasha
– chk.buddi
Nov 22 '18 at 15:34
Thank you Andrew Sasha
– chk.buddi
Nov 22 '18 at 15:34
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53397387%2frestrict-security-authentication-for-read-access%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cCo8fU YVa oRPvX