User input in Python, after a false statement and recall the function to force the correct entry, the true...
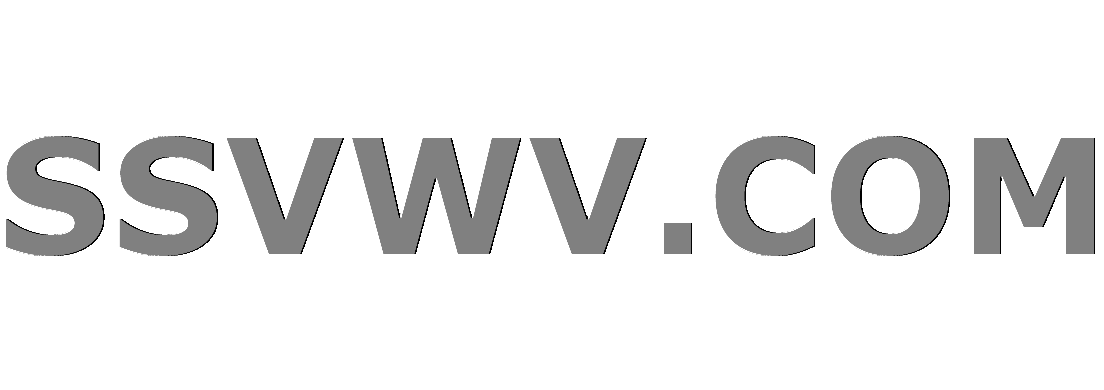
Multi tool use
It is my second question here in the group, I really tried to find the answer everywhere, probably I used the wrong terms when looking for it. I am a completely new in Python. Please, tell me If the answer is available in your community or in anywhere else, and I will delete this post. Thank you.
So, I am doing a Python online course, and in the assignment I have to create a database for a movie store, so I have to create menus and actions like, view the list of movies, add a movie, search a movie, delete a movie and so on...
I am stuck in the process to add a movie, that is when I ask the user to insert the correct 'movie year' in format '2018' and the correct category (which is a dictionary)...
The problem is, if the users do their job correctly since begin, everything perfectly works, i.e. the true statements in the IF's and the Try(Except) work great, but when the users input a wrong format either in the 'movie year' or a different number for the category in the category movie, the IF's and Try(Except) FALSE statements work and make the users insert their answer again, however, the new TRUE statements don't do what they should do, which are to 'return' (capture) the user input, it is returning 'None' instead.
Please, help me out with this.
Thank you.
By the way, I am coding in PyCharm, if that matters. Cheers.
Assignment 1 - Database for a movie store
def save_dict_to_file(dic):
f = open('dict.txt', 'w')
f.write(str(dic))
f.close()
def load_dict_from_file():
f = open('dict.txt', 'r')
data = f.read()
f.close()
return eval(data)
def open_or_loading():
print('nnHello to Movies.basenn'
'Do you want to load an existent database or create a new one?nn'
'Choose 1 for loading an existent.n'
'Choose 2 for create a new database.n')
user_answer = input('Option: ')
if user_answer == '1':
database = load_dict_from_file()
elif user_answer == '2':
database = {}
save_dict_to_file(database)
else:
print('Wrong option, try again.n')
open_or_loading()
def view_all_list():
for key, value in database.items():
print(key, value)
def add_movie():
last_inserted = (len(database))
movie_name = input('What is the name of the movie: ')
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
return movie_year
movie_year = year_movie()
movie_director = input('Directed by: ')
def category_movie():
print('Choose one category:nn'
'1 - Actionn'
'2 - Adventuren'
'3 - Avant-garde / Experimentaln'
'4 - Comedyn'
'5 - Comedy-Draman'
'6 - Crimen'
'7 - Draman'
'8 - Epicn'
'9 - Family-Childrenn'
'10 - Fantasyn'
'11 - Historical-Filmn'
'12 - Horrorn'
'13 - Musicaln'
'14 - Mysteryn'
'15 - Romancen'
'16 - Sci-Fi / Science-Fictionn'
'17 - Spy Filmn'
'18 - Thrillern'
'19 - Warn'
'20 - Westernn'
'21 - Adultn')
category = int(input('Category: '))
def switch_demo(argument):
switcher = {
1: 'Action',
2: 'Adventure',
3: 'Avant-garde / Experimental',
4: 'Comedy',
5: 'Comedy-Drama',
6: 'Crime',
7: 'Drama',
8: 'Epic',
9: 'Family-Children',
10: 'Fantasy',
11: 'Historical-Film',
12: 'Horror',
13: 'Musical',
14: 'Mystery',
15: 'Romance',
16: 'Sci-Fi / Science-Fiction',
17: 'Spy Film',
18: 'Thriller',
19: 'War',
20: 'Western',
21: 'Adult'
}
return switcher.get(category)
if category < 1 or category > 21:
print('nInvalid Categoryn')
category_movie()
switch_demo(category)
else:
return switch_demo(category)
movie_category = category_movie()
database[last_inserted + 1] = {}
database[last_inserted + 1]['Movie Name'] = movie_name.upper()
database[last_inserted + 1]['Movie Year'] = movie_year
database[last_inserted + 1]['Director Name'] = movie_director.upper()
database[last_inserted + 1]['Movie Category'] = movie_category
save_dict_to_file(database)
print(f'nMovie {movie_name} added to databasen')
menu_initial()
def menu_initial():
print('nnChose one option:nn'
'Select 1 to view the movies list.n'
'Select 2 to add a new movie.n'
'Select 3 to find a movie.n'
'Select 4 to delete a movie.n'
'Select 5 to exit outn')
user_selection = (input('Option: '))
if user_selection == '1':
view_all_list()
menu_initial()
elif user_selection == '2':
add_movie()
menu_initial()
elif user_selection == '3':
print('Finding')
menu_initial()
elif user_selection == '4':
print('deleting')
menu_initial()
elif user_selection == '5':
print('Thanks for using Movie.Base. Bye-bye!')
exit()
else:
print('Option do not exist')
menu_initial()
menu_initial()
open_or_loading()
python python-3.x pycharm
add a comment |
It is my second question here in the group, I really tried to find the answer everywhere, probably I used the wrong terms when looking for it. I am a completely new in Python. Please, tell me If the answer is available in your community or in anywhere else, and I will delete this post. Thank you.
So, I am doing a Python online course, and in the assignment I have to create a database for a movie store, so I have to create menus and actions like, view the list of movies, add a movie, search a movie, delete a movie and so on...
I am stuck in the process to add a movie, that is when I ask the user to insert the correct 'movie year' in format '2018' and the correct category (which is a dictionary)...
The problem is, if the users do their job correctly since begin, everything perfectly works, i.e. the true statements in the IF's and the Try(Except) work great, but when the users input a wrong format either in the 'movie year' or a different number for the category in the category movie, the IF's and Try(Except) FALSE statements work and make the users insert their answer again, however, the new TRUE statements don't do what they should do, which are to 'return' (capture) the user input, it is returning 'None' instead.
Please, help me out with this.
Thank you.
By the way, I am coding in PyCharm, if that matters. Cheers.
Assignment 1 - Database for a movie store
def save_dict_to_file(dic):
f = open('dict.txt', 'w')
f.write(str(dic))
f.close()
def load_dict_from_file():
f = open('dict.txt', 'r')
data = f.read()
f.close()
return eval(data)
def open_or_loading():
print('nnHello to Movies.basenn'
'Do you want to load an existent database or create a new one?nn'
'Choose 1 for loading an existent.n'
'Choose 2 for create a new database.n')
user_answer = input('Option: ')
if user_answer == '1':
database = load_dict_from_file()
elif user_answer == '2':
database = {}
save_dict_to_file(database)
else:
print('Wrong option, try again.n')
open_or_loading()
def view_all_list():
for key, value in database.items():
print(key, value)
def add_movie():
last_inserted = (len(database))
movie_name = input('What is the name of the movie: ')
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
return movie_year
movie_year = year_movie()
movie_director = input('Directed by: ')
def category_movie():
print('Choose one category:nn'
'1 - Actionn'
'2 - Adventuren'
'3 - Avant-garde / Experimentaln'
'4 - Comedyn'
'5 - Comedy-Draman'
'6 - Crimen'
'7 - Draman'
'8 - Epicn'
'9 - Family-Childrenn'
'10 - Fantasyn'
'11 - Historical-Filmn'
'12 - Horrorn'
'13 - Musicaln'
'14 - Mysteryn'
'15 - Romancen'
'16 - Sci-Fi / Science-Fictionn'
'17 - Spy Filmn'
'18 - Thrillern'
'19 - Warn'
'20 - Westernn'
'21 - Adultn')
category = int(input('Category: '))
def switch_demo(argument):
switcher = {
1: 'Action',
2: 'Adventure',
3: 'Avant-garde / Experimental',
4: 'Comedy',
5: 'Comedy-Drama',
6: 'Crime',
7: 'Drama',
8: 'Epic',
9: 'Family-Children',
10: 'Fantasy',
11: 'Historical-Film',
12: 'Horror',
13: 'Musical',
14: 'Mystery',
15: 'Romance',
16: 'Sci-Fi / Science-Fiction',
17: 'Spy Film',
18: 'Thriller',
19: 'War',
20: 'Western',
21: 'Adult'
}
return switcher.get(category)
if category < 1 or category > 21:
print('nInvalid Categoryn')
category_movie()
switch_demo(category)
else:
return switch_demo(category)
movie_category = category_movie()
database[last_inserted + 1] = {}
database[last_inserted + 1]['Movie Name'] = movie_name.upper()
database[last_inserted + 1]['Movie Year'] = movie_year
database[last_inserted + 1]['Director Name'] = movie_director.upper()
database[last_inserted + 1]['Movie Category'] = movie_category
save_dict_to_file(database)
print(f'nMovie {movie_name} added to databasen')
menu_initial()
def menu_initial():
print('nnChose one option:nn'
'Select 1 to view the movies list.n'
'Select 2 to add a new movie.n'
'Select 3 to find a movie.n'
'Select 4 to delete a movie.n'
'Select 5 to exit outn')
user_selection = (input('Option: '))
if user_selection == '1':
view_all_list()
menu_initial()
elif user_selection == '2':
add_movie()
menu_initial()
elif user_selection == '3':
print('Finding')
menu_initial()
elif user_selection == '4':
print('deleting')
menu_initial()
elif user_selection == '5':
print('Thanks for using Movie.Base. Bye-bye!')
exit()
else:
print('Option do not exist')
menu_initial()
menu_initial()
open_or_loading()
python python-3.x pycharm
add a comment |
It is my second question here in the group, I really tried to find the answer everywhere, probably I used the wrong terms when looking for it. I am a completely new in Python. Please, tell me If the answer is available in your community or in anywhere else, and I will delete this post. Thank you.
So, I am doing a Python online course, and in the assignment I have to create a database for a movie store, so I have to create menus and actions like, view the list of movies, add a movie, search a movie, delete a movie and so on...
I am stuck in the process to add a movie, that is when I ask the user to insert the correct 'movie year' in format '2018' and the correct category (which is a dictionary)...
The problem is, if the users do their job correctly since begin, everything perfectly works, i.e. the true statements in the IF's and the Try(Except) work great, but when the users input a wrong format either in the 'movie year' or a different number for the category in the category movie, the IF's and Try(Except) FALSE statements work and make the users insert their answer again, however, the new TRUE statements don't do what they should do, which are to 'return' (capture) the user input, it is returning 'None' instead.
Please, help me out with this.
Thank you.
By the way, I am coding in PyCharm, if that matters. Cheers.
Assignment 1 - Database for a movie store
def save_dict_to_file(dic):
f = open('dict.txt', 'w')
f.write(str(dic))
f.close()
def load_dict_from_file():
f = open('dict.txt', 'r')
data = f.read()
f.close()
return eval(data)
def open_or_loading():
print('nnHello to Movies.basenn'
'Do you want to load an existent database or create a new one?nn'
'Choose 1 for loading an existent.n'
'Choose 2 for create a new database.n')
user_answer = input('Option: ')
if user_answer == '1':
database = load_dict_from_file()
elif user_answer == '2':
database = {}
save_dict_to_file(database)
else:
print('Wrong option, try again.n')
open_or_loading()
def view_all_list():
for key, value in database.items():
print(key, value)
def add_movie():
last_inserted = (len(database))
movie_name = input('What is the name of the movie: ')
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
return movie_year
movie_year = year_movie()
movie_director = input('Directed by: ')
def category_movie():
print('Choose one category:nn'
'1 - Actionn'
'2 - Adventuren'
'3 - Avant-garde / Experimentaln'
'4 - Comedyn'
'5 - Comedy-Draman'
'6 - Crimen'
'7 - Draman'
'8 - Epicn'
'9 - Family-Childrenn'
'10 - Fantasyn'
'11 - Historical-Filmn'
'12 - Horrorn'
'13 - Musicaln'
'14 - Mysteryn'
'15 - Romancen'
'16 - Sci-Fi / Science-Fictionn'
'17 - Spy Filmn'
'18 - Thrillern'
'19 - Warn'
'20 - Westernn'
'21 - Adultn')
category = int(input('Category: '))
def switch_demo(argument):
switcher = {
1: 'Action',
2: 'Adventure',
3: 'Avant-garde / Experimental',
4: 'Comedy',
5: 'Comedy-Drama',
6: 'Crime',
7: 'Drama',
8: 'Epic',
9: 'Family-Children',
10: 'Fantasy',
11: 'Historical-Film',
12: 'Horror',
13: 'Musical',
14: 'Mystery',
15: 'Romance',
16: 'Sci-Fi / Science-Fiction',
17: 'Spy Film',
18: 'Thriller',
19: 'War',
20: 'Western',
21: 'Adult'
}
return switcher.get(category)
if category < 1 or category > 21:
print('nInvalid Categoryn')
category_movie()
switch_demo(category)
else:
return switch_demo(category)
movie_category = category_movie()
database[last_inserted + 1] = {}
database[last_inserted + 1]['Movie Name'] = movie_name.upper()
database[last_inserted + 1]['Movie Year'] = movie_year
database[last_inserted + 1]['Director Name'] = movie_director.upper()
database[last_inserted + 1]['Movie Category'] = movie_category
save_dict_to_file(database)
print(f'nMovie {movie_name} added to databasen')
menu_initial()
def menu_initial():
print('nnChose one option:nn'
'Select 1 to view the movies list.n'
'Select 2 to add a new movie.n'
'Select 3 to find a movie.n'
'Select 4 to delete a movie.n'
'Select 5 to exit outn')
user_selection = (input('Option: '))
if user_selection == '1':
view_all_list()
menu_initial()
elif user_selection == '2':
add_movie()
menu_initial()
elif user_selection == '3':
print('Finding')
menu_initial()
elif user_selection == '4':
print('deleting')
menu_initial()
elif user_selection == '5':
print('Thanks for using Movie.Base. Bye-bye!')
exit()
else:
print('Option do not exist')
menu_initial()
menu_initial()
open_or_loading()
python python-3.x pycharm
It is my second question here in the group, I really tried to find the answer everywhere, probably I used the wrong terms when looking for it. I am a completely new in Python. Please, tell me If the answer is available in your community or in anywhere else, and I will delete this post. Thank you.
So, I am doing a Python online course, and in the assignment I have to create a database for a movie store, so I have to create menus and actions like, view the list of movies, add a movie, search a movie, delete a movie and so on...
I am stuck in the process to add a movie, that is when I ask the user to insert the correct 'movie year' in format '2018' and the correct category (which is a dictionary)...
The problem is, if the users do their job correctly since begin, everything perfectly works, i.e. the true statements in the IF's and the Try(Except) work great, but when the users input a wrong format either in the 'movie year' or a different number for the category in the category movie, the IF's and Try(Except) FALSE statements work and make the users insert their answer again, however, the new TRUE statements don't do what they should do, which are to 'return' (capture) the user input, it is returning 'None' instead.
Please, help me out with this.
Thank you.
By the way, I am coding in PyCharm, if that matters. Cheers.
Assignment 1 - Database for a movie store
def save_dict_to_file(dic):
f = open('dict.txt', 'w')
f.write(str(dic))
f.close()
def load_dict_from_file():
f = open('dict.txt', 'r')
data = f.read()
f.close()
return eval(data)
def open_or_loading():
print('nnHello to Movies.basenn'
'Do you want to load an existent database or create a new one?nn'
'Choose 1 for loading an existent.n'
'Choose 2 for create a new database.n')
user_answer = input('Option: ')
if user_answer == '1':
database = load_dict_from_file()
elif user_answer == '2':
database = {}
save_dict_to_file(database)
else:
print('Wrong option, try again.n')
open_or_loading()
def view_all_list():
for key, value in database.items():
print(key, value)
def add_movie():
last_inserted = (len(database))
movie_name = input('What is the name of the movie: ')
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
year_movie()
else:
return movie_year
movie_year = year_movie()
movie_director = input('Directed by: ')
def category_movie():
print('Choose one category:nn'
'1 - Actionn'
'2 - Adventuren'
'3 - Avant-garde / Experimentaln'
'4 - Comedyn'
'5 - Comedy-Draman'
'6 - Crimen'
'7 - Draman'
'8 - Epicn'
'9 - Family-Childrenn'
'10 - Fantasyn'
'11 - Historical-Filmn'
'12 - Horrorn'
'13 - Musicaln'
'14 - Mysteryn'
'15 - Romancen'
'16 - Sci-Fi / Science-Fictionn'
'17 - Spy Filmn'
'18 - Thrillern'
'19 - Warn'
'20 - Westernn'
'21 - Adultn')
category = int(input('Category: '))
def switch_demo(argument):
switcher = {
1: 'Action',
2: 'Adventure',
3: 'Avant-garde / Experimental',
4: 'Comedy',
5: 'Comedy-Drama',
6: 'Crime',
7: 'Drama',
8: 'Epic',
9: 'Family-Children',
10: 'Fantasy',
11: 'Historical-Film',
12: 'Horror',
13: 'Musical',
14: 'Mystery',
15: 'Romance',
16: 'Sci-Fi / Science-Fiction',
17: 'Spy Film',
18: 'Thriller',
19: 'War',
20: 'Western',
21: 'Adult'
}
return switcher.get(category)
if category < 1 or category > 21:
print('nInvalid Categoryn')
category_movie()
switch_demo(category)
else:
return switch_demo(category)
movie_category = category_movie()
database[last_inserted + 1] = {}
database[last_inserted + 1]['Movie Name'] = movie_name.upper()
database[last_inserted + 1]['Movie Year'] = movie_year
database[last_inserted + 1]['Director Name'] = movie_director.upper()
database[last_inserted + 1]['Movie Category'] = movie_category
save_dict_to_file(database)
print(f'nMovie {movie_name} added to databasen')
menu_initial()
def menu_initial():
print('nnChose one option:nn'
'Select 1 to view the movies list.n'
'Select 2 to add a new movie.n'
'Select 3 to find a movie.n'
'Select 4 to delete a movie.n'
'Select 5 to exit outn')
user_selection = (input('Option: '))
if user_selection == '1':
view_all_list()
menu_initial()
elif user_selection == '2':
add_movie()
menu_initial()
elif user_selection == '3':
print('Finding')
menu_initial()
elif user_selection == '4':
print('deleting')
menu_initial()
elif user_selection == '5':
print('Thanks for using Movie.Base. Bye-bye!')
exit()
else:
print('Option do not exist')
menu_initial()
menu_initial()
open_or_loading()
python python-3.x pycharm
python python-3.x pycharm
edited Nov 19 '18 at 12:12
Shyllenno
asked Nov 19 '18 at 12:04


ShyllennoShyllenno
65
65
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
In the method year_movie you need to always return something, otherwise you get None
. You're calling this method recursively when the error is incorrect but you don't return the value. Try adding return
keyword in the recursive calls:
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
return movie_year
Also, in category_movie()
:
if category < 1 or category > 21:
print('nInvalid Categoryn')
category = category_movie() # <-- Assign this value
switch_demo(category)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374275%2fuser-input-in-python-after-a-false-statement-and-recall-the-function-to-force-t%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In the method year_movie you need to always return something, otherwise you get None
. You're calling this method recursively when the error is incorrect but you don't return the value. Try adding return
keyword in the recursive calls:
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
return movie_year
Also, in category_movie()
:
if category < 1 or category > 21:
print('nInvalid Categoryn')
category = category_movie() # <-- Assign this value
switch_demo(category)
add a comment |
In the method year_movie you need to always return something, otherwise you get None
. You're calling this method recursively when the error is incorrect but you don't return the value. Try adding return
keyword in the recursive calls:
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
return movie_year
Also, in category_movie()
:
if category < 1 or category > 21:
print('nInvalid Categoryn')
category = category_movie() # <-- Assign this value
switch_demo(category)
add a comment |
In the method year_movie you need to always return something, otherwise you get None
. You're calling this method recursively when the error is incorrect but you don't return the value. Try adding return
keyword in the recursive calls:
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
return movie_year
Also, in category_movie()
:
if category < 1 or category > 21:
print('nInvalid Categoryn')
category = category_movie() # <-- Assign this value
switch_demo(category)
In the method year_movie you need to always return something, otherwise you get None
. You're calling this method recursively when the error is incorrect but you don't return the value. Try adding return
keyword in the recursive calls:
def year_movie():
try:
movie_year = int(input('Released in year: '))
except:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
if movie_year < 1000 or movie_year > 9999:
print('You must insert a year format: e.g.: 2018')
return year_movie() ## return from recursive call
else:
return movie_year
Also, in category_movie()
:
if category < 1 or category > 21:
print('nInvalid Categoryn')
category = category_movie() # <-- Assign this value
switch_demo(category)
edited Nov 19 '18 at 12:18


Carlos Mermingas
2,28621229
2,28621229
answered Nov 19 '18 at 12:13
Milo BemMilo Bem
822418
822418
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53374275%2fuser-input-in-python-after-a-false-statement-and-recall-the-function-to-force-t%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7SRFoU,BxBk42LLwQnf2pN5R oz1MgreOXOuV atlVYWkUoT