WPF bind DataGrid column ComboBox from database table using id
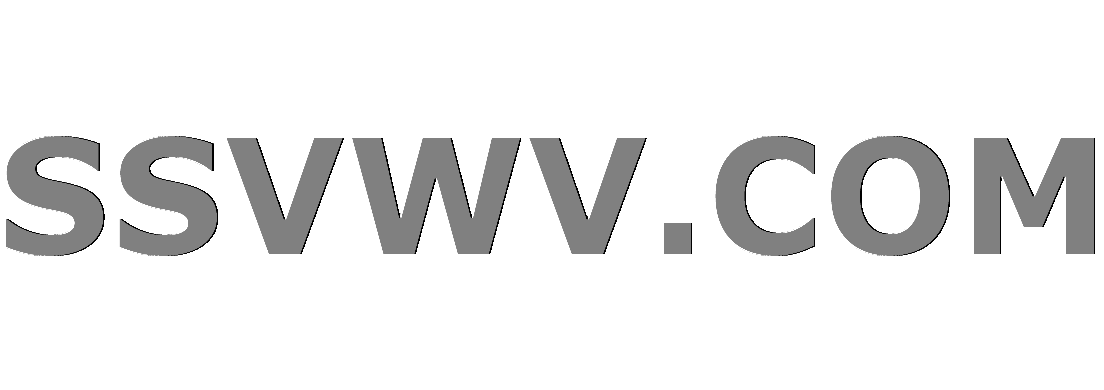
Multi tool use
up vote
0
down vote
favorite
I have classes
[Table(Name = "Categories")]
class Category
{
[Column(Name = "CategoryID", IsPrimaryKey = true, IsDbGenerated = true)]
private int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
}
[Table(Name = "Products")]
class Product
{
[Column(Name = "ProductID", IsPrimaryKey = true, IsDbGenerated = true)]
public int ProductID { get; set; }
[Column(Name = "CategoryID")]
public int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
[Column(Name = "Price")]
public double Price { get; set; }
}
and i need to create DataGrid column as ComboBox using CategoryID from Products and put all categories in ComboBox from Categories for editing, how can i make it with using DataContext
c# wpf xaml binding datacontext
add a comment |
up vote
0
down vote
favorite
I have classes
[Table(Name = "Categories")]
class Category
{
[Column(Name = "CategoryID", IsPrimaryKey = true, IsDbGenerated = true)]
private int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
}
[Table(Name = "Products")]
class Product
{
[Column(Name = "ProductID", IsPrimaryKey = true, IsDbGenerated = true)]
public int ProductID { get; set; }
[Column(Name = "CategoryID")]
public int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
[Column(Name = "Price")]
public double Price { get; set; }
}
and i need to create DataGrid column as ComboBox using CategoryID from Products and put all categories in ComboBox from Categories for editing, how can i make it with using DataContext
c# wpf xaml binding datacontext
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have classes
[Table(Name = "Categories")]
class Category
{
[Column(Name = "CategoryID", IsPrimaryKey = true, IsDbGenerated = true)]
private int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
}
[Table(Name = "Products")]
class Product
{
[Column(Name = "ProductID", IsPrimaryKey = true, IsDbGenerated = true)]
public int ProductID { get; set; }
[Column(Name = "CategoryID")]
public int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
[Column(Name = "Price")]
public double Price { get; set; }
}
and i need to create DataGrid column as ComboBox using CategoryID from Products and put all categories in ComboBox from Categories for editing, how can i make it with using DataContext
c# wpf xaml binding datacontext
I have classes
[Table(Name = "Categories")]
class Category
{
[Column(Name = "CategoryID", IsPrimaryKey = true, IsDbGenerated = true)]
private int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
}
[Table(Name = "Products")]
class Product
{
[Column(Name = "ProductID", IsPrimaryKey = true, IsDbGenerated = true)]
public int ProductID { get; set; }
[Column(Name = "CategoryID")]
public int CategoryID { get; set; }
[Column(Name = "Name")]
public string Name { get; set; }
[Column(Name = "Price")]
public double Price { get; set; }
}
and i need to create DataGrid column as ComboBox using CategoryID from Products and put all categories in ComboBox from Categories for editing, how can i make it with using DataContext
c# wpf xaml binding datacontext
c# wpf xaml binding datacontext
edited Nov 13 at 2:28
asked Nov 12 at 23:34


Rabbit_Killer
84
84
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Here I have created ObservableCollection of Product and Category with name ProductSet & ListOfCategory in the Window level.
public ObservableCollection<Category> ListOfCategory { get; set; }
public ObservableCollection<Product> ProductSet { get; set; }
I have created both the ObservableCollection in the Code behind of the Window, You can create this in view model which is better approch.
I have named the Window as "Window1" x:Name="Window1"
As you need to bind the data context, did the elementBinding with Window1 and also set the ItemsSource="{Binding ProductSet}". So what happens here is the ProuctSet is searched for DataContext, which is in turn searched inside Window1 Class.
For DataGridComboBoxColumn, set the ItemsSource using the Style and search for the Datagrid DataContext which has ListOfCategory using Relative Source.
<DataGrid AutoGenerateColumns="False" x:Name="DataGrid1" HorizontalAlignment="Left" Height="135" Margin="21,288,0,0" VerticalAlignment="Top" Width="729" DataContext="{Binding ElementName=Window1}" ItemsSource="{Binding ProductSet}" >
<DataGrid.Columns>
<DataGridTextColumn Header="ProductID" Width="175" Binding="{Binding ProductID}"></DataGridTextColumn>
<DataGridComboBoxColumn Header="Category" DisplayMemberPath="Name" SelectedValuePath="CategoryID" SelectedItemBinding="{Binding ListOfCategory}">
<DataGridComboBoxColumn.ElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory, RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.ElementStyle>
<DataGridComboBoxColumn.EditingElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory,RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.EditingElementStyle>
</DataGridComboBoxColumn>
<DataGridTextColumn Header="Name" Width="175" Binding="{Binding Name}"></DataGridTextColumn>
<DataGridTextColumn Header="Price " Width="175" Binding="{Binding Price }"></DataGridTextColumn>
</DataGrid.Columns>
</DataGrid>
If you going to bind using View model, then provide the Class Name of the Viewmodel instead of Element Binding.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Here I have created ObservableCollection of Product and Category with name ProductSet & ListOfCategory in the Window level.
public ObservableCollection<Category> ListOfCategory { get; set; }
public ObservableCollection<Product> ProductSet { get; set; }
I have created both the ObservableCollection in the Code behind of the Window, You can create this in view model which is better approch.
I have named the Window as "Window1" x:Name="Window1"
As you need to bind the data context, did the elementBinding with Window1 and also set the ItemsSource="{Binding ProductSet}". So what happens here is the ProuctSet is searched for DataContext, which is in turn searched inside Window1 Class.
For DataGridComboBoxColumn, set the ItemsSource using the Style and search for the Datagrid DataContext which has ListOfCategory using Relative Source.
<DataGrid AutoGenerateColumns="False" x:Name="DataGrid1" HorizontalAlignment="Left" Height="135" Margin="21,288,0,0" VerticalAlignment="Top" Width="729" DataContext="{Binding ElementName=Window1}" ItemsSource="{Binding ProductSet}" >
<DataGrid.Columns>
<DataGridTextColumn Header="ProductID" Width="175" Binding="{Binding ProductID}"></DataGridTextColumn>
<DataGridComboBoxColumn Header="Category" DisplayMemberPath="Name" SelectedValuePath="CategoryID" SelectedItemBinding="{Binding ListOfCategory}">
<DataGridComboBoxColumn.ElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory, RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.ElementStyle>
<DataGridComboBoxColumn.EditingElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory,RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.EditingElementStyle>
</DataGridComboBoxColumn>
<DataGridTextColumn Header="Name" Width="175" Binding="{Binding Name}"></DataGridTextColumn>
<DataGridTextColumn Header="Price " Width="175" Binding="{Binding Price }"></DataGridTextColumn>
</DataGrid.Columns>
</DataGrid>
If you going to bind using View model, then provide the Class Name of the Viewmodel instead of Element Binding.
add a comment |
up vote
0
down vote
accepted
Here I have created ObservableCollection of Product and Category with name ProductSet & ListOfCategory in the Window level.
public ObservableCollection<Category> ListOfCategory { get; set; }
public ObservableCollection<Product> ProductSet { get; set; }
I have created both the ObservableCollection in the Code behind of the Window, You can create this in view model which is better approch.
I have named the Window as "Window1" x:Name="Window1"
As you need to bind the data context, did the elementBinding with Window1 and also set the ItemsSource="{Binding ProductSet}". So what happens here is the ProuctSet is searched for DataContext, which is in turn searched inside Window1 Class.
For DataGridComboBoxColumn, set the ItemsSource using the Style and search for the Datagrid DataContext which has ListOfCategory using Relative Source.
<DataGrid AutoGenerateColumns="False" x:Name="DataGrid1" HorizontalAlignment="Left" Height="135" Margin="21,288,0,0" VerticalAlignment="Top" Width="729" DataContext="{Binding ElementName=Window1}" ItemsSource="{Binding ProductSet}" >
<DataGrid.Columns>
<DataGridTextColumn Header="ProductID" Width="175" Binding="{Binding ProductID}"></DataGridTextColumn>
<DataGridComboBoxColumn Header="Category" DisplayMemberPath="Name" SelectedValuePath="CategoryID" SelectedItemBinding="{Binding ListOfCategory}">
<DataGridComboBoxColumn.ElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory, RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.ElementStyle>
<DataGridComboBoxColumn.EditingElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory,RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.EditingElementStyle>
</DataGridComboBoxColumn>
<DataGridTextColumn Header="Name" Width="175" Binding="{Binding Name}"></DataGridTextColumn>
<DataGridTextColumn Header="Price " Width="175" Binding="{Binding Price }"></DataGridTextColumn>
</DataGrid.Columns>
</DataGrid>
If you going to bind using View model, then provide the Class Name of the Viewmodel instead of Element Binding.
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Here I have created ObservableCollection of Product and Category with name ProductSet & ListOfCategory in the Window level.
public ObservableCollection<Category> ListOfCategory { get; set; }
public ObservableCollection<Product> ProductSet { get; set; }
I have created both the ObservableCollection in the Code behind of the Window, You can create this in view model which is better approch.
I have named the Window as "Window1" x:Name="Window1"
As you need to bind the data context, did the elementBinding with Window1 and also set the ItemsSource="{Binding ProductSet}". So what happens here is the ProuctSet is searched for DataContext, which is in turn searched inside Window1 Class.
For DataGridComboBoxColumn, set the ItemsSource using the Style and search for the Datagrid DataContext which has ListOfCategory using Relative Source.
<DataGrid AutoGenerateColumns="False" x:Name="DataGrid1" HorizontalAlignment="Left" Height="135" Margin="21,288,0,0" VerticalAlignment="Top" Width="729" DataContext="{Binding ElementName=Window1}" ItemsSource="{Binding ProductSet}" >
<DataGrid.Columns>
<DataGridTextColumn Header="ProductID" Width="175" Binding="{Binding ProductID}"></DataGridTextColumn>
<DataGridComboBoxColumn Header="Category" DisplayMemberPath="Name" SelectedValuePath="CategoryID" SelectedItemBinding="{Binding ListOfCategory}">
<DataGridComboBoxColumn.ElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory, RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.ElementStyle>
<DataGridComboBoxColumn.EditingElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory,RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.EditingElementStyle>
</DataGridComboBoxColumn>
<DataGridTextColumn Header="Name" Width="175" Binding="{Binding Name}"></DataGridTextColumn>
<DataGridTextColumn Header="Price " Width="175" Binding="{Binding Price }"></DataGridTextColumn>
</DataGrid.Columns>
</DataGrid>
If you going to bind using View model, then provide the Class Name of the Viewmodel instead of Element Binding.
Here I have created ObservableCollection of Product and Category with name ProductSet & ListOfCategory in the Window level.
public ObservableCollection<Category> ListOfCategory { get; set; }
public ObservableCollection<Product> ProductSet { get; set; }
I have created both the ObservableCollection in the Code behind of the Window, You can create this in view model which is better approch.
I have named the Window as "Window1" x:Name="Window1"
As you need to bind the data context, did the elementBinding with Window1 and also set the ItemsSource="{Binding ProductSet}". So what happens here is the ProuctSet is searched for DataContext, which is in turn searched inside Window1 Class.
For DataGridComboBoxColumn, set the ItemsSource using the Style and search for the Datagrid DataContext which has ListOfCategory using Relative Source.
<DataGrid AutoGenerateColumns="False" x:Name="DataGrid1" HorizontalAlignment="Left" Height="135" Margin="21,288,0,0" VerticalAlignment="Top" Width="729" DataContext="{Binding ElementName=Window1}" ItemsSource="{Binding ProductSet}" >
<DataGrid.Columns>
<DataGridTextColumn Header="ProductID" Width="175" Binding="{Binding ProductID}"></DataGridTextColumn>
<DataGridComboBoxColumn Header="Category" DisplayMemberPath="Name" SelectedValuePath="CategoryID" SelectedItemBinding="{Binding ListOfCategory}">
<DataGridComboBoxColumn.ElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory, RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.ElementStyle>
<DataGridComboBoxColumn.EditingElementStyle>
<Style TargetType="ComboBox">
<Setter Property="ItemsSource" Value="{Binding Path=DataContext.ListOfCategory,RelativeSource={RelativeSource FindAncestor, AncestorType={x:Type DataGrid}}}" />
</Style>
</DataGridComboBoxColumn.EditingElementStyle>
</DataGridComboBoxColumn>
<DataGridTextColumn Header="Name" Width="175" Binding="{Binding Name}"></DataGridTextColumn>
<DataGridTextColumn Header="Price " Width="175" Binding="{Binding Price }"></DataGridTextColumn>
</DataGrid.Columns>
</DataGrid>
If you going to bind using View model, then provide the Class Name of the Viewmodel instead of Element Binding.
answered Nov 13 at 7:38
Satish Pai
1659
1659
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271645%2fwpf-bind-datagrid-column-combobox-from-database-table-using-id%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Aczo9u0PLCFm1Fo90jb9HM5u,K2d8Ty0jGj