Why does cout fail to output strings sometimes, but printf has no problem?
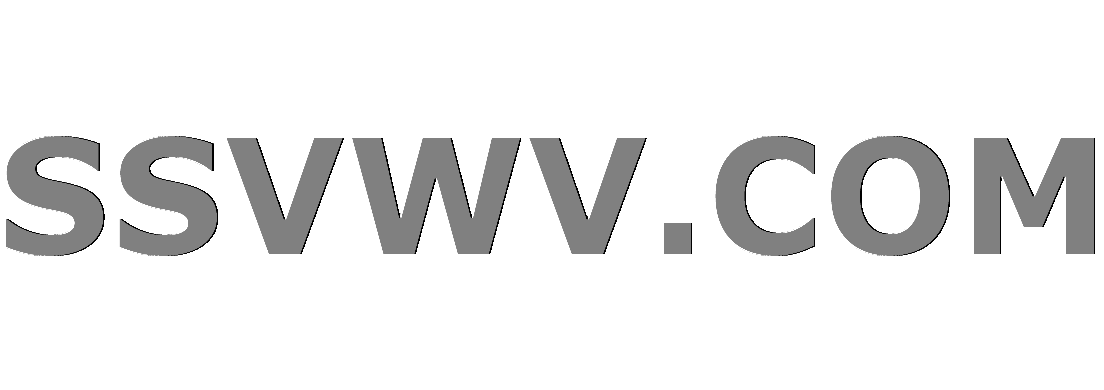
Multi tool use
up vote
0
down vote
favorite
Problem: when printing items in a hash table, non top-level items do not print correctly.
I add items line-by-line from a text file. First, number of lines are determined, and then, the hash table is constructed, with the size found in the first loop of the file.
hash constructor
hash::hash(unsigned int tSize)
{
this->tableSize = tSize;
hashTable.resize(tableSize);
for (int i = 0; i < tableSize; i++)
{
hashTable[i] = new item;
hashTable[i]->firstLetters = "__";
hashTable[i]->str = "____";
hashTable[i]->next = NULL;
}
}
adding items, setting values, and printing them
void hash::add(std::string key)
{
int index = hafn(key);
if (hashTable[index]->str == "____")
{
hashTable[index]->str = key;
setFirstLetters(index,key[0],key[1]);
}
else
{
item *iter = hashTable[index];
item *n_ptr = new item;
n_ptr->str = key;
setFirstLetters(n_ptr, key[0], key[1]);
n_ptr->next = NULL;
while (iter->next != NULL)
{
iter = iter->next;
}
iter->next = n_ptr;
}
}
void hash::setFirstLetters(item *n_ptr, char a, char b)
{
n_ptr->firstLetters[0] = a;
n_ptr->firstLetters[1] = b;
}
void hash::setFirstLetters(int index, char a, char b)
{
hashTable[index]->firstLetters[0] = a;
hashTable[index]->firstLetters[1] = b;
}
void hash::print()
{
int num;
for (int i = 0; i < tableSize; i++)
{
num = numInIndex(i);
printer(num, i);
if (num > 1)
{
int c = 0;
for (int j = num - 1; j > 0; j--)
{
printer(c, num, i);
c++;
}
}
}
}
void hash::printer(int num, int i)
{
item *iter = hashTable[i];
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << iter->firstLetters << endl;
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
void hash::printer(int numIn, int num, int i)
{
item *iter = hashTable[i];
for (int j = 0; j < numIn; j++)
{
iter = iter->next;
}
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << std::flush;
cout << iter->firstLetters << endl; //this does not work, though the pointer is pointing to the correct values
//printf("%sn", iter->firstLetters.c_str()); //this works, even without flushing
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
struct item
{
std::string str;
std::string firstLetters;
item *next;
};
The problem is that firstLetters
does not print correctly. firstLetters
is set correctly. However, in third- and later level items (ie, using the same hash index), firstLetters
does not print at all.
In order to be more clear, here is an output example:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
# of items = 3
-------------------------------
A correct example of output would be this:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
go
# of items = 3
-------------------------------
c++ hash printf cout string-hashing
|
show 11 more comments
up vote
0
down vote
favorite
Problem: when printing items in a hash table, non top-level items do not print correctly.
I add items line-by-line from a text file. First, number of lines are determined, and then, the hash table is constructed, with the size found in the first loop of the file.
hash constructor
hash::hash(unsigned int tSize)
{
this->tableSize = tSize;
hashTable.resize(tableSize);
for (int i = 0; i < tableSize; i++)
{
hashTable[i] = new item;
hashTable[i]->firstLetters = "__";
hashTable[i]->str = "____";
hashTable[i]->next = NULL;
}
}
adding items, setting values, and printing them
void hash::add(std::string key)
{
int index = hafn(key);
if (hashTable[index]->str == "____")
{
hashTable[index]->str = key;
setFirstLetters(index,key[0],key[1]);
}
else
{
item *iter = hashTable[index];
item *n_ptr = new item;
n_ptr->str = key;
setFirstLetters(n_ptr, key[0], key[1]);
n_ptr->next = NULL;
while (iter->next != NULL)
{
iter = iter->next;
}
iter->next = n_ptr;
}
}
void hash::setFirstLetters(item *n_ptr, char a, char b)
{
n_ptr->firstLetters[0] = a;
n_ptr->firstLetters[1] = b;
}
void hash::setFirstLetters(int index, char a, char b)
{
hashTable[index]->firstLetters[0] = a;
hashTable[index]->firstLetters[1] = b;
}
void hash::print()
{
int num;
for (int i = 0; i < tableSize; i++)
{
num = numInIndex(i);
printer(num, i);
if (num > 1)
{
int c = 0;
for (int j = num - 1; j > 0; j--)
{
printer(c, num, i);
c++;
}
}
}
}
void hash::printer(int num, int i)
{
item *iter = hashTable[i];
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << iter->firstLetters << endl;
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
void hash::printer(int numIn, int num, int i)
{
item *iter = hashTable[i];
for (int j = 0; j < numIn; j++)
{
iter = iter->next;
}
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << std::flush;
cout << iter->firstLetters << endl; //this does not work, though the pointer is pointing to the correct values
//printf("%sn", iter->firstLetters.c_str()); //this works, even without flushing
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
struct item
{
std::string str;
std::string firstLetters;
item *next;
};
The problem is that firstLetters
does not print correctly. firstLetters
is set correctly. However, in third- and later level items (ie, using the same hash index), firstLetters
does not print at all.
In order to be more clear, here is an output example:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
# of items = 3
-------------------------------
A correct example of output would be this:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
go
# of items = 3
-------------------------------
c++ hash printf cout string-hashing
I have been using the debugger continually. On the line thatfirstLetters
is printed, that variable is correct insidestruct item
– Adam
Nov 12 at 23:42
You seem to be expecting us to debug your uncompilable code.
– Neil Butterworth
Nov 12 at 23:44
I am not. The code does compile. I haven't given every single piece of code, only the relevant ones. I just need help on situations thatcout
will not print, even though the output stream has been flushed.
– Adam
Nov 12 at 23:45
2
Bug in there somewhere that isn't jumping out at me. Could we trouble you for a Minimal, Complete, and Verifiable example? This is important because if you don't know what the bug is, how can you know what is and what isn't relevant? The real beauty of the MCVE is usually you get in part way and the reduced code to bug ratio reveals the bug to you.
– user4581301
Nov 12 at 23:46
1
@Adam You are initializingfirstLetters = "__"
only for the initial top-levelitem
objects that thehash
constructor creates, but you are NOT initializingfirstLetters
to anything in the secondaryitem
objects thathash::add()
creates, son_ptr->firstLetters
is blank whensetFirstLetters(n_ptr, ...)
is called, sosetFirstLetters()
tries to index into invalid memory. If you wantfirstLetters
to alows be initialized as"__", you should add a constructor to the
item` struct for that.
– Remy Lebeau
Nov 13 at 0:14
|
show 11 more comments
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Problem: when printing items in a hash table, non top-level items do not print correctly.
I add items line-by-line from a text file. First, number of lines are determined, and then, the hash table is constructed, with the size found in the first loop of the file.
hash constructor
hash::hash(unsigned int tSize)
{
this->tableSize = tSize;
hashTable.resize(tableSize);
for (int i = 0; i < tableSize; i++)
{
hashTable[i] = new item;
hashTable[i]->firstLetters = "__";
hashTable[i]->str = "____";
hashTable[i]->next = NULL;
}
}
adding items, setting values, and printing them
void hash::add(std::string key)
{
int index = hafn(key);
if (hashTable[index]->str == "____")
{
hashTable[index]->str = key;
setFirstLetters(index,key[0],key[1]);
}
else
{
item *iter = hashTable[index];
item *n_ptr = new item;
n_ptr->str = key;
setFirstLetters(n_ptr, key[0], key[1]);
n_ptr->next = NULL;
while (iter->next != NULL)
{
iter = iter->next;
}
iter->next = n_ptr;
}
}
void hash::setFirstLetters(item *n_ptr, char a, char b)
{
n_ptr->firstLetters[0] = a;
n_ptr->firstLetters[1] = b;
}
void hash::setFirstLetters(int index, char a, char b)
{
hashTable[index]->firstLetters[0] = a;
hashTable[index]->firstLetters[1] = b;
}
void hash::print()
{
int num;
for (int i = 0; i < tableSize; i++)
{
num = numInIndex(i);
printer(num, i);
if (num > 1)
{
int c = 0;
for (int j = num - 1; j > 0; j--)
{
printer(c, num, i);
c++;
}
}
}
}
void hash::printer(int num, int i)
{
item *iter = hashTable[i];
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << iter->firstLetters << endl;
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
void hash::printer(int numIn, int num, int i)
{
item *iter = hashTable[i];
for (int j = 0; j < numIn; j++)
{
iter = iter->next;
}
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << std::flush;
cout << iter->firstLetters << endl; //this does not work, though the pointer is pointing to the correct values
//printf("%sn", iter->firstLetters.c_str()); //this works, even without flushing
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
struct item
{
std::string str;
std::string firstLetters;
item *next;
};
The problem is that firstLetters
does not print correctly. firstLetters
is set correctly. However, in third- and later level items (ie, using the same hash index), firstLetters
does not print at all.
In order to be more clear, here is an output example:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
# of items = 3
-------------------------------
A correct example of output would be this:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
go
# of items = 3
-------------------------------
c++ hash printf cout string-hashing
Problem: when printing items in a hash table, non top-level items do not print correctly.
I add items line-by-line from a text file. First, number of lines are determined, and then, the hash table is constructed, with the size found in the first loop of the file.
hash constructor
hash::hash(unsigned int tSize)
{
this->tableSize = tSize;
hashTable.resize(tableSize);
for (int i = 0; i < tableSize; i++)
{
hashTable[i] = new item;
hashTable[i]->firstLetters = "__";
hashTable[i]->str = "____";
hashTable[i]->next = NULL;
}
}
adding items, setting values, and printing them
void hash::add(std::string key)
{
int index = hafn(key);
if (hashTable[index]->str == "____")
{
hashTable[index]->str = key;
setFirstLetters(index,key[0],key[1]);
}
else
{
item *iter = hashTable[index];
item *n_ptr = new item;
n_ptr->str = key;
setFirstLetters(n_ptr, key[0], key[1]);
n_ptr->next = NULL;
while (iter->next != NULL)
{
iter = iter->next;
}
iter->next = n_ptr;
}
}
void hash::setFirstLetters(item *n_ptr, char a, char b)
{
n_ptr->firstLetters[0] = a;
n_ptr->firstLetters[1] = b;
}
void hash::setFirstLetters(int index, char a, char b)
{
hashTable[index]->firstLetters[0] = a;
hashTable[index]->firstLetters[1] = b;
}
void hash::print()
{
int num;
for (int i = 0; i < tableSize; i++)
{
num = numInIndex(i);
printer(num, i);
if (num > 1)
{
int c = 0;
for (int j = num - 1; j > 0; j--)
{
printer(c, num, i);
c++;
}
}
}
}
void hash::printer(int num, int i)
{
item *iter = hashTable[i];
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << iter->firstLetters << endl;
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
void hash::printer(int numIn, int num, int i)
{
item *iter = hashTable[i];
for (int j = 0; j < numIn; j++)
{
iter = iter->next;
}
cout << "-------------------------------" << endl;
cout << "index = " << i << endl;
cout << iter->str << endl;
cout << std::flush;
cout << iter->firstLetters << endl; //this does not work, though the pointer is pointing to the correct values
//printf("%sn", iter->firstLetters.c_str()); //this works, even without flushing
cout << "# of items = " << num << endl;
cout << "-------------------------------" << endl;
cout << endl;
}
struct item
{
std::string str;
std::string firstLetters;
item *next;
};
The problem is that firstLetters
does not print correctly. firstLetters
is set correctly. However, in third- and later level items (ie, using the same hash index), firstLetters
does not print at all.
In order to be more clear, here is an output example:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
# of items = 3
-------------------------------
A correct example of output would be this:
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
will
wi
# of items = 3
-------------------------------
-------------------------------
index = 15
good
go
# of items = 3
-------------------------------
c++ hash printf cout string-hashing
c++ hash printf cout string-hashing
edited Nov 13 at 0:28
asked Nov 12 at 23:33
Adam
125
125
I have been using the debugger continually. On the line thatfirstLetters
is printed, that variable is correct insidestruct item
– Adam
Nov 12 at 23:42
You seem to be expecting us to debug your uncompilable code.
– Neil Butterworth
Nov 12 at 23:44
I am not. The code does compile. I haven't given every single piece of code, only the relevant ones. I just need help on situations thatcout
will not print, even though the output stream has been flushed.
– Adam
Nov 12 at 23:45
2
Bug in there somewhere that isn't jumping out at me. Could we trouble you for a Minimal, Complete, and Verifiable example? This is important because if you don't know what the bug is, how can you know what is and what isn't relevant? The real beauty of the MCVE is usually you get in part way and the reduced code to bug ratio reveals the bug to you.
– user4581301
Nov 12 at 23:46
1
@Adam You are initializingfirstLetters = "__"
only for the initial top-levelitem
objects that thehash
constructor creates, but you are NOT initializingfirstLetters
to anything in the secondaryitem
objects thathash::add()
creates, son_ptr->firstLetters
is blank whensetFirstLetters(n_ptr, ...)
is called, sosetFirstLetters()
tries to index into invalid memory. If you wantfirstLetters
to alows be initialized as"__", you should add a constructor to the
item` struct for that.
– Remy Lebeau
Nov 13 at 0:14
|
show 11 more comments
I have been using the debugger continually. On the line thatfirstLetters
is printed, that variable is correct insidestruct item
– Adam
Nov 12 at 23:42
You seem to be expecting us to debug your uncompilable code.
– Neil Butterworth
Nov 12 at 23:44
I am not. The code does compile. I haven't given every single piece of code, only the relevant ones. I just need help on situations thatcout
will not print, even though the output stream has been flushed.
– Adam
Nov 12 at 23:45
2
Bug in there somewhere that isn't jumping out at me. Could we trouble you for a Minimal, Complete, and Verifiable example? This is important because if you don't know what the bug is, how can you know what is and what isn't relevant? The real beauty of the MCVE is usually you get in part way and the reduced code to bug ratio reveals the bug to you.
– user4581301
Nov 12 at 23:46
1
@Adam You are initializingfirstLetters = "__"
only for the initial top-levelitem
objects that thehash
constructor creates, but you are NOT initializingfirstLetters
to anything in the secondaryitem
objects thathash::add()
creates, son_ptr->firstLetters
is blank whensetFirstLetters(n_ptr, ...)
is called, sosetFirstLetters()
tries to index into invalid memory. If you wantfirstLetters
to alows be initialized as"__", you should add a constructor to the
item` struct for that.
– Remy Lebeau
Nov 13 at 0:14
I have been using the debugger continually. On the line that
firstLetters
is printed, that variable is correct inside struct item
– Adam
Nov 12 at 23:42
I have been using the debugger continually. On the line that
firstLetters
is printed, that variable is correct inside struct item
– Adam
Nov 12 at 23:42
You seem to be expecting us to debug your uncompilable code.
– Neil Butterworth
Nov 12 at 23:44
You seem to be expecting us to debug your uncompilable code.
– Neil Butterworth
Nov 12 at 23:44
I am not. The code does compile. I haven't given every single piece of code, only the relevant ones. I just need help on situations that
cout
will not print, even though the output stream has been flushed.– Adam
Nov 12 at 23:45
I am not. The code does compile. I haven't given every single piece of code, only the relevant ones. I just need help on situations that
cout
will not print, even though the output stream has been flushed.– Adam
Nov 12 at 23:45
2
2
Bug in there somewhere that isn't jumping out at me. Could we trouble you for a Minimal, Complete, and Verifiable example? This is important because if you don't know what the bug is, how can you know what is and what isn't relevant? The real beauty of the MCVE is usually you get in part way and the reduced code to bug ratio reveals the bug to you.
– user4581301
Nov 12 at 23:46
Bug in there somewhere that isn't jumping out at me. Could we trouble you for a Minimal, Complete, and Verifiable example? This is important because if you don't know what the bug is, how can you know what is and what isn't relevant? The real beauty of the MCVE is usually you get in part way and the reduced code to bug ratio reveals the bug to you.
– user4581301
Nov 12 at 23:46
1
1
@Adam You are initializing
firstLetters = "__"
only for the initial top-level item
objects that the hash
constructor creates, but you are NOT initializing firstLetters
to anything in the secondary item
objects that hash::add()
creates, so n_ptr->firstLetters
is blank when setFirstLetters(n_ptr, ...)
is called, so setFirstLetters()
tries to index into invalid memory. If you want firstLetters
to alows be initialized as "__", you should add a constructor to the
item` struct for that.– Remy Lebeau
Nov 13 at 0:14
@Adam You are initializing
firstLetters = "__"
only for the initial top-level item
objects that the hash
constructor creates, but you are NOT initializing firstLetters
to anything in the secondary item
objects that hash::add()
creates, so n_ptr->firstLetters
is blank when setFirstLetters(n_ptr, ...)
is called, so setFirstLetters()
tries to index into invalid memory. If you want firstLetters
to alows be initialized as "__", you should add a constructor to the
item` struct for that.– Remy Lebeau
Nov 13 at 0:14
|
show 11 more comments
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271641%2fwhy-does-cout-fail-to-output-strings-sometimes-but-printf-has-no-problem%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oeji,inXIqwLCu6Dw4N4EWT6SBcuD4
I have been using the debugger continually. On the line that
firstLetters
is printed, that variable is correct insidestruct item
– Adam
Nov 12 at 23:42
You seem to be expecting us to debug your uncompilable code.
– Neil Butterworth
Nov 12 at 23:44
I am not. The code does compile. I haven't given every single piece of code, only the relevant ones. I just need help on situations that
cout
will not print, even though the output stream has been flushed.– Adam
Nov 12 at 23:45
2
Bug in there somewhere that isn't jumping out at me. Could we trouble you for a Minimal, Complete, and Verifiable example? This is important because if you don't know what the bug is, how can you know what is and what isn't relevant? The real beauty of the MCVE is usually you get in part way and the reduced code to bug ratio reveals the bug to you.
– user4581301
Nov 12 at 23:46
1
@Adam You are initializing
firstLetters = "__"
only for the initial top-levelitem
objects that thehash
constructor creates, but you are NOT initializingfirstLetters
to anything in the secondaryitem
objects thathash::add()
creates, son_ptr->firstLetters
is blank whensetFirstLetters(n_ptr, ...)
is called, sosetFirstLetters()
tries to index into invalid memory. If you wantfirstLetters
to alows be initialized as"__", you should add a constructor to the
item` struct for that.– Remy Lebeau
Nov 13 at 0:14