Issues developing Fibonacci Search algorithm with R.
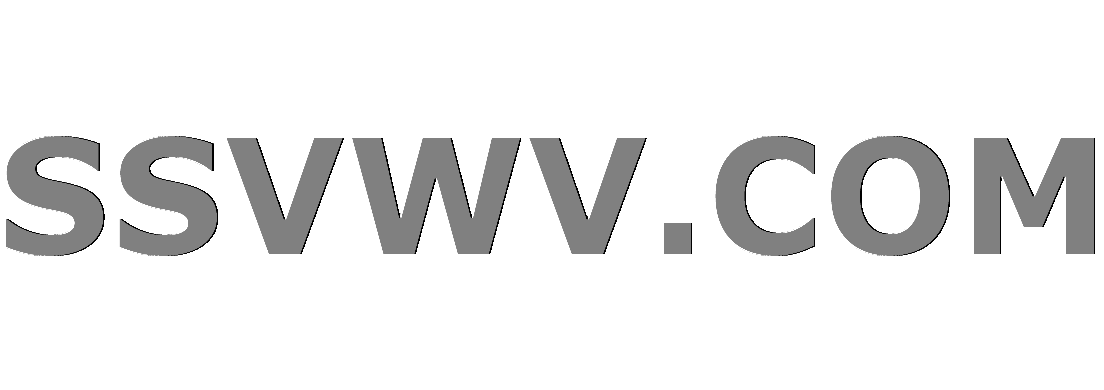
Multi tool use
up vote
0
down vote
favorite
[edited] I compiled this code for an Operations Research class. I ended up submitting another format that relied on R packages; however, I feel that I am close to a functioning algorithm.
I derived this code from another R program I complied for the 'Golden Search' algorithm. That program conducted 'bracket' searches until it found the optimal min/max. Each time I run this algorithm, it fails to conduct a 'bracket' search. After multiple hours, I have failed to find the issue. Any help finding the error in my code would be appreciated.
My submitted (and confirmed) algorithm returned a minimum (x) value of 0.8095 with a f(x) value of -24.3445 utilizing a Fibonacci search method.
# Step One: Initialization
## Input user-defined function
f = function(x)
{
(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))
}
## Input user-defined parameters
Lb <- 0 ### Lower Bound
Ub <- 2 ### Upper Bound
Tl <- .3 ### Tolerance
n <- (Ub-Lb)/Tl
n<- as.integer(n)
fibonacci.search = function(f, lower.bound, upper.bound, tolerance)
{
iteration = 0
Fn = fibonacci(n, sequence = FALSE)
### Use the fibonacci ratios to set the initial test points
x1 = lower.bound + ((fib(n-2)/fib(n)) * (upper.bound - lower.bound))
x2 = lower.bound + ((fib(n-1)/fib(n)) * (upper.bound))
### Evaluate the function at the test points
f1 = f(x1)
f2 = f(x2)
while ((lower.bound - upper.bound) > tolerance)
{
iteration = iteration + 1
n = n - 1
cat('', 'n')
cat('Iteration #', iteration, 'n')
cat('f1 =', f1, 'n')
cat('f2 =', f2, 'n')
if (f1 < f2)
{
cat('f(x1) < f(x2)', 'n')
# If f(x1) < f(x2), then the new interval is [x1,b]:
# a becomes the previous x1, b does not change
lower.bound = x1
upper.bound = upper.bound
# x1 becomes the previous x2, n = n-1
x1 = x2
f1 = f2
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Upper Test Point = ', x1, 'n')
### Find the new x2 using the formula in Step2.
x2 = lower.bound + (fib(n-1)/fib(n)) * (upper.bound)
cat('New Lower Test Point = ', x1, 'n')
f2 = f(x2)
}
else
{
cat('f(x1) > f(x2)', 'n')
# If f(x1) > f(x2), then the new interval is [a,x2]:
# a does not change, b becomes the previous x2
upper.bound = x2
lower.bound = lower.bound
# x2 becomes the previous x1, n = n-1
x2 = x1
f2 = f1
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Lower Test Point = ', x1, 'n')
# Find the new x1 using the formula in Step2.
x1 = lower.bound + (fib(n-2)/fib(n)) * (upper.bound - lower.bound)
cat('New Upper Test Point = ', x2, 'n')
f1 = f(x1)
}
}
### Use the mid-point of the final interval as the estimate of the optimzer
cat('', 'n')
cat('Final Lower Bound =', lower.bound, 'n')
cat('Final Upper Bound =', upper.bound, 'n')
estimated.minimizer = (lower.bound + upper.bound)/2
cat('Estimated Minimum Value (x) =', estimated.minimizer, 'n')
cat('Estimated F(x)', f(estimated.minimizer),'n')
# Step Three: Results
## Plot the curve of the user-defined function
curve(f, from = Lb, to = Ub, main = expression("(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))"))
## Plot the solution as a point
points(estimated.minimizer, f(estimated.minimizer), col="red", pch=19)
## Draw a square around the optima to highlight it
rect(estimated.minimizer-0.1, f(estimated.minimizer)-01, estimated.minimizer+0.1, f(estimated.minimizer)+01, lwd=2)
}
# Step Two: Loop Function
fibonacci.search(f, Lb, Ub, Tl)
optimization fibonacci-numbers programming
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
[edited] I compiled this code for an Operations Research class. I ended up submitting another format that relied on R packages; however, I feel that I am close to a functioning algorithm.
I derived this code from another R program I complied for the 'Golden Search' algorithm. That program conducted 'bracket' searches until it found the optimal min/max. Each time I run this algorithm, it fails to conduct a 'bracket' search. After multiple hours, I have failed to find the issue. Any help finding the error in my code would be appreciated.
My submitted (and confirmed) algorithm returned a minimum (x) value of 0.8095 with a f(x) value of -24.3445 utilizing a Fibonacci search method.
# Step One: Initialization
## Input user-defined function
f = function(x)
{
(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))
}
## Input user-defined parameters
Lb <- 0 ### Lower Bound
Ub <- 2 ### Upper Bound
Tl <- .3 ### Tolerance
n <- (Ub-Lb)/Tl
n<- as.integer(n)
fibonacci.search = function(f, lower.bound, upper.bound, tolerance)
{
iteration = 0
Fn = fibonacci(n, sequence = FALSE)
### Use the fibonacci ratios to set the initial test points
x1 = lower.bound + ((fib(n-2)/fib(n)) * (upper.bound - lower.bound))
x2 = lower.bound + ((fib(n-1)/fib(n)) * (upper.bound))
### Evaluate the function at the test points
f1 = f(x1)
f2 = f(x2)
while ((lower.bound - upper.bound) > tolerance)
{
iteration = iteration + 1
n = n - 1
cat('', 'n')
cat('Iteration #', iteration, 'n')
cat('f1 =', f1, 'n')
cat('f2 =', f2, 'n')
if (f1 < f2)
{
cat('f(x1) < f(x2)', 'n')
# If f(x1) < f(x2), then the new interval is [x1,b]:
# a becomes the previous x1, b does not change
lower.bound = x1
upper.bound = upper.bound
# x1 becomes the previous x2, n = n-1
x1 = x2
f1 = f2
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Upper Test Point = ', x1, 'n')
### Find the new x2 using the formula in Step2.
x2 = lower.bound + (fib(n-1)/fib(n)) * (upper.bound)
cat('New Lower Test Point = ', x1, 'n')
f2 = f(x2)
}
else
{
cat('f(x1) > f(x2)', 'n')
# If f(x1) > f(x2), then the new interval is [a,x2]:
# a does not change, b becomes the previous x2
upper.bound = x2
lower.bound = lower.bound
# x2 becomes the previous x1, n = n-1
x2 = x1
f2 = f1
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Lower Test Point = ', x1, 'n')
# Find the new x1 using the formula in Step2.
x1 = lower.bound + (fib(n-2)/fib(n)) * (upper.bound - lower.bound)
cat('New Upper Test Point = ', x2, 'n')
f1 = f(x1)
}
}
### Use the mid-point of the final interval as the estimate of the optimzer
cat('', 'n')
cat('Final Lower Bound =', lower.bound, 'n')
cat('Final Upper Bound =', upper.bound, 'n')
estimated.minimizer = (lower.bound + upper.bound)/2
cat('Estimated Minimum Value (x) =', estimated.minimizer, 'n')
cat('Estimated F(x)', f(estimated.minimizer),'n')
# Step Three: Results
## Plot the curve of the user-defined function
curve(f, from = Lb, to = Ub, main = expression("(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))"))
## Plot the solution as a point
points(estimated.minimizer, f(estimated.minimizer), col="red", pch=19)
## Draw a square around the optima to highlight it
rect(estimated.minimizer-0.1, f(estimated.minimizer)-01, estimated.minimizer+0.1, f(estimated.minimizer)+01, lwd=2)
}
# Step Two: Loop Function
fibonacci.search(f, Lb, Ub, Tl)
optimization fibonacci-numbers programming
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
[edited] I compiled this code for an Operations Research class. I ended up submitting another format that relied on R packages; however, I feel that I am close to a functioning algorithm.
I derived this code from another R program I complied for the 'Golden Search' algorithm. That program conducted 'bracket' searches until it found the optimal min/max. Each time I run this algorithm, it fails to conduct a 'bracket' search. After multiple hours, I have failed to find the issue. Any help finding the error in my code would be appreciated.
My submitted (and confirmed) algorithm returned a minimum (x) value of 0.8095 with a f(x) value of -24.3445 utilizing a Fibonacci search method.
# Step One: Initialization
## Input user-defined function
f = function(x)
{
(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))
}
## Input user-defined parameters
Lb <- 0 ### Lower Bound
Ub <- 2 ### Upper Bound
Tl <- .3 ### Tolerance
n <- (Ub-Lb)/Tl
n<- as.integer(n)
fibonacci.search = function(f, lower.bound, upper.bound, tolerance)
{
iteration = 0
Fn = fibonacci(n, sequence = FALSE)
### Use the fibonacci ratios to set the initial test points
x1 = lower.bound + ((fib(n-2)/fib(n)) * (upper.bound - lower.bound))
x2 = lower.bound + ((fib(n-1)/fib(n)) * (upper.bound))
### Evaluate the function at the test points
f1 = f(x1)
f2 = f(x2)
while ((lower.bound - upper.bound) > tolerance)
{
iteration = iteration + 1
n = n - 1
cat('', 'n')
cat('Iteration #', iteration, 'n')
cat('f1 =', f1, 'n')
cat('f2 =', f2, 'n')
if (f1 < f2)
{
cat('f(x1) < f(x2)', 'n')
# If f(x1) < f(x2), then the new interval is [x1,b]:
# a becomes the previous x1, b does not change
lower.bound = x1
upper.bound = upper.bound
# x1 becomes the previous x2, n = n-1
x1 = x2
f1 = f2
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Upper Test Point = ', x1, 'n')
### Find the new x2 using the formula in Step2.
x2 = lower.bound + (fib(n-1)/fib(n)) * (upper.bound)
cat('New Lower Test Point = ', x1, 'n')
f2 = f(x2)
}
else
{
cat('f(x1) > f(x2)', 'n')
# If f(x1) > f(x2), then the new interval is [a,x2]:
# a does not change, b becomes the previous x2
upper.bound = x2
lower.bound = lower.bound
# x2 becomes the previous x1, n = n-1
x2 = x1
f2 = f1
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Lower Test Point = ', x1, 'n')
# Find the new x1 using the formula in Step2.
x1 = lower.bound + (fib(n-2)/fib(n)) * (upper.bound - lower.bound)
cat('New Upper Test Point = ', x2, 'n')
f1 = f(x1)
}
}
### Use the mid-point of the final interval as the estimate of the optimzer
cat('', 'n')
cat('Final Lower Bound =', lower.bound, 'n')
cat('Final Upper Bound =', upper.bound, 'n')
estimated.minimizer = (lower.bound + upper.bound)/2
cat('Estimated Minimum Value (x) =', estimated.minimizer, 'n')
cat('Estimated F(x)', f(estimated.minimizer),'n')
# Step Three: Results
## Plot the curve of the user-defined function
curve(f, from = Lb, to = Ub, main = expression("(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))"))
## Plot the solution as a point
points(estimated.minimizer, f(estimated.minimizer), col="red", pch=19)
## Draw a square around the optima to highlight it
rect(estimated.minimizer-0.1, f(estimated.minimizer)-01, estimated.minimizer+0.1, f(estimated.minimizer)+01, lwd=2)
}
# Step Two: Loop Function
fibonacci.search(f, Lb, Ub, Tl)
optimization fibonacci-numbers programming
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
[edited] I compiled this code for an Operations Research class. I ended up submitting another format that relied on R packages; however, I feel that I am close to a functioning algorithm.
I derived this code from another R program I complied for the 'Golden Search' algorithm. That program conducted 'bracket' searches until it found the optimal min/max. Each time I run this algorithm, it fails to conduct a 'bracket' search. After multiple hours, I have failed to find the issue. Any help finding the error in my code would be appreciated.
My submitted (and confirmed) algorithm returned a minimum (x) value of 0.8095 with a f(x) value of -24.3445 utilizing a Fibonacci search method.
# Step One: Initialization
## Input user-defined function
f = function(x)
{
(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))
}
## Input user-defined parameters
Lb <- 0 ### Lower Bound
Ub <- 2 ### Upper Bound
Tl <- .3 ### Tolerance
n <- (Ub-Lb)/Tl
n<- as.integer(n)
fibonacci.search = function(f, lower.bound, upper.bound, tolerance)
{
iteration = 0
Fn = fibonacci(n, sequence = FALSE)
### Use the fibonacci ratios to set the initial test points
x1 = lower.bound + ((fib(n-2)/fib(n)) * (upper.bound - lower.bound))
x2 = lower.bound + ((fib(n-1)/fib(n)) * (upper.bound))
### Evaluate the function at the test points
f1 = f(x1)
f2 = f(x2)
while ((lower.bound - upper.bound) > tolerance)
{
iteration = iteration + 1
n = n - 1
cat('', 'n')
cat('Iteration #', iteration, 'n')
cat('f1 =', f1, 'n')
cat('f2 =', f2, 'n')
if (f1 < f2)
{
cat('f(x1) < f(x2)', 'n')
# If f(x1) < f(x2), then the new interval is [x1,b]:
# a becomes the previous x1, b does not change
lower.bound = x1
upper.bound = upper.bound
# x1 becomes the previous x2, n = n-1
x1 = x2
f1 = f2
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Upper Test Point = ', x1, 'n')
### Find the new x2 using the formula in Step2.
x2 = lower.bound + (fib(n-1)/fib(n)) * (upper.bound)
cat('New Lower Test Point = ', x1, 'n')
f2 = f(x2)
}
else
{
cat('f(x1) > f(x2)', 'n')
# If f(x1) > f(x2), then the new interval is [a,x2]:
# a does not change, b becomes the previous x2
upper.bound = x2
lower.bound = lower.bound
# x2 becomes the previous x1, n = n-1
x2 = x1
f2 = f1
cat('New Upper Bound =', upper.bound, 'n')
cat('New Lower Bound =', lower.bound, 'n')
cat('New Lower Test Point = ', x1, 'n')
# Find the new x1 using the formula in Step2.
x1 = lower.bound + (fib(n-2)/fib(n)) * (upper.bound - lower.bound)
cat('New Upper Test Point = ', x2, 'n')
f1 = f(x1)
}
}
### Use the mid-point of the final interval as the estimate of the optimzer
cat('', 'n')
cat('Final Lower Bound =', lower.bound, 'n')
cat('Final Upper Bound =', upper.bound, 'n')
estimated.minimizer = (lower.bound + upper.bound)/2
cat('Estimated Minimum Value (x) =', estimated.minimizer, 'n')
cat('Estimated F(x)', f(estimated.minimizer),'n')
# Step Three: Results
## Plot the curve of the user-defined function
curve(f, from = Lb, to = Ub, main = expression("(x^4)-(14*(x^3))+(60*(x^2))-(70*(x))"))
## Plot the solution as a point
points(estimated.minimizer, f(estimated.minimizer), col="red", pch=19)
## Draw a square around the optima to highlight it
rect(estimated.minimizer-0.1, f(estimated.minimizer)-01, estimated.minimizer+0.1, f(estimated.minimizer)+01, lwd=2)
}
# Step Two: Loop Function
fibonacci.search(f, Lb, Ub, Tl)
optimization fibonacci-numbers programming
optimization fibonacci-numbers programming
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
edited yesterday
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 9 at 22:54
Josh K
11
11
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Josh K is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Josh K is a new contributor. Be nice, and check out our Code of Conduct.
Josh K is a new contributor. Be nice, and check out our Code of Conduct.
Josh K is a new contributor. Be nice, and check out our Code of Conduct.
Josh K is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fmath.stackexchange.com%2fquestions%2f2992046%2fissues-developing-fibonacci-search-algorithm-with-r%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
e2zrkbG 5dkXu,2wzTHog7sK7I2WS5wqasToIHWHxOPrube,EBdYAfJKD22