How to send CORS request from React?
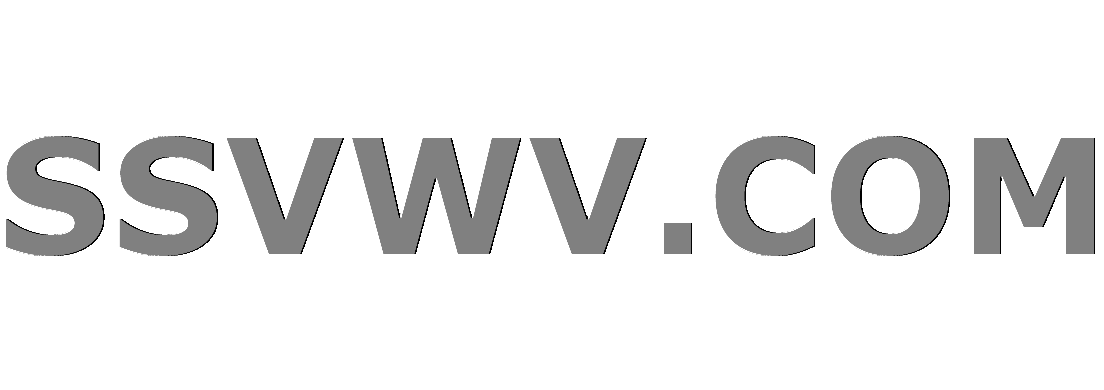
Multi tool use
up vote
0
down vote
favorite
I have problem with sending CORS request with token in header.
Fetch code:
fetchApi() {
fetch('http://some-link.com',
{
headers: {
'Accept': 'application/json',
'auth-token': 'xxxxxxxxx'
},
method: "GET"
}).then(response => response.json())
.then(function(response){
this.setState({hits:response});
}).catch(function(error) { console.log(error); });
console.log(this.state.hits);
};
Console log:
Access to fetch at 'http://some-link.com' from origin 'http://localhost:3000' has been blocked by CORS policy: Request header field auth-token is not allowed by Access-Control-Allow-Headers in preflight response.
Network request log:
General
Request URL: http://some-link.com
Request Method: OPTIONS
Status Code: 200 OK
Remote Address: some_ip:80
Referrer Policy: no-referrer-when-downgrade
Response Headers
Access-Control-Allow-Headers: origin, x-requested-with, content-type, auth_token
Access-Control-Allow-Methods: POST, GET, PUT, DELETE, OPTIONS
Access-Control-Allow-Origin: *
Cache-Control: no-cache, private
Connection: Keep-Alive
Content-Length: 8
Content-Type: application/json
Date: Tue, 13 Nov 2018 13:30:35 GMT
Keep-Alive: timeout=5, max=100
Server: Apache/2.4.10 (Debian)
Request Headers
Provisional headers are shown
Access-Control-Request-Headers: auth-token
Access-Control-Request-Method: GET
Origin: http://localhost:3000
Referer: http://localhost:3000/
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/70.0.3538.77 Safari/537.36
Any ideas what might be wrong?
reactjs http
add a comment |
up vote
0
down vote
favorite
I have problem with sending CORS request with token in header.
Fetch code:
fetchApi() {
fetch('http://some-link.com',
{
headers: {
'Accept': 'application/json',
'auth-token': 'xxxxxxxxx'
},
method: "GET"
}).then(response => response.json())
.then(function(response){
this.setState({hits:response});
}).catch(function(error) { console.log(error); });
console.log(this.state.hits);
};
Console log:
Access to fetch at 'http://some-link.com' from origin 'http://localhost:3000' has been blocked by CORS policy: Request header field auth-token is not allowed by Access-Control-Allow-Headers in preflight response.
Network request log:
General
Request URL: http://some-link.com
Request Method: OPTIONS
Status Code: 200 OK
Remote Address: some_ip:80
Referrer Policy: no-referrer-when-downgrade
Response Headers
Access-Control-Allow-Headers: origin, x-requested-with, content-type, auth_token
Access-Control-Allow-Methods: POST, GET, PUT, DELETE, OPTIONS
Access-Control-Allow-Origin: *
Cache-Control: no-cache, private
Connection: Keep-Alive
Content-Length: 8
Content-Type: application/json
Date: Tue, 13 Nov 2018 13:30:35 GMT
Keep-Alive: timeout=5, max=100
Server: Apache/2.4.10 (Debian)
Request Headers
Provisional headers are shown
Access-Control-Request-Headers: auth-token
Access-Control-Request-Method: GET
Origin: http://localhost:3000
Referer: http://localhost:3000/
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/70.0.3538.77 Safari/537.36
Any ideas what might be wrong?
reactjs http
The same way as you would from anywhere but React. There's nothing in React that would be responsible for HTTP requests. It's auth_token vs auth-token header.
– estus
Nov 13 at 13:51
Fetch is a PITA, it's very low level and you have to do a lot of the work yourself. You can use something like axios to get a fully feature HTTP Client.
– AndyJ
Nov 13 at 14:09
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have problem with sending CORS request with token in header.
Fetch code:
fetchApi() {
fetch('http://some-link.com',
{
headers: {
'Accept': 'application/json',
'auth-token': 'xxxxxxxxx'
},
method: "GET"
}).then(response => response.json())
.then(function(response){
this.setState({hits:response});
}).catch(function(error) { console.log(error); });
console.log(this.state.hits);
};
Console log:
Access to fetch at 'http://some-link.com' from origin 'http://localhost:3000' has been blocked by CORS policy: Request header field auth-token is not allowed by Access-Control-Allow-Headers in preflight response.
Network request log:
General
Request URL: http://some-link.com
Request Method: OPTIONS
Status Code: 200 OK
Remote Address: some_ip:80
Referrer Policy: no-referrer-when-downgrade
Response Headers
Access-Control-Allow-Headers: origin, x-requested-with, content-type, auth_token
Access-Control-Allow-Methods: POST, GET, PUT, DELETE, OPTIONS
Access-Control-Allow-Origin: *
Cache-Control: no-cache, private
Connection: Keep-Alive
Content-Length: 8
Content-Type: application/json
Date: Tue, 13 Nov 2018 13:30:35 GMT
Keep-Alive: timeout=5, max=100
Server: Apache/2.4.10 (Debian)
Request Headers
Provisional headers are shown
Access-Control-Request-Headers: auth-token
Access-Control-Request-Method: GET
Origin: http://localhost:3000
Referer: http://localhost:3000/
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/70.0.3538.77 Safari/537.36
Any ideas what might be wrong?
reactjs http
I have problem with sending CORS request with token in header.
Fetch code:
fetchApi() {
fetch('http://some-link.com',
{
headers: {
'Accept': 'application/json',
'auth-token': 'xxxxxxxxx'
},
method: "GET"
}).then(response => response.json())
.then(function(response){
this.setState({hits:response});
}).catch(function(error) { console.log(error); });
console.log(this.state.hits);
};
Console log:
Access to fetch at 'http://some-link.com' from origin 'http://localhost:3000' has been blocked by CORS policy: Request header field auth-token is not allowed by Access-Control-Allow-Headers in preflight response.
Network request log:
General
Request URL: http://some-link.com
Request Method: OPTIONS
Status Code: 200 OK
Remote Address: some_ip:80
Referrer Policy: no-referrer-when-downgrade
Response Headers
Access-Control-Allow-Headers: origin, x-requested-with, content-type, auth_token
Access-Control-Allow-Methods: POST, GET, PUT, DELETE, OPTIONS
Access-Control-Allow-Origin: *
Cache-Control: no-cache, private
Connection: Keep-Alive
Content-Length: 8
Content-Type: application/json
Date: Tue, 13 Nov 2018 13:30:35 GMT
Keep-Alive: timeout=5, max=100
Server: Apache/2.4.10 (Debian)
Request Headers
Provisional headers are shown
Access-Control-Request-Headers: auth-token
Access-Control-Request-Method: GET
Origin: http://localhost:3000
Referer: http://localhost:3000/
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/70.0.3538.77 Safari/537.36
Any ideas what might be wrong?
reactjs http
reactjs http
asked Nov 13 at 13:41
Rado
12
12
The same way as you would from anywhere but React. There's nothing in React that would be responsible for HTTP requests. It's auth_token vs auth-token header.
– estus
Nov 13 at 13:51
Fetch is a PITA, it's very low level and you have to do a lot of the work yourself. You can use something like axios to get a fully feature HTTP Client.
– AndyJ
Nov 13 at 14:09
add a comment |
The same way as you would from anywhere but React. There's nothing in React that would be responsible for HTTP requests. It's auth_token vs auth-token header.
– estus
Nov 13 at 13:51
Fetch is a PITA, it's very low level and you have to do a lot of the work yourself. You can use something like axios to get a fully feature HTTP Client.
– AndyJ
Nov 13 at 14:09
The same way as you would from anywhere but React. There's nothing in React that would be responsible for HTTP requests. It's auth_token vs auth-token header.
– estus
Nov 13 at 13:51
The same way as you would from anywhere but React. There's nothing in React that would be responsible for HTTP requests. It's auth_token vs auth-token header.
– estus
Nov 13 at 13:51
Fetch is a PITA, it's very low level and you have to do a lot of the work yourself. You can use something like axios to get a fully feature HTTP Client.
– AndyJ
Nov 13 at 14:09
Fetch is a PITA, it's very low level and you have to do a lot of the work yourself. You can use something like axios to get a fully feature HTTP Client.
– AndyJ
Nov 13 at 14:09
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
You have to change the server settings to allow the header "auth-token".
Once you do this, your request will work.
You are currently allowing "auth_token", not "auth-token".
Alternatively, change the header name in the frontend like so:
headers: {
'Accept': 'application/json',
'auth_token': 'xxxxxxxxx'
}
Jesus, how stupid am I... Thanks and cheers
– Rado
Nov 13 at 16:04
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
You have to change the server settings to allow the header "auth-token".
Once you do this, your request will work.
You are currently allowing "auth_token", not "auth-token".
Alternatively, change the header name in the frontend like so:
headers: {
'Accept': 'application/json',
'auth_token': 'xxxxxxxxx'
}
Jesus, how stupid am I... Thanks and cheers
– Rado
Nov 13 at 16:04
add a comment |
up vote
0
down vote
You have to change the server settings to allow the header "auth-token".
Once you do this, your request will work.
You are currently allowing "auth_token", not "auth-token".
Alternatively, change the header name in the frontend like so:
headers: {
'Accept': 'application/json',
'auth_token': 'xxxxxxxxx'
}
Jesus, how stupid am I... Thanks and cheers
– Rado
Nov 13 at 16:04
add a comment |
up vote
0
down vote
up vote
0
down vote
You have to change the server settings to allow the header "auth-token".
Once you do this, your request will work.
You are currently allowing "auth_token", not "auth-token".
Alternatively, change the header name in the frontend like so:
headers: {
'Accept': 'application/json',
'auth_token': 'xxxxxxxxx'
}
You have to change the server settings to allow the header "auth-token".
Once you do this, your request will work.
You are currently allowing "auth_token", not "auth-token".
Alternatively, change the header name in the frontend like so:
headers: {
'Accept': 'application/json',
'auth_token': 'xxxxxxxxx'
}
answered Nov 13 at 14:52


Rupert
1,056620
1,056620
Jesus, how stupid am I... Thanks and cheers
– Rado
Nov 13 at 16:04
add a comment |
Jesus, how stupid am I... Thanks and cheers
– Rado
Nov 13 at 16:04
Jesus, how stupid am I... Thanks and cheers
– Rado
Nov 13 at 16:04
Jesus, how stupid am I... Thanks and cheers
– Rado
Nov 13 at 16:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53282348%2fhow-to-send-cors-request-from-react%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UC njKaPqf nAPmgm68hN29Gtruau0DcM2a3kZKi m ObpG9uAgHRD3j Kb RDxdF 0 HsM n,6BDsZPQJ,k2
The same way as you would from anywhere but React. There's nothing in React that would be responsible for HTTP requests. It's auth_token vs auth-token header.
– estus
Nov 13 at 13:51
Fetch is a PITA, it's very low level and you have to do a lot of the work yourself. You can use something like axios to get a fully feature HTTP Client.
– AndyJ
Nov 13 at 14:09