How to make a list of partial sums using forEach
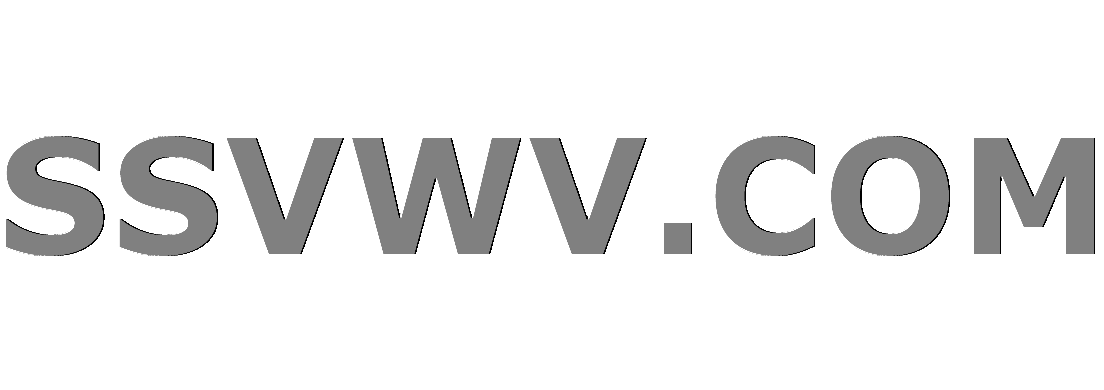
Multi tool use
I have an array of arrays which looks like this:
changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
I want to get the next value in the array by adding the last value
values = [ [1, 2, 3, 2], [1, 0, -1], [1, 2] ];
so far I have tried to use a forEach:
changes.forEach(change => {
let i = changes.indexOf(change);
let newValue = change[i] + change[i + 1]
});
I think I am on the right lines but I cannot get this approach to work, or maybe there is a better way to do it.
javascript arrays ecmascript-6 foreach
|
show 4 more comments
I have an array of arrays which looks like this:
changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
I want to get the next value in the array by adding the last value
values = [ [1, 2, 3, 2], [1, 0, -1], [1, 2] ];
so far I have tried to use a forEach:
changes.forEach(change => {
let i = changes.indexOf(change);
let newValue = change[i] + change[i + 1]
});
I think I am on the right lines but I cannot get this approach to work, or maybe there is a better way to do it.
javascript arrays ecmascript-6 foreach
1
Please elaborate this "I want to be able to increment the values based on the next value in the array to get this result:"
– Syed Mehtab Hassan
Mar 20 at 10:51
1
This is just a cumulative sum wrapped in.map
. The answer by Thomas is better than anything you'll find there though.
– JollyJoker
Mar 20 at 12:21
2
@JollyJoker Thomas simulate.reduce
method, why not use .reduce directly ?
– R3tep
Mar 20 at 12:26
1
@Thomas Look my answer, you can use an array as accumulator.
– R3tep
Mar 20 at 12:52
1
Never useforEach
if you want to produce a result.
– Bergi
Mar 20 at 13:55
|
show 4 more comments
I have an array of arrays which looks like this:
changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
I want to get the next value in the array by adding the last value
values = [ [1, 2, 3, 2], [1, 0, -1], [1, 2] ];
so far I have tried to use a forEach:
changes.forEach(change => {
let i = changes.indexOf(change);
let newValue = change[i] + change[i + 1]
});
I think I am on the right lines but I cannot get this approach to work, or maybe there is a better way to do it.
javascript arrays ecmascript-6 foreach
I have an array of arrays which looks like this:
changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
I want to get the next value in the array by adding the last value
values = [ [1, 2, 3, 2], [1, 0, -1], [1, 2] ];
so far I have tried to use a forEach:
changes.forEach(change => {
let i = changes.indexOf(change);
let newValue = change[i] + change[i + 1]
});
I think I am on the right lines but I cannot get this approach to work, or maybe there is a better way to do it.
javascript arrays ecmascript-6 foreach
javascript arrays ecmascript-6 foreach
edited Mar 20 at 14:51
Solomon Ucko
7902822
7902822
asked Mar 20 at 10:47


Team CafeTeam Cafe
1215
1215
1
Please elaborate this "I want to be able to increment the values based on the next value in the array to get this result:"
– Syed Mehtab Hassan
Mar 20 at 10:51
1
This is just a cumulative sum wrapped in.map
. The answer by Thomas is better than anything you'll find there though.
– JollyJoker
Mar 20 at 12:21
2
@JollyJoker Thomas simulate.reduce
method, why not use .reduce directly ?
– R3tep
Mar 20 at 12:26
1
@Thomas Look my answer, you can use an array as accumulator.
– R3tep
Mar 20 at 12:52
1
Never useforEach
if you want to produce a result.
– Bergi
Mar 20 at 13:55
|
show 4 more comments
1
Please elaborate this "I want to be able to increment the values based on the next value in the array to get this result:"
– Syed Mehtab Hassan
Mar 20 at 10:51
1
This is just a cumulative sum wrapped in.map
. The answer by Thomas is better than anything you'll find there though.
– JollyJoker
Mar 20 at 12:21
2
@JollyJoker Thomas simulate.reduce
method, why not use .reduce directly ?
– R3tep
Mar 20 at 12:26
1
@Thomas Look my answer, you can use an array as accumulator.
– R3tep
Mar 20 at 12:52
1
Never useforEach
if you want to produce a result.
– Bergi
Mar 20 at 13:55
1
1
Please elaborate this "I want to be able to increment the values based on the next value in the array to get this result:"
– Syed Mehtab Hassan
Mar 20 at 10:51
Please elaborate this "I want to be able to increment the values based on the next value in the array to get this result:"
– Syed Mehtab Hassan
Mar 20 at 10:51
1
1
This is just a cumulative sum wrapped in
.map
. The answer by Thomas is better than anything you'll find there though.– JollyJoker
Mar 20 at 12:21
This is just a cumulative sum wrapped in
.map
. The answer by Thomas is better than anything you'll find there though.– JollyJoker
Mar 20 at 12:21
2
2
@JollyJoker Thomas simulate
.reduce
method, why not use .reduce directly ?– R3tep
Mar 20 at 12:26
@JollyJoker Thomas simulate
.reduce
method, why not use .reduce directly ?– R3tep
Mar 20 at 12:26
1
1
@Thomas Look my answer, you can use an array as accumulator.
– R3tep
Mar 20 at 12:52
@Thomas Look my answer, you can use an array as accumulator.
– R3tep
Mar 20 at 12:52
1
1
Never use
forEach
if you want to produce a result.– Bergi
Mar 20 at 13:55
Never use
forEach
if you want to produce a result.– Bergi
Mar 20 at 13:55
|
show 4 more comments
7 Answers
7
active
oldest
votes
You could save a sum and add the values.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.map((s => v => s += v)(0)));
console.log(result);
By using forEach
, you need to take the object reference and the previous value or zero.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
array.forEach(a => a.forEach((v, i, a) => a[i] = (a[i - 1] || 0) + v));
console.log(array);
1
That first approach looks nice, but is really inefficient.
– T.J. Crowder
Mar 20 at 10:58
12
but man does it look nice
– Jeremy Thille
Mar 20 at 10:58
1
@T.J.Crowder could you explain or point me in the right direction, why the first approach is inefficient?
– Thomas
Mar 20 at 11:01
1
@Thomas - Look at all the functions it's creating and executing. Your solution is great -- as concise (way more so than mine) and still efficient.
– T.J. Crowder
Mar 20 at 11:01
2
really, for three items in the outer array? if you really want to speed up the execution, you never use some array methods, instead use nestedfor
loops and create new arrays.
– Nina Scholz
Mar 20 at 11:05
|
show 4 more comments
A version with map.
const changes = [
[1, 1, 1, -1],
[1, -1, -1],
[1, 1]
];
const values = changes.map(array => {
let acc = 0;
return array.map(v => acc += v);
});
console.log(values);
.as-console-wrapper{top:0;max-height:100%!important}
And this doesn't change the source Array.
Note that the array of arrays structure is just a needless complication. The question is actually about the cumulative sum of an array. I'd split out the outer mapping function into something calledcumulativeSum
– JollyJoker
Mar 20 at 12:25
add a comment |
New ESNext features of generators are nice for this.
Here I've created a simple sumpUp
generator that you can re-use.
function* sumUp(a) {
let sum = 0;
for (const v of a) yield sum += v;
}
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const values = changes.map(a => [...sumUp(a)]);
console.log(values);
add a comment |
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ]
let values =
changes.forEach(arr => {
let accu = 0
let nestedArr =
arr.forEach(n => {
accu += n
nestedArr.push(accu)
})
values.push(nestedArr)
})
console.log(values)
add a comment |
You may use map function of Array
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const result = changes.map((v) => v.slice(0).map((t, i, arr) => i === 0 ? t : (arr[i] += arr[i - 1])))
console.log(changes);
console.log(result);
Update
Use slice to clone array. This will prevent changes to the original array.
3
This modifies the source array, which is probably not a good idea.
– T.J. Crowder
Mar 20 at 10:56
Yes. Andslice
will help to prevent that. Thanks for the tip
– Alexander
Mar 20 at 11:02
add a comment |
Here is an easier to read way that iterates over the outer list of arrays. A copy of the inner array is made to keep the initial values (like [1, 1, 1, -1]). It then iterates over each value in the copied array and adds it to each index after it in the original array.
var changes = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
changes.forEach(subArray => {
var subArrayCopy = subArray.slice(); // Create a copy of the current sub array (i.e. subArrayCopy = [1, 1, 1, -1];)
subArrayCopy.forEach((val, index) => { // Iterate through each value in the copy
for (var i = subArray.length - 1; i > index; i--) { // For each element from the end to the current index
subArray[i] += val; // Add the copy's current index value to the original array
}
});
})
console.log(changes);
add a comment |
Another way,
You can use .map
to return your new array with the desired results. By using .reduce
with an array as an accumulator, you can generate the subarray.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.reduce((ac, v, i) => {
const lastVal = ac[i-1] || 0;
return [...ac, lastVal + v];
}, ));
console.log(result);
// shorter
result = array.map(a => a.reduce((ac, v, i) => [...ac, (ac[i-1] || 0) + v], ));
console.log(result);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55258925%2fhow-to-make-a-list-of-partial-sums-using-foreach%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
7 Answers
7
active
oldest
votes
7 Answers
7
active
oldest
votes
active
oldest
votes
active
oldest
votes
You could save a sum and add the values.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.map((s => v => s += v)(0)));
console.log(result);
By using forEach
, you need to take the object reference and the previous value or zero.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
array.forEach(a => a.forEach((v, i, a) => a[i] = (a[i - 1] || 0) + v));
console.log(array);
1
That first approach looks nice, but is really inefficient.
– T.J. Crowder
Mar 20 at 10:58
12
but man does it look nice
– Jeremy Thille
Mar 20 at 10:58
1
@T.J.Crowder could you explain or point me in the right direction, why the first approach is inefficient?
– Thomas
Mar 20 at 11:01
1
@Thomas - Look at all the functions it's creating and executing. Your solution is great -- as concise (way more so than mine) and still efficient.
– T.J. Crowder
Mar 20 at 11:01
2
really, for three items in the outer array? if you really want to speed up the execution, you never use some array methods, instead use nestedfor
loops and create new arrays.
– Nina Scholz
Mar 20 at 11:05
|
show 4 more comments
You could save a sum and add the values.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.map((s => v => s += v)(0)));
console.log(result);
By using forEach
, you need to take the object reference and the previous value or zero.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
array.forEach(a => a.forEach((v, i, a) => a[i] = (a[i - 1] || 0) + v));
console.log(array);
1
That first approach looks nice, but is really inefficient.
– T.J. Crowder
Mar 20 at 10:58
12
but man does it look nice
– Jeremy Thille
Mar 20 at 10:58
1
@T.J.Crowder could you explain or point me in the right direction, why the first approach is inefficient?
– Thomas
Mar 20 at 11:01
1
@Thomas - Look at all the functions it's creating and executing. Your solution is great -- as concise (way more so than mine) and still efficient.
– T.J. Crowder
Mar 20 at 11:01
2
really, for three items in the outer array? if you really want to speed up the execution, you never use some array methods, instead use nestedfor
loops and create new arrays.
– Nina Scholz
Mar 20 at 11:05
|
show 4 more comments
You could save a sum and add the values.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.map((s => v => s += v)(0)));
console.log(result);
By using forEach
, you need to take the object reference and the previous value or zero.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
array.forEach(a => a.forEach((v, i, a) => a[i] = (a[i - 1] || 0) + v));
console.log(array);
You could save a sum and add the values.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.map((s => v => s += v)(0)));
console.log(result);
By using forEach
, you need to take the object reference and the previous value or zero.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
array.forEach(a => a.forEach((v, i, a) => a[i] = (a[i - 1] || 0) + v));
console.log(array);
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.map((s => v => s += v)(0)));
console.log(result);
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.map((s => v => s += v)(0)));
console.log(result);
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
array.forEach(a => a.forEach((v, i, a) => a[i] = (a[i - 1] || 0) + v));
console.log(array);
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
array.forEach(a => a.forEach((v, i, a) => a[i] = (a[i - 1] || 0) + v));
console.log(array);
answered Mar 20 at 10:54


Nina ScholzNina Scholz
195k15107178
195k15107178
1
That first approach looks nice, but is really inefficient.
– T.J. Crowder
Mar 20 at 10:58
12
but man does it look nice
– Jeremy Thille
Mar 20 at 10:58
1
@T.J.Crowder could you explain or point me in the right direction, why the first approach is inefficient?
– Thomas
Mar 20 at 11:01
1
@Thomas - Look at all the functions it's creating and executing. Your solution is great -- as concise (way more so than mine) and still efficient.
– T.J. Crowder
Mar 20 at 11:01
2
really, for three items in the outer array? if you really want to speed up the execution, you never use some array methods, instead use nestedfor
loops and create new arrays.
– Nina Scholz
Mar 20 at 11:05
|
show 4 more comments
1
That first approach looks nice, but is really inefficient.
– T.J. Crowder
Mar 20 at 10:58
12
but man does it look nice
– Jeremy Thille
Mar 20 at 10:58
1
@T.J.Crowder could you explain or point me in the right direction, why the first approach is inefficient?
– Thomas
Mar 20 at 11:01
1
@Thomas - Look at all the functions it's creating and executing. Your solution is great -- as concise (way more so than mine) and still efficient.
– T.J. Crowder
Mar 20 at 11:01
2
really, for three items in the outer array? if you really want to speed up the execution, you never use some array methods, instead use nestedfor
loops and create new arrays.
– Nina Scholz
Mar 20 at 11:05
1
1
That first approach looks nice, but is really inefficient.
– T.J. Crowder
Mar 20 at 10:58
That first approach looks nice, but is really inefficient.
– T.J. Crowder
Mar 20 at 10:58
12
12
but man does it look nice
– Jeremy Thille
Mar 20 at 10:58
but man does it look nice
– Jeremy Thille
Mar 20 at 10:58
1
1
@T.J.Crowder could you explain or point me in the right direction, why the first approach is inefficient?
– Thomas
Mar 20 at 11:01
@T.J.Crowder could you explain or point me in the right direction, why the first approach is inefficient?
– Thomas
Mar 20 at 11:01
1
1
@Thomas - Look at all the functions it's creating and executing. Your solution is great -- as concise (way more so than mine) and still efficient.
– T.J. Crowder
Mar 20 at 11:01
@Thomas - Look at all the functions it's creating and executing. Your solution is great -- as concise (way more so than mine) and still efficient.
– T.J. Crowder
Mar 20 at 11:01
2
2
really, for three items in the outer array? if you really want to speed up the execution, you never use some array methods, instead use nested
for
loops and create new arrays.– Nina Scholz
Mar 20 at 11:05
really, for three items in the outer array? if you really want to speed up the execution, you never use some array methods, instead use nested
for
loops and create new arrays.– Nina Scholz
Mar 20 at 11:05
|
show 4 more comments
A version with map.
const changes = [
[1, 1, 1, -1],
[1, -1, -1],
[1, 1]
];
const values = changes.map(array => {
let acc = 0;
return array.map(v => acc += v);
});
console.log(values);
.as-console-wrapper{top:0;max-height:100%!important}
And this doesn't change the source Array.
Note that the array of arrays structure is just a needless complication. The question is actually about the cumulative sum of an array. I'd split out the outer mapping function into something calledcumulativeSum
– JollyJoker
Mar 20 at 12:25
add a comment |
A version with map.
const changes = [
[1, 1, 1, -1],
[1, -1, -1],
[1, 1]
];
const values = changes.map(array => {
let acc = 0;
return array.map(v => acc += v);
});
console.log(values);
.as-console-wrapper{top:0;max-height:100%!important}
And this doesn't change the source Array.
Note that the array of arrays structure is just a needless complication. The question is actually about the cumulative sum of an array. I'd split out the outer mapping function into something calledcumulativeSum
– JollyJoker
Mar 20 at 12:25
add a comment |
A version with map.
const changes = [
[1, 1, 1, -1],
[1, -1, -1],
[1, 1]
];
const values = changes.map(array => {
let acc = 0;
return array.map(v => acc += v);
});
console.log(values);
.as-console-wrapper{top:0;max-height:100%!important}
And this doesn't change the source Array.
A version with map.
const changes = [
[1, 1, 1, -1],
[1, -1, -1],
[1, 1]
];
const values = changes.map(array => {
let acc = 0;
return array.map(v => acc += v);
});
console.log(values);
.as-console-wrapper{top:0;max-height:100%!important}
And this doesn't change the source Array.
const changes = [
[1, 1, 1, -1],
[1, -1, -1],
[1, 1]
];
const values = changes.map(array => {
let acc = 0;
return array.map(v => acc += v);
});
console.log(values);
.as-console-wrapper{top:0;max-height:100%!important}
const changes = [
[1, 1, 1, -1],
[1, -1, -1],
[1, 1]
];
const values = changes.map(array => {
let acc = 0;
return array.map(v => acc += v);
});
console.log(values);
.as-console-wrapper{top:0;max-height:100%!important}
edited Mar 20 at 11:01
T.J. Crowder
697k12312401336
697k12312401336
answered Mar 20 at 10:59
ThomasThomas
5,2161510
5,2161510
Note that the array of arrays structure is just a needless complication. The question is actually about the cumulative sum of an array. I'd split out the outer mapping function into something calledcumulativeSum
– JollyJoker
Mar 20 at 12:25
add a comment |
Note that the array of arrays structure is just a needless complication. The question is actually about the cumulative sum of an array. I'd split out the outer mapping function into something calledcumulativeSum
– JollyJoker
Mar 20 at 12:25
Note that the array of arrays structure is just a needless complication. The question is actually about the cumulative sum of an array. I'd split out the outer mapping function into something called
cumulativeSum
– JollyJoker
Mar 20 at 12:25
Note that the array of arrays structure is just a needless complication. The question is actually about the cumulative sum of an array. I'd split out the outer mapping function into something called
cumulativeSum
– JollyJoker
Mar 20 at 12:25
add a comment |
New ESNext features of generators are nice for this.
Here I've created a simple sumpUp
generator that you can re-use.
function* sumUp(a) {
let sum = 0;
for (const v of a) yield sum += v;
}
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const values = changes.map(a => [...sumUp(a)]);
console.log(values);
add a comment |
New ESNext features of generators are nice for this.
Here I've created a simple sumpUp
generator that you can re-use.
function* sumUp(a) {
let sum = 0;
for (const v of a) yield sum += v;
}
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const values = changes.map(a => [...sumUp(a)]);
console.log(values);
add a comment |
New ESNext features of generators are nice for this.
Here I've created a simple sumpUp
generator that you can re-use.
function* sumUp(a) {
let sum = 0;
for (const v of a) yield sum += v;
}
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const values = changes.map(a => [...sumUp(a)]);
console.log(values);
New ESNext features of generators are nice for this.
Here I've created a simple sumpUp
generator that you can re-use.
function* sumUp(a) {
let sum = 0;
for (const v of a) yield sum += v;
}
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const values = changes.map(a => [...sumUp(a)]);
console.log(values);
function* sumUp(a) {
let sum = 0;
for (const v of a) yield sum += v;
}
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const values = changes.map(a => [...sumUp(a)]);
console.log(values);
function* sumUp(a) {
let sum = 0;
for (const v of a) yield sum += v;
}
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const values = changes.map(a => [...sumUp(a)]);
console.log(values);
edited Mar 20 at 11:18
answered Mar 20 at 11:13
KeithKeith
9,1031821
9,1031821
add a comment |
add a comment |
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ]
let values =
changes.forEach(arr => {
let accu = 0
let nestedArr =
arr.forEach(n => {
accu += n
nestedArr.push(accu)
})
values.push(nestedArr)
})
console.log(values)
add a comment |
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ]
let values =
changes.forEach(arr => {
let accu = 0
let nestedArr =
arr.forEach(n => {
accu += n
nestedArr.push(accu)
})
values.push(nestedArr)
})
console.log(values)
add a comment |
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ]
let values =
changes.forEach(arr => {
let accu = 0
let nestedArr =
arr.forEach(n => {
accu += n
nestedArr.push(accu)
})
values.push(nestedArr)
})
console.log(values)
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ]
let values =
changes.forEach(arr => {
let accu = 0
let nestedArr =
arr.forEach(n => {
accu += n
nestedArr.push(accu)
})
values.push(nestedArr)
})
console.log(values)
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ]
let values =
changes.forEach(arr => {
let accu = 0
let nestedArr =
arr.forEach(n => {
accu += n
nestedArr.push(accu)
})
values.push(nestedArr)
})
console.log(values)
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ]
let values =
changes.forEach(arr => {
let accu = 0
let nestedArr =
arr.forEach(n => {
accu += n
nestedArr.push(accu)
})
values.push(nestedArr)
})
console.log(values)
answered Mar 20 at 10:52
holydragonholydragon
2,74221230
2,74221230
add a comment |
add a comment |
You may use map function of Array
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const result = changes.map((v) => v.slice(0).map((t, i, arr) => i === 0 ? t : (arr[i] += arr[i - 1])))
console.log(changes);
console.log(result);
Update
Use slice to clone array. This will prevent changes to the original array.
3
This modifies the source array, which is probably not a good idea.
– T.J. Crowder
Mar 20 at 10:56
Yes. Andslice
will help to prevent that. Thanks for the tip
– Alexander
Mar 20 at 11:02
add a comment |
You may use map function of Array
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const result = changes.map((v) => v.slice(0).map((t, i, arr) => i === 0 ? t : (arr[i] += arr[i - 1])))
console.log(changes);
console.log(result);
Update
Use slice to clone array. This will prevent changes to the original array.
3
This modifies the source array, which is probably not a good idea.
– T.J. Crowder
Mar 20 at 10:56
Yes. Andslice
will help to prevent that. Thanks for the tip
– Alexander
Mar 20 at 11:02
add a comment |
You may use map function of Array
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const result = changes.map((v) => v.slice(0).map((t, i, arr) => i === 0 ? t : (arr[i] += arr[i - 1])))
console.log(changes);
console.log(result);
Update
Use slice to clone array. This will prevent changes to the original array.
You may use map function of Array
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const result = changes.map((v) => v.slice(0).map((t, i, arr) => i === 0 ? t : (arr[i] += arr[i - 1])))
console.log(changes);
console.log(result);
Update
Use slice to clone array. This will prevent changes to the original array.
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const result = changes.map((v) => v.slice(0).map((t, i, arr) => i === 0 ? t : (arr[i] += arr[i - 1])))
console.log(changes);
console.log(result);
const changes = [ [1, 1, 1, -1], [1, -1, -1], [1, 1] ];
const result = changes.map((v) => v.slice(0).map((t, i, arr) => i === 0 ? t : (arr[i] += arr[i - 1])))
console.log(changes);
console.log(result);
edited Mar 20 at 11:01
answered Mar 20 at 10:51


AlexanderAlexander
1,023214
1,023214
3
This modifies the source array, which is probably not a good idea.
– T.J. Crowder
Mar 20 at 10:56
Yes. Andslice
will help to prevent that. Thanks for the tip
– Alexander
Mar 20 at 11:02
add a comment |
3
This modifies the source array, which is probably not a good idea.
– T.J. Crowder
Mar 20 at 10:56
Yes. Andslice
will help to prevent that. Thanks for the tip
– Alexander
Mar 20 at 11:02
3
3
This modifies the source array, which is probably not a good idea.
– T.J. Crowder
Mar 20 at 10:56
This modifies the source array, which is probably not a good idea.
– T.J. Crowder
Mar 20 at 10:56
Yes. And
slice
will help to prevent that. Thanks for the tip– Alexander
Mar 20 at 11:02
Yes. And
slice
will help to prevent that. Thanks for the tip– Alexander
Mar 20 at 11:02
add a comment |
Here is an easier to read way that iterates over the outer list of arrays. A copy of the inner array is made to keep the initial values (like [1, 1, 1, -1]). It then iterates over each value in the copied array and adds it to each index after it in the original array.
var changes = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
changes.forEach(subArray => {
var subArrayCopy = subArray.slice(); // Create a copy of the current sub array (i.e. subArrayCopy = [1, 1, 1, -1];)
subArrayCopy.forEach((val, index) => { // Iterate through each value in the copy
for (var i = subArray.length - 1; i > index; i--) { // For each element from the end to the current index
subArray[i] += val; // Add the copy's current index value to the original array
}
});
})
console.log(changes);
add a comment |
Here is an easier to read way that iterates over the outer list of arrays. A copy of the inner array is made to keep the initial values (like [1, 1, 1, -1]). It then iterates over each value in the copied array and adds it to each index after it in the original array.
var changes = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
changes.forEach(subArray => {
var subArrayCopy = subArray.slice(); // Create a copy of the current sub array (i.e. subArrayCopy = [1, 1, 1, -1];)
subArrayCopy.forEach((val, index) => { // Iterate through each value in the copy
for (var i = subArray.length - 1; i > index; i--) { // For each element from the end to the current index
subArray[i] += val; // Add the copy's current index value to the original array
}
});
})
console.log(changes);
add a comment |
Here is an easier to read way that iterates over the outer list of arrays. A copy of the inner array is made to keep the initial values (like [1, 1, 1, -1]). It then iterates over each value in the copied array and adds it to each index after it in the original array.
var changes = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
changes.forEach(subArray => {
var subArrayCopy = subArray.slice(); // Create a copy of the current sub array (i.e. subArrayCopy = [1, 1, 1, -1];)
subArrayCopy.forEach((val, index) => { // Iterate through each value in the copy
for (var i = subArray.length - 1; i > index; i--) { // For each element from the end to the current index
subArray[i] += val; // Add the copy's current index value to the original array
}
});
})
console.log(changes);
Here is an easier to read way that iterates over the outer list of arrays. A copy of the inner array is made to keep the initial values (like [1, 1, 1, -1]). It then iterates over each value in the copied array and adds it to each index after it in the original array.
var changes = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
changes.forEach(subArray => {
var subArrayCopy = subArray.slice(); // Create a copy of the current sub array (i.e. subArrayCopy = [1, 1, 1, -1];)
subArrayCopy.forEach((val, index) => { // Iterate through each value in the copy
for (var i = subArray.length - 1; i > index; i--) { // For each element from the end to the current index
subArray[i] += val; // Add the copy's current index value to the original array
}
});
})
console.log(changes);
var changes = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
changes.forEach(subArray => {
var subArrayCopy = subArray.slice(); // Create a copy of the current sub array (i.e. subArrayCopy = [1, 1, 1, -1];)
subArrayCopy.forEach((val, index) => { // Iterate through each value in the copy
for (var i = subArray.length - 1; i > index; i--) { // For each element from the end to the current index
subArray[i] += val; // Add the copy's current index value to the original array
}
});
})
console.log(changes);
var changes = [[1, 1, 1, -1], [1, -1, -1], [1, 1]];
changes.forEach(subArray => {
var subArrayCopy = subArray.slice(); // Create a copy of the current sub array (i.e. subArrayCopy = [1, 1, 1, -1];)
subArrayCopy.forEach((val, index) => { // Iterate through each value in the copy
for (var i = subArray.length - 1; i > index; i--) { // For each element from the end to the current index
subArray[i] += val; // Add the copy's current index value to the original array
}
});
})
console.log(changes);
answered Mar 20 at 13:06
Nick GNick G
1,029614
1,029614
add a comment |
add a comment |
Another way,
You can use .map
to return your new array with the desired results. By using .reduce
with an array as an accumulator, you can generate the subarray.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.reduce((ac, v, i) => {
const lastVal = ac[i-1] || 0;
return [...ac, lastVal + v];
}, ));
console.log(result);
// shorter
result = array.map(a => a.reduce((ac, v, i) => [...ac, (ac[i-1] || 0) + v], ));
console.log(result);
add a comment |
Another way,
You can use .map
to return your new array with the desired results. By using .reduce
with an array as an accumulator, you can generate the subarray.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.reduce((ac, v, i) => {
const lastVal = ac[i-1] || 0;
return [...ac, lastVal + v];
}, ));
console.log(result);
// shorter
result = array.map(a => a.reduce((ac, v, i) => [...ac, (ac[i-1] || 0) + v], ));
console.log(result);
add a comment |
Another way,
You can use .map
to return your new array with the desired results. By using .reduce
with an array as an accumulator, you can generate the subarray.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.reduce((ac, v, i) => {
const lastVal = ac[i-1] || 0;
return [...ac, lastVal + v];
}, ));
console.log(result);
// shorter
result = array.map(a => a.reduce((ac, v, i) => [...ac, (ac[i-1] || 0) + v], ));
console.log(result);
Another way,
You can use .map
to return your new array with the desired results. By using .reduce
with an array as an accumulator, you can generate the subarray.
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.reduce((ac, v, i) => {
const lastVal = ac[i-1] || 0;
return [...ac, lastVal + v];
}, ));
console.log(result);
// shorter
result = array.map(a => a.reduce((ac, v, i) => [...ac, (ac[i-1] || 0) + v], ));
console.log(result);
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.reduce((ac, v, i) => {
const lastVal = ac[i-1] || 0;
return [...ac, lastVal + v];
}, ));
console.log(result);
// shorter
result = array.map(a => a.reduce((ac, v, i) => [...ac, (ac[i-1] || 0) + v], ));
console.log(result);
var array = [[1, 1, 1, -1], [1, -1, -1], [1, 1]],
result = array.map(a => a.reduce((ac, v, i) => {
const lastVal = ac[i-1] || 0;
return [...ac, lastVal + v];
}, ));
console.log(result);
// shorter
result = array.map(a => a.reduce((ac, v, i) => [...ac, (ac[i-1] || 0) + v], ));
console.log(result);
edited Mar 20 at 14:37
answered Mar 20 at 12:12
R3tepR3tep
8,14382962
8,14382962
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55258925%2fhow-to-make-a-list-of-partial-sums-using-foreach%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
z7oWRcmcEl66IvwL6QQJ,ZCpnKBurfxWg GrwWHIXXwPpF0NTf Dt
1
Please elaborate this "I want to be able to increment the values based on the next value in the array to get this result:"
– Syed Mehtab Hassan
Mar 20 at 10:51
1
This is just a cumulative sum wrapped in
.map
. The answer by Thomas is better than anything you'll find there though.– JollyJoker
Mar 20 at 12:21
2
@JollyJoker Thomas simulate
.reduce
method, why not use .reduce directly ?– R3tep
Mar 20 at 12:26
1
@Thomas Look my answer, you can use an array as accumulator.
– R3tep
Mar 20 at 12:52
1
Never use
forEach
if you want to produce a result.– Bergi
Mar 20 at 13:55