Extracting numeric values from a string containing key:value pairs
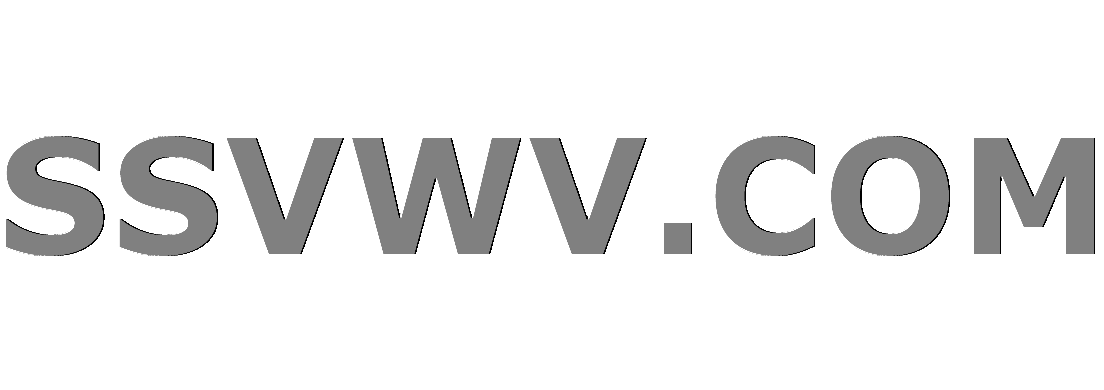
Multi tool use
$begingroup$
I am writing function numericValues(text: String): List[Int]
to extract patterns """([a-z]+)s*:s*(d+)"""
and return the list of the numeric values :
numericValues("a123 : 0 abc:123 123:abc xyz:1") // List(123, 1)
I would write numericValues
like this:
def numericValues(text: String): List[Int] = {
val regex = """([a-z]+)s*:s*(d+)""".r
regex.findAllIn(text).toList.flatMap {s =>
PartialFunction.condOpt(s) { case regex(_, num) => num.toInt }
}
}
I guess the condOpt
invocation is redundant and I wonder how to simplify this implementation. Also, I'd appreciate comments on improvements to style and best practice.
regex scala
$endgroup$
add a comment |
$begingroup$
I am writing function numericValues(text: String): List[Int]
to extract patterns """([a-z]+)s*:s*(d+)"""
and return the list of the numeric values :
numericValues("a123 : 0 abc:123 123:abc xyz:1") // List(123, 1)
I would write numericValues
like this:
def numericValues(text: String): List[Int] = {
val regex = """([a-z]+)s*:s*(d+)""".r
regex.findAllIn(text).toList.flatMap {s =>
PartialFunction.condOpt(s) { case regex(_, num) => num.toInt }
}
}
I guess the condOpt
invocation is redundant and I wonder how to simplify this implementation. Also, I'd appreciate comments on improvements to style and best practice.
regex scala
$endgroup$
add a comment |
$begingroup$
I am writing function numericValues(text: String): List[Int]
to extract patterns """([a-z]+)s*:s*(d+)"""
and return the list of the numeric values :
numericValues("a123 : 0 abc:123 123:abc xyz:1") // List(123, 1)
I would write numericValues
like this:
def numericValues(text: String): List[Int] = {
val regex = """([a-z]+)s*:s*(d+)""".r
regex.findAllIn(text).toList.flatMap {s =>
PartialFunction.condOpt(s) { case regex(_, num) => num.toInt }
}
}
I guess the condOpt
invocation is redundant and I wonder how to simplify this implementation. Also, I'd appreciate comments on improvements to style and best practice.
regex scala
$endgroup$
I am writing function numericValues(text: String): List[Int]
to extract patterns """([a-z]+)s*:s*(d+)"""
and return the list of the numeric values :
numericValues("a123 : 0 abc:123 123:abc xyz:1") // List(123, 1)
I would write numericValues
like this:
def numericValues(text: String): List[Int] = {
val regex = """([a-z]+)s*:s*(d+)""".r
regex.findAllIn(text).toList.flatMap {s =>
PartialFunction.condOpt(s) { case regex(_, num) => num.toInt }
}
}
I guess the condOpt
invocation is redundant and I wonder how to simplify this implementation. Also, I'd appreciate comments on improvements to style and best practice.
regex scala
regex scala
edited Mar 20 at 21:30
Michael
asked Mar 20 at 10:20
MichaelMichael
1285
1285
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
You usually want a zero-width word boundary b
on either end of the regex, to avoid matching things like 1a:1a
.
There's no need to capture [a-z]+
since you are throwing it away.
You can use lookbehind (?<=…)
assertions to require a match to be preceded by whatever, without including the whatever in the match result. This means no need for capturing parenthesis, only the integer is included in the match, and the final map is simply _.toInt
. Variable-width lookbehind was introduced in Java 9; older versions have only fixed-width lookbehind.
Finally, removing the variable makes braces unnecessary.
def numericValues(text: String): List[Int] = """(?<=b[a-z]+s*:s*)d+b""".r.findAllIn(text).toList.map(_.toInt)
With only fixed-width lookbehind, you could postprocess the matches to remove non-numerics:
….map(_.replaceAll("[^0-9]", "").toInt)
Or, more idiomatically and less hacky, just capture the digits and extract the capture groups:
"""b[a-z]+s*:s*(d+)b""".r.findAllIn(text).matchData.toList.map(_.group(1).toInt)
$endgroup$
$begingroup$
Thanks a lot. What if I have only fixed-width lookbehind ?
$endgroup$
– Michael
Mar 20 at 13:00
1
$begingroup$
then you can't use variable-width lookbehind :) See edit.
$endgroup$
– Oh My Goodness
Mar 20 at 13:56
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
return StackExchange.using("mathjaxEditing", function () {
StackExchange.MarkdownEditor.creationCallbacks.add(function (editor, postfix) {
StackExchange.mathjaxEditing.prepareWmdForMathJax(editor, postfix, [["\$", "\$"]]);
});
});
}, "mathjax-editing");
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215831%2fextracting-numeric-values-from-a-string-containing-keyvalue-pairs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
You usually want a zero-width word boundary b
on either end of the regex, to avoid matching things like 1a:1a
.
There's no need to capture [a-z]+
since you are throwing it away.
You can use lookbehind (?<=…)
assertions to require a match to be preceded by whatever, without including the whatever in the match result. This means no need for capturing parenthesis, only the integer is included in the match, and the final map is simply _.toInt
. Variable-width lookbehind was introduced in Java 9; older versions have only fixed-width lookbehind.
Finally, removing the variable makes braces unnecessary.
def numericValues(text: String): List[Int] = """(?<=b[a-z]+s*:s*)d+b""".r.findAllIn(text).toList.map(_.toInt)
With only fixed-width lookbehind, you could postprocess the matches to remove non-numerics:
….map(_.replaceAll("[^0-9]", "").toInt)
Or, more idiomatically and less hacky, just capture the digits and extract the capture groups:
"""b[a-z]+s*:s*(d+)b""".r.findAllIn(text).matchData.toList.map(_.group(1).toInt)
$endgroup$
$begingroup$
Thanks a lot. What if I have only fixed-width lookbehind ?
$endgroup$
– Michael
Mar 20 at 13:00
1
$begingroup$
then you can't use variable-width lookbehind :) See edit.
$endgroup$
– Oh My Goodness
Mar 20 at 13:56
add a comment |
$begingroup$
You usually want a zero-width word boundary b
on either end of the regex, to avoid matching things like 1a:1a
.
There's no need to capture [a-z]+
since you are throwing it away.
You can use lookbehind (?<=…)
assertions to require a match to be preceded by whatever, without including the whatever in the match result. This means no need for capturing parenthesis, only the integer is included in the match, and the final map is simply _.toInt
. Variable-width lookbehind was introduced in Java 9; older versions have only fixed-width lookbehind.
Finally, removing the variable makes braces unnecessary.
def numericValues(text: String): List[Int] = """(?<=b[a-z]+s*:s*)d+b""".r.findAllIn(text).toList.map(_.toInt)
With only fixed-width lookbehind, you could postprocess the matches to remove non-numerics:
….map(_.replaceAll("[^0-9]", "").toInt)
Or, more idiomatically and less hacky, just capture the digits and extract the capture groups:
"""b[a-z]+s*:s*(d+)b""".r.findAllIn(text).matchData.toList.map(_.group(1).toInt)
$endgroup$
$begingroup$
Thanks a lot. What if I have only fixed-width lookbehind ?
$endgroup$
– Michael
Mar 20 at 13:00
1
$begingroup$
then you can't use variable-width lookbehind :) See edit.
$endgroup$
– Oh My Goodness
Mar 20 at 13:56
add a comment |
$begingroup$
You usually want a zero-width word boundary b
on either end of the regex, to avoid matching things like 1a:1a
.
There's no need to capture [a-z]+
since you are throwing it away.
You can use lookbehind (?<=…)
assertions to require a match to be preceded by whatever, without including the whatever in the match result. This means no need for capturing parenthesis, only the integer is included in the match, and the final map is simply _.toInt
. Variable-width lookbehind was introduced in Java 9; older versions have only fixed-width lookbehind.
Finally, removing the variable makes braces unnecessary.
def numericValues(text: String): List[Int] = """(?<=b[a-z]+s*:s*)d+b""".r.findAllIn(text).toList.map(_.toInt)
With only fixed-width lookbehind, you could postprocess the matches to remove non-numerics:
….map(_.replaceAll("[^0-9]", "").toInt)
Or, more idiomatically and less hacky, just capture the digits and extract the capture groups:
"""b[a-z]+s*:s*(d+)b""".r.findAllIn(text).matchData.toList.map(_.group(1).toInt)
$endgroup$
You usually want a zero-width word boundary b
on either end of the regex, to avoid matching things like 1a:1a
.
There's no need to capture [a-z]+
since you are throwing it away.
You can use lookbehind (?<=…)
assertions to require a match to be preceded by whatever, without including the whatever in the match result. This means no need for capturing parenthesis, only the integer is included in the match, and the final map is simply _.toInt
. Variable-width lookbehind was introduced in Java 9; older versions have only fixed-width lookbehind.
Finally, removing the variable makes braces unnecessary.
def numericValues(text: String): List[Int] = """(?<=b[a-z]+s*:s*)d+b""".r.findAllIn(text).toList.map(_.toInt)
With only fixed-width lookbehind, you could postprocess the matches to remove non-numerics:
….map(_.replaceAll("[^0-9]", "").toInt)
Or, more idiomatically and less hacky, just capture the digits and extract the capture groups:
"""b[a-z]+s*:s*(d+)b""".r.findAllIn(text).matchData.toList.map(_.group(1).toInt)
edited Mar 20 at 13:54
answered Mar 20 at 12:35


Oh My GoodnessOh My Goodness
1,984315
1,984315
$begingroup$
Thanks a lot. What if I have only fixed-width lookbehind ?
$endgroup$
– Michael
Mar 20 at 13:00
1
$begingroup$
then you can't use variable-width lookbehind :) See edit.
$endgroup$
– Oh My Goodness
Mar 20 at 13:56
add a comment |
$begingroup$
Thanks a lot. What if I have only fixed-width lookbehind ?
$endgroup$
– Michael
Mar 20 at 13:00
1
$begingroup$
then you can't use variable-width lookbehind :) See edit.
$endgroup$
– Oh My Goodness
Mar 20 at 13:56
$begingroup$
Thanks a lot. What if I have only fixed-width lookbehind ?
$endgroup$
– Michael
Mar 20 at 13:00
$begingroup$
Thanks a lot. What if I have only fixed-width lookbehind ?
$endgroup$
– Michael
Mar 20 at 13:00
1
1
$begingroup$
then you can't use variable-width lookbehind :) See edit.
$endgroup$
– Oh My Goodness
Mar 20 at 13:56
$begingroup$
then you can't use variable-width lookbehind :) See edit.
$endgroup$
– Oh My Goodness
Mar 20 at 13:56
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f215831%2fextracting-numeric-values-from-a-string-containing-keyvalue-pairs%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
JoZ,HBG RqGLyhcoycFknFs4iv6 pNu3TdTc9zBGDL3KP8kwEpfZsCW kt