graphql query is embedded inside req.body
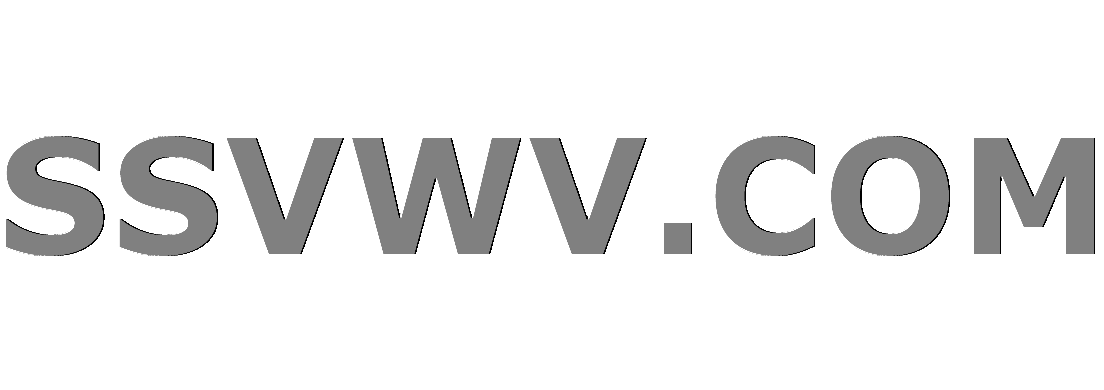
Multi tool use
So I'm trying to move from REST to GraphQL but there's a minor thing that makes it a little bit harder is that queries e.g.
www.example.com?test=test
the query: { test: "test" }
is actually under body: { query: { test: "test" } }
in GraphQL. So I was wondering if there was some middleware or something that could move this back out.
I'm using body-parser
via
var bodyParser =
require("body-parser")
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({
extended: true
}))
javascript node.js express graphql
add a comment |
So I'm trying to move from REST to GraphQL but there's a minor thing that makes it a little bit harder is that queries e.g.
www.example.com?test=test
the query: { test: "test" }
is actually under body: { query: { test: "test" } }
in GraphQL. So I was wondering if there was some middleware or something that could move this back out.
I'm using body-parser
via
var bodyParser =
require("body-parser")
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({
extended: true
}))
javascript node.js express graphql
add a comment |
So I'm trying to move from REST to GraphQL but there's a minor thing that makes it a little bit harder is that queries e.g.
www.example.com?test=test
the query: { test: "test" }
is actually under body: { query: { test: "test" } }
in GraphQL. So I was wondering if there was some middleware or something that could move this back out.
I'm using body-parser
via
var bodyParser =
require("body-parser")
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({
extended: true
}))
javascript node.js express graphql
So I'm trying to move from REST to GraphQL but there's a minor thing that makes it a little bit harder is that queries e.g.
www.example.com?test=test
the query: { test: "test" }
is actually under body: { query: { test: "test" } }
in GraphQL. So I was wondering if there was some middleware or something that could move this back out.
I'm using body-parser
via
var bodyParser =
require("body-parser")
app.use(bodyParser.json())
app.use(bodyParser.urlencoded({
extended: true
}))
javascript node.js express graphql
javascript node.js express graphql
asked Nov 22 '18 at 0:50
A. LauA. Lau
3,43062263
3,43062263
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Unless there's a specific reason you want to validate and execute the GraphQL document yourself, you should probably use an existing library for that. Two of the more popular solutions for express:
//express-graphql
const graphqlHTTP = require('express-graphql');
const { makeExecutableSchema } = require('graphql-tools');
const schema = makeExecutableSchema({
typeDefs,
resolvers,
});
app.use('/graphql', graphqlHTTP({
schema,
graphiql: true,
}));
app.listen(4000);
// apollo-server-express
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const server = new ApolloServer({ typeDefs, resolvers });
server.applyMiddleware({ app });
app.listen(4000);
You can also just use apollo-server
, which runs apollo-server-express under the hood.
const { ApolloServer, gql } = require('apollo-server');
// pass in a schema
const server = new ApolloServer({
schema
});
// or let Apollo make it for you
const server = new ApolloServer({
typeDefs,
resolvers,
});
server.listen()
None of these solutions require you to include body-parser
and provide a ton of extra features out-of-the-box.
I've kind of rethought how to perform the resolvers. Because since I'm using express and was using REST, I was basically passing everything via(req, res)
but having thought about it for a while, I should really do preliminary validation via another function, which then calls another function to actually execute read/writes onto my mongo db.
– A. Lau
Nov 22 '18 at 7:50
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53422461%2fgraphql-query-is-embedded-inside-req-body%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Unless there's a specific reason you want to validate and execute the GraphQL document yourself, you should probably use an existing library for that. Two of the more popular solutions for express:
//express-graphql
const graphqlHTTP = require('express-graphql');
const { makeExecutableSchema } = require('graphql-tools');
const schema = makeExecutableSchema({
typeDefs,
resolvers,
});
app.use('/graphql', graphqlHTTP({
schema,
graphiql: true,
}));
app.listen(4000);
// apollo-server-express
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const server = new ApolloServer({ typeDefs, resolvers });
server.applyMiddleware({ app });
app.listen(4000);
You can also just use apollo-server
, which runs apollo-server-express under the hood.
const { ApolloServer, gql } = require('apollo-server');
// pass in a schema
const server = new ApolloServer({
schema
});
// or let Apollo make it for you
const server = new ApolloServer({
typeDefs,
resolvers,
});
server.listen()
None of these solutions require you to include body-parser
and provide a ton of extra features out-of-the-box.
I've kind of rethought how to perform the resolvers. Because since I'm using express and was using REST, I was basically passing everything via(req, res)
but having thought about it for a while, I should really do preliminary validation via another function, which then calls another function to actually execute read/writes onto my mongo db.
– A. Lau
Nov 22 '18 at 7:50
add a comment |
Unless there's a specific reason you want to validate and execute the GraphQL document yourself, you should probably use an existing library for that. Two of the more popular solutions for express:
//express-graphql
const graphqlHTTP = require('express-graphql');
const { makeExecutableSchema } = require('graphql-tools');
const schema = makeExecutableSchema({
typeDefs,
resolvers,
});
app.use('/graphql', graphqlHTTP({
schema,
graphiql: true,
}));
app.listen(4000);
// apollo-server-express
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const server = new ApolloServer({ typeDefs, resolvers });
server.applyMiddleware({ app });
app.listen(4000);
You can also just use apollo-server
, which runs apollo-server-express under the hood.
const { ApolloServer, gql } = require('apollo-server');
// pass in a schema
const server = new ApolloServer({
schema
});
// or let Apollo make it for you
const server = new ApolloServer({
typeDefs,
resolvers,
});
server.listen()
None of these solutions require you to include body-parser
and provide a ton of extra features out-of-the-box.
I've kind of rethought how to perform the resolvers. Because since I'm using express and was using REST, I was basically passing everything via(req, res)
but having thought about it for a while, I should really do preliminary validation via another function, which then calls another function to actually execute read/writes onto my mongo db.
– A. Lau
Nov 22 '18 at 7:50
add a comment |
Unless there's a specific reason you want to validate and execute the GraphQL document yourself, you should probably use an existing library for that. Two of the more popular solutions for express:
//express-graphql
const graphqlHTTP = require('express-graphql');
const { makeExecutableSchema } = require('graphql-tools');
const schema = makeExecutableSchema({
typeDefs,
resolvers,
});
app.use('/graphql', graphqlHTTP({
schema,
graphiql: true,
}));
app.listen(4000);
// apollo-server-express
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const server = new ApolloServer({ typeDefs, resolvers });
server.applyMiddleware({ app });
app.listen(4000);
You can also just use apollo-server
, which runs apollo-server-express under the hood.
const { ApolloServer, gql } = require('apollo-server');
// pass in a schema
const server = new ApolloServer({
schema
});
// or let Apollo make it for you
const server = new ApolloServer({
typeDefs,
resolvers,
});
server.listen()
None of these solutions require you to include body-parser
and provide a ton of extra features out-of-the-box.
Unless there's a specific reason you want to validate and execute the GraphQL document yourself, you should probably use an existing library for that. Two of the more popular solutions for express:
//express-graphql
const graphqlHTTP = require('express-graphql');
const { makeExecutableSchema } = require('graphql-tools');
const schema = makeExecutableSchema({
typeDefs,
resolvers,
});
app.use('/graphql', graphqlHTTP({
schema,
graphiql: true,
}));
app.listen(4000);
// apollo-server-express
const express = require('express');
const { ApolloServer, gql } = require('apollo-server-express');
const server = new ApolloServer({ typeDefs, resolvers });
server.applyMiddleware({ app });
app.listen(4000);
You can also just use apollo-server
, which runs apollo-server-express under the hood.
const { ApolloServer, gql } = require('apollo-server');
// pass in a schema
const server = new ApolloServer({
schema
});
// or let Apollo make it for you
const server = new ApolloServer({
typeDefs,
resolvers,
});
server.listen()
None of these solutions require you to include body-parser
and provide a ton of extra features out-of-the-box.
answered Nov 22 '18 at 3:54


Daniel ReardenDaniel Rearden
16.6k12045
16.6k12045
I've kind of rethought how to perform the resolvers. Because since I'm using express and was using REST, I was basically passing everything via(req, res)
but having thought about it for a while, I should really do preliminary validation via another function, which then calls another function to actually execute read/writes onto my mongo db.
– A. Lau
Nov 22 '18 at 7:50
add a comment |
I've kind of rethought how to perform the resolvers. Because since I'm using express and was using REST, I was basically passing everything via(req, res)
but having thought about it for a while, I should really do preliminary validation via another function, which then calls another function to actually execute read/writes onto my mongo db.
– A. Lau
Nov 22 '18 at 7:50
I've kind of rethought how to perform the resolvers. Because since I'm using express and was using REST, I was basically passing everything via
(req, res)
but having thought about it for a while, I should really do preliminary validation via another function, which then calls another function to actually execute read/writes onto my mongo db.– A. Lau
Nov 22 '18 at 7:50
I've kind of rethought how to perform the resolvers. Because since I'm using express and was using REST, I was basically passing everything via
(req, res)
but having thought about it for a while, I should really do preliminary validation via another function, which then calls another function to actually execute read/writes onto my mongo db.– A. Lau
Nov 22 '18 at 7:50
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53422461%2fgraphql-query-is-embedded-inside-req-body%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kbNOeQ,aTa0cQo,X9mQWpnNkNcOiPnvawdvf,pbe,omM,uPaZlranOXSH