Configure LocaldateTime in Spring Rest API
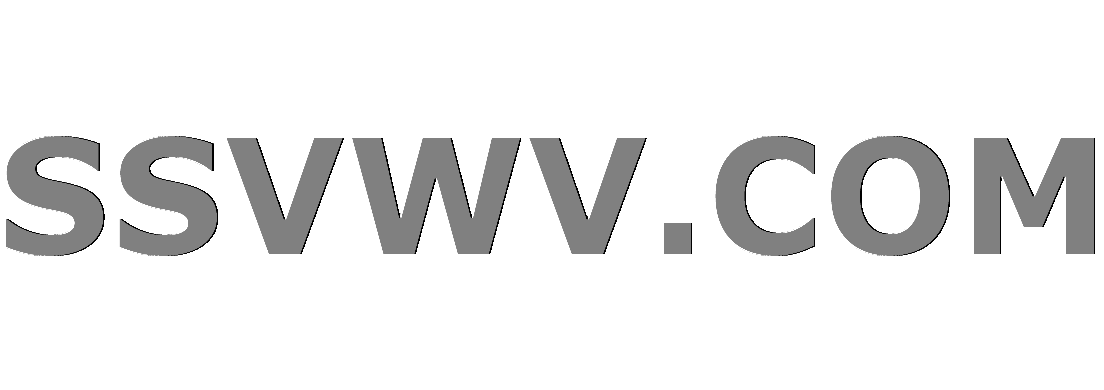
Multi tool use
I use Java 10 with latest Spring spring-boot-starter-parent 2.1.0.RELEASE
POM configuration:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.7</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.module</groupId>
<artifactId>jackson-module-jaxb-annotations</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.jaxrs</groupId>
<artifactId>jackson-jaxrs-json-provider</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<dependency>
<groupId>org.codehaus.woodstox</groupId>
<artifactId>woodstox-core-asl</artifactId>
<version>4.4.1</version>
</dependency>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-core</artifactId>
<version>2.3.0.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-jdk8</artifactId>
<version>1.2.0.Final</version>
</dependency>
<dependency>
<groupId>org.jxls</groupId>
<artifactId>jxls-poi</artifactId>
<version>1.0.15</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
</dependencies>
Rest Endpoint:
@GetMapping("{id}")
public ResponseEntity<?> get(@PathVariable String id) {
return transactionRepository
.findById(Integer.parseInt(id))
.map(mapper::toDTO)
.map(ResponseEntity::ok)
.orElseGet(() -> notFound().build());
}
DTO:
public class PaymentTransactionsDTO {
private Integer id;
private String status;
private LocalDateTime created_at;
private String merchant;
.... getters and setters
}
But when I try to return JSON data for LocalDateTime created_at I get empty result. I suppose that LocalDateTime is not properly converted into JSON value.
Can you advice how I can fix this issue?
spring spring-boot spring-data-jpa spring-data
add a comment |
I use Java 10 with latest Spring spring-boot-starter-parent 2.1.0.RELEASE
POM configuration:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.7</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.module</groupId>
<artifactId>jackson-module-jaxb-annotations</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.jaxrs</groupId>
<artifactId>jackson-jaxrs-json-provider</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<dependency>
<groupId>org.codehaus.woodstox</groupId>
<artifactId>woodstox-core-asl</artifactId>
<version>4.4.1</version>
</dependency>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-core</artifactId>
<version>2.3.0.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-jdk8</artifactId>
<version>1.2.0.Final</version>
</dependency>
<dependency>
<groupId>org.jxls</groupId>
<artifactId>jxls-poi</artifactId>
<version>1.0.15</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
</dependencies>
Rest Endpoint:
@GetMapping("{id}")
public ResponseEntity<?> get(@PathVariable String id) {
return transactionRepository
.findById(Integer.parseInt(id))
.map(mapper::toDTO)
.map(ResponseEntity::ok)
.orElseGet(() -> notFound().build());
}
DTO:
public class PaymentTransactionsDTO {
private Integer id;
private String status;
private LocalDateTime created_at;
private String merchant;
.... getters and setters
}
But when I try to return JSON data for LocalDateTime created_at I get empty result. I suppose that LocalDateTime is not properly converted into JSON value.
Can you advice how I can fix this issue?
spring spring-boot spring-data-jpa spring-data
You need to add converter and register in spring eg. baeldung.com/spring-mvc-custom-data-binder
– Vipul
Nov 21 '18 at 7:47
Can you paste official answer so I can rate it, please?
– user1285928
Nov 21 '18 at 8:53
isn't your issue resolved with the converter.
– Vipul
Nov 22 '18 at 4:59
add a comment |
I use Java 10 with latest Spring spring-boot-starter-parent 2.1.0.RELEASE
POM configuration:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.7</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.module</groupId>
<artifactId>jackson-module-jaxb-annotations</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.jaxrs</groupId>
<artifactId>jackson-jaxrs-json-provider</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<dependency>
<groupId>org.codehaus.woodstox</groupId>
<artifactId>woodstox-core-asl</artifactId>
<version>4.4.1</version>
</dependency>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-core</artifactId>
<version>2.3.0.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-jdk8</artifactId>
<version>1.2.0.Final</version>
</dependency>
<dependency>
<groupId>org.jxls</groupId>
<artifactId>jxls-poi</artifactId>
<version>1.0.15</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
</dependencies>
Rest Endpoint:
@GetMapping("{id}")
public ResponseEntity<?> get(@PathVariable String id) {
return transactionRepository
.findById(Integer.parseInt(id))
.map(mapper::toDTO)
.map(ResponseEntity::ok)
.orElseGet(() -> notFound().build());
}
DTO:
public class PaymentTransactionsDTO {
private Integer id;
private String status;
private LocalDateTime created_at;
private String merchant;
.... getters and setters
}
But when I try to return JSON data for LocalDateTime created_at I get empty result. I suppose that LocalDateTime is not properly converted into JSON value.
Can you advice how I can fix this issue?
spring spring-boot spring-data-jpa spring-data
I use Java 10 with latest Spring spring-boot-starter-parent 2.1.0.RELEASE
POM configuration:
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.0.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
<version>2.9.7</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.module</groupId>
<artifactId>jackson-module-jaxb-annotations</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.jaxrs</groupId>
<artifactId>jackson-jaxrs-json-provider</artifactId>
<version>2.9.7</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.6</version>
</dependency>
<dependency>
<groupId>org.codehaus.woodstox</groupId>
<artifactId>woodstox-core-asl</artifactId>
<version>4.4.1</version>
</dependency>
<dependency>
<groupId>javax.xml.bind</groupId>
<artifactId>jaxb-api</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-core</artifactId>
<version>2.3.0.1</version>
</dependency>
<dependency>
<groupId>com.sun.xml.bind</groupId>
<artifactId>jaxb-impl</artifactId>
<version>2.3.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-jdk8</artifactId>
<version>1.2.0.Final</version>
</dependency>
<dependency>
<groupId>org.jxls</groupId>
<artifactId>jxls-poi</artifactId>
<version>1.0.15</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
<dependency>
<groupId>javax</groupId>
<artifactId>javaee-api</artifactId>
<version>8.0</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth</groupId>
<artifactId>spring-security-oauth2</artifactId>
<version>2.3.4.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.security.oauth.boot</groupId>
<artifactId>spring-security-oauth2-autoconfigure</artifactId>
<version>2.1.0.RELEASE</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
</dependencies>
Rest Endpoint:
@GetMapping("{id}")
public ResponseEntity<?> get(@PathVariable String id) {
return transactionRepository
.findById(Integer.parseInt(id))
.map(mapper::toDTO)
.map(ResponseEntity::ok)
.orElseGet(() -> notFound().build());
}
DTO:
public class PaymentTransactionsDTO {
private Integer id;
private String status;
private LocalDateTime created_at;
private String merchant;
.... getters and setters
}
But when I try to return JSON data for LocalDateTime created_at I get empty result. I suppose that LocalDateTime is not properly converted into JSON value.
Can you advice how I can fix this issue?
spring spring-boot spring-data-jpa spring-data
spring spring-boot spring-data-jpa spring-data
asked Nov 18 '18 at 22:04
user1285928user1285928
3911869129
3911869129
You need to add converter and register in spring eg. baeldung.com/spring-mvc-custom-data-binder
– Vipul
Nov 21 '18 at 7:47
Can you paste official answer so I can rate it, please?
– user1285928
Nov 21 '18 at 8:53
isn't your issue resolved with the converter.
– Vipul
Nov 22 '18 at 4:59
add a comment |
You need to add converter and register in spring eg. baeldung.com/spring-mvc-custom-data-binder
– Vipul
Nov 21 '18 at 7:47
Can you paste official answer so I can rate it, please?
– user1285928
Nov 21 '18 at 8:53
isn't your issue resolved with the converter.
– Vipul
Nov 22 '18 at 4:59
You need to add converter and register in spring eg. baeldung.com/spring-mvc-custom-data-binder
– Vipul
Nov 21 '18 at 7:47
You need to add converter and register in spring eg. baeldung.com/spring-mvc-custom-data-binder
– Vipul
Nov 21 '18 at 7:47
Can you paste official answer so I can rate it, please?
– user1285928
Nov 21 '18 at 8:53
Can you paste official answer so I can rate it, please?
– user1285928
Nov 21 '18 at 8:53
isn't your issue resolved with the converter.
– Vipul
Nov 22 '18 at 4:59
isn't your issue resolved with the converter.
– Vipul
Nov 22 '18 at 4:59
add a comment |
4 Answers
4
active
oldest
votes
JSON serialization is driven by Jackson's ObjectMapper
, which I recommend configuring explicitely. For proper serialization of the Java 8 date and time objects, make sure to
- register the
JavaTimeModule
- disable writing dates as timestamps
- setting the date format (use
StdDateFormat
)
Description of StdDateFormat
:
Default
DateFormat
implementation used by standard Date
serializers and deserializers. For serialization defaults to using an
ISO-8601 compliant format (format String "yyyy-MM-dd'T'HH:mm:ss.SSSZ")
and for deserialization, both ISO-8601 and RFC-1123.
Recommended configuration:
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper mapper = new ObjectMapper();
mapper.setAnnotationIntrospector(new JacksonAnnotationIntrospector());
mapper.registerModule(new JavaTimeModule());
mapper.disable(SerializationFeature.CLOSE_CLOSEABLE.WRITE_DATES_AS_TIMESTAMPS);
mapper.setDateFormat(new StdDateFormat());
return mapper;
}
}
Examples of serialized date and time objects:
LocalDate
: 2018-11-21
LocalTime
: 11:13:13.274
LocalDateTime
: 2018-11-21T11:13:13.274
ZonedDateTime
: 2018-11-21T11:13:13.274+01:00
Edit: standalone dependencies (already included transitively in spring-boot-starter-web
):
com.fasterxml.jackson.core:jackson-annotations
com.fasterxml.jackson.core:jackson-databind
com.fasterxml.jackson.datatype:jackson-datatype-jsr310
What do you mean byregister the JavaTimeModule
? Add maven dependency?
– user1285928
Nov 21 '18 at 10:28
It means the linemapper.registerModule(new JavaTimeModule());
. I edited the post to list the explicit jackson modules required, however they are already included inspring-boot-starter-web
.
– Peter Walser
Nov 21 '18 at 11:23
add a comment |
Try using @JsonFormat on your created_at field.
@JsonFormat(pattern="yyyy-MM-dd")
@DateTimeFormat(iso = DateTimeFormat.ISO.TIME)
private LocalDateTime created_at;
No, it's not fixing the issue.
– user1285928
Nov 19 '18 at 11:25
Probably I'm applying this annotation on DTO not in JPA Entity?
– user1285928
Nov 20 '18 at 19:17
You need to apply it on DTO only. You can use a workaround by taking string instead of local date time and convert your local date time into string. This is not the best approach but may be helpful.
– Pooja Aggarwal
Nov 21 '18 at 1:37
Also you can try solutions from stackoverflow.com/questions/28802544/…
– Pooja Aggarwal
Nov 21 '18 at 1:39
add a comment |
You need to add converter and register in spring eg.
baeldung.com/spring-mvc-custom-data-binder
add a comment |
You can type your PaymentTransactionsDTO "created_at" attribute as a String and use a converter to convert the String to LocalDate (type of the attribute created_at of your entity "PaymentTransactions")
@Component
public class PaymentTransactionsConverter implements Converter<PaymentTransactionsDTO, PaymentTransactions> {
@Override
public PaymentTransactions convert(PaymentTransactionsDTO paymentTransactionsDTO) {
PaymentTransactions paymentTransactions = new PaymentTransactions();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
...
paymentTransactions.setCreated_at(LocalDate.parse(paymentTransactionsDTO.getCreated_at(), formatter));
return paymentTransactions;
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53365917%2fconfigure-localdatetime-in-spring-rest-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
JSON serialization is driven by Jackson's ObjectMapper
, which I recommend configuring explicitely. For proper serialization of the Java 8 date and time objects, make sure to
- register the
JavaTimeModule
- disable writing dates as timestamps
- setting the date format (use
StdDateFormat
)
Description of StdDateFormat
:
Default
DateFormat
implementation used by standard Date
serializers and deserializers. For serialization defaults to using an
ISO-8601 compliant format (format String "yyyy-MM-dd'T'HH:mm:ss.SSSZ")
and for deserialization, both ISO-8601 and RFC-1123.
Recommended configuration:
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper mapper = new ObjectMapper();
mapper.setAnnotationIntrospector(new JacksonAnnotationIntrospector());
mapper.registerModule(new JavaTimeModule());
mapper.disable(SerializationFeature.CLOSE_CLOSEABLE.WRITE_DATES_AS_TIMESTAMPS);
mapper.setDateFormat(new StdDateFormat());
return mapper;
}
}
Examples of serialized date and time objects:
LocalDate
: 2018-11-21
LocalTime
: 11:13:13.274
LocalDateTime
: 2018-11-21T11:13:13.274
ZonedDateTime
: 2018-11-21T11:13:13.274+01:00
Edit: standalone dependencies (already included transitively in spring-boot-starter-web
):
com.fasterxml.jackson.core:jackson-annotations
com.fasterxml.jackson.core:jackson-databind
com.fasterxml.jackson.datatype:jackson-datatype-jsr310
What do you mean byregister the JavaTimeModule
? Add maven dependency?
– user1285928
Nov 21 '18 at 10:28
It means the linemapper.registerModule(new JavaTimeModule());
. I edited the post to list the explicit jackson modules required, however they are already included inspring-boot-starter-web
.
– Peter Walser
Nov 21 '18 at 11:23
add a comment |
JSON serialization is driven by Jackson's ObjectMapper
, which I recommend configuring explicitely. For proper serialization of the Java 8 date and time objects, make sure to
- register the
JavaTimeModule
- disable writing dates as timestamps
- setting the date format (use
StdDateFormat
)
Description of StdDateFormat
:
Default
DateFormat
implementation used by standard Date
serializers and deserializers. For serialization defaults to using an
ISO-8601 compliant format (format String "yyyy-MM-dd'T'HH:mm:ss.SSSZ")
and for deserialization, both ISO-8601 and RFC-1123.
Recommended configuration:
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper mapper = new ObjectMapper();
mapper.setAnnotationIntrospector(new JacksonAnnotationIntrospector());
mapper.registerModule(new JavaTimeModule());
mapper.disable(SerializationFeature.CLOSE_CLOSEABLE.WRITE_DATES_AS_TIMESTAMPS);
mapper.setDateFormat(new StdDateFormat());
return mapper;
}
}
Examples of serialized date and time objects:
LocalDate
: 2018-11-21
LocalTime
: 11:13:13.274
LocalDateTime
: 2018-11-21T11:13:13.274
ZonedDateTime
: 2018-11-21T11:13:13.274+01:00
Edit: standalone dependencies (already included transitively in spring-boot-starter-web
):
com.fasterxml.jackson.core:jackson-annotations
com.fasterxml.jackson.core:jackson-databind
com.fasterxml.jackson.datatype:jackson-datatype-jsr310
What do you mean byregister the JavaTimeModule
? Add maven dependency?
– user1285928
Nov 21 '18 at 10:28
It means the linemapper.registerModule(new JavaTimeModule());
. I edited the post to list the explicit jackson modules required, however they are already included inspring-boot-starter-web
.
– Peter Walser
Nov 21 '18 at 11:23
add a comment |
JSON serialization is driven by Jackson's ObjectMapper
, which I recommend configuring explicitely. For proper serialization of the Java 8 date and time objects, make sure to
- register the
JavaTimeModule
- disable writing dates as timestamps
- setting the date format (use
StdDateFormat
)
Description of StdDateFormat
:
Default
DateFormat
implementation used by standard Date
serializers and deserializers. For serialization defaults to using an
ISO-8601 compliant format (format String "yyyy-MM-dd'T'HH:mm:ss.SSSZ")
and for deserialization, both ISO-8601 and RFC-1123.
Recommended configuration:
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper mapper = new ObjectMapper();
mapper.setAnnotationIntrospector(new JacksonAnnotationIntrospector());
mapper.registerModule(new JavaTimeModule());
mapper.disable(SerializationFeature.CLOSE_CLOSEABLE.WRITE_DATES_AS_TIMESTAMPS);
mapper.setDateFormat(new StdDateFormat());
return mapper;
}
}
Examples of serialized date and time objects:
LocalDate
: 2018-11-21
LocalTime
: 11:13:13.274
LocalDateTime
: 2018-11-21T11:13:13.274
ZonedDateTime
: 2018-11-21T11:13:13.274+01:00
Edit: standalone dependencies (already included transitively in spring-boot-starter-web
):
com.fasterxml.jackson.core:jackson-annotations
com.fasterxml.jackson.core:jackson-databind
com.fasterxml.jackson.datatype:jackson-datatype-jsr310
JSON serialization is driven by Jackson's ObjectMapper
, which I recommend configuring explicitely. For proper serialization of the Java 8 date and time objects, make sure to
- register the
JavaTimeModule
- disable writing dates as timestamps
- setting the date format (use
StdDateFormat
)
Description of StdDateFormat
:
Default
DateFormat
implementation used by standard Date
serializers and deserializers. For serialization defaults to using an
ISO-8601 compliant format (format String "yyyy-MM-dd'T'HH:mm:ss.SSSZ")
and for deserialization, both ISO-8601 and RFC-1123.
Recommended configuration:
@Configuration
public class JacksonConfig {
@Bean
public ObjectMapper objectMapper() {
ObjectMapper mapper = new ObjectMapper();
mapper.setAnnotationIntrospector(new JacksonAnnotationIntrospector());
mapper.registerModule(new JavaTimeModule());
mapper.disable(SerializationFeature.CLOSE_CLOSEABLE.WRITE_DATES_AS_TIMESTAMPS);
mapper.setDateFormat(new StdDateFormat());
return mapper;
}
}
Examples of serialized date and time objects:
LocalDate
: 2018-11-21
LocalTime
: 11:13:13.274
LocalDateTime
: 2018-11-21T11:13:13.274
ZonedDateTime
: 2018-11-21T11:13:13.274+01:00
Edit: standalone dependencies (already included transitively in spring-boot-starter-web
):
com.fasterxml.jackson.core:jackson-annotations
com.fasterxml.jackson.core:jackson-databind
com.fasterxml.jackson.datatype:jackson-datatype-jsr310
edited Nov 21 '18 at 11:22
answered Nov 21 '18 at 10:01
Peter WalserPeter Walser
10.4k23555
10.4k23555
What do you mean byregister the JavaTimeModule
? Add maven dependency?
– user1285928
Nov 21 '18 at 10:28
It means the linemapper.registerModule(new JavaTimeModule());
. I edited the post to list the explicit jackson modules required, however they are already included inspring-boot-starter-web
.
– Peter Walser
Nov 21 '18 at 11:23
add a comment |
What do you mean byregister the JavaTimeModule
? Add maven dependency?
– user1285928
Nov 21 '18 at 10:28
It means the linemapper.registerModule(new JavaTimeModule());
. I edited the post to list the explicit jackson modules required, however they are already included inspring-boot-starter-web
.
– Peter Walser
Nov 21 '18 at 11:23
What do you mean by
register the JavaTimeModule
? Add maven dependency?– user1285928
Nov 21 '18 at 10:28
What do you mean by
register the JavaTimeModule
? Add maven dependency?– user1285928
Nov 21 '18 at 10:28
It means the line
mapper.registerModule(new JavaTimeModule());
. I edited the post to list the explicit jackson modules required, however they are already included in spring-boot-starter-web
.– Peter Walser
Nov 21 '18 at 11:23
It means the line
mapper.registerModule(new JavaTimeModule());
. I edited the post to list the explicit jackson modules required, however they are already included in spring-boot-starter-web
.– Peter Walser
Nov 21 '18 at 11:23
add a comment |
Try using @JsonFormat on your created_at field.
@JsonFormat(pattern="yyyy-MM-dd")
@DateTimeFormat(iso = DateTimeFormat.ISO.TIME)
private LocalDateTime created_at;
No, it's not fixing the issue.
– user1285928
Nov 19 '18 at 11:25
Probably I'm applying this annotation on DTO not in JPA Entity?
– user1285928
Nov 20 '18 at 19:17
You need to apply it on DTO only. You can use a workaround by taking string instead of local date time and convert your local date time into string. This is not the best approach but may be helpful.
– Pooja Aggarwal
Nov 21 '18 at 1:37
Also you can try solutions from stackoverflow.com/questions/28802544/…
– Pooja Aggarwal
Nov 21 '18 at 1:39
add a comment |
Try using @JsonFormat on your created_at field.
@JsonFormat(pattern="yyyy-MM-dd")
@DateTimeFormat(iso = DateTimeFormat.ISO.TIME)
private LocalDateTime created_at;
No, it's not fixing the issue.
– user1285928
Nov 19 '18 at 11:25
Probably I'm applying this annotation on DTO not in JPA Entity?
– user1285928
Nov 20 '18 at 19:17
You need to apply it on DTO only. You can use a workaround by taking string instead of local date time and convert your local date time into string. This is not the best approach but may be helpful.
– Pooja Aggarwal
Nov 21 '18 at 1:37
Also you can try solutions from stackoverflow.com/questions/28802544/…
– Pooja Aggarwal
Nov 21 '18 at 1:39
add a comment |
Try using @JsonFormat on your created_at field.
@JsonFormat(pattern="yyyy-MM-dd")
@DateTimeFormat(iso = DateTimeFormat.ISO.TIME)
private LocalDateTime created_at;
Try using @JsonFormat on your created_at field.
@JsonFormat(pattern="yyyy-MM-dd")
@DateTimeFormat(iso = DateTimeFormat.ISO.TIME)
private LocalDateTime created_at;
answered Nov 19 '18 at 5:26
Pooja AggarwalPooja Aggarwal
860111
860111
No, it's not fixing the issue.
– user1285928
Nov 19 '18 at 11:25
Probably I'm applying this annotation on DTO not in JPA Entity?
– user1285928
Nov 20 '18 at 19:17
You need to apply it on DTO only. You can use a workaround by taking string instead of local date time and convert your local date time into string. This is not the best approach but may be helpful.
– Pooja Aggarwal
Nov 21 '18 at 1:37
Also you can try solutions from stackoverflow.com/questions/28802544/…
– Pooja Aggarwal
Nov 21 '18 at 1:39
add a comment |
No, it's not fixing the issue.
– user1285928
Nov 19 '18 at 11:25
Probably I'm applying this annotation on DTO not in JPA Entity?
– user1285928
Nov 20 '18 at 19:17
You need to apply it on DTO only. You can use a workaround by taking string instead of local date time and convert your local date time into string. This is not the best approach but may be helpful.
– Pooja Aggarwal
Nov 21 '18 at 1:37
Also you can try solutions from stackoverflow.com/questions/28802544/…
– Pooja Aggarwal
Nov 21 '18 at 1:39
No, it's not fixing the issue.
– user1285928
Nov 19 '18 at 11:25
No, it's not fixing the issue.
– user1285928
Nov 19 '18 at 11:25
Probably I'm applying this annotation on DTO not in JPA Entity?
– user1285928
Nov 20 '18 at 19:17
Probably I'm applying this annotation on DTO not in JPA Entity?
– user1285928
Nov 20 '18 at 19:17
You need to apply it on DTO only. You can use a workaround by taking string instead of local date time and convert your local date time into string. This is not the best approach but may be helpful.
– Pooja Aggarwal
Nov 21 '18 at 1:37
You need to apply it on DTO only. You can use a workaround by taking string instead of local date time and convert your local date time into string. This is not the best approach but may be helpful.
– Pooja Aggarwal
Nov 21 '18 at 1:37
Also you can try solutions from stackoverflow.com/questions/28802544/…
– Pooja Aggarwal
Nov 21 '18 at 1:39
Also you can try solutions from stackoverflow.com/questions/28802544/…
– Pooja Aggarwal
Nov 21 '18 at 1:39
add a comment |
You need to add converter and register in spring eg.
baeldung.com/spring-mvc-custom-data-binder
add a comment |
You need to add converter and register in spring eg.
baeldung.com/spring-mvc-custom-data-binder
add a comment |
You need to add converter and register in spring eg.
baeldung.com/spring-mvc-custom-data-binder
You need to add converter and register in spring eg.
baeldung.com/spring-mvc-custom-data-binder
answered Nov 21 '18 at 9:52
VipulVipul
439312
439312
add a comment |
add a comment |
You can type your PaymentTransactionsDTO "created_at" attribute as a String and use a converter to convert the String to LocalDate (type of the attribute created_at of your entity "PaymentTransactions")
@Component
public class PaymentTransactionsConverter implements Converter<PaymentTransactionsDTO, PaymentTransactions> {
@Override
public PaymentTransactions convert(PaymentTransactionsDTO paymentTransactionsDTO) {
PaymentTransactions paymentTransactions = new PaymentTransactions();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
...
paymentTransactions.setCreated_at(LocalDate.parse(paymentTransactionsDTO.getCreated_at(), formatter));
return paymentTransactions;
}
}
add a comment |
You can type your PaymentTransactionsDTO "created_at" attribute as a String and use a converter to convert the String to LocalDate (type of the attribute created_at of your entity "PaymentTransactions")
@Component
public class PaymentTransactionsConverter implements Converter<PaymentTransactionsDTO, PaymentTransactions> {
@Override
public PaymentTransactions convert(PaymentTransactionsDTO paymentTransactionsDTO) {
PaymentTransactions paymentTransactions = new PaymentTransactions();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
...
paymentTransactions.setCreated_at(LocalDate.parse(paymentTransactionsDTO.getCreated_at(), formatter));
return paymentTransactions;
}
}
add a comment |
You can type your PaymentTransactionsDTO "created_at" attribute as a String and use a converter to convert the String to LocalDate (type of the attribute created_at of your entity "PaymentTransactions")
@Component
public class PaymentTransactionsConverter implements Converter<PaymentTransactionsDTO, PaymentTransactions> {
@Override
public PaymentTransactions convert(PaymentTransactionsDTO paymentTransactionsDTO) {
PaymentTransactions paymentTransactions = new PaymentTransactions();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
...
paymentTransactions.setCreated_at(LocalDate.parse(paymentTransactionsDTO.getCreated_at(), formatter));
return paymentTransactions;
}
}
You can type your PaymentTransactionsDTO "created_at" attribute as a String and use a converter to convert the String to LocalDate (type of the attribute created_at of your entity "PaymentTransactions")
@Component
public class PaymentTransactionsConverter implements Converter<PaymentTransactionsDTO, PaymentTransactions> {
@Override
public PaymentTransactions convert(PaymentTransactionsDTO paymentTransactionsDTO) {
PaymentTransactions paymentTransactions = new PaymentTransactions();
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
...
paymentTransactions.setCreated_at(LocalDate.parse(paymentTransactionsDTO.getCreated_at(), formatter));
return paymentTransactions;
}
}
answered Nov 21 '18 at 10:13


vebenveben
1,1922921
1,1922921
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53365917%2fconfigure-localdatetime-in-spring-rest-api%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
E b4,zKSv85ng4jXE v,rjg4Oi,uLscutTKO9dH,qQ3,2Zj,0tz6ZEI2dU BxkjWPtPfH 4yQV
You need to add converter and register in spring eg. baeldung.com/spring-mvc-custom-data-binder
– Vipul
Nov 21 '18 at 7:47
Can you paste official answer so I can rate it, please?
– user1285928
Nov 21 '18 at 8:53
isn't your issue resolved with the converter.
– Vipul
Nov 22 '18 at 4:59