Why can't you modify the data returned by a Mongoose Query (ex: findById)
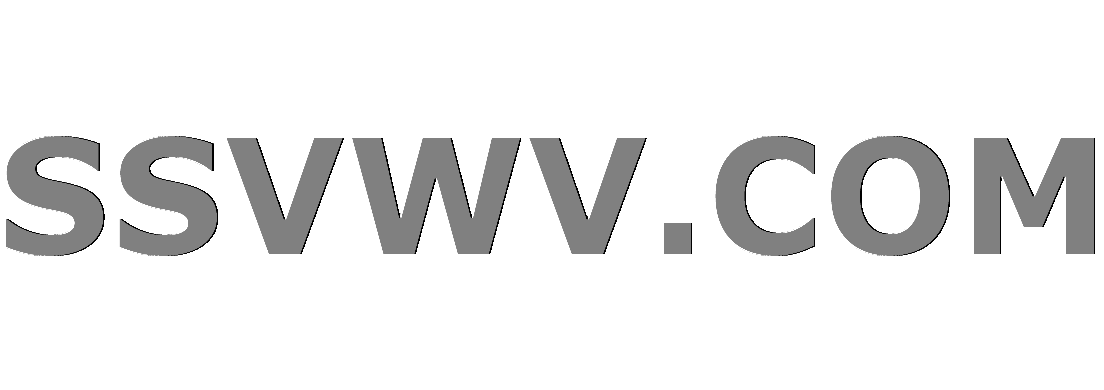
Multi tool use
When I try to change any part of the data returned by a Mongoose Query it has no effect.
I was trying to figure this out for about 2 hours yesterday, with all kinds of _.clone()
s, using temporary storage variables, etc. Finally, just when I though I was going crazy, I found a solution. So I figured somebody in the future (fyuuuture!) might have the save issue.
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q; //has no effect
if(++counter == len) {
res.send(data);
}
});
});
});
node.js mongoose
add a comment |
When I try to change any part of the data returned by a Mongoose Query it has no effect.
I was trying to figure this out for about 2 hours yesterday, with all kinds of _.clone()
s, using temporary storage variables, etc. Finally, just when I though I was going crazy, I found a solution. So I figured somebody in the future (fyuuuture!) might have the save issue.
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q; //has no effect
if(++counter == len) {
res.send(data);
}
});
});
});
node.js mongoose
1
possible duplicate of How do you turn a Mongoose document into a plain object?
– Blakes Seven
Jul 21 '15 at 9:56
add a comment |
When I try to change any part of the data returned by a Mongoose Query it has no effect.
I was trying to figure this out for about 2 hours yesterday, with all kinds of _.clone()
s, using temporary storage variables, etc. Finally, just when I though I was going crazy, I found a solution. So I figured somebody in the future (fyuuuture!) might have the save issue.
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q; //has no effect
if(++counter == len) {
res.send(data);
}
});
});
});
node.js mongoose
When I try to change any part of the data returned by a Mongoose Query it has no effect.
I was trying to figure this out for about 2 hours yesterday, with all kinds of _.clone()
s, using temporary storage variables, etc. Finally, just when I though I was going crazy, I found a solution. So I figured somebody in the future (fyuuuture!) might have the save issue.
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q; //has no effect
if(++counter == len) {
res.send(data);
}
});
});
});
node.js mongoose
node.js mongoose
asked Jan 24 '13 at 15:03


ToliToli
2,13141935
2,13141935
1
possible duplicate of How do you turn a Mongoose document into a plain object?
– Blakes Seven
Jul 21 '15 at 9:56
add a comment |
1
possible duplicate of How do you turn a Mongoose document into a plain object?
– Blakes Seven
Jul 21 '15 at 9:56
1
1
possible duplicate of How do you turn a Mongoose document into a plain object?
– Blakes Seven
Jul 21 '15 at 9:56
possible duplicate of How do you turn a Mongoose document into a plain object?
– Blakes Seven
Jul 21 '15 at 9:56
add a comment |
2 Answers
2
active
oldest
votes
For cases like this where you want a plain JS object instead of a full model instance, you can call lean()
on the query chain like so:
Survey.findById(req.params.id).lean().exec(function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
This way data
is already a plain JS object you can manipulate as you need to.
8
Btw @JohnnyHK just wanted to say thanks again. A year and a half later was helping a client debug something. He spent a weekend trying to figure something out, turns out it was because he was trying to modify the Mongoose Object ;P
– Toli
Jul 14 '14 at 13:54
2 years later and still crushing it. Didn't even realize lean() was there.
– Petrogad
Dec 17 '15 at 12:43
When usingfindOne
, I can just modify the object and then calldata.save()
which seems to work just fine (I'm appending to an array)
– developius
Feb 8 '16 at 20:38
What about when usingaggregate
instead of a simplefind
?
– Fizzix
Jun 1 '16 at 0:04
1
@Fizzixaggregate
always provides its results as plain objects, so there's no need forlean()
.
– JohnnyHK
Jun 1 '16 at 3:59
|
show 2 more comments
I think the Mongoose documentation doesn't make this clear enough, but the data returned in the query (although you can res.send() it) is actually a Mongoose Document object, and NOT a JSON object. But you can fix this with one line...
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
var data = data.toJSON(); //turns it into JSON YAY!
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
12
You can also usetoObject()
, which does the same thing astoJSON()
but with a less confusing name.
– JohnnyHK
May 31 '15 at 14:35
1
Will this also get rid of virtuals put on by the developer as well?
– mjwrazor
Sep 14 '16 at 19:14
4
TypeError: data.toObject is not a function
I got this, same withtoJSON
– Luzan Baral
May 23 '18 at 11:05
Instead of modifying theresult
, I was able to modifyresult._doc
.
– nth-chile
Nov 22 '18 at 1:18
add a comment |
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f14504385%2fwhy-cant-you-modify-the-data-returned-by-a-mongoose-query-ex-findbyid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
For cases like this where you want a plain JS object instead of a full model instance, you can call lean()
on the query chain like so:
Survey.findById(req.params.id).lean().exec(function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
This way data
is already a plain JS object you can manipulate as you need to.
8
Btw @JohnnyHK just wanted to say thanks again. A year and a half later was helping a client debug something. He spent a weekend trying to figure something out, turns out it was because he was trying to modify the Mongoose Object ;P
– Toli
Jul 14 '14 at 13:54
2 years later and still crushing it. Didn't even realize lean() was there.
– Petrogad
Dec 17 '15 at 12:43
When usingfindOne
, I can just modify the object and then calldata.save()
which seems to work just fine (I'm appending to an array)
– developius
Feb 8 '16 at 20:38
What about when usingaggregate
instead of a simplefind
?
– Fizzix
Jun 1 '16 at 0:04
1
@Fizzixaggregate
always provides its results as plain objects, so there's no need forlean()
.
– JohnnyHK
Jun 1 '16 at 3:59
|
show 2 more comments
For cases like this where you want a plain JS object instead of a full model instance, you can call lean()
on the query chain like so:
Survey.findById(req.params.id).lean().exec(function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
This way data
is already a plain JS object you can manipulate as you need to.
8
Btw @JohnnyHK just wanted to say thanks again. A year and a half later was helping a client debug something. He spent a weekend trying to figure something out, turns out it was because he was trying to modify the Mongoose Object ;P
– Toli
Jul 14 '14 at 13:54
2 years later and still crushing it. Didn't even realize lean() was there.
– Petrogad
Dec 17 '15 at 12:43
When usingfindOne
, I can just modify the object and then calldata.save()
which seems to work just fine (I'm appending to an array)
– developius
Feb 8 '16 at 20:38
What about when usingaggregate
instead of a simplefind
?
– Fizzix
Jun 1 '16 at 0:04
1
@Fizzixaggregate
always provides its results as plain objects, so there's no need forlean()
.
– JohnnyHK
Jun 1 '16 at 3:59
|
show 2 more comments
For cases like this where you want a plain JS object instead of a full model instance, you can call lean()
on the query chain like so:
Survey.findById(req.params.id).lean().exec(function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
This way data
is already a plain JS object you can manipulate as you need to.
For cases like this where you want a plain JS object instead of a full model instance, you can call lean()
on the query chain like so:
Survey.findById(req.params.id).lean().exec(function(err, data){
var len = data.survey_questions.length;
var counter = 0;
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
This way data
is already a plain JS object you can manipulate as you need to.
answered Jan 24 '13 at 21:09
JohnnyHKJohnnyHK
213k42453378
213k42453378
8
Btw @JohnnyHK just wanted to say thanks again. A year and a half later was helping a client debug something. He spent a weekend trying to figure something out, turns out it was because he was trying to modify the Mongoose Object ;P
– Toli
Jul 14 '14 at 13:54
2 years later and still crushing it. Didn't even realize lean() was there.
– Petrogad
Dec 17 '15 at 12:43
When usingfindOne
, I can just modify the object and then calldata.save()
which seems to work just fine (I'm appending to an array)
– developius
Feb 8 '16 at 20:38
What about when usingaggregate
instead of a simplefind
?
– Fizzix
Jun 1 '16 at 0:04
1
@Fizzixaggregate
always provides its results as plain objects, so there's no need forlean()
.
– JohnnyHK
Jun 1 '16 at 3:59
|
show 2 more comments
8
Btw @JohnnyHK just wanted to say thanks again. A year and a half later was helping a client debug something. He spent a weekend trying to figure something out, turns out it was because he was trying to modify the Mongoose Object ;P
– Toli
Jul 14 '14 at 13:54
2 years later and still crushing it. Didn't even realize lean() was there.
– Petrogad
Dec 17 '15 at 12:43
When usingfindOne
, I can just modify the object and then calldata.save()
which seems to work just fine (I'm appending to an array)
– developius
Feb 8 '16 at 20:38
What about when usingaggregate
instead of a simplefind
?
– Fizzix
Jun 1 '16 at 0:04
1
@Fizzixaggregate
always provides its results as plain objects, so there's no need forlean()
.
– JohnnyHK
Jun 1 '16 at 3:59
8
8
Btw @JohnnyHK just wanted to say thanks again. A year and a half later was helping a client debug something. He spent a weekend trying to figure something out, turns out it was because he was trying to modify the Mongoose Object ;P
– Toli
Jul 14 '14 at 13:54
Btw @JohnnyHK just wanted to say thanks again. A year and a half later was helping a client debug something. He spent a weekend trying to figure something out, turns out it was because he was trying to modify the Mongoose Object ;P
– Toli
Jul 14 '14 at 13:54
2 years later and still crushing it. Didn't even realize lean() was there.
– Petrogad
Dec 17 '15 at 12:43
2 years later and still crushing it. Didn't even realize lean() was there.
– Petrogad
Dec 17 '15 at 12:43
When using
findOne
, I can just modify the object and then call data.save()
which seems to work just fine (I'm appending to an array)– developius
Feb 8 '16 at 20:38
When using
findOne
, I can just modify the object and then call data.save()
which seems to work just fine (I'm appending to an array)– developius
Feb 8 '16 at 20:38
What about when using
aggregate
instead of a simple find
?– Fizzix
Jun 1 '16 at 0:04
What about when using
aggregate
instead of a simple find
?– Fizzix
Jun 1 '16 at 0:04
1
1
@Fizzix
aggregate
always provides its results as plain objects, so there's no need for lean()
.– JohnnyHK
Jun 1 '16 at 3:59
@Fizzix
aggregate
always provides its results as plain objects, so there's no need for lean()
.– JohnnyHK
Jun 1 '16 at 3:59
|
show 2 more comments
I think the Mongoose documentation doesn't make this clear enough, but the data returned in the query (although you can res.send() it) is actually a Mongoose Document object, and NOT a JSON object. But you can fix this with one line...
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
var data = data.toJSON(); //turns it into JSON YAY!
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
12
You can also usetoObject()
, which does the same thing astoJSON()
but with a less confusing name.
– JohnnyHK
May 31 '15 at 14:35
1
Will this also get rid of virtuals put on by the developer as well?
– mjwrazor
Sep 14 '16 at 19:14
4
TypeError: data.toObject is not a function
I got this, same withtoJSON
– Luzan Baral
May 23 '18 at 11:05
Instead of modifying theresult
, I was able to modifyresult._doc
.
– nth-chile
Nov 22 '18 at 1:18
add a comment |
I think the Mongoose documentation doesn't make this clear enough, but the data returned in the query (although you can res.send() it) is actually a Mongoose Document object, and NOT a JSON object. But you can fix this with one line...
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
var data = data.toJSON(); //turns it into JSON YAY!
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
12
You can also usetoObject()
, which does the same thing astoJSON()
but with a less confusing name.
– JohnnyHK
May 31 '15 at 14:35
1
Will this also get rid of virtuals put on by the developer as well?
– mjwrazor
Sep 14 '16 at 19:14
4
TypeError: data.toObject is not a function
I got this, same withtoJSON
– Luzan Baral
May 23 '18 at 11:05
Instead of modifying theresult
, I was able to modifyresult._doc
.
– nth-chile
Nov 22 '18 at 1:18
add a comment |
I think the Mongoose documentation doesn't make this clear enough, but the data returned in the query (although you can res.send() it) is actually a Mongoose Document object, and NOT a JSON object. But you can fix this with one line...
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
var data = data.toJSON(); //turns it into JSON YAY!
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
I think the Mongoose documentation doesn't make this clear enough, but the data returned in the query (although you can res.send() it) is actually a Mongoose Document object, and NOT a JSON object. But you can fix this with one line...
Survey.findById(req.params.id, function(err, data){
var len = data.survey_questions.length;
var counter = 0;
var data = data.toJSON(); //turns it into JSON YAY!
_.each(data.survey_questions, function(sq){
Question.findById(sq.question, function(err, q){
sq.question = q;
if(++counter == len) {
res.send(data);
}
});
});
});
answered Jan 24 '13 at 15:03


ToliToli
2,13141935
2,13141935
12
You can also usetoObject()
, which does the same thing astoJSON()
but with a less confusing name.
– JohnnyHK
May 31 '15 at 14:35
1
Will this also get rid of virtuals put on by the developer as well?
– mjwrazor
Sep 14 '16 at 19:14
4
TypeError: data.toObject is not a function
I got this, same withtoJSON
– Luzan Baral
May 23 '18 at 11:05
Instead of modifying theresult
, I was able to modifyresult._doc
.
– nth-chile
Nov 22 '18 at 1:18
add a comment |
12
You can also usetoObject()
, which does the same thing astoJSON()
but with a less confusing name.
– JohnnyHK
May 31 '15 at 14:35
1
Will this also get rid of virtuals put on by the developer as well?
– mjwrazor
Sep 14 '16 at 19:14
4
TypeError: data.toObject is not a function
I got this, same withtoJSON
– Luzan Baral
May 23 '18 at 11:05
Instead of modifying theresult
, I was able to modifyresult._doc
.
– nth-chile
Nov 22 '18 at 1:18
12
12
You can also use
toObject()
, which does the same thing as toJSON()
but with a less confusing name.– JohnnyHK
May 31 '15 at 14:35
You can also use
toObject()
, which does the same thing as toJSON()
but with a less confusing name.– JohnnyHK
May 31 '15 at 14:35
1
1
Will this also get rid of virtuals put on by the developer as well?
– mjwrazor
Sep 14 '16 at 19:14
Will this also get rid of virtuals put on by the developer as well?
– mjwrazor
Sep 14 '16 at 19:14
4
4
TypeError: data.toObject is not a function
I got this, same with toJSON
– Luzan Baral
May 23 '18 at 11:05
TypeError: data.toObject is not a function
I got this, same with toJSON
– Luzan Baral
May 23 '18 at 11:05
Instead of modifying the
result
, I was able to modify result._doc
.– nth-chile
Nov 22 '18 at 1:18
Instead of modifying the
result
, I was able to modify result._doc
.– nth-chile
Nov 22 '18 at 1:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f14504385%2fwhy-cant-you-modify-the-data-returned-by-a-mongoose-query-ex-findbyid%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
KHy9exPsqf,to,MUCt7Dg5pLiYSlF
1
possible duplicate of How do you turn a Mongoose document into a plain object?
– Blakes Seven
Jul 21 '15 at 9:56