Trouble with JavaScript collection of document elements
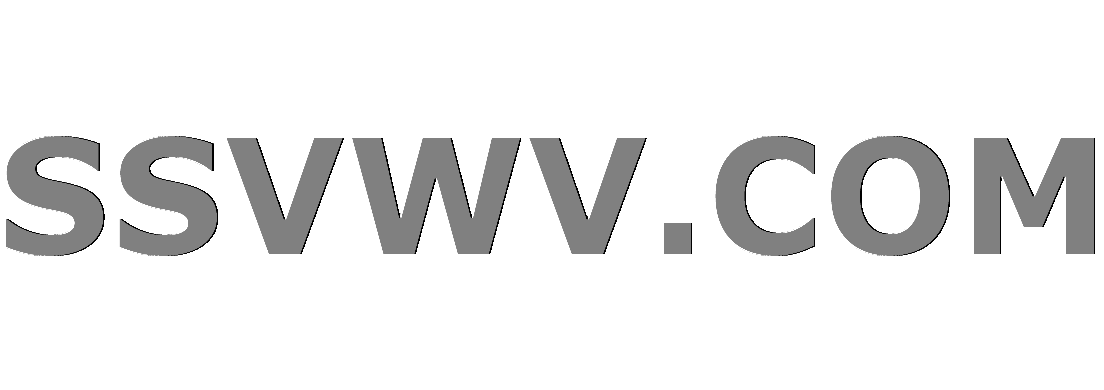
Multi tool use
I'm not sure if I'm on the wrong course altogether, or just missing a minor bit. I have a page that has sections, subsections, and subsubsections. The latter are elements that all share a common formatting:
<select id="SubSubFlood" class='hidden'>
<option></option>
</select>
<select id="SubSubHome" class='hidden'>
<option></option>
</select>
I can't name each one explicitly because they're dynamically generated, but they all start with "SubSub". I'm trying to create code that will change all of the SubSubs to class='hidden', then change the one I want to be visible to class='unhidden'. Here is my attempt:
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (item in SubSub) {
if (item.ID.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
Where am I missing the boat? How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
javascript regex for-loop getelementsbytagname
add a comment |
I'm not sure if I'm on the wrong course altogether, or just missing a minor bit. I have a page that has sections, subsections, and subsubsections. The latter are elements that all share a common formatting:
<select id="SubSubFlood" class='hidden'>
<option></option>
</select>
<select id="SubSubHome" class='hidden'>
<option></option>
</select>
I can't name each one explicitly because they're dynamically generated, but they all start with "SubSub". I'm trying to create code that will change all of the SubSubs to class='hidden', then change the one I want to be visible to class='unhidden'. Here is my attempt:
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (item in SubSub) {
if (item.ID.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
Where am I missing the boat? How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
javascript regex for-loop getelementsbytagname
1
Can't usefor ... in
loop on an HTMLCollection. You'll need to use a traditionalfor
loop. Alternatively, if you usedocument.querySelectorAll('select')
you will get a NodeList and can then useforEach
.
– Randy Casburn
Nov 21 '18 at 15:03
@RandyCasburnfor...in
is for iterating over object properties, not for arrays - for those it isfor ... of
and that is safe on anything that isiterable
(includingNodeList
andHTMLCollection
).
– connexo
Nov 21 '18 at 15:17
item.ID
I assume will always returnundefined
. Maybe you meantitem.id
?
– connexo
Nov 21 '18 at 15:22
add a comment |
I'm not sure if I'm on the wrong course altogether, or just missing a minor bit. I have a page that has sections, subsections, and subsubsections. The latter are elements that all share a common formatting:
<select id="SubSubFlood" class='hidden'>
<option></option>
</select>
<select id="SubSubHome" class='hidden'>
<option></option>
</select>
I can't name each one explicitly because they're dynamically generated, but they all start with "SubSub". I'm trying to create code that will change all of the SubSubs to class='hidden', then change the one I want to be visible to class='unhidden'. Here is my attempt:
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (item in SubSub) {
if (item.ID.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
Where am I missing the boat? How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
javascript regex for-loop getelementsbytagname
I'm not sure if I'm on the wrong course altogether, or just missing a minor bit. I have a page that has sections, subsections, and subsubsections. The latter are elements that all share a common formatting:
<select id="SubSubFlood" class='hidden'>
<option></option>
</select>
<select id="SubSubHome" class='hidden'>
<option></option>
</select>
I can't name each one explicitly because they're dynamically generated, but they all start with "SubSub". I'm trying to create code that will change all of the SubSubs to class='hidden', then change the one I want to be visible to class='unhidden'. Here is my attempt:
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (item in SubSub) {
if (item.ID.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
Where am I missing the boat? How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
javascript regex for-loop getelementsbytagname
javascript regex for-loop getelementsbytagname
asked Nov 21 '18 at 14:56


Bob CooperBob Cooper
163
163
1
Can't usefor ... in
loop on an HTMLCollection. You'll need to use a traditionalfor
loop. Alternatively, if you usedocument.querySelectorAll('select')
you will get a NodeList and can then useforEach
.
– Randy Casburn
Nov 21 '18 at 15:03
@RandyCasburnfor...in
is for iterating over object properties, not for arrays - for those it isfor ... of
and that is safe on anything that isiterable
(includingNodeList
andHTMLCollection
).
– connexo
Nov 21 '18 at 15:17
item.ID
I assume will always returnundefined
. Maybe you meantitem.id
?
– connexo
Nov 21 '18 at 15:22
add a comment |
1
Can't usefor ... in
loop on an HTMLCollection. You'll need to use a traditionalfor
loop. Alternatively, if you usedocument.querySelectorAll('select')
you will get a NodeList and can then useforEach
.
– Randy Casburn
Nov 21 '18 at 15:03
@RandyCasburnfor...in
is for iterating over object properties, not for arrays - for those it isfor ... of
and that is safe on anything that isiterable
(includingNodeList
andHTMLCollection
).
– connexo
Nov 21 '18 at 15:17
item.ID
I assume will always returnundefined
. Maybe you meantitem.id
?
– connexo
Nov 21 '18 at 15:22
1
1
Can't use
for ... in
loop on an HTMLCollection. You'll need to use a traditional for
loop. Alternatively, if you use document.querySelectorAll('select')
you will get a NodeList and can then use forEach
.– Randy Casburn
Nov 21 '18 at 15:03
Can't use
for ... in
loop on an HTMLCollection. You'll need to use a traditional for
loop. Alternatively, if you use document.querySelectorAll('select')
you will get a NodeList and can then use forEach
.– Randy Casburn
Nov 21 '18 at 15:03
@RandyCasburn
for...in
is for iterating over object properties, not for arrays - for those it is for ... of
and that is safe on anything that is iterable
(including NodeList
and HTMLCollection
).– connexo
Nov 21 '18 at 15:17
@RandyCasburn
for...in
is for iterating over object properties, not for arrays - for those it is for ... of
and that is safe on anything that is iterable
(including NodeList
and HTMLCollection
).– connexo
Nov 21 '18 at 15:17
item.ID
I assume will always return undefined
. Maybe you meant item.id
?– connexo
Nov 21 '18 at 15:22
item.ID
I assume will always return undefined
. Maybe you meant item.id
?– connexo
Nov 21 '18 at 15:22
add a comment |
3 Answers
3
active
oldest
votes
SubSub
will contain an array like object, so when you put it in a for each
loop, you'll be enumerating it's properties, not the elements themselves. So item in the loop will not be the first select, it'll be 0
. I suggest changing it to a normal for
loop
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (var i =0; i < SubSub.length; i++) {
if (SubSub[i].id.match(/SubSub.*/)) {
SubSub[i].className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome')
.hidden{
display:none;
}
<select id="SubSubFlood" class='hidden'>
<option>Flood</option>
</select>
<select id="SubSubHome" class='hidden'>
<option>Home</option>
</select>
P.S. is there any reason for having an unhidden
class, can't you just remove the hidden
class?
Exactly what I needed. Tested it out, and it did just what I needed it to.
– Bob Cooper
Nov 21 '18 at 19:49
add a comment |
This replies to the question:
How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
[...document.querySelectorAll('[id^="SubSub"]')].forEach(section => section.className.add('hidden'))
will achieve just that.
So let's break that up:
First of all use an attribute selector that matches all elements that have an
id
value starting withSubSub
:[id^="SubSub"]
.
Query the document for all of these:
document.querySelectorAll('[id^="SubSub"]')
Spread the
NodeList
you get to into anarray
using...
(spread operator):
[...document.querySelectorAll('[id^="SubSub"]')]
Alternatively, you could also use
Array.from(iterable)
:
Array.from(document.querySelectorAll('[id^="SubSub"]'))
so it's cross-browser safe to use
forEach
on the result:
[...document.querySelectorAll('[id^="SubSub"]')].forEach((section) => {})
In that loop, simply add the classname to the
classList
:
section.className.add('hidden')
If Nodelist.forEach not supported in the browser highly unlikely spread operator is
– charlietfl
Nov 21 '18 at 15:05
I guess because it's hard to read and because it has no explanation whatsoever
– Oram
Nov 21 '18 at 15:05
1
This solution just hides all elements by settings their class to "hidden". But the question also says, a single one should get aunhidden
class.
– David
Nov 21 '18 at 15:07
1
Guys, where does the answer mention it is only meant to replace parts of the code?
– David
Nov 21 '18 at 15:11
1
I rather doubt OP is transpiling with babel
– charlietfl
Nov 21 '18 at 15:15
|
show 8 more comments
The problem is that getElementsByTagName
returns HTMLCollection
which "not work" with for-in, instead use
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
console.log(SubSub);
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
console.log(item);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
I also develop a little improvement to connexo solution:
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414757%2ftrouble-with-javascript-collection-of-document-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
SubSub
will contain an array like object, so when you put it in a for each
loop, you'll be enumerating it's properties, not the elements themselves. So item in the loop will not be the first select, it'll be 0
. I suggest changing it to a normal for
loop
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (var i =0; i < SubSub.length; i++) {
if (SubSub[i].id.match(/SubSub.*/)) {
SubSub[i].className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome')
.hidden{
display:none;
}
<select id="SubSubFlood" class='hidden'>
<option>Flood</option>
</select>
<select id="SubSubHome" class='hidden'>
<option>Home</option>
</select>
P.S. is there any reason for having an unhidden
class, can't you just remove the hidden
class?
Exactly what I needed. Tested it out, and it did just what I needed it to.
– Bob Cooper
Nov 21 '18 at 19:49
add a comment |
SubSub
will contain an array like object, so when you put it in a for each
loop, you'll be enumerating it's properties, not the elements themselves. So item in the loop will not be the first select, it'll be 0
. I suggest changing it to a normal for
loop
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (var i =0; i < SubSub.length; i++) {
if (SubSub[i].id.match(/SubSub.*/)) {
SubSub[i].className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome')
.hidden{
display:none;
}
<select id="SubSubFlood" class='hidden'>
<option>Flood</option>
</select>
<select id="SubSubHome" class='hidden'>
<option>Home</option>
</select>
P.S. is there any reason for having an unhidden
class, can't you just remove the hidden
class?
Exactly what I needed. Tested it out, and it did just what I needed it to.
– Bob Cooper
Nov 21 '18 at 19:49
add a comment |
SubSub
will contain an array like object, so when you put it in a for each
loop, you'll be enumerating it's properties, not the elements themselves. So item in the loop will not be the first select, it'll be 0
. I suggest changing it to a normal for
loop
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (var i =0; i < SubSub.length; i++) {
if (SubSub[i].id.match(/SubSub.*/)) {
SubSub[i].className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome')
.hidden{
display:none;
}
<select id="SubSubFlood" class='hidden'>
<option>Flood</option>
</select>
<select id="SubSubHome" class='hidden'>
<option>Home</option>
</select>
P.S. is there any reason for having an unhidden
class, can't you just remove the hidden
class?
SubSub
will contain an array like object, so when you put it in a for each
loop, you'll be enumerating it's properties, not the elements themselves. So item in the loop will not be the first select, it'll be 0
. I suggest changing it to a normal for
loop
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (var i =0; i < SubSub.length; i++) {
if (SubSub[i].id.match(/SubSub.*/)) {
SubSub[i].className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome')
.hidden{
display:none;
}
<select id="SubSubFlood" class='hidden'>
<option>Flood</option>
</select>
<select id="SubSubHome" class='hidden'>
<option>Home</option>
</select>
P.S. is there any reason for having an unhidden
class, can't you just remove the hidden
class?
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (var i =0; i < SubSub.length; i++) {
if (SubSub[i].id.match(/SubSub.*/)) {
SubSub[i].className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome')
.hidden{
display:none;
}
<select id="SubSubFlood" class='hidden'>
<option>Flood</option>
</select>
<select id="SubSubHome" class='hidden'>
<option>Home</option>
</select>
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
var item;
for (var i =0; i < SubSub.length; i++) {
if (SubSub[i].id.match(/SubSub.*/)) {
SubSub[i].className = 'hidden';
}
}
item = document.getElementById(SelectID);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome')
.hidden{
display:none;
}
<select id="SubSubFlood" class='hidden'>
<option>Flood</option>
</select>
<select id="SubSubHome" class='hidden'>
<option>Home</option>
</select>
answered Nov 21 '18 at 15:03


GeorgeGeorge
4,51311832
4,51311832
Exactly what I needed. Tested it out, and it did just what I needed it to.
– Bob Cooper
Nov 21 '18 at 19:49
add a comment |
Exactly what I needed. Tested it out, and it did just what I needed it to.
– Bob Cooper
Nov 21 '18 at 19:49
Exactly what I needed. Tested it out, and it did just what I needed it to.
– Bob Cooper
Nov 21 '18 at 19:49
Exactly what I needed. Tested it out, and it did just what I needed it to.
– Bob Cooper
Nov 21 '18 at 19:49
add a comment |
This replies to the question:
How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
[...document.querySelectorAll('[id^="SubSub"]')].forEach(section => section.className.add('hidden'))
will achieve just that.
So let's break that up:
First of all use an attribute selector that matches all elements that have an
id
value starting withSubSub
:[id^="SubSub"]
.
Query the document for all of these:
document.querySelectorAll('[id^="SubSub"]')
Spread the
NodeList
you get to into anarray
using...
(spread operator):
[...document.querySelectorAll('[id^="SubSub"]')]
Alternatively, you could also use
Array.from(iterable)
:
Array.from(document.querySelectorAll('[id^="SubSub"]'))
so it's cross-browser safe to use
forEach
on the result:
[...document.querySelectorAll('[id^="SubSub"]')].forEach((section) => {})
In that loop, simply add the classname to the
classList
:
section.className.add('hidden')
If Nodelist.forEach not supported in the browser highly unlikely spread operator is
– charlietfl
Nov 21 '18 at 15:05
I guess because it's hard to read and because it has no explanation whatsoever
– Oram
Nov 21 '18 at 15:05
1
This solution just hides all elements by settings their class to "hidden". But the question also says, a single one should get aunhidden
class.
– David
Nov 21 '18 at 15:07
1
Guys, where does the answer mention it is only meant to replace parts of the code?
– David
Nov 21 '18 at 15:11
1
I rather doubt OP is transpiling with babel
– charlietfl
Nov 21 '18 at 15:15
|
show 8 more comments
This replies to the question:
How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
[...document.querySelectorAll('[id^="SubSub"]')].forEach(section => section.className.add('hidden'))
will achieve just that.
So let's break that up:
First of all use an attribute selector that matches all elements that have an
id
value starting withSubSub
:[id^="SubSub"]
.
Query the document for all of these:
document.querySelectorAll('[id^="SubSub"]')
Spread the
NodeList
you get to into anarray
using...
(spread operator):
[...document.querySelectorAll('[id^="SubSub"]')]
Alternatively, you could also use
Array.from(iterable)
:
Array.from(document.querySelectorAll('[id^="SubSub"]'))
so it's cross-browser safe to use
forEach
on the result:
[...document.querySelectorAll('[id^="SubSub"]')].forEach((section) => {})
In that loop, simply add the classname to the
classList
:
section.className.add('hidden')
If Nodelist.forEach not supported in the browser highly unlikely spread operator is
– charlietfl
Nov 21 '18 at 15:05
I guess because it's hard to read and because it has no explanation whatsoever
– Oram
Nov 21 '18 at 15:05
1
This solution just hides all elements by settings their class to "hidden". But the question also says, a single one should get aunhidden
class.
– David
Nov 21 '18 at 15:07
1
Guys, where does the answer mention it is only meant to replace parts of the code?
– David
Nov 21 '18 at 15:11
1
I rather doubt OP is transpiling with babel
– charlietfl
Nov 21 '18 at 15:15
|
show 8 more comments
This replies to the question:
How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
[...document.querySelectorAll('[id^="SubSub"]')].forEach(section => section.className.add('hidden'))
will achieve just that.
So let's break that up:
First of all use an attribute selector that matches all elements that have an
id
value starting withSubSub
:[id^="SubSub"]
.
Query the document for all of these:
document.querySelectorAll('[id^="SubSub"]')
Spread the
NodeList
you get to into anarray
using...
(spread operator):
[...document.querySelectorAll('[id^="SubSub"]')]
Alternatively, you could also use
Array.from(iterable)
:
Array.from(document.querySelectorAll('[id^="SubSub"]'))
so it's cross-browser safe to use
forEach
on the result:
[...document.querySelectorAll('[id^="SubSub"]')].forEach((section) => {})
In that loop, simply add the classname to the
classList
:
section.className.add('hidden')
This replies to the question:
How do I get JavaScript to change every tag with an ID that starts with "SubSub" to class="hidden"?
[...document.querySelectorAll('[id^="SubSub"]')].forEach(section => section.className.add('hidden'))
will achieve just that.
So let's break that up:
First of all use an attribute selector that matches all elements that have an
id
value starting withSubSub
:[id^="SubSub"]
.
Query the document for all of these:
document.querySelectorAll('[id^="SubSub"]')
Spread the
NodeList
you get to into anarray
using...
(spread operator):
[...document.querySelectorAll('[id^="SubSub"]')]
Alternatively, you could also use
Array.from(iterable)
:
Array.from(document.querySelectorAll('[id^="SubSub"]'))
so it's cross-browser safe to use
forEach
on the result:
[...document.querySelectorAll('[id^="SubSub"]')].forEach((section) => {})
In that loop, simply add the classname to the
classList
:
section.className.add('hidden')
edited Nov 21 '18 at 15:24
answered Nov 21 '18 at 15:03


connexoconnexo
22.8k83762
22.8k83762
If Nodelist.forEach not supported in the browser highly unlikely spread operator is
– charlietfl
Nov 21 '18 at 15:05
I guess because it's hard to read and because it has no explanation whatsoever
– Oram
Nov 21 '18 at 15:05
1
This solution just hides all elements by settings their class to "hidden". But the question also says, a single one should get aunhidden
class.
– David
Nov 21 '18 at 15:07
1
Guys, where does the answer mention it is only meant to replace parts of the code?
– David
Nov 21 '18 at 15:11
1
I rather doubt OP is transpiling with babel
– charlietfl
Nov 21 '18 at 15:15
|
show 8 more comments
If Nodelist.forEach not supported in the browser highly unlikely spread operator is
– charlietfl
Nov 21 '18 at 15:05
I guess because it's hard to read and because it has no explanation whatsoever
– Oram
Nov 21 '18 at 15:05
1
This solution just hides all elements by settings their class to "hidden". But the question also says, a single one should get aunhidden
class.
– David
Nov 21 '18 at 15:07
1
Guys, where does the answer mention it is only meant to replace parts of the code?
– David
Nov 21 '18 at 15:11
1
I rather doubt OP is transpiling with babel
– charlietfl
Nov 21 '18 at 15:15
If Nodelist.forEach not supported in the browser highly unlikely spread operator is
– charlietfl
Nov 21 '18 at 15:05
If Nodelist.forEach not supported in the browser highly unlikely spread operator is
– charlietfl
Nov 21 '18 at 15:05
I guess because it's hard to read and because it has no explanation whatsoever
– Oram
Nov 21 '18 at 15:05
I guess because it's hard to read and because it has no explanation whatsoever
– Oram
Nov 21 '18 at 15:05
1
1
This solution just hides all elements by settings their class to "hidden". But the question also says, a single one should get a
unhidden
class.– David
Nov 21 '18 at 15:07
This solution just hides all elements by settings their class to "hidden". But the question also says, a single one should get a
unhidden
class.– David
Nov 21 '18 at 15:07
1
1
Guys, where does the answer mention it is only meant to replace parts of the code?
– David
Nov 21 '18 at 15:11
Guys, where does the answer mention it is only meant to replace parts of the code?
– David
Nov 21 '18 at 15:11
1
1
I rather doubt OP is transpiling with babel
– charlietfl
Nov 21 '18 at 15:15
I rather doubt OP is transpiling with babel
– charlietfl
Nov 21 '18 at 15:15
|
show 8 more comments
The problem is that getElementsByTagName
returns HTMLCollection
which "not work" with for-in, instead use
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
console.log(SubSub);
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
console.log(item);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
I also develop a little improvement to connexo solution:
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
add a comment |
The problem is that getElementsByTagName
returns HTMLCollection
which "not work" with for-in, instead use
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
console.log(SubSub);
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
console.log(item);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
I also develop a little improvement to connexo solution:
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
add a comment |
The problem is that getElementsByTagName
returns HTMLCollection
which "not work" with for-in, instead use
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
console.log(SubSub);
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
console.log(item);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
I also develop a little improvement to connexo solution:
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
The problem is that getElementsByTagName
returns HTMLCollection
which "not work" with for-in, instead use
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
console.log(SubSub);
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
console.log(item);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
I also develop a little improvement to connexo solution:
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
console.log(SubSub);
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
console.log(item);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
function ShowSubSub(SelectID) {
var SubSub = document.getElementsByTagName("Select");
console.log(SubSub);
for (let i =0; i<SubSub.length; i++) {
let item = SubSub[i];
if (item.id.match(/SubSub.*/)) {
item.className = 'hidden';
}
}
item = document.getElementById(SelectID);
console.log(item);
item.className = 'unhidden';
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
function ShowSubSub(SelectID)
{
[...document.querySelectorAll('[id^="SubSub"]')].map(s =>
s.id==SelectID ? s.className=('unhidden') : s.className=('hidden')
)
}
ShowSubSub('SubSubHome');
.hidden {
background: red;
}
.unhidden {
background: blue;
}
<select id="SubSubFlood" >
<option></option>
</select>
<select id="SubSubHome" >
<option></option>
</select>
edited Nov 21 '18 at 15:32
answered Nov 21 '18 at 15:06


Kamil KiełczewskiKamil Kiełczewski
13.2k87196
13.2k87196
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414757%2ftrouble-with-javascript-collection-of-document-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
jM7eW4jOSv sHRDsi137cdtQgECeWMwyrzTBW hvpeDH7e0UMhMTvnqaG y5y9NucYCBmUewn
1
Can't use
for ... in
loop on an HTMLCollection. You'll need to use a traditionalfor
loop. Alternatively, if you usedocument.querySelectorAll('select')
you will get a NodeList and can then useforEach
.– Randy Casburn
Nov 21 '18 at 15:03
@RandyCasburn
for...in
is for iterating over object properties, not for arrays - for those it isfor ... of
and that is safe on anything that isiterable
(includingNodeList
andHTMLCollection
).– connexo
Nov 21 '18 at 15:17
item.ID
I assume will always returnundefined
. Maybe you meantitem.id
?– connexo
Nov 21 '18 at 15:22