How do I log every request and errors in logfile
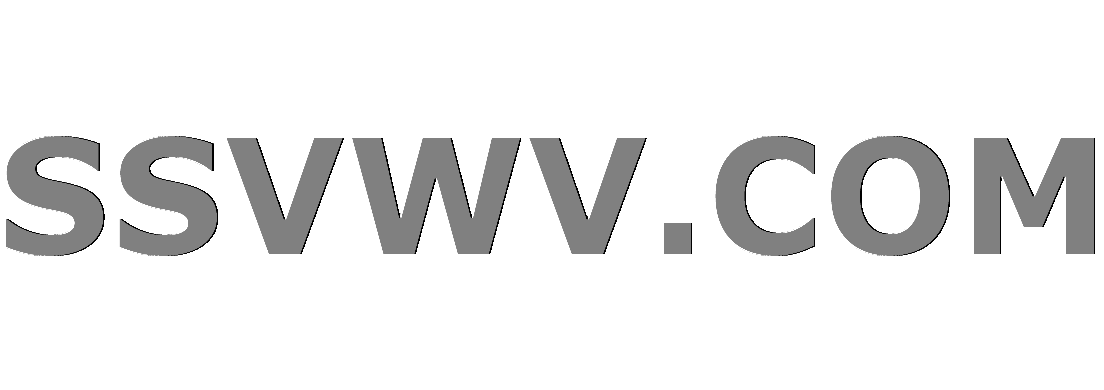
Multi tool use
I have created one middleware to Log every request and errors of API, can any one tell me the best practice to do that?
logging.js
const winston = require('winston');
module.exports = function(req, res, next) {
const logger = winston.createLogger({
levels: winston.config.syslog.levels,
transports: [
new winston.transports.File({
filename: 'Logs/combined.log',
level: 'info'
})
],
exitOnError: false
});
var logmsg = {
'Request IP':req.ip,
'Method':req.method,
'URL':req.originalUrl,
'statusCode':res.statusCode,
'headers':req.headers,
'Time':new Date()
};
process.on('unhandledRejection', (reason, p) => {
logger.error('exception:'+reason);
res.status(200).json({
'statuscode': 200,
'message': 'Validation Error',
'responsedata': 'Unhandled Exception Occured'
});
});
logger.log('info', logmsg);
next();
}
node.js
add a comment |
I have created one middleware to Log every request and errors of API, can any one tell me the best practice to do that?
logging.js
const winston = require('winston');
module.exports = function(req, res, next) {
const logger = winston.createLogger({
levels: winston.config.syslog.levels,
transports: [
new winston.transports.File({
filename: 'Logs/combined.log',
level: 'info'
})
],
exitOnError: false
});
var logmsg = {
'Request IP':req.ip,
'Method':req.method,
'URL':req.originalUrl,
'statusCode':res.statusCode,
'headers':req.headers,
'Time':new Date()
};
process.on('unhandledRejection', (reason, p) => {
logger.error('exception:'+reason);
res.status(200).json({
'statuscode': 200,
'message': 'Validation Error',
'responsedata': 'Unhandled Exception Occured'
});
});
logger.log('info', logmsg);
next();
}
node.js
add a comment |
I have created one middleware to Log every request and errors of API, can any one tell me the best practice to do that?
logging.js
const winston = require('winston');
module.exports = function(req, res, next) {
const logger = winston.createLogger({
levels: winston.config.syslog.levels,
transports: [
new winston.transports.File({
filename: 'Logs/combined.log',
level: 'info'
})
],
exitOnError: false
});
var logmsg = {
'Request IP':req.ip,
'Method':req.method,
'URL':req.originalUrl,
'statusCode':res.statusCode,
'headers':req.headers,
'Time':new Date()
};
process.on('unhandledRejection', (reason, p) => {
logger.error('exception:'+reason);
res.status(200).json({
'statuscode': 200,
'message': 'Validation Error',
'responsedata': 'Unhandled Exception Occured'
});
});
logger.log('info', logmsg);
next();
}
node.js
I have created one middleware to Log every request and errors of API, can any one tell me the best practice to do that?
logging.js
const winston = require('winston');
module.exports = function(req, res, next) {
const logger = winston.createLogger({
levels: winston.config.syslog.levels,
transports: [
new winston.transports.File({
filename: 'Logs/combined.log',
level: 'info'
})
],
exitOnError: false
});
var logmsg = {
'Request IP':req.ip,
'Method':req.method,
'URL':req.originalUrl,
'statusCode':res.statusCode,
'headers':req.headers,
'Time':new Date()
};
process.on('unhandledRejection', (reason, p) => {
logger.error('exception:'+reason);
res.status(200).json({
'statuscode': 200,
'message': 'Validation Error',
'responsedata': 'Unhandled Exception Occured'
});
});
logger.log('info', logmsg);
next();
}
node.js
node.js
edited Nov 21 '18 at 12:33
Sridhar
5,69842937
5,69842937
asked Nov 21 '18 at 10:10


Juned AnsariJuned Ansari
2,21222141
2,21222141
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Assuming you are using express or similar, add the following to where you configure your express:
const logging = require('$path/to/logging')
const app = express.createServer(options)
app.all('*', logging)
This will make the middleware get called for all requests.
You should also initialize winston outside of the middleware call, since it is a one-time operation. So move the winston.createLogger
call outside of the function scope.
As another note, process.on('unhandledRejection', handler)
is a global hook, so you should also not use it inside the middleware function scope. You will end up with 1 listener per request, which are never cleaned up, and which will all fire when an unhandled rejection occurs. (They will fail, since the responses are already sent.)
add a comment |
You can use a middleware, which will log your all request. This middleware should be place at the top of all routes.
app.use((req,res,next)=> {
console.log(`${req.method}- ${req.protocol}:// ${req.get('host')}{req.originalUr}`)
next();
})
You can use try-catch to get the error and transfer all errors to a common function.
try{
// all code
}catch(err){
return sendError(res, err);
}
sendError(res, err){
return res.status(200).json({
'statuscode': 200,
'message': err.message,
'responsedata': 'Unhandled Exception Occured'
});
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53409687%2fhow-do-i-log-every-request-and-errors-in-logfile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Assuming you are using express or similar, add the following to where you configure your express:
const logging = require('$path/to/logging')
const app = express.createServer(options)
app.all('*', logging)
This will make the middleware get called for all requests.
You should also initialize winston outside of the middleware call, since it is a one-time operation. So move the winston.createLogger
call outside of the function scope.
As another note, process.on('unhandledRejection', handler)
is a global hook, so you should also not use it inside the middleware function scope. You will end up with 1 listener per request, which are never cleaned up, and which will all fire when an unhandled rejection occurs. (They will fail, since the responses are already sent.)
add a comment |
Assuming you are using express or similar, add the following to where you configure your express:
const logging = require('$path/to/logging')
const app = express.createServer(options)
app.all('*', logging)
This will make the middleware get called for all requests.
You should also initialize winston outside of the middleware call, since it is a one-time operation. So move the winston.createLogger
call outside of the function scope.
As another note, process.on('unhandledRejection', handler)
is a global hook, so you should also not use it inside the middleware function scope. You will end up with 1 listener per request, which are never cleaned up, and which will all fire when an unhandled rejection occurs. (They will fail, since the responses are already sent.)
add a comment |
Assuming you are using express or similar, add the following to where you configure your express:
const logging = require('$path/to/logging')
const app = express.createServer(options)
app.all('*', logging)
This will make the middleware get called for all requests.
You should also initialize winston outside of the middleware call, since it is a one-time operation. So move the winston.createLogger
call outside of the function scope.
As another note, process.on('unhandledRejection', handler)
is a global hook, so you should also not use it inside the middleware function scope. You will end up with 1 listener per request, which are never cleaned up, and which will all fire when an unhandled rejection occurs. (They will fail, since the responses are already sent.)
Assuming you are using express or similar, add the following to where you configure your express:
const logging = require('$path/to/logging')
const app = express.createServer(options)
app.all('*', logging)
This will make the middleware get called for all requests.
You should also initialize winston outside of the middleware call, since it is a one-time operation. So move the winston.createLogger
call outside of the function scope.
As another note, process.on('unhandledRejection', handler)
is a global hook, so you should also not use it inside the middleware function scope. You will end up with 1 listener per request, which are never cleaned up, and which will all fire when an unhandled rejection occurs. (They will fail, since the responses are already sent.)
answered Nov 21 '18 at 10:42
mtkoponemtkopone
3,44721516
3,44721516
add a comment |
add a comment |
You can use a middleware, which will log your all request. This middleware should be place at the top of all routes.
app.use((req,res,next)=> {
console.log(`${req.method}- ${req.protocol}:// ${req.get('host')}{req.originalUr}`)
next();
})
You can use try-catch to get the error and transfer all errors to a common function.
try{
// all code
}catch(err){
return sendError(res, err);
}
sendError(res, err){
return res.status(200).json({
'statuscode': 200,
'message': err.message,
'responsedata': 'Unhandled Exception Occured'
});
}
add a comment |
You can use a middleware, which will log your all request. This middleware should be place at the top of all routes.
app.use((req,res,next)=> {
console.log(`${req.method}- ${req.protocol}:// ${req.get('host')}{req.originalUr}`)
next();
})
You can use try-catch to get the error and transfer all errors to a common function.
try{
// all code
}catch(err){
return sendError(res, err);
}
sendError(res, err){
return res.status(200).json({
'statuscode': 200,
'message': err.message,
'responsedata': 'Unhandled Exception Occured'
});
}
add a comment |
You can use a middleware, which will log your all request. This middleware should be place at the top of all routes.
app.use((req,res,next)=> {
console.log(`${req.method}- ${req.protocol}:// ${req.get('host')}{req.originalUr}`)
next();
})
You can use try-catch to get the error and transfer all errors to a common function.
try{
// all code
}catch(err){
return sendError(res, err);
}
sendError(res, err){
return res.status(200).json({
'statuscode': 200,
'message': err.message,
'responsedata': 'Unhandled Exception Occured'
});
}
You can use a middleware, which will log your all request. This middleware should be place at the top of all routes.
app.use((req,res,next)=> {
console.log(`${req.method}- ${req.protocol}:// ${req.get('host')}{req.originalUr}`)
next();
})
You can use try-catch to get the error and transfer all errors to a common function.
try{
// all code
}catch(err){
return sendError(res, err);
}
sendError(res, err){
return res.status(200).json({
'statuscode': 200,
'message': err.message,
'responsedata': 'Unhandled Exception Occured'
});
}
edited Nov 21 '18 at 11:20
answered Nov 21 '18 at 10:41
Biplab MalakarBiplab Malakar
42837
42837
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53409687%2fhow-do-i-log-every-request-and-errors-in-logfile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
y8aLAo1XxUg2DNod2LXk4Wk58g,4L40