Pass Parameter to Base Class Constructor while creating Derived class Object
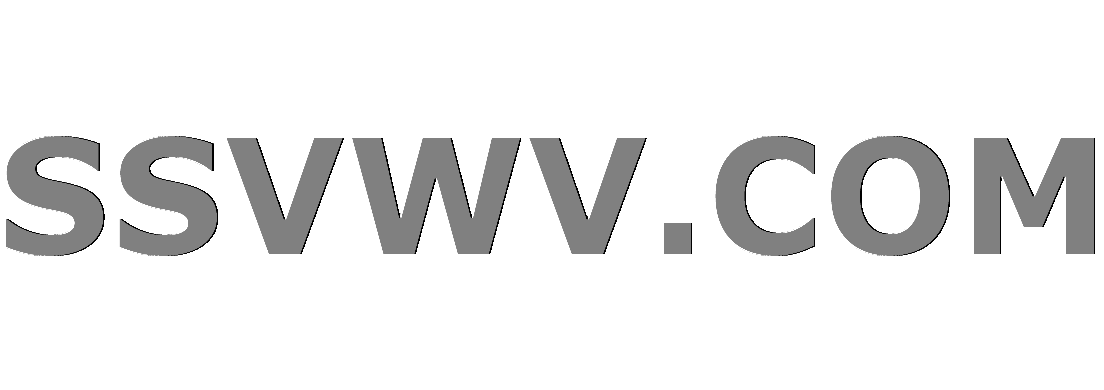
Multi tool use
Consider two classes A
and B
class A
{
public:
A(int);
~A();
};
class B : public A
{
public:
B(int);
~B();
};
int main()
{
A* aobj;
B* bobj = new bobj(5);
}
Now the class B
inherits A
.
I want to create an object of B
. I am aware that creating a derived class object, will also invoke the base class constructor , but that is the default constructor without any parameters.
What i want is that B
to take a parameter (say 5), and pass it on to the constructor of A
.
Please show some code to demonstrate this concept.
c++ oop constructor
add a comment |
Consider two classes A
and B
class A
{
public:
A(int);
~A();
};
class B : public A
{
public:
B(int);
~B();
};
int main()
{
A* aobj;
B* bobj = new bobj(5);
}
Now the class B
inherits A
.
I want to create an object of B
. I am aware that creating a derived class object, will also invoke the base class constructor , but that is the default constructor without any parameters.
What i want is that B
to take a parameter (say 5), and pass it on to the constructor of A
.
Please show some code to demonstrate this concept.
c++ oop constructor
2
B::B(int val) : A(val) {}
– Andrew
May 16 '13 at 11:11
2
I thought you want to make the destructor take arguments, didn't look like a typo to me. And no need from!!
. Down-vote reverted, remark deleted.
– Kiril Kirov
May 16 '13 at 11:21
add a comment |
Consider two classes A
and B
class A
{
public:
A(int);
~A();
};
class B : public A
{
public:
B(int);
~B();
};
int main()
{
A* aobj;
B* bobj = new bobj(5);
}
Now the class B
inherits A
.
I want to create an object of B
. I am aware that creating a derived class object, will also invoke the base class constructor , but that is the default constructor without any parameters.
What i want is that B
to take a parameter (say 5), and pass it on to the constructor of A
.
Please show some code to demonstrate this concept.
c++ oop constructor
Consider two classes A
and B
class A
{
public:
A(int);
~A();
};
class B : public A
{
public:
B(int);
~B();
};
int main()
{
A* aobj;
B* bobj = new bobj(5);
}
Now the class B
inherits A
.
I want to create an object of B
. I am aware that creating a derived class object, will also invoke the base class constructor , but that is the default constructor without any parameters.
What i want is that B
to take a parameter (say 5), and pass it on to the constructor of A
.
Please show some code to demonstrate this concept.
c++ oop constructor
c++ oop constructor
edited May 16 '13 at 15:15


Barath Ravikumar
4,30311336
4,30311336
asked May 16 '13 at 11:09
CodeCrusaderCodeCrusader
1942212
1942212
2
B::B(int val) : A(val) {}
– Andrew
May 16 '13 at 11:11
2
I thought you want to make the destructor take arguments, didn't look like a typo to me. And no need from!!
. Down-vote reverted, remark deleted.
– Kiril Kirov
May 16 '13 at 11:21
add a comment |
2
B::B(int val) : A(val) {}
– Andrew
May 16 '13 at 11:11
2
I thought you want to make the destructor take arguments, didn't look like a typo to me. And no need from!!
. Down-vote reverted, remark deleted.
– Kiril Kirov
May 16 '13 at 11:21
2
2
B::B(int val) : A(val) {}
– Andrew
May 16 '13 at 11:11
B::B(int val) : A(val) {}
– Andrew
May 16 '13 at 11:11
2
2
I thought you want to make the destructor take arguments, didn't look like a typo to me. And no need from
!!
. Down-vote reverted, remark deleted.– Kiril Kirov
May 16 '13 at 11:21
I thought you want to make the destructor take arguments, didn't look like a typo to me. And no need from
!!
. Down-vote reverted, remark deleted.– Kiril Kirov
May 16 '13 at 11:21
add a comment |
4 Answers
4
active
oldest
votes
Use base member initialisation:
class B : public A
{
public:
B(int a) : A(a)
{
}
~B();
};
add a comment |
B::B(int x):A(x)
{
//Body of B constructor
}
add a comment |
If you are using your derived class constructor just to pass arguments to base class then you can also do it in a shorter way in C++11:
class B : public A
{
using A::A;
};
Note that I have not even used "public" access specifier before it. This is not required since inherited constructors are implicitly declared with same access level as in the base class.
For more details, please refer to section Inheriting constructors at: https://en.cppreference.com/w/cpp/language/using_declaration
Also you may refer to https://softwareengineering.stackexchange.com/a/307648 to understand limitations on constructor inheritance.
add a comment |
class A
{ public:
int aval;
A(int a):aval(a){};
~A();
};
class B : public A
{ public:
int bval;
B(int a,int b):bval(a),A(b){};
~B();
};
int main()
{
B *bobj = new bobj(5,6);
//or
A *aobj=new bobj(5,6);
}
In this case you are assigning the values for base class and derived class .
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f16585856%2fpass-parameter-to-base-class-constructor-while-creating-derived-class-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use base member initialisation:
class B : public A
{
public:
B(int a) : A(a)
{
}
~B();
};
add a comment |
Use base member initialisation:
class B : public A
{
public:
B(int a) : A(a)
{
}
~B();
};
add a comment |
Use base member initialisation:
class B : public A
{
public:
B(int a) : A(a)
{
}
~B();
};
Use base member initialisation:
class B : public A
{
public:
B(int a) : A(a)
{
}
~B();
};
edited Feb 14 '14 at 8:54
answered May 16 '13 at 11:14


BathshebaBathsheba
179k27254381
179k27254381
add a comment |
add a comment |
B::B(int x):A(x)
{
//Body of B constructor
}
add a comment |
B::B(int x):A(x)
{
//Body of B constructor
}
add a comment |
B::B(int x):A(x)
{
//Body of B constructor
}
B::B(int x):A(x)
{
//Body of B constructor
}
answered May 16 '13 at 11:11
SomeWittyUsernameSomeWittyUsername
14.9k22967
14.9k22967
add a comment |
add a comment |
If you are using your derived class constructor just to pass arguments to base class then you can also do it in a shorter way in C++11:
class B : public A
{
using A::A;
};
Note that I have not even used "public" access specifier before it. This is not required since inherited constructors are implicitly declared with same access level as in the base class.
For more details, please refer to section Inheriting constructors at: https://en.cppreference.com/w/cpp/language/using_declaration
Also you may refer to https://softwareengineering.stackexchange.com/a/307648 to understand limitations on constructor inheritance.
add a comment |
If you are using your derived class constructor just to pass arguments to base class then you can also do it in a shorter way in C++11:
class B : public A
{
using A::A;
};
Note that I have not even used "public" access specifier before it. This is not required since inherited constructors are implicitly declared with same access level as in the base class.
For more details, please refer to section Inheriting constructors at: https://en.cppreference.com/w/cpp/language/using_declaration
Also you may refer to https://softwareengineering.stackexchange.com/a/307648 to understand limitations on constructor inheritance.
add a comment |
If you are using your derived class constructor just to pass arguments to base class then you can also do it in a shorter way in C++11:
class B : public A
{
using A::A;
};
Note that I have not even used "public" access specifier before it. This is not required since inherited constructors are implicitly declared with same access level as in the base class.
For more details, please refer to section Inheriting constructors at: https://en.cppreference.com/w/cpp/language/using_declaration
Also you may refer to https://softwareengineering.stackexchange.com/a/307648 to understand limitations on constructor inheritance.
If you are using your derived class constructor just to pass arguments to base class then you can also do it in a shorter way in C++11:
class B : public A
{
using A::A;
};
Note that I have not even used "public" access specifier before it. This is not required since inherited constructors are implicitly declared with same access level as in the base class.
For more details, please refer to section Inheriting constructors at: https://en.cppreference.com/w/cpp/language/using_declaration
Also you may refer to https://softwareengineering.stackexchange.com/a/307648 to understand limitations on constructor inheritance.
answered Jul 19 '18 at 15:46
Mandeep SinghMandeep Singh
658
658
add a comment |
add a comment |
class A
{ public:
int aval;
A(int a):aval(a){};
~A();
};
class B : public A
{ public:
int bval;
B(int a,int b):bval(a),A(b){};
~B();
};
int main()
{
B *bobj = new bobj(5,6);
//or
A *aobj=new bobj(5,6);
}
In this case you are assigning the values for base class and derived class .
add a comment |
class A
{ public:
int aval;
A(int a):aval(a){};
~A();
};
class B : public A
{ public:
int bval;
B(int a,int b):bval(a),A(b){};
~B();
};
int main()
{
B *bobj = new bobj(5,6);
//or
A *aobj=new bobj(5,6);
}
In this case you are assigning the values for base class and derived class .
add a comment |
class A
{ public:
int aval;
A(int a):aval(a){};
~A();
};
class B : public A
{ public:
int bval;
B(int a,int b):bval(a),A(b){};
~B();
};
int main()
{
B *bobj = new bobj(5,6);
//or
A *aobj=new bobj(5,6);
}
In this case you are assigning the values for base class and derived class .
class A
{ public:
int aval;
A(int a):aval(a){};
~A();
};
class B : public A
{ public:
int bval;
B(int a,int b):bval(a),A(b){};
~B();
};
int main()
{
B *bobj = new bobj(5,6);
//or
A *aobj=new bobj(5,6);
}
In this case you are assigning the values for base class and derived class .
answered Nov 20 '18 at 11:58
WilsonWilson
112
112
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f16585856%2fpass-parameter-to-base-class-constructor-while-creating-derived-class-object%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fzX6EmdOR5WWTjd4,ikpI58Ny5bOOT fQT M5qZimPHeNOziv9elNHlJhljSPbq7bZkSsudi,9 RfOpR v5DA50 cprusCD
2
B::B(int val) : A(val) {}
– Andrew
May 16 '13 at 11:11
2
I thought you want to make the destructor take arguments, didn't look like a typo to me. And no need from
!!
. Down-vote reverted, remark deleted.– Kiril Kirov
May 16 '13 at 11:21