Avoid typescript casting inside a switch
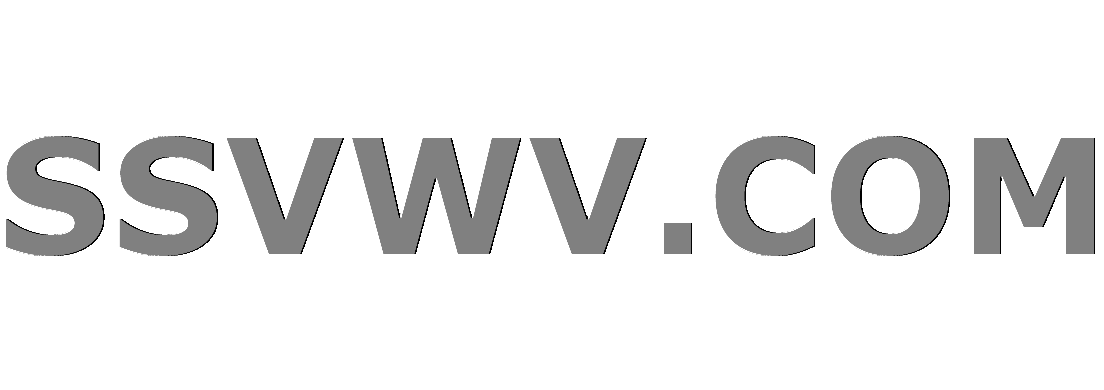
Multi tool use
Consider the following code:
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export interface FooBarAction<T extends FooBarTypes> {
type: T;
data: FooBarTypeMap[T];
}
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction((action as FooBarAction<"FOO">));
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
Now I would like to avoid the casting (action as FooBarAction<"FOO">) as seen in doSomthingBasedOnType, as the very definition if the interface makes this the only possibility inside this switch. Is there something I can change in my code for this to work, or is this simply a bug in TypeScript?
typescript casting typescript-typings typescript-generics
add a comment |
Consider the following code:
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export interface FooBarAction<T extends FooBarTypes> {
type: T;
data: FooBarTypeMap[T];
}
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction((action as FooBarAction<"FOO">));
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
Now I would like to avoid the casting (action as FooBarAction<"FOO">) as seen in doSomthingBasedOnType, as the very definition if the interface makes this the only possibility inside this switch. Is there something I can change in my code for this to work, or is this simply a bug in TypeScript?
typescript casting typescript-typings typescript-generics
add a comment |
Consider the following code:
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export interface FooBarAction<T extends FooBarTypes> {
type: T;
data: FooBarTypeMap[T];
}
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction((action as FooBarAction<"FOO">));
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
Now I would like to avoid the casting (action as FooBarAction<"FOO">) as seen in doSomthingBasedOnType, as the very definition if the interface makes this the only possibility inside this switch. Is there something I can change in my code for this to work, or is this simply a bug in TypeScript?
typescript casting typescript-typings typescript-generics
Consider the following code:
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export interface FooBarAction<T extends FooBarTypes> {
type: T;
data: FooBarTypeMap[T];
}
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction((action as FooBarAction<"FOO">));
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
Now I would like to avoid the casting (action as FooBarAction<"FOO">) as seen in doSomthingBasedOnType, as the very definition if the interface makes this the only possibility inside this switch. Is there something I can change in my code for this to work, or is this simply a bug in TypeScript?
typescript casting typescript-typings typescript-generics
typescript casting typescript-typings typescript-generics
asked Nov 20 '18 at 11:56


MrMamenMrMamen
508
508
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You need to transform FooBarAction
into a discriminated union. At the moment your version of FooBarAction
is not very strict, while type
must be one of "FOO" | "BAR"
and data
must be one of FooBarTypeMap[FooBarTypes] = FooInterface | BarInterface
there is no relation between the two. So this could be allowed:
let o : FooBarAction2<FooBarTypes> = {
type: "BAR",
data: {} as FooInterface
}
The discriminated union version would look like this:
export type FooBarAction = {
type: "FOO";
data: FooInterface;
} | {
type: "BAR";
data: BarInterface;
}
const doSomthingBasedOnType = (action: FooBarAction): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
// We use extract to get a specific type from the union
const FooAction = (action: Extract<FooBarAction, { type: "FOO" }>): void => {
//do something with action.data
};
You can also create a union from a union of types using the distributive behavior of conditional types:
interface FooInterface { foo: number}
interface BarInterface { bar: number}
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export type FooBarAction<T extends FooBarTypes> = T extends any ? {
type: T;
data: FooBarTypeMap[T];
}: never;
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
Seems to be working, but could you explain this part: T extends any ? { type: T; data: FooBarTypeMap[T]; }: never;
– MrMamen
Nov 20 '18 at 13:41
@MrMamen This is called "Distributive conditional types" you can read more about it here: typescriptlang.org/docs/handbook/advanced-types.html
– Titian Cernicova-Dragomir
Nov 20 '18 at 13:43
I've expanded the interfaces to include their own type. Using this I was hoping I could generate a (generic) FooBarAction, but I'm struggeling with typings.interface FooInterface { foo: number, type: "FOO"} interface BarInterface { bar: number, type: "BAR"} const createFooBarAction = (fooBarData: FooBarTypeMap[FooBarTypes]): FooBarAction<FooBarTypes> => ({ type: fooBarData.type, data: fooBarData })
– MrMamen
Nov 22 '18 at 9:31
@MrMamen could you please post it as a new question with the full code ? I can't answer right now maybe someone else will and the comments are a really bad place to put a lot of code :)
– Titian Cernicova-Dragomir
Nov 22 '18 at 9:33
Good point, created one here: stackoverflow.com/questions/53428841/…
– MrMamen
Nov 22 '18 at 10:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53392498%2favoid-typescript-casting-inside-a-switch%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You need to transform FooBarAction
into a discriminated union. At the moment your version of FooBarAction
is not very strict, while type
must be one of "FOO" | "BAR"
and data
must be one of FooBarTypeMap[FooBarTypes] = FooInterface | BarInterface
there is no relation between the two. So this could be allowed:
let o : FooBarAction2<FooBarTypes> = {
type: "BAR",
data: {} as FooInterface
}
The discriminated union version would look like this:
export type FooBarAction = {
type: "FOO";
data: FooInterface;
} | {
type: "BAR";
data: BarInterface;
}
const doSomthingBasedOnType = (action: FooBarAction): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
// We use extract to get a specific type from the union
const FooAction = (action: Extract<FooBarAction, { type: "FOO" }>): void => {
//do something with action.data
};
You can also create a union from a union of types using the distributive behavior of conditional types:
interface FooInterface { foo: number}
interface BarInterface { bar: number}
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export type FooBarAction<T extends FooBarTypes> = T extends any ? {
type: T;
data: FooBarTypeMap[T];
}: never;
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
Seems to be working, but could you explain this part: T extends any ? { type: T; data: FooBarTypeMap[T]; }: never;
– MrMamen
Nov 20 '18 at 13:41
@MrMamen This is called "Distributive conditional types" you can read more about it here: typescriptlang.org/docs/handbook/advanced-types.html
– Titian Cernicova-Dragomir
Nov 20 '18 at 13:43
I've expanded the interfaces to include their own type. Using this I was hoping I could generate a (generic) FooBarAction, but I'm struggeling with typings.interface FooInterface { foo: number, type: "FOO"} interface BarInterface { bar: number, type: "BAR"} const createFooBarAction = (fooBarData: FooBarTypeMap[FooBarTypes]): FooBarAction<FooBarTypes> => ({ type: fooBarData.type, data: fooBarData })
– MrMamen
Nov 22 '18 at 9:31
@MrMamen could you please post it as a new question with the full code ? I can't answer right now maybe someone else will and the comments are a really bad place to put a lot of code :)
– Titian Cernicova-Dragomir
Nov 22 '18 at 9:33
Good point, created one here: stackoverflow.com/questions/53428841/…
– MrMamen
Nov 22 '18 at 10:28
add a comment |
You need to transform FooBarAction
into a discriminated union. At the moment your version of FooBarAction
is not very strict, while type
must be one of "FOO" | "BAR"
and data
must be one of FooBarTypeMap[FooBarTypes] = FooInterface | BarInterface
there is no relation between the two. So this could be allowed:
let o : FooBarAction2<FooBarTypes> = {
type: "BAR",
data: {} as FooInterface
}
The discriminated union version would look like this:
export type FooBarAction = {
type: "FOO";
data: FooInterface;
} | {
type: "BAR";
data: BarInterface;
}
const doSomthingBasedOnType = (action: FooBarAction): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
// We use extract to get a specific type from the union
const FooAction = (action: Extract<FooBarAction, { type: "FOO" }>): void => {
//do something with action.data
};
You can also create a union from a union of types using the distributive behavior of conditional types:
interface FooInterface { foo: number}
interface BarInterface { bar: number}
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export type FooBarAction<T extends FooBarTypes> = T extends any ? {
type: T;
data: FooBarTypeMap[T];
}: never;
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
Seems to be working, but could you explain this part: T extends any ? { type: T; data: FooBarTypeMap[T]; }: never;
– MrMamen
Nov 20 '18 at 13:41
@MrMamen This is called "Distributive conditional types" you can read more about it here: typescriptlang.org/docs/handbook/advanced-types.html
– Titian Cernicova-Dragomir
Nov 20 '18 at 13:43
I've expanded the interfaces to include their own type. Using this I was hoping I could generate a (generic) FooBarAction, but I'm struggeling with typings.interface FooInterface { foo: number, type: "FOO"} interface BarInterface { bar: number, type: "BAR"} const createFooBarAction = (fooBarData: FooBarTypeMap[FooBarTypes]): FooBarAction<FooBarTypes> => ({ type: fooBarData.type, data: fooBarData })
– MrMamen
Nov 22 '18 at 9:31
@MrMamen could you please post it as a new question with the full code ? I can't answer right now maybe someone else will and the comments are a really bad place to put a lot of code :)
– Titian Cernicova-Dragomir
Nov 22 '18 at 9:33
Good point, created one here: stackoverflow.com/questions/53428841/…
– MrMamen
Nov 22 '18 at 10:28
add a comment |
You need to transform FooBarAction
into a discriminated union. At the moment your version of FooBarAction
is not very strict, while type
must be one of "FOO" | "BAR"
and data
must be one of FooBarTypeMap[FooBarTypes] = FooInterface | BarInterface
there is no relation between the two. So this could be allowed:
let o : FooBarAction2<FooBarTypes> = {
type: "BAR",
data: {} as FooInterface
}
The discriminated union version would look like this:
export type FooBarAction = {
type: "FOO";
data: FooInterface;
} | {
type: "BAR";
data: BarInterface;
}
const doSomthingBasedOnType = (action: FooBarAction): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
// We use extract to get a specific type from the union
const FooAction = (action: Extract<FooBarAction, { type: "FOO" }>): void => {
//do something with action.data
};
You can also create a union from a union of types using the distributive behavior of conditional types:
interface FooInterface { foo: number}
interface BarInterface { bar: number}
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export type FooBarAction<T extends FooBarTypes> = T extends any ? {
type: T;
data: FooBarTypeMap[T];
}: never;
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
You need to transform FooBarAction
into a discriminated union. At the moment your version of FooBarAction
is not very strict, while type
must be one of "FOO" | "BAR"
and data
must be one of FooBarTypeMap[FooBarTypes] = FooInterface | BarInterface
there is no relation between the two. So this could be allowed:
let o : FooBarAction2<FooBarTypes> = {
type: "BAR",
data: {} as FooInterface
}
The discriminated union version would look like this:
export type FooBarAction = {
type: "FOO";
data: FooInterface;
} | {
type: "BAR";
data: BarInterface;
}
const doSomthingBasedOnType = (action: FooBarAction): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
// We use extract to get a specific type from the union
const FooAction = (action: Extract<FooBarAction, { type: "FOO" }>): void => {
//do something with action.data
};
You can also create a union from a union of types using the distributive behavior of conditional types:
interface FooInterface { foo: number}
interface BarInterface { bar: number}
interface FooBarTypeMap {
FOO: FooInterface;
BAR: BarInterface;
}
type FooBarTypes = "FOO" | "BAR";
export type FooBarAction<T extends FooBarTypes> = T extends any ? {
type: T;
data: FooBarTypeMap[T];
}: never;
const doSomthingBasedOnType = (action: FooBarAction<FooBarTypes>): void => {
switch (action.type) {
case "FOO":
FooAction(action);
}
};
const FooAction = (action: FooBarAction<"FOO">): void => {
//do something with action.data
};
edited Nov 20 '18 at 12:11
answered Nov 20 '18 at 12:04


Titian Cernicova-DragomirTitian Cernicova-Dragomir
65.6k34361
65.6k34361
Seems to be working, but could you explain this part: T extends any ? { type: T; data: FooBarTypeMap[T]; }: never;
– MrMamen
Nov 20 '18 at 13:41
@MrMamen This is called "Distributive conditional types" you can read more about it here: typescriptlang.org/docs/handbook/advanced-types.html
– Titian Cernicova-Dragomir
Nov 20 '18 at 13:43
I've expanded the interfaces to include their own type. Using this I was hoping I could generate a (generic) FooBarAction, but I'm struggeling with typings.interface FooInterface { foo: number, type: "FOO"} interface BarInterface { bar: number, type: "BAR"} const createFooBarAction = (fooBarData: FooBarTypeMap[FooBarTypes]): FooBarAction<FooBarTypes> => ({ type: fooBarData.type, data: fooBarData })
– MrMamen
Nov 22 '18 at 9:31
@MrMamen could you please post it as a new question with the full code ? I can't answer right now maybe someone else will and the comments are a really bad place to put a lot of code :)
– Titian Cernicova-Dragomir
Nov 22 '18 at 9:33
Good point, created one here: stackoverflow.com/questions/53428841/…
– MrMamen
Nov 22 '18 at 10:28
add a comment |
Seems to be working, but could you explain this part: T extends any ? { type: T; data: FooBarTypeMap[T]; }: never;
– MrMamen
Nov 20 '18 at 13:41
@MrMamen This is called "Distributive conditional types" you can read more about it here: typescriptlang.org/docs/handbook/advanced-types.html
– Titian Cernicova-Dragomir
Nov 20 '18 at 13:43
I've expanded the interfaces to include their own type. Using this I was hoping I could generate a (generic) FooBarAction, but I'm struggeling with typings.interface FooInterface { foo: number, type: "FOO"} interface BarInterface { bar: number, type: "BAR"} const createFooBarAction = (fooBarData: FooBarTypeMap[FooBarTypes]): FooBarAction<FooBarTypes> => ({ type: fooBarData.type, data: fooBarData })
– MrMamen
Nov 22 '18 at 9:31
@MrMamen could you please post it as a new question with the full code ? I can't answer right now maybe someone else will and the comments are a really bad place to put a lot of code :)
– Titian Cernicova-Dragomir
Nov 22 '18 at 9:33
Good point, created one here: stackoverflow.com/questions/53428841/…
– MrMamen
Nov 22 '18 at 10:28
Seems to be working, but could you explain this part: T extends any ? { type: T; data: FooBarTypeMap[T]; }: never;
– MrMamen
Nov 20 '18 at 13:41
Seems to be working, but could you explain this part: T extends any ? { type: T; data: FooBarTypeMap[T]; }: never;
– MrMamen
Nov 20 '18 at 13:41
@MrMamen This is called "Distributive conditional types" you can read more about it here: typescriptlang.org/docs/handbook/advanced-types.html
– Titian Cernicova-Dragomir
Nov 20 '18 at 13:43
@MrMamen This is called "Distributive conditional types" you can read more about it here: typescriptlang.org/docs/handbook/advanced-types.html
– Titian Cernicova-Dragomir
Nov 20 '18 at 13:43
I've expanded the interfaces to include their own type. Using this I was hoping I could generate a (generic) FooBarAction, but I'm struggeling with typings.
interface FooInterface { foo: number, type: "FOO"} interface BarInterface { bar: number, type: "BAR"} const createFooBarAction = (fooBarData: FooBarTypeMap[FooBarTypes]): FooBarAction<FooBarTypes> => ({ type: fooBarData.type, data: fooBarData })
– MrMamen
Nov 22 '18 at 9:31
I've expanded the interfaces to include their own type. Using this I was hoping I could generate a (generic) FooBarAction, but I'm struggeling with typings.
interface FooInterface { foo: number, type: "FOO"} interface BarInterface { bar: number, type: "BAR"} const createFooBarAction = (fooBarData: FooBarTypeMap[FooBarTypes]): FooBarAction<FooBarTypes> => ({ type: fooBarData.type, data: fooBarData })
– MrMamen
Nov 22 '18 at 9:31
@MrMamen could you please post it as a new question with the full code ? I can't answer right now maybe someone else will and the comments are a really bad place to put a lot of code :)
– Titian Cernicova-Dragomir
Nov 22 '18 at 9:33
@MrMamen could you please post it as a new question with the full code ? I can't answer right now maybe someone else will and the comments are a really bad place to put a lot of code :)
– Titian Cernicova-Dragomir
Nov 22 '18 at 9:33
Good point, created one here: stackoverflow.com/questions/53428841/…
– MrMamen
Nov 22 '18 at 10:28
Good point, created one here: stackoverflow.com/questions/53428841/…
– MrMamen
Nov 22 '18 at 10:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53392498%2favoid-typescript-casting-inside-a-switch%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9aLpx,BrrFbDHT8tzWE6mOfvJRAH oOfrbm5Re KZ gtmAka d3RQhhiKx,4S7Q56R hqR45Yen