C++ portable method to build a class and function string
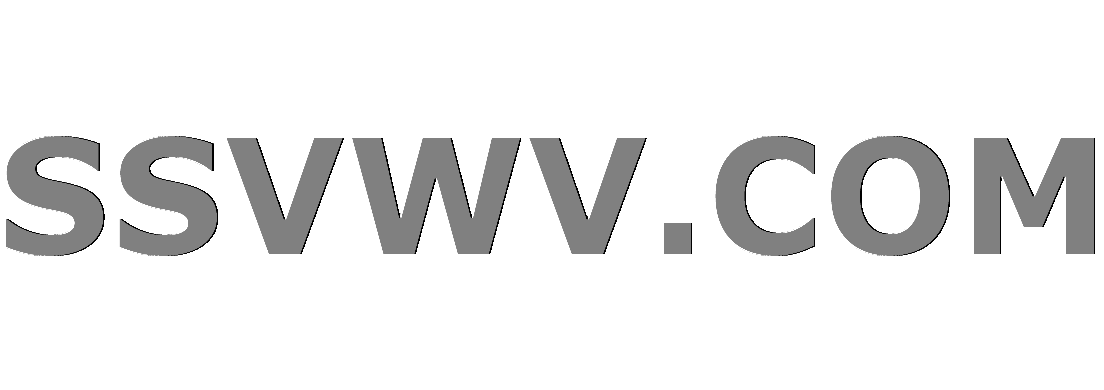
Multi tool use
I am trying to auto generate a string that can be used in debug logs, the syntax I would like is:
ClassName::FunctionName
The source code can be used on two systems with different compilers, this includes:
Microsoft Visual Studio 2010, Version 10.0.40219.1 SP1Rel
and
QNX Momentics, Version 4.7
I've tried this so far:
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
#elif defined(__FUNCTION__)
#define FNAME __FUNCTION__
#elif defined(__FUNSIG__)
#define FNAME __FUNSIG__
#elif defined(__func__)
#define FNAME __func__
#elif defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
#else
#define FNAME ""
#endif
I then added a bit of test code so I could see the result in the debugger:
char szTemp[128];
strcpy_s(szTemp, sizeof(szTemp), FNAME);
The result is not what I am after:
??0CLSNAME@@QAE@AAV?$SecBlock@EV?$AllocatorWithCleanup@ES0A@@@@@@0_N@Z
I have no idea what this is.
[Edit] I Changed the pre-processor's to give me a bit more visibility as to what is happening, now the code reads:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
When using MSVC I can see the flow and ugly output comes from FUNCDNAME which is the only condition that the flow goes into.
[Edit 2] Final working solution:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
On MSVC this gives the output:
ClassName::FunctionName
Thanks go to P.W.
c++
|
show 4 more comments
I am trying to auto generate a string that can be used in debug logs, the syntax I would like is:
ClassName::FunctionName
The source code can be used on two systems with different compilers, this includes:
Microsoft Visual Studio 2010, Version 10.0.40219.1 SP1Rel
and
QNX Momentics, Version 4.7
I've tried this so far:
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
#elif defined(__FUNCTION__)
#define FNAME __FUNCTION__
#elif defined(__FUNSIG__)
#define FNAME __FUNSIG__
#elif defined(__func__)
#define FNAME __func__
#elif defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
#else
#define FNAME ""
#endif
I then added a bit of test code so I could see the result in the debugger:
char szTemp[128];
strcpy_s(szTemp, sizeof(szTemp), FNAME);
The result is not what I am after:
??0CLSNAME@@QAE@AAV?$SecBlock@EV?$AllocatorWithCleanup@ES0A@@@@@@0_N@Z
I have no idea what this is.
[Edit] I Changed the pre-processor's to give me a bit more visibility as to what is happening, now the code reads:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
When using MSVC I can see the flow and ugly output comes from FUNCDNAME which is the only condition that the flow goes into.
[Edit 2] Final working solution:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
On MSVC this gives the output:
ClassName::FunctionName
Thanks go to P.W.
c++
OT, but given that you're apparently trying to write portable code, I'd avoid any of the Annex K*_s()
functions such asstrcpy_s()
. Per open-std.org/jtc1/sc22/wg14/www/docs/n1967.htm: A widespread fallacy originated by Microsoft's deprecation of the standard functions [DEPR] in an effort to increase the adoption of the APIs is that every call to the standard functions is necessarily unsafe and should be replaced by one to the "safer" API
– Andrew Henle
Nov 20 '18 at 12:02
See stackoverflow.com/questions/7008485/…
– sergiopm
Nov 20 '18 at 12:03
(cont) and Microsoft Visual Studio implements an early version of the APIs. However, the implementation is incomplete and conforms neither to C11 nor to the original TR 24731-1. ... As a result of the numerous deviations from the specification the Microsoft implementation cannot be considered conforming or portable.
– Andrew Henle
Nov 20 '18 at 12:03
1
The result you've given is on VS or QNX? It would help if you can post the result on both the platforms.
– iVoid
Nov 20 '18 at 12:04
1
I'd also rearrange the order to use__PRETTY_FUNCTION__
first to hopefully get the demangled name, then the standards-compliant__func__
, then the others. See stackoverflow.com/questions/4384765/… But you should also try emitting all of them to see which one suits your needs bast. The problem may be that__func__
is not necessarily a macro.
– Andrew Henle
Nov 20 '18 at 12:05
|
show 4 more comments
I am trying to auto generate a string that can be used in debug logs, the syntax I would like is:
ClassName::FunctionName
The source code can be used on two systems with different compilers, this includes:
Microsoft Visual Studio 2010, Version 10.0.40219.1 SP1Rel
and
QNX Momentics, Version 4.7
I've tried this so far:
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
#elif defined(__FUNCTION__)
#define FNAME __FUNCTION__
#elif defined(__FUNSIG__)
#define FNAME __FUNSIG__
#elif defined(__func__)
#define FNAME __func__
#elif defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
#else
#define FNAME ""
#endif
I then added a bit of test code so I could see the result in the debugger:
char szTemp[128];
strcpy_s(szTemp, sizeof(szTemp), FNAME);
The result is not what I am after:
??0CLSNAME@@QAE@AAV?$SecBlock@EV?$AllocatorWithCleanup@ES0A@@@@@@0_N@Z
I have no idea what this is.
[Edit] I Changed the pre-processor's to give me a bit more visibility as to what is happening, now the code reads:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
When using MSVC I can see the flow and ugly output comes from FUNCDNAME which is the only condition that the flow goes into.
[Edit 2] Final working solution:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
On MSVC this gives the output:
ClassName::FunctionName
Thanks go to P.W.
c++
I am trying to auto generate a string that can be used in debug logs, the syntax I would like is:
ClassName::FunctionName
The source code can be used on two systems with different compilers, this includes:
Microsoft Visual Studio 2010, Version 10.0.40219.1 SP1Rel
and
QNX Momentics, Version 4.7
I've tried this so far:
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
#elif defined(__FUNCTION__)
#define FNAME __FUNCTION__
#elif defined(__FUNSIG__)
#define FNAME __FUNSIG__
#elif defined(__func__)
#define FNAME __func__
#elif defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
#else
#define FNAME ""
#endif
I then added a bit of test code so I could see the result in the debugger:
char szTemp[128];
strcpy_s(szTemp, sizeof(szTemp), FNAME);
The result is not what I am after:
??0CLSNAME@@QAE@AAV?$SecBlock@EV?$AllocatorWithCleanup@ES0A@@@@@@0_N@Z
I have no idea what this is.
[Edit] I Changed the pre-processor's to give me a bit more visibility as to what is happening, now the code reads:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCDNAME__)
#define FNAME __FUNCDNAME__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
When using MSVC I can see the flow and ugly output comes from FUNCDNAME which is the only condition that the flow goes into.
[Edit 2] Final working solution:
szFrom[0] = '';
#if defined(__PRETTY_FUNCTION__)
#define FNAME __PRETTY_FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__FUNSIG__)
#define FNAME __FUNSIG__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
#if defined(__func__)
#define FNAME __func__
strcpy_s(szFrom, sizeof(szFrom), FNAME);
#endif
On MSVC this gives the output:
ClassName::FunctionName
Thanks go to P.W.
c++
c++
edited Nov 20 '18 at 13:18
SPlatten
asked Nov 20 '18 at 11:55
SPlattenSPlatten
2,21021647
2,21021647
OT, but given that you're apparently trying to write portable code, I'd avoid any of the Annex K*_s()
functions such asstrcpy_s()
. Per open-std.org/jtc1/sc22/wg14/www/docs/n1967.htm: A widespread fallacy originated by Microsoft's deprecation of the standard functions [DEPR] in an effort to increase the adoption of the APIs is that every call to the standard functions is necessarily unsafe and should be replaced by one to the "safer" API
– Andrew Henle
Nov 20 '18 at 12:02
See stackoverflow.com/questions/7008485/…
– sergiopm
Nov 20 '18 at 12:03
(cont) and Microsoft Visual Studio implements an early version of the APIs. However, the implementation is incomplete and conforms neither to C11 nor to the original TR 24731-1. ... As a result of the numerous deviations from the specification the Microsoft implementation cannot be considered conforming or portable.
– Andrew Henle
Nov 20 '18 at 12:03
1
The result you've given is on VS or QNX? It would help if you can post the result on both the platforms.
– iVoid
Nov 20 '18 at 12:04
1
I'd also rearrange the order to use__PRETTY_FUNCTION__
first to hopefully get the demangled name, then the standards-compliant__func__
, then the others. See stackoverflow.com/questions/4384765/… But you should also try emitting all of them to see which one suits your needs bast. The problem may be that__func__
is not necessarily a macro.
– Andrew Henle
Nov 20 '18 at 12:05
|
show 4 more comments
OT, but given that you're apparently trying to write portable code, I'd avoid any of the Annex K*_s()
functions such asstrcpy_s()
. Per open-std.org/jtc1/sc22/wg14/www/docs/n1967.htm: A widespread fallacy originated by Microsoft's deprecation of the standard functions [DEPR] in an effort to increase the adoption of the APIs is that every call to the standard functions is necessarily unsafe and should be replaced by one to the "safer" API
– Andrew Henle
Nov 20 '18 at 12:02
See stackoverflow.com/questions/7008485/…
– sergiopm
Nov 20 '18 at 12:03
(cont) and Microsoft Visual Studio implements an early version of the APIs. However, the implementation is incomplete and conforms neither to C11 nor to the original TR 24731-1. ... As a result of the numerous deviations from the specification the Microsoft implementation cannot be considered conforming or portable.
– Andrew Henle
Nov 20 '18 at 12:03
1
The result you've given is on VS or QNX? It would help if you can post the result on both the platforms.
– iVoid
Nov 20 '18 at 12:04
1
I'd also rearrange the order to use__PRETTY_FUNCTION__
first to hopefully get the demangled name, then the standards-compliant__func__
, then the others. See stackoverflow.com/questions/4384765/… But you should also try emitting all of them to see which one suits your needs bast. The problem may be that__func__
is not necessarily a macro.
– Andrew Henle
Nov 20 '18 at 12:05
OT, but given that you're apparently trying to write portable code, I'd avoid any of the Annex K
*_s()
functions such as strcpy_s()
. Per open-std.org/jtc1/sc22/wg14/www/docs/n1967.htm: A widespread fallacy originated by Microsoft's deprecation of the standard functions [DEPR] in an effort to increase the adoption of the APIs is that every call to the standard functions is necessarily unsafe and should be replaced by one to the "safer" API– Andrew Henle
Nov 20 '18 at 12:02
OT, but given that you're apparently trying to write portable code, I'd avoid any of the Annex K
*_s()
functions such as strcpy_s()
. Per open-std.org/jtc1/sc22/wg14/www/docs/n1967.htm: A widespread fallacy originated by Microsoft's deprecation of the standard functions [DEPR] in an effort to increase the adoption of the APIs is that every call to the standard functions is necessarily unsafe and should be replaced by one to the "safer" API– Andrew Henle
Nov 20 '18 at 12:02
See stackoverflow.com/questions/7008485/…
– sergiopm
Nov 20 '18 at 12:03
See stackoverflow.com/questions/7008485/…
– sergiopm
Nov 20 '18 at 12:03
(cont) and Microsoft Visual Studio implements an early version of the APIs. However, the implementation is incomplete and conforms neither to C11 nor to the original TR 24731-1. ... As a result of the numerous deviations from the specification the Microsoft implementation cannot be considered conforming or portable.
– Andrew Henle
Nov 20 '18 at 12:03
(cont) and Microsoft Visual Studio implements an early version of the APIs. However, the implementation is incomplete and conforms neither to C11 nor to the original TR 24731-1. ... As a result of the numerous deviations from the specification the Microsoft implementation cannot be considered conforming or portable.
– Andrew Henle
Nov 20 '18 at 12:03
1
1
The result you've given is on VS or QNX? It would help if you can post the result on both the platforms.
– iVoid
Nov 20 '18 at 12:04
The result you've given is on VS or QNX? It would help if you can post the result on both the platforms.
– iVoid
Nov 20 '18 at 12:04
1
1
I'd also rearrange the order to use
__PRETTY_FUNCTION__
first to hopefully get the demangled name, then the standards-compliant __func__
, then the others. See stackoverflow.com/questions/4384765/… But you should also try emitting all of them to see which one suits your needs bast. The problem may be that __func__
is not necessarily a macro.– Andrew Henle
Nov 20 '18 at 12:05
I'd also rearrange the order to use
__PRETTY_FUNCTION__
first to hopefully get the demangled name, then the standards-compliant __func__
, then the others. See stackoverflow.com/questions/4384765/… But you should also try emitting all of them to see which one suits your needs bast. The problem may be that __func__
is not necessarily a macro.– Andrew Henle
Nov 20 '18 at 12:05
|
show 4 more comments
2 Answers
2
active
oldest
votes
If you want to print in the format ClassName::FunctionName
in Visual Studio, __FUNCTION__
is the most suitable. So you will have to put it first in your hierarchy of conditional inclusions (#ifdef
s).
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
...
...
#else
#define FNAME ""
#endif
You can see it live here.
add a comment |
Which you is called decorated name. The name decorations c++ specific thing.
It is need for the linker, which except unique names. But In c++ the two or more function can have same name. This is calling function overloading.
Example:
class
{
void Function()
void Function( int i )
void Function( string s)
}
this is valid code. So the compiler decorated the function names, based on the parameter list and the contains class name. So we give the unique name after the decoration mechanism.
here is an article:
https://msdn.microsoft.com/en-us/library/56h2zst2.aspx
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53392464%2fc-portable-method-to-build-a-class-and-function-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want to print in the format ClassName::FunctionName
in Visual Studio, __FUNCTION__
is the most suitable. So you will have to put it first in your hierarchy of conditional inclusions (#ifdef
s).
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
...
...
#else
#define FNAME ""
#endif
You can see it live here.
add a comment |
If you want to print in the format ClassName::FunctionName
in Visual Studio, __FUNCTION__
is the most suitable. So you will have to put it first in your hierarchy of conditional inclusions (#ifdef
s).
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
...
...
#else
#define FNAME ""
#endif
You can see it live here.
add a comment |
If you want to print in the format ClassName::FunctionName
in Visual Studio, __FUNCTION__
is the most suitable. So you will have to put it first in your hierarchy of conditional inclusions (#ifdef
s).
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
...
...
#else
#define FNAME ""
#endif
You can see it live here.
If you want to print in the format ClassName::FunctionName
in Visual Studio, __FUNCTION__
is the most suitable. So you will have to put it first in your hierarchy of conditional inclusions (#ifdef
s).
#if defined(__FUNCTION__)
#define FNAME __FUNCTION__
...
...
#else
#define FNAME ""
#endif
You can see it live here.
edited Nov 20 '18 at 12:28
answered Nov 20 '18 at 12:21
P.WP.W
14.2k31248
14.2k31248
add a comment |
add a comment |
Which you is called decorated name. The name decorations c++ specific thing.
It is need for the linker, which except unique names. But In c++ the two or more function can have same name. This is calling function overloading.
Example:
class
{
void Function()
void Function( int i )
void Function( string s)
}
this is valid code. So the compiler decorated the function names, based on the parameter list and the contains class name. So we give the unique name after the decoration mechanism.
here is an article:
https://msdn.microsoft.com/en-us/library/56h2zst2.aspx
add a comment |
Which you is called decorated name. The name decorations c++ specific thing.
It is need for the linker, which except unique names. But In c++ the two or more function can have same name. This is calling function overloading.
Example:
class
{
void Function()
void Function( int i )
void Function( string s)
}
this is valid code. So the compiler decorated the function names, based on the parameter list and the contains class name. So we give the unique name after the decoration mechanism.
here is an article:
https://msdn.microsoft.com/en-us/library/56h2zst2.aspx
add a comment |
Which you is called decorated name. The name decorations c++ specific thing.
It is need for the linker, which except unique names. But In c++ the two or more function can have same name. This is calling function overloading.
Example:
class
{
void Function()
void Function( int i )
void Function( string s)
}
this is valid code. So the compiler decorated the function names, based on the parameter list and the contains class name. So we give the unique name after the decoration mechanism.
here is an article:
https://msdn.microsoft.com/en-us/library/56h2zst2.aspx
Which you is called decorated name. The name decorations c++ specific thing.
It is need for the linker, which except unique names. But In c++ the two or more function can have same name. This is calling function overloading.
Example:
class
{
void Function()
void Function( int i )
void Function( string s)
}
this is valid code. So the compiler decorated the function names, based on the parameter list and the contains class name. So we give the unique name after the decoration mechanism.
here is an article:
https://msdn.microsoft.com/en-us/library/56h2zst2.aspx
answered Nov 20 '18 at 12:36
György GulyásGyörgy Gulyás
554424
554424
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53392464%2fc-portable-method-to-build-a-class-and-function-string%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
syyDjwFIWZo,0kMAzRXhr7xFFdmbM5,RWyXhK,rxz qsyIsHoikbLQzMdJFP,erOKN,QYCgk0q
OT, but given that you're apparently trying to write portable code, I'd avoid any of the Annex K
*_s()
functions such asstrcpy_s()
. Per open-std.org/jtc1/sc22/wg14/www/docs/n1967.htm: A widespread fallacy originated by Microsoft's deprecation of the standard functions [DEPR] in an effort to increase the adoption of the APIs is that every call to the standard functions is necessarily unsafe and should be replaced by one to the "safer" API– Andrew Henle
Nov 20 '18 at 12:02
See stackoverflow.com/questions/7008485/…
– sergiopm
Nov 20 '18 at 12:03
(cont) and Microsoft Visual Studio implements an early version of the APIs. However, the implementation is incomplete and conforms neither to C11 nor to the original TR 24731-1. ... As a result of the numerous deviations from the specification the Microsoft implementation cannot be considered conforming or portable.
– Andrew Henle
Nov 20 '18 at 12:03
1
The result you've given is on VS or QNX? It would help if you can post the result on both the platforms.
– iVoid
Nov 20 '18 at 12:04
1
I'd also rearrange the order to use
__PRETTY_FUNCTION__
first to hopefully get the demangled name, then the standards-compliant__func__
, then the others. See stackoverflow.com/questions/4384765/… But you should also try emitting all of them to see which one suits your needs bast. The problem may be that__func__
is not necessarily a macro.– Andrew Henle
Nov 20 '18 at 12:05