Spring Boot shutdown hook
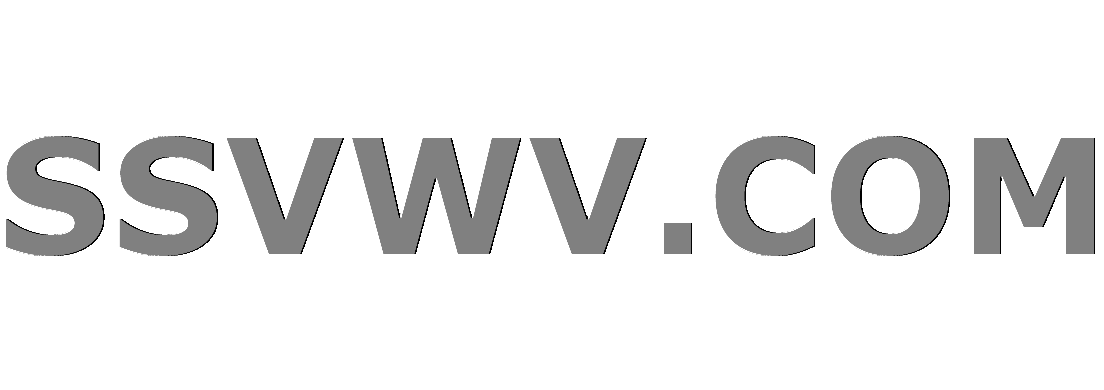
Multi tool use
How can I register/add a custom shutdown routine that shall fire when my Spring Boot application shuts down?
Scenario: I deploy my Spring Boot application to a Jetty servlet container (i.e., no embedded Jetty). My application uses Logback for logging, and I want to change logging levels during runtime using Logback's MBean JMX configurator. Its documentation states that to avoid memory leaks, on shutdown a specific LoggerContext shutdown method has to be called.
What are good ways to listen on Spring Boot shutdown events?
I have tried:
public static void main(String args) throws Exception {
ConfigurableApplicationContext cac = SpringApplication.run(Example.class, args);
cac.addApplicationListener(new ApplicationListener<ContextClosedEvent>() {
@Override
public void onApplicationEvent(ContextClosedEvent event) {
logger.info("Do something");
}
});
}
but this registered listener does not get called when the application shuts down.
java spring-boot
add a comment |
How can I register/add a custom shutdown routine that shall fire when my Spring Boot application shuts down?
Scenario: I deploy my Spring Boot application to a Jetty servlet container (i.e., no embedded Jetty). My application uses Logback for logging, and I want to change logging levels during runtime using Logback's MBean JMX configurator. Its documentation states that to avoid memory leaks, on shutdown a specific LoggerContext shutdown method has to be called.
What are good ways to listen on Spring Boot shutdown events?
I have tried:
public static void main(String args) throws Exception {
ConfigurableApplicationContext cac = SpringApplication.run(Example.class, args);
cac.addApplicationListener(new ApplicationListener<ContextClosedEvent>() {
@Override
public void onApplicationEvent(ContextClosedEvent event) {
logger.info("Do something");
}
});
}
but this registered listener does not get called when the application shuts down.
java spring-boot
You are registering the listener after the context is created, basically rendering it useless. If you want it to participate you need to register it as a bean in your application context like any other bean.
– M. Deinum
Nov 2 '14 at 17:07
add a comment |
How can I register/add a custom shutdown routine that shall fire when my Spring Boot application shuts down?
Scenario: I deploy my Spring Boot application to a Jetty servlet container (i.e., no embedded Jetty). My application uses Logback for logging, and I want to change logging levels during runtime using Logback's MBean JMX configurator. Its documentation states that to avoid memory leaks, on shutdown a specific LoggerContext shutdown method has to be called.
What are good ways to listen on Spring Boot shutdown events?
I have tried:
public static void main(String args) throws Exception {
ConfigurableApplicationContext cac = SpringApplication.run(Example.class, args);
cac.addApplicationListener(new ApplicationListener<ContextClosedEvent>() {
@Override
public void onApplicationEvent(ContextClosedEvent event) {
logger.info("Do something");
}
});
}
but this registered listener does not get called when the application shuts down.
java spring-boot
How can I register/add a custom shutdown routine that shall fire when my Spring Boot application shuts down?
Scenario: I deploy my Spring Boot application to a Jetty servlet container (i.e., no embedded Jetty). My application uses Logback for logging, and I want to change logging levels during runtime using Logback's MBean JMX configurator. Its documentation states that to avoid memory leaks, on shutdown a specific LoggerContext shutdown method has to be called.
What are good ways to listen on Spring Boot shutdown events?
I have tried:
public static void main(String args) throws Exception {
ConfigurableApplicationContext cac = SpringApplication.run(Example.class, args);
cac.addApplicationListener(new ApplicationListener<ContextClosedEvent>() {
@Override
public void onApplicationEvent(ContextClosedEvent event) {
logger.info("Do something");
}
});
}
but this registered listener does not get called when the application shuts down.
java spring-boot
java spring-boot
edited Oct 31 '14 at 22:57


Riggs
665516
665516
asked Oct 31 '14 at 15:29
AbdullAbdull
14.6k1486135
14.6k1486135
You are registering the listener after the context is created, basically rendering it useless. If you want it to participate you need to register it as a bean in your application context like any other bean.
– M. Deinum
Nov 2 '14 at 17:07
add a comment |
You are registering the listener after the context is created, basically rendering it useless. If you want it to participate you need to register it as a bean in your application context like any other bean.
– M. Deinum
Nov 2 '14 at 17:07
You are registering the listener after the context is created, basically rendering it useless. If you want it to participate you need to register it as a bean in your application context like any other bean.
– M. Deinum
Nov 2 '14 at 17:07
You are registering the listener after the context is created, basically rendering it useless. If you want it to participate you need to register it as a bean in your application context like any other bean.
– M. Deinum
Nov 2 '14 at 17:07
add a comment |
4 Answers
4
active
oldest
votes
http://docs.spring.io/spring-boot/docs/current-SNAPSHOT/reference/htmlsingle/#boot-features-application-exit
Each SpringApplication will register a shutdown hook with the JVM to ensure that the ApplicationContext is closed gracefully on exit. All the standard Spring lifecycle callbacks (such as the DisposableBean interface, or the @PreDestroy annotation) can be used.
In addition, beans may implement the org.springframework.boot.ExitCodeGenerator interface if they wish to return a specific exit code when the application ends.
I have updated my question to show my current unsuccessful effort
– Abdull
Oct 31 '14 at 16:24
Updated link docs.spring.io/spring-boot/docs/current/reference/html/…
– Kenny
Dec 18 '18 at 7:32
add a comment |
Your listener is registered too late (that line will never be reached until the context has already closed). It should suffice to make it a @Bean
.
add a comment |
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletListenerRegistrationBean;
import org.springframework.boot.web.support.SpringBootServletInitializer;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
@EnableAutoConfiguration
public class Application extends SpringBootServletInitializer {
public static void main(
String args) {
SpringApplication.run(Application.class,
args);
}
@NotNull
@Bean
ServletListenerRegistrationBean<ServletContextListener> myServletListener() {
ServletListenerRegistrationBean<ServletContextListener> srb =
new ServletListenerRegistrationBean<>();
srb.setListener(new ExampleServletContextListener());
return srb;
}
}
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
public class ExampleServletContextListener implements ServletContextListener {
@Override
public void contextInitialized(
ServletContextEvent sce) {
// Context Initialised
}
@Override
public void contextDestroyed(
ServletContextEvent sce) {
// Here - what you want to do that context shutdown
}
}
add a comment |
have you tried this as mentioned by @cfrick ?
@SpringBootApplication
@Slf4j
public class SpringBootShutdownHookApplication {
public static void main(String args) {
SpringApplication.run(SpringBootShutdownHookApplication.class, args);
}
@PreDestroy
public void onExit() {
log.info("###STOPing###");
try {
Thread.sleep(5 * 1000);
} catch (InterruptedException e) {
log.error("", e);;
}
log.info("###STOP FROM THE LIFECYCLE###");
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f26678208%2fspring-boot-shutdown-hook%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
http://docs.spring.io/spring-boot/docs/current-SNAPSHOT/reference/htmlsingle/#boot-features-application-exit
Each SpringApplication will register a shutdown hook with the JVM to ensure that the ApplicationContext is closed gracefully on exit. All the standard Spring lifecycle callbacks (such as the DisposableBean interface, or the @PreDestroy annotation) can be used.
In addition, beans may implement the org.springframework.boot.ExitCodeGenerator interface if they wish to return a specific exit code when the application ends.
I have updated my question to show my current unsuccessful effort
– Abdull
Oct 31 '14 at 16:24
Updated link docs.spring.io/spring-boot/docs/current/reference/html/…
– Kenny
Dec 18 '18 at 7:32
add a comment |
http://docs.spring.io/spring-boot/docs/current-SNAPSHOT/reference/htmlsingle/#boot-features-application-exit
Each SpringApplication will register a shutdown hook with the JVM to ensure that the ApplicationContext is closed gracefully on exit. All the standard Spring lifecycle callbacks (such as the DisposableBean interface, or the @PreDestroy annotation) can be used.
In addition, beans may implement the org.springframework.boot.ExitCodeGenerator interface if they wish to return a specific exit code when the application ends.
I have updated my question to show my current unsuccessful effort
– Abdull
Oct 31 '14 at 16:24
Updated link docs.spring.io/spring-boot/docs/current/reference/html/…
– Kenny
Dec 18 '18 at 7:32
add a comment |
http://docs.spring.io/spring-boot/docs/current-SNAPSHOT/reference/htmlsingle/#boot-features-application-exit
Each SpringApplication will register a shutdown hook with the JVM to ensure that the ApplicationContext is closed gracefully on exit. All the standard Spring lifecycle callbacks (such as the DisposableBean interface, or the @PreDestroy annotation) can be used.
In addition, beans may implement the org.springframework.boot.ExitCodeGenerator interface if they wish to return a specific exit code when the application ends.
http://docs.spring.io/spring-boot/docs/current-SNAPSHOT/reference/htmlsingle/#boot-features-application-exit
Each SpringApplication will register a shutdown hook with the JVM to ensure that the ApplicationContext is closed gracefully on exit. All the standard Spring lifecycle callbacks (such as the DisposableBean interface, or the @PreDestroy annotation) can be used.
In addition, beans may implement the org.springframework.boot.ExitCodeGenerator interface if they wish to return a specific exit code when the application ends.
answered Oct 31 '14 at 15:49


cfrickcfrick
18.2k13552
18.2k13552
I have updated my question to show my current unsuccessful effort
– Abdull
Oct 31 '14 at 16:24
Updated link docs.spring.io/spring-boot/docs/current/reference/html/…
– Kenny
Dec 18 '18 at 7:32
add a comment |
I have updated my question to show my current unsuccessful effort
– Abdull
Oct 31 '14 at 16:24
Updated link docs.spring.io/spring-boot/docs/current/reference/html/…
– Kenny
Dec 18 '18 at 7:32
I have updated my question to show my current unsuccessful effort
– Abdull
Oct 31 '14 at 16:24
I have updated my question to show my current unsuccessful effort
– Abdull
Oct 31 '14 at 16:24
Updated link docs.spring.io/spring-boot/docs/current/reference/html/…
– Kenny
Dec 18 '18 at 7:32
Updated link docs.spring.io/spring-boot/docs/current/reference/html/…
– Kenny
Dec 18 '18 at 7:32
add a comment |
Your listener is registered too late (that line will never be reached until the context has already closed). It should suffice to make it a @Bean
.
add a comment |
Your listener is registered too late (that line will never be reached until the context has already closed). It should suffice to make it a @Bean
.
add a comment |
Your listener is registered too late (that line will never be reached until the context has already closed). It should suffice to make it a @Bean
.
Your listener is registered too late (that line will never be reached until the context has already closed). It should suffice to make it a @Bean
.
answered Nov 1 '14 at 12:07


Dave SyerDave Syer
42.8k9116113
42.8k9116113
add a comment |
add a comment |
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletListenerRegistrationBean;
import org.springframework.boot.web.support.SpringBootServletInitializer;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
@EnableAutoConfiguration
public class Application extends SpringBootServletInitializer {
public static void main(
String args) {
SpringApplication.run(Application.class,
args);
}
@NotNull
@Bean
ServletListenerRegistrationBean<ServletContextListener> myServletListener() {
ServletListenerRegistrationBean<ServletContextListener> srb =
new ServletListenerRegistrationBean<>();
srb.setListener(new ExampleServletContextListener());
return srb;
}
}
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
public class ExampleServletContextListener implements ServletContextListener {
@Override
public void contextInitialized(
ServletContextEvent sce) {
// Context Initialised
}
@Override
public void contextDestroyed(
ServletContextEvent sce) {
// Here - what you want to do that context shutdown
}
}
add a comment |
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletListenerRegistrationBean;
import org.springframework.boot.web.support.SpringBootServletInitializer;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
@EnableAutoConfiguration
public class Application extends SpringBootServletInitializer {
public static void main(
String args) {
SpringApplication.run(Application.class,
args);
}
@NotNull
@Bean
ServletListenerRegistrationBean<ServletContextListener> myServletListener() {
ServletListenerRegistrationBean<ServletContextListener> srb =
new ServletListenerRegistrationBean<>();
srb.setListener(new ExampleServletContextListener());
return srb;
}
}
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
public class ExampleServletContextListener implements ServletContextListener {
@Override
public void contextInitialized(
ServletContextEvent sce) {
// Context Initialised
}
@Override
public void contextDestroyed(
ServletContextEvent sce) {
// Here - what you want to do that context shutdown
}
}
add a comment |
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletListenerRegistrationBean;
import org.springframework.boot.web.support.SpringBootServletInitializer;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
@EnableAutoConfiguration
public class Application extends SpringBootServletInitializer {
public static void main(
String args) {
SpringApplication.run(Application.class,
args);
}
@NotNull
@Bean
ServletListenerRegistrationBean<ServletContextListener> myServletListener() {
ServletListenerRegistrationBean<ServletContextListener> srb =
new ServletListenerRegistrationBean<>();
srb.setListener(new ExampleServletContextListener());
return srb;
}
}
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
public class ExampleServletContextListener implements ServletContextListener {
@Override
public void contextInitialized(
ServletContextEvent sce) {
// Context Initialised
}
@Override
public void contextDestroyed(
ServletContextEvent sce) {
// Here - what you want to do that context shutdown
}
}
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.ServletListenerRegistrationBean;
import org.springframework.boot.web.support.SpringBootServletInitializer;
import org.springframework.context.annotation.Bean;
@SpringBootApplication
@EnableAutoConfiguration
public class Application extends SpringBootServletInitializer {
public static void main(
String args) {
SpringApplication.run(Application.class,
args);
}
@NotNull
@Bean
ServletListenerRegistrationBean<ServletContextListener> myServletListener() {
ServletListenerRegistrationBean<ServletContextListener> srb =
new ServletListenerRegistrationBean<>();
srb.setListener(new ExampleServletContextListener());
return srb;
}
}
import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
public class ExampleServletContextListener implements ServletContextListener {
@Override
public void contextInitialized(
ServletContextEvent sce) {
// Context Initialised
}
@Override
public void contextDestroyed(
ServletContextEvent sce) {
// Here - what you want to do that context shutdown
}
}
edited Aug 18 '18 at 14:25


geisterfurz007
2,38821834
2,38821834
answered Jan 10 '18 at 6:20


harsh.tibrewalharsh.tibrewal
30627
30627
add a comment |
add a comment |
have you tried this as mentioned by @cfrick ?
@SpringBootApplication
@Slf4j
public class SpringBootShutdownHookApplication {
public static void main(String args) {
SpringApplication.run(SpringBootShutdownHookApplication.class, args);
}
@PreDestroy
public void onExit() {
log.info("###STOPing###");
try {
Thread.sleep(5 * 1000);
} catch (InterruptedException e) {
log.error("", e);;
}
log.info("###STOP FROM THE LIFECYCLE###");
}
}
add a comment |
have you tried this as mentioned by @cfrick ?
@SpringBootApplication
@Slf4j
public class SpringBootShutdownHookApplication {
public static void main(String args) {
SpringApplication.run(SpringBootShutdownHookApplication.class, args);
}
@PreDestroy
public void onExit() {
log.info("###STOPing###");
try {
Thread.sleep(5 * 1000);
} catch (InterruptedException e) {
log.error("", e);;
}
log.info("###STOP FROM THE LIFECYCLE###");
}
}
add a comment |
have you tried this as mentioned by @cfrick ?
@SpringBootApplication
@Slf4j
public class SpringBootShutdownHookApplication {
public static void main(String args) {
SpringApplication.run(SpringBootShutdownHookApplication.class, args);
}
@PreDestroy
public void onExit() {
log.info("###STOPing###");
try {
Thread.sleep(5 * 1000);
} catch (InterruptedException e) {
log.error("", e);;
}
log.info("###STOP FROM THE LIFECYCLE###");
}
}
have you tried this as mentioned by @cfrick ?
@SpringBootApplication
@Slf4j
public class SpringBootShutdownHookApplication {
public static void main(String args) {
SpringApplication.run(SpringBootShutdownHookApplication.class, args);
}
@PreDestroy
public void onExit() {
log.info("###STOPing###");
try {
Thread.sleep(5 * 1000);
} catch (InterruptedException e) {
log.error("", e);;
}
log.info("###STOP FROM THE LIFECYCLE###");
}
}
answered Nov 19 '18 at 7:16
lizhipenglizhipeng
612
612
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f26678208%2fspring-boot-shutdown-hook%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
N1cNM5,v5CCW4x29Dvi B6f7MQVf,MV UwE,CG7wcQl1isHm6tyX0N3ARrMp5bLQZ2S6I
You are registering the listener after the context is created, basically rendering it useless. If you want it to participate you need to register it as a bean in your application context like any other bean.
– M. Deinum
Nov 2 '14 at 17:07