Cannot insert data, foreign key error on postgresql
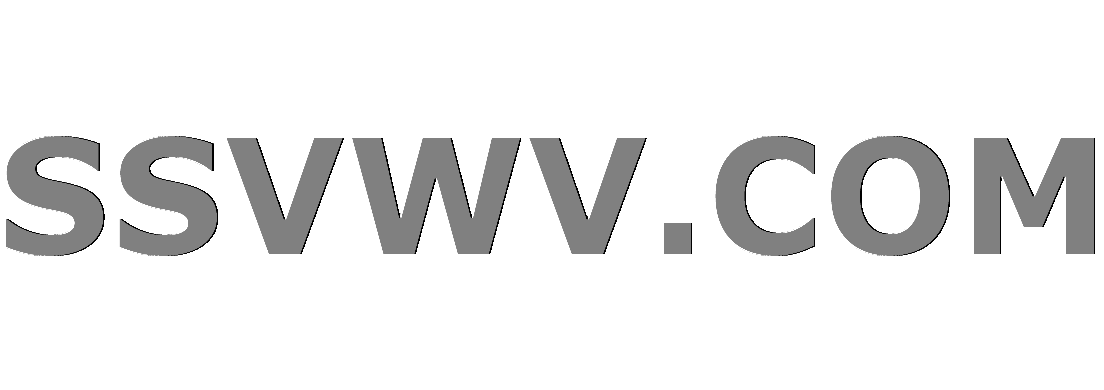
Multi tool use
I have 3 tables, where the table INTRUSIONS have foreign keys belonging to CCTVS and ALARMS tables. I want the foreign keys to be nullable in my INTRUSIONS table. I am not sure why but I cannot insert data into my 'INTRUSIONS' table. Here is my code:
CREATE TABLE
REMOTE_SECURITY.CCTVS(CCTV_ID serial);
CREATE TABLE
REMOTE_SECURITY.ALARMS(ALARM_ID serial);
CREATE TABLE
REMOTE_SECURITY.INTRUSIONS(INTRUSION_ID serial,CCTV_ID serial,ALARM_ID serial);
ALTER TABLE
REMOTE_SECURITY.CCTVS ADD CONSTRAINT
CCTVS_PK PRIMARY KEY
(CCTV_ID)
;
ALTER TABLE
REMOTE_SECURITY.ALARMS ADD CONSTRAINT
ALARMS_PK PRIMARY KEY
(ALARM_ID)
;
This code doesn't work:
INSERT
INTO
REMOTE_SECURITY.INTRUSIONS
(
INTRUSION_ID
, CCTV_ID
, ALARM_ID
) VALUES (
1
, NULL
, 1
)
;
Here is the error:
[2018-11-19 19:35:59] [23502] ERROR: null value in column "cctv_id" violates not-null constraint
[2018-11-19 19:35:59] Detail: Failing row contains (1, null, 1, 2010-02-01 07:00:01).
sql postgresql sql-insert
add a comment |
I have 3 tables, where the table INTRUSIONS have foreign keys belonging to CCTVS and ALARMS tables. I want the foreign keys to be nullable in my INTRUSIONS table. I am not sure why but I cannot insert data into my 'INTRUSIONS' table. Here is my code:
CREATE TABLE
REMOTE_SECURITY.CCTVS(CCTV_ID serial);
CREATE TABLE
REMOTE_SECURITY.ALARMS(ALARM_ID serial);
CREATE TABLE
REMOTE_SECURITY.INTRUSIONS(INTRUSION_ID serial,CCTV_ID serial,ALARM_ID serial);
ALTER TABLE
REMOTE_SECURITY.CCTVS ADD CONSTRAINT
CCTVS_PK PRIMARY KEY
(CCTV_ID)
;
ALTER TABLE
REMOTE_SECURITY.ALARMS ADD CONSTRAINT
ALARMS_PK PRIMARY KEY
(ALARM_ID)
;
This code doesn't work:
INSERT
INTO
REMOTE_SECURITY.INTRUSIONS
(
INTRUSION_ID
, CCTV_ID
, ALARM_ID
) VALUES (
1
, NULL
, 1
)
;
Here is the error:
[2018-11-19 19:35:59] [23502] ERROR: null value in column "cctv_id" violates not-null constraint
[2018-11-19 19:35:59] Detail: Failing row contains (1, null, 1, 2010-02-01 07:00:01).
sql postgresql sql-insert
add a comment |
I have 3 tables, where the table INTRUSIONS have foreign keys belonging to CCTVS and ALARMS tables. I want the foreign keys to be nullable in my INTRUSIONS table. I am not sure why but I cannot insert data into my 'INTRUSIONS' table. Here is my code:
CREATE TABLE
REMOTE_SECURITY.CCTVS(CCTV_ID serial);
CREATE TABLE
REMOTE_SECURITY.ALARMS(ALARM_ID serial);
CREATE TABLE
REMOTE_SECURITY.INTRUSIONS(INTRUSION_ID serial,CCTV_ID serial,ALARM_ID serial);
ALTER TABLE
REMOTE_SECURITY.CCTVS ADD CONSTRAINT
CCTVS_PK PRIMARY KEY
(CCTV_ID)
;
ALTER TABLE
REMOTE_SECURITY.ALARMS ADD CONSTRAINT
ALARMS_PK PRIMARY KEY
(ALARM_ID)
;
This code doesn't work:
INSERT
INTO
REMOTE_SECURITY.INTRUSIONS
(
INTRUSION_ID
, CCTV_ID
, ALARM_ID
) VALUES (
1
, NULL
, 1
)
;
Here is the error:
[2018-11-19 19:35:59] [23502] ERROR: null value in column "cctv_id" violates not-null constraint
[2018-11-19 19:35:59] Detail: Failing row contains (1, null, 1, 2010-02-01 07:00:01).
sql postgresql sql-insert
I have 3 tables, where the table INTRUSIONS have foreign keys belonging to CCTVS and ALARMS tables. I want the foreign keys to be nullable in my INTRUSIONS table. I am not sure why but I cannot insert data into my 'INTRUSIONS' table. Here is my code:
CREATE TABLE
REMOTE_SECURITY.CCTVS(CCTV_ID serial);
CREATE TABLE
REMOTE_SECURITY.ALARMS(ALARM_ID serial);
CREATE TABLE
REMOTE_SECURITY.INTRUSIONS(INTRUSION_ID serial,CCTV_ID serial,ALARM_ID serial);
ALTER TABLE
REMOTE_SECURITY.CCTVS ADD CONSTRAINT
CCTVS_PK PRIMARY KEY
(CCTV_ID)
;
ALTER TABLE
REMOTE_SECURITY.ALARMS ADD CONSTRAINT
ALARMS_PK PRIMARY KEY
(ALARM_ID)
;
This code doesn't work:
INSERT
INTO
REMOTE_SECURITY.INTRUSIONS
(
INTRUSION_ID
, CCTV_ID
, ALARM_ID
) VALUES (
1
, NULL
, 1
)
;
Here is the error:
[2018-11-19 19:35:59] [23502] ERROR: null value in column "cctv_id" violates not-null constraint
[2018-11-19 19:35:59] Detail: Failing row contains (1, null, 1, 2010-02-01 07:00:01).
sql postgresql sql-insert
sql postgresql sql-insert
edited Nov 21 '18 at 7:16
a_horse_with_no_name
296k46451546
296k46451546
asked Nov 19 '18 at 19:46
AmorosoAmoroso
1571212
1571212
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
The foreign key forces the column to be non null. If you want a foreign key association it won't know how to relate null to another table. From the documentation
A foreign key constraint specifies that the values in a column (or a group of columns) must match the values appearing in some row of another table. We say this maintains the referential integrity between two related tables.
https://www.postgresql.org/docs/9.4/ddl-constraints.html
add a comment |
Your Error
are clear.. You cannot put Null
value to Foreign Key
or Serial
.. Maybe you are wrong in the concept design your schema
..
CREATE TABLE tablename (
colname SERIAL
);
is equivalent to specifying:
CREATE SEQUENCE tablename_colname_seq;
CREATE TABLE tablename (
colname integer NOT NULL DEFAULT nextval('tablename_colname_seq')
);
ALTER SEQUENCE tablename_colname_seq OWNED BY tablename.colname;
As noted by Documentation Serial
data type hold Not Null
so it can't be Null
and Other answer really great tell about constraint foreign key..
As Documentation FK
said
We say this maintains the referential integrity between two related
tables
So.. The value in FK
are referencing to Parents value which it is the PK
.. PK
cannot have Null
so FK
too..
add a comment |
A foreign key column (intrusions.cctv_id
or intrusions.alarm_id
in your case) should not be defined as serial
as you don't want to generate new values every time you insert into them. You use the generated values from the referenced primary key column(s)
As dwir182 has pointed out, a serial
column is implicitly defined as not null
. By fixxing the incorrect definition of the FK columns, you will be able to insert NULL values.
So you want:
CREATE TABLE remote_security.intrusions
(
intrusion_id serial,
-- no serial for the foreign key columns!
cctv_id integer references cctvs,
alarm_id integer references alarms
);
Then you can do the following:
insert into remote_security.cctvs values (default); -- creates id = 1;
insert into remote_security.alarms values (default); -- creates id = 1;
-- do not specify a value for the serial column!
insert into remote_security.intrusions
(intrusion_id, cctv_id, alarm_id)
values
(default, null, 1);
See the online example: https://rextester.com/ELPHK12991
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53381631%2fcannot-insert-data-foreign-key-error-on-postgresql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
The foreign key forces the column to be non null. If you want a foreign key association it won't know how to relate null to another table. From the documentation
A foreign key constraint specifies that the values in a column (or a group of columns) must match the values appearing in some row of another table. We say this maintains the referential integrity between two related tables.
https://www.postgresql.org/docs/9.4/ddl-constraints.html
add a comment |
The foreign key forces the column to be non null. If you want a foreign key association it won't know how to relate null to another table. From the documentation
A foreign key constraint specifies that the values in a column (or a group of columns) must match the values appearing in some row of another table. We say this maintains the referential integrity between two related tables.
https://www.postgresql.org/docs/9.4/ddl-constraints.html
add a comment |
The foreign key forces the column to be non null. If you want a foreign key association it won't know how to relate null to another table. From the documentation
A foreign key constraint specifies that the values in a column (or a group of columns) must match the values appearing in some row of another table. We say this maintains the referential integrity between two related tables.
https://www.postgresql.org/docs/9.4/ddl-constraints.html
The foreign key forces the column to be non null. If you want a foreign key association it won't know how to relate null to another table. From the documentation
A foreign key constraint specifies that the values in a column (or a group of columns) must match the values appearing in some row of another table. We say this maintains the referential integrity between two related tables.
https://www.postgresql.org/docs/9.4/ddl-constraints.html
answered Nov 19 '18 at 21:20
Nick EllisNick Ellis
699718
699718
add a comment |
add a comment |
Your Error
are clear.. You cannot put Null
value to Foreign Key
or Serial
.. Maybe you are wrong in the concept design your schema
..
CREATE TABLE tablename (
colname SERIAL
);
is equivalent to specifying:
CREATE SEQUENCE tablename_colname_seq;
CREATE TABLE tablename (
colname integer NOT NULL DEFAULT nextval('tablename_colname_seq')
);
ALTER SEQUENCE tablename_colname_seq OWNED BY tablename.colname;
As noted by Documentation Serial
data type hold Not Null
so it can't be Null
and Other answer really great tell about constraint foreign key..
As Documentation FK
said
We say this maintains the referential integrity between two related
tables
So.. The value in FK
are referencing to Parents value which it is the PK
.. PK
cannot have Null
so FK
too..
add a comment |
Your Error
are clear.. You cannot put Null
value to Foreign Key
or Serial
.. Maybe you are wrong in the concept design your schema
..
CREATE TABLE tablename (
colname SERIAL
);
is equivalent to specifying:
CREATE SEQUENCE tablename_colname_seq;
CREATE TABLE tablename (
colname integer NOT NULL DEFAULT nextval('tablename_colname_seq')
);
ALTER SEQUENCE tablename_colname_seq OWNED BY tablename.colname;
As noted by Documentation Serial
data type hold Not Null
so it can't be Null
and Other answer really great tell about constraint foreign key..
As Documentation FK
said
We say this maintains the referential integrity between two related
tables
So.. The value in FK
are referencing to Parents value which it is the PK
.. PK
cannot have Null
so FK
too..
add a comment |
Your Error
are clear.. You cannot put Null
value to Foreign Key
or Serial
.. Maybe you are wrong in the concept design your schema
..
CREATE TABLE tablename (
colname SERIAL
);
is equivalent to specifying:
CREATE SEQUENCE tablename_colname_seq;
CREATE TABLE tablename (
colname integer NOT NULL DEFAULT nextval('tablename_colname_seq')
);
ALTER SEQUENCE tablename_colname_seq OWNED BY tablename.colname;
As noted by Documentation Serial
data type hold Not Null
so it can't be Null
and Other answer really great tell about constraint foreign key..
As Documentation FK
said
We say this maintains the referential integrity between two related
tables
So.. The value in FK
are referencing to Parents value which it is the PK
.. PK
cannot have Null
so FK
too..
Your Error
are clear.. You cannot put Null
value to Foreign Key
or Serial
.. Maybe you are wrong in the concept design your schema
..
CREATE TABLE tablename (
colname SERIAL
);
is equivalent to specifying:
CREATE SEQUENCE tablename_colname_seq;
CREATE TABLE tablename (
colname integer NOT NULL DEFAULT nextval('tablename_colname_seq')
);
ALTER SEQUENCE tablename_colname_seq OWNED BY tablename.colname;
As noted by Documentation Serial
data type hold Not Null
so it can't be Null
and Other answer really great tell about constraint foreign key..
As Documentation FK
said
We say this maintains the referential integrity between two related
tables
So.. The value in FK
are referencing to Parents value which it is the PK
.. PK
cannot have Null
so FK
too..
answered Nov 21 '18 at 2:23


dwir182dwir182
1,418618
1,418618
add a comment |
add a comment |
A foreign key column (intrusions.cctv_id
or intrusions.alarm_id
in your case) should not be defined as serial
as you don't want to generate new values every time you insert into them. You use the generated values from the referenced primary key column(s)
As dwir182 has pointed out, a serial
column is implicitly defined as not null
. By fixxing the incorrect definition of the FK columns, you will be able to insert NULL values.
So you want:
CREATE TABLE remote_security.intrusions
(
intrusion_id serial,
-- no serial for the foreign key columns!
cctv_id integer references cctvs,
alarm_id integer references alarms
);
Then you can do the following:
insert into remote_security.cctvs values (default); -- creates id = 1;
insert into remote_security.alarms values (default); -- creates id = 1;
-- do not specify a value for the serial column!
insert into remote_security.intrusions
(intrusion_id, cctv_id, alarm_id)
values
(default, null, 1);
See the online example: https://rextester.com/ELPHK12991
add a comment |
A foreign key column (intrusions.cctv_id
or intrusions.alarm_id
in your case) should not be defined as serial
as you don't want to generate new values every time you insert into them. You use the generated values from the referenced primary key column(s)
As dwir182 has pointed out, a serial
column is implicitly defined as not null
. By fixxing the incorrect definition of the FK columns, you will be able to insert NULL values.
So you want:
CREATE TABLE remote_security.intrusions
(
intrusion_id serial,
-- no serial for the foreign key columns!
cctv_id integer references cctvs,
alarm_id integer references alarms
);
Then you can do the following:
insert into remote_security.cctvs values (default); -- creates id = 1;
insert into remote_security.alarms values (default); -- creates id = 1;
-- do not specify a value for the serial column!
insert into remote_security.intrusions
(intrusion_id, cctv_id, alarm_id)
values
(default, null, 1);
See the online example: https://rextester.com/ELPHK12991
add a comment |
A foreign key column (intrusions.cctv_id
or intrusions.alarm_id
in your case) should not be defined as serial
as you don't want to generate new values every time you insert into them. You use the generated values from the referenced primary key column(s)
As dwir182 has pointed out, a serial
column is implicitly defined as not null
. By fixxing the incorrect definition of the FK columns, you will be able to insert NULL values.
So you want:
CREATE TABLE remote_security.intrusions
(
intrusion_id serial,
-- no serial for the foreign key columns!
cctv_id integer references cctvs,
alarm_id integer references alarms
);
Then you can do the following:
insert into remote_security.cctvs values (default); -- creates id = 1;
insert into remote_security.alarms values (default); -- creates id = 1;
-- do not specify a value for the serial column!
insert into remote_security.intrusions
(intrusion_id, cctv_id, alarm_id)
values
(default, null, 1);
See the online example: https://rextester.com/ELPHK12991
A foreign key column (intrusions.cctv_id
or intrusions.alarm_id
in your case) should not be defined as serial
as you don't want to generate new values every time you insert into them. You use the generated values from the referenced primary key column(s)
As dwir182 has pointed out, a serial
column is implicitly defined as not null
. By fixxing the incorrect definition of the FK columns, you will be able to insert NULL values.
So you want:
CREATE TABLE remote_security.intrusions
(
intrusion_id serial,
-- no serial for the foreign key columns!
cctv_id integer references cctvs,
alarm_id integer references alarms
);
Then you can do the following:
insert into remote_security.cctvs values (default); -- creates id = 1;
insert into remote_security.alarms values (default); -- creates id = 1;
-- do not specify a value for the serial column!
insert into remote_security.intrusions
(intrusion_id, cctv_id, alarm_id)
values
(default, null, 1);
See the online example: https://rextester.com/ELPHK12991
answered Nov 21 '18 at 7:30
a_horse_with_no_namea_horse_with_no_name
296k46451546
296k46451546
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53381631%2fcannot-insert-data-foreign-key-error-on-postgresql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
L6X2gHgi mmiv7kJ CF7HskkoKUETcwvW3eRM W1 3j3K0GFxCngr,fWSWt79 b