passport/passport-civic: Error exchanging code for data: [Bad Request] missing authToken
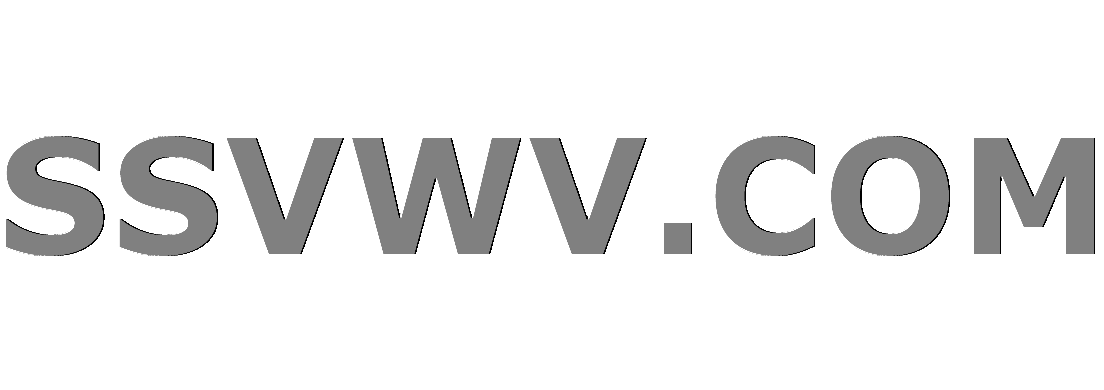
Multi tool use
up vote
0
down vote
favorite
I am trying to configure passport-civic
, here is my code:
var createError = require('http-errors');
var express = require('express');
var path = require('path');
var cookieParser = require('cookie-parser');
var logger = require('morgan');
var passport = require('passport');
var CivicStrategy = require('passport-civic').Strategy;
var indexRouter = require('./routes/index');
var usersRouter = require('./routes/users');
var loginRouter = require('./routes/login');
var app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'jade');
app.use(logger('dev'));
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(cookieParser());
app.use(require('express-session')({ secret: 'xxx', resave: true, saveUninitialized: true }));
app.use(passport.initialize());
app.use(passport.session());
app.use(express.static(path.join(__dirname, 'public')));
app.use('/', indexRouter);
app.use('/users', usersRouter);
app.use('/login', loginRouter);
passport.use(new CivicStrategy({
appId: 'xxx',
prvKey: 'xxx',
appSecret: 'xxx'
},
function(profile, done) {
User.findOrCreate({ civicId: profile.userId }, function (err, user) {
return done(err, user);
});
}
));
passport.serializeUser(function(user, done) {
done(null, user.id);
});
passport.deserializeUser(function(id, done) {
User.findById(id, function (err, user) {
done(err, user);
});
});
app.get('/auth/civic', passport.authenticate('civic'));
app.post('/auth/civic',
passport.authenticate('civic', { failureRedirect: '/login' }),
function(req, res) {
// Successful authentication, redirect home.
res.redirect('/');
}
);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
next(createError(404));
});
// error handler
app.use(function(err, req, res, next) {
// set locals, only providing error in development
res.locals.message = err.message;
res.locals.error = req.app.get('env') === 'development' ? err : {};
// render the error page
res.status(err.status || 500);
res.render('error');
});
module.exports = app;
The error when I reach /auth/civic
:
Error: Error exchanging code for data: [Bad Request] missing authToken
at Object._callee$ (/Volumes/EamonWD/omniatm/omni-atm/node_modules/passport-civic/node_modules/civic-sip-api/dist/index.js:107:26)
at tryCatch (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:62:40)
at Generator.invoke [as _invoke] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:296:22)
at Generator.prototype.(anonymous function) [as throw] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:114:21)
at step (/Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:17:30)
at /Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:30:13
at process._tickCallback (internal/process/next_tick.js:68:7)
You can find the passport-civic
documentation here: https://www.npmjs.com/package/passport-civic
Here is passport
documentation: http://www.passportjs.org/docs/
UPDATE
When creating the civic
app in their dashboard, they asked for whitelist
domains - could this error be because I am trying it on localhost and not the domain I whitelisted (omniatm.eamondev.com
)?
UPDATE
I tried it on my domain ominatm.eamondev.com
and it seems like the whitelist
isn't the problem as I still get the error.
javascript node.js express authentication passport.js
add a comment |
up vote
0
down vote
favorite
I am trying to configure passport-civic
, here is my code:
var createError = require('http-errors');
var express = require('express');
var path = require('path');
var cookieParser = require('cookie-parser');
var logger = require('morgan');
var passport = require('passport');
var CivicStrategy = require('passport-civic').Strategy;
var indexRouter = require('./routes/index');
var usersRouter = require('./routes/users');
var loginRouter = require('./routes/login');
var app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'jade');
app.use(logger('dev'));
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(cookieParser());
app.use(require('express-session')({ secret: 'xxx', resave: true, saveUninitialized: true }));
app.use(passport.initialize());
app.use(passport.session());
app.use(express.static(path.join(__dirname, 'public')));
app.use('/', indexRouter);
app.use('/users', usersRouter);
app.use('/login', loginRouter);
passport.use(new CivicStrategy({
appId: 'xxx',
prvKey: 'xxx',
appSecret: 'xxx'
},
function(profile, done) {
User.findOrCreate({ civicId: profile.userId }, function (err, user) {
return done(err, user);
});
}
));
passport.serializeUser(function(user, done) {
done(null, user.id);
});
passport.deserializeUser(function(id, done) {
User.findById(id, function (err, user) {
done(err, user);
});
});
app.get('/auth/civic', passport.authenticate('civic'));
app.post('/auth/civic',
passport.authenticate('civic', { failureRedirect: '/login' }),
function(req, res) {
// Successful authentication, redirect home.
res.redirect('/');
}
);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
next(createError(404));
});
// error handler
app.use(function(err, req, res, next) {
// set locals, only providing error in development
res.locals.message = err.message;
res.locals.error = req.app.get('env') === 'development' ? err : {};
// render the error page
res.status(err.status || 500);
res.render('error');
});
module.exports = app;
The error when I reach /auth/civic
:
Error: Error exchanging code for data: [Bad Request] missing authToken
at Object._callee$ (/Volumes/EamonWD/omniatm/omni-atm/node_modules/passport-civic/node_modules/civic-sip-api/dist/index.js:107:26)
at tryCatch (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:62:40)
at Generator.invoke [as _invoke] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:296:22)
at Generator.prototype.(anonymous function) [as throw] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:114:21)
at step (/Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:17:30)
at /Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:30:13
at process._tickCallback (internal/process/next_tick.js:68:7)
You can find the passport-civic
documentation here: https://www.npmjs.com/package/passport-civic
Here is passport
documentation: http://www.passportjs.org/docs/
UPDATE
When creating the civic
app in their dashboard, they asked for whitelist
domains - could this error be because I am trying it on localhost and not the domain I whitelisted (omniatm.eamondev.com
)?
UPDATE
I tried it on my domain ominatm.eamondev.com
and it seems like the whitelist
isn't the problem as I still get the error.
javascript node.js express authentication passport.js
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to configure passport-civic
, here is my code:
var createError = require('http-errors');
var express = require('express');
var path = require('path');
var cookieParser = require('cookie-parser');
var logger = require('morgan');
var passport = require('passport');
var CivicStrategy = require('passport-civic').Strategy;
var indexRouter = require('./routes/index');
var usersRouter = require('./routes/users');
var loginRouter = require('./routes/login');
var app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'jade');
app.use(logger('dev'));
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(cookieParser());
app.use(require('express-session')({ secret: 'xxx', resave: true, saveUninitialized: true }));
app.use(passport.initialize());
app.use(passport.session());
app.use(express.static(path.join(__dirname, 'public')));
app.use('/', indexRouter);
app.use('/users', usersRouter);
app.use('/login', loginRouter);
passport.use(new CivicStrategy({
appId: 'xxx',
prvKey: 'xxx',
appSecret: 'xxx'
},
function(profile, done) {
User.findOrCreate({ civicId: profile.userId }, function (err, user) {
return done(err, user);
});
}
));
passport.serializeUser(function(user, done) {
done(null, user.id);
});
passport.deserializeUser(function(id, done) {
User.findById(id, function (err, user) {
done(err, user);
});
});
app.get('/auth/civic', passport.authenticate('civic'));
app.post('/auth/civic',
passport.authenticate('civic', { failureRedirect: '/login' }),
function(req, res) {
// Successful authentication, redirect home.
res.redirect('/');
}
);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
next(createError(404));
});
// error handler
app.use(function(err, req, res, next) {
// set locals, only providing error in development
res.locals.message = err.message;
res.locals.error = req.app.get('env') === 'development' ? err : {};
// render the error page
res.status(err.status || 500);
res.render('error');
});
module.exports = app;
The error when I reach /auth/civic
:
Error: Error exchanging code for data: [Bad Request] missing authToken
at Object._callee$ (/Volumes/EamonWD/omniatm/omni-atm/node_modules/passport-civic/node_modules/civic-sip-api/dist/index.js:107:26)
at tryCatch (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:62:40)
at Generator.invoke [as _invoke] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:296:22)
at Generator.prototype.(anonymous function) [as throw] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:114:21)
at step (/Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:17:30)
at /Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:30:13
at process._tickCallback (internal/process/next_tick.js:68:7)
You can find the passport-civic
documentation here: https://www.npmjs.com/package/passport-civic
Here is passport
documentation: http://www.passportjs.org/docs/
UPDATE
When creating the civic
app in their dashboard, they asked for whitelist
domains - could this error be because I am trying it on localhost and not the domain I whitelisted (omniatm.eamondev.com
)?
UPDATE
I tried it on my domain ominatm.eamondev.com
and it seems like the whitelist
isn't the problem as I still get the error.
javascript node.js express authentication passport.js
I am trying to configure passport-civic
, here is my code:
var createError = require('http-errors');
var express = require('express');
var path = require('path');
var cookieParser = require('cookie-parser');
var logger = require('morgan');
var passport = require('passport');
var CivicStrategy = require('passport-civic').Strategy;
var indexRouter = require('./routes/index');
var usersRouter = require('./routes/users');
var loginRouter = require('./routes/login');
var app = express();
// view engine setup
app.set('views', path.join(__dirname, 'views'));
app.set('view engine', 'jade');
app.use(logger('dev'));
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(cookieParser());
app.use(require('express-session')({ secret: 'xxx', resave: true, saveUninitialized: true }));
app.use(passport.initialize());
app.use(passport.session());
app.use(express.static(path.join(__dirname, 'public')));
app.use('/', indexRouter);
app.use('/users', usersRouter);
app.use('/login', loginRouter);
passport.use(new CivicStrategy({
appId: 'xxx',
prvKey: 'xxx',
appSecret: 'xxx'
},
function(profile, done) {
User.findOrCreate({ civicId: profile.userId }, function (err, user) {
return done(err, user);
});
}
));
passport.serializeUser(function(user, done) {
done(null, user.id);
});
passport.deserializeUser(function(id, done) {
User.findById(id, function (err, user) {
done(err, user);
});
});
app.get('/auth/civic', passport.authenticate('civic'));
app.post('/auth/civic',
passport.authenticate('civic', { failureRedirect: '/login' }),
function(req, res) {
// Successful authentication, redirect home.
res.redirect('/');
}
);
// catch 404 and forward to error handler
app.use(function(req, res, next) {
next(createError(404));
});
// error handler
app.use(function(err, req, res, next) {
// set locals, only providing error in development
res.locals.message = err.message;
res.locals.error = req.app.get('env') === 'development' ? err : {};
// render the error page
res.status(err.status || 500);
res.render('error');
});
module.exports = app;
The error when I reach /auth/civic
:
Error: Error exchanging code for data: [Bad Request] missing authToken
at Object._callee$ (/Volumes/EamonWD/omniatm/omni-atm/node_modules/passport-civic/node_modules/civic-sip-api/dist/index.js:107:26)
at tryCatch (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:62:40)
at Generator.invoke [as _invoke] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:296:22)
at Generator.prototype.(anonymous function) [as throw] (/Volumes/EamonWD/omniatm/omni-atm/node_modules/regenerator-runtime/runtime.js:114:21)
at step (/Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:17:30)
at /Volumes/EamonWD/omniatm/omni-atm/node_modules/babel-runtime/helpers/asyncToGenerator.js:30:13
at process._tickCallback (internal/process/next_tick.js:68:7)
You can find the passport-civic
documentation here: https://www.npmjs.com/package/passport-civic
Here is passport
documentation: http://www.passportjs.org/docs/
UPDATE
When creating the civic
app in their dashboard, they asked for whitelist
domains - could this error be because I am trying it on localhost and not the domain I whitelisted (omniatm.eamondev.com
)?
UPDATE
I tried it on my domain ominatm.eamondev.com
and it seems like the whitelist
isn't the problem as I still get the error.
javascript node.js express authentication passport.js
javascript node.js express authentication passport.js
edited Nov 15 at 13:29
asked Nov 12 at 23:13


ewizard
1,06122467
1,06122467
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53271454%2fpassport-passport-civic-error-exchanging-code-for-data-bad-request-missing-a%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2C6Ghy3I0mBBAWSaLIqEAlAIpgXI1c dyW UWO T6qrio8IN3z1XNKFE3KU