Getting the first characters of each string in an array in Javascript
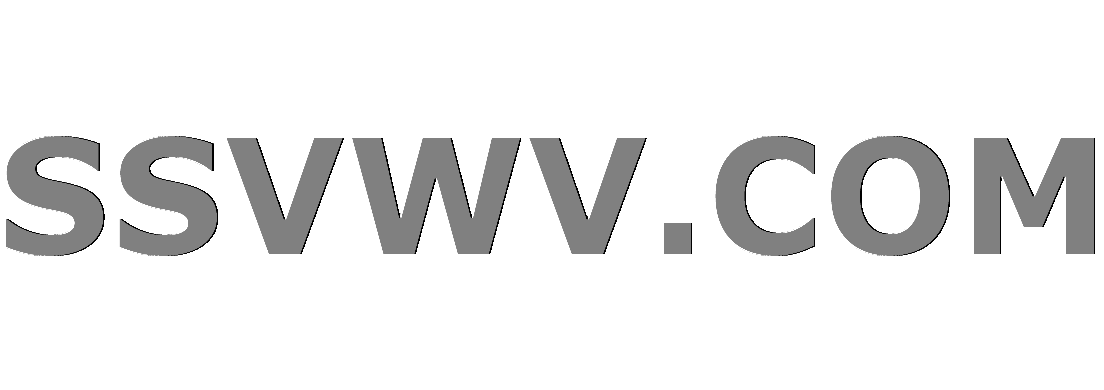
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
for(animal = 0; animal <= animals.length-1; animal++) {
return animals[animal].charAt(animal);
}
});
console.log(secretMessage.join(''));
Hi, thru this piece of code i want to output the string HelloWorld
, which is formed by the first characters of each string/element in the animals array. However, the output is instead HHHHHHHHHH
. I don't know if the for loop is the problem here?
Could someone please tell me why the code produces such an output and how i can modify it in order for the desired result to be returned successfully?
I'm just a novice for now & that's why your help will play a tremendous part in my growth as a programmer. Thanks in advance!
javascript arrays loops
add a comment |
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
for(animal = 0; animal <= animals.length-1; animal++) {
return animals[animal].charAt(animal);
}
});
console.log(secretMessage.join(''));
Hi, thru this piece of code i want to output the string HelloWorld
, which is formed by the first characters of each string/element in the animals array. However, the output is instead HHHHHHHHHH
. I don't know if the for loop is the problem here?
Could someone please tell me why the code produces such an output and how i can modify it in order for the desired result to be returned successfully?
I'm just a novice for now & that's why your help will play a tremendous part in my growth as a programmer. Thanks in advance!
javascript arrays loops
Possible duplicate of How to read first character of string inside an array without using string.charAt()?
– shawon191
Oct 31 '17 at 12:49
animals.map(function(s) { return s[0]; }).join("");
– Yogesh Chuahan
Oct 31 '17 at 13:01
add a comment |
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
for(animal = 0; animal <= animals.length-1; animal++) {
return animals[animal].charAt(animal);
}
});
console.log(secretMessage.join(''));
Hi, thru this piece of code i want to output the string HelloWorld
, which is formed by the first characters of each string/element in the animals array. However, the output is instead HHHHHHHHHH
. I don't know if the for loop is the problem here?
Could someone please tell me why the code produces such an output and how i can modify it in order for the desired result to be returned successfully?
I'm just a novice for now & that's why your help will play a tremendous part in my growth as a programmer. Thanks in advance!
javascript arrays loops
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
for(animal = 0; animal <= animals.length-1; animal++) {
return animals[animal].charAt(animal);
}
});
console.log(secretMessage.join(''));
Hi, thru this piece of code i want to output the string HelloWorld
, which is formed by the first characters of each string/element in the animals array. However, the output is instead HHHHHHHHHH
. I don't know if the for loop is the problem here?
Could someone please tell me why the code produces such an output and how i can modify it in order for the desired result to be returned successfully?
I'm just a novice for now & that's why your help will play a tremendous part in my growth as a programmer. Thanks in advance!
javascript arrays loops
javascript arrays loops
edited Oct 31 '17 at 13:07
Sandeep Sukhija
8311021
8311021
asked Oct 31 '17 at 12:43
jack coltranejack coltrane
1816
1816
Possible duplicate of How to read first character of string inside an array without using string.charAt()?
– shawon191
Oct 31 '17 at 12:49
animals.map(function(s) { return s[0]; }).join("");
– Yogesh Chuahan
Oct 31 '17 at 13:01
add a comment |
Possible duplicate of How to read first character of string inside an array without using string.charAt()?
– shawon191
Oct 31 '17 at 12:49
animals.map(function(s) { return s[0]; }).join("");
– Yogesh Chuahan
Oct 31 '17 at 13:01
Possible duplicate of How to read first character of string inside an array without using string.charAt()?
– shawon191
Oct 31 '17 at 12:49
Possible duplicate of How to read first character of string inside an array without using string.charAt()?
– shawon191
Oct 31 '17 at 12:49
animals.map(function(s) { return s[0]; }).join("");
– Yogesh Chuahan
Oct 31 '17 at 13:01
animals.map(function(s) { return s[0]; }).join("");
– Yogesh Chuahan
Oct 31 '17 at 13:01
add a comment |
8 Answers
8
active
oldest
votes
Map
is a for loop
in and of itself.
Try:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.map((animal) => animal[0]).join('')
// Log.
console.log(secretMessage) // "HelloWorld"
Or alternatively:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.reduce((accumulator, animal) => accumulator + animal[0], '')
// Log.
console.log(secretMessage) // "HelloWorld"
add a comment |
The map method does the for loop for you. So all you need in the function is animal[0]
let secretMessage = animals.map(function(animal) {
return animal[0]
});
This will return every first character in every string in the array, animals. Everything else you have is right.
add a comment |
You don't need inner loop:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0); // or simply animal[0]
});
console.log(secretMessage.join(''));
add a comment |
The error here is that you have a loop inside the map function, which already loops the array.
The correct code is
animals.map(x=>x[0]).join('');
//or
animals.map(function(animal){
return animal[0]; //First letter of word
}).join(''); //Array -> String
add a comment |
This should be the code:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0);
});
console.log(secretMessage.join(''));
add a comment |
Just do this.
let animals = [
'Hen', 'elephant', 'llama',
'leopard', 'ostrich', 'Whale',
'octopus', 'rabbit', 'lion', 'dog'
];
console.log(animals.map(e => e[0]).join(""));
1
For ES5 or less use this: animals.map(function(s) { return s[0]; }).join(""); For ES6: () => { }
– Yogesh Chuahan
Oct 31 '17 at 13:02
add a comment |
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animals) {
return animals[0];
});
console.log(secretMessage.join(''));
or
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(animals => animals[0]);
console.log(secretMessage.join(''));
add a comment |
.map()
array
function take callback function as an argument and return new array so that can use this method to get our result see the syntax below:
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
const secretMessage = animals.map(animals => {
return animals[0];
})
console.log(secretMessage.join(''));
now when .map method is applied on the animals array its return only the first element of the each string because we use animals[0]
which read only first element of each string so our output is HelloWorld
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f47035752%2fgetting-the-first-characters-of-each-string-in-an-array-in-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
8 Answers
8
active
oldest
votes
8 Answers
8
active
oldest
votes
active
oldest
votes
active
oldest
votes
Map
is a for loop
in and of itself.
Try:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.map((animal) => animal[0]).join('')
// Log.
console.log(secretMessage) // "HelloWorld"
Or alternatively:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.reduce((accumulator, animal) => accumulator + animal[0], '')
// Log.
console.log(secretMessage) // "HelloWorld"
add a comment |
Map
is a for loop
in and of itself.
Try:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.map((animal) => animal[0]).join('')
// Log.
console.log(secretMessage) // "HelloWorld"
Or alternatively:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.reduce((accumulator, animal) => accumulator + animal[0], '')
// Log.
console.log(secretMessage) // "HelloWorld"
add a comment |
Map
is a for loop
in and of itself.
Try:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.map((animal) => animal[0]).join('')
// Log.
console.log(secretMessage) // "HelloWorld"
Or alternatively:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.reduce((accumulator, animal) => accumulator + animal[0], '')
// Log.
console.log(secretMessage) // "HelloWorld"
Map
is a for loop
in and of itself.
Try:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.map((animal) => animal[0]).join('')
// Log.
console.log(secretMessage) // "HelloWorld"
Or alternatively:
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.reduce((accumulator, animal) => accumulator + animal[0], '')
// Log.
console.log(secretMessage) // "HelloWorld"
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.map((animal) => animal[0]).join('')
// Log.
console.log(secretMessage) // "HelloWorld"
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.map((animal) => animal[0]).join('')
// Log.
console.log(secretMessage) // "HelloWorld"
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.reduce((accumulator, animal) => accumulator + animal[0], '')
// Log.
console.log(secretMessage) // "HelloWorld"
// Animals.
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog']
// Secret Message.
const secretMessage = animals.reduce((accumulator, animal) => accumulator + animal[0], '')
// Log.
console.log(secretMessage) // "HelloWorld"
edited May 10 '18 at 7:53
answered Oct 31 '17 at 12:47
Arman CharanArman Charan
3,29821121
3,29821121
add a comment |
add a comment |
The map method does the for loop for you. So all you need in the function is animal[0]
let secretMessage = animals.map(function(animal) {
return animal[0]
});
This will return every first character in every string in the array, animals. Everything else you have is right.
add a comment |
The map method does the for loop for you. So all you need in the function is animal[0]
let secretMessage = animals.map(function(animal) {
return animal[0]
});
This will return every first character in every string in the array, animals. Everything else you have is right.
add a comment |
The map method does the for loop for you. So all you need in the function is animal[0]
let secretMessage = animals.map(function(animal) {
return animal[0]
});
This will return every first character in every string in the array, animals. Everything else you have is right.
The map method does the for loop for you. So all you need in the function is animal[0]
let secretMessage = animals.map(function(animal) {
return animal[0]
});
This will return every first character in every string in the array, animals. Everything else you have is right.
answered Oct 31 '17 at 14:19


Joseph CarrickJoseph Carrick
312
312
add a comment |
add a comment |
You don't need inner loop:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0); // or simply animal[0]
});
console.log(secretMessage.join(''));
add a comment |
You don't need inner loop:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0); // or simply animal[0]
});
console.log(secretMessage.join(''));
add a comment |
You don't need inner loop:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0); // or simply animal[0]
});
console.log(secretMessage.join(''));
You don't need inner loop:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0); // or simply animal[0]
});
console.log(secretMessage.join(''));
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0); // or simply animal[0]
});
console.log(secretMessage.join(''));
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0); // or simply animal[0]
});
console.log(secretMessage.join(''));
answered Oct 31 '17 at 12:46
dfsqdfsq
168k21184213
168k21184213
add a comment |
add a comment |
The error here is that you have a loop inside the map function, which already loops the array.
The correct code is
animals.map(x=>x[0]).join('');
//or
animals.map(function(animal){
return animal[0]; //First letter of word
}).join(''); //Array -> String
add a comment |
The error here is that you have a loop inside the map function, which already loops the array.
The correct code is
animals.map(x=>x[0]).join('');
//or
animals.map(function(animal){
return animal[0]; //First letter of word
}).join(''); //Array -> String
add a comment |
The error here is that you have a loop inside the map function, which already loops the array.
The correct code is
animals.map(x=>x[0]).join('');
//or
animals.map(function(animal){
return animal[0]; //First letter of word
}).join(''); //Array -> String
The error here is that you have a loop inside the map function, which already loops the array.
The correct code is
animals.map(x=>x[0]).join('');
//or
animals.map(function(animal){
return animal[0]; //First letter of word
}).join(''); //Array -> String
answered Oct 31 '17 at 12:46


Luca De NardiLuca De Nardi
1,500624
1,500624
add a comment |
add a comment |
This should be the code:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0);
});
console.log(secretMessage.join(''));
add a comment |
This should be the code:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0);
});
console.log(secretMessage.join(''));
add a comment |
This should be the code:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0);
});
console.log(secretMessage.join(''));
This should be the code:
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animal) {
return animal.charAt(0);
});
console.log(secretMessage.join(''));
answered Oct 31 '17 at 12:46


Kenji MukaiKenji Mukai
60148
60148
add a comment |
add a comment |
Just do this.
let animals = [
'Hen', 'elephant', 'llama',
'leopard', 'ostrich', 'Whale',
'octopus', 'rabbit', 'lion', 'dog'
];
console.log(animals.map(e => e[0]).join(""));
1
For ES5 or less use this: animals.map(function(s) { return s[0]; }).join(""); For ES6: () => { }
– Yogesh Chuahan
Oct 31 '17 at 13:02
add a comment |
Just do this.
let animals = [
'Hen', 'elephant', 'llama',
'leopard', 'ostrich', 'Whale',
'octopus', 'rabbit', 'lion', 'dog'
];
console.log(animals.map(e => e[0]).join(""));
1
For ES5 or less use this: animals.map(function(s) { return s[0]; }).join(""); For ES6: () => { }
– Yogesh Chuahan
Oct 31 '17 at 13:02
add a comment |
Just do this.
let animals = [
'Hen', 'elephant', 'llama',
'leopard', 'ostrich', 'Whale',
'octopus', 'rabbit', 'lion', 'dog'
];
console.log(animals.map(e => e[0]).join(""));
Just do this.
let animals = [
'Hen', 'elephant', 'llama',
'leopard', 'ostrich', 'Whale',
'octopus', 'rabbit', 'lion', 'dog'
];
console.log(animals.map(e => e[0]).join(""));
let animals = [
'Hen', 'elephant', 'llama',
'leopard', 'ostrich', 'Whale',
'octopus', 'rabbit', 'lion', 'dog'
];
console.log(animals.map(e => e[0]).join(""));
let animals = [
'Hen', 'elephant', 'llama',
'leopard', 'ostrich', 'Whale',
'octopus', 'rabbit', 'lion', 'dog'
];
console.log(animals.map(e => e[0]).join(""));
edited Oct 31 '17 at 12:52
answered Oct 31 '17 at 12:46


marmeladzemarmeladze
4,39131433
4,39131433
1
For ES5 or less use this: animals.map(function(s) { return s[0]; }).join(""); For ES6: () => { }
– Yogesh Chuahan
Oct 31 '17 at 13:02
add a comment |
1
For ES5 or less use this: animals.map(function(s) { return s[0]; }).join(""); For ES6: () => { }
– Yogesh Chuahan
Oct 31 '17 at 13:02
1
1
For ES5 or less use this: animals.map(function(s) { return s[0]; }).join(""); For ES6: () => { }
– Yogesh Chuahan
Oct 31 '17 at 13:02
For ES5 or less use this: animals.map(function(s) { return s[0]; }).join(""); For ES6: () => { }
– Yogesh Chuahan
Oct 31 '17 at 13:02
add a comment |
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animals) {
return animals[0];
});
console.log(secretMessage.join(''));
or
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(animals => animals[0]);
console.log(secretMessage.join(''));
add a comment |
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animals) {
return animals[0];
});
console.log(secretMessage.join(''));
or
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(animals => animals[0]);
console.log(secretMessage.join(''));
add a comment |
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animals) {
return animals[0];
});
console.log(secretMessage.join(''));
or
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(animals => animals[0]);
console.log(secretMessage.join(''));
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(function(animals) {
return animals[0];
});
console.log(secretMessage.join(''));
or
let animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
let secretMessage = animals.map(animals => animals[0]);
console.log(secretMessage.join(''));
answered Mar 30 '18 at 15:08
Kenny HeenatigalaKenny Heenatigala
12
12
add a comment |
add a comment |
.map()
array
function take callback function as an argument and return new array so that can use this method to get our result see the syntax below:
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
const secretMessage = animals.map(animals => {
return animals[0];
})
console.log(secretMessage.join(''));
now when .map method is applied on the animals array its return only the first element of the each string because we use animals[0]
which read only first element of each string so our output is HelloWorld
add a comment |
.map()
array
function take callback function as an argument and return new array so that can use this method to get our result see the syntax below:
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
const secretMessage = animals.map(animals => {
return animals[0];
})
console.log(secretMessage.join(''));
now when .map method is applied on the animals array its return only the first element of the each string because we use animals[0]
which read only first element of each string so our output is HelloWorld
add a comment |
.map()
array
function take callback function as an argument and return new array so that can use this method to get our result see the syntax below:
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
const secretMessage = animals.map(animals => {
return animals[0];
})
console.log(secretMessage.join(''));
now when .map method is applied on the animals array its return only the first element of the each string because we use animals[0]
which read only first element of each string so our output is HelloWorld
.map()
array
function take callback function as an argument and return new array so that can use this method to get our result see the syntax below:
const animals = ['Hen', 'elephant', 'llama', 'leopard', 'ostrich', 'Whale', 'octopus', 'rabbit', 'lion', 'dog'];
const secretMessage = animals.map(animals => {
return animals[0];
})
console.log(secretMessage.join(''));
now when .map method is applied on the animals array its return only the first element of the each string because we use animals[0]
which read only first element of each string so our output is HelloWorld
edited Nov 23 '18 at 6:13


Ram Ghadiyaram
17.6k84980
17.6k84980
answered Nov 23 '18 at 6:07


Mukesh SutharMukesh Suthar
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f47035752%2fgetting-the-first-characters-of-each-string-in-an-array-in-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
n5XkTLoPsze7y xooMy4y1ronnhE 27Xeqf2 DQpeC6hw1rThADNQFU 3eTuOmy
Possible duplicate of How to read first character of string inside an array without using string.charAt()?
– shawon191
Oct 31 '17 at 12:49
animals.map(function(s) { return s[0]; }).join("");
– Yogesh Chuahan
Oct 31 '17 at 13:01