How to replace parts of a string and a more. Swift 4
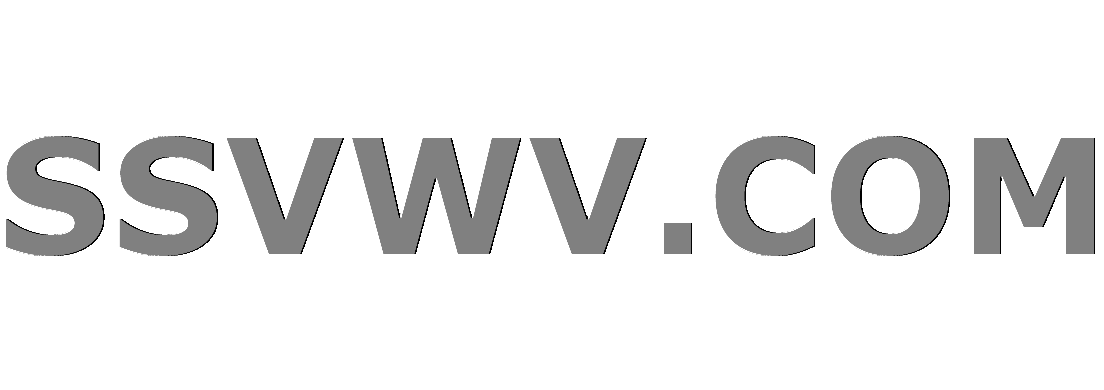
Multi tool use
I have a string:
"Location: <<LesionLoc>><br>
Quality: <<TESTTEST>><br>"
End result should be:
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span>
Quality: <span class="span_dropdowntext" keyword="TESTTEST" style="color:blue;font-weight:bold;"contenteditable="False"> TESTTEST</span>
As you can see, all I have to do is:
Replace
<<
with<span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">
Replace
>>
with</span>
And lastly, (which is what is giving me a hard time), inserting the strings "LesionLoc" and "TESTTEST" into the
keyword=/"/"
part.
Replacing is easy, just use string.replacingOcurences but then I thought it'd be better if I can loop through all the <<
and >>
, replace it inside the loop and also insert the strings into the keyword part.
I'm really lost in this. Someone has mentioned using index and substring functions to return the new string, but I'm not sure how to approach this.
Thanks.
swift string indexing substring
add a comment |
I have a string:
"Location: <<LesionLoc>><br>
Quality: <<TESTTEST>><br>"
End result should be:
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span>
Quality: <span class="span_dropdowntext" keyword="TESTTEST" style="color:blue;font-weight:bold;"contenteditable="False"> TESTTEST</span>
As you can see, all I have to do is:
Replace
<<
with<span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">
Replace
>>
with</span>
And lastly, (which is what is giving me a hard time), inserting the strings "LesionLoc" and "TESTTEST" into the
keyword=/"/"
part.
Replacing is easy, just use string.replacingOcurences but then I thought it'd be better if I can loop through all the <<
and >>
, replace it inside the loop and also insert the strings into the keyword part.
I'm really lost in this. Someone has mentioned using index and substring functions to return the new string, but I'm not sure how to approach this.
Thanks.
swift string indexing substring
add a comment |
I have a string:
"Location: <<LesionLoc>><br>
Quality: <<TESTTEST>><br>"
End result should be:
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span>
Quality: <span class="span_dropdowntext" keyword="TESTTEST" style="color:blue;font-weight:bold;"contenteditable="False"> TESTTEST</span>
As you can see, all I have to do is:
Replace
<<
with<span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">
Replace
>>
with</span>
And lastly, (which is what is giving me a hard time), inserting the strings "LesionLoc" and "TESTTEST" into the
keyword=/"/"
part.
Replacing is easy, just use string.replacingOcurences but then I thought it'd be better if I can loop through all the <<
and >>
, replace it inside the loop and also insert the strings into the keyword part.
I'm really lost in this. Someone has mentioned using index and substring functions to return the new string, but I'm not sure how to approach this.
Thanks.
swift string indexing substring
I have a string:
"Location: <<LesionLoc>><br>
Quality: <<TESTTEST>><br>"
End result should be:
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span>
Quality: <span class="span_dropdowntext" keyword="TESTTEST" style="color:blue;font-weight:bold;"contenteditable="False"> TESTTEST</span>
As you can see, all I have to do is:
Replace
<<
with<span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">
Replace
>>
with</span>
And lastly, (which is what is giving me a hard time), inserting the strings "LesionLoc" and "TESTTEST" into the
keyword=/"/"
part.
Replacing is easy, just use string.replacingOcurences but then I thought it'd be better if I can loop through all the <<
and >>
, replace it inside the loop and also insert the strings into the keyword part.
I'm really lost in this. Someone has mentioned using index and substring functions to return the new string, but I'm not sure how to approach this.
Thanks.
swift string indexing substring
swift string indexing substring
asked Nov 20 '18 at 1:13
alwonggalwongg
164
164
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Firstly, you must find your keyword (in this case is LesionLoc
and TESTTEST
). You can find it by using the NSRegularExpression
with regex format <<[a-z0-9]+>>
Next, replace your <<
with
<span class="span_dropdowntext" keyword="<KEYWORD>"style="color:blue;font-weight:bold;"contenteditable="False">
and >>
with </span>
.
You can using this very simple code
var string = "Location: <<LesionLoc>><br>nQuality: <<TESTTEST>><br>"
do {
let regex = try NSRegularExpression(pattern: "<<[a-z0-9]+>>", options: NSRegularExpression.Options.caseInsensitive)
for text in regex.matches(in: string, options: , range: NSRange(location: 0, length: string.count)).reversed() {
let keyword = (string as NSString).substring(with: text.range).replacingOccurrences(of: "<<", with: "").replacingOccurrences(of: ">>", with: "")
let newString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">(keyword)</span>"
string = (string as NSString).replacingCharacters(in: text.range, with: newString)
}
print(string)
} catch {
print(error.localizedDescription)
}
1
Works great! Thank you very much!
– alwongg
Nov 20 '18 at 14:31
add a comment |
private func replaceString(_ str : String)->String{
guard let keyword = findKeyword(str) else{
fatalError()
}
let replacementString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">"
var newString = str.replacingOccurrences(of: ">>", with: "</span>")
newString = newString.replacingOccurrences(of: "<<", with: replacementString)
return newString
}
private func findKeyword(_ s : String)->String?{
var str = Array(s)
let firstKey = "<<"
let secondKey = ">>"
var startingIndex : Int = 0
var movingIndex : Int = 0
while movingIndex < str.count + 1 - secondKey.count && startingIndex < str.count + 1 - firstKey.count{
if String(str[startingIndex..<startingIndex + firstKey.count]) != firstKey{
startingIndex += 1
}else{
if movingIndex < startingIndex + firstKey.count{
movingIndex = startingIndex + firstKey.count
}else{
if String(str[movingIndex..<movingIndex + secondKey.count]) != secondKey{
movingIndex += 1
}else{
return String(str[startingIndex+firstKey.count..<movingIndex])
}
}
}
}
return nil
}
Now, you can use the replaceString function to do the work
let string = "Location: <<LesionLoc>><br>"
print(replaceString(string))
your end result will be
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span><br>
There is a "br" at the end of the result string. I don't know if you wanna to remove that or not.. But if you do, you should know how to .
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384861%2fhow-to-replace-parts-of-a-string-and-a-more-swift-4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Firstly, you must find your keyword (in this case is LesionLoc
and TESTTEST
). You can find it by using the NSRegularExpression
with regex format <<[a-z0-9]+>>
Next, replace your <<
with
<span class="span_dropdowntext" keyword="<KEYWORD>"style="color:blue;font-weight:bold;"contenteditable="False">
and >>
with </span>
.
You can using this very simple code
var string = "Location: <<LesionLoc>><br>nQuality: <<TESTTEST>><br>"
do {
let regex = try NSRegularExpression(pattern: "<<[a-z0-9]+>>", options: NSRegularExpression.Options.caseInsensitive)
for text in regex.matches(in: string, options: , range: NSRange(location: 0, length: string.count)).reversed() {
let keyword = (string as NSString).substring(with: text.range).replacingOccurrences(of: "<<", with: "").replacingOccurrences(of: ">>", with: "")
let newString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">(keyword)</span>"
string = (string as NSString).replacingCharacters(in: text.range, with: newString)
}
print(string)
} catch {
print(error.localizedDescription)
}
1
Works great! Thank you very much!
– alwongg
Nov 20 '18 at 14:31
add a comment |
Firstly, you must find your keyword (in this case is LesionLoc
and TESTTEST
). You can find it by using the NSRegularExpression
with regex format <<[a-z0-9]+>>
Next, replace your <<
with
<span class="span_dropdowntext" keyword="<KEYWORD>"style="color:blue;font-weight:bold;"contenteditable="False">
and >>
with </span>
.
You can using this very simple code
var string = "Location: <<LesionLoc>><br>nQuality: <<TESTTEST>><br>"
do {
let regex = try NSRegularExpression(pattern: "<<[a-z0-9]+>>", options: NSRegularExpression.Options.caseInsensitive)
for text in regex.matches(in: string, options: , range: NSRange(location: 0, length: string.count)).reversed() {
let keyword = (string as NSString).substring(with: text.range).replacingOccurrences(of: "<<", with: "").replacingOccurrences(of: ">>", with: "")
let newString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">(keyword)</span>"
string = (string as NSString).replacingCharacters(in: text.range, with: newString)
}
print(string)
} catch {
print(error.localizedDescription)
}
1
Works great! Thank you very much!
– alwongg
Nov 20 '18 at 14:31
add a comment |
Firstly, you must find your keyword (in this case is LesionLoc
and TESTTEST
). You can find it by using the NSRegularExpression
with regex format <<[a-z0-9]+>>
Next, replace your <<
with
<span class="span_dropdowntext" keyword="<KEYWORD>"style="color:blue;font-weight:bold;"contenteditable="False">
and >>
with </span>
.
You can using this very simple code
var string = "Location: <<LesionLoc>><br>nQuality: <<TESTTEST>><br>"
do {
let regex = try NSRegularExpression(pattern: "<<[a-z0-9]+>>", options: NSRegularExpression.Options.caseInsensitive)
for text in regex.matches(in: string, options: , range: NSRange(location: 0, length: string.count)).reversed() {
let keyword = (string as NSString).substring(with: text.range).replacingOccurrences(of: "<<", with: "").replacingOccurrences(of: ">>", with: "")
let newString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">(keyword)</span>"
string = (string as NSString).replacingCharacters(in: text.range, with: newString)
}
print(string)
} catch {
print(error.localizedDescription)
}
Firstly, you must find your keyword (in this case is LesionLoc
and TESTTEST
). You can find it by using the NSRegularExpression
with regex format <<[a-z0-9]+>>
Next, replace your <<
with
<span class="span_dropdowntext" keyword="<KEYWORD>"style="color:blue;font-weight:bold;"contenteditable="False">
and >>
with </span>
.
You can using this very simple code
var string = "Location: <<LesionLoc>><br>nQuality: <<TESTTEST>><br>"
do {
let regex = try NSRegularExpression(pattern: "<<[a-z0-9]+>>", options: NSRegularExpression.Options.caseInsensitive)
for text in regex.matches(in: string, options: , range: NSRange(location: 0, length: string.count)).reversed() {
let keyword = (string as NSString).substring(with: text.range).replacingOccurrences(of: "<<", with: "").replacingOccurrences(of: ">>", with: "")
let newString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">(keyword)</span>"
string = (string as NSString).replacingCharacters(in: text.range, with: newString)
}
print(string)
} catch {
print(error.localizedDescription)
}
answered Nov 20 '18 at 2:15
Quoc NguyenQuoc Nguyen
1,66851420
1,66851420
1
Works great! Thank you very much!
– alwongg
Nov 20 '18 at 14:31
add a comment |
1
Works great! Thank you very much!
– alwongg
Nov 20 '18 at 14:31
1
1
Works great! Thank you very much!
– alwongg
Nov 20 '18 at 14:31
Works great! Thank you very much!
– alwongg
Nov 20 '18 at 14:31
add a comment |
private func replaceString(_ str : String)->String{
guard let keyword = findKeyword(str) else{
fatalError()
}
let replacementString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">"
var newString = str.replacingOccurrences(of: ">>", with: "</span>")
newString = newString.replacingOccurrences(of: "<<", with: replacementString)
return newString
}
private func findKeyword(_ s : String)->String?{
var str = Array(s)
let firstKey = "<<"
let secondKey = ">>"
var startingIndex : Int = 0
var movingIndex : Int = 0
while movingIndex < str.count + 1 - secondKey.count && startingIndex < str.count + 1 - firstKey.count{
if String(str[startingIndex..<startingIndex + firstKey.count]) != firstKey{
startingIndex += 1
}else{
if movingIndex < startingIndex + firstKey.count{
movingIndex = startingIndex + firstKey.count
}else{
if String(str[movingIndex..<movingIndex + secondKey.count]) != secondKey{
movingIndex += 1
}else{
return String(str[startingIndex+firstKey.count..<movingIndex])
}
}
}
}
return nil
}
Now, you can use the replaceString function to do the work
let string = "Location: <<LesionLoc>><br>"
print(replaceString(string))
your end result will be
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span><br>
There is a "br" at the end of the result string. I don't know if you wanna to remove that or not.. But if you do, you should know how to .
add a comment |
private func replaceString(_ str : String)->String{
guard let keyword = findKeyword(str) else{
fatalError()
}
let replacementString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">"
var newString = str.replacingOccurrences(of: ">>", with: "</span>")
newString = newString.replacingOccurrences(of: "<<", with: replacementString)
return newString
}
private func findKeyword(_ s : String)->String?{
var str = Array(s)
let firstKey = "<<"
let secondKey = ">>"
var startingIndex : Int = 0
var movingIndex : Int = 0
while movingIndex < str.count + 1 - secondKey.count && startingIndex < str.count + 1 - firstKey.count{
if String(str[startingIndex..<startingIndex + firstKey.count]) != firstKey{
startingIndex += 1
}else{
if movingIndex < startingIndex + firstKey.count{
movingIndex = startingIndex + firstKey.count
}else{
if String(str[movingIndex..<movingIndex + secondKey.count]) != secondKey{
movingIndex += 1
}else{
return String(str[startingIndex+firstKey.count..<movingIndex])
}
}
}
}
return nil
}
Now, you can use the replaceString function to do the work
let string = "Location: <<LesionLoc>><br>"
print(replaceString(string))
your end result will be
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span><br>
There is a "br" at the end of the result string. I don't know if you wanna to remove that or not.. But if you do, you should know how to .
add a comment |
private func replaceString(_ str : String)->String{
guard let keyword = findKeyword(str) else{
fatalError()
}
let replacementString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">"
var newString = str.replacingOccurrences(of: ">>", with: "</span>")
newString = newString.replacingOccurrences(of: "<<", with: replacementString)
return newString
}
private func findKeyword(_ s : String)->String?{
var str = Array(s)
let firstKey = "<<"
let secondKey = ">>"
var startingIndex : Int = 0
var movingIndex : Int = 0
while movingIndex < str.count + 1 - secondKey.count && startingIndex < str.count + 1 - firstKey.count{
if String(str[startingIndex..<startingIndex + firstKey.count]) != firstKey{
startingIndex += 1
}else{
if movingIndex < startingIndex + firstKey.count{
movingIndex = startingIndex + firstKey.count
}else{
if String(str[movingIndex..<movingIndex + secondKey.count]) != secondKey{
movingIndex += 1
}else{
return String(str[startingIndex+firstKey.count..<movingIndex])
}
}
}
}
return nil
}
Now, you can use the replaceString function to do the work
let string = "Location: <<LesionLoc>><br>"
print(replaceString(string))
your end result will be
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span><br>
There is a "br" at the end of the result string. I don't know if you wanna to remove that or not.. But if you do, you should know how to .
private func replaceString(_ str : String)->String{
guard let keyword = findKeyword(str) else{
fatalError()
}
let replacementString = "<span class="span_dropdowntext" keyword="(keyword)" style="color:blue;font-weight:bold;"contenteditable="False">"
var newString = str.replacingOccurrences(of: ">>", with: "</span>")
newString = newString.replacingOccurrences(of: "<<", with: replacementString)
return newString
}
private func findKeyword(_ s : String)->String?{
var str = Array(s)
let firstKey = "<<"
let secondKey = ">>"
var startingIndex : Int = 0
var movingIndex : Int = 0
while movingIndex < str.count + 1 - secondKey.count && startingIndex < str.count + 1 - firstKey.count{
if String(str[startingIndex..<startingIndex + firstKey.count]) != firstKey{
startingIndex += 1
}else{
if movingIndex < startingIndex + firstKey.count{
movingIndex = startingIndex + firstKey.count
}else{
if String(str[movingIndex..<movingIndex + secondKey.count]) != secondKey{
movingIndex += 1
}else{
return String(str[startingIndex+firstKey.count..<movingIndex])
}
}
}
}
return nil
}
Now, you can use the replaceString function to do the work
let string = "Location: <<LesionLoc>><br>"
print(replaceString(string))
your end result will be
Location: <span class="span_dropdowntext" keyword="LesionLoc" style="color:blue;font-weight:bold;"contenteditable="False">LesionLoc</span><br>
There is a "br" at the end of the result string. I don't know if you wanna to remove that or not.. But if you do, you should know how to .
answered Nov 20 '18 at 2:38
progammingBeignnerprogammingBeignner
3391315
3391315
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384861%2fhow-to-replace-parts-of-a-string-and-a-more-swift-4%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
m,OnM Z gD4V RZ,j7QDZz0G,qk,dAUS,vL