Overload a class constructor that takes an rvalue reference
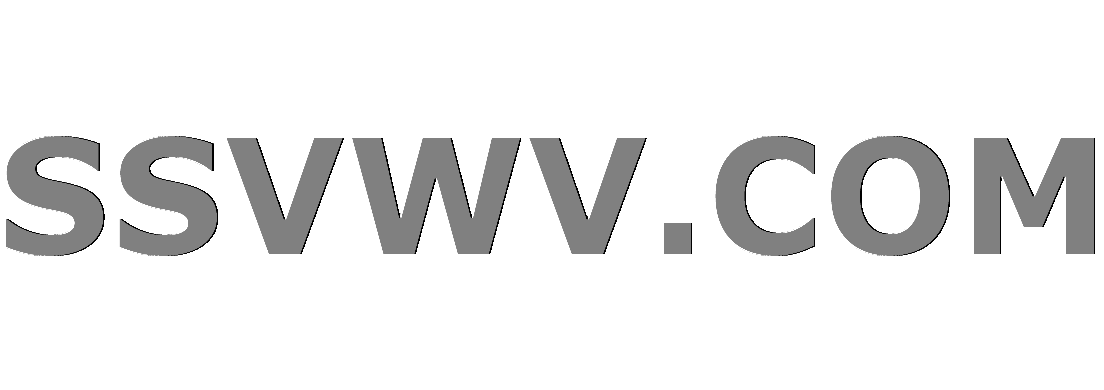
Multi tool use
I would like to do something like the following:
class Foo
{
Foo(int &&a, int b, std::string s="");
// does not compile because a is not an rvalue:
// Foo(int &&a, std::string s) : Foo(a, 0, s) {}
Foo(int &&a, std::string s) : Foo(std::move(a), 0, s) {} // move a
}
- Is this a valid way to overload a constructor in general?
- And specifically one that takes an rvalue reference as a parameter?
Edited based on comments
To clarify, I'm new to move semantics (and an amateur programmer) and I'm simply not sure if this is a good way to handle this situation.
I added the first question based on a comment (now deleted) that suggested this is not the correct way to overload constructors.
c++ move-semantics rvalue-reference constructor-overloading stdmove
add a comment |
I would like to do something like the following:
class Foo
{
Foo(int &&a, int b, std::string s="");
// does not compile because a is not an rvalue:
// Foo(int &&a, std::string s) : Foo(a, 0, s) {}
Foo(int &&a, std::string s) : Foo(std::move(a), 0, s) {} // move a
}
- Is this a valid way to overload a constructor in general?
- And specifically one that takes an rvalue reference as a parameter?
Edited based on comments
To clarify, I'm new to move semantics (and an amateur programmer) and I'm simply not sure if this is a good way to handle this situation.
I added the first question based on a comment (now deleted) that suggested this is not the correct way to overload constructors.
c++ move-semantics rvalue-reference constructor-overloading stdmove
2
Why don't you want to usestd::move
? What do you mean by "better"?
– Rakete1111
Nov 17 '18 at 17:59
2
If it did compile, the default argument would make which constructor to call ambiguous.
– Neil Butterworth
Nov 17 '18 at 18:01
2
As you already recognizedstd::move
is the right way to do it.
– Werner Henze
Nov 17 '18 at 18:01
Thanks for your comments. I've edited the question accordingly. @Ripi2 I'll look into what is.
– bur
Nov 17 '18 at 18:07
add a comment |
I would like to do something like the following:
class Foo
{
Foo(int &&a, int b, std::string s="");
// does not compile because a is not an rvalue:
// Foo(int &&a, std::string s) : Foo(a, 0, s) {}
Foo(int &&a, std::string s) : Foo(std::move(a), 0, s) {} // move a
}
- Is this a valid way to overload a constructor in general?
- And specifically one that takes an rvalue reference as a parameter?
Edited based on comments
To clarify, I'm new to move semantics (and an amateur programmer) and I'm simply not sure if this is a good way to handle this situation.
I added the first question based on a comment (now deleted) that suggested this is not the correct way to overload constructors.
c++ move-semantics rvalue-reference constructor-overloading stdmove
I would like to do something like the following:
class Foo
{
Foo(int &&a, int b, std::string s="");
// does not compile because a is not an rvalue:
// Foo(int &&a, std::string s) : Foo(a, 0, s) {}
Foo(int &&a, std::string s) : Foo(std::move(a), 0, s) {} // move a
}
- Is this a valid way to overload a constructor in general?
- And specifically one that takes an rvalue reference as a parameter?
Edited based on comments
To clarify, I'm new to move semantics (and an amateur programmer) and I'm simply not sure if this is a good way to handle this situation.
I added the first question based on a comment (now deleted) that suggested this is not the correct way to overload constructors.
c++ move-semantics rvalue-reference constructor-overloading stdmove
c++ move-semantics rvalue-reference constructor-overloading stdmove
edited Nov 17 '18 at 18:33
asked Nov 17 '18 at 17:57
bur
17713
17713
2
Why don't you want to usestd::move
? What do you mean by "better"?
– Rakete1111
Nov 17 '18 at 17:59
2
If it did compile, the default argument would make which constructor to call ambiguous.
– Neil Butterworth
Nov 17 '18 at 18:01
2
As you already recognizedstd::move
is the right way to do it.
– Werner Henze
Nov 17 '18 at 18:01
Thanks for your comments. I've edited the question accordingly. @Ripi2 I'll look into what is.
– bur
Nov 17 '18 at 18:07
add a comment |
2
Why don't you want to usestd::move
? What do you mean by "better"?
– Rakete1111
Nov 17 '18 at 17:59
2
If it did compile, the default argument would make which constructor to call ambiguous.
– Neil Butterworth
Nov 17 '18 at 18:01
2
As you already recognizedstd::move
is the right way to do it.
– Werner Henze
Nov 17 '18 at 18:01
Thanks for your comments. I've edited the question accordingly. @Ripi2 I'll look into what is.
– bur
Nov 17 '18 at 18:07
2
2
Why don't you want to use
std::move
? What do you mean by "better"?– Rakete1111
Nov 17 '18 at 17:59
Why don't you want to use
std::move
? What do you mean by "better"?– Rakete1111
Nov 17 '18 at 17:59
2
2
If it did compile, the default argument would make which constructor to call ambiguous.
– Neil Butterworth
Nov 17 '18 at 18:01
If it did compile, the default argument would make which constructor to call ambiguous.
– Neil Butterworth
Nov 17 '18 at 18:01
2
2
As you already recognized
std::move
is the right way to do it.– Werner Henze
Nov 17 '18 at 18:01
As you already recognized
std::move
is the right way to do it.– Werner Henze
Nov 17 '18 at 18:01
Thanks for your comments. I've edited the question accordingly. @Ripi2 I'll look into what is.
– bur
Nov 17 '18 at 18:07
Thanks for your comments. I've edited the question accordingly. @Ripi2 I'll look into what is.
– bur
Nov 17 '18 at 18:07
add a comment |
1 Answer
1
active
oldest
votes
Is this a valid way to overload a constructor in general?
And specifically one that takes an rvalue reference as a parameter?
It is valid to overload constructors in general, even ones that have rvalue reference arguments.
In particular, your example is ill-formed. As you point out in the comments, the example fails to compile. To fix it, you must pass an rvalue to the constructor that you delegate to. The correct way to convert a rvalue reference variable into an rvalue is to use std::move
. So, what you must do is what you already know:
The only option I can see is to
std::move(a)
.
To future readers: I edited the question before I saw this answer. Thanks, this answers my second question, and I can infer that the answer to my first question is yes.
– bur
Nov 17 '18 at 18:19
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53353971%2foverload-a-class-constructor-that-takes-an-rvalue-reference%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Is this a valid way to overload a constructor in general?
And specifically one that takes an rvalue reference as a parameter?
It is valid to overload constructors in general, even ones that have rvalue reference arguments.
In particular, your example is ill-formed. As you point out in the comments, the example fails to compile. To fix it, you must pass an rvalue to the constructor that you delegate to. The correct way to convert a rvalue reference variable into an rvalue is to use std::move
. So, what you must do is what you already know:
The only option I can see is to
std::move(a)
.
To future readers: I edited the question before I saw this answer. Thanks, this answers my second question, and I can infer that the answer to my first question is yes.
– bur
Nov 17 '18 at 18:19
add a comment |
Is this a valid way to overload a constructor in general?
And specifically one that takes an rvalue reference as a parameter?
It is valid to overload constructors in general, even ones that have rvalue reference arguments.
In particular, your example is ill-formed. As you point out in the comments, the example fails to compile. To fix it, you must pass an rvalue to the constructor that you delegate to. The correct way to convert a rvalue reference variable into an rvalue is to use std::move
. So, what you must do is what you already know:
The only option I can see is to
std::move(a)
.
To future readers: I edited the question before I saw this answer. Thanks, this answers my second question, and I can infer that the answer to my first question is yes.
– bur
Nov 17 '18 at 18:19
add a comment |
Is this a valid way to overload a constructor in general?
And specifically one that takes an rvalue reference as a parameter?
It is valid to overload constructors in general, even ones that have rvalue reference arguments.
In particular, your example is ill-formed. As you point out in the comments, the example fails to compile. To fix it, you must pass an rvalue to the constructor that you delegate to. The correct way to convert a rvalue reference variable into an rvalue is to use std::move
. So, what you must do is what you already know:
The only option I can see is to
std::move(a)
.
Is this a valid way to overload a constructor in general?
And specifically one that takes an rvalue reference as a parameter?
It is valid to overload constructors in general, even ones that have rvalue reference arguments.
In particular, your example is ill-formed. As you point out in the comments, the example fails to compile. To fix it, you must pass an rvalue to the constructor that you delegate to. The correct way to convert a rvalue reference variable into an rvalue is to use std::move
. So, what you must do is what you already know:
The only option I can see is to
std::move(a)
.
answered Nov 17 '18 at 18:12
eerorika
76.9k556117
76.9k556117
To future readers: I edited the question before I saw this answer. Thanks, this answers my second question, and I can infer that the answer to my first question is yes.
– bur
Nov 17 '18 at 18:19
add a comment |
To future readers: I edited the question before I saw this answer. Thanks, this answers my second question, and I can infer that the answer to my first question is yes.
– bur
Nov 17 '18 at 18:19
To future readers: I edited the question before I saw this answer. Thanks, this answers my second question, and I can infer that the answer to my first question is yes.
– bur
Nov 17 '18 at 18:19
To future readers: I edited the question before I saw this answer. Thanks, this answers my second question, and I can infer that the answer to my first question is yes.
– bur
Nov 17 '18 at 18:19
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53353971%2foverload-a-class-constructor-that-takes-an-rvalue-reference%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
q45QT0omg8 ewsy4opgyONXuqu EGL tuJSNKGQAfoZennILqlaMzf i
2
Why don't you want to use
std::move
? What do you mean by "better"?– Rakete1111
Nov 17 '18 at 17:59
2
If it did compile, the default argument would make which constructor to call ambiguous.
– Neil Butterworth
Nov 17 '18 at 18:01
2
As you already recognized
std::move
is the right way to do it.– Werner Henze
Nov 17 '18 at 18:01
Thanks for your comments. I've edited the question accordingly. @Ripi2 I'll look into what is.
– bur
Nov 17 '18 at 18:07