How to check if object is an array of a certain type?
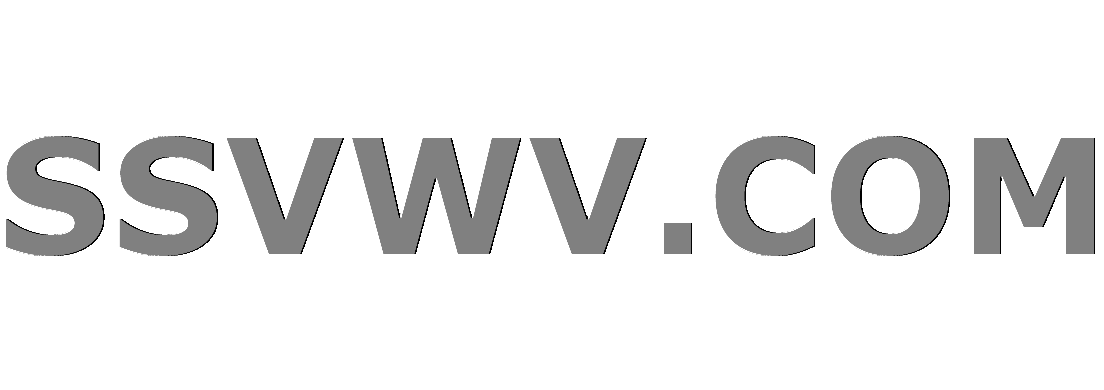
Multi tool use
This works fine:
var expectedType = typeof(string);
object value = "...";
if (value.GetType().IsAssignableFrom(expectedType))
{
...
}
But how do I check if value is a string array without setting expectedType
to typeof(string)
? I want to do something like:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType().IsArrayOf(expectedType)) // <---
{
...
}
Is this possible?
c# .net arrays reflection types
add a comment |
This works fine:
var expectedType = typeof(string);
object value = "...";
if (value.GetType().IsAssignableFrom(expectedType))
{
...
}
But how do I check if value is a string array without setting expectedType
to typeof(string)
? I want to do something like:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType().IsArrayOf(expectedType)) // <---
{
...
}
Is this possible?
c# .net arrays reflection types
1
Do you want to know if the object was declared as a string. or if an object contains only instances of a certain type?
– Chris Marasti-Georg
Mar 11 '11 at 15:34
add a comment |
This works fine:
var expectedType = typeof(string);
object value = "...";
if (value.GetType().IsAssignableFrom(expectedType))
{
...
}
But how do I check if value is a string array without setting expectedType
to typeof(string)
? I want to do something like:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType().IsArrayOf(expectedType)) // <---
{
...
}
Is this possible?
c# .net arrays reflection types
This works fine:
var expectedType = typeof(string);
object value = "...";
if (value.GetType().IsAssignableFrom(expectedType))
{
...
}
But how do I check if value is a string array without setting expectedType
to typeof(string)
? I want to do something like:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType().IsArrayOf(expectedType)) // <---
{
...
}
Is this possible?
c# .net arrays reflection types
c# .net arrays reflection types
edited Mar 11 '11 at 15:32


Oded
409k70744910
409k70744910
asked Mar 11 '11 at 15:30
AllrameestAllrameest
2,30912446
2,30912446
1
Do you want to know if the object was declared as a string. or if an object contains only instances of a certain type?
– Chris Marasti-Georg
Mar 11 '11 at 15:34
add a comment |
1
Do you want to know if the object was declared as a string. or if an object contains only instances of a certain type?
– Chris Marasti-Georg
Mar 11 '11 at 15:34
1
1
Do you want to know if the object was declared as a string. or if an object contains only instances of a certain type?
– Chris Marasti-Georg
Mar 11 '11 at 15:34
Do you want to know if the object was declared as a string. or if an object contains only instances of a certain type?
– Chris Marasti-Georg
Mar 11 '11 at 15:34
add a comment |
6 Answers
6
active
oldest
votes
Use Type.IsArray and Type.GetElementType() to check the element type of an array.
Type valueType = value.GetType();
if (valueType.IsArray && expectedType.IsAssignableFrom(valueType.GetElementType())
{
...
}
Beware the Type.IsAssignableFrom(). If you want to check the type for an exact match you should check for equality (typeA == typeB
). If you want to check if a given type is the type itself or a subclass (or an interface) then you should use Type.IsAssignableFrom()
:
typeof(BaseClass).IsAssignableFrom(typeof(ExpectedSubclass))
add a comment |
You can use extension methods (not that you have to but makes it more readable):
public static class TypeExtensions
{
public static bool IsArrayOf<T>(this Type type)
{
return type == typeof (T);
}
}
And then use:
Console.WriteLine(new string[0].GetType().IsArrayOf<string>());
add a comment |
The neatest and securest way to do it that found is using MakeArrayType
:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType() == expectedType.MakeArrayType())
{
...
}
add a comment |
value.GetType().GetElementType() == typeof(string)
as an added bonus (but I'm not 100% sure. This is the code I use...)
var ienum = value.GetType().GetInterface("IEnumerable`1");
if (ienum != null) {
var baseTypeForIEnum = ienum.GetGenericArguments()[0]
}
with this you can look for List, IEnumerable... and get the T.
17
That's one risky magic string right there
– Kieren Johnstone
Nov 17 '12 at 11:16
add a comment |
Do you actually need to know the type of the array? Or do you only need the elements to be of a certain type?
If the latter, you can simply filter only the elements that match what you want:
string strings = array.OfType<string>();
add a comment |
if(objVal.GetType().Name == "Object")
true for array
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f5274865%2fhow-to-check-if-object-is-an-array-of-a-certain-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
6 Answers
6
active
oldest
votes
6 Answers
6
active
oldest
votes
active
oldest
votes
active
oldest
votes
Use Type.IsArray and Type.GetElementType() to check the element type of an array.
Type valueType = value.GetType();
if (valueType.IsArray && expectedType.IsAssignableFrom(valueType.GetElementType())
{
...
}
Beware the Type.IsAssignableFrom(). If you want to check the type for an exact match you should check for equality (typeA == typeB
). If you want to check if a given type is the type itself or a subclass (or an interface) then you should use Type.IsAssignableFrom()
:
typeof(BaseClass).IsAssignableFrom(typeof(ExpectedSubclass))
add a comment |
Use Type.IsArray and Type.GetElementType() to check the element type of an array.
Type valueType = value.GetType();
if (valueType.IsArray && expectedType.IsAssignableFrom(valueType.GetElementType())
{
...
}
Beware the Type.IsAssignableFrom(). If you want to check the type for an exact match you should check for equality (typeA == typeB
). If you want to check if a given type is the type itself or a subclass (or an interface) then you should use Type.IsAssignableFrom()
:
typeof(BaseClass).IsAssignableFrom(typeof(ExpectedSubclass))
add a comment |
Use Type.IsArray and Type.GetElementType() to check the element type of an array.
Type valueType = value.GetType();
if (valueType.IsArray && expectedType.IsAssignableFrom(valueType.GetElementType())
{
...
}
Beware the Type.IsAssignableFrom(). If you want to check the type for an exact match you should check for equality (typeA == typeB
). If you want to check if a given type is the type itself or a subclass (or an interface) then you should use Type.IsAssignableFrom()
:
typeof(BaseClass).IsAssignableFrom(typeof(ExpectedSubclass))
Use Type.IsArray and Type.GetElementType() to check the element type of an array.
Type valueType = value.GetType();
if (valueType.IsArray && expectedType.IsAssignableFrom(valueType.GetElementType())
{
...
}
Beware the Type.IsAssignableFrom(). If you want to check the type for an exact match you should check for equality (typeA == typeB
). If you want to check if a given type is the type itself or a subclass (or an interface) then you should use Type.IsAssignableFrom()
:
typeof(BaseClass).IsAssignableFrom(typeof(ExpectedSubclass))
edited Mar 11 '11 at 15:48
answered Mar 11 '11 at 15:34
StefanStefan
12.4k14755
12.4k14755
add a comment |
add a comment |
You can use extension methods (not that you have to but makes it more readable):
public static class TypeExtensions
{
public static bool IsArrayOf<T>(this Type type)
{
return type == typeof (T);
}
}
And then use:
Console.WriteLine(new string[0].GetType().IsArrayOf<string>());
add a comment |
You can use extension methods (not that you have to but makes it more readable):
public static class TypeExtensions
{
public static bool IsArrayOf<T>(this Type type)
{
return type == typeof (T);
}
}
And then use:
Console.WriteLine(new string[0].GetType().IsArrayOf<string>());
add a comment |
You can use extension methods (not that you have to but makes it more readable):
public static class TypeExtensions
{
public static bool IsArrayOf<T>(this Type type)
{
return type == typeof (T);
}
}
And then use:
Console.WriteLine(new string[0].GetType().IsArrayOf<string>());
You can use extension methods (not that you have to but makes it more readable):
public static class TypeExtensions
{
public static bool IsArrayOf<T>(this Type type)
{
return type == typeof (T);
}
}
And then use:
Console.WriteLine(new string[0].GetType().IsArrayOf<string>());
answered Mar 11 '11 at 15:37
AliostadAliostad
69.2k13134190
69.2k13134190
add a comment |
add a comment |
The neatest and securest way to do it that found is using MakeArrayType
:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType() == expectedType.MakeArrayType())
{
...
}
add a comment |
The neatest and securest way to do it that found is using MakeArrayType
:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType() == expectedType.MakeArrayType())
{
...
}
add a comment |
The neatest and securest way to do it that found is using MakeArrayType
:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType() == expectedType.MakeArrayType())
{
...
}
The neatest and securest way to do it that found is using MakeArrayType
:
var expectedType = typeof(string);
object value = new {"...", "---"};
if (value.GetType() == expectedType.MakeArrayType())
{
...
}
edited Apr 29 '16 at 9:14
answered Dec 19 '12 at 16:56
NatxoNatxo
2,49511832
2,49511832
add a comment |
add a comment |
value.GetType().GetElementType() == typeof(string)
as an added bonus (but I'm not 100% sure. This is the code I use...)
var ienum = value.GetType().GetInterface("IEnumerable`1");
if (ienum != null) {
var baseTypeForIEnum = ienum.GetGenericArguments()[0]
}
with this you can look for List, IEnumerable... and get the T.
17
That's one risky magic string right there
– Kieren Johnstone
Nov 17 '12 at 11:16
add a comment |
value.GetType().GetElementType() == typeof(string)
as an added bonus (but I'm not 100% sure. This is the code I use...)
var ienum = value.GetType().GetInterface("IEnumerable`1");
if (ienum != null) {
var baseTypeForIEnum = ienum.GetGenericArguments()[0]
}
with this you can look for List, IEnumerable... and get the T.
17
That's one risky magic string right there
– Kieren Johnstone
Nov 17 '12 at 11:16
add a comment |
value.GetType().GetElementType() == typeof(string)
as an added bonus (but I'm not 100% sure. This is the code I use...)
var ienum = value.GetType().GetInterface("IEnumerable`1");
if (ienum != null) {
var baseTypeForIEnum = ienum.GetGenericArguments()[0]
}
with this you can look for List, IEnumerable... and get the T.
value.GetType().GetElementType() == typeof(string)
as an added bonus (but I'm not 100% sure. This is the code I use...)
var ienum = value.GetType().GetInterface("IEnumerable`1");
if (ienum != null) {
var baseTypeForIEnum = ienum.GetGenericArguments()[0]
}
with this you can look for List, IEnumerable... and get the T.
edited Mar 11 '11 at 15:46
answered Mar 11 '11 at 15:38
xanatosxanatos
88.9k7140196
88.9k7140196
17
That's one risky magic string right there
– Kieren Johnstone
Nov 17 '12 at 11:16
add a comment |
17
That's one risky magic string right there
– Kieren Johnstone
Nov 17 '12 at 11:16
17
17
That's one risky magic string right there
– Kieren Johnstone
Nov 17 '12 at 11:16
That's one risky magic string right there
– Kieren Johnstone
Nov 17 '12 at 11:16
add a comment |
Do you actually need to know the type of the array? Or do you only need the elements to be of a certain type?
If the latter, you can simply filter only the elements that match what you want:
string strings = array.OfType<string>();
add a comment |
Do you actually need to know the type of the array? Or do you only need the elements to be of a certain type?
If the latter, you can simply filter only the elements that match what you want:
string strings = array.OfType<string>();
add a comment |
Do you actually need to know the type of the array? Or do you only need the elements to be of a certain type?
If the latter, you can simply filter only the elements that match what you want:
string strings = array.OfType<string>();
Do you actually need to know the type of the array? Or do you only need the elements to be of a certain type?
If the latter, you can simply filter only the elements that match what you want:
string strings = array.OfType<string>();
answered Mar 11 '11 at 15:36
jdmichaljdmichal
9,36533437
9,36533437
add a comment |
add a comment |
if(objVal.GetType().Name == "Object")
true for array
add a comment |
if(objVal.GetType().Name == "Object")
true for array
add a comment |
if(objVal.GetType().Name == "Object")
true for array
if(objVal.GetType().Name == "Object")
true for array
answered Apr 19 '18 at 17:49
snbsnb
16518
16518
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f5274865%2fhow-to-check-if-object-is-an-array-of-a-certain-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uK3VpT3 SdVIcR,KRFC845fK8 M0X,0ZvE7GukOeak14
1
Do you want to know if the object was declared as a string. or if an object contains only instances of a certain type?
– Chris Marasti-Georg
Mar 11 '11 at 15:34