How can I change ascii string to hex and vice versa in python 3.7?
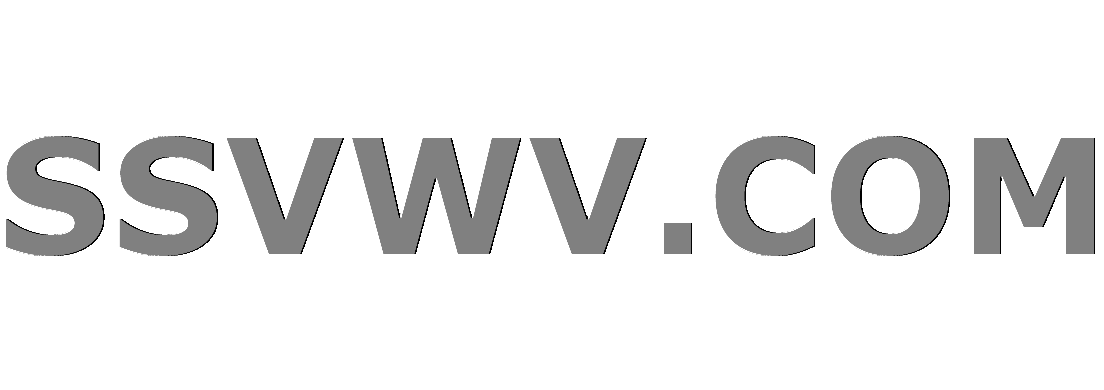
Multi tool use
I look some solution in this site but those not works in python 3.7.
So, I asked a new question.
Hex string of "the" is "746865"
I want to a solution to convert "the" to "746865" and "746865" to "the"
data-conversion python-3.7
add a comment |
I look some solution in this site but those not works in python 3.7.
So, I asked a new question.
Hex string of "the" is "746865"
I want to a solution to convert "the" to "746865" and "746865" to "the"
data-conversion python-3.7
@KenWhite use this converter browserling.com/tools/text-to-hex
– user10303745
Sep 1 '18 at 14:12
add a comment |
I look some solution in this site but those not works in python 3.7.
So, I asked a new question.
Hex string of "the" is "746865"
I want to a solution to convert "the" to "746865" and "746865" to "the"
data-conversion python-3.7
I look some solution in this site but those not works in python 3.7.
So, I asked a new question.
Hex string of "the" is "746865"
I want to a solution to convert "the" to "746865" and "746865" to "the"
data-conversion python-3.7
data-conversion python-3.7
asked Sep 1 '18 at 13:58
user10303745
@KenWhite use this converter browserling.com/tools/text-to-hex
– user10303745
Sep 1 '18 at 14:12
add a comment |
@KenWhite use this converter browserling.com/tools/text-to-hex
– user10303745
Sep 1 '18 at 14:12
@KenWhite use this converter browserling.com/tools/text-to-hex
– user10303745
Sep 1 '18 at 14:12
@KenWhite use this converter browserling.com/tools/text-to-hex
– user10303745
Sep 1 '18 at 14:12
add a comment |
4 Answers
4
active
oldest
votes
#!/usr/bin/python3
"""
Program name: txt_to_ASC.py
The program transfers
a string of letters -> the corresponding
string of hexadecimal ASCII-codes,
eg. the -> 746865
Only letters in [abc...xyzABC...XYZ] should be input.
"""
print("Transfer letters to hex ASCII-codes")
print("Input range is [abc...xyzABC...XYZ].")
print()
string = input("Input set of letters, eg. the: ")
print("hex ASCII-code: " + " "*15, end = "")
def str_to_hasc(x):
global glo
byt = bytes(x, 'utf-8')
bythex = byt.hex()
for b1 in bythex:
y = print(b1, end = "")
glo = str(y)
return glo
str_to_hasc(string)
Usually it's better to explain a solution instead of just posting some rows of anonymous code. You can read How do I write a good answer, and also Explaining entirely code-based answers
– Anh Pham
Nov 19 '18 at 13:28
add a comment |
Given that your string contains ascii only (each char is in range 0-0xff), you can use the following snippet:
In [28]: s = '746865'
In [29]: import math
In [30]: int(s, base=16).to_bytes(math.ceil(len(s) / 2), byteorder='big').decode('ascii')
Out[30]: 'the'
Firstly you need to convert a string into integer with base of 16, then convert it to bytes (assuming 2 chars per byte) and then convert bytes back to string using decode
add a comment |
If you have a byte string, then:
>>> import binascii
>>> binascii.hexlify(b'the')
b'746865'
If you have a Unicode string, you can encode it:
>>> s = 'the'
>>> binascii.hexlify(s)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: a bytes-like object is required, not 'str'
>>> binascii.hexlify(s.encode())
b'746865'
The result is a byte string, you can decode it to get a Unicode string:
>>> binascii.hexlify(s.encode()).decode()
'746865'
The reverse, of course, is:
>>> binascii.unhexlify(b'746865')
b'the'
Whyb
is used inhexlify
?
– user10303745
Sep 1 '18 at 16:57
@ alhelal Byte strings in Python 3 are represented that way.
– Mark Tolonen
Sep 1 '18 at 20:24
add a comment |
#!/usr/bin/python3
"""
Program name: ASC_to_txt.py
The program's input is a string of hexadecimal digits.
The string is a bytes object, and each byte is supposed to be
the hex ASCII-code of a (capital or small) letter.
The program's output is the string of the corresponding letters.
Example
Input: 746865
First subresult: ['7','4','6','8','6','5']
Second subresult: ['0x74', '0x68', '0x65']
Third subresult: [116, 104, 101]
Final result: the
References
Contribution by alhelal to stackoverflow.com (20180901)
Contribution by QintenG to stackoverflow.com (20170104)
Mark Pilgrim, Dive into Python 3, section 4.6
"""
import string
print("The program converts a string of hex ASCII-codes")
print("into the corresponding string of letters.")
print("Input range is [41, 42, ..., 5a] U [61, 62, ..., 7a]. n")
x = input("Input the hex ASCII-codes, eg. 746865: ")
result_1 =
for i in range(0,len(x)//2):
for j in range(0,2):
result_1.extend(x[2*i+j])
# First subresult
lenres_1 = len(result_1)
result_2 =
for i in range(0,len(result_1) - 1,2):
temp = ""
temp = temp + "0x" + result_1[i] #0, 2, 4
temp = temp + result_1[i + 1] #1, 3, 5
result_2.append(temp)
# Second subresult
result_3 =
for i in range(0,len(result_2)):
result_3.append(int(result_2[i],16))
# Third subresult
by = bytes(result_3)
result_4 = by.decode('utf-8')
# Final result
print("Corresponding string of letters:" + " "*6, result_4, end = "n")
1
The two programs, ASC_to_txt.py and txt_to_ASC.py, both input and output strings.
– jeppe
Nov 19 '18 at 13:31
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52128805%2fhow-can-i-change-ascii-string-to-hex-and-vice-versa-in-python-3-7%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
#!/usr/bin/python3
"""
Program name: txt_to_ASC.py
The program transfers
a string of letters -> the corresponding
string of hexadecimal ASCII-codes,
eg. the -> 746865
Only letters in [abc...xyzABC...XYZ] should be input.
"""
print("Transfer letters to hex ASCII-codes")
print("Input range is [abc...xyzABC...XYZ].")
print()
string = input("Input set of letters, eg. the: ")
print("hex ASCII-code: " + " "*15, end = "")
def str_to_hasc(x):
global glo
byt = bytes(x, 'utf-8')
bythex = byt.hex()
for b1 in bythex:
y = print(b1, end = "")
glo = str(y)
return glo
str_to_hasc(string)
Usually it's better to explain a solution instead of just posting some rows of anonymous code. You can read How do I write a good answer, and also Explaining entirely code-based answers
– Anh Pham
Nov 19 '18 at 13:28
add a comment |
#!/usr/bin/python3
"""
Program name: txt_to_ASC.py
The program transfers
a string of letters -> the corresponding
string of hexadecimal ASCII-codes,
eg. the -> 746865
Only letters in [abc...xyzABC...XYZ] should be input.
"""
print("Transfer letters to hex ASCII-codes")
print("Input range is [abc...xyzABC...XYZ].")
print()
string = input("Input set of letters, eg. the: ")
print("hex ASCII-code: " + " "*15, end = "")
def str_to_hasc(x):
global glo
byt = bytes(x, 'utf-8')
bythex = byt.hex()
for b1 in bythex:
y = print(b1, end = "")
glo = str(y)
return glo
str_to_hasc(string)
Usually it's better to explain a solution instead of just posting some rows of anonymous code. You can read How do I write a good answer, and also Explaining entirely code-based answers
– Anh Pham
Nov 19 '18 at 13:28
add a comment |
#!/usr/bin/python3
"""
Program name: txt_to_ASC.py
The program transfers
a string of letters -> the corresponding
string of hexadecimal ASCII-codes,
eg. the -> 746865
Only letters in [abc...xyzABC...XYZ] should be input.
"""
print("Transfer letters to hex ASCII-codes")
print("Input range is [abc...xyzABC...XYZ].")
print()
string = input("Input set of letters, eg. the: ")
print("hex ASCII-code: " + " "*15, end = "")
def str_to_hasc(x):
global glo
byt = bytes(x, 'utf-8')
bythex = byt.hex()
for b1 in bythex:
y = print(b1, end = "")
glo = str(y)
return glo
str_to_hasc(string)
#!/usr/bin/python3
"""
Program name: txt_to_ASC.py
The program transfers
a string of letters -> the corresponding
string of hexadecimal ASCII-codes,
eg. the -> 746865
Only letters in [abc...xyzABC...XYZ] should be input.
"""
print("Transfer letters to hex ASCII-codes")
print("Input range is [abc...xyzABC...XYZ].")
print()
string = input("Input set of letters, eg. the: ")
print("hex ASCII-code: " + " "*15, end = "")
def str_to_hasc(x):
global glo
byt = bytes(x, 'utf-8')
bythex = byt.hex()
for b1 in bythex:
y = print(b1, end = "")
glo = str(y)
return glo
str_to_hasc(string)
answered Nov 19 '18 at 13:09
jeppejeppe
92
92
Usually it's better to explain a solution instead of just posting some rows of anonymous code. You can read How do I write a good answer, and also Explaining entirely code-based answers
– Anh Pham
Nov 19 '18 at 13:28
add a comment |
Usually it's better to explain a solution instead of just posting some rows of anonymous code. You can read How do I write a good answer, and also Explaining entirely code-based answers
– Anh Pham
Nov 19 '18 at 13:28
Usually it's better to explain a solution instead of just posting some rows of anonymous code. You can read How do I write a good answer, and also Explaining entirely code-based answers
– Anh Pham
Nov 19 '18 at 13:28
Usually it's better to explain a solution instead of just posting some rows of anonymous code. You can read How do I write a good answer, and also Explaining entirely code-based answers
– Anh Pham
Nov 19 '18 at 13:28
add a comment |
Given that your string contains ascii only (each char is in range 0-0xff), you can use the following snippet:
In [28]: s = '746865'
In [29]: import math
In [30]: int(s, base=16).to_bytes(math.ceil(len(s) / 2), byteorder='big').decode('ascii')
Out[30]: 'the'
Firstly you need to convert a string into integer with base of 16, then convert it to bytes (assuming 2 chars per byte) and then convert bytes back to string using decode
add a comment |
Given that your string contains ascii only (each char is in range 0-0xff), you can use the following snippet:
In [28]: s = '746865'
In [29]: import math
In [30]: int(s, base=16).to_bytes(math.ceil(len(s) / 2), byteorder='big').decode('ascii')
Out[30]: 'the'
Firstly you need to convert a string into integer with base of 16, then convert it to bytes (assuming 2 chars per byte) and then convert bytes back to string using decode
add a comment |
Given that your string contains ascii only (each char is in range 0-0xff), you can use the following snippet:
In [28]: s = '746865'
In [29]: import math
In [30]: int(s, base=16).to_bytes(math.ceil(len(s) / 2), byteorder='big').decode('ascii')
Out[30]: 'the'
Firstly you need to convert a string into integer with base of 16, then convert it to bytes (assuming 2 chars per byte) and then convert bytes back to string using decode
Given that your string contains ascii only (each char is in range 0-0xff), you can use the following snippet:
In [28]: s = '746865'
In [29]: import math
In [30]: int(s, base=16).to_bytes(math.ceil(len(s) / 2), byteorder='big').decode('ascii')
Out[30]: 'the'
Firstly you need to convert a string into integer with base of 16, then convert it to bytes (assuming 2 chars per byte) and then convert bytes back to string using decode
answered Sep 1 '18 at 14:16
soonsoon
20.6k44572
20.6k44572
add a comment |
add a comment |
If you have a byte string, then:
>>> import binascii
>>> binascii.hexlify(b'the')
b'746865'
If you have a Unicode string, you can encode it:
>>> s = 'the'
>>> binascii.hexlify(s)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: a bytes-like object is required, not 'str'
>>> binascii.hexlify(s.encode())
b'746865'
The result is a byte string, you can decode it to get a Unicode string:
>>> binascii.hexlify(s.encode()).decode()
'746865'
The reverse, of course, is:
>>> binascii.unhexlify(b'746865')
b'the'
Whyb
is used inhexlify
?
– user10303745
Sep 1 '18 at 16:57
@ alhelal Byte strings in Python 3 are represented that way.
– Mark Tolonen
Sep 1 '18 at 20:24
add a comment |
If you have a byte string, then:
>>> import binascii
>>> binascii.hexlify(b'the')
b'746865'
If you have a Unicode string, you can encode it:
>>> s = 'the'
>>> binascii.hexlify(s)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: a bytes-like object is required, not 'str'
>>> binascii.hexlify(s.encode())
b'746865'
The result is a byte string, you can decode it to get a Unicode string:
>>> binascii.hexlify(s.encode()).decode()
'746865'
The reverse, of course, is:
>>> binascii.unhexlify(b'746865')
b'the'
Whyb
is used inhexlify
?
– user10303745
Sep 1 '18 at 16:57
@ alhelal Byte strings in Python 3 are represented that way.
– Mark Tolonen
Sep 1 '18 at 20:24
add a comment |
If you have a byte string, then:
>>> import binascii
>>> binascii.hexlify(b'the')
b'746865'
If you have a Unicode string, you can encode it:
>>> s = 'the'
>>> binascii.hexlify(s)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: a bytes-like object is required, not 'str'
>>> binascii.hexlify(s.encode())
b'746865'
The result is a byte string, you can decode it to get a Unicode string:
>>> binascii.hexlify(s.encode()).decode()
'746865'
The reverse, of course, is:
>>> binascii.unhexlify(b'746865')
b'the'
If you have a byte string, then:
>>> import binascii
>>> binascii.hexlify(b'the')
b'746865'
If you have a Unicode string, you can encode it:
>>> s = 'the'
>>> binascii.hexlify(s)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: a bytes-like object is required, not 'str'
>>> binascii.hexlify(s.encode())
b'746865'
The result is a byte string, you can decode it to get a Unicode string:
>>> binascii.hexlify(s.encode()).decode()
'746865'
The reverse, of course, is:
>>> binascii.unhexlify(b'746865')
b'the'
answered Sep 1 '18 at 14:23


Mark TolonenMark Tolonen
92.2k12110176
92.2k12110176
Whyb
is used inhexlify
?
– user10303745
Sep 1 '18 at 16:57
@ alhelal Byte strings in Python 3 are represented that way.
– Mark Tolonen
Sep 1 '18 at 20:24
add a comment |
Whyb
is used inhexlify
?
– user10303745
Sep 1 '18 at 16:57
@ alhelal Byte strings in Python 3 are represented that way.
– Mark Tolonen
Sep 1 '18 at 20:24
Why
b
is used in hexlify
?– user10303745
Sep 1 '18 at 16:57
Why
b
is used in hexlify
?– user10303745
Sep 1 '18 at 16:57
@ alhelal Byte strings in Python 3 are represented that way.
– Mark Tolonen
Sep 1 '18 at 20:24
@ alhelal Byte strings in Python 3 are represented that way.
– Mark Tolonen
Sep 1 '18 at 20:24
add a comment |
#!/usr/bin/python3
"""
Program name: ASC_to_txt.py
The program's input is a string of hexadecimal digits.
The string is a bytes object, and each byte is supposed to be
the hex ASCII-code of a (capital or small) letter.
The program's output is the string of the corresponding letters.
Example
Input: 746865
First subresult: ['7','4','6','8','6','5']
Second subresult: ['0x74', '0x68', '0x65']
Third subresult: [116, 104, 101]
Final result: the
References
Contribution by alhelal to stackoverflow.com (20180901)
Contribution by QintenG to stackoverflow.com (20170104)
Mark Pilgrim, Dive into Python 3, section 4.6
"""
import string
print("The program converts a string of hex ASCII-codes")
print("into the corresponding string of letters.")
print("Input range is [41, 42, ..., 5a] U [61, 62, ..., 7a]. n")
x = input("Input the hex ASCII-codes, eg. 746865: ")
result_1 =
for i in range(0,len(x)//2):
for j in range(0,2):
result_1.extend(x[2*i+j])
# First subresult
lenres_1 = len(result_1)
result_2 =
for i in range(0,len(result_1) - 1,2):
temp = ""
temp = temp + "0x" + result_1[i] #0, 2, 4
temp = temp + result_1[i + 1] #1, 3, 5
result_2.append(temp)
# Second subresult
result_3 =
for i in range(0,len(result_2)):
result_3.append(int(result_2[i],16))
# Third subresult
by = bytes(result_3)
result_4 = by.decode('utf-8')
# Final result
print("Corresponding string of letters:" + " "*6, result_4, end = "n")
1
The two programs, ASC_to_txt.py and txt_to_ASC.py, both input and output strings.
– jeppe
Nov 19 '18 at 13:31
add a comment |
#!/usr/bin/python3
"""
Program name: ASC_to_txt.py
The program's input is a string of hexadecimal digits.
The string is a bytes object, and each byte is supposed to be
the hex ASCII-code of a (capital or small) letter.
The program's output is the string of the corresponding letters.
Example
Input: 746865
First subresult: ['7','4','6','8','6','5']
Second subresult: ['0x74', '0x68', '0x65']
Third subresult: [116, 104, 101]
Final result: the
References
Contribution by alhelal to stackoverflow.com (20180901)
Contribution by QintenG to stackoverflow.com (20170104)
Mark Pilgrim, Dive into Python 3, section 4.6
"""
import string
print("The program converts a string of hex ASCII-codes")
print("into the corresponding string of letters.")
print("Input range is [41, 42, ..., 5a] U [61, 62, ..., 7a]. n")
x = input("Input the hex ASCII-codes, eg. 746865: ")
result_1 =
for i in range(0,len(x)//2):
for j in range(0,2):
result_1.extend(x[2*i+j])
# First subresult
lenres_1 = len(result_1)
result_2 =
for i in range(0,len(result_1) - 1,2):
temp = ""
temp = temp + "0x" + result_1[i] #0, 2, 4
temp = temp + result_1[i + 1] #1, 3, 5
result_2.append(temp)
# Second subresult
result_3 =
for i in range(0,len(result_2)):
result_3.append(int(result_2[i],16))
# Third subresult
by = bytes(result_3)
result_4 = by.decode('utf-8')
# Final result
print("Corresponding string of letters:" + " "*6, result_4, end = "n")
1
The two programs, ASC_to_txt.py and txt_to_ASC.py, both input and output strings.
– jeppe
Nov 19 '18 at 13:31
add a comment |
#!/usr/bin/python3
"""
Program name: ASC_to_txt.py
The program's input is a string of hexadecimal digits.
The string is a bytes object, and each byte is supposed to be
the hex ASCII-code of a (capital or small) letter.
The program's output is the string of the corresponding letters.
Example
Input: 746865
First subresult: ['7','4','6','8','6','5']
Second subresult: ['0x74', '0x68', '0x65']
Third subresult: [116, 104, 101]
Final result: the
References
Contribution by alhelal to stackoverflow.com (20180901)
Contribution by QintenG to stackoverflow.com (20170104)
Mark Pilgrim, Dive into Python 3, section 4.6
"""
import string
print("The program converts a string of hex ASCII-codes")
print("into the corresponding string of letters.")
print("Input range is [41, 42, ..., 5a] U [61, 62, ..., 7a]. n")
x = input("Input the hex ASCII-codes, eg. 746865: ")
result_1 =
for i in range(0,len(x)//2):
for j in range(0,2):
result_1.extend(x[2*i+j])
# First subresult
lenres_1 = len(result_1)
result_2 =
for i in range(0,len(result_1) - 1,2):
temp = ""
temp = temp + "0x" + result_1[i] #0, 2, 4
temp = temp + result_1[i + 1] #1, 3, 5
result_2.append(temp)
# Second subresult
result_3 =
for i in range(0,len(result_2)):
result_3.append(int(result_2[i],16))
# Third subresult
by = bytes(result_3)
result_4 = by.decode('utf-8')
# Final result
print("Corresponding string of letters:" + " "*6, result_4, end = "n")
#!/usr/bin/python3
"""
Program name: ASC_to_txt.py
The program's input is a string of hexadecimal digits.
The string is a bytes object, and each byte is supposed to be
the hex ASCII-code of a (capital or small) letter.
The program's output is the string of the corresponding letters.
Example
Input: 746865
First subresult: ['7','4','6','8','6','5']
Second subresult: ['0x74', '0x68', '0x65']
Third subresult: [116, 104, 101]
Final result: the
References
Contribution by alhelal to stackoverflow.com (20180901)
Contribution by QintenG to stackoverflow.com (20170104)
Mark Pilgrim, Dive into Python 3, section 4.6
"""
import string
print("The program converts a string of hex ASCII-codes")
print("into the corresponding string of letters.")
print("Input range is [41, 42, ..., 5a] U [61, 62, ..., 7a]. n")
x = input("Input the hex ASCII-codes, eg. 746865: ")
result_1 =
for i in range(0,len(x)//2):
for j in range(0,2):
result_1.extend(x[2*i+j])
# First subresult
lenres_1 = len(result_1)
result_2 =
for i in range(0,len(result_1) - 1,2):
temp = ""
temp = temp + "0x" + result_1[i] #0, 2, 4
temp = temp + result_1[i + 1] #1, 3, 5
result_2.append(temp)
# Second subresult
result_3 =
for i in range(0,len(result_2)):
result_3.append(int(result_2[i],16))
# Third subresult
by = bytes(result_3)
result_4 = by.decode('utf-8')
# Final result
print("Corresponding string of letters:" + " "*6, result_4, end = "n")
answered Nov 19 '18 at 13:19
jeppejeppe
92
92
1
The two programs, ASC_to_txt.py and txt_to_ASC.py, both input and output strings.
– jeppe
Nov 19 '18 at 13:31
add a comment |
1
The two programs, ASC_to_txt.py and txt_to_ASC.py, both input and output strings.
– jeppe
Nov 19 '18 at 13:31
1
1
The two programs, ASC_to_txt.py and txt_to_ASC.py, both input and output strings.
– jeppe
Nov 19 '18 at 13:31
The two programs, ASC_to_txt.py and txt_to_ASC.py, both input and output strings.
– jeppe
Nov 19 '18 at 13:31
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52128805%2fhow-can-i-change-ascii-string-to-hex-and-vice-versa-in-python-3-7%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
N6,M,q77sIszbz5jqNGg06j74W494hUFmDnLZ
@KenWhite use this converter browserling.com/tools/text-to-hex
– user10303745
Sep 1 '18 at 14:12