client wants to transfer the large file to the server [on hold]
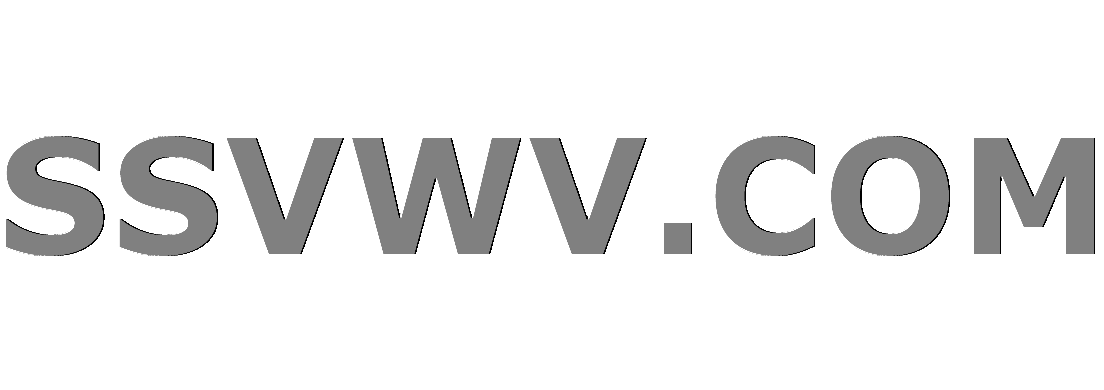
Multi tool use
up vote
0
down vote
favorite
Client.py
import socket
import sys
HOST = '192.168.100.232' # server name goes in here
PORT = 5005
def put(commandName):
socket1 = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket1.connect((HOST, PORT))
socket1.send(commandName)
string = commandName.split(' ', 1)
inputFile = string[1]
with open(inputFile, 'rb') as file_to_send:
for data in file_to_send:
socket1.sendall(data)
print 'PUT Successful'
socket1.close()
return
msg = raw_input('Enter your name: ')
while(1):
print '"put [filename]" to send the file the server '
print '"quit" to exit'
sys.stdout.write('%s> ' % msg)
inputCommand = sys.stdin.readline().strip()
if (inputCommand == 'quit'):
socket.send('quit')
break
else:
string = inputCommand.split(' ', 1)
if (string[0] == 'put'):
put(inputCommand)
Server.py
import socket
import sys
HOST = '192.168.100.232'
PORT = 5005
socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket.bind((HOST, PORT))
socket.listen(1)
while (1):
conn, addr = socket.accept()
print 'New client connected ..'
reqCommand = conn.recv(1024)
print 'Client> %s' %(reqCommand)
if (reqCommand == 'quit'):
break
else:
string = reqCommand.split(' ', 1) #in case of 'put' and 'get' method
reqFile = string[1]
if (string[0] == 'put'):
with open(reqFile, 'wb') as file_to_write:
while True:
data = conn.recv(1024)
if not data:
break
file_to_write.write(data)
file_to_write.close()
break
print 'Receive Successful'
conn.close()
socket.close()
Both script run: https://i.stack.imgur.com/O7uUs.png
But i receive the corrupt file on the server.can anyone tell me what's wrong.
server python sockets
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by PerlDuck, karel, Zanna, Thomas, Tom Brossman yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This is not about Ubuntu. Questions about other Linux distributions can be asked on Unix & Linux, those about Windows on Super User, those about Apple products on Ask Different and generic programming questions on Stack Overflow." – PerlDuck, karel, Zanna, Thomas, Tom Brossman
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
up vote
0
down vote
favorite
Client.py
import socket
import sys
HOST = '192.168.100.232' # server name goes in here
PORT = 5005
def put(commandName):
socket1 = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket1.connect((HOST, PORT))
socket1.send(commandName)
string = commandName.split(' ', 1)
inputFile = string[1]
with open(inputFile, 'rb') as file_to_send:
for data in file_to_send:
socket1.sendall(data)
print 'PUT Successful'
socket1.close()
return
msg = raw_input('Enter your name: ')
while(1):
print '"put [filename]" to send the file the server '
print '"quit" to exit'
sys.stdout.write('%s> ' % msg)
inputCommand = sys.stdin.readline().strip()
if (inputCommand == 'quit'):
socket.send('quit')
break
else:
string = inputCommand.split(' ', 1)
if (string[0] == 'put'):
put(inputCommand)
Server.py
import socket
import sys
HOST = '192.168.100.232'
PORT = 5005
socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket.bind((HOST, PORT))
socket.listen(1)
while (1):
conn, addr = socket.accept()
print 'New client connected ..'
reqCommand = conn.recv(1024)
print 'Client> %s' %(reqCommand)
if (reqCommand == 'quit'):
break
else:
string = reqCommand.split(' ', 1) #in case of 'put' and 'get' method
reqFile = string[1]
if (string[0] == 'put'):
with open(reqFile, 'wb') as file_to_write:
while True:
data = conn.recv(1024)
if not data:
break
file_to_write.write(data)
file_to_write.close()
break
print 'Receive Successful'
conn.close()
socket.close()
Both script run: https://i.stack.imgur.com/O7uUs.png
But i receive the corrupt file on the server.can anyone tell me what's wrong.
server python sockets
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by PerlDuck, karel, Zanna, Thomas, Tom Brossman yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This is not about Ubuntu. Questions about other Linux distributions can be asked on Unix & Linux, those about Windows on Super User, those about Apple products on Ask Different and generic programming questions on Stack Overflow." – PerlDuck, karel, Zanna, Thomas, Tom Brossman
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Client.py
import socket
import sys
HOST = '192.168.100.232' # server name goes in here
PORT = 5005
def put(commandName):
socket1 = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket1.connect((HOST, PORT))
socket1.send(commandName)
string = commandName.split(' ', 1)
inputFile = string[1]
with open(inputFile, 'rb') as file_to_send:
for data in file_to_send:
socket1.sendall(data)
print 'PUT Successful'
socket1.close()
return
msg = raw_input('Enter your name: ')
while(1):
print '"put [filename]" to send the file the server '
print '"quit" to exit'
sys.stdout.write('%s> ' % msg)
inputCommand = sys.stdin.readline().strip()
if (inputCommand == 'quit'):
socket.send('quit')
break
else:
string = inputCommand.split(' ', 1)
if (string[0] == 'put'):
put(inputCommand)
Server.py
import socket
import sys
HOST = '192.168.100.232'
PORT = 5005
socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket.bind((HOST, PORT))
socket.listen(1)
while (1):
conn, addr = socket.accept()
print 'New client connected ..'
reqCommand = conn.recv(1024)
print 'Client> %s' %(reqCommand)
if (reqCommand == 'quit'):
break
else:
string = reqCommand.split(' ', 1) #in case of 'put' and 'get' method
reqFile = string[1]
if (string[0] == 'put'):
with open(reqFile, 'wb') as file_to_write:
while True:
data = conn.recv(1024)
if not data:
break
file_to_write.write(data)
file_to_write.close()
break
print 'Receive Successful'
conn.close()
socket.close()
Both script run: https://i.stack.imgur.com/O7uUs.png
But i receive the corrupt file on the server.can anyone tell me what's wrong.
server python sockets
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Client.py
import socket
import sys
HOST = '192.168.100.232' # server name goes in here
PORT = 5005
def put(commandName):
socket1 = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket1.connect((HOST, PORT))
socket1.send(commandName)
string = commandName.split(' ', 1)
inputFile = string[1]
with open(inputFile, 'rb') as file_to_send:
for data in file_to_send:
socket1.sendall(data)
print 'PUT Successful'
socket1.close()
return
msg = raw_input('Enter your name: ')
while(1):
print '"put [filename]" to send the file the server '
print '"quit" to exit'
sys.stdout.write('%s> ' % msg)
inputCommand = sys.stdin.readline().strip()
if (inputCommand == 'quit'):
socket.send('quit')
break
else:
string = inputCommand.split(' ', 1)
if (string[0] == 'put'):
put(inputCommand)
Server.py
import socket
import sys
HOST = '192.168.100.232'
PORT = 5005
socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
socket.bind((HOST, PORT))
socket.listen(1)
while (1):
conn, addr = socket.accept()
print 'New client connected ..'
reqCommand = conn.recv(1024)
print 'Client> %s' %(reqCommand)
if (reqCommand == 'quit'):
break
else:
string = reqCommand.split(' ', 1) #in case of 'put' and 'get' method
reqFile = string[1]
if (string[0] == 'put'):
with open(reqFile, 'wb') as file_to_write:
while True:
data = conn.recv(1024)
if not data:
break
file_to_write.write(data)
file_to_write.close()
break
print 'Receive Successful'
conn.close()
socket.close()
Both script run: https://i.stack.imgur.com/O7uUs.png
But i receive the corrupt file on the server.can anyone tell me what's wrong.
server python sockets
server python sockets
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 13 at 10:53


Shubham Gupta
11
11
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Shubham Gupta is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
put on hold as off-topic by PerlDuck, karel, Zanna, Thomas, Tom Brossman yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This is not about Ubuntu. Questions about other Linux distributions can be asked on Unix & Linux, those about Windows on Super User, those about Apple products on Ask Different and generic programming questions on Stack Overflow." – PerlDuck, karel, Zanna, Thomas, Tom Brossman
If this question can be reworded to fit the rules in the help center, please edit the question.
put on hold as off-topic by PerlDuck, karel, Zanna, Thomas, Tom Brossman yesterday
This question appears to be off-topic. The users who voted to close gave this specific reason:
- "This is not about Ubuntu. Questions about other Linux distributions can be asked on Unix & Linux, those about Windows on Super User, those about Apple products on Ask Different and generic programming questions on Stack Overflow." – PerlDuck, karel, Zanna, Thomas, Tom Brossman
If this question can be reworded to fit the rules in the help center, please edit the question.
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
eyR4CPBvh,zHbU8ZGmHw1QK3ZZz2jaA5KDuRCdFwRSa5IK3vltmOf2 fBVbmY8HqiqpVBeY,i scmd,yns8L0UllkZTdB