PowerShell - Update Variable Value from another Variable
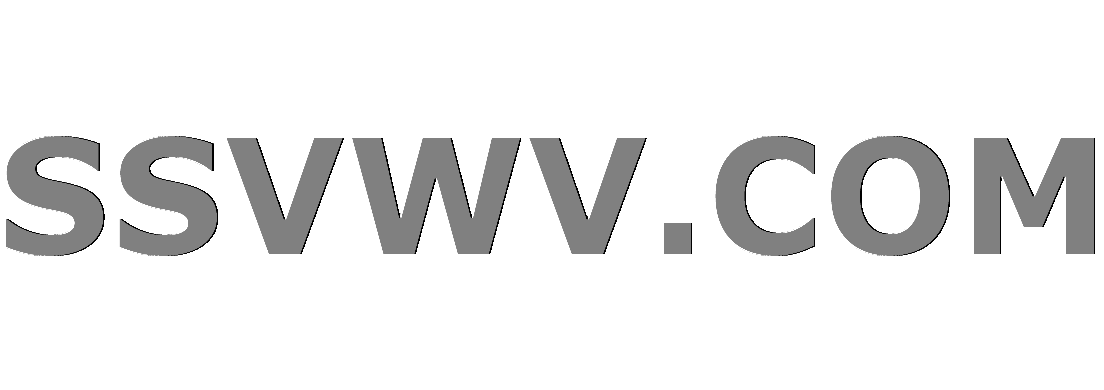
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am trying to update a number of values in $Table1 from another $Table2.
Say I have $Table1 (in this case an imported CSV file):
Model ModelID Blah
abc 0 Blah
ghi 0 Blah
mno 0 Blah
and I have $Table2 (in this case, obtained from a data source):
name id
abc 11
def 12
ghi 13
jkl 14
mno 15
pqr 16
etc.
I am trying to update the values in $Table1."ModelID" from $Table2."id"
WHERE $Table1."Model" = $Table2."name"
In SQL, I would do something like:
UPDATE $Table1
SET ModelID = $Table2."id"
WHERE $Table1."Model" = $Table2."name"
How do I do conditional updates based on joins on columns in a Variable in PowerShell?
I was looking at:
-replace... (I can't seem to do conditional replaces based on joins)
Add-Member -MemberType NoteProperty "modelID" -Value ... (again, I can't seem to set the value based on joins)
foreach($item in $Table1)
{
$Table1."ModelID" = $Table2."id"
where ?????
}.. (again, I can't seem to set the value based on joins)
Am I over-egging the pudding here?
powershell
add a comment |
I am trying to update a number of values in $Table1 from another $Table2.
Say I have $Table1 (in this case an imported CSV file):
Model ModelID Blah
abc 0 Blah
ghi 0 Blah
mno 0 Blah
and I have $Table2 (in this case, obtained from a data source):
name id
abc 11
def 12
ghi 13
jkl 14
mno 15
pqr 16
etc.
I am trying to update the values in $Table1."ModelID" from $Table2."id"
WHERE $Table1."Model" = $Table2."name"
In SQL, I would do something like:
UPDATE $Table1
SET ModelID = $Table2."id"
WHERE $Table1."Model" = $Table2."name"
How do I do conditional updates based on joins on columns in a Variable in PowerShell?
I was looking at:
-replace... (I can't seem to do conditional replaces based on joins)
Add-Member -MemberType NoteProperty "modelID" -Value ... (again, I can't seem to set the value based on joins)
foreach($item in $Table1)
{
$Table1."ModelID" = $Table2."id"
where ?????
}.. (again, I can't seem to set the value based on joins)
Am I over-egging the pudding here?
powershell
add a comment |
I am trying to update a number of values in $Table1 from another $Table2.
Say I have $Table1 (in this case an imported CSV file):
Model ModelID Blah
abc 0 Blah
ghi 0 Blah
mno 0 Blah
and I have $Table2 (in this case, obtained from a data source):
name id
abc 11
def 12
ghi 13
jkl 14
mno 15
pqr 16
etc.
I am trying to update the values in $Table1."ModelID" from $Table2."id"
WHERE $Table1."Model" = $Table2."name"
In SQL, I would do something like:
UPDATE $Table1
SET ModelID = $Table2."id"
WHERE $Table1."Model" = $Table2."name"
How do I do conditional updates based on joins on columns in a Variable in PowerShell?
I was looking at:
-replace... (I can't seem to do conditional replaces based on joins)
Add-Member -MemberType NoteProperty "modelID" -Value ... (again, I can't seem to set the value based on joins)
foreach($item in $Table1)
{
$Table1."ModelID" = $Table2."id"
where ?????
}.. (again, I can't seem to set the value based on joins)
Am I over-egging the pudding here?
powershell
I am trying to update a number of values in $Table1 from another $Table2.
Say I have $Table1 (in this case an imported CSV file):
Model ModelID Blah
abc 0 Blah
ghi 0 Blah
mno 0 Blah
and I have $Table2 (in this case, obtained from a data source):
name id
abc 11
def 12
ghi 13
jkl 14
mno 15
pqr 16
etc.
I am trying to update the values in $Table1."ModelID" from $Table2."id"
WHERE $Table1."Model" = $Table2."name"
In SQL, I would do something like:
UPDATE $Table1
SET ModelID = $Table2."id"
WHERE $Table1."Model" = $Table2."name"
How do I do conditional updates based on joins on columns in a Variable in PowerShell?
I was looking at:
-replace... (I can't seem to do conditional replaces based on joins)
Add-Member -MemberType NoteProperty "modelID" -Value ... (again, I can't seem to set the value based on joins)
foreach($item in $Table1)
{
$Table1."ModelID" = $Table2."id"
where ?????
}.. (again, I can't seem to set the value based on joins)
Am I over-egging the pudding here?
powershell
powershell
asked Nov 23 '18 at 4:17


SimonSimon
506
506
add a comment |
add a comment |
5 Answers
5
active
oldest
votes
This is VERY messy but it seems to get the job done.
$Table2 = import-csv C:temptest.csv
$Table1 = import-csv C:tempTest55.csv
Foreach($item in $Table1){
Foreach($tab2 in $Table2){
If($tab2.name -match $item.model){
$item.ModelID = $tab2.id
}
}
}
Thank you SO much @Drew. Worked a treat.
– Simon
Nov 23 '18 at 5:52
add a comment |
Here is the code can help out I believe.
foreach($i in $t1)
{
foreach($j in $t2)
{
if($i.'model' -eq $j.'id')
{
$i.'modelid' = $j.'name'
break
}
}
}
For every item in table1, looking for the pattern in table2, if find a match, change the value in table1.
add a comment |
A less dirty variant using a hash table to lookup the ID from Model.
Using here strings as source.
## Q:Test20181123SO_53440594.ps1
$table1 = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Hashtable2 = @{}
@"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv | ForEach-Object {$Hashtable2.Add($_.Name,$_.Id)}
ForEach ($Row in $table1){
if($Hashtable2.Containskey($Row.Model)){
$Row.ModelID = $Hashtable2[$Row.Model]
} else {
"Model {0} not present in `$table2" -f $Row.Model
}
}
$table1
Sample output:
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
add a comment |
here's an alternate method. it uses the "operate against the collection" technique [introduced in v4, i think]. a hashtable is still the fastest way to do this when the lookup list is large, tho. [grin]
$OneTable = @'
Model, ModelID, Blah
abc, 0, Blah
ghi, 0, Blah
mno, 0, Blah
'@ | ConvertFrom-Csv
# removed one line [ghi, 13] to allow for "no match" error test
$TwoTable = @'
Name, ID
abc, 11
def, 12
jkl, 14
mno, 15
pqr, 16
'@ | ConvertFrom-Csv
foreach ($OT_Item in $OneTable)
{
$Lookup = $TwoTable -match $OT_Item.Model
if ($Lookup)
{
$OT_Item.ModelID = $Lookup.ID
}
else
{
Write-Warning ('No matching Model was found for [ {0} ].' -f $OT_Item.Model)
}
}
$OneTable
output ...
WARNING: No matching Model was found for [ ghi ].
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 0 Blah
mno 15 Blah
add a comment |
What about this way ...
$Data = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Reference = @"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv
$Data | ForEach-Object {
$Local:ThisModelKey = $_.Model
if ($Reference.Name -contains $ThisModelKey) {
$_.ModelID = (@($Reference | Where-Object { $_.Name -like $ThisModelKey } ))[0].ID
}
}
The result is ...
$Data
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440594%2fpowershell-update-variable-value-from-another-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is VERY messy but it seems to get the job done.
$Table2 = import-csv C:temptest.csv
$Table1 = import-csv C:tempTest55.csv
Foreach($item in $Table1){
Foreach($tab2 in $Table2){
If($tab2.name -match $item.model){
$item.ModelID = $tab2.id
}
}
}
Thank you SO much @Drew. Worked a treat.
– Simon
Nov 23 '18 at 5:52
add a comment |
This is VERY messy but it seems to get the job done.
$Table2 = import-csv C:temptest.csv
$Table1 = import-csv C:tempTest55.csv
Foreach($item in $Table1){
Foreach($tab2 in $Table2){
If($tab2.name -match $item.model){
$item.ModelID = $tab2.id
}
}
}
Thank you SO much @Drew. Worked a treat.
– Simon
Nov 23 '18 at 5:52
add a comment |
This is VERY messy but it seems to get the job done.
$Table2 = import-csv C:temptest.csv
$Table1 = import-csv C:tempTest55.csv
Foreach($item in $Table1){
Foreach($tab2 in $Table2){
If($tab2.name -match $item.model){
$item.ModelID = $tab2.id
}
}
}
This is VERY messy but it seems to get the job done.
$Table2 = import-csv C:temptest.csv
$Table1 = import-csv C:tempTest55.csv
Foreach($item in $Table1){
Foreach($tab2 in $Table2){
If($tab2.name -match $item.model){
$item.ModelID = $tab2.id
}
}
}
answered Nov 23 '18 at 4:49
DrewDrew
1,476418
1,476418
Thank you SO much @Drew. Worked a treat.
– Simon
Nov 23 '18 at 5:52
add a comment |
Thank you SO much @Drew. Worked a treat.
– Simon
Nov 23 '18 at 5:52
Thank you SO much @Drew. Worked a treat.
– Simon
Nov 23 '18 at 5:52
Thank you SO much @Drew. Worked a treat.
– Simon
Nov 23 '18 at 5:52
add a comment |
Here is the code can help out I believe.
foreach($i in $t1)
{
foreach($j in $t2)
{
if($i.'model' -eq $j.'id')
{
$i.'modelid' = $j.'name'
break
}
}
}
For every item in table1, looking for the pattern in table2, if find a match, change the value in table1.
add a comment |
Here is the code can help out I believe.
foreach($i in $t1)
{
foreach($j in $t2)
{
if($i.'model' -eq $j.'id')
{
$i.'modelid' = $j.'name'
break
}
}
}
For every item in table1, looking for the pattern in table2, if find a match, change the value in table1.
add a comment |
Here is the code can help out I believe.
foreach($i in $t1)
{
foreach($j in $t2)
{
if($i.'model' -eq $j.'id')
{
$i.'modelid' = $j.'name'
break
}
}
}
For every item in table1, looking for the pattern in table2, if find a match, change the value in table1.
Here is the code can help out I believe.
foreach($i in $t1)
{
foreach($j in $t2)
{
if($i.'model' -eq $j.'id')
{
$i.'modelid' = $j.'name'
break
}
}
}
For every item in table1, looking for the pattern in table2, if find a match, change the value in table1.
edited Nov 23 '18 at 4:55
answered Nov 23 '18 at 4:49
Larry SongLarry Song
49137
49137
add a comment |
add a comment |
A less dirty variant using a hash table to lookup the ID from Model.
Using here strings as source.
## Q:Test20181123SO_53440594.ps1
$table1 = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Hashtable2 = @{}
@"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv | ForEach-Object {$Hashtable2.Add($_.Name,$_.Id)}
ForEach ($Row in $table1){
if($Hashtable2.Containskey($Row.Model)){
$Row.ModelID = $Hashtable2[$Row.Model]
} else {
"Model {0} not present in `$table2" -f $Row.Model
}
}
$table1
Sample output:
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
add a comment |
A less dirty variant using a hash table to lookup the ID from Model.
Using here strings as source.
## Q:Test20181123SO_53440594.ps1
$table1 = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Hashtable2 = @{}
@"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv | ForEach-Object {$Hashtable2.Add($_.Name,$_.Id)}
ForEach ($Row in $table1){
if($Hashtable2.Containskey($Row.Model)){
$Row.ModelID = $Hashtable2[$Row.Model]
} else {
"Model {0} not present in `$table2" -f $Row.Model
}
}
$table1
Sample output:
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
add a comment |
A less dirty variant using a hash table to lookup the ID from Model.
Using here strings as source.
## Q:Test20181123SO_53440594.ps1
$table1 = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Hashtable2 = @{}
@"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv | ForEach-Object {$Hashtable2.Add($_.Name,$_.Id)}
ForEach ($Row in $table1){
if($Hashtable2.Containskey($Row.Model)){
$Row.ModelID = $Hashtable2[$Row.Model]
} else {
"Model {0} not present in `$table2" -f $Row.Model
}
}
$table1
Sample output:
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
A less dirty variant using a hash table to lookup the ID from Model.
Using here strings as source.
## Q:Test20181123SO_53440594.ps1
$table1 = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Hashtable2 = @{}
@"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv | ForEach-Object {$Hashtable2.Add($_.Name,$_.Id)}
ForEach ($Row in $table1){
if($Hashtable2.Containskey($Row.Model)){
$Row.ModelID = $Hashtable2[$Row.Model]
} else {
"Model {0} not present in `$table2" -f $Row.Model
}
}
$table1
Sample output:
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
answered Nov 23 '18 at 11:41


LotPingsLotPings
20.7k61633
20.7k61633
add a comment |
add a comment |
here's an alternate method. it uses the "operate against the collection" technique [introduced in v4, i think]. a hashtable is still the fastest way to do this when the lookup list is large, tho. [grin]
$OneTable = @'
Model, ModelID, Blah
abc, 0, Blah
ghi, 0, Blah
mno, 0, Blah
'@ | ConvertFrom-Csv
# removed one line [ghi, 13] to allow for "no match" error test
$TwoTable = @'
Name, ID
abc, 11
def, 12
jkl, 14
mno, 15
pqr, 16
'@ | ConvertFrom-Csv
foreach ($OT_Item in $OneTable)
{
$Lookup = $TwoTable -match $OT_Item.Model
if ($Lookup)
{
$OT_Item.ModelID = $Lookup.ID
}
else
{
Write-Warning ('No matching Model was found for [ {0} ].' -f $OT_Item.Model)
}
}
$OneTable
output ...
WARNING: No matching Model was found for [ ghi ].
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 0 Blah
mno 15 Blah
add a comment |
here's an alternate method. it uses the "operate against the collection" technique [introduced in v4, i think]. a hashtable is still the fastest way to do this when the lookup list is large, tho. [grin]
$OneTable = @'
Model, ModelID, Blah
abc, 0, Blah
ghi, 0, Blah
mno, 0, Blah
'@ | ConvertFrom-Csv
# removed one line [ghi, 13] to allow for "no match" error test
$TwoTable = @'
Name, ID
abc, 11
def, 12
jkl, 14
mno, 15
pqr, 16
'@ | ConvertFrom-Csv
foreach ($OT_Item in $OneTable)
{
$Lookup = $TwoTable -match $OT_Item.Model
if ($Lookup)
{
$OT_Item.ModelID = $Lookup.ID
}
else
{
Write-Warning ('No matching Model was found for [ {0} ].' -f $OT_Item.Model)
}
}
$OneTable
output ...
WARNING: No matching Model was found for [ ghi ].
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 0 Blah
mno 15 Blah
add a comment |
here's an alternate method. it uses the "operate against the collection" technique [introduced in v4, i think]. a hashtable is still the fastest way to do this when the lookup list is large, tho. [grin]
$OneTable = @'
Model, ModelID, Blah
abc, 0, Blah
ghi, 0, Blah
mno, 0, Blah
'@ | ConvertFrom-Csv
# removed one line [ghi, 13] to allow for "no match" error test
$TwoTable = @'
Name, ID
abc, 11
def, 12
jkl, 14
mno, 15
pqr, 16
'@ | ConvertFrom-Csv
foreach ($OT_Item in $OneTable)
{
$Lookup = $TwoTable -match $OT_Item.Model
if ($Lookup)
{
$OT_Item.ModelID = $Lookup.ID
}
else
{
Write-Warning ('No matching Model was found for [ {0} ].' -f $OT_Item.Model)
}
}
$OneTable
output ...
WARNING: No matching Model was found for [ ghi ].
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 0 Blah
mno 15 Blah
here's an alternate method. it uses the "operate against the collection" technique [introduced in v4, i think]. a hashtable is still the fastest way to do this when the lookup list is large, tho. [grin]
$OneTable = @'
Model, ModelID, Blah
abc, 0, Blah
ghi, 0, Blah
mno, 0, Blah
'@ | ConvertFrom-Csv
# removed one line [ghi, 13] to allow for "no match" error test
$TwoTable = @'
Name, ID
abc, 11
def, 12
jkl, 14
mno, 15
pqr, 16
'@ | ConvertFrom-Csv
foreach ($OT_Item in $OneTable)
{
$Lookup = $TwoTable -match $OT_Item.Model
if ($Lookup)
{
$OT_Item.ModelID = $Lookup.ID
}
else
{
Write-Warning ('No matching Model was found for [ {0} ].' -f $OT_Item.Model)
}
}
$OneTable
output ...
WARNING: No matching Model was found for [ ghi ].
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 0 Blah
mno 15 Blah
answered Nov 23 '18 at 17:31
Lee_DaileyLee_Dailey
2,8911811
2,8911811
add a comment |
add a comment |
What about this way ...
$Data = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Reference = @"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv
$Data | ForEach-Object {
$Local:ThisModelKey = $_.Model
if ($Reference.Name -contains $ThisModelKey) {
$_.ModelID = (@($Reference | Where-Object { $_.Name -like $ThisModelKey } ))[0].ID
}
}
The result is ...
$Data
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
add a comment |
What about this way ...
$Data = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Reference = @"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv
$Data | ForEach-Object {
$Local:ThisModelKey = $_.Model
if ($Reference.Name -contains $ThisModelKey) {
$_.ModelID = (@($Reference | Where-Object { $_.Name -like $ThisModelKey } ))[0].ID
}
}
The result is ...
$Data
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
add a comment |
What about this way ...
$Data = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Reference = @"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv
$Data | ForEach-Object {
$Local:ThisModelKey = $_.Model
if ($Reference.Name -contains $ThisModelKey) {
$_.ModelID = (@($Reference | Where-Object { $_.Name -like $ThisModelKey } ))[0].ID
}
}
The result is ...
$Data
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
What about this way ...
$Data = @"
Model,ModelID,Blah
abc,0,Blah
ghi,0,Blah
mno,0,Blah
"@ | ConvertFrom-Csv
$Reference = @"
Name,Id
abc,11
def,12
ghi,13
jkl,14
mno,15
pqr,16
"@ | ConvertFrom-Csv
$Data | ForEach-Object {
$Local:ThisModelKey = $_.Model
if ($Reference.Name -contains $ThisModelKey) {
$_.ModelID = (@($Reference | Where-Object { $_.Name -like $ThisModelKey } ))[0].ID
}
}
The result is ...
$Data
Model ModelID Blah
----- ------- ----
abc 11 Blah
ghi 13 Blah
mno 15 Blah
answered Nov 23 '18 at 19:27
BuxmaniakBuxmaniak
1644
1644
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53440594%2fpowershell-update-variable-value-from-another-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
oBhdY1R1F,oUwPJkW6YF ya71hg9wM,Q7V41xdsCnMXsZ JboUD0v8E,hao uwOjUDKJ 9HzyN0wIFDKpEYPhUbI4z6Vp7mE