What is causing my POST request to fail (CORS with Express/Angular)?
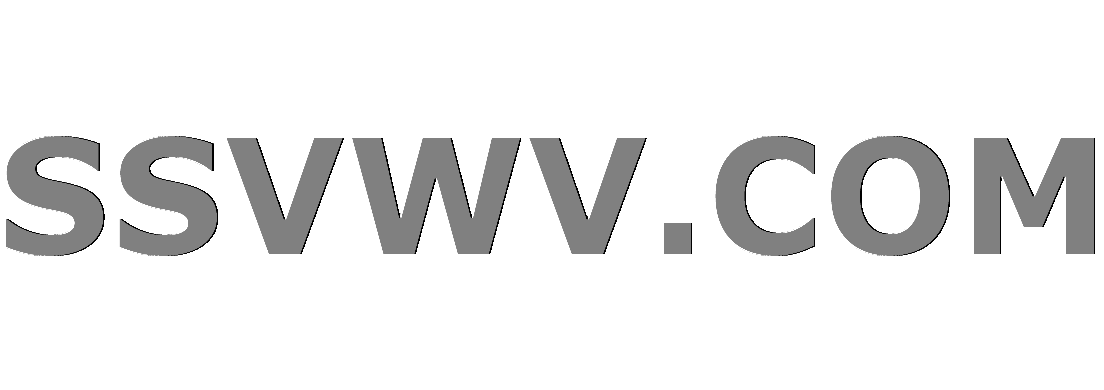
Multi tool use
At this point, I feel like I've tried everything I can think of and that has been searched.
The "pure" version of my code is as follows:
Server.js - Express
const express = require('express'),
path = require('path'),
bodyParser = require('body-parser'),
cors = require('cors'),
mongoose = require('mongoose'),
config = require('./DB');
businessRoute = require('../routes/business.route');
mongoose.Promise = global.Promise;
mongoose.connect(config.DB, { useNewUrlParser: true}).then(
() => { console.log('Database is connected') },
err => { console.log('Can not connect to the database'+ err) }
);
const app = express();
app.use(bodyParser.json());
app.use(cors());
businessRoute.all('*', cors());
app.use('/business', businessRoute);
let port = process.env.PORT || 4000;
const server = app.listen(function(){
console.log('Listening on port ' + port);
});
business.route.js - Express, concat.
const express = require('express');
const app = express();
const businessRoutes = express.Router();
// Require Business model in our routes module
let Business = require('../models/Business');
// Defined store route
businessRoutes.route('/add').post(function(req, res) {
let business = new Business(req.body);
business.save()
.then(business => {
res.status(200).json({'business': 'business added successfully'});
})
.catch(err => {
res.status(400).send("Unable to save to database");
});
});
business.server.ts - Angular
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BusinessService {
uri = 'http://localhost:4000/business';
constructor(private http: HttpClient) { }
addBusiness(person_name, business_name, business_gst_number): void {
const obj = {
person_name: person_name,
business_name: business_name,
business_gst_number: business_gst_number
};
console.log(obj);
this.http.post(`${this.uri}/add`, obj).subscribe(
res => console.log('Done')
);
}
}
What has been Tried
I've attempted to use the following code, which has been recommended on numerous sites, including SO:
app.use(function(req, res, next) {
res.setHeader("Access-Control-Allow-Origin", "*");
res.setHeader('Access-Control-Allow-Methods', 'POST,GET,OPTIONS,PUT,DELETE');
res.setHeader("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
next();
});
I've attempted to remove the cors module for the code, using the attempted solution as a replacement. I used the code in the express router. I've so used both the module and the code in conjuction in both the server.js and router, as well as (and what is still in the code) businessRoute.all('*', cors());
I'm missing something, I have to be. Any help is appreciated.
As requested, here is the network inspector information from Chrome:
And it did give me an OPTIONS error:
node.js angular express cors
|
show 1 more comment
At this point, I feel like I've tried everything I can think of and that has been searched.
The "pure" version of my code is as follows:
Server.js - Express
const express = require('express'),
path = require('path'),
bodyParser = require('body-parser'),
cors = require('cors'),
mongoose = require('mongoose'),
config = require('./DB');
businessRoute = require('../routes/business.route');
mongoose.Promise = global.Promise;
mongoose.connect(config.DB, { useNewUrlParser: true}).then(
() => { console.log('Database is connected') },
err => { console.log('Can not connect to the database'+ err) }
);
const app = express();
app.use(bodyParser.json());
app.use(cors());
businessRoute.all('*', cors());
app.use('/business', businessRoute);
let port = process.env.PORT || 4000;
const server = app.listen(function(){
console.log('Listening on port ' + port);
});
business.route.js - Express, concat.
const express = require('express');
const app = express();
const businessRoutes = express.Router();
// Require Business model in our routes module
let Business = require('../models/Business');
// Defined store route
businessRoutes.route('/add').post(function(req, res) {
let business = new Business(req.body);
business.save()
.then(business => {
res.status(200).json({'business': 'business added successfully'});
})
.catch(err => {
res.status(400).send("Unable to save to database");
});
});
business.server.ts - Angular
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BusinessService {
uri = 'http://localhost:4000/business';
constructor(private http: HttpClient) { }
addBusiness(person_name, business_name, business_gst_number): void {
const obj = {
person_name: person_name,
business_name: business_name,
business_gst_number: business_gst_number
};
console.log(obj);
this.http.post(`${this.uri}/add`, obj).subscribe(
res => console.log('Done')
);
}
}
What has been Tried
I've attempted to use the following code, which has been recommended on numerous sites, including SO:
app.use(function(req, res, next) {
res.setHeader("Access-Control-Allow-Origin", "*");
res.setHeader('Access-Control-Allow-Methods', 'POST,GET,OPTIONS,PUT,DELETE');
res.setHeader("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
next();
});
I've attempted to remove the cors module for the code, using the attempted solution as a replacement. I used the code in the express router. I've so used both the module and the code in conjuction in both the server.js and router, as well as (and what is still in the code) businessRoute.all('*', cors());
I'm missing something, I have to be. Any help is appreciated.
As requested, here is the network inspector information from Chrome:
And it did give me an OPTIONS error:
node.js angular express cors
1
Can you try in chrome? You might get a more explicit error message. And can you post a screenshot of the network instpector, showing the response for the OPTIONS request?
– David
Nov 17 '18 at 8:46
Hey! Sorry for the delay:OPTIONS http://localhost:4000/business/add net::ERR_CONNECTION_REFUSED
is what Chrome shows. The network inspector will be posted in a moment.
– Colby Hunter
Nov 17 '18 at 10:27
1
Looks like you cannot even get a response from the API for the OPTIONS request. Just checking, does the API work with GET requests?
– David
Nov 17 '18 at 10:49
I set up thebusinessRoutes.route('/').get()
withres.json({'message': 'Testing'});
. I sent the request through Postman as a GET request forhttp://localhost:4000/business/
and recieved "Could not get any response".
– Colby Hunter
Nov 17 '18 at 11:11
1
It's not a cors issue then. What if you try using the machine IP address or 127.0.0.1 instead of localhost?
– David
Nov 17 '18 at 12:40
|
show 1 more comment
At this point, I feel like I've tried everything I can think of and that has been searched.
The "pure" version of my code is as follows:
Server.js - Express
const express = require('express'),
path = require('path'),
bodyParser = require('body-parser'),
cors = require('cors'),
mongoose = require('mongoose'),
config = require('./DB');
businessRoute = require('../routes/business.route');
mongoose.Promise = global.Promise;
mongoose.connect(config.DB, { useNewUrlParser: true}).then(
() => { console.log('Database is connected') },
err => { console.log('Can not connect to the database'+ err) }
);
const app = express();
app.use(bodyParser.json());
app.use(cors());
businessRoute.all('*', cors());
app.use('/business', businessRoute);
let port = process.env.PORT || 4000;
const server = app.listen(function(){
console.log('Listening on port ' + port);
});
business.route.js - Express, concat.
const express = require('express');
const app = express();
const businessRoutes = express.Router();
// Require Business model in our routes module
let Business = require('../models/Business');
// Defined store route
businessRoutes.route('/add').post(function(req, res) {
let business = new Business(req.body);
business.save()
.then(business => {
res.status(200).json({'business': 'business added successfully'});
})
.catch(err => {
res.status(400).send("Unable to save to database");
});
});
business.server.ts - Angular
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BusinessService {
uri = 'http://localhost:4000/business';
constructor(private http: HttpClient) { }
addBusiness(person_name, business_name, business_gst_number): void {
const obj = {
person_name: person_name,
business_name: business_name,
business_gst_number: business_gst_number
};
console.log(obj);
this.http.post(`${this.uri}/add`, obj).subscribe(
res => console.log('Done')
);
}
}
What has been Tried
I've attempted to use the following code, which has been recommended on numerous sites, including SO:
app.use(function(req, res, next) {
res.setHeader("Access-Control-Allow-Origin", "*");
res.setHeader('Access-Control-Allow-Methods', 'POST,GET,OPTIONS,PUT,DELETE');
res.setHeader("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
next();
});
I've attempted to remove the cors module for the code, using the attempted solution as a replacement. I used the code in the express router. I've so used both the module and the code in conjuction in both the server.js and router, as well as (and what is still in the code) businessRoute.all('*', cors());
I'm missing something, I have to be. Any help is appreciated.
As requested, here is the network inspector information from Chrome:
And it did give me an OPTIONS error:
node.js angular express cors
At this point, I feel like I've tried everything I can think of and that has been searched.
The "pure" version of my code is as follows:
Server.js - Express
const express = require('express'),
path = require('path'),
bodyParser = require('body-parser'),
cors = require('cors'),
mongoose = require('mongoose'),
config = require('./DB');
businessRoute = require('../routes/business.route');
mongoose.Promise = global.Promise;
mongoose.connect(config.DB, { useNewUrlParser: true}).then(
() => { console.log('Database is connected') },
err => { console.log('Can not connect to the database'+ err) }
);
const app = express();
app.use(bodyParser.json());
app.use(cors());
businessRoute.all('*', cors());
app.use('/business', businessRoute);
let port = process.env.PORT || 4000;
const server = app.listen(function(){
console.log('Listening on port ' + port);
});
business.route.js - Express, concat.
const express = require('express');
const app = express();
const businessRoutes = express.Router();
// Require Business model in our routes module
let Business = require('../models/Business');
// Defined store route
businessRoutes.route('/add').post(function(req, res) {
let business = new Business(req.body);
business.save()
.then(business => {
res.status(200).json({'business': 'business added successfully'});
})
.catch(err => {
res.status(400).send("Unable to save to database");
});
});
business.server.ts - Angular
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Injectable({
providedIn: 'root'
})
export class BusinessService {
uri = 'http://localhost:4000/business';
constructor(private http: HttpClient) { }
addBusiness(person_name, business_name, business_gst_number): void {
const obj = {
person_name: person_name,
business_name: business_name,
business_gst_number: business_gst_number
};
console.log(obj);
this.http.post(`${this.uri}/add`, obj).subscribe(
res => console.log('Done')
);
}
}
What has been Tried
I've attempted to use the following code, which has been recommended on numerous sites, including SO:
app.use(function(req, res, next) {
res.setHeader("Access-Control-Allow-Origin", "*");
res.setHeader('Access-Control-Allow-Methods', 'POST,GET,OPTIONS,PUT,DELETE');
res.setHeader("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
next();
});
I've attempted to remove the cors module for the code, using the attempted solution as a replacement. I used the code in the express router. I've so used both the module and the code in conjuction in both the server.js and router, as well as (and what is still in the code) businessRoute.all('*', cors());
I'm missing something, I have to be. Any help is appreciated.
As requested, here is the network inspector information from Chrome:
And it did give me an OPTIONS error:
node.js angular express cors
node.js angular express cors
edited Nov 17 '18 at 10:28
asked Nov 17 '18 at 8:34
Colby Hunter
859
859
1
Can you try in chrome? You might get a more explicit error message. And can you post a screenshot of the network instpector, showing the response for the OPTIONS request?
– David
Nov 17 '18 at 8:46
Hey! Sorry for the delay:OPTIONS http://localhost:4000/business/add net::ERR_CONNECTION_REFUSED
is what Chrome shows. The network inspector will be posted in a moment.
– Colby Hunter
Nov 17 '18 at 10:27
1
Looks like you cannot even get a response from the API for the OPTIONS request. Just checking, does the API work with GET requests?
– David
Nov 17 '18 at 10:49
I set up thebusinessRoutes.route('/').get()
withres.json({'message': 'Testing'});
. I sent the request through Postman as a GET request forhttp://localhost:4000/business/
and recieved "Could not get any response".
– Colby Hunter
Nov 17 '18 at 11:11
1
It's not a cors issue then. What if you try using the machine IP address or 127.0.0.1 instead of localhost?
– David
Nov 17 '18 at 12:40
|
show 1 more comment
1
Can you try in chrome? You might get a more explicit error message. And can you post a screenshot of the network instpector, showing the response for the OPTIONS request?
– David
Nov 17 '18 at 8:46
Hey! Sorry for the delay:OPTIONS http://localhost:4000/business/add net::ERR_CONNECTION_REFUSED
is what Chrome shows. The network inspector will be posted in a moment.
– Colby Hunter
Nov 17 '18 at 10:27
1
Looks like you cannot even get a response from the API for the OPTIONS request. Just checking, does the API work with GET requests?
– David
Nov 17 '18 at 10:49
I set up thebusinessRoutes.route('/').get()
withres.json({'message': 'Testing'});
. I sent the request through Postman as a GET request forhttp://localhost:4000/business/
and recieved "Could not get any response".
– Colby Hunter
Nov 17 '18 at 11:11
1
It's not a cors issue then. What if you try using the machine IP address or 127.0.0.1 instead of localhost?
– David
Nov 17 '18 at 12:40
1
1
Can you try in chrome? You might get a more explicit error message. And can you post a screenshot of the network instpector, showing the response for the OPTIONS request?
– David
Nov 17 '18 at 8:46
Can you try in chrome? You might get a more explicit error message. And can you post a screenshot of the network instpector, showing the response for the OPTIONS request?
– David
Nov 17 '18 at 8:46
Hey! Sorry for the delay:
OPTIONS http://localhost:4000/business/add net::ERR_CONNECTION_REFUSED
is what Chrome shows. The network inspector will be posted in a moment.– Colby Hunter
Nov 17 '18 at 10:27
Hey! Sorry for the delay:
OPTIONS http://localhost:4000/business/add net::ERR_CONNECTION_REFUSED
is what Chrome shows. The network inspector will be posted in a moment.– Colby Hunter
Nov 17 '18 at 10:27
1
1
Looks like you cannot even get a response from the API for the OPTIONS request. Just checking, does the API work with GET requests?
– David
Nov 17 '18 at 10:49
Looks like you cannot even get a response from the API for the OPTIONS request. Just checking, does the API work with GET requests?
– David
Nov 17 '18 at 10:49
I set up the
businessRoutes.route('/').get()
with res.json({'message': 'Testing'});
. I sent the request through Postman as a GET request for http://localhost:4000/business/
and recieved "Could not get any response".– Colby Hunter
Nov 17 '18 at 11:11
I set up the
businessRoutes.route('/').get()
with res.json({'message': 'Testing'});
. I sent the request through Postman as a GET request for http://localhost:4000/business/
and recieved "Could not get any response".– Colby Hunter
Nov 17 '18 at 11:11
1
1
It's not a cors issue then. What if you try using the machine IP address or 127.0.0.1 instead of localhost?
– David
Nov 17 '18 at 12:40
It's not a cors issue then. What if you try using the machine IP address or 127.0.0.1 instead of localhost?
– David
Nov 17 '18 at 12:40
|
show 1 more comment
3 Answers
3
active
oldest
votes
I found the issue, thanks to David. The issue was in:
const server = app.listen(port, function(){
console.log('Listening on port ' + port);
});
I was missing port
in app.listen()
.
Thank you for everyone who has helped. I'm inclined to delete the question as it does not have to do with CORS at all, but I was given a warning about deleting questions.
A Request For The Community
Editing the question to something like "Why is Express refusing my API requests?" would be a more accurate question, but make the previously posted answers irrelevant. On top of this, Ebin's answer is unique to all the answers I have seen about the CORS issue, and believe it may help others.
For these reasons, I'm leaving it up to the community and how they feel about the question staying, and if they have any suggestions for improving it to make it worth staying.
add a comment |
I had a same error then I used this
app.use((req,res,next)=>{
res.header("Access-Control-Allow-Origin", "*");
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
if(req.method ==='OPTION'){
res.header("Access-Control-Allow-Methods", 'GET,POST,PUT,DELETE,PATCH');
return res.status(200).json({});
}
next();
});
then it was worked, try this
I used the code on its own and even placed the code in businessRoute.all('*', yourCode) and I'm still getting the same error.
– Colby Hunter
Nov 17 '18 at 9:09
add a comment |
Try removing const app = express();
from your business.route.js
and businessRoute.all('*', cors());
from your server.js
I'd use app.use(cors())
before anything else too
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53349575%2fwhat-is-causing-my-post-request-to-fail-cors-with-express-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I found the issue, thanks to David. The issue was in:
const server = app.listen(port, function(){
console.log('Listening on port ' + port);
});
I was missing port
in app.listen()
.
Thank you for everyone who has helped. I'm inclined to delete the question as it does not have to do with CORS at all, but I was given a warning about deleting questions.
A Request For The Community
Editing the question to something like "Why is Express refusing my API requests?" would be a more accurate question, but make the previously posted answers irrelevant. On top of this, Ebin's answer is unique to all the answers I have seen about the CORS issue, and believe it may help others.
For these reasons, I'm leaving it up to the community and how they feel about the question staying, and if they have any suggestions for improving it to make it worth staying.
add a comment |
I found the issue, thanks to David. The issue was in:
const server = app.listen(port, function(){
console.log('Listening on port ' + port);
});
I was missing port
in app.listen()
.
Thank you for everyone who has helped. I'm inclined to delete the question as it does not have to do with CORS at all, but I was given a warning about deleting questions.
A Request For The Community
Editing the question to something like "Why is Express refusing my API requests?" would be a more accurate question, but make the previously posted answers irrelevant. On top of this, Ebin's answer is unique to all the answers I have seen about the CORS issue, and believe it may help others.
For these reasons, I'm leaving it up to the community and how they feel about the question staying, and if they have any suggestions for improving it to make it worth staying.
add a comment |
I found the issue, thanks to David. The issue was in:
const server = app.listen(port, function(){
console.log('Listening on port ' + port);
});
I was missing port
in app.listen()
.
Thank you for everyone who has helped. I'm inclined to delete the question as it does not have to do with CORS at all, but I was given a warning about deleting questions.
A Request For The Community
Editing the question to something like "Why is Express refusing my API requests?" would be a more accurate question, but make the previously posted answers irrelevant. On top of this, Ebin's answer is unique to all the answers I have seen about the CORS issue, and believe it may help others.
For these reasons, I'm leaving it up to the community and how they feel about the question staying, and if they have any suggestions for improving it to make it worth staying.
I found the issue, thanks to David. The issue was in:
const server = app.listen(port, function(){
console.log('Listening on port ' + port);
});
I was missing port
in app.listen()
.
Thank you for everyone who has helped. I'm inclined to delete the question as it does not have to do with CORS at all, but I was given a warning about deleting questions.
A Request For The Community
Editing the question to something like "Why is Express refusing my API requests?" would be a more accurate question, but make the previously posted answers irrelevant. On top of this, Ebin's answer is unique to all the answers I have seen about the CORS issue, and believe it may help others.
For these reasons, I'm leaving it up to the community and how they feel about the question staying, and if they have any suggestions for improving it to make it worth staying.
answered Nov 17 '18 at 15:35
Colby Hunter
859
859
add a comment |
add a comment |
I had a same error then I used this
app.use((req,res,next)=>{
res.header("Access-Control-Allow-Origin", "*");
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
if(req.method ==='OPTION'){
res.header("Access-Control-Allow-Methods", 'GET,POST,PUT,DELETE,PATCH');
return res.status(200).json({});
}
next();
});
then it was worked, try this
I used the code on its own and even placed the code in businessRoute.all('*', yourCode) and I'm still getting the same error.
– Colby Hunter
Nov 17 '18 at 9:09
add a comment |
I had a same error then I used this
app.use((req,res,next)=>{
res.header("Access-Control-Allow-Origin", "*");
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
if(req.method ==='OPTION'){
res.header("Access-Control-Allow-Methods", 'GET,POST,PUT,DELETE,PATCH');
return res.status(200).json({});
}
next();
});
then it was worked, try this
I used the code on its own and even placed the code in businessRoute.all('*', yourCode) and I'm still getting the same error.
– Colby Hunter
Nov 17 '18 at 9:09
add a comment |
I had a same error then I used this
app.use((req,res,next)=>{
res.header("Access-Control-Allow-Origin", "*");
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
if(req.method ==='OPTION'){
res.header("Access-Control-Allow-Methods", 'GET,POST,PUT,DELETE,PATCH');
return res.status(200).json({});
}
next();
});
then it was worked, try this
I had a same error then I used this
app.use((req,res,next)=>{
res.header("Access-Control-Allow-Origin", "*");
res.header("Access-Control-Allow-Headers", "Origin, X-Requested-With, Content-Type, Accept");
if(req.method ==='OPTION'){
res.header("Access-Control-Allow-Methods", 'GET,POST,PUT,DELETE,PATCH');
return res.status(200).json({});
}
next();
});
then it was worked, try this
edited Nov 17 '18 at 8:46
Ebin Manuval
788822
788822
answered Nov 17 '18 at 8:42


Prabodha Ranasinghe
596
596
I used the code on its own and even placed the code in businessRoute.all('*', yourCode) and I'm still getting the same error.
– Colby Hunter
Nov 17 '18 at 9:09
add a comment |
I used the code on its own and even placed the code in businessRoute.all('*', yourCode) and I'm still getting the same error.
– Colby Hunter
Nov 17 '18 at 9:09
I used the code on its own and even placed the code in businessRoute.all('*', yourCode) and I'm still getting the same error.
– Colby Hunter
Nov 17 '18 at 9:09
I used the code on its own and even placed the code in businessRoute.all('*', yourCode) and I'm still getting the same error.
– Colby Hunter
Nov 17 '18 at 9:09
add a comment |
Try removing const app = express();
from your business.route.js
and businessRoute.all('*', cors());
from your server.js
I'd use app.use(cors())
before anything else too
add a comment |
Try removing const app = express();
from your business.route.js
and businessRoute.all('*', cors());
from your server.js
I'd use app.use(cors())
before anything else too
add a comment |
Try removing const app = express();
from your business.route.js
and businessRoute.all('*', cors());
from your server.js
I'd use app.use(cors())
before anything else too
Try removing const app = express();
from your business.route.js
and businessRoute.all('*', cors());
from your server.js
I'd use app.use(cors())
before anything else too
answered Nov 17 '18 at 12:13


Maty
67
67
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53349575%2fwhat-is-causing-my-post-request-to-fail-cors-with-express-angular%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Ik5B6SnZcu5uKHO ORyYujSKUHLBzSY8IR3
1
Can you try in chrome? You might get a more explicit error message. And can you post a screenshot of the network instpector, showing the response for the OPTIONS request?
– David
Nov 17 '18 at 8:46
Hey! Sorry for the delay:
OPTIONS http://localhost:4000/business/add net::ERR_CONNECTION_REFUSED
is what Chrome shows. The network inspector will be posted in a moment.– Colby Hunter
Nov 17 '18 at 10:27
1
Looks like you cannot even get a response from the API for the OPTIONS request. Just checking, does the API work with GET requests?
– David
Nov 17 '18 at 10:49
I set up the
businessRoutes.route('/').get()
withres.json({'message': 'Testing'});
. I sent the request through Postman as a GET request forhttp://localhost:4000/business/
and recieved "Could not get any response".– Colby Hunter
Nov 17 '18 at 11:11
1
It's not a cors issue then. What if you try using the machine IP address or 127.0.0.1 instead of localhost?
– David
Nov 17 '18 at 12:40