Crop and save facial landmarks using PIL
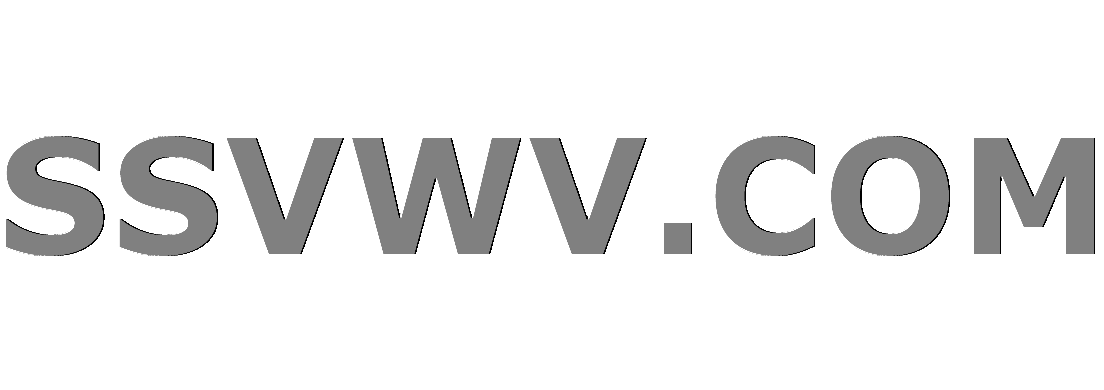
Multi tool use
I want to crop the top lip and bottom and save it into one image.
I am using this GitHub library face_recognition
This is the code:
from PIL import Image, ImageDraw
import face_recognition
# Load the jpg file into a numpy array
image = face_recognition.load_image_file("me.jpg")
# Find all facial features in all the faces in the image
face_landmarks_list = face_recognition.face_landmarks(image)
for face_landmarks in face_landmarks_list:
pil_image = Image.fromarray(image)
d = ImageDraw.Draw(pil_image, 'RGBA')
# Gloss the lips
d.polygon(face_landmarks['top_lip'], fill=(150, 0, 0, 128), outline=None)
d.polygon(face_landmarks['bottom_lip'], fill=(150, 0, 0, 128), outline=None)
d.line(face_landmarks['top_lip'], fill=(150, 0, 0, 64), width=8)
d.line(face_landmarks['bottom_lip'], fill=(150, 0, 0, 64), width=8)
# cropped_top_lip = image.crop(face_landmarks['top_lip'])
# cropped_top_lip.save('top_lip.jpg')
# cropped_bottom_lip = image.crop(face_landmarks['bottom_lip'])
# cropped_bottom_lip.save('bottom_lip.jpg')
pil_image.save('me2.jpg')
This return the full image, I want only the lips part.
This is a print of face_landmarks['top_lip']
:
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726,
922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942),
(516, 942)]
python image-processing face-recognition
add a comment |
I want to crop the top lip and bottom and save it into one image.
I am using this GitHub library face_recognition
This is the code:
from PIL import Image, ImageDraw
import face_recognition
# Load the jpg file into a numpy array
image = face_recognition.load_image_file("me.jpg")
# Find all facial features in all the faces in the image
face_landmarks_list = face_recognition.face_landmarks(image)
for face_landmarks in face_landmarks_list:
pil_image = Image.fromarray(image)
d = ImageDraw.Draw(pil_image, 'RGBA')
# Gloss the lips
d.polygon(face_landmarks['top_lip'], fill=(150, 0, 0, 128), outline=None)
d.polygon(face_landmarks['bottom_lip'], fill=(150, 0, 0, 128), outline=None)
d.line(face_landmarks['top_lip'], fill=(150, 0, 0, 64), width=8)
d.line(face_landmarks['bottom_lip'], fill=(150, 0, 0, 64), width=8)
# cropped_top_lip = image.crop(face_landmarks['top_lip'])
# cropped_top_lip.save('top_lip.jpg')
# cropped_bottom_lip = image.crop(face_landmarks['bottom_lip'])
# cropped_bottom_lip.save('bottom_lip.jpg')
pil_image.save('me2.jpg')
This return the full image, I want only the lips part.
This is a print of face_landmarks['top_lip']
:
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726,
922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942),
(516, 942)]
python image-processing face-recognition
add a comment |
I want to crop the top lip and bottom and save it into one image.
I am using this GitHub library face_recognition
This is the code:
from PIL import Image, ImageDraw
import face_recognition
# Load the jpg file into a numpy array
image = face_recognition.load_image_file("me.jpg")
# Find all facial features in all the faces in the image
face_landmarks_list = face_recognition.face_landmarks(image)
for face_landmarks in face_landmarks_list:
pil_image = Image.fromarray(image)
d = ImageDraw.Draw(pil_image, 'RGBA')
# Gloss the lips
d.polygon(face_landmarks['top_lip'], fill=(150, 0, 0, 128), outline=None)
d.polygon(face_landmarks['bottom_lip'], fill=(150, 0, 0, 128), outline=None)
d.line(face_landmarks['top_lip'], fill=(150, 0, 0, 64), width=8)
d.line(face_landmarks['bottom_lip'], fill=(150, 0, 0, 64), width=8)
# cropped_top_lip = image.crop(face_landmarks['top_lip'])
# cropped_top_lip.save('top_lip.jpg')
# cropped_bottom_lip = image.crop(face_landmarks['bottom_lip'])
# cropped_bottom_lip.save('bottom_lip.jpg')
pil_image.save('me2.jpg')
This return the full image, I want only the lips part.
This is a print of face_landmarks['top_lip']
:
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726,
922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942),
(516, 942)]
python image-processing face-recognition
I want to crop the top lip and bottom and save it into one image.
I am using this GitHub library face_recognition
This is the code:
from PIL import Image, ImageDraw
import face_recognition
# Load the jpg file into a numpy array
image = face_recognition.load_image_file("me.jpg")
# Find all facial features in all the faces in the image
face_landmarks_list = face_recognition.face_landmarks(image)
for face_landmarks in face_landmarks_list:
pil_image = Image.fromarray(image)
d = ImageDraw.Draw(pil_image, 'RGBA')
# Gloss the lips
d.polygon(face_landmarks['top_lip'], fill=(150, 0, 0, 128), outline=None)
d.polygon(face_landmarks['bottom_lip'], fill=(150, 0, 0, 128), outline=None)
d.line(face_landmarks['top_lip'], fill=(150, 0, 0, 64), width=8)
d.line(face_landmarks['bottom_lip'], fill=(150, 0, 0, 64), width=8)
# cropped_top_lip = image.crop(face_landmarks['top_lip'])
# cropped_top_lip.save('top_lip.jpg')
# cropped_bottom_lip = image.crop(face_landmarks['bottom_lip'])
# cropped_bottom_lip.save('bottom_lip.jpg')
pil_image.save('me2.jpg')
This return the full image, I want only the lips part.
This is a print of face_landmarks['top_lip']
:
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726,
922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942),
(516, 942)]
python image-processing face-recognition
python image-processing face-recognition
asked Nov 19 '18 at 7:59


RoyaumeIXRoyaumeIX
1,2491725
1,2491725
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The .crop
method takes a list containing 4 co-ordinates of the cropping area -
[
X_top_left_corner, Y_top_left_corner,
X_bottom_right_corner, Y_bottom_right_corner
]
What I can tell from the co-ordinates of face_landmarks['top_lip']
, I think, these are the points on the outline of the lip. So, you need to find the left-top-most corner, and right-bottom-most corner, and pass that to crop
method.
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726, 922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942), (516, 942)]
To find the top-left corner, you'll need to find the least X co-ordinate and the least Y co-ordinate from this data. And these two co-ordinates can be present in separate tuples.
For the bottom-right corner, you'll need the highest X co-ordinate and the highest y co-ordinate. Again, these two can be present in separate tuples.
You can determine the corners like this:
x1 = min(my_list)[0] # top left corner X
y1 = min(my_list, key=lambda pair: pair[1])[1] # top left corner Y
x2 = max(my_list)[0] # bottom right corner X
y2 = max(my_list, key=lambda pair: pair[1])[1] # bottom right corner Y
In this case the least X co-ordinate is 498
and the least Y co-ordinate is 922
. These will make your top-left corner.
The highest X co-ordinate is 772
and the highest Y co-ordinate is 946
. These will be your bottom-right corner.
This should work for cropping the top lip:
# im.crop([x1, y1, x2, y2])
im.crop([498, 922, 772, 946])
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53370471%2fcrop-and-save-facial-landmarks-using-pil%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The .crop
method takes a list containing 4 co-ordinates of the cropping area -
[
X_top_left_corner, Y_top_left_corner,
X_bottom_right_corner, Y_bottom_right_corner
]
What I can tell from the co-ordinates of face_landmarks['top_lip']
, I think, these are the points on the outline of the lip. So, you need to find the left-top-most corner, and right-bottom-most corner, and pass that to crop
method.
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726, 922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942), (516, 942)]
To find the top-left corner, you'll need to find the least X co-ordinate and the least Y co-ordinate from this data. And these two co-ordinates can be present in separate tuples.
For the bottom-right corner, you'll need the highest X co-ordinate and the highest y co-ordinate. Again, these two can be present in separate tuples.
You can determine the corners like this:
x1 = min(my_list)[0] # top left corner X
y1 = min(my_list, key=lambda pair: pair[1])[1] # top left corner Y
x2 = max(my_list)[0] # bottom right corner X
y2 = max(my_list, key=lambda pair: pair[1])[1] # bottom right corner Y
In this case the least X co-ordinate is 498
and the least Y co-ordinate is 922
. These will make your top-left corner.
The highest X co-ordinate is 772
and the highest Y co-ordinate is 946
. These will be your bottom-right corner.
This should work for cropping the top lip:
# im.crop([x1, y1, x2, y2])
im.crop([498, 922, 772, 946])
add a comment |
The .crop
method takes a list containing 4 co-ordinates of the cropping area -
[
X_top_left_corner, Y_top_left_corner,
X_bottom_right_corner, Y_bottom_right_corner
]
What I can tell from the co-ordinates of face_landmarks['top_lip']
, I think, these are the points on the outline of the lip. So, you need to find the left-top-most corner, and right-bottom-most corner, and pass that to crop
method.
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726, 922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942), (516, 942)]
To find the top-left corner, you'll need to find the least X co-ordinate and the least Y co-ordinate from this data. And these two co-ordinates can be present in separate tuples.
For the bottom-right corner, you'll need the highest X co-ordinate and the highest y co-ordinate. Again, these two can be present in separate tuples.
You can determine the corners like this:
x1 = min(my_list)[0] # top left corner X
y1 = min(my_list, key=lambda pair: pair[1])[1] # top left corner Y
x2 = max(my_list)[0] # bottom right corner X
y2 = max(my_list, key=lambda pair: pair[1])[1] # bottom right corner Y
In this case the least X co-ordinate is 498
and the least Y co-ordinate is 922
. These will make your top-left corner.
The highest X co-ordinate is 772
and the highest Y co-ordinate is 946
. These will be your bottom-right corner.
This should work for cropping the top lip:
# im.crop([x1, y1, x2, y2])
im.crop([498, 922, 772, 946])
add a comment |
The .crop
method takes a list containing 4 co-ordinates of the cropping area -
[
X_top_left_corner, Y_top_left_corner,
X_bottom_right_corner, Y_bottom_right_corner
]
What I can tell from the co-ordinates of face_landmarks['top_lip']
, I think, these are the points on the outline of the lip. So, you need to find the left-top-most corner, and right-bottom-most corner, and pass that to crop
method.
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726, 922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942), (516, 942)]
To find the top-left corner, you'll need to find the least X co-ordinate and the least Y co-ordinate from this data. And these two co-ordinates can be present in separate tuples.
For the bottom-right corner, you'll need the highest X co-ordinate and the highest y co-ordinate. Again, these two can be present in separate tuples.
You can determine the corners like this:
x1 = min(my_list)[0] # top left corner X
y1 = min(my_list, key=lambda pair: pair[1])[1] # top left corner Y
x2 = max(my_list)[0] # bottom right corner X
y2 = max(my_list, key=lambda pair: pair[1])[1] # bottom right corner Y
In this case the least X co-ordinate is 498
and the least Y co-ordinate is 922
. These will make your top-left corner.
The highest X co-ordinate is 772
and the highest Y co-ordinate is 946
. These will be your bottom-right corner.
This should work for cropping the top lip:
# im.crop([x1, y1, x2, y2])
im.crop([498, 922, 772, 946])
The .crop
method takes a list containing 4 co-ordinates of the cropping area -
[
X_top_left_corner, Y_top_left_corner,
X_bottom_right_corner, Y_bottom_right_corner
]
What I can tell from the co-ordinates of face_landmarks['top_lip']
, I think, these are the points on the outline of the lip. So, you need to find the left-top-most corner, and right-bottom-most corner, and pass that to crop
method.
[(498, 937), (546, 926), (597, 924), (637, 930), (676, 922), (726, 922), (772, 929), (756, 935), (677, 940), (637, 946), (597, 942), (516, 942)]
To find the top-left corner, you'll need to find the least X co-ordinate and the least Y co-ordinate from this data. And these two co-ordinates can be present in separate tuples.
For the bottom-right corner, you'll need the highest X co-ordinate and the highest y co-ordinate. Again, these two can be present in separate tuples.
You can determine the corners like this:
x1 = min(my_list)[0] # top left corner X
y1 = min(my_list, key=lambda pair: pair[1])[1] # top left corner Y
x2 = max(my_list)[0] # bottom right corner X
y2 = max(my_list, key=lambda pair: pair[1])[1] # bottom right corner Y
In this case the least X co-ordinate is 498
and the least Y co-ordinate is 922
. These will make your top-left corner.
The highest X co-ordinate is 772
and the highest Y co-ordinate is 946
. These will be your bottom-right corner.
This should work for cropping the top lip:
# im.crop([x1, y1, x2, y2])
im.crop([498, 922, 772, 946])
edited Nov 21 '18 at 6:13
answered Nov 19 '18 at 8:16


xyresxyres
9,28732345
9,28732345
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53370471%2fcrop-and-save-facial-landmarks-using-pil%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
bRU 0,sl E7lMQ7RTgd