iterating over arrays in MYSQL
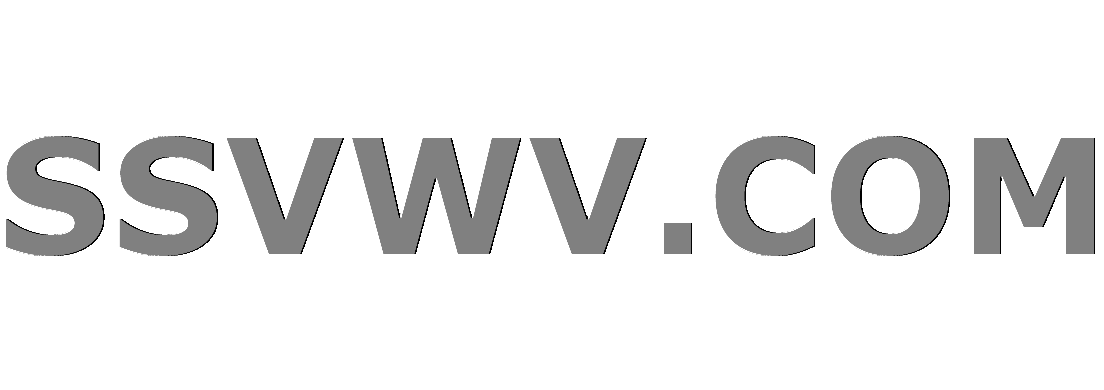
Multi tool use
up vote
0
down vote
favorite
Is there a way to pass an array into a MySQL query and return the results as another array ?(apart from using cursors which would be an overkill for my use case)
For a single id, my query looks like this.
SET @userId = '04b452cd59dcc656'
Select user_account_number from userstore where u_id = @userId ;
Instead of sending each id at a time, I am trying to send a list and return a list
SET @userId = ('04b452cd59dcc656','eqwe52cddasfsd656');
<query returning the list of account numbers>
Also - I think this would be efficient over just sending one id at a time. Thoughts ?
mysql sql
add a comment |
up vote
0
down vote
favorite
Is there a way to pass an array into a MySQL query and return the results as another array ?(apart from using cursors which would be an overkill for my use case)
For a single id, my query looks like this.
SET @userId = '04b452cd59dcc656'
Select user_account_number from userstore where u_id = @userId ;
Instead of sending each id at a time, I am trying to send a list and return a list
SET @userId = ('04b452cd59dcc656','eqwe52cddasfsd656');
<query returning the list of account numbers>
Also - I think this would be efficient over just sending one id at a time. Thoughts ?
mysql sql
Mysql does not implement any arrays. You can use string concatenation to dynamically create sql statements and to create concatenated, comma delimited output.
– Shadow
Nov 14 at 23:08
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Is there a way to pass an array into a MySQL query and return the results as another array ?(apart from using cursors which would be an overkill for my use case)
For a single id, my query looks like this.
SET @userId = '04b452cd59dcc656'
Select user_account_number from userstore where u_id = @userId ;
Instead of sending each id at a time, I am trying to send a list and return a list
SET @userId = ('04b452cd59dcc656','eqwe52cddasfsd656');
<query returning the list of account numbers>
Also - I think this would be efficient over just sending one id at a time. Thoughts ?
mysql sql
Is there a way to pass an array into a MySQL query and return the results as another array ?(apart from using cursors which would be an overkill for my use case)
For a single id, my query looks like this.
SET @userId = '04b452cd59dcc656'
Select user_account_number from userstore where u_id = @userId ;
Instead of sending each id at a time, I am trying to send a list and return a list
SET @userId = ('04b452cd59dcc656','eqwe52cddasfsd656');
<query returning the list of account numbers>
Also - I think this would be efficient over just sending one id at a time. Thoughts ?
mysql sql
mysql sql
asked Nov 14 at 22:52
user5566364
5618
5618
Mysql does not implement any arrays. You can use string concatenation to dynamically create sql statements and to create concatenated, comma delimited output.
– Shadow
Nov 14 at 23:08
add a comment |
Mysql does not implement any arrays. You can use string concatenation to dynamically create sql statements and to create concatenated, comma delimited output.
– Shadow
Nov 14 at 23:08
Mysql does not implement any arrays. You can use string concatenation to dynamically create sql statements and to create concatenated, comma delimited output.
– Shadow
Nov 14 at 23:08
Mysql does not implement any arrays. You can use string concatenation to dynamically create sql statements and to create concatenated, comma delimited output.
– Shadow
Nov 14 at 23:08
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
You can use IN
:
select user_account_number
from userstore
where u_id in ('04b452cd59dcc656', 'eqwe52cddasfsd656') ;
Using variables is trickier. If you know a maximum number, you can do:
select user_account_number
from userstore
where u_id in (@id1, @id2);
Not satisfying, but it does the job. Similarly unsatisfying is FIND_IN_SET()
:
set @ids = '04b452cd59dcc656,eqwe52cddasfsd656';
select user_account_number
from userstore
where find_in_set(u_id, @ids) > 0;
Alas, this won't use an index.
Finally there is dynamic SQL:
set @sql = concat('select user_account_number from userstore where u_id in (''',
replace(ids, ',', ''','''),
''')'
);
prepare s from @sql;
execute s;
Thanks. The first two does not work as the length of the array is not fixed and is not known to me before hand. The third one works. But , why do you call it 'not satisfying' ?And also did not follow your comment FIND_IN_SET is 'similarly unsatisfying'. Similarly unsatisfying to what ? I don't see anything else in that query that can be unsatisfying. :O
– user5566364
Nov 15 at 0:39
@user5566364 . . . Having to use dynamic SQL to solve this problem is a sad reality of the SQL language.
– Gordon Linoff
Nov 15 at 0:46
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
You can use IN
:
select user_account_number
from userstore
where u_id in ('04b452cd59dcc656', 'eqwe52cddasfsd656') ;
Using variables is trickier. If you know a maximum number, you can do:
select user_account_number
from userstore
where u_id in (@id1, @id2);
Not satisfying, but it does the job. Similarly unsatisfying is FIND_IN_SET()
:
set @ids = '04b452cd59dcc656,eqwe52cddasfsd656';
select user_account_number
from userstore
where find_in_set(u_id, @ids) > 0;
Alas, this won't use an index.
Finally there is dynamic SQL:
set @sql = concat('select user_account_number from userstore where u_id in (''',
replace(ids, ',', ''','''),
''')'
);
prepare s from @sql;
execute s;
Thanks. The first two does not work as the length of the array is not fixed and is not known to me before hand. The third one works. But , why do you call it 'not satisfying' ?And also did not follow your comment FIND_IN_SET is 'similarly unsatisfying'. Similarly unsatisfying to what ? I don't see anything else in that query that can be unsatisfying. :O
– user5566364
Nov 15 at 0:39
@user5566364 . . . Having to use dynamic SQL to solve this problem is a sad reality of the SQL language.
– Gordon Linoff
Nov 15 at 0:46
add a comment |
up vote
0
down vote
accepted
You can use IN
:
select user_account_number
from userstore
where u_id in ('04b452cd59dcc656', 'eqwe52cddasfsd656') ;
Using variables is trickier. If you know a maximum number, you can do:
select user_account_number
from userstore
where u_id in (@id1, @id2);
Not satisfying, but it does the job. Similarly unsatisfying is FIND_IN_SET()
:
set @ids = '04b452cd59dcc656,eqwe52cddasfsd656';
select user_account_number
from userstore
where find_in_set(u_id, @ids) > 0;
Alas, this won't use an index.
Finally there is dynamic SQL:
set @sql = concat('select user_account_number from userstore where u_id in (''',
replace(ids, ',', ''','''),
''')'
);
prepare s from @sql;
execute s;
Thanks. The first two does not work as the length of the array is not fixed and is not known to me before hand. The third one works. But , why do you call it 'not satisfying' ?And also did not follow your comment FIND_IN_SET is 'similarly unsatisfying'. Similarly unsatisfying to what ? I don't see anything else in that query that can be unsatisfying. :O
– user5566364
Nov 15 at 0:39
@user5566364 . . . Having to use dynamic SQL to solve this problem is a sad reality of the SQL language.
– Gordon Linoff
Nov 15 at 0:46
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
You can use IN
:
select user_account_number
from userstore
where u_id in ('04b452cd59dcc656', 'eqwe52cddasfsd656') ;
Using variables is trickier. If you know a maximum number, you can do:
select user_account_number
from userstore
where u_id in (@id1, @id2);
Not satisfying, but it does the job. Similarly unsatisfying is FIND_IN_SET()
:
set @ids = '04b452cd59dcc656,eqwe52cddasfsd656';
select user_account_number
from userstore
where find_in_set(u_id, @ids) > 0;
Alas, this won't use an index.
Finally there is dynamic SQL:
set @sql = concat('select user_account_number from userstore where u_id in (''',
replace(ids, ',', ''','''),
''')'
);
prepare s from @sql;
execute s;
You can use IN
:
select user_account_number
from userstore
where u_id in ('04b452cd59dcc656', 'eqwe52cddasfsd656') ;
Using variables is trickier. If you know a maximum number, you can do:
select user_account_number
from userstore
where u_id in (@id1, @id2);
Not satisfying, but it does the job. Similarly unsatisfying is FIND_IN_SET()
:
set @ids = '04b452cd59dcc656,eqwe52cddasfsd656';
select user_account_number
from userstore
where find_in_set(u_id, @ids) > 0;
Alas, this won't use an index.
Finally there is dynamic SQL:
set @sql = concat('select user_account_number from userstore where u_id in (''',
replace(ids, ',', ''','''),
''')'
);
prepare s from @sql;
execute s;
answered Nov 15 at 0:28
Gordon Linoff
751k34286394
751k34286394
Thanks. The first two does not work as the length of the array is not fixed and is not known to me before hand. The third one works. But , why do you call it 'not satisfying' ?And also did not follow your comment FIND_IN_SET is 'similarly unsatisfying'. Similarly unsatisfying to what ? I don't see anything else in that query that can be unsatisfying. :O
– user5566364
Nov 15 at 0:39
@user5566364 . . . Having to use dynamic SQL to solve this problem is a sad reality of the SQL language.
– Gordon Linoff
Nov 15 at 0:46
add a comment |
Thanks. The first two does not work as the length of the array is not fixed and is not known to me before hand. The third one works. But , why do you call it 'not satisfying' ?And also did not follow your comment FIND_IN_SET is 'similarly unsatisfying'. Similarly unsatisfying to what ? I don't see anything else in that query that can be unsatisfying. :O
– user5566364
Nov 15 at 0:39
@user5566364 . . . Having to use dynamic SQL to solve this problem is a sad reality of the SQL language.
– Gordon Linoff
Nov 15 at 0:46
Thanks. The first two does not work as the length of the array is not fixed and is not known to me before hand. The third one works. But , why do you call it 'not satisfying' ?And also did not follow your comment FIND_IN_SET is 'similarly unsatisfying'. Similarly unsatisfying to what ? I don't see anything else in that query that can be unsatisfying. :O
– user5566364
Nov 15 at 0:39
Thanks. The first two does not work as the length of the array is not fixed and is not known to me before hand. The third one works. But , why do you call it 'not satisfying' ?And also did not follow your comment FIND_IN_SET is 'similarly unsatisfying'. Similarly unsatisfying to what ? I don't see anything else in that query that can be unsatisfying. :O
– user5566364
Nov 15 at 0:39
@user5566364 . . . Having to use dynamic SQL to solve this problem is a sad reality of the SQL language.
– Gordon Linoff
Nov 15 at 0:46
@user5566364 . . . Having to use dynamic SQL to solve this problem is a sad reality of the SQL language.
– Gordon Linoff
Nov 15 at 0:46
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53309928%2fiterating-over-arrays-in-mysql%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
IvI0MGraAOnXGu3G r0UIu 9 qplWJRBvbZsQV5X6 8d5vtpAGn,0q,JT Q,VdIAsZi5PcvvhqlY8Brp4Fm
Mysql does not implement any arrays. You can use string concatenation to dynamically create sql statements and to create concatenated, comma delimited output.
– Shadow
Nov 14 at 23:08