What really happens when setText() is called on an EditText?
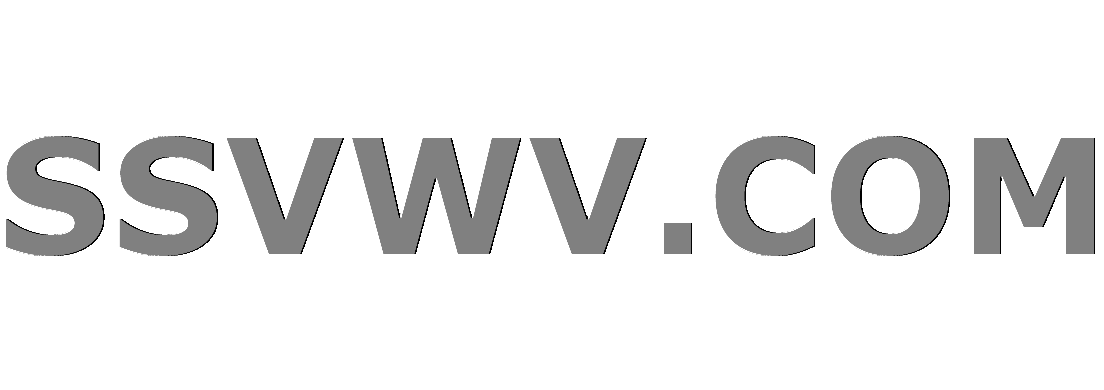
Multi tool use
up vote
1
down vote
favorite
This is a part of the code:
editText.setText("Some Text", TextView.BufferType.EDITABLE);
Editable editable = (Editable) editText.getText();
// value of editable.toString() here is "Some Text"
editText.setText("Another Text", TextView.BufferType.EDITABLE);
// value of editable.toString() is still "Some Text"
Why the value of editable.toString() did not change? Thanks


add a comment |
up vote
1
down vote
favorite
This is a part of the code:
editText.setText("Some Text", TextView.BufferType.EDITABLE);
Editable editable = (Editable) editText.getText();
// value of editable.toString() here is "Some Text"
editText.setText("Another Text", TextView.BufferType.EDITABLE);
// value of editable.toString() is still "Some Text"
Why the value of editable.toString() did not change? Thanks


add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
This is a part of the code:
editText.setText("Some Text", TextView.BufferType.EDITABLE);
Editable editable = (Editable) editText.getText();
// value of editable.toString() here is "Some Text"
editText.setText("Another Text", TextView.BufferType.EDITABLE);
// value of editable.toString() is still "Some Text"
Why the value of editable.toString() did not change? Thanks


This is a part of the code:
editText.setText("Some Text", TextView.BufferType.EDITABLE);
Editable editable = (Editable) editText.getText();
// value of editable.toString() here is "Some Text"
editText.setText("Another Text", TextView.BufferType.EDITABLE);
// value of editable.toString() is still "Some Text"
Why the value of editable.toString() did not change? Thanks




asked Nov 13 at 15:33
H. Riantsoa
326
326
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
You assigned editText.getText()
to a variable. That means its value won't change.
When you call setText()
, the original text is overwritten with the new CharSequence; the original instance of the Editable that getText()
returns is no longer part of the TextView, so your editable
variable is no longer attached to the TextView.
Take a look at TextView's getEditableText()
(this is what EditText calls from getText()
):
public Editable getEditableText() {
return (mText instanceof Editable) ? (Editable) mText : null;
}
If mText
is an Editable Object, then it'll return it. Otherwise, it'll return null
.
setText()
eventually makes its way to setTextInternal()
:
private void setTextInternal(@Nullable CharSequence text) {
mText = text;
mSpannable = (text instanceof Spannable) ? (Spannable) text : null;
mPrecomputed = (text instanceof PrecomputedText) ? (PrecomputedText) text : null;
}
As you can see, it just overwrites the mText
field, meaning your Editable instance is no longer the instance that the EditText has.
TextView.java
I agree with most of this answer, but simply assigning a value to a variable doesn't mean that the value will never change.SpannableStringBuilder
implementsEditable
, and so the results of itstoString()
method can change over time.
– Ben P.
Nov 13 at 15:46
@BenP. yes, but OP isn't using a SpannableStringBuilder. OP is using an EditText, which does overwrite the variable returned bygetText()
. That means that theeditable
variable OP has won't change whensetText()
is called.
– TheWanderer
Nov 13 at 15:50
My point is just that the blanket statement "You assigned X to a variable, therefore X's value won't change" is not true in general. It may be true in this case, but it would be a bad lesson to "learn" for java programming in general.
– Ben P.
Nov 13 at 15:53
It wasn't meant as a blanket statement.
– TheWanderer
Nov 13 at 15:54
` Editable t = mEditableFactory.newEditable(text); text = t; ` also helped me a lot. Thanks
– H. Riantsoa
Nov 13 at 16:54
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
You assigned editText.getText()
to a variable. That means its value won't change.
When you call setText()
, the original text is overwritten with the new CharSequence; the original instance of the Editable that getText()
returns is no longer part of the TextView, so your editable
variable is no longer attached to the TextView.
Take a look at TextView's getEditableText()
(this is what EditText calls from getText()
):
public Editable getEditableText() {
return (mText instanceof Editable) ? (Editable) mText : null;
}
If mText
is an Editable Object, then it'll return it. Otherwise, it'll return null
.
setText()
eventually makes its way to setTextInternal()
:
private void setTextInternal(@Nullable CharSequence text) {
mText = text;
mSpannable = (text instanceof Spannable) ? (Spannable) text : null;
mPrecomputed = (text instanceof PrecomputedText) ? (PrecomputedText) text : null;
}
As you can see, it just overwrites the mText
field, meaning your Editable instance is no longer the instance that the EditText has.
TextView.java
I agree with most of this answer, but simply assigning a value to a variable doesn't mean that the value will never change.SpannableStringBuilder
implementsEditable
, and so the results of itstoString()
method can change over time.
– Ben P.
Nov 13 at 15:46
@BenP. yes, but OP isn't using a SpannableStringBuilder. OP is using an EditText, which does overwrite the variable returned bygetText()
. That means that theeditable
variable OP has won't change whensetText()
is called.
– TheWanderer
Nov 13 at 15:50
My point is just that the blanket statement "You assigned X to a variable, therefore X's value won't change" is not true in general. It may be true in this case, but it would be a bad lesson to "learn" for java programming in general.
– Ben P.
Nov 13 at 15:53
It wasn't meant as a blanket statement.
– TheWanderer
Nov 13 at 15:54
` Editable t = mEditableFactory.newEditable(text); text = t; ` also helped me a lot. Thanks
– H. Riantsoa
Nov 13 at 16:54
add a comment |
up vote
2
down vote
accepted
You assigned editText.getText()
to a variable. That means its value won't change.
When you call setText()
, the original text is overwritten with the new CharSequence; the original instance of the Editable that getText()
returns is no longer part of the TextView, so your editable
variable is no longer attached to the TextView.
Take a look at TextView's getEditableText()
(this is what EditText calls from getText()
):
public Editable getEditableText() {
return (mText instanceof Editable) ? (Editable) mText : null;
}
If mText
is an Editable Object, then it'll return it. Otherwise, it'll return null
.
setText()
eventually makes its way to setTextInternal()
:
private void setTextInternal(@Nullable CharSequence text) {
mText = text;
mSpannable = (text instanceof Spannable) ? (Spannable) text : null;
mPrecomputed = (text instanceof PrecomputedText) ? (PrecomputedText) text : null;
}
As you can see, it just overwrites the mText
field, meaning your Editable instance is no longer the instance that the EditText has.
TextView.java
I agree with most of this answer, but simply assigning a value to a variable doesn't mean that the value will never change.SpannableStringBuilder
implementsEditable
, and so the results of itstoString()
method can change over time.
– Ben P.
Nov 13 at 15:46
@BenP. yes, but OP isn't using a SpannableStringBuilder. OP is using an EditText, which does overwrite the variable returned bygetText()
. That means that theeditable
variable OP has won't change whensetText()
is called.
– TheWanderer
Nov 13 at 15:50
My point is just that the blanket statement "You assigned X to a variable, therefore X's value won't change" is not true in general. It may be true in this case, but it would be a bad lesson to "learn" for java programming in general.
– Ben P.
Nov 13 at 15:53
It wasn't meant as a blanket statement.
– TheWanderer
Nov 13 at 15:54
` Editable t = mEditableFactory.newEditable(text); text = t; ` also helped me a lot. Thanks
– H. Riantsoa
Nov 13 at 16:54
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
You assigned editText.getText()
to a variable. That means its value won't change.
When you call setText()
, the original text is overwritten with the new CharSequence; the original instance of the Editable that getText()
returns is no longer part of the TextView, so your editable
variable is no longer attached to the TextView.
Take a look at TextView's getEditableText()
(this is what EditText calls from getText()
):
public Editable getEditableText() {
return (mText instanceof Editable) ? (Editable) mText : null;
}
If mText
is an Editable Object, then it'll return it. Otherwise, it'll return null
.
setText()
eventually makes its way to setTextInternal()
:
private void setTextInternal(@Nullable CharSequence text) {
mText = text;
mSpannable = (text instanceof Spannable) ? (Spannable) text : null;
mPrecomputed = (text instanceof PrecomputedText) ? (PrecomputedText) text : null;
}
As you can see, it just overwrites the mText
field, meaning your Editable instance is no longer the instance that the EditText has.
TextView.java
You assigned editText.getText()
to a variable. That means its value won't change.
When you call setText()
, the original text is overwritten with the new CharSequence; the original instance of the Editable that getText()
returns is no longer part of the TextView, so your editable
variable is no longer attached to the TextView.
Take a look at TextView's getEditableText()
(this is what EditText calls from getText()
):
public Editable getEditableText() {
return (mText instanceof Editable) ? (Editable) mText : null;
}
If mText
is an Editable Object, then it'll return it. Otherwise, it'll return null
.
setText()
eventually makes its way to setTextInternal()
:
private void setTextInternal(@Nullable CharSequence text) {
mText = text;
mSpannable = (text instanceof Spannable) ? (Spannable) text : null;
mPrecomputed = (text instanceof PrecomputedText) ? (PrecomputedText) text : null;
}
As you can see, it just overwrites the mText
field, meaning your Editable instance is no longer the instance that the EditText has.
TextView.java
answered Nov 13 at 15:40


TheWanderer
5,73611026
5,73611026
I agree with most of this answer, but simply assigning a value to a variable doesn't mean that the value will never change.SpannableStringBuilder
implementsEditable
, and so the results of itstoString()
method can change over time.
– Ben P.
Nov 13 at 15:46
@BenP. yes, but OP isn't using a SpannableStringBuilder. OP is using an EditText, which does overwrite the variable returned bygetText()
. That means that theeditable
variable OP has won't change whensetText()
is called.
– TheWanderer
Nov 13 at 15:50
My point is just that the blanket statement "You assigned X to a variable, therefore X's value won't change" is not true in general. It may be true in this case, but it would be a bad lesson to "learn" for java programming in general.
– Ben P.
Nov 13 at 15:53
It wasn't meant as a blanket statement.
– TheWanderer
Nov 13 at 15:54
` Editable t = mEditableFactory.newEditable(text); text = t; ` also helped me a lot. Thanks
– H. Riantsoa
Nov 13 at 16:54
add a comment |
I agree with most of this answer, but simply assigning a value to a variable doesn't mean that the value will never change.SpannableStringBuilder
implementsEditable
, and so the results of itstoString()
method can change over time.
– Ben P.
Nov 13 at 15:46
@BenP. yes, but OP isn't using a SpannableStringBuilder. OP is using an EditText, which does overwrite the variable returned bygetText()
. That means that theeditable
variable OP has won't change whensetText()
is called.
– TheWanderer
Nov 13 at 15:50
My point is just that the blanket statement "You assigned X to a variable, therefore X's value won't change" is not true in general. It may be true in this case, but it would be a bad lesson to "learn" for java programming in general.
– Ben P.
Nov 13 at 15:53
It wasn't meant as a blanket statement.
– TheWanderer
Nov 13 at 15:54
` Editable t = mEditableFactory.newEditable(text); text = t; ` also helped me a lot. Thanks
– H. Riantsoa
Nov 13 at 16:54
I agree with most of this answer, but simply assigning a value to a variable doesn't mean that the value will never change.
SpannableStringBuilder
implements Editable
, and so the results of its toString()
method can change over time.– Ben P.
Nov 13 at 15:46
I agree with most of this answer, but simply assigning a value to a variable doesn't mean that the value will never change.
SpannableStringBuilder
implements Editable
, and so the results of its toString()
method can change over time.– Ben P.
Nov 13 at 15:46
@BenP. yes, but OP isn't using a SpannableStringBuilder. OP is using an EditText, which does overwrite the variable returned by
getText()
. That means that the editable
variable OP has won't change when setText()
is called.– TheWanderer
Nov 13 at 15:50
@BenP. yes, but OP isn't using a SpannableStringBuilder. OP is using an EditText, which does overwrite the variable returned by
getText()
. That means that the editable
variable OP has won't change when setText()
is called.– TheWanderer
Nov 13 at 15:50
My point is just that the blanket statement "You assigned X to a variable, therefore X's value won't change" is not true in general. It may be true in this case, but it would be a bad lesson to "learn" for java programming in general.
– Ben P.
Nov 13 at 15:53
My point is just that the blanket statement "You assigned X to a variable, therefore X's value won't change" is not true in general. It may be true in this case, but it would be a bad lesson to "learn" for java programming in general.
– Ben P.
Nov 13 at 15:53
It wasn't meant as a blanket statement.
– TheWanderer
Nov 13 at 15:54
It wasn't meant as a blanket statement.
– TheWanderer
Nov 13 at 15:54
` Editable t = mEditableFactory.newEditable(text); text = t; ` also helped me a lot. Thanks
– H. Riantsoa
Nov 13 at 16:54
` Editable t = mEditableFactory.newEditable(text); text = t; ` also helped me a lot. Thanks
– H. Riantsoa
Nov 13 at 16:54
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53284390%2fwhat-really-happens-when-settext-is-called-on-an-edittext%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3x2,rbZvgWq R V5gk WkejyJFZfQ3,0 pUfR,kosPBPrAvSwIdXHI