Problems loading view with jquery in php MVC framework
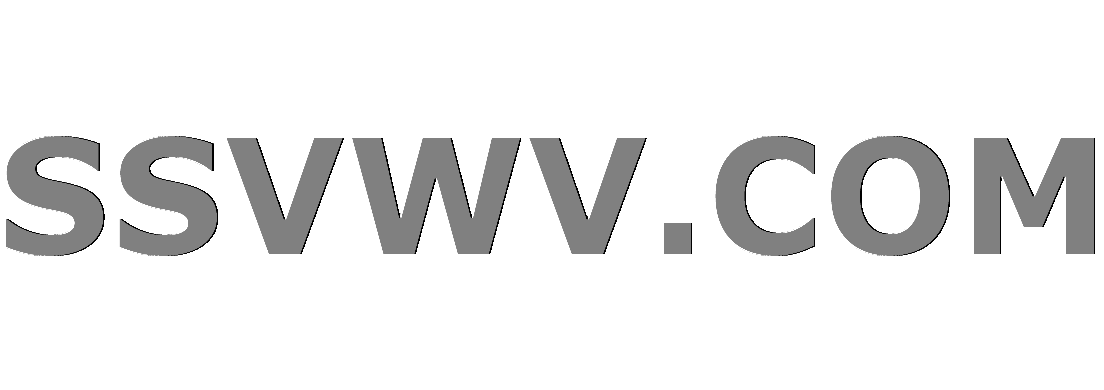
Multi tool use
up vote
0
down vote
favorite
I am trying to develop my first "big project" in php. I used the simple open-source MVC framework provided by traversy. You can download it here
I decided to use a lot of ajax & jquery because of the nature of the project. I am getting to a point where I need to reload small parts of the page whithout refreshing the whole page. That's why I use the .load()
jquery function. The part I want to load is in my app/views folder & needs to access php $_SESSION
variables (it's not a static view and I don't want to store it in the 'public' folder even though it would kind of work this way).
The problem is that the view is not loading because of how the framework is made (loading controllers based on urls). Here is the 'Core.php' file of the framework :
<?php
/*
* App Core Class
* Creates URL & loads core controller
* URL FORMAT - /controller/method/params
*/
class Core {
protected $currentController = 'Pages';
protected $currentMethod = 'index';
protected $params = ;
public function __construct(){
$url = $this->getUrl();
// Look in controllers for first value
if(file_exists('../app/controllers/' . ucwords($url[0]). '.php')){
// If exists, set as controller
$this->currentController = ucwords($url[0]);
// Unset 0 Index
unset($url[0]);
}
// Require the controller
require_once '../app/controllers/'. $this->currentController . '.php';
// Instantiate controller class
$this->currentController = new $this->currentController;
// Check for second part of url
if(isset($url[1])){
// Check to see if method exists in controller
$url[1] = dashesToCamelCase($url[1]);
if(method_exists($this->currentController, $url[1])){
$this->currentMethod = $url[1];
// Unset 1 index
unset($url[1]);
}
}
// Get params
$this->params = $url ? array_values($url) : ;
// Call a callback with array of params
call_user_func_array([$this->currentController, $this->currentMethod], $this->params);
}
public function getUrl(){
if(isset($_GET['url'])){
$url = rtrim($_GET['url'], '/');
$url = filter_var($url, FILTER_SANITIZE_URL);
$url = explode('/', $url);
return $url;
}
}
}
Here is the 'Pages.php' controller :
<?php
class Pages extends Controller {
public function __construct(){
}
public function index(){
/*$data = [
'title' => 'Welcome'
];*/
if(isLoggedIn()){
redirect('projects');
} else {
redirect('users/login');
}
$this->view('pages/index', $data);
}
public function about(){
$data = [
'title' => 'About Us'
];
$this->view('pages/about', $data);
}
public function newProject(){
$data = [
'title' => 'About Us'
];
$this->view('cdp/new-project', $data);
}
}
?>
And here is the Controller.php file :
<?php
/*
* Base Controller
* Loads the models and views
*/
class Controller {
// Load model
public function model($model){
// Require model file
require_once '../app/models/' . $model . '.php';
// Instatiate model
return new $model();
}
// Load view
public function view($view, $data = ){
// Check for view file
if(file_exists('../app/views/' . $view . '.php')){
require_once '../app/views/' . $view . '.php';
} else {
// View does not exist
die('View does not exist');
}
}
}
The problem obviously happens in the index()
function in the Pages.php file since the loaded part displays the 'projects' page. But that's because it is the default behaviour if the controller file doesn't exist (Core.php).
How to change this behaviour and prevent the redirect ? Maybe I need to rewrite Core.php or Pages.php completely but I have no idea how since the framework part was a tutorial.
I hope you have enough infos, tried to be as clear as possible.
Thanks.
php jquery model-view-controller
add a comment |
up vote
0
down vote
favorite
I am trying to develop my first "big project" in php. I used the simple open-source MVC framework provided by traversy. You can download it here
I decided to use a lot of ajax & jquery because of the nature of the project. I am getting to a point where I need to reload small parts of the page whithout refreshing the whole page. That's why I use the .load()
jquery function. The part I want to load is in my app/views folder & needs to access php $_SESSION
variables (it's not a static view and I don't want to store it in the 'public' folder even though it would kind of work this way).
The problem is that the view is not loading because of how the framework is made (loading controllers based on urls). Here is the 'Core.php' file of the framework :
<?php
/*
* App Core Class
* Creates URL & loads core controller
* URL FORMAT - /controller/method/params
*/
class Core {
protected $currentController = 'Pages';
protected $currentMethod = 'index';
protected $params = ;
public function __construct(){
$url = $this->getUrl();
// Look in controllers for first value
if(file_exists('../app/controllers/' . ucwords($url[0]). '.php')){
// If exists, set as controller
$this->currentController = ucwords($url[0]);
// Unset 0 Index
unset($url[0]);
}
// Require the controller
require_once '../app/controllers/'. $this->currentController . '.php';
// Instantiate controller class
$this->currentController = new $this->currentController;
// Check for second part of url
if(isset($url[1])){
// Check to see if method exists in controller
$url[1] = dashesToCamelCase($url[1]);
if(method_exists($this->currentController, $url[1])){
$this->currentMethod = $url[1];
// Unset 1 index
unset($url[1]);
}
}
// Get params
$this->params = $url ? array_values($url) : ;
// Call a callback with array of params
call_user_func_array([$this->currentController, $this->currentMethod], $this->params);
}
public function getUrl(){
if(isset($_GET['url'])){
$url = rtrim($_GET['url'], '/');
$url = filter_var($url, FILTER_SANITIZE_URL);
$url = explode('/', $url);
return $url;
}
}
}
Here is the 'Pages.php' controller :
<?php
class Pages extends Controller {
public function __construct(){
}
public function index(){
/*$data = [
'title' => 'Welcome'
];*/
if(isLoggedIn()){
redirect('projects');
} else {
redirect('users/login');
}
$this->view('pages/index', $data);
}
public function about(){
$data = [
'title' => 'About Us'
];
$this->view('pages/about', $data);
}
public function newProject(){
$data = [
'title' => 'About Us'
];
$this->view('cdp/new-project', $data);
}
}
?>
And here is the Controller.php file :
<?php
/*
* Base Controller
* Loads the models and views
*/
class Controller {
// Load model
public function model($model){
// Require model file
require_once '../app/models/' . $model . '.php';
// Instatiate model
return new $model();
}
// Load view
public function view($view, $data = ){
// Check for view file
if(file_exists('../app/views/' . $view . '.php')){
require_once '../app/views/' . $view . '.php';
} else {
// View does not exist
die('View does not exist');
}
}
}
The problem obviously happens in the index()
function in the Pages.php file since the loaded part displays the 'projects' page. But that's because it is the default behaviour if the controller file doesn't exist (Core.php).
How to change this behaviour and prevent the redirect ? Maybe I need to rewrite Core.php or Pages.php completely but I have no idea how since the framework part was a tutorial.
I hope you have enough infos, tried to be as clear as possible.
Thanks.
php jquery model-view-controller
How do you pass url to jQuery load() function?
– Rüzgar
Nov 13 at 15:08
With something like this :$('.selector').load('views/inc/partIwantToLoad.php')
(I do this in asucccess:
after ajax call but maybe that's not relevant) And BTW, initially (before jquery/ajax calls), I call this same view with arequire()
& it's working fine.
– Guillaume Jarry
Nov 13 at 15:13
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am trying to develop my first "big project" in php. I used the simple open-source MVC framework provided by traversy. You can download it here
I decided to use a lot of ajax & jquery because of the nature of the project. I am getting to a point where I need to reload small parts of the page whithout refreshing the whole page. That's why I use the .load()
jquery function. The part I want to load is in my app/views folder & needs to access php $_SESSION
variables (it's not a static view and I don't want to store it in the 'public' folder even though it would kind of work this way).
The problem is that the view is not loading because of how the framework is made (loading controllers based on urls). Here is the 'Core.php' file of the framework :
<?php
/*
* App Core Class
* Creates URL & loads core controller
* URL FORMAT - /controller/method/params
*/
class Core {
protected $currentController = 'Pages';
protected $currentMethod = 'index';
protected $params = ;
public function __construct(){
$url = $this->getUrl();
// Look in controllers for first value
if(file_exists('../app/controllers/' . ucwords($url[0]). '.php')){
// If exists, set as controller
$this->currentController = ucwords($url[0]);
// Unset 0 Index
unset($url[0]);
}
// Require the controller
require_once '../app/controllers/'. $this->currentController . '.php';
// Instantiate controller class
$this->currentController = new $this->currentController;
// Check for second part of url
if(isset($url[1])){
// Check to see if method exists in controller
$url[1] = dashesToCamelCase($url[1]);
if(method_exists($this->currentController, $url[1])){
$this->currentMethod = $url[1];
// Unset 1 index
unset($url[1]);
}
}
// Get params
$this->params = $url ? array_values($url) : ;
// Call a callback with array of params
call_user_func_array([$this->currentController, $this->currentMethod], $this->params);
}
public function getUrl(){
if(isset($_GET['url'])){
$url = rtrim($_GET['url'], '/');
$url = filter_var($url, FILTER_SANITIZE_URL);
$url = explode('/', $url);
return $url;
}
}
}
Here is the 'Pages.php' controller :
<?php
class Pages extends Controller {
public function __construct(){
}
public function index(){
/*$data = [
'title' => 'Welcome'
];*/
if(isLoggedIn()){
redirect('projects');
} else {
redirect('users/login');
}
$this->view('pages/index', $data);
}
public function about(){
$data = [
'title' => 'About Us'
];
$this->view('pages/about', $data);
}
public function newProject(){
$data = [
'title' => 'About Us'
];
$this->view('cdp/new-project', $data);
}
}
?>
And here is the Controller.php file :
<?php
/*
* Base Controller
* Loads the models and views
*/
class Controller {
// Load model
public function model($model){
// Require model file
require_once '../app/models/' . $model . '.php';
// Instatiate model
return new $model();
}
// Load view
public function view($view, $data = ){
// Check for view file
if(file_exists('../app/views/' . $view . '.php')){
require_once '../app/views/' . $view . '.php';
} else {
// View does not exist
die('View does not exist');
}
}
}
The problem obviously happens in the index()
function in the Pages.php file since the loaded part displays the 'projects' page. But that's because it is the default behaviour if the controller file doesn't exist (Core.php).
How to change this behaviour and prevent the redirect ? Maybe I need to rewrite Core.php or Pages.php completely but I have no idea how since the framework part was a tutorial.
I hope you have enough infos, tried to be as clear as possible.
Thanks.
php jquery model-view-controller
I am trying to develop my first "big project" in php. I used the simple open-source MVC framework provided by traversy. You can download it here
I decided to use a lot of ajax & jquery because of the nature of the project. I am getting to a point where I need to reload small parts of the page whithout refreshing the whole page. That's why I use the .load()
jquery function. The part I want to load is in my app/views folder & needs to access php $_SESSION
variables (it's not a static view and I don't want to store it in the 'public' folder even though it would kind of work this way).
The problem is that the view is not loading because of how the framework is made (loading controllers based on urls). Here is the 'Core.php' file of the framework :
<?php
/*
* App Core Class
* Creates URL & loads core controller
* URL FORMAT - /controller/method/params
*/
class Core {
protected $currentController = 'Pages';
protected $currentMethod = 'index';
protected $params = ;
public function __construct(){
$url = $this->getUrl();
// Look in controllers for first value
if(file_exists('../app/controllers/' . ucwords($url[0]). '.php')){
// If exists, set as controller
$this->currentController = ucwords($url[0]);
// Unset 0 Index
unset($url[0]);
}
// Require the controller
require_once '../app/controllers/'. $this->currentController . '.php';
// Instantiate controller class
$this->currentController = new $this->currentController;
// Check for second part of url
if(isset($url[1])){
// Check to see if method exists in controller
$url[1] = dashesToCamelCase($url[1]);
if(method_exists($this->currentController, $url[1])){
$this->currentMethod = $url[1];
// Unset 1 index
unset($url[1]);
}
}
// Get params
$this->params = $url ? array_values($url) : ;
// Call a callback with array of params
call_user_func_array([$this->currentController, $this->currentMethod], $this->params);
}
public function getUrl(){
if(isset($_GET['url'])){
$url = rtrim($_GET['url'], '/');
$url = filter_var($url, FILTER_SANITIZE_URL);
$url = explode('/', $url);
return $url;
}
}
}
Here is the 'Pages.php' controller :
<?php
class Pages extends Controller {
public function __construct(){
}
public function index(){
/*$data = [
'title' => 'Welcome'
];*/
if(isLoggedIn()){
redirect('projects');
} else {
redirect('users/login');
}
$this->view('pages/index', $data);
}
public function about(){
$data = [
'title' => 'About Us'
];
$this->view('pages/about', $data);
}
public function newProject(){
$data = [
'title' => 'About Us'
];
$this->view('cdp/new-project', $data);
}
}
?>
And here is the Controller.php file :
<?php
/*
* Base Controller
* Loads the models and views
*/
class Controller {
// Load model
public function model($model){
// Require model file
require_once '../app/models/' . $model . '.php';
// Instatiate model
return new $model();
}
// Load view
public function view($view, $data = ){
// Check for view file
if(file_exists('../app/views/' . $view . '.php')){
require_once '../app/views/' . $view . '.php';
} else {
// View does not exist
die('View does not exist');
}
}
}
The problem obviously happens in the index()
function in the Pages.php file since the loaded part displays the 'projects' page. But that's because it is the default behaviour if the controller file doesn't exist (Core.php).
How to change this behaviour and prevent the redirect ? Maybe I need to rewrite Core.php or Pages.php completely but I have no idea how since the framework part was a tutorial.
I hope you have enough infos, tried to be as clear as possible.
Thanks.
php jquery model-view-controller
php jquery model-view-controller
edited Nov 13 at 15:11
asked Nov 13 at 14:33
Guillaume Jarry
12
12
How do you pass url to jQuery load() function?
– Rüzgar
Nov 13 at 15:08
With something like this :$('.selector').load('views/inc/partIwantToLoad.php')
(I do this in asucccess:
after ajax call but maybe that's not relevant) And BTW, initially (before jquery/ajax calls), I call this same view with arequire()
& it's working fine.
– Guillaume Jarry
Nov 13 at 15:13
add a comment |
How do you pass url to jQuery load() function?
– Rüzgar
Nov 13 at 15:08
With something like this :$('.selector').load('views/inc/partIwantToLoad.php')
(I do this in asucccess:
after ajax call but maybe that's not relevant) And BTW, initially (before jquery/ajax calls), I call this same view with arequire()
& it's working fine.
– Guillaume Jarry
Nov 13 at 15:13
How do you pass url to jQuery load() function?
– Rüzgar
Nov 13 at 15:08
How do you pass url to jQuery load() function?
– Rüzgar
Nov 13 at 15:08
With something like this :
$('.selector').load('views/inc/partIwantToLoad.php')
(I do this in a succcess:
after ajax call but maybe that's not relevant) And BTW, initially (before jquery/ajax calls), I call this same view with a require()
& it's working fine.– Guillaume Jarry
Nov 13 at 15:13
With something like this :
$('.selector').load('views/inc/partIwantToLoad.php')
(I do this in a succcess:
after ajax call but maybe that's not relevant) And BTW, initially (before jquery/ajax calls), I call this same view with a require()
& it's working fine.– Guillaume Jarry
Nov 13 at 15:13
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53283318%2fproblems-loading-view-with-jquery-in-php-mvc-framework%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
srF BL0V0 lpXyqVHMr6dy82xTE vSM,CQ3X8Hz8 aoc2xFwHKBX9hGCKZ,6
How do you pass url to jQuery load() function?
– Rüzgar
Nov 13 at 15:08
With something like this :
$('.selector').load('views/inc/partIwantToLoad.php')
(I do this in asucccess:
after ajax call but maybe that's not relevant) And BTW, initially (before jquery/ajax calls), I call this same view with arequire()
& it's working fine.– Guillaume Jarry
Nov 13 at 15:13