Evaluating polynomial with function
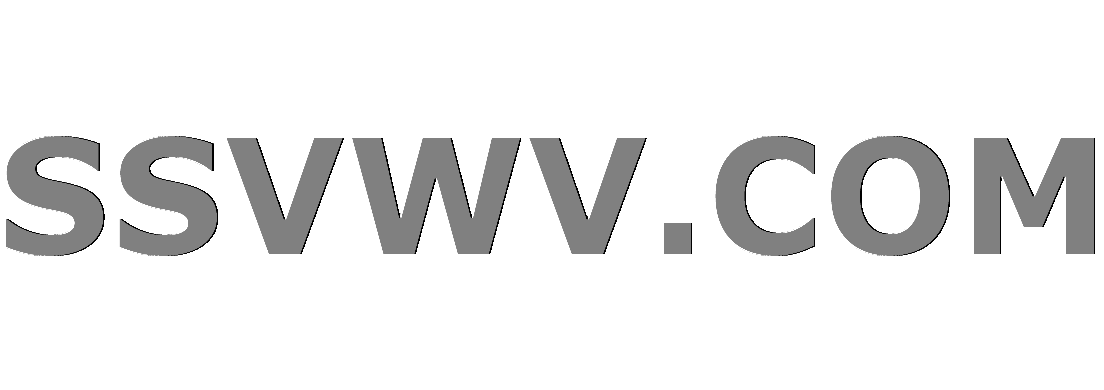
Multi tool use
up vote
0
down vote
favorite
Im trying to evaluate a polynomial P(x):
However i dont think im entering the polynomial itself correctly in my function.
Code for function:
directpoly1 <- function(x, coef, seqcoef = seq(coef) - 1) {
sum(coef*x^seqcoef)
}
directpoly <- function(x, coef) {
seqcoef <- seq(coef) - 1
sapply(x, directpoly1, coef, seqcoef)
}
Code for using function:
directpoly(x=seq(-10,10, length=5000000), rep(c(2,-1),20))
Any ideas on how to enter it correctly?
r polynomials
add a comment |
up vote
0
down vote
favorite
Im trying to evaluate a polynomial P(x):
However i dont think im entering the polynomial itself correctly in my function.
Code for function:
directpoly1 <- function(x, coef, seqcoef = seq(coef) - 1) {
sum(coef*x^seqcoef)
}
directpoly <- function(x, coef) {
seqcoef <- seq(coef) - 1
sapply(x, directpoly1, coef, seqcoef)
}
Code for using function:
directpoly(x=seq(-10,10, length=5000000), rep(c(2,-1),20))
Any ideas on how to enter it correctly?
r polynomials
1
Tryseqcoef = seq_along(coef)
. This means you will need to include the zero coefficients in the vectorcoef
.
– Rui Barradas
Nov 13 at 12:22
Thx! Can you see if im using the function correctly, so when evaluating P(x) with my function, is P(x) then correctly typed in?
– torvin
Nov 13 at 12:27
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Im trying to evaluate a polynomial P(x):
However i dont think im entering the polynomial itself correctly in my function.
Code for function:
directpoly1 <- function(x, coef, seqcoef = seq(coef) - 1) {
sum(coef*x^seqcoef)
}
directpoly <- function(x, coef) {
seqcoef <- seq(coef) - 1
sapply(x, directpoly1, coef, seqcoef)
}
Code for using function:
directpoly(x=seq(-10,10, length=5000000), rep(c(2,-1),20))
Any ideas on how to enter it correctly?
r polynomials
Im trying to evaluate a polynomial P(x):
However i dont think im entering the polynomial itself correctly in my function.
Code for function:
directpoly1 <- function(x, coef, seqcoef = seq(coef) - 1) {
sum(coef*x^seqcoef)
}
directpoly <- function(x, coef) {
seqcoef <- seq(coef) - 1
sapply(x, directpoly1, coef, seqcoef)
}
Code for using function:
directpoly(x=seq(-10,10, length=5000000), rep(c(2,-1),20))
Any ideas on how to enter it correctly?
r polynomials
r polynomials
asked Nov 13 at 12:04


torvin
284
284
1
Tryseqcoef = seq_along(coef)
. This means you will need to include the zero coefficients in the vectorcoef
.
– Rui Barradas
Nov 13 at 12:22
Thx! Can you see if im using the function correctly, so when evaluating P(x) with my function, is P(x) then correctly typed in?
– torvin
Nov 13 at 12:27
add a comment |
1
Tryseqcoef = seq_along(coef)
. This means you will need to include the zero coefficients in the vectorcoef
.
– Rui Barradas
Nov 13 at 12:22
Thx! Can you see if im using the function correctly, so when evaluating P(x) with my function, is P(x) then correctly typed in?
– torvin
Nov 13 at 12:27
1
1
Try
seqcoef = seq_along(coef)
. This means you will need to include the zero coefficients in the vector coef
.– Rui Barradas
Nov 13 at 12:22
Try
seqcoef = seq_along(coef)
. This means you will need to include the zero coefficients in the vector coef
.– Rui Barradas
Nov 13 at 12:22
Thx! Can you see if im using the function correctly, so when evaluating P(x) with my function, is P(x) then correctly typed in?
– torvin
Nov 13 at 12:27
Thx! Can you see if im using the function correctly, so when evaluating P(x) with my function, is P(x) then correctly typed in?
– torvin
Nov 13 at 12:27
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Here are two ways of writing your function.
- With a
sapply
loop. Makes the code more readable and some times faster. - With a standard
for
loop.
There is no need to write a separate function outside the function to be called with the x
values, I have placed that function in the body of the main functions.
Then both functions are tested, with a smaller input vector. As you can see, the for
loop function is faster.
directpoly <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
sapply(x, f)
}
directpoly2 <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
y <- numeric(length(x))
for(i in seq_along(y))
y[i] <- f(x[i])
y
}
library(microbenchmark)
library(ggplot2)
x <- seq(-10, 10, length = 50000)
mb <- microbenchmark(
Sapply = directpoly(x),
Forloop = directpoly2(x)
)
autoplot(mb)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Here are two ways of writing your function.
- With a
sapply
loop. Makes the code more readable and some times faster. - With a standard
for
loop.
There is no need to write a separate function outside the function to be called with the x
values, I have placed that function in the body of the main functions.
Then both functions are tested, with a smaller input vector. As you can see, the for
loop function is faster.
directpoly <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
sapply(x, f)
}
directpoly2 <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
y <- numeric(length(x))
for(i in seq_along(y))
y[i] <- f(x[i])
y
}
library(microbenchmark)
library(ggplot2)
x <- seq(-10, 10, length = 50000)
mb <- microbenchmark(
Sapply = directpoly(x),
Forloop = directpoly2(x)
)
autoplot(mb)
add a comment |
up vote
0
down vote
accepted
Here are two ways of writing your function.
- With a
sapply
loop. Makes the code more readable and some times faster. - With a standard
for
loop.
There is no need to write a separate function outside the function to be called with the x
values, I have placed that function in the body of the main functions.
Then both functions are tested, with a smaller input vector. As you can see, the for
loop function is faster.
directpoly <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
sapply(x, f)
}
directpoly2 <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
y <- numeric(length(x))
for(i in seq_along(y))
y[i] <- f(x[i])
y
}
library(microbenchmark)
library(ggplot2)
x <- seq(-10, 10, length = 50000)
mb <- microbenchmark(
Sapply = directpoly(x),
Forloop = directpoly2(x)
)
autoplot(mb)
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Here are two ways of writing your function.
- With a
sapply
loop. Makes the code more readable and some times faster. - With a standard
for
loop.
There is no need to write a separate function outside the function to be called with the x
values, I have placed that function in the body of the main functions.
Then both functions are tested, with a smaller input vector. As you can see, the for
loop function is faster.
directpoly <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
sapply(x, f)
}
directpoly2 <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
y <- numeric(length(x))
for(i in seq_along(y))
y[i] <- f(x[i])
y
}
library(microbenchmark)
library(ggplot2)
x <- seq(-10, 10, length = 50000)
mb <- microbenchmark(
Sapply = directpoly(x),
Forloop = directpoly2(x)
)
autoplot(mb)
Here are two ways of writing your function.
- With a
sapply
loop. Makes the code more readable and some times faster. - With a standard
for
loop.
There is no need to write a separate function outside the function to be called with the x
values, I have placed that function in the body of the main functions.
Then both functions are tested, with a smaller input vector. As you can see, the for
loop function is faster.
directpoly <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
sapply(x, f)
}
directpoly2 <- function(x, n = 39, coef = NULL){
f <- function(y) sum(coef * y^seqcoef)
if(all(is.null(coef))) coef <- rep(c(2, -1), length.out = n + 1)
seqcoef <- rev(seq_along(coef) - 1)
y <- numeric(length(x))
for(i in seq_along(y))
y[i] <- f(x[i])
y
}
library(microbenchmark)
library(ggplot2)
x <- seq(-10, 10, length = 50000)
mb <- microbenchmark(
Sapply = directpoly(x),
Forloop = directpoly2(x)
)
autoplot(mb)
answered Nov 13 at 14:10
Rui Barradas
14.8k31730
14.8k31730
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53280664%2fevaluating-polynomial-with-function%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
mOxpWNYsgMSz
1
Try
seqcoef = seq_along(coef)
. This means you will need to include the zero coefficients in the vectorcoef
.– Rui Barradas
Nov 13 at 12:22
Thx! Can you see if im using the function correctly, so when evaluating P(x) with my function, is P(x) then correctly typed in?
– torvin
Nov 13 at 12:27