Initialize Map instance from Map entries
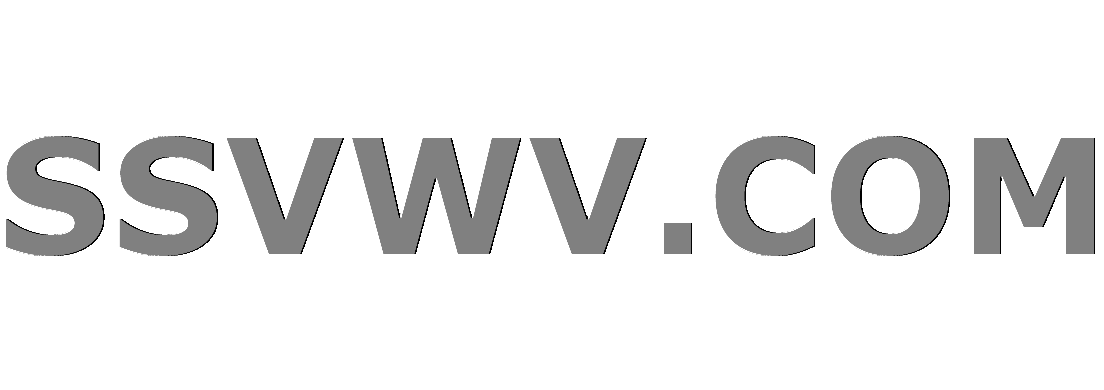
Multi tool use
Say I have some map entries like so:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.of(a,b,c); // error here
I get this error:
Cannot resolve method
'of(java.util.Map.Entry,
java.util.Map.Entry,
java.util.Map.Entry)'
I just want to make a new Map from entries in a map, how can I do this?
Not the question is specifically about how to init a Map given Map.Entry instances.
java hashmap java-10
|
show 1 more comment
Say I have some map entries like so:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.of(a,b,c); // error here
I get this error:
Cannot resolve method
'of(java.util.Map.Entry,
java.util.Map.Entry,
java.util.Map.Entry)'
I just want to make a new Map from entries in a map, how can I do this?
Not the question is specifically about how to init a Map given Map.Entry instances.
java hashmap java-10
1
I think Map.ofEntries will suffice. Suprising that Map.of is not overloaded to support entries, but I guess I know why.
– rakim
Feb 4 at 5:34
1
@RobbyCornelissen it is Java version 11, the var keyword was introduced, please
– rakim
Feb 4 at 5:34
Actually it goes back to Java version 10, not 11, so..
– rakim
Feb 4 at 5:41
5
more preciselyjava-9
– Common Man
Feb 4 at 5:48
2
@CommonMan Thevar
keyword was introduced in Java 10 not Java 9.
– Mark Rotteveel
Feb 4 at 10:07
|
show 1 more comment
Say I have some map entries like so:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.of(a,b,c); // error here
I get this error:
Cannot resolve method
'of(java.util.Map.Entry,
java.util.Map.Entry,
java.util.Map.Entry)'
I just want to make a new Map from entries in a map, how can I do this?
Not the question is specifically about how to init a Map given Map.Entry instances.
java hashmap java-10
Say I have some map entries like so:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.of(a,b,c); // error here
I get this error:
Cannot resolve method
'of(java.util.Map.Entry,
java.util.Map.Entry,
java.util.Map.Entry)'
I just want to make a new Map from entries in a map, how can I do this?
Not the question is specifically about how to init a Map given Map.Entry instances.
java hashmap java-10
java hashmap java-10
edited Feb 5 at 2:50
rakim
asked Feb 4 at 5:32


rakimrakim
362111
362111
1
I think Map.ofEntries will suffice. Suprising that Map.of is not overloaded to support entries, but I guess I know why.
– rakim
Feb 4 at 5:34
1
@RobbyCornelissen it is Java version 11, the var keyword was introduced, please
– rakim
Feb 4 at 5:34
Actually it goes back to Java version 10, not 11, so..
– rakim
Feb 4 at 5:41
5
more preciselyjava-9
– Common Man
Feb 4 at 5:48
2
@CommonMan Thevar
keyword was introduced in Java 10 not Java 9.
– Mark Rotteveel
Feb 4 at 10:07
|
show 1 more comment
1
I think Map.ofEntries will suffice. Suprising that Map.of is not overloaded to support entries, but I guess I know why.
– rakim
Feb 4 at 5:34
1
@RobbyCornelissen it is Java version 11, the var keyword was introduced, please
– rakim
Feb 4 at 5:34
Actually it goes back to Java version 10, not 11, so..
– rakim
Feb 4 at 5:41
5
more preciselyjava-9
– Common Man
Feb 4 at 5:48
2
@CommonMan Thevar
keyword was introduced in Java 10 not Java 9.
– Mark Rotteveel
Feb 4 at 10:07
1
1
I think Map.ofEntries will suffice. Suprising that Map.of is not overloaded to support entries, but I guess I know why.
– rakim
Feb 4 at 5:34
I think Map.ofEntries will suffice. Suprising that Map.of is not overloaded to support entries, but I guess I know why.
– rakim
Feb 4 at 5:34
1
1
@RobbyCornelissen it is Java version 11, the var keyword was introduced, please
– rakim
Feb 4 at 5:34
@RobbyCornelissen it is Java version 11, the var keyword was introduced, please
– rakim
Feb 4 at 5:34
Actually it goes back to Java version 10, not 11, so..
– rakim
Feb 4 at 5:41
Actually it goes back to Java version 10, not 11, so..
– rakim
Feb 4 at 5:41
5
5
more precisely
java-9
– Common Man
Feb 4 at 5:48
more precisely
java-9
– Common Man
Feb 4 at 5:48
2
2
@CommonMan The
var
keyword was introduced in Java 10 not Java 9.– Mark Rotteveel
Feb 4 at 10:07
@CommonMan The
var
keyword was introduced in Java 10 not Java 9.– Mark Rotteveel
Feb 4 at 10:07
|
show 1 more comment
5 Answers
5
active
oldest
votes
Replace
Map.of(a,b,c);
with
Map.ofEntries(a,b,c);
If you want to still use Map.of()
then you shall paste keys and values explicitly.
Map.Entry()
returns an immutableMap.Entry
containing the given
key and value. These entries are suitable for populating Map instances
using theMap.ofEntries()
method.
When to use Map.of()
and when to use Map.ofEntries()
add a comment |
From jdk-9 you can use Map.of()
to create Map
with key value pairs
Map<String, Object> map = Map.of("a", new Object(), "b", new Object(), "c", new Object());
And also by using SimpleEntry
Map<String, Object> map = Map.ofEntries(
new AbstractMap.SimpleEntry<>("a", new Object()),
new AbstractMap.SimpleEntry<>("b", new Object()),
new AbstractMap.SimpleEntry<>("c", new Object()));
Or by using Map.ofEntries
OP suggestion
Map.ofEntries was what I was looking for
– rakim
Feb 4 at 5:39
add a comment |
The simple answer is:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c); // ! use Map.ofEntries not Map.of
And the type of Map.entry(key,val)
is Map.Entry<K,V>
, in case you were wondering.
add a comment |
Use this
var m = Map.ofEntries(a, b, c);
instead of
var m = Map.of(a,b,c);
add a comment |
To create a map from entries Use either:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c);
or:
var m = Map.ofEntries(
entry("a", new Object()),
entry("b", new Object()),
entry("c", new Object()));
You can also create the map without explicitly creating the entries:
var m = Map.of("a", new Object(),
"b", new Object(),
"c", new Object());
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54510598%2finitialize-mapstring-object-instance-from-map-entries%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
Replace
Map.of(a,b,c);
with
Map.ofEntries(a,b,c);
If you want to still use Map.of()
then you shall paste keys and values explicitly.
Map.Entry()
returns an immutableMap.Entry
containing the given
key and value. These entries are suitable for populating Map instances
using theMap.ofEntries()
method.
When to use Map.of()
and when to use Map.ofEntries()
add a comment |
Replace
Map.of(a,b,c);
with
Map.ofEntries(a,b,c);
If you want to still use Map.of()
then you shall paste keys and values explicitly.
Map.Entry()
returns an immutableMap.Entry
containing the given
key and value. These entries are suitable for populating Map instances
using theMap.ofEntries()
method.
When to use Map.of()
and when to use Map.ofEntries()
add a comment |
Replace
Map.of(a,b,c);
with
Map.ofEntries(a,b,c);
If you want to still use Map.of()
then you shall paste keys and values explicitly.
Map.Entry()
returns an immutableMap.Entry
containing the given
key and value. These entries are suitable for populating Map instances
using theMap.ofEntries()
method.
When to use Map.of()
and when to use Map.ofEntries()
Replace
Map.of(a,b,c);
with
Map.ofEntries(a,b,c);
If you want to still use Map.of()
then you shall paste keys and values explicitly.
Map.Entry()
returns an immutableMap.Entry
containing the given
key and value. These entries are suitable for populating Map instances
using theMap.ofEntries()
method.
When to use Map.of()
and when to use Map.ofEntries()
edited Feb 4 at 5:52


nullpointer
49k11101197
49k11101197
answered Feb 4 at 5:41


Common ManCommon Man
1,43721327
1,43721327
add a comment |
add a comment |
From jdk-9 you can use Map.of()
to create Map
with key value pairs
Map<String, Object> map = Map.of("a", new Object(), "b", new Object(), "c", new Object());
And also by using SimpleEntry
Map<String, Object> map = Map.ofEntries(
new AbstractMap.SimpleEntry<>("a", new Object()),
new AbstractMap.SimpleEntry<>("b", new Object()),
new AbstractMap.SimpleEntry<>("c", new Object()));
Or by using Map.ofEntries
OP suggestion
Map.ofEntries was what I was looking for
– rakim
Feb 4 at 5:39
add a comment |
From jdk-9 you can use Map.of()
to create Map
with key value pairs
Map<String, Object> map = Map.of("a", new Object(), "b", new Object(), "c", new Object());
And also by using SimpleEntry
Map<String, Object> map = Map.ofEntries(
new AbstractMap.SimpleEntry<>("a", new Object()),
new AbstractMap.SimpleEntry<>("b", new Object()),
new AbstractMap.SimpleEntry<>("c", new Object()));
Or by using Map.ofEntries
OP suggestion
Map.ofEntries was what I was looking for
– rakim
Feb 4 at 5:39
add a comment |
From jdk-9 you can use Map.of()
to create Map
with key value pairs
Map<String, Object> map = Map.of("a", new Object(), "b", new Object(), "c", new Object());
And also by using SimpleEntry
Map<String, Object> map = Map.ofEntries(
new AbstractMap.SimpleEntry<>("a", new Object()),
new AbstractMap.SimpleEntry<>("b", new Object()),
new AbstractMap.SimpleEntry<>("c", new Object()));
Or by using Map.ofEntries
OP suggestion
From jdk-9 you can use Map.of()
to create Map
with key value pairs
Map<String, Object> map = Map.of("a", new Object(), "b", new Object(), "c", new Object());
And also by using SimpleEntry
Map<String, Object> map = Map.ofEntries(
new AbstractMap.SimpleEntry<>("a", new Object()),
new AbstractMap.SimpleEntry<>("b", new Object()),
new AbstractMap.SimpleEntry<>("c", new Object()));
Or by using Map.ofEntries
OP suggestion
edited Feb 4 at 5:49
answered Feb 4 at 5:38
DeadpoolDeadpool
6,0392528
6,0392528
Map.ofEntries was what I was looking for
– rakim
Feb 4 at 5:39
add a comment |
Map.ofEntries was what I was looking for
– rakim
Feb 4 at 5:39
Map.ofEntries was what I was looking for
– rakim
Feb 4 at 5:39
Map.ofEntries was what I was looking for
– rakim
Feb 4 at 5:39
add a comment |
The simple answer is:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c); // ! use Map.ofEntries not Map.of
And the type of Map.entry(key,val)
is Map.Entry<K,V>
, in case you were wondering.
add a comment |
The simple answer is:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c); // ! use Map.ofEntries not Map.of
And the type of Map.entry(key,val)
is Map.Entry<K,V>
, in case you were wondering.
add a comment |
The simple answer is:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c); // ! use Map.ofEntries not Map.of
And the type of Map.entry(key,val)
is Map.Entry<K,V>
, in case you were wondering.
The simple answer is:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c); // ! use Map.ofEntries not Map.of
And the type of Map.entry(key,val)
is Map.Entry<K,V>
, in case you were wondering.
answered Feb 4 at 5:39


rakimrakim
362111
362111
add a comment |
add a comment |
Use this
var m = Map.ofEntries(a, b, c);
instead of
var m = Map.of(a,b,c);
add a comment |
Use this
var m = Map.ofEntries(a, b, c);
instead of
var m = Map.of(a,b,c);
add a comment |
Use this
var m = Map.ofEntries(a, b, c);
instead of
var m = Map.of(a,b,c);
Use this
var m = Map.ofEntries(a, b, c);
instead of
var m = Map.of(a,b,c);
answered Feb 4 at 5:42
Mukit09Mukit09
1,05011023
1,05011023
add a comment |
add a comment |
To create a map from entries Use either:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c);
or:
var m = Map.ofEntries(
entry("a", new Object()),
entry("b", new Object()),
entry("c", new Object()));
You can also create the map without explicitly creating the entries:
var m = Map.of("a", new Object(),
"b", new Object(),
"c", new Object());
add a comment |
To create a map from entries Use either:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c);
or:
var m = Map.ofEntries(
entry("a", new Object()),
entry("b", new Object()),
entry("c", new Object()));
You can also create the map without explicitly creating the entries:
var m = Map.of("a", new Object(),
"b", new Object(),
"c", new Object());
add a comment |
To create a map from entries Use either:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c);
or:
var m = Map.ofEntries(
entry("a", new Object()),
entry("b", new Object()),
entry("c", new Object()));
You can also create the map without explicitly creating the entries:
var m = Map.of("a", new Object(),
"b", new Object(),
"c", new Object());
To create a map from entries Use either:
var a = Map.entry("a", new Object());
var b = Map.entry("b", new Object());
var c = Map.entry("c", new Object());
var m = Map.ofEntries(a,b,c);
or:
var m = Map.ofEntries(
entry("a", new Object()),
entry("b", new Object()),
entry("c", new Object()));
You can also create the map without explicitly creating the entries:
var m = Map.of("a", new Object(),
"b", new Object(),
"c", new Object());
answered Feb 4 at 7:59


ETOETO
2,6231627
2,6231627
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54510598%2finitialize-mapstring-object-instance-from-map-entries%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vUROC,t e1 2nhIsfYo QO6WNt7cdOj,2AY4T,JsAzoHME4ZHhREGtonRKrTv5Ys8A
1
I think Map.ofEntries will suffice. Suprising that Map.of is not overloaded to support entries, but I guess I know why.
– rakim
Feb 4 at 5:34
1
@RobbyCornelissen it is Java version 11, the var keyword was introduced, please
– rakim
Feb 4 at 5:34
Actually it goes back to Java version 10, not 11, so..
– rakim
Feb 4 at 5:41
5
more precisely
java-9
– Common Man
Feb 4 at 5:48
2
@CommonMan The
var
keyword was introduced in Java 10 not Java 9.– Mark Rotteveel
Feb 4 at 10:07