beautify by serializing class to generate token in C#
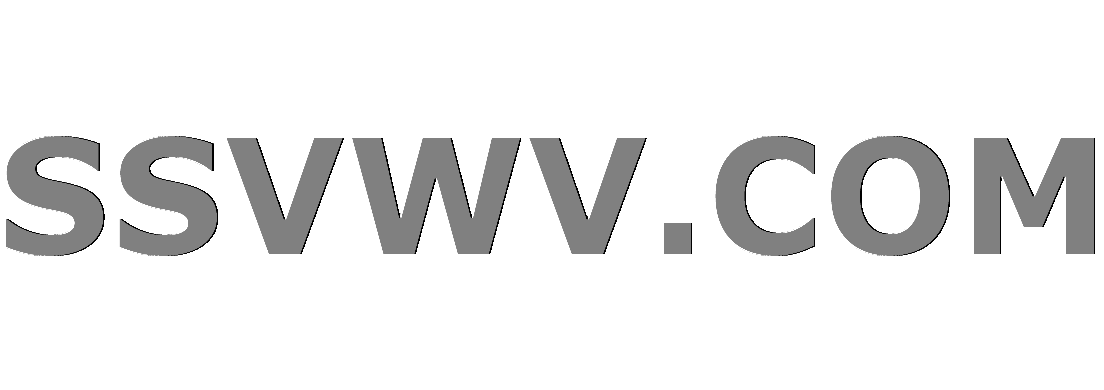
Multi tool use
up vote
0
down vote
favorite
I need to prepare some token make request over rest API. I already did this and works fine but as you guess its kinda look like very dirty way to handle this.
var dict = new Dictionary<string, string>();
dict.Add("Parameter1", tokenData.par1);
dict.Add("Parameter2", tokenData.par2);
using (HttpClient cliesssnt = new HttpClient())
{
cliesssnt.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var token =new FormUrlEncodedContent(dict);
HttpResponseMessage respossnse =
cliesssnt.PostAsync("https://someurl", token ).Result;
var result= respossnse.Content.ReadAsStringAsync().Result;
}
class:
public class ERaporAuthVM
{
[JsonProperty("Parameter1")]
public int par1 { get; set; }
[JsonProperty("Parameter2")]
public string par2 { get; set; }
}
I wish I could do something like this to preapre this token;
var token = JsonSerializer<ERaporAuthVM>(tokenData);
but how can I make it work this way?
asp.net rest
add a comment |
up vote
0
down vote
favorite
I need to prepare some token make request over rest API. I already did this and works fine but as you guess its kinda look like very dirty way to handle this.
var dict = new Dictionary<string, string>();
dict.Add("Parameter1", tokenData.par1);
dict.Add("Parameter2", tokenData.par2);
using (HttpClient cliesssnt = new HttpClient())
{
cliesssnt.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var token =new FormUrlEncodedContent(dict);
HttpResponseMessage respossnse =
cliesssnt.PostAsync("https://someurl", token ).Result;
var result= respossnse.Content.ReadAsStringAsync().Result;
}
class:
public class ERaporAuthVM
{
[JsonProperty("Parameter1")]
public int par1 { get; set; }
[JsonProperty("Parameter2")]
public string par2 { get; set; }
}
I wish I could do something like this to preapre this token;
var token = JsonSerializer<ERaporAuthVM>(tokenData);
but how can I make it work this way?
asp.net rest
You can try to createdict
dynamically via reflection
– Slava Utesinov
Nov 13 at 9:49
@SlavaUtesinov "Talk is cheap, show me the code!" Linus Torvalds. :)
– TyForHelpDude
Nov 13 at 9:51
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I need to prepare some token make request over rest API. I already did this and works fine but as you guess its kinda look like very dirty way to handle this.
var dict = new Dictionary<string, string>();
dict.Add("Parameter1", tokenData.par1);
dict.Add("Parameter2", tokenData.par2);
using (HttpClient cliesssnt = new HttpClient())
{
cliesssnt.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var token =new FormUrlEncodedContent(dict);
HttpResponseMessage respossnse =
cliesssnt.PostAsync("https://someurl", token ).Result;
var result= respossnse.Content.ReadAsStringAsync().Result;
}
class:
public class ERaporAuthVM
{
[JsonProperty("Parameter1")]
public int par1 { get; set; }
[JsonProperty("Parameter2")]
public string par2 { get; set; }
}
I wish I could do something like this to preapre this token;
var token = JsonSerializer<ERaporAuthVM>(tokenData);
but how can I make it work this way?
asp.net rest
I need to prepare some token make request over rest API. I already did this and works fine but as you guess its kinda look like very dirty way to handle this.
var dict = new Dictionary<string, string>();
dict.Add("Parameter1", tokenData.par1);
dict.Add("Parameter2", tokenData.par2);
using (HttpClient cliesssnt = new HttpClient())
{
cliesssnt.DefaultRequestHeaders.Accept.Add(new MediaTypeWithQualityHeaderValue("application/json"));
var token =new FormUrlEncodedContent(dict);
HttpResponseMessage respossnse =
cliesssnt.PostAsync("https://someurl", token ).Result;
var result= respossnse.Content.ReadAsStringAsync().Result;
}
class:
public class ERaporAuthVM
{
[JsonProperty("Parameter1")]
public int par1 { get; set; }
[JsonProperty("Parameter2")]
public string par2 { get; set; }
}
I wish I could do something like this to preapre this token;
var token = JsonSerializer<ERaporAuthVM>(tokenData);
but how can I make it work this way?
asp.net rest
asp.net rest
asked Nov 13 at 9:42
TyForHelpDude
1,43522546
1,43522546
You can try to createdict
dynamically via reflection
– Slava Utesinov
Nov 13 at 9:49
@SlavaUtesinov "Talk is cheap, show me the code!" Linus Torvalds. :)
– TyForHelpDude
Nov 13 at 9:51
add a comment |
You can try to createdict
dynamically via reflection
– Slava Utesinov
Nov 13 at 9:49
@SlavaUtesinov "Talk is cheap, show me the code!" Linus Torvalds. :)
– TyForHelpDude
Nov 13 at 9:51
You can try to create
dict
dynamically via reflection– Slava Utesinov
Nov 13 at 9:49
You can try to create
dict
dynamically via reflection– Slava Utesinov
Nov 13 at 9:49
@SlavaUtesinov "Talk is cheap, show me the code!" Linus Torvalds. :)
– TyForHelpDude
Nov 13 at 9:51
@SlavaUtesinov "Talk is cheap, show me the code!" Linus Torvalds. :)
– TyForHelpDude
Nov 13 at 9:51
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
public FormUrlEncodedContent JsonSerializer<T>(T input) where T : class
{
var result = new Dictionary<string, string>();
foreach(var prop in input.GetType().GetProperties())
{
var value = prop.GetValue(input);
//to-do: get name from JsonPropertyAttribute if exists
result.Add(prop.Name, value == null ? null : value.ToString());
}
return new FormUrlEncodedContent(result);
}
Usage:
var token = JsonSerializer(tokenData);
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
public FormUrlEncodedContent JsonSerializer<T>(T input) where T : class
{
var result = new Dictionary<string, string>();
foreach(var prop in input.GetType().GetProperties())
{
var value = prop.GetValue(input);
//to-do: get name from JsonPropertyAttribute if exists
result.Add(prop.Name, value == null ? null : value.ToString());
}
return new FormUrlEncodedContent(result);
}
Usage:
var token = JsonSerializer(tokenData);
add a comment |
up vote
1
down vote
accepted
public FormUrlEncodedContent JsonSerializer<T>(T input) where T : class
{
var result = new Dictionary<string, string>();
foreach(var prop in input.GetType().GetProperties())
{
var value = prop.GetValue(input);
//to-do: get name from JsonPropertyAttribute if exists
result.Add(prop.Name, value == null ? null : value.ToString());
}
return new FormUrlEncodedContent(result);
}
Usage:
var token = JsonSerializer(tokenData);
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
public FormUrlEncodedContent JsonSerializer<T>(T input) where T : class
{
var result = new Dictionary<string, string>();
foreach(var prop in input.GetType().GetProperties())
{
var value = prop.GetValue(input);
//to-do: get name from JsonPropertyAttribute if exists
result.Add(prop.Name, value == null ? null : value.ToString());
}
return new FormUrlEncodedContent(result);
}
Usage:
var token = JsonSerializer(tokenData);
public FormUrlEncodedContent JsonSerializer<T>(T input) where T : class
{
var result = new Dictionary<string, string>();
foreach(var prop in input.GetType().GetProperties())
{
var value = prop.GetValue(input);
//to-do: get name from JsonPropertyAttribute if exists
result.Add(prop.Name, value == null ? null : value.ToString());
}
return new FormUrlEncodedContent(result);
}
Usage:
var token = JsonSerializer(tokenData);
edited Nov 14 at 6:04
answered Nov 13 at 9:55


Slava Utesinov
10.6k2822
10.6k2822
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53278040%2fbeautify-by-serializing-class-to-generate-token-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
m,Tn5SekN Ec yR3X4g,pM,34mz,Jo5D
You can try to create
dict
dynamically via reflection– Slava Utesinov
Nov 13 at 9:49
@SlavaUtesinov "Talk is cheap, show me the code!" Linus Torvalds. :)
– TyForHelpDude
Nov 13 at 9:51