Unity Animation won't play if not enough time since last animation
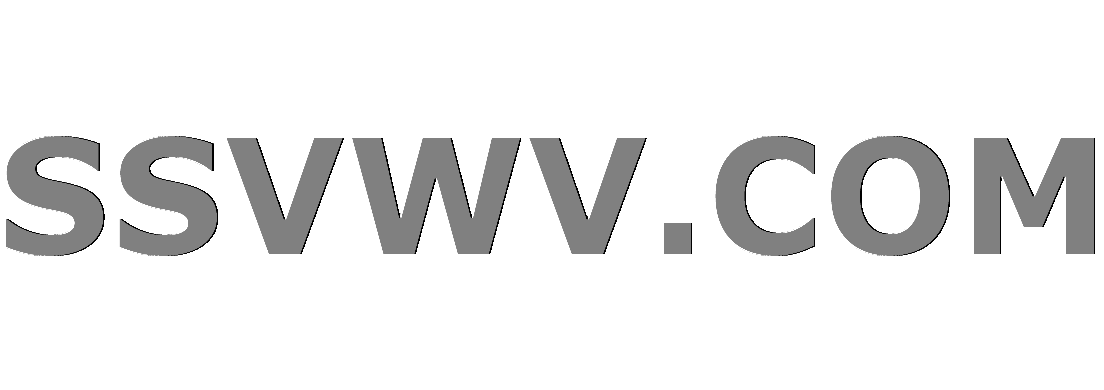
Multi tool use
I'm making a MIDI visualizer, and the first instrument I decided to try was the cowbell. Just to start, I'm storing a list of times of when the cowbell should play (I'll get to MIDI parsing later). It then checks if the current time is past or equal to the time of when the note should play.
for (int i = 0; i < times.Length; i++) { // For each note
bool notePlayed = false;
while (!notePlayed) { // While current note hasn't been played (waiting for its time)
if (Time.timeSinceLevelLoad >= timesAdjusted [i]) { // If the current time is past or equal to the time it should play
anim.Play ("hit"); // Play animation
Debug.Log ("hit!");
notePlayed = true; // The note has been played.
}
yield return null;
}
}
This works, but only if the time between the previous note and the current note is big enough for the animation to finish. So, how can I get the animation to play regardless of whether or not the animation has finished?
unity3d animation midi
add a comment |
I'm making a MIDI visualizer, and the first instrument I decided to try was the cowbell. Just to start, I'm storing a list of times of when the cowbell should play (I'll get to MIDI parsing later). It then checks if the current time is past or equal to the time of when the note should play.
for (int i = 0; i < times.Length; i++) { // For each note
bool notePlayed = false;
while (!notePlayed) { // While current note hasn't been played (waiting for its time)
if (Time.timeSinceLevelLoad >= timesAdjusted [i]) { // If the current time is past or equal to the time it should play
anim.Play ("hit"); // Play animation
Debug.Log ("hit!");
notePlayed = true; // The note has been played.
}
yield return null;
}
}
This works, but only if the time between the previous note and the current note is big enough for the animation to finish. So, how can I get the animation to play regardless of whether or not the animation has finished?
unity3d animation midi
So my issue was unrelated. In my code, I forgot to make sure some numbers for time calculations were casted to float, so basically all my note times were quantized to the quarter note. Many hours wasted...
– wyskoj
Nov 21 '18 at 3:39
add a comment |
I'm making a MIDI visualizer, and the first instrument I decided to try was the cowbell. Just to start, I'm storing a list of times of when the cowbell should play (I'll get to MIDI parsing later). It then checks if the current time is past or equal to the time of when the note should play.
for (int i = 0; i < times.Length; i++) { // For each note
bool notePlayed = false;
while (!notePlayed) { // While current note hasn't been played (waiting for its time)
if (Time.timeSinceLevelLoad >= timesAdjusted [i]) { // If the current time is past or equal to the time it should play
anim.Play ("hit"); // Play animation
Debug.Log ("hit!");
notePlayed = true; // The note has been played.
}
yield return null;
}
}
This works, but only if the time between the previous note and the current note is big enough for the animation to finish. So, how can I get the animation to play regardless of whether or not the animation has finished?
unity3d animation midi
I'm making a MIDI visualizer, and the first instrument I decided to try was the cowbell. Just to start, I'm storing a list of times of when the cowbell should play (I'll get to MIDI parsing later). It then checks if the current time is past or equal to the time of when the note should play.
for (int i = 0; i < times.Length; i++) { // For each note
bool notePlayed = false;
while (!notePlayed) { // While current note hasn't been played (waiting for its time)
if (Time.timeSinceLevelLoad >= timesAdjusted [i]) { // If the current time is past or equal to the time it should play
anim.Play ("hit"); // Play animation
Debug.Log ("hit!");
notePlayed = true; // The note has been played.
}
yield return null;
}
}
This works, but only if the time between the previous note and the current note is big enough for the animation to finish. So, how can I get the animation to play regardless of whether or not the animation has finished?
unity3d animation midi
unity3d animation midi
asked Nov 19 '18 at 23:19


wyskojwyskoj
1135
1135
So my issue was unrelated. In my code, I forgot to make sure some numbers for time calculations were casted to float, so basically all my note times were quantized to the quarter note. Many hours wasted...
– wyskoj
Nov 21 '18 at 3:39
add a comment |
So my issue was unrelated. In my code, I forgot to make sure some numbers for time calculations were casted to float, so basically all my note times were quantized to the quarter note. Many hours wasted...
– wyskoj
Nov 21 '18 at 3:39
So my issue was unrelated. In my code, I forgot to make sure some numbers for time calculations were casted to float, so basically all my note times were quantized to the quarter note. Many hours wasted...
– wyskoj
Nov 21 '18 at 3:39
So my issue was unrelated. In my code, I forgot to make sure some numbers for time calculations were casted to float, so basically all my note times were quantized to the quarter note. Many hours wasted...
– wyskoj
Nov 21 '18 at 3:39
add a comment |
2 Answers
2
active
oldest
votes
You are using "Play" method which will play an animation state regardless of your transition (Idle->Hit) , plus there's no condition defined in your transitions which will create an infinite loop between your states.
To resolve your main issue, you have to use set "normalizedTime" parameter to 0, like:
anim.Play ("hit",0,0); //assuming "hit" state is in your 0 layer
add a comment |
Disable has exit time checkbox to change animation without waiting another
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384038%2funity-animation-wont-play-if-not-enough-time-since-last-animation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are using "Play" method which will play an animation state regardless of your transition (Idle->Hit) , plus there's no condition defined in your transitions which will create an infinite loop between your states.
To resolve your main issue, you have to use set "normalizedTime" parameter to 0, like:
anim.Play ("hit",0,0); //assuming "hit" state is in your 0 layer
add a comment |
You are using "Play" method which will play an animation state regardless of your transition (Idle->Hit) , plus there's no condition defined in your transitions which will create an infinite loop between your states.
To resolve your main issue, you have to use set "normalizedTime" parameter to 0, like:
anim.Play ("hit",0,0); //assuming "hit" state is in your 0 layer
add a comment |
You are using "Play" method which will play an animation state regardless of your transition (Idle->Hit) , plus there's no condition defined in your transitions which will create an infinite loop between your states.
To resolve your main issue, you have to use set "normalizedTime" parameter to 0, like:
anim.Play ("hit",0,0); //assuming "hit" state is in your 0 layer
You are using "Play" method which will play an animation state regardless of your transition (Idle->Hit) , plus there's no condition defined in your transitions which will create an infinite loop between your states.
To resolve your main issue, you have to use set "normalizedTime" parameter to 0, like:
anim.Play ("hit",0,0); //assuming "hit" state is in your 0 layer
answered Nov 20 '18 at 7:11
HesamomHesamom
917
917
add a comment |
add a comment |
Disable has exit time checkbox to change animation without waiting another
add a comment |
Disable has exit time checkbox to change animation without waiting another
add a comment |
Disable has exit time checkbox to change animation without waiting another
Disable has exit time checkbox to change animation without waiting another
answered Nov 20 '18 at 7:47


phantasmphantasm
1315
1315
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384038%2funity-animation-wont-play-if-not-enough-time-since-last-animation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XyaxzAV0IZWmZ5oekHENXeGfm91 tHabJZkKyHc1IZX3nibS5BwR7MhPchllCD,uATBqHk5QA0xQzHO
So my issue was unrelated. In my code, I forgot to make sure some numbers for time calculations were casted to float, so basically all my note times were quantized to the quarter note. Many hours wasted...
– wyskoj
Nov 21 '18 at 3:39