Problem in downloading data using Download Handlers in ShinyApp
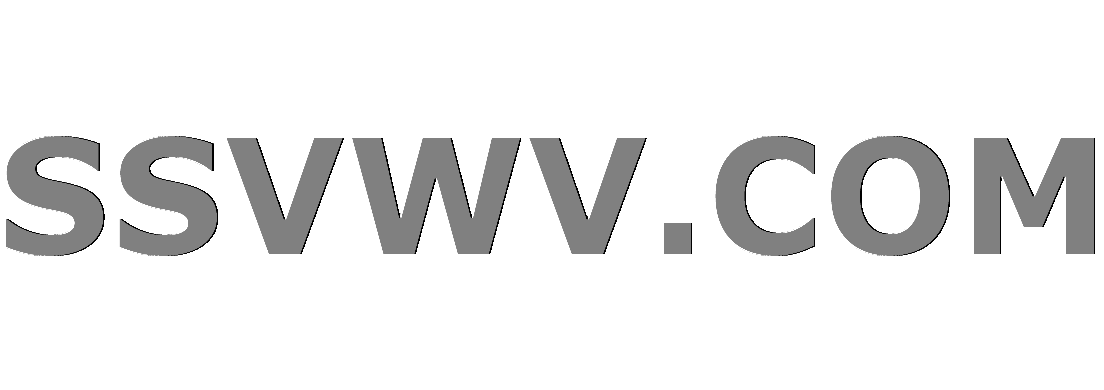
Multi tool use
My original data of mtcar gets downloaded using Download Handlers in ShinyApp whereas i want the the modified data (using SelectInputs) to be downloaded through Handlers.
I have attached my codes as well, please let me know whats wrong with them. Many thanks:)
library(shiny)
library(tidyr)
library(dplyr)
library(readr)
library(DT)
data_table <- mtcars
# Define UI
ui <- fluidPage(
downloadButton('downLoadFilter',"Download the filtered data"),
selectInput(inputId = "cyl",
label = "cyl:",
choices = c("All",
unique(as.character(data_table$cyl))),
selected = "4",
multiple = TRUE),
selectInput(inputId = "vs",
label = "vs:",
choices = c("All",
unique(as.character(data_table$vs))),
selected = "1",
multiple = TRUE),
DT::dataTableOutput('ex1'))
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else if(input$vs != 'All'){
return(data_table[data_table$vs == input$vs,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength =
10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive
thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
shinyApp(ui = ui, server = server)
r datatables shiny shiny-server dt
add a comment |
My original data of mtcar gets downloaded using Download Handlers in ShinyApp whereas i want the the modified data (using SelectInputs) to be downloaded through Handlers.
I have attached my codes as well, please let me know whats wrong with them. Many thanks:)
library(shiny)
library(tidyr)
library(dplyr)
library(readr)
library(DT)
data_table <- mtcars
# Define UI
ui <- fluidPage(
downloadButton('downLoadFilter',"Download the filtered data"),
selectInput(inputId = "cyl",
label = "cyl:",
choices = c("All",
unique(as.character(data_table$cyl))),
selected = "4",
multiple = TRUE),
selectInput(inputId = "vs",
label = "vs:",
choices = c("All",
unique(as.character(data_table$vs))),
selected = "1",
multiple = TRUE),
DT::dataTableOutput('ex1'))
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else if(input$vs != 'All'){
return(data_table[data_table$vs == input$vs,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength =
10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive
thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
shinyApp(ui = ui, server = server)
r datatables shiny shiny-server dt
don't remove the code for original answer, others need your question in the future, add a second section if needed
– Soren Havelund Welling
Nov 20 '18 at 7:29
I placed the code for your new question in comments of Vishesh Shrivastav answer.
– Soren Havelund Welling
Nov 20 '18 at 7:41
add a comment |
My original data of mtcar gets downloaded using Download Handlers in ShinyApp whereas i want the the modified data (using SelectInputs) to be downloaded through Handlers.
I have attached my codes as well, please let me know whats wrong with them. Many thanks:)
library(shiny)
library(tidyr)
library(dplyr)
library(readr)
library(DT)
data_table <- mtcars
# Define UI
ui <- fluidPage(
downloadButton('downLoadFilter',"Download the filtered data"),
selectInput(inputId = "cyl",
label = "cyl:",
choices = c("All",
unique(as.character(data_table$cyl))),
selected = "4",
multiple = TRUE),
selectInput(inputId = "vs",
label = "vs:",
choices = c("All",
unique(as.character(data_table$vs))),
selected = "1",
multiple = TRUE),
DT::dataTableOutput('ex1'))
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else if(input$vs != 'All'){
return(data_table[data_table$vs == input$vs,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength =
10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive
thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
shinyApp(ui = ui, server = server)
r datatables shiny shiny-server dt
My original data of mtcar gets downloaded using Download Handlers in ShinyApp whereas i want the the modified data (using SelectInputs) to be downloaded through Handlers.
I have attached my codes as well, please let me know whats wrong with them. Many thanks:)
library(shiny)
library(tidyr)
library(dplyr)
library(readr)
library(DT)
data_table <- mtcars
# Define UI
ui <- fluidPage(
downloadButton('downLoadFilter',"Download the filtered data"),
selectInput(inputId = "cyl",
label = "cyl:",
choices = c("All",
unique(as.character(data_table$cyl))),
selected = "4",
multiple = TRUE),
selectInput(inputId = "vs",
label = "vs:",
choices = c("All",
unique(as.character(data_table$vs))),
selected = "1",
multiple = TRUE),
DT::dataTableOutput('ex1'))
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else if(input$vs != 'All'){
return(data_table[data_table$vs == input$vs,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength =
10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive
thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
shinyApp(ui = ui, server = server)
r datatables shiny shiny-server dt
r datatables shiny shiny-server dt
edited Nov 20 '18 at 6:41
Doctor
asked Nov 19 '18 at 22:18
DoctorDoctor
238
238
don't remove the code for original answer, others need your question in the future, add a second section if needed
– Soren Havelund Welling
Nov 20 '18 at 7:29
I placed the code for your new question in comments of Vishesh Shrivastav answer.
– Soren Havelund Welling
Nov 20 '18 at 7:41
add a comment |
don't remove the code for original answer, others need your question in the future, add a second section if needed
– Soren Havelund Welling
Nov 20 '18 at 7:29
I placed the code for your new question in comments of Vishesh Shrivastav answer.
– Soren Havelund Welling
Nov 20 '18 at 7:41
don't remove the code for original answer, others need your question in the future, add a second section if needed
– Soren Havelund Welling
Nov 20 '18 at 7:29
don't remove the code for original answer, others need your question in the future, add a second section if needed
– Soren Havelund Welling
Nov 20 '18 at 7:29
I placed the code for your new question in comments of Vishesh Shrivastav answer.
– Soren Havelund Welling
Nov 20 '18 at 7:41
I placed the code for your new question in comments of Vishesh Shrivastav answer.
– Soren Havelund Welling
Nov 20 '18 at 7:41
add a comment |
1 Answer
1
active
oldest
votes
You are updating thedata()
only inside your renderDataTable
. You need to make it a reactive and then use it for being rendered as DataTable and being downloaded.
Change your server to:
# Define server logic
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength = 10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
Thank you so much mate, I have updated the codes (at top section) as you suggested. However i'm facing a problem in filtering out vs column using else if statement. Can you please have a look? Many thanks
– Doctor
Nov 20 '18 at 6:44
thedata <- reactive({ include_by_cyl = if(input$cyl != 'All') data_table$cyl %in% input$cyl else rep(T,nrow(data_table)); include_by_vs = if(input$cyl != 'All') data_table$vs %in% input$vs else rep(T,nrow(data_table)); return(data_table[include_by_cyl & include_by_vs,]) })
– Soren Havelund Welling
Nov 20 '18 at 7:43
semi colons is for line breaks...
– Soren Havelund Welling
Nov 20 '18 at 7:45
thanks mate, I tried running it, but it doesn't work when you put 'cyl=All" and then change 'vs' to any value in selectInput
– Doctor
Nov 20 '18 at 9:49
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53383462%2fproblem-in-downloading-data-using-download-handlers-in-shinyapp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You are updating thedata()
only inside your renderDataTable
. You need to make it a reactive and then use it for being rendered as DataTable and being downloaded.
Change your server to:
# Define server logic
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength = 10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
Thank you so much mate, I have updated the codes (at top section) as you suggested. However i'm facing a problem in filtering out vs column using else if statement. Can you please have a look? Many thanks
– Doctor
Nov 20 '18 at 6:44
thedata <- reactive({ include_by_cyl = if(input$cyl != 'All') data_table$cyl %in% input$cyl else rep(T,nrow(data_table)); include_by_vs = if(input$cyl != 'All') data_table$vs %in% input$vs else rep(T,nrow(data_table)); return(data_table[include_by_cyl & include_by_vs,]) })
– Soren Havelund Welling
Nov 20 '18 at 7:43
semi colons is for line breaks...
– Soren Havelund Welling
Nov 20 '18 at 7:45
thanks mate, I tried running it, but it doesn't work when you put 'cyl=All" and then change 'vs' to any value in selectInput
– Doctor
Nov 20 '18 at 9:49
add a comment |
You are updating thedata()
only inside your renderDataTable
. You need to make it a reactive and then use it for being rendered as DataTable and being downloaded.
Change your server to:
# Define server logic
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength = 10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
Thank you so much mate, I have updated the codes (at top section) as you suggested. However i'm facing a problem in filtering out vs column using else if statement. Can you please have a look? Many thanks
– Doctor
Nov 20 '18 at 6:44
thedata <- reactive({ include_by_cyl = if(input$cyl != 'All') data_table$cyl %in% input$cyl else rep(T,nrow(data_table)); include_by_vs = if(input$cyl != 'All') data_table$vs %in% input$vs else rep(T,nrow(data_table)); return(data_table[include_by_cyl & include_by_vs,]) })
– Soren Havelund Welling
Nov 20 '18 at 7:43
semi colons is for line breaks...
– Soren Havelund Welling
Nov 20 '18 at 7:45
thanks mate, I tried running it, but it doesn't work when you put 'cyl=All" and then change 'vs' to any value in selectInput
– Doctor
Nov 20 '18 at 9:49
add a comment |
You are updating thedata()
only inside your renderDataTable
. You need to make it a reactive and then use it for being rendered as DataTable and being downloaded.
Change your server to:
# Define server logic
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength = 10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
You are updating thedata()
only inside your renderDataTable
. You need to make it a reactive and then use it for being rendered as DataTable and being downloaded.
Change your server to:
# Define server logic
server <- function(input, output) {
thedata <- reactive({
if(input$cyl != 'All'){
return(data_table[data_table$cyl == input$cyl,])
}
else{
return(data_table)
}
})
output$ex1 <- DT::renderDataTable(DT::datatable(filter = 'top',
escape = FALSE,
options = list(pageLength = 10, scrollX='500px',autoWidth = TRUE),{
thedata() # Call reactive thedata()
}))
output$downLoadFilter <- downloadHandler(
filename = function() {
paste('Filtered data-', Sys.Date(), '.csv', sep = '')
},
content = function(path){
write_csv(thedata(),path) # Call reactive thedata()
})}
edited Nov 20 '18 at 1:12
answered Nov 20 '18 at 0:36
Vishesh ShrivastavVishesh Shrivastav
1,0812722
1,0812722
Thank you so much mate, I have updated the codes (at top section) as you suggested. However i'm facing a problem in filtering out vs column using else if statement. Can you please have a look? Many thanks
– Doctor
Nov 20 '18 at 6:44
thedata <- reactive({ include_by_cyl = if(input$cyl != 'All') data_table$cyl %in% input$cyl else rep(T,nrow(data_table)); include_by_vs = if(input$cyl != 'All') data_table$vs %in% input$vs else rep(T,nrow(data_table)); return(data_table[include_by_cyl & include_by_vs,]) })
– Soren Havelund Welling
Nov 20 '18 at 7:43
semi colons is for line breaks...
– Soren Havelund Welling
Nov 20 '18 at 7:45
thanks mate, I tried running it, but it doesn't work when you put 'cyl=All" and then change 'vs' to any value in selectInput
– Doctor
Nov 20 '18 at 9:49
add a comment |
Thank you so much mate, I have updated the codes (at top section) as you suggested. However i'm facing a problem in filtering out vs column using else if statement. Can you please have a look? Many thanks
– Doctor
Nov 20 '18 at 6:44
thedata <- reactive({ include_by_cyl = if(input$cyl != 'All') data_table$cyl %in% input$cyl else rep(T,nrow(data_table)); include_by_vs = if(input$cyl != 'All') data_table$vs %in% input$vs else rep(T,nrow(data_table)); return(data_table[include_by_cyl & include_by_vs,]) })
– Soren Havelund Welling
Nov 20 '18 at 7:43
semi colons is for line breaks...
– Soren Havelund Welling
Nov 20 '18 at 7:45
thanks mate, I tried running it, but it doesn't work when you put 'cyl=All" and then change 'vs' to any value in selectInput
– Doctor
Nov 20 '18 at 9:49
Thank you so much mate, I have updated the codes (at top section) as you suggested. However i'm facing a problem in filtering out vs column using else if statement. Can you please have a look? Many thanks
– Doctor
Nov 20 '18 at 6:44
Thank you so much mate, I have updated the codes (at top section) as you suggested. However i'm facing a problem in filtering out vs column using else if statement. Can you please have a look? Many thanks
– Doctor
Nov 20 '18 at 6:44
thedata <- reactive({ include_by_cyl = if(input$cyl != 'All') data_table$cyl %in% input$cyl else rep(T,nrow(data_table)); include_by_vs = if(input$cyl != 'All') data_table$vs %in% input$vs else rep(T,nrow(data_table)); return(data_table[include_by_cyl & include_by_vs,]) })
– Soren Havelund Welling
Nov 20 '18 at 7:43
thedata <- reactive({ include_by_cyl = if(input$cyl != 'All') data_table$cyl %in% input$cyl else rep(T,nrow(data_table)); include_by_vs = if(input$cyl != 'All') data_table$vs %in% input$vs else rep(T,nrow(data_table)); return(data_table[include_by_cyl & include_by_vs,]) })
– Soren Havelund Welling
Nov 20 '18 at 7:43
semi colons is for line breaks...
– Soren Havelund Welling
Nov 20 '18 at 7:45
semi colons is for line breaks...
– Soren Havelund Welling
Nov 20 '18 at 7:45
thanks mate, I tried running it, but it doesn't work when you put 'cyl=All" and then change 'vs' to any value in selectInput
– Doctor
Nov 20 '18 at 9:49
thanks mate, I tried running it, but it doesn't work when you put 'cyl=All" and then change 'vs' to any value in selectInput
– Doctor
Nov 20 '18 at 9:49
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53383462%2fproblem-in-downloading-data-using-download-handlers-in-shinyapp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LHi4kZD Fppoqqm s8VgVVEkm38VxrPk X9U HV,FWzZ5I u7 l0F U OpBY
don't remove the code for original answer, others need your question in the future, add a second section if needed
– Soren Havelund Welling
Nov 20 '18 at 7:29
I placed the code for your new question in comments of Vishesh Shrivastav answer.
– Soren Havelund Welling
Nov 20 '18 at 7:41