Wait for two actions inside component ngrx store
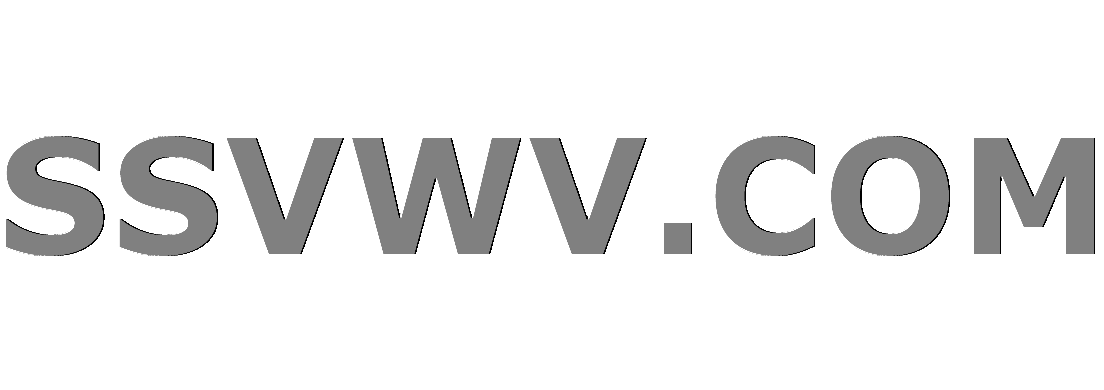
Multi tool use
I have 2 actions named METADATA_SUCCESS_ACTION_1 & SUCCESS_ACTION_2.
How can i wait for this 2 actions to complete and then subscribe for the consolidated data.
this.actions
.pipe(
ofType(METADATA_SUCCESS_ACTION_1),
take(1)
)
.subscribe((data1: any) => {
console.log(data1)
});
this.actions
.pipe(
ofType(SUCCESS_ACTION_2),
take(1)
)
.subscribe((data2: any) => {
console.log(data2)
});
I want to wait for both success to happen and then work on both the data as metadata as well as success data
angular ngrx ngrx-store
add a comment |
I have 2 actions named METADATA_SUCCESS_ACTION_1 & SUCCESS_ACTION_2.
How can i wait for this 2 actions to complete and then subscribe for the consolidated data.
this.actions
.pipe(
ofType(METADATA_SUCCESS_ACTION_1),
take(1)
)
.subscribe((data1: any) => {
console.log(data1)
});
this.actions
.pipe(
ofType(SUCCESS_ACTION_2),
take(1)
)
.subscribe((data2: any) => {
console.log(data2)
});
I want to wait for both success to happen and then work on both the data as metadata as well as success data
angular ngrx ngrx-store
add a comment |
I have 2 actions named METADATA_SUCCESS_ACTION_1 & SUCCESS_ACTION_2.
How can i wait for this 2 actions to complete and then subscribe for the consolidated data.
this.actions
.pipe(
ofType(METADATA_SUCCESS_ACTION_1),
take(1)
)
.subscribe((data1: any) => {
console.log(data1)
});
this.actions
.pipe(
ofType(SUCCESS_ACTION_2),
take(1)
)
.subscribe((data2: any) => {
console.log(data2)
});
I want to wait for both success to happen and then work on both the data as metadata as well as success data
angular ngrx ngrx-store
I have 2 actions named METADATA_SUCCESS_ACTION_1 & SUCCESS_ACTION_2.
How can i wait for this 2 actions to complete and then subscribe for the consolidated data.
this.actions
.pipe(
ofType(METADATA_SUCCESS_ACTION_1),
take(1)
)
.subscribe((data1: any) => {
console.log(data1)
});
this.actions
.pipe(
ofType(SUCCESS_ACTION_2),
take(1)
)
.subscribe((data2: any) => {
console.log(data2)
});
I want to wait for both success to happen and then work on both the data as metadata as well as success data
angular ngrx ngrx-store
angular ngrx ngrx-store
edited Nov 19 '18 at 18:53
Daniel W Strimpel
3,3961617
3,3961617
asked Nov 19 '18 at 17:34


Mayur PatilMayur Patil
287
287
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
It sounds like you can make use of the forkJoin
operator here (When all observables complete, emit the last emitted value from each.). From the documentation:
Why use forkJoin?
This operator is best used when you have a group of observables and
only care about the final emitted value of each. One common use case
for this is if you wish to issue multiple requests on page load (or
some other event) and only want to take action when a response has
been receieved for all. In this way it is similar to how you might use
Promise.all
.
Be aware that if any of the inner observables supplied to
forkJoin
error you will lose the value of any other observables that would or
have already completed if you do notcatch
the error correctly on the
inner observable. If you are only concerned with all inner observables
completing successfully you can catch the error on the outside.
It's also worth noting that if you have an observable that emits more
than one item, and you are concerned with the previous emissions
forkJoin
is not the correct choice. In these cases you may better off
with an operator likecombineLatest
orzip
.
To use it, you can use the following code for your example above.
forkJoin(
this.actions.pipe(ofType(METADATA_SUCCESS_ACTION_1), take(1)),
this.actions.pipe(ofType(SUCCESS_ACTION_2), take(1))
).subscribe(([data1, data2]) => {
console.log(data1);
console.log(data2);
})
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53379916%2fwait-for-two-actions-inside-component-ngrx-store%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It sounds like you can make use of the forkJoin
operator here (When all observables complete, emit the last emitted value from each.). From the documentation:
Why use forkJoin?
This operator is best used when you have a group of observables and
only care about the final emitted value of each. One common use case
for this is if you wish to issue multiple requests on page load (or
some other event) and only want to take action when a response has
been receieved for all. In this way it is similar to how you might use
Promise.all
.
Be aware that if any of the inner observables supplied to
forkJoin
error you will lose the value of any other observables that would or
have already completed if you do notcatch
the error correctly on the
inner observable. If you are only concerned with all inner observables
completing successfully you can catch the error on the outside.
It's also worth noting that if you have an observable that emits more
than one item, and you are concerned with the previous emissions
forkJoin
is not the correct choice. In these cases you may better off
with an operator likecombineLatest
orzip
.
To use it, you can use the following code for your example above.
forkJoin(
this.actions.pipe(ofType(METADATA_SUCCESS_ACTION_1), take(1)),
this.actions.pipe(ofType(SUCCESS_ACTION_2), take(1))
).subscribe(([data1, data2]) => {
console.log(data1);
console.log(data2);
})
add a comment |
It sounds like you can make use of the forkJoin
operator here (When all observables complete, emit the last emitted value from each.). From the documentation:
Why use forkJoin?
This operator is best used when you have a group of observables and
only care about the final emitted value of each. One common use case
for this is if you wish to issue multiple requests on page load (or
some other event) and only want to take action when a response has
been receieved for all. In this way it is similar to how you might use
Promise.all
.
Be aware that if any of the inner observables supplied to
forkJoin
error you will lose the value of any other observables that would or
have already completed if you do notcatch
the error correctly on the
inner observable. If you are only concerned with all inner observables
completing successfully you can catch the error on the outside.
It's also worth noting that if you have an observable that emits more
than one item, and you are concerned with the previous emissions
forkJoin
is not the correct choice. In these cases you may better off
with an operator likecombineLatest
orzip
.
To use it, you can use the following code for your example above.
forkJoin(
this.actions.pipe(ofType(METADATA_SUCCESS_ACTION_1), take(1)),
this.actions.pipe(ofType(SUCCESS_ACTION_2), take(1))
).subscribe(([data1, data2]) => {
console.log(data1);
console.log(data2);
})
add a comment |
It sounds like you can make use of the forkJoin
operator here (When all observables complete, emit the last emitted value from each.). From the documentation:
Why use forkJoin?
This operator is best used when you have a group of observables and
only care about the final emitted value of each. One common use case
for this is if you wish to issue multiple requests on page load (or
some other event) and only want to take action when a response has
been receieved for all. In this way it is similar to how you might use
Promise.all
.
Be aware that if any of the inner observables supplied to
forkJoin
error you will lose the value of any other observables that would or
have already completed if you do notcatch
the error correctly on the
inner observable. If you are only concerned with all inner observables
completing successfully you can catch the error on the outside.
It's also worth noting that if you have an observable that emits more
than one item, and you are concerned with the previous emissions
forkJoin
is not the correct choice. In these cases you may better off
with an operator likecombineLatest
orzip
.
To use it, you can use the following code for your example above.
forkJoin(
this.actions.pipe(ofType(METADATA_SUCCESS_ACTION_1), take(1)),
this.actions.pipe(ofType(SUCCESS_ACTION_2), take(1))
).subscribe(([data1, data2]) => {
console.log(data1);
console.log(data2);
})
It sounds like you can make use of the forkJoin
operator here (When all observables complete, emit the last emitted value from each.). From the documentation:
Why use forkJoin?
This operator is best used when you have a group of observables and
only care about the final emitted value of each. One common use case
for this is if you wish to issue multiple requests on page load (or
some other event) and only want to take action when a response has
been receieved for all. In this way it is similar to how you might use
Promise.all
.
Be aware that if any of the inner observables supplied to
forkJoin
error you will lose the value of any other observables that would or
have already completed if you do notcatch
the error correctly on the
inner observable. If you are only concerned with all inner observables
completing successfully you can catch the error on the outside.
It's also worth noting that if you have an observable that emits more
than one item, and you are concerned with the previous emissions
forkJoin
is not the correct choice. In these cases you may better off
with an operator likecombineLatest
orzip
.
To use it, you can use the following code for your example above.
forkJoin(
this.actions.pipe(ofType(METADATA_SUCCESS_ACTION_1), take(1)),
this.actions.pipe(ofType(SUCCESS_ACTION_2), take(1))
).subscribe(([data1, data2]) => {
console.log(data1);
console.log(data2);
})
answered Nov 19 '18 at 19:08
Daniel W StrimpelDaniel W Strimpel
3,3961617
3,3961617
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53379916%2fwait-for-two-actions-inside-component-ngrx-store%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ZbIZpN2O4dVE4N9oUIZDTyHfzUk472X5BrHS,j avSrwx 88ltg0eO0,wHP