Symfony request stays empty while sending file from javascript
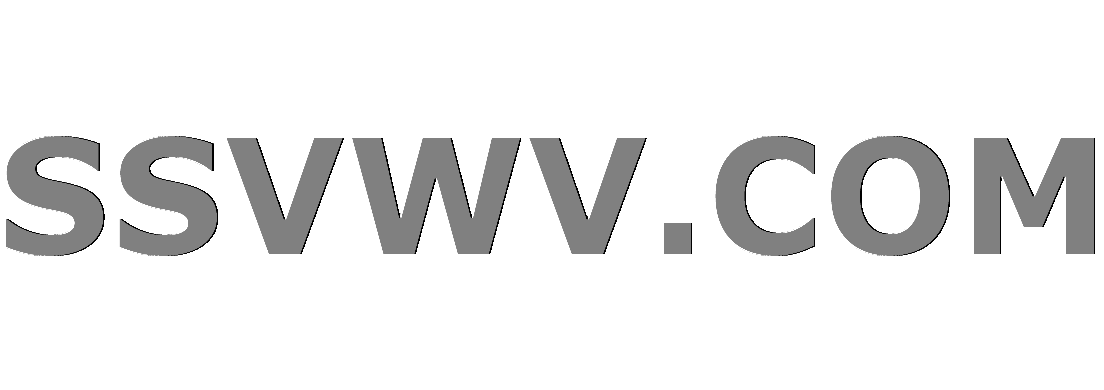
Multi tool use
I'm trying to send a file using React.js and the dropzone package.
I can send the file but the request in my php controller doesn't contain the file.
Here is the code:
Javascript:
handleDrop = files => {
// const uploaders = files.map(file => {
const formData = new FormData();
formData.append("file", files[0], 'picture');
return axios.post("http://localhost:8000/upload", formData, {
headers: {
'accept': 'application/json',
'Content-Type': `multipart/form-data; boundary=${formData._boundary}`,
}})
.then(response => {
const data = response.data;
console.log(data);
})
// })
};
render() {
return (
<div>
<NavigationBar>
displaySearchFields={'none'}
</NavigationBar>
<Dropzone onDrop={this.handleDrop}>
<div>Try dropping some files here, or click to select files to upload.</div>
</Dropzone>
</div>
);
}
Php:
/**
* @Route("/upload", methods={"POST", "OPTIONS"}, name="upload")
*/
public function uploadImageToDatabase(Request $request)
{
$data = $request->files->get("file");
$this->saveImage($data);
return new JsonResponse($request);
}
public function saveImage($image){
$fp = fopen("D:TempimageToEncode.png", "w");
fwrite($fp, $image);
fclose($fp);
}
Does anyone know what I am doing wrong?
javascript php reactjs symfony
|
show 1 more comment
I'm trying to send a file using React.js and the dropzone package.
I can send the file but the request in my php controller doesn't contain the file.
Here is the code:
Javascript:
handleDrop = files => {
// const uploaders = files.map(file => {
const formData = new FormData();
formData.append("file", files[0], 'picture');
return axios.post("http://localhost:8000/upload", formData, {
headers: {
'accept': 'application/json',
'Content-Type': `multipart/form-data; boundary=${formData._boundary}`,
}})
.then(response => {
const data = response.data;
console.log(data);
})
// })
};
render() {
return (
<div>
<NavigationBar>
displaySearchFields={'none'}
</NavigationBar>
<Dropzone onDrop={this.handleDrop}>
<div>Try dropping some files here, or click to select files to upload.</div>
</Dropzone>
</div>
);
}
Php:
/**
* @Route("/upload", methods={"POST", "OPTIONS"}, name="upload")
*/
public function uploadImageToDatabase(Request $request)
{
$data = $request->files->get("file");
$this->saveImage($data);
return new JsonResponse($request);
}
public function saveImage($image){
$fp = fopen("D:TempimageToEncode.png", "w");
fwrite($fp, $image);
fclose($fp);
}
Does anyone know what I am doing wrong?
javascript php reactjs symfony
I'm quite sure thatFormData
take a<form>
selector as parameter
– Preciel
Nov 19 '18 at 19:18
I didn't see people do that on the posts that I found though, but it seems logical anyway :D how should I do it you think?
– Jonas
Nov 19 '18 at 19:54
You won't needformData.append
btw
– Preciel
Nov 19 '18 at 20:01
How do you mean? Sorry New at javascript
– Jonas
Nov 19 '18 at 21:04
Doingconst formData=new FormData(document.getElementById(MyFormId))
will include every inputs in the form. Thus you don't need to append any data
– Preciel
Nov 19 '18 at 21:22
|
show 1 more comment
I'm trying to send a file using React.js and the dropzone package.
I can send the file but the request in my php controller doesn't contain the file.
Here is the code:
Javascript:
handleDrop = files => {
// const uploaders = files.map(file => {
const formData = new FormData();
formData.append("file", files[0], 'picture');
return axios.post("http://localhost:8000/upload", formData, {
headers: {
'accept': 'application/json',
'Content-Type': `multipart/form-data; boundary=${formData._boundary}`,
}})
.then(response => {
const data = response.data;
console.log(data);
})
// })
};
render() {
return (
<div>
<NavigationBar>
displaySearchFields={'none'}
</NavigationBar>
<Dropzone onDrop={this.handleDrop}>
<div>Try dropping some files here, or click to select files to upload.</div>
</Dropzone>
</div>
);
}
Php:
/**
* @Route("/upload", methods={"POST", "OPTIONS"}, name="upload")
*/
public function uploadImageToDatabase(Request $request)
{
$data = $request->files->get("file");
$this->saveImage($data);
return new JsonResponse($request);
}
public function saveImage($image){
$fp = fopen("D:TempimageToEncode.png", "w");
fwrite($fp, $image);
fclose($fp);
}
Does anyone know what I am doing wrong?
javascript php reactjs symfony
I'm trying to send a file using React.js and the dropzone package.
I can send the file but the request in my php controller doesn't contain the file.
Here is the code:
Javascript:
handleDrop = files => {
// const uploaders = files.map(file => {
const formData = new FormData();
formData.append("file", files[0], 'picture');
return axios.post("http://localhost:8000/upload", formData, {
headers: {
'accept': 'application/json',
'Content-Type': `multipart/form-data; boundary=${formData._boundary}`,
}})
.then(response => {
const data = response.data;
console.log(data);
})
// })
};
render() {
return (
<div>
<NavigationBar>
displaySearchFields={'none'}
</NavigationBar>
<Dropzone onDrop={this.handleDrop}>
<div>Try dropping some files here, or click to select files to upload.</div>
</Dropzone>
</div>
);
}
Php:
/**
* @Route("/upload", methods={"POST", "OPTIONS"}, name="upload")
*/
public function uploadImageToDatabase(Request $request)
{
$data = $request->files->get("file");
$this->saveImage($data);
return new JsonResponse($request);
}
public function saveImage($image){
$fp = fopen("D:TempimageToEncode.png", "w");
fwrite($fp, $image);
fclose($fp);
}
Does anyone know what I am doing wrong?
javascript php reactjs symfony
javascript php reactjs symfony
asked Nov 19 '18 at 16:10
JonasJonas
237
237
I'm quite sure thatFormData
take a<form>
selector as parameter
– Preciel
Nov 19 '18 at 19:18
I didn't see people do that on the posts that I found though, but it seems logical anyway :D how should I do it you think?
– Jonas
Nov 19 '18 at 19:54
You won't needformData.append
btw
– Preciel
Nov 19 '18 at 20:01
How do you mean? Sorry New at javascript
– Jonas
Nov 19 '18 at 21:04
Doingconst formData=new FormData(document.getElementById(MyFormId))
will include every inputs in the form. Thus you don't need to append any data
– Preciel
Nov 19 '18 at 21:22
|
show 1 more comment
I'm quite sure thatFormData
take a<form>
selector as parameter
– Preciel
Nov 19 '18 at 19:18
I didn't see people do that on the posts that I found though, but it seems logical anyway :D how should I do it you think?
– Jonas
Nov 19 '18 at 19:54
You won't needformData.append
btw
– Preciel
Nov 19 '18 at 20:01
How do you mean? Sorry New at javascript
– Jonas
Nov 19 '18 at 21:04
Doingconst formData=new FormData(document.getElementById(MyFormId))
will include every inputs in the form. Thus you don't need to append any data
– Preciel
Nov 19 '18 at 21:22
I'm quite sure that
FormData
take a <form>
selector as parameter– Preciel
Nov 19 '18 at 19:18
I'm quite sure that
FormData
take a <form>
selector as parameter– Preciel
Nov 19 '18 at 19:18
I didn't see people do that on the posts that I found though, but it seems logical anyway :D how should I do it you think?
– Jonas
Nov 19 '18 at 19:54
I didn't see people do that on the posts that I found though, but it seems logical anyway :D how should I do it you think?
– Jonas
Nov 19 '18 at 19:54
You won't need
formData.append
btw– Preciel
Nov 19 '18 at 20:01
You won't need
formData.append
btw– Preciel
Nov 19 '18 at 20:01
How do you mean? Sorry New at javascript
– Jonas
Nov 19 '18 at 21:04
How do you mean? Sorry New at javascript
– Jonas
Nov 19 '18 at 21:04
Doing
const formData=new FormData(document.getElementById(MyFormId))
will include every inputs in the form. Thus you don't need to append any data– Preciel
Nov 19 '18 at 21:22
Doing
const formData=new FormData(document.getElementById(MyFormId))
will include every inputs in the form. Thus you don't need to append any data– Preciel
Nov 19 '18 at 21:22
|
show 1 more comment
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378618%2fsymfony-request-stays-empty-while-sending-file-from-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53378618%2fsymfony-request-stays-empty-while-sending-file-from-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
f lViLhJZla5mc0Ptmfhq feG3 g054fjX10miV ix5dUc1QW
I'm quite sure that
FormData
take a<form>
selector as parameter– Preciel
Nov 19 '18 at 19:18
I didn't see people do that on the posts that I found though, but it seems logical anyway :D how should I do it you think?
– Jonas
Nov 19 '18 at 19:54
You won't need
formData.append
btw– Preciel
Nov 19 '18 at 20:01
How do you mean? Sorry New at javascript
– Jonas
Nov 19 '18 at 21:04
Doing
const formData=new FormData(document.getElementById(MyFormId))
will include every inputs in the form. Thus you don't need to append any data– Preciel
Nov 19 '18 at 21:22